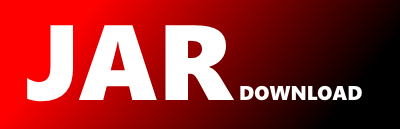
cn.hyperchain.sdk.service.params.InspectorRuleParam Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of litesdk Show documentation
Show all versions of litesdk Show documentation
A Java client tool for Hyperchain
package cn.hyperchain.sdk.service.params;
import com.google.gson.annotations.Expose;
import com.google.gson.annotations.SerializedName;
import java.util.List;
public class InspectorRuleParam {
@Expose
@SerializedName("allow_anyone")
private boolean allowAnyone;
@Expose
@SerializedName("authorized_roles")
private List authorizedRoles;
@Expose
@SerializedName("forbidden_roles")
private List forbiddenRoles;
@Expose
private int id;
@Expose
private String name;
@Expose
private List method;
public static class Builder {
InspectorRuleParam ruleParam;
public Builder() {
ruleParam = new InspectorRuleParam();
}
public Builder allowAnyone(boolean allowAnyone) {
ruleParam.allowAnyone = allowAnyone;
return this;
}
public Builder authorizedRoles(List roles) {
ruleParam.authorizedRoles = roles;
return this;
}
public Builder forbiddenRoles(List roles) {
ruleParam.forbiddenRoles = roles;
return this;
}
public Builder id(int id) {
ruleParam.id = id;
return this;
}
public Builder name(String name) {
ruleParam.name = name;
return this;
}
public Builder methods(List methods) {
ruleParam.method = methods;
return this;
}
public InspectorRuleParam build() {
return this.ruleParam;
}
}
public boolean isAllowAnyone() {
return allowAnyone;
}
public void setAllowAnyone(boolean allowAnyone) {
this.allowAnyone = allowAnyone;
}
public List getAuthorizedRoles() {
return authorizedRoles;
}
public void setAuthorizedRoles(List authorizedRoles) {
this.authorizedRoles = authorizedRoles;
}
public List getForbiddenRoles() {
return forbiddenRoles;
}
public void setForbiddenRoles(List forbiddenRoles) {
this.forbiddenRoles = forbiddenRoles;
}
public int getId() {
return id;
}
public void setId(int id) {
this.id = id;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public List getMethod() {
return method;
}
public void setMethod(List method) {
this.method = method;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy