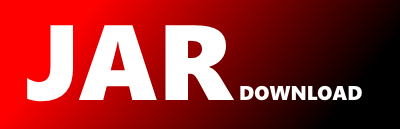
cn.ipokerface.admin.service.AdminServiceImpl Maven / Gradle / Ivy
package cn.ipokerface.admin.service;
import cn.ipokerface.admin.AdminCacheService;
import cn.ipokerface.admin.entity.AdminAccount;
import cn.ipokerface.admin.enums.AccountStatus;
import cn.ipokerface.admin.exception.AccountForbiddenException;
import cn.ipokerface.admin.exception.AccountWrongException;
import cn.ipokerface.admin.exception.PasswordRequireException;
import cn.ipokerface.admin.exception.PasswordWrongException;
import cn.ipokerface.admin.mapper.AdminAccountMapper;
import cn.ipokerface.admin.model.AccountModel;
import cn.ipokerface.common.utils.DigestUtils;
import cn.ipokerface.common.utils.StringUtils;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.beans.factory.annotation.Value;
import org.springframework.stereotype.Service;
import java.util.List;
/**
* Created by PokerFace
* Create Date 2019-12-26.
* Email: [email protected]
* Version 1.0.0
*
* Description:
*/
@Service
public class AdminServiceImpl implements AdminService {
@Autowired
private AdminAccountMapper adminAccountMapper;
@Autowired
private AdminCacheService adminCacheService;
@Override
public AdminAccount login(String username, String password, String ip) {
AdminAccount adminAccount = adminAccountMapper.selectByUsername(username);
if (adminAccount == null) {
throw new AccountWrongException();
}
if (AccountStatus.AccountStatus_Forbidden.equals(adminAccount.getStatus())) {
throw new AccountForbiddenException();
}
if (!DigestUtils.md5Hex(password + adminAccount.getSalt()).equals(adminAccount.getPassword())) {
throw new PasswordWrongException();
}
return adminAccount;
}
@Override
public void resetPassword(Long accountId, String password, String newPassword) {
if (StringUtils.isEmpty(password) || StringUtils.isEmpty(newPassword)) {
throw new PasswordRequireException();
}
AdminAccount systemAccount = adminAccountMapper.selectByPrimaryKey(accountId);
if (systemAccount == null) {
throw new AccountWrongException();
}
// 验证密码
String md5password = DigestUtils.md5Hex(password + systemAccount.getSalt());
if (!md5password.equals(systemAccount.getPassword()))
throw new PasswordWrongException();
adminAccountMapper.updatePassword(accountId,DigestUtils.md5Hex(newPassword + systemAccount.getSalt()));
}
}