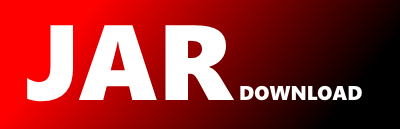
cn.hutool.poi.excel.InternalExcelUtil Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of hutool-poi Show documentation
Show all versions of hutool-poi Show documentation
Hutool POI工具类(对MS Office操作)
package cn.hutool.poi.excel;
import java.util.ArrayList;
import java.util.Calendar;
import java.util.Date;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import org.apache.poi.POIXMLDocumentPart;
import org.apache.poi.hssf.usermodel.HSSFClientAnchor;
import org.apache.poi.hssf.usermodel.HSSFPicture;
import org.apache.poi.hssf.usermodel.HSSFPictureData;
import org.apache.poi.hssf.usermodel.HSSFShape;
import org.apache.poi.hssf.usermodel.HSSFSheet;
import org.apache.poi.hssf.usermodel.HSSFWorkbook;
import org.apache.poi.ss.usermodel.Cell;
import org.apache.poi.ss.usermodel.CellStyle;
import org.apache.poi.ss.usermodel.CellType;
import org.apache.poi.ss.usermodel.PictureData;
import org.apache.poi.ss.usermodel.RichTextString;
import org.apache.poi.ss.usermodel.Row;
import org.apache.poi.ss.usermodel.Sheet;
import org.apache.poi.ss.usermodel.Workbook;
import org.apache.poi.ss.util.CellRangeAddress;
import org.apache.poi.ss.util.RegionUtil;
import org.apache.poi.xssf.usermodel.XSSFDrawing;
import org.apache.poi.xssf.usermodel.XSSFPicture;
import org.apache.poi.xssf.usermodel.XSSFShape;
import org.apache.poi.xssf.usermodel.XSSFSheet;
import org.apache.poi.xssf.usermodel.XSSFWorkbook;
import org.openxmlformats.schemas.drawingml.x2006.spreadsheetDrawing.CTMarker;
import cn.hutool.core.collection.CollectionUtil;
import cn.hutool.core.date.DateUtil;
import cn.hutool.core.lang.Assert;
import cn.hutool.core.util.StrUtil;
import cn.hutool.poi.excel.editors.TrimEditor;
/**
* Excel内部工具类,主要针对行等操作支持
*
* @author looly
*/
public class InternalExcelUtil {
/**
* 获取单元格值
*
* @param cell {@link Cell}单元格
* @param isTrimCellValue 如果单元格类型为字符串,是否去掉两边空白符
* @return 值,类型可能为:Date、Double、Boolean、String
*/
public static Object getCellValue(Cell cell, boolean isTrimCellValue) {
return getCellValue(cell, cell.getCellTypeEnum(), isTrimCellValue);
}
/**
* 获取单元格值
*
* @param cell {@link Cell}单元格
* @param cellEditor 单元格值编辑器。可以通过此编辑器对单元格值做自定义操作
* @return 值,类型可能为:Date、Double、Boolean、String
*/
public static Object getCellValue(Cell cell, CellEditor cellEditor) {
if (null == cell) {
return null;
}
return getCellValue(cell, cell.getCellTypeEnum(), cellEditor);
}
/**
* 获取单元格值
*
* @param cell {@link Cell}单元格
* @param cellType 单元格值类型{@link CellType}枚举
* @param isTrimCellValue 如果单元格类型为字符串,是否去掉两边空白符
* @return 值,类型可能为:Date、Double、Boolean、String
*/
public static Object getCellValue(Cell cell, CellType cellType, final boolean isTrimCellValue) {
return getCellValue(cell, cellType, isTrimCellValue ? new TrimEditor() : null);
}
/**
* 获取单元格值
* 如果单元格值为数字格式,则判断其格式中是否有小数部分,无则返回Long类型,否则返回Double类型
*
* @param cell {@link Cell}单元格
* @param cellType 单元格值类型{@link CellType}枚举,如果为{@code null}默认使用cell的类型
* @param cellEditor 单元格值编辑器。可以通过此编辑器对单元格值做自定义操作
* @return 值,类型可能为:Date、Double、Boolean、String
*/
public static Object getCellValue(Cell cell, CellType cellType, CellEditor cellEditor) {
if (null == cell) {
return null;
}
if (null == cellType) {
cellType = cell.getCellTypeEnum();
}
Object value;
switch (cellType) {
case NUMERIC:
value = getNumericValue(cell);
break;
case BOOLEAN:
value = cell.getBooleanCellValue();
break;
case FORMULA:
// 遇到公式时查找公式结果类型
value = getCellValue(cell, cell.getCachedFormulaResultTypeEnum(), cellEditor);
break;
case BLANK:
value = StrUtil.EMPTY;
break;
default:
value = cell.getStringCellValue();
}
return null == cellEditor ? value : cellEditor.edit(cell, value);
}
/**
* 设置单元格值
*
* @param cell 单元格
* @param value 值
* @param styleSet 单元格样式集,包括日期等样式
*/
public static void setCellValue(Cell cell, Object value, StyleSet styleSet) {
if (null != styleSet.cellStyle) {
cell.setCellStyle(styleSet.cellStyle);
}
if (null == value) {
cell.setCellValue(StrUtil.EMPTY);
} else if (value instanceof Date) {
if (null != styleSet && null != styleSet.cellStyleForDate) {
cell.setCellStyle(styleSet.cellStyleForDate);
}
cell.setCellValue((Date) value);
} else if (value instanceof Calendar) {
cell.setCellValue((Calendar) value);
} else if (value instanceof Boolean) {
cell.setCellValue((Boolean) value);
} else if (value instanceof RichTextString) {
cell.setCellValue((RichTextString) value);
} else if (value instanceof Number) {
if ((value instanceof Double || value instanceof Float) && null != styleSet && null != styleSet.cellStyleForNumber) {
cell.setCellStyle(styleSet.cellStyleForNumber);
}
cell.setCellValue(((Number) value).doubleValue());
} else {
cell.setCellValue(value.toString());
}
}
/**
* 获取已有行或创建新行
* @param row Excel表的行
* @param cellIndex 列号
* @return {@link Row}
* @since 4.0.2
*/
public static Cell getOrCreateCell(Row row, int cellIndex) {
Cell cell = row.getCell(cellIndex);
if(null == cell) {
cell = row.createCell(cellIndex);
}
return cell;
}
/**
* 获取已有行或创建新行
* @param sheet Excel表
* @param rowIndex 行号
* @return {@link Row}
* @since 4.0.2
*/
public static Row getOrCreateRow(Sheet sheet, int rowIndex) {
Row row = sheet.getRow(rowIndex);
if(null == row) {
row = sheet.createRow(rowIndex);
}
return row;
}
/**
* 读取一行
*
* @param row 行
* @param cellEditor 单元格编辑器
* @return 单元格值列表
*/
public static List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy