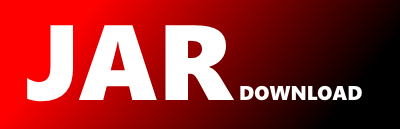
cn.ipokerface.aps.notification.NotificationAps Maven / Gradle / Ivy
Show all versions of apple-pns-java Show documentation
package cn.ipokerface.aps.notification;
import com.alibaba.fastjson.annotation.JSONField;
/**
* Created by PokerFace
* Create Date 2020-08-23.
* Email: [email protected]
* Version 1.0.0
*
* Description: https://developer.apple.com/documentation/usernotifications/setting_up_a_remote_notification_server/generating_a_remote_notification
*/
public class NotificationAps {
/**
* The information for displaying an alert. A dictionary is recommended. If you specify a string, the alert displays
* your string as the body text. For a list of dictionary keys, see Table 2.
*
* {@link NotificationAlert}
*
*/
private NotificationAlert alert;
/**
* The number to display in a badge on your app’s icon. Specify 0 to remove the current badge, if any.
*
*/
private Integer badge;
/**
* use string or jsonString to define sound resources.
* A dictionary that contains sound information for critical alerts.
* For regular notifications, use the sound string instead.
* more see
* https://developer.apple.com/documentation/usernotifications/setting_up_a_remote_notification_server/generating_a_remote_notification
*
*/
private String sound;
/**
* An app-specific identifier for grouping related notifications. This value corresponds to the
* threadIdentifier property in the UNNotificationContent object.
*/
@JSONField(name = "thread-id")
private String threadId;
/**
* The notification’s type. This string must correspond to the identifier of one of the UNNotificationCategory
* objects you register at launch time. See Declaring Your Actionable Notification Types.
*/
private String category;
/**
* The background notification flag. To perform a silent background update, specify the value 1 and don't
* include the alert, badge, or sound keys in your payload. See Pushing Background Updates to Your App.
*/
@JSONField(name = "content-available")
private Integer contentAvailable;
/**
* The notification service app extension flag. If the value is 1, the system passes the notification to your
* notification service app extension before delivery. Use your extension to modify the notification’s content.
* See Modifying Content in Newly Delivered Notifications.
*/
@JSONField(name = "mutable-content")
private Integer mutableContent;
/**
* The identifier of the window brought forward. The value of this key will be populated on the
* UNNotificationContent object created from the push payload. Access the value using the UNNotificationContent
* object's targetContentIdentifier property.
*/
@JSONField(name = "target-content-id")
private String targetContentId;
public NotificationAlert getAlert() {
return alert;
}
public void setAlert(NotificationAlert alert) {
this.alert = alert;
}
public int getBadge() {
return badge;
}
public void setBadge(int badge) {
this.badge = badge;
}
public String getSound() {
return sound;
}
public void setSound(String sound) {
this.sound = sound;
}
public String getThreadId() {
return threadId;
}
public void setThreadId(String threadId) {
this.threadId = threadId;
}
public String getCategory() {
return category;
}
public void setCategory(String category) {
this.category = category;
}
public Integer getContentAvailable() {
return contentAvailable;
}
public void setContentAvailable(Integer contentAvailable) {
this.contentAvailable = contentAvailable;
}
public Integer getMutableContent() {
return mutableContent;
}
public void setMutableContent(Integer mutableContent) {
this.mutableContent = mutableContent;
}
public String getTargetContentId() {
return targetContentId;
}
public void setTargetContentId(String targetContentId) {
this.targetContentId = targetContentId;
}
public static class Builder{
private NotificationAlert alert;
private int badge = 0;
private String sound;
private String threadId;
private String category;
private Integer contentAvailable;
private Integer mutableContent;
private String targetContentId;
public Builder() {
}
public Builder alert(NotificationAlert alert){
this.alert = alert;
return this;
}
public Builder badge(int badge){
this.badge = badge;
return this;
}
public Builder sound(String sound){
this.sound = sound;
return this;
}
/**
* set push sound as 'default'
*
*
* @return builder
*/
public Builder soundDefault() {
this.sound = "default";
return this;
}
public Builder threadId(String threadId){
this.threadId = threadId;
return this;
}
public Builder category(String category){
this.category = category;
return this;
}
public Builder contentAvailable(Integer contentAvailable){
this.contentAvailable = contentAvailable;
return this;
}
public Builder mutableContent(Integer mutableContent){
this.mutableContent = mutableContent;
return this;
}
public Builder targetContentId(String targetContentId){
this.targetContentId = targetContentId;
return this;
}
/**
* build for Notification Aps
*
* @return
*/
public NotificationAps build(){
NotificationAps notificationAps = new NotificationAps();
notificationAps.alert = this.alert;
notificationAps.badge = this.badge;
notificationAps.sound = this.sound;
notificationAps.threadId = this.threadId;
notificationAps.category = this.category;
notificationAps.contentAvailable = this.contentAvailable;
notificationAps.mutableContent = this.mutableContent;
notificationAps.targetContentId = this.targetContentId;
return notificationAps;
}
}
}