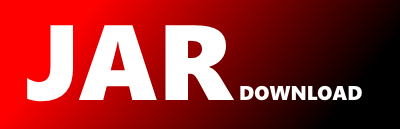
cn.imaq.autumn.aop.HookChain Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of autumn-aop Show documentation
Show all versions of autumn-aop Show documentation
Aspect Oriented Programming support for Autumn
package cn.imaq.autumn.aop;
import cn.imaq.autumn.core.context.AutumnContext;
import lombok.Getter;
import java.lang.reflect.InvocationTargetException;
import java.lang.reflect.Method;
import java.util.Iterator;
public class HookChain {
private Iterator hookItr;
@Getter
private AutumnContext context;
@Getter
private Object target;
@Getter
private Method realMethod;
@Getter
private Object[] args;
public HookChain(Iterator hookItr, AutumnContext context, Object target, Method realMethod, Object[] args) {
this.hookItr = hookItr;
this.context = context;
this.target = target;
this.realMethod = realMethod;
this.args = args;
}
public Object proceed() throws Throwable {
try {
if (hookItr.hasNext()) {
return invokeHook(hookItr.next().getHook());
}
return realMethod.invoke(target, args);
} catch (InvocationTargetException e) {
throw e.getCause();
}
}
private Object invokeHook(Method hook) throws InvocationTargetException, IllegalAccessException {
if (hook.getParameterCount() > 0) {
return hook.invoke(context.getBeanByType(hook.getDeclaringClass()), this);
} else {
hook.invoke(context.getBeanByType(hook.getDeclaringClass()));
return realMethod.invoke(target, args);
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy