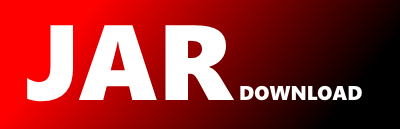
cn.hutool.poi.excel.reader.MapSheetReader Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of hutool-all Show documentation
Show all versions of hutool-all Show documentation
Hutool是一个小而全的Java工具类库,通过静态方法封装,降低相关API的学习成本,提高工作效率,使Java拥有函数式语言般的优雅,让Java语言也可以“甜甜的”。
package cn.hutool.poi.excel.reader;
import cn.hutool.core.collection.CollUtil;
import cn.hutool.core.collection.IterUtil;
import cn.hutool.core.collection.ListUtil;
import cn.hutool.core.util.StrUtil;
import org.apache.poi.ss.usermodel.Sheet;
import java.util.ArrayList;
import java.util.List;
import java.util.Map;
/**
* 读取{@link Sheet}为Map的List列表形式
*
* @author looly
* @since 5.4.4
*/
public class MapSheetReader extends AbstractSheetReader>> {
private final int headerRowIndex;
/**
* 构造
*
* @param headerRowIndex 标题所在行,如果标题行在读取的内容行中间,这行做为数据将忽略
* @param startRowIndex 起始行(包含,从0开始计数)
* @param endRowIndex 结束行(包含,从0开始计数)
*/
public MapSheetReader(int headerRowIndex, int startRowIndex, int endRowIndex) {
super(startRowIndex, endRowIndex);
this.headerRowIndex = headerRowIndex;
}
@Override
public List
© 2015 - 2024 Weber Informatics LLC | Privacy Policy