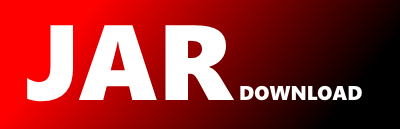
cn.hyperchain.sdk.response.auth.InspectorRulesResponse Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of litesdk Show documentation
Show all versions of litesdk Show documentation
A Java client tool for Hyperchain
package cn.hyperchain.sdk.response.auth;
import com.google.gson.annotations.Expose;
import com.google.gson.annotations.SerializedName;
import java.util.List;
public class InspectorRulesResponse extends Response {
@Expose
private List result;
public List getRules() {
return result;
}
@Override
public String toString() {
return "InspectorRulesResponse{" +
"result=" + result +
", jsonrpc='" + jsonrpc + '\'' +
", id='" + id + '\'' +
", code=" + code +
", message='" + message + '\'' +
", namespace='" + namespace + '\'' +
'}';
}
public class InspectorRule {
@Expose
@SerializedName("allow_anyone")
private boolean allowAnyone;
@Expose
@SerializedName("authorized_roles")
private List authorizedRoles;
@Expose
@SerializedName("forbidden_roles")
private List forbiddenRoles;
@Expose
private int id;
@Expose
private String name;
@Expose
private List method;
public boolean isAllowAnyone() {
return allowAnyone;
}
public void setAllowAnyone(boolean allowAnyone) {
this.allowAnyone = allowAnyone;
}
public List getAuthorizedRoles() {
return authorizedRoles;
}
public void setAuthorizedRoles(List authorizedRoles) {
this.authorizedRoles = authorizedRoles;
}
public List getForbiddenRoles() {
return forbiddenRoles;
}
public void setForbiddenRoles(List forbiddenRoles) {
this.forbiddenRoles = forbiddenRoles;
}
public int getId() {
return id;
}
public void setId(int id) {
this.id = id;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public List getMethod() {
return method;
}
public void setMethod(List method) {
this.method = method;
}
@Override
public String toString() {
return "InspectorRule{" +
"allowAnyone=" + allowAnyone +
", authorizedRoles=" + authorizedRoles +
", forbiddenRoles=" + forbiddenRoles +
", id=" + id +
", name='" + name + '\'' +
", method=" + method +
'}';
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy