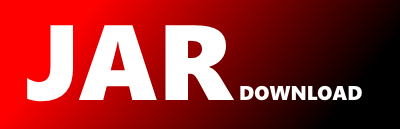
cn.hyperchain.sdk.service.ArchiveService Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of litesdk Show documentation
Show all versions of litesdk Show documentation
A Java client tool for Hyperchain
package cn.hyperchain.sdk.service;
import cn.hyperchain.sdk.request.Request;
import cn.hyperchain.sdk.response.archive.ArchiveBoolResponse;
import cn.hyperchain.sdk.response.archive.ArchiveFilterIdResponse;
import cn.hyperchain.sdk.response.archive.ArchiveResponse;
import java.math.BigInteger;
public interface ArchiveService {
/**
* @see ArchiveService#snapshot(String, int...)
*/
Request snapshot(BigInteger blockNumber, int... nodeIds);
/**
* make snapshot.
*
* @param blockNumber block number
* @param nodeIds specific ids
* @return {@link Request} of {@link ArchiveFilterIdResponse}
*/
Request snapshot(String blockNumber, int... nodeIds);
/**
* check if the snapshot exists.
*
* @param filterId filter id
* @param nodeIds specific ids
* @return {@link Request} of {@link ArchiveBoolResponse}
*/
Request querySnapshotExist(String filterId, int... nodeIds);
/**
* check if the snapshot is correct.
*
* @param filterId filter id
* @param nodeIds specific ids
* @return {@link Request} of {@link ArchiveBoolResponse}
*/
Request checkSnapshot(String filterId, int... nodeIds);
/**
* delete snapshot.
*
* @param filterId filter id
* @param nodeIds specific ids
* @return {@link Request} of {@link ArchiveBoolResponse}
*/
Request deleteSnapshot(String filterId, int... nodeIds);
/**
* list snapshot.
*
* @param nodeIds specific ids
* @return {@link Request} of {@link ArchiveBoolResponse}
*/
Request listSnapshot(int... nodeIds);
/**
* read snapshot.
*
* @param filterId filter id
* @param nodeIds specific ids
* @return {@link Request} of {@link ArchiveBoolResponse}
*/
Request readSnapshot(String filterId, int... nodeIds);
/**
* data archive.
*
* @param filterId filter id
* @param sync if synchronization
* @param nodeIds specific ids
* @return {@link Request} of {@link ArchiveBoolResponse}
*/
Request archive(String filterId, boolean sync, int... nodeIds);
/**
* direct archive.
*
* @param blkNumber block number
* @param nodeIds specific ids
* @return {@link Request} of {@link ArchiveBoolResponse}
*/
Request archiveNoPredict(BigInteger blkNumber, int... nodeIds);
/**
* restore data from archive.
*
* @param filterId filter id
* @param sync if synchronization
* @param nodeIds specific ids
* @return {@link Request} of {@link ArchiveBoolResponse}
*/
Request restore(String filterId, boolean sync, int... nodeIds);
/**
* restore all data.
*
* @param sync if synchronization
* @param nodeIds specific ids
* @return {@link Request} of {@link ArchiveBoolResponse}
*/
Request restoreAll(boolean sync, int... nodeIds);
/**
* query archive.
*
* @param filterId filter id
* @param nodeIds specific ids
* @return {@link Request} of {@link ArchiveBoolResponse}
*/
Request queryArchive(String filterId, int... nodeIds);
/**
* read pending archives.
*
* @param nodeIds specific ids
* @return {@link Request} of {@link ArchiveResponse}
*/
Request pending(int... nodeIds);
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy