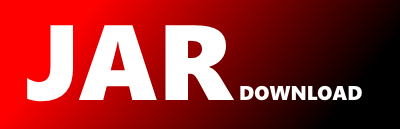
cn.ipokerface.common.utils.AesEncryptUtil Maven / Gradle / Ivy
Show all versions of common Show documentation
package cn.ipokerface.common.utils;
import javax.crypto.BadPaddingException;
import javax.crypto.Cipher;
import javax.crypto.IllegalBlockSizeException;
import javax.crypto.NoSuchPaddingException;
import javax.crypto.spec.IvParameterSpec;
import javax.crypto.spec.SecretKeySpec;
import java.io.UnsupportedEncodingException;
import java.security.InvalidAlgorithmParameterException;
import java.security.InvalidKeyException;
import java.security.NoSuchAlgorithmException;
import java.util.Base64;
/**
* Created by PokerFace
* Create Date 2020-08-27.
* Email:
* Version 1.0.0
*
* Description:
*/
public class AesEncryptUtil {
private static final String encoding = "UTF-8";
private static final String algorithm = "AES/CBC/PKCS5Padding";
private static final String algorithm_type = "AES";
/**
* 加密字符串
*
* @param content 需要加密的内容
* @param key 加密密钥 key
* @param iv 加密向量 16位
* @return encrypted data
* @throws InvalidKeyException InvalidKeyException
* @throws NoSuchAlgorithmException NoSuchAlgorithmException
* @throws NoSuchPaddingException NoSuchPaddingException
* @throws UnsupportedEncodingException UnsupportedEncodingException
* @throws InvalidAlgorithmParameterException InvalidAlgorithmParameterException
* @throws IllegalBlockSizeException IllegalBlockSizeException
* @throws BadPaddingException BadPaddingException
*/
public static String encrypt(String content, String key, String iv) throws InvalidKeyException,
NoSuchAlgorithmException, NoSuchPaddingException, UnsupportedEncodingException,
InvalidAlgorithmParameterException, IllegalBlockSizeException, BadPaddingException{
byte[] contentBytes = content.getBytes(encoding);
byte[] keyBytes = key.getBytes(encoding);
byte[] ivBytes = iv.getBytes(encoding);
byte[] encrypted = encrypt(contentBytes, keyBytes, ivBytes);
return Base64.getEncoder().encodeToString(encrypted);
}
/**
* 加密
* content 需要加密的byte数组
* key 需要加密时的密钥, 自定义
* iv 加密时的向量, 可自定义 但必须为16位
*
* @param content value to decode
* @param key decode key
* @param iv iv value
* @return decoded data
* @throws InvalidKeyException InvalidKeyException
* @throws NoSuchAlgorithmException NoSuchAlgorithmException
* @throws NoSuchPaddingException NoSuchPaddingException
* @throws UnsupportedEncodingException UnsupportedEncodingException
* @throws InvalidAlgorithmParameterException InvalidAlgorithmParameterException
* @throws IllegalBlockSizeException IllegalBlockSizeException
* @throws BadPaddingException BadPaddingException
*/
public static byte[] encrypt(byte[] content, byte[] key, byte[] iv) throws InvalidKeyException,
NoSuchAlgorithmException, NoSuchPaddingException, UnsupportedEncodingException,
InvalidAlgorithmParameterException, IllegalBlockSizeException, BadPaddingException {
Cipher cipher = initCipher(key, iv, Cipher.ENCRYPT_MODE);
byte[] encrypted = cipher.doFinal(content);
return encrypted;
}
/**
* 解密运算
* content 由key,iv 加密后经过base64处理的字符串
*
* @param content 需要解密的数据
* @param key 解密时的key
* @param iv 解密时的iv
* @return 解密后的值
* @throws InvalidKeyException InvalidKeyException
* @throws NoSuchAlgorithmException NoSuchAlgorithmException
* @throws NoSuchPaddingException NoSuchPaddingException
* @throws UnsupportedEncodingException UnsupportedEncodingException
* @throws InvalidAlgorithmParameterException InvalidAlgorithmParameterException
* @throws IllegalBlockSizeException IllegalBlockSizeException
* @throws BadPaddingException BadPaddingException
*/
public static String decrypt(String content, String key, String iv) throws InvalidKeyException,
NoSuchAlgorithmException, NoSuchPaddingException, UnsupportedEncodingException,
InvalidAlgorithmParameterException, IllegalBlockSizeException, BadPaddingException {
byte[] contentBytes = Base64.getDecoder().decode(content);
byte[] keyBytes = key.getBytes(encoding);
byte[] ivBytes = iv.getBytes(encoding);
byte[] decrypted = decrypt(contentBytes, keyBytes, ivBytes);
return new String(decrypted, encoding);
}
/**
* 进行解密运算
* content: 由AES 和key ,iv 加密运算的加密串
* key: 加密时的key
* iv: 加密时的iv
*
* @param content 需要解密的数据
* @param key 解密时的key
* @param iv 解密时的iv
* @return 解密后的值
* @throws InvalidKeyException InvalidKeyException
* @throws NoSuchAlgorithmException NoSuchAlgorithmException
* @throws NoSuchPaddingException NoSuchPaddingException
* @throws UnsupportedEncodingException UnsupportedEncodingException
* @throws InvalidAlgorithmParameterException InvalidAlgorithmParameterException
* @throws IllegalBlockSizeException IllegalBlockSizeException
* @throws BadPaddingException BadPaddingException
*/
public static byte[] decrypt(byte[] content, byte[] key, byte[] iv) throws InvalidKeyException,
NoSuchAlgorithmException, NoSuchPaddingException, UnsupportedEncodingException,
InvalidAlgorithmParameterException, IllegalBlockSizeException, BadPaddingException {
Cipher cipher = initCipher(key, iv, Cipher.DECRYPT_MODE);
byte[] decrypted = cipher.doFinal(content);
return decrypted;
}
/**
* 获得运算器
*
* @param key
* @param iv 运算向量, 必须是16位的byte[]
* @param mode 运算模式 {@link Cipher#ENCRYPT_MODE} {@link Cipher#DECRYPT_MODE}
* @return 运算器
* @throws UnsupportedEncodingException UnsupportedEncodingException
* @throws NoSuchAlgorithmException NoSuchAlgorithmException
* @throws NoSuchPaddingException NoSuchPaddingException
* @throws InvalidKeyException InvalidKeyException
* @throws InvalidAlgorithmParameterException InvalidAlgorithmParameterException
*/
private static Cipher initCipher(byte[] key, byte[] iv, int mode) throws UnsupportedEncodingException, NoSuchAlgorithmException,
NoSuchPaddingException,InvalidKeyException, InvalidAlgorithmParameterException {
// 注意,为了能与 iOS 统一
// 这里的 key 不可以使用 KeyGenerator、SecureRandom、SecretKey 生成
// 生产指定参数
SecretKeySpec secretKeySpec = new SecretKeySpec(key, algorithm_type);
// 获取向量参数
IvParameterSpec ivParameterSpec = new IvParameterSpec(iv);
// 指定加密的算法、工作模式和填充方式
Cipher cipher = Cipher.getInstance(algorithm);
// 初始化运算器
cipher.init(mode, secretKeySpec, ivParameterSpec);
return cipher;
}
}