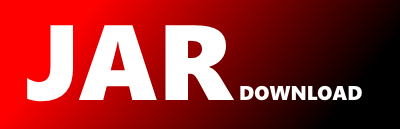
org.shoulder.crypto.negotiation.cache.MemoryNegotiationResultCache Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of shoulder-crypto-negotiation Show documentation
Show all versions of shoulder-crypto-negotiation Show documentation
Shoulder 提供的 协商加密模块,用于非信任网络环境下的安全通信。基于 DH + ECC 实现先进的加密算法协商算法,比传统的 DH + DES 协商算法性能显著更高,更安全。
package org.shoulder.crypto.negotiation.cache;
import jakarta.annotation.Nonnull;
import jakarta.annotation.Nullable;
import org.shoulder.crypto.negotiation.dto.NegotiationResult;
import java.util.Map;
import java.util.concurrent.ConcurrentHashMap;
/**
* 密钥协商结果缓存
*
* @author lym
*/
public class MemoryNegotiationResultCache implements NegotiationResultCache {
/**
* 客户端缓存,key为对方应用标识
*/
private static final Map clientKeyExchangeResultMap = new ConcurrentHashMap<>(8);
/**
* 作为服务方缓存,key为 xSessionId
*/
private static final Map serverKeyExchangeResultMap = new ConcurrentHashMap<>(8);
@Override
public void put(@Nonnull String cacheKey, @Nonnull NegotiationResult negotiationResult, boolean asClient) {
Map keyExchangeResultMap = getKeyExchangeCacheMap(asClient);
keyExchangeResultMap.put(cacheKey, negotiationResult);
}
@Override
@Nullable
public NegotiationResult get(String cacheKey, boolean asClient) {
Map keyExchangeResultMap = getKeyExchangeCacheMap(asClient);
long now = System.currentTimeMillis();
NegotiationResult cacheResult = keyExchangeResultMap.get(cacheKey);
if (cacheResult == null) {
// 不存在
return null;
}
if (now > cacheResult.getExpireTime()) {
// 过期,清理,并返回 null
keyExchangeResultMap.remove(cacheKey);
return null;
} else {
// 存在且未过期
return cacheResult;
}
}
@Override
public void delete(String cacheKey, boolean asClient) {
Map keyExchangeCache = getKeyExchangeCacheMap(asClient);
keyExchangeCache.remove(cacheKey);
}
private Map getKeyExchangeCacheMap(boolean asClient) {
return asClient ? clientKeyExchangeResultMap : serverKeyExchangeResultMap;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy