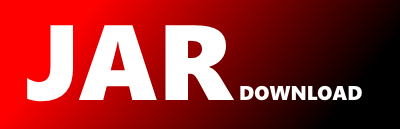
org.shoulder.web.template.crud.SaveController Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of shoulder-web Show documentation
Show all versions of shoulder-web Show documentation
shoulder WEB 模块,基于Spring Boot Web提供了 Controller AOP 日志、AOP异常处理,统一返回值,健康检查,租户、用户解析,Web
安全防护,通用CrudController,动态字典,标签管理,HTTP client AOP日志、AOP异常处理等能力,助力Web飞速开发。
The newest version!
package org.shoulder.web.template.crud;
import com.baomidou.mybatisplus.core.toolkit.reflect.GenericTypeUtils;
import io.swagger.v3.oas.annotations.Operation;
import jakarta.validation.Valid;
import jakarta.validation.constraints.NotNull;
import org.shoulder.core.dto.response.BaseResult;
import org.shoulder.core.exception.CommonErrorCodeEnum;
import org.shoulder.core.model.Operable;
import org.shoulder.core.util.AssertUtils;
import org.shoulder.data.mybatis.template.entity.BaseEntity;
import org.shoulder.data.mybatis.template.entity.BizEntity;
import org.shoulder.log.operation.annotation.OperationLog;
import org.shoulder.log.operation.annotation.OperationLogParam;
import org.shoulder.log.operation.context.OpLogContextHolder;
import org.shoulder.validate.groups.Create;
import org.springframework.transaction.annotation.Transactional;
import org.springframework.validation.annotation.Validated;
import org.springframework.web.bind.annotation.PostMapping;
import org.springframework.web.bind.annotation.RequestBody;
import java.io.Serializable;
/**
* 新增 API
*
* 暴露以下接口:
* POST / 新建一个,可根据 bizId 或 biz源字段自动幂等
*
* @param 实体
* @param DTO
* @author lym
*/
public interface SaveController<
ENTITY extends BaseEntity extends Serializable>,
SAVE_DTO extends Serializable,
SAVE_RESULT_DTO extends Serializable>
extends BaseController {
/**
* 新增
* service.save —— mapper.insert
*
* @param dto 保存参数
* @return ok
*/
@Operation(summary = "新增")
@PostMapping
@OperationLog(operation = OperationLog.Operations.CREATE)
@Validated(Create.class)
@Transactional(rollbackFor = Exception.class)
default BaseResult save(@OperationLogParam @RequestBody @Valid @NotNull SAVE_DTO dto) {
ENTITY entity = handleBeforeSaveAndConvertToEntity(dto);
if (Operable.class.isAssignableFrom(getEntityClass())) {
if (entity != null) {
OpLogContextHolder.setOperableObject(entity);
}
OpLogContextHolder.getLog().setObjectType(getEntityObjectType());
}
AssertUtils.notNull(entity, CommonErrorCodeEnum.ILLEGAL_PARAM);
if (entity instanceof BizEntity extends Serializable> bizEntity) {
if (bizEntity.getBizId() == null) {
String bizId = generateBizId(entity);
bizEntity.setBizId(bizId);
}
ENTITY dataInDb = getService().lockByBizId(bizEntity.getBizId());
// 数据已存在
AssertUtils.isNull(dataInDb, CommonErrorCodeEnum.DATA_ALREADY_EXISTS);
}
getService().save(entity);
return BaseResult.success(convertEntityToSaveResultDTO(entity));
}
String generateBizId(ENTITY entity);
/**
* 新增前扩展点
*
* @param dto DTO
* @return entity
*/
default ENTITY handleBeforeSaveAndConvertToEntity(SAVE_DTO dto) {
return getConversionService().convert(dto, getEntityClass());
}
@SuppressWarnings("unchecked")
default Class getSaveResultDTOClass() {
return (Class) GenericTypeUtils.resolveTypeArguments(this.getClass(), SaveController.class)[2];
}
default SAVE_RESULT_DTO convertEntityToSaveResultDTO(ENTITY entity) {
return getConversionService().convert(entity, getSaveResultDTOClass());
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy