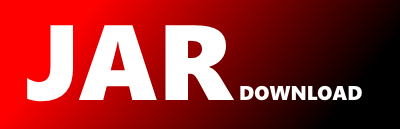
cn.ipokerface.aps.notification.Notification Maven / Gradle / Ivy
Show all versions of apple-pns-java Show documentation
package cn.ipokerface.aps.notification;
import cn.ipokerface.aps.Constant;
import com.alibaba.fastjson.JSON;
import org.apache.commons.lang3.StringUtils;
import java.util.HashMap;
import java.util.Map;
import java.util.UUID;
/**
* Created by PokerFace
* Create Date 2020-08-23.
* Email: [email protected]
* Version 1.0.0
*
* Description:
*/
public class Notification {
/**
* user's device token...
*
*/
private String deviceToken;
/**
* {@link Priority}
* {@link Priority#Immediately}
* {@link Priority#PowerConsideration}
* The priority of the notification. If you omit this header, APNs sets the notification priority to 10.
*
* Specify 10 to send the notification immediately. A value of 10 is appropriate for notifications that trigger an
* alert, play a sound, or badge the app’s icon. Specifying this priority for a notification that has a payload
* containing the content-available key causes an error.
*
* Specify 5 to send the notification based on power considerations on the user’s device. Use this priority for
* notifications that have a payload that includes the content-available key. Notifications with this priority might
* be grouped and delivered in bursts to the user’s device. They may also be throttled, and in some cases not delivered.
*
* see
* https://developer.apple.com/documentation/usernotifications/setting_up_a_remote_notification_server/sending_notification_requests_to_apns/}
*
*/
private Priority priority;
/**
* expire time in second (UTC) ...
* The date at which the notification is no longer valid. This value is a UNIX epoch expressed in seconds (UTC).
* If the value is nonzero, APNs stores the notification and tries to deliver it at least once, repeating the attempt
* as needed until the specified date. If the value is 0, APNs attempts to deliver the notification only once and doesn’t store it.
*
* see apns documention
* https://developer.apple.com/documentation/usernotifications/setting_up_a_remote_notification_server/sending_notification_requests_to_apns/}
*/
private long expiration;
/**
* push type.
* (Required for watchOS 6 and later; recommended for macOS, iOS, tvOS, and iPadOS) The value of this header must
* accurately reflect the contents of your notification’s payload. If there is a mismatch, or if the header is missing
* on required systems, APNs may return an error, delay the delivery of the notification, or drop it altogether.
* {@link Type}
*/
private Type type;
/**
* The topic for the notification. In general, the topic is your app’s bundle ID, but it may have a suffix
* based on the push notification’s type.
*/
private String topic;
/**
* (Required for token-based authentication) The value of this header is bearer , where
* is the encrypted token that authorizes you to send notifications for the specified topic.
* APNs ignores this header if you use certificate-based authentication. For more information, see Establishing
* a Token-Based Connection to APNs.
*
*/
private String authorization;
/**
* An identifier you use to coalesce multiple notifications into a single notification for the user. Typically,
* each notification request causes a new notification to be displayed on the user’s device. When sending the same
* notification more than once, use the same value in this header to coalesce the requests.
* The value of this key must not exceed 64 bytes.
*/
private String apnsCollapseId;
/**
* 推送数据
*
*/
private NotificationAps aps;
/**
* 推送自定义数据
*/
private Map payload;
private String apnsId;
private Notification() {
}
public String getDeviceToken() {
return deviceToken;
}
public Priority getPriority() {
return priority;
}
public long getExpiration() {
return expiration;
}
public Type getType() {
return type;
}
public String getTopic() {
return topic;
}
public String getAuthorization() {
return authorization;
}
public String getApnsCollapseId() {
return apnsCollapseId;
}
public NotificationAps getAps() {
return aps;
}
public Map getPayload() {
return payload;
}
public String getApnsId() {
return apnsId;
}
/**
*
* {"aps": {"alert": {"title": "测试"}}, "custom": "aaa"}
*
* @return
*/
public String payloadJson() {
if (this.payload == null) this.payload = new HashMap<>();
this.payload.put(Constant.payload_key_aps, this.aps);
return JSON.toJSONString(this.payload);
}
@Override
public String toString() {
return "Notification{" +
"deviceToken='" + deviceToken + '\'' +
", priority=" + priority +
", expiration=" + expiration +
", type=" + type +
", topic='" + topic + '\'' +
", authorization='" + authorization + '\'' +
", apnsCollapseId='" + apnsCollapseId + '\'' +
", aps=" + aps +
", payload=" + payload +
", apnsId='" + apnsId + '\'' +
'}';
}
public static class Builder {
private String deviceToken;
private long expiration = 0;
private Priority priority = Priority.Immediately;
private Type type = Type.alert;
private String topic;
private String authorization;
private String apnsCollapseId;
private NotificationAps aps;
private Map extension;
public Builder(){
}
/**
* user's deviceToken
*
* @param deviceToken
* @return
*/
public Builder device(String deviceToken) {
this.deviceToken = deviceToken;
return this;
}
/**
* expiration time seconds
* {@link Notification#expiration}
*
* @param expireSeconds
* @return
*/
public Builder expiration(long expireSeconds) {
this.expiration = expireSeconds;
return this;
}
/**
* {@link Priority}
*
* @param priority
* @return
*/
public Builder priority(Priority priority) {
this.priority = priority;
return this;
}
/**
* {@link Type}
*
* @param type
* @return
*/
public Builder type(Type type) {
this.type = type;
return this;
}
/**
* default PushClient topic means bundle id
* {@link cn.ipokerface.aps.PushClient}
*
* @param topic
* @return
*/
public Builder topic(String topic) {
this.topic = topic;
return this;
}
/**
* {@link Notification#authorization}
*
* @param authorization
* @return
*/
public Builder authorization(String authorization) {
this.authorization = authorization;
return this;
}
/**
* {@link Notification#apnsCollapseId}
* @param apnsCollapseId
* @return
*/
public Builder apnsCollapseId(String apnsCollapseId) {
this.apnsCollapseId = apnsCollapseId;
return this;
}
/**
* payload data.
* {@link NotificationAps}
*
* @param aps
* @return
*/
public Builder payload(NotificationAps aps) {
this.aps = aps;
return this;
}
/**
* custom data.
*
* @param name
* @param value
* @return
*/
public Builder extension(String name, Object value) {
if (StringUtils.isEmpty(name) || value == null) return this;
if (this.extension == null) extension = new HashMap<>();
extension.put(name, value);
return this;
}
/**
* build for Notification
* {@link Notification}
*
* @return
*/
public Notification build() {
Notification notification = new Notification();
notification.deviceToken = this.deviceToken;
notification.priority = this.priority;
notification.expiration = this.expiration;
notification.type = this.type;
notification.authorization = this.authorization;
notification.topic = this.topic;
notification.apnsCollapseId = this.apnsCollapseId;
notification.aps = this.aps;
if (this.extension == null) this.extension = new HashMap<>();
if (aps != null) {
extension.put(Constant.payload_key_aps, aps);
}
notification.payload = this.extension;
notification.apnsId = UUID.randomUUID().toString().toLowerCase();
return notification;
}
}
public enum Priority {
Immediately(10),
PowerConsideration(5);
private final int code;
private Priority(int code) {
this.code = code;
}
public int getCode() {
return this.code;
}
}
/**
* apns-push-type
*
* see
* https://developer.apple.com/documentation/usernotifications/setting_up_a_remote_notification_server/sending_notification_requests_to_apns/}
*
*
*/
public enum Type {
/**
* Use the alert push type for notifications that trigger a user interaction—for example,
* an alert, badge, or sound. If you set this push type, the apns-topic header field must
* use your app’s bundle ID as the topic. For more information, see Generating a Remote Notification.
*
* The alert push type is required on watchOS 6 and later. It is recommended on macOS, iOS, tvOS, and iPadOS.
*/
alert,
/**
* Use the background push type for notifications that deliver content in the background, and don’t
* trigger any user interactions. If you set this push type, the apns-topic header field must use
* your app’s bundle ID as the topic. For more information, see Pushing Background Updates to Your App.
*
* The background push type is required on watchOS 6 and later. It is recommended on macOS, iOS, tvOS, and iPadOS.
*
*/
background,
/**
* Use the voip push type for notifications that provide information about an incoming Voice-over-IP (VoIP) call.
* For more information, see Responding to VoIP Notifications from PushKit.
*
* If you set this push type, the apns-topic header field must use your app’s
* bundle ID with .voip appended to the end. If you’re using certificate-based authentication, you must also
* register the certificate for VoIP services. The topic is then part of the 1.2.840.113635.100.6.3.4
* or 1.2.840.113635.100.6.3.6 extension.
*
* The voip push type is not available on watchOS. It is recommended on macOS,
* iOS, tvOS, and iPadOS.
*/
voip,
/**
* Use the complication push type for notifications that contain update information for a watchOS app’s
* complications. For more information, see Updating Your Complication.
*
* If you set this push type, the apns-topic header field must use your app’s bundle ID with
* .complication appended to the end. If you’re using certificate-based authentication, you must also
* register the certificate for WatchKit services. The topic is then part of the 1.2.840.113635.100.6.3.6 extension.
*
* The complication push type is recommended for watchOS and iOS. It is not available on macOS,
* tvOS, and iPadOS.
*/
complication,
/**
* Use the fileprovider push type to signal changes to a File Provider extension. If you set this push type,
* the apns-topic header field must use your app’s bundle ID with .pushkit.fileprovider appended to the end.
* For more information, see Using Push Notifications to Signal Changes.
*
* The fileprovider push type is not available on watchOS. It is recommended on macOS, iOS, tvOS, and iPadOS.
*
*/
fileprovider,
/**
* Use the mdm push type for notifications that tell managed devices to contact the MDM server. If you set this
* push type, you must use the topic from the UID attribute in the subject of your MDM push certificate.
* For more information, see Device Management.
*
* The mdm push type is not available on watchOS. It is recommended on macOS, iOS, tvOS, and iPadOS.
*/
mdm;
@Override
public String toString() {
return name();
}
}
}