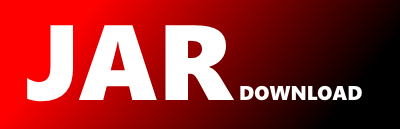
cn.hutool.socket.aio.AioClient Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of hutool-all Show documentation
Show all versions of hutool-all Show documentation
Hutool是一个小而全的Java工具类库,通过静态方法封装,降低相关API的学习成本,提高工作效率,使Java拥有函数式语言般的优雅,让Java语言也可以“甜甜的”。
package cn.hutool.socket.aio;
import cn.hutool.socket.ChannelUtil;
import cn.hutool.socket.SocketConfig;
import java.io.Closeable;
import java.io.IOException;
import java.net.InetSocketAddress;
import java.net.SocketOption;
import java.nio.ByteBuffer;
import java.nio.channels.AsynchronousSocketChannel;
/**
* Aio Socket客户端
*
* @author looly
* @since 4.5.0
*/
public class AioClient implements Closeable{
private final AioSession session;
/**
* 构造
*
* @param address 地址
* @param ioAction IO处理类
*/
public AioClient(InetSocketAddress address, IoAction ioAction) {
this(address, ioAction, new SocketConfig());
}
/**
* 构造
*
* @param address 地址
* @param ioAction IO处理类
* @param config 配置项
*/
public AioClient(InetSocketAddress address, IoAction ioAction, SocketConfig config) {
this(createChannel(address, config.getThreadPoolSize()), ioAction, config);
}
/**
* 构造
*
* @param channel {@link AsynchronousSocketChannel}
* @param ioAction IO处理类
* @param config 配置项
*/
public AioClient(AsynchronousSocketChannel channel, IoAction ioAction, SocketConfig config) {
this.session = new AioSession(channel, ioAction, config);
ioAction.accept(this.session);
}
/**
* 设置 Socket 的 Option 选项
* 选项见:{@link java.net.StandardSocketOptions}
*
* @param 选项泛型
* @param name {@link SocketOption} 枚举
* @param value SocketOption参数
* @return this
* @throws IOException IO异常
*/
public AioClient setOption(SocketOption name, T value) throws IOException {
this.session.getChannel().setOption(name, value);
return this;
}
/**
* 获取IO处理器
*
* @return {@link IoAction}
*/
public IoAction getIoAction() {
return this.session.getIoAction();
}
/**
* 从服务端读取数据
*
* @return this
*/
public AioClient read() {
this.session.read();
return this;
}
/**
* 写数据到服务端
*
* @param data 数据
* @return this
*/
public AioClient write(ByteBuffer data) {
this.session.write(data);
return this;
}
/**
* 关闭客户端
*/
@Override
public void close() {
this.session.close();
}
// ------------------------------------------------------------------------------------- Private method start
/**
* 初始化
*
* @param address 地址和端口
* @param poolSize 线程池大小
* @return this
*/
private static AsynchronousSocketChannel createChannel(InetSocketAddress address, int poolSize) {
return ChannelUtil.connect(ChannelUtil.createFixedGroup(poolSize), address);
}
// ------------------------------------------------------------------------------------- Private method end
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy