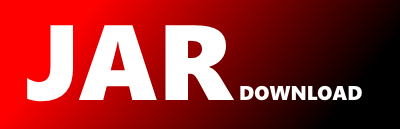
cn.hutool.core.thread.DelegatedExecutorService Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of hutool-all Show documentation
Show all versions of hutool-all Show documentation
Hutool是一个小而全的Java工具类库,通过静态方法封装,降低相关API的学习成本,提高工作效率,使Java拥有函数式语言般的优雅,让Java语言也可以“甜甜的”。
package cn.hutool.core.thread;
import cn.hutool.core.lang.Assert;
import java.util.Collection;
import java.util.List;
import java.util.concurrent.AbstractExecutorService;
import java.util.concurrent.Callable;
import java.util.concurrent.ExecutionException;
import java.util.concurrent.ExecutorService;
import java.util.concurrent.Future;
import java.util.concurrent.TimeUnit;
import java.util.concurrent.TimeoutException;
/**
* ExecutorService代理
*
* @author loolly
*/
public class DelegatedExecutorService extends AbstractExecutorService {
private final ExecutorService e;
/**
* 构造
*
* @param executor {@link ExecutorService}
*/
public DelegatedExecutorService(ExecutorService executor) {
Assert.notNull(executor, "executor must be not null !");
e = executor;
}
@Override
public void execute(Runnable command) {
e.execute(command);
}
@Override
public void shutdown() {
e.shutdown();
}
@Override
public List shutdownNow() {
return e.shutdownNow();
}
@Override
public boolean isShutdown() {
return e.isShutdown();
}
@Override
public boolean isTerminated() {
return e.isTerminated();
}
@Override
public boolean awaitTermination(long timeout, TimeUnit unit) throws InterruptedException {
return e.awaitTermination(timeout, unit);
}
@Override
public Future> submit(Runnable task) {
return e.submit(task);
}
@Override
public Future submit(Callable task) {
return e.submit(task);
}
@Override
public Future submit(Runnable task, T result) {
return e.submit(task, result);
}
@Override
public List> invokeAll(Collection extends Callable> tasks) throws InterruptedException {
return e.invokeAll(tasks);
}
@Override
public List> invokeAll(Collection extends Callable> tasks, long timeout, TimeUnit unit)
throws InterruptedException {
return e.invokeAll(tasks, timeout, unit);
}
@Override
public T invokeAny(Collection extends Callable> tasks)
throws InterruptedException, ExecutionException {
return e.invokeAny(tasks);
}
@Override
public T invokeAny(Collection extends Callable> tasks, long timeout, TimeUnit unit)
throws InterruptedException, ExecutionException, TimeoutException {
return e.invokeAny(tasks, timeout, unit);
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy