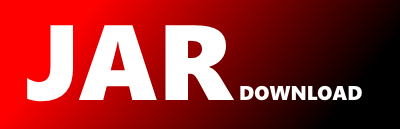
cn.hyperchain.sdk.response.PageResult Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of litesdk Show documentation
Show all versions of litesdk Show documentation
A Java client tool for Hyperchain
package cn.hyperchain.sdk.response;
import cn.hyperchain.sdk.response.block.BlockResponse;
import cn.hyperchain.sdk.response.tx.TxResponse;
import com.google.gson.Gson;
import com.google.gson.GsonBuilder;
import com.google.gson.JsonArray;
import com.google.gson.JsonElement;
import com.google.gson.annotations.Expose;
import java.util.ArrayList;
import java.util.List;
public class PageResult {
@Expose
private String hasmore;
@Expose
private JsonElement data;
Gson gson = new GsonBuilder().excludeFieldsWithoutExposeAnnotation().create();
/**
* parse result to real result(blocks or transactions).
*
* @param clz can only be TxResponse.Transaction.class or BlockResponse.Block.class
* @return parse result
*/
public List parseResult(Class clz) {
if (clz != BlockResponse.Block.class && clz != TxResponse.Transaction.class) {
throw new RuntimeException("method argument `class` must be BlockResponse.Block.class or TxResponse.Transaction.class!");
}
ArrayList results = new ArrayList<>();
if (data.isJsonArray()) {
JsonArray jsonArray = data.getAsJsonArray();
for (JsonElement jsonElement : jsonArray) {
results.add((T) gson.fromJson(jsonElement, clz));
}
} else {
T block = (T) gson.fromJson(data, clz);
results.add(block);
}
return results;
}
@Override
public String toString() {
return "PageResult{" +
"hasmore='" + hasmore + '\'' +
", data=" + data +
'}';
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy