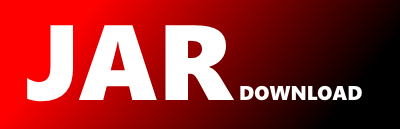
cn.ipokerface.admin.entity.AdminAccount Maven / Gradle / Ivy
package cn.ipokerface.admin.entity;
import cn.ipokerface.admin.enums.AccountStatus;
import cn.ipokerface.common.model.entity.BaseTableEntity;
/**
*
* [ADMIN_ACCOUNT]
*/
public class AdminAccount extends BaseTableEntity {
/**
* 用户名
* [USERNAME]
* 2021-04-07T11:25:23.406
*/
private String username;
/**
* 用户手机号
* [MOBILE]
* 2021-04-07T11:25:23.409
*/
private String mobile;
/**
* 名字
* [NAME]
* 2021-04-07T11:25:23.409
*/
private String name;
/**
* 密码
* [PASSWORD]
* 2021-04-07T11:25:23.409
*/
private String password;
/**
* 加密参数
* [SALT]
* 2021-04-07T11:25:23.409
*/
private String salt;
/**
* 账号状态 AccountStatus
* [STATUS]
* 2021-04-07T11:25:23.409
*/
private AccountStatus status;
/**
* 备注字段
* [REMARK]
* 2021-04-07T11:25:23.409
*/
private String remark;
/**
* This method was generated by MyBatis Generator.
* This method returns the value of the database column ADMIN_ACCOUNT.USERNAME
*
* @return the value of ADMIN_ACCOUNT.USERNAME
*
* .generated Wed Apr 07 11:25:23 CST 2021
*/
public String getUsername() {
return username;
}
/**
* This method was generated by MyBatis Generator.
* This method sets the value of the database column ADMIN_ACCOUNT.USERNAME
*
* @param username the value for ADMIN_ACCOUNT.USERNAME
*
* .generated Wed Apr 07 11:25:23 CST 2021
*/
public void setUsername(String username) {
this.username = username;
}
/**
* This method was generated by MyBatis Generator.
* This method returns the value of the database column ADMIN_ACCOUNT.MOBILE
*
* @return the value of ADMIN_ACCOUNT.MOBILE
*
* .generated Wed Apr 07 11:25:23 CST 2021
*/
public String getMobile() {
return mobile;
}
/**
* This method was generated by MyBatis Generator.
* This method sets the value of the database column ADMIN_ACCOUNT.MOBILE
*
* @param mobile the value for ADMIN_ACCOUNT.MOBILE
*
* .generated Wed Apr 07 11:25:23 CST 2021
*/
public void setMobile(String mobile) {
this.mobile = mobile;
}
/**
* This method was generated by MyBatis Generator.
* This method returns the value of the database column ADMIN_ACCOUNT.NAME
*
* @return the value of ADMIN_ACCOUNT.NAME
*
* .generated Wed Apr 07 11:25:23 CST 2021
*/
public String getName() {
return name;
}
/**
* This method was generated by MyBatis Generator.
* This method sets the value of the database column ADMIN_ACCOUNT.NAME
*
* @param name the value for ADMIN_ACCOUNT.NAME
*
* .generated Wed Apr 07 11:25:23 CST 2021
*/
public void setName(String name) {
this.name = name;
}
/**
* This method was generated by MyBatis Generator.
* This method returns the value of the database column ADMIN_ACCOUNT.PASSWORD
*
* @return the value of ADMIN_ACCOUNT.PASSWORD
*
* .generated Wed Apr 07 11:25:23 CST 2021
*/
public String getPassword() {
return password;
}
/**
* This method was generated by MyBatis Generator.
* This method sets the value of the database column ADMIN_ACCOUNT.PASSWORD
*
* @param password the value for ADMIN_ACCOUNT.PASSWORD
*
* .generated Wed Apr 07 11:25:23 CST 2021
*/
public void setPassword(String password) {
this.password = password;
}
/**
* This method was generated by MyBatis Generator.
* This method returns the value of the database column ADMIN_ACCOUNT.SALT
*
* @return the value of ADMIN_ACCOUNT.SALT
*
* .generated Wed Apr 07 11:25:23 CST 2021
*/
public String getSalt() {
return salt;
}
/**
* This method was generated by MyBatis Generator.
* This method sets the value of the database column ADMIN_ACCOUNT.SALT
*
* @param salt the value for ADMIN_ACCOUNT.SALT
*
* .generated Wed Apr 07 11:25:23 CST 2021
*/
public void setSalt(String salt) {
this.salt = salt;
}
/**
* This method was generated by MyBatis Generator.
* This method returns the value of the database column ADMIN_ACCOUNT.STATUS
*
* @return the value of ADMIN_ACCOUNT.STATUS
*
* .generated Wed Apr 07 11:25:23 CST 2021
*/
public AccountStatus getStatus() {
return status;
}
/**
* This method was generated by MyBatis Generator.
* This method sets the value of the database column ADMIN_ACCOUNT.STATUS
*
* @param status the value for ADMIN_ACCOUNT.STATUS
*
* .generated Wed Apr 07 11:25:23 CST 2021
*/
public void setStatus(AccountStatus status) {
this.status = status;
}
/**
* This method was generated by MyBatis Generator.
* This method returns the value of the database column ADMIN_ACCOUNT.REMARK
*
* @return the value of ADMIN_ACCOUNT.REMARK
*
* .generated Wed Apr 07 11:25:23 CST 2021
*/
public String getRemark() {
return remark;
}
/**
* This method was generated by MyBatis Generator.
* This method sets the value of the database column ADMIN_ACCOUNT.REMARK
*
* @param remark the value for ADMIN_ACCOUNT.REMARK
*
* .generated Wed Apr 07 11:25:23 CST 2021
*/
public void setRemark(String remark) {
this.remark = remark;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy