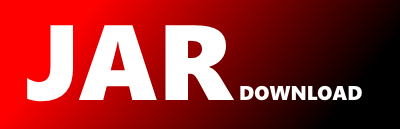
org.shoulder.web.template.dictionary.controller.DictionaryItemEnumController Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of shoulder-web Show documentation
Show all versions of shoulder-web Show documentation
shoulder WEB 模块,基于Spring Boot Web提供了 Controller AOP 日志、AOP异常处理,统一返回值,健康检查,租户、用户解析,Web
安全防护,通用CrudController,动态字典,标签管理,HTTP client AOP日志、AOP异常处理等能力,助力Web飞速开发。
The newest version!
package org.shoulder.web.template.dictionary.controller;
import io.swagger.v3.oas.annotations.Operation;
import io.swagger.v3.oas.annotations.Parameter;
import io.swagger.v3.oas.annotations.Parameters;
import io.swagger.v3.oas.annotations.tags.Tag;
import org.shoulder.core.converter.ShoulderConversionService;
import org.shoulder.core.dictionary.model.DictionaryItemEnum;
import org.shoulder.core.dictionary.spi.DictionaryEnumStore;
import org.shoulder.core.dto.response.BaseResult;
import org.shoulder.core.dto.response.ListResult;
import org.shoulder.log.operation.annotation.OperationLogParam;
import org.shoulder.web.template.dictionary.dto.DictionaryBatchQueryParam;
import org.shoulder.web.template.dictionary.dto.DictionaryItemDTO;
import org.shoulder.web.template.dictionary.dto.DictionaryTypeDTO;
import org.springframework.validation.annotation.Validated;
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.PathVariable;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.RequestMethod;
import org.springframework.web.bind.annotation.RestController;
import java.util.List;
import java.util.stream.Collectors;
@Tag(name = "DictionaryItemEnumController", description = "枚举字典-枚举项查询(只读)")
@RestController
@RequestMapping(value = "${shoulder.web.ext.dictionary.path:/api/v1/dictionary}")
public class DictionaryItemEnumController implements DictionaryItemController {
/**
* 字典枚举存储
*/
private final DictionaryEnumStore dictionaryEnumStore;
protected final ShoulderConversionService conversionService;
public DictionaryItemEnumController(DictionaryEnumStore dictionaryEnumStore, ShoulderConversionService conversionService) {
this.dictionaryEnumStore = dictionaryEnumStore;
this.conversionService = conversionService;
}
/**
* 查询单个字典的所有字典项
*
* @param dictionaryType 字典项类型
* @return 查询结果
*/
@Parameters({
@Parameter(name = "dictionaryType", description = "字典类型"),
})
@Operation(summary = "查询单个字典项", description = "查询单个字典项")
@GetMapping("/listByType/{dictionaryType}")
public BaseResult> listByType(@OperationLogParam @PathVariable("dictionaryType") String dictionaryType) {
List dictionaryItemList = query(dictionaryType);
//dictionaryItemList.sort(DictionaryItemDTO::compareTo); // 枚举添加的时候已经排序了,暂不需要这行代码
return BaseResult.success(dictionaryItemList);
}
/**
* 根据类型查询多个字典项
*
* @param batchQueryParam 字典项类型 List
* @return 查询结果
*/
@Parameters({
@Parameter(name = "batchQueryParam", description = "字典类型 list"),
})
@Operation(summary = "查询多个字典项", description = "查询多个字典项")
@RequestMapping(value = "/listByTypes", method = { RequestMethod.GET, RequestMethod.POST })
public BaseResult> listAllByTypes(@Validated DictionaryBatchQueryParam batchQueryParam) {
List dictionaryList = batchQueryParam.getDictionaryTypeList().stream()
.map(type -> new DictionaryTypeDTO(type, query(type)))
.collect(Collectors.toList());
return BaseResult.success(dictionaryList);
}
@SuppressWarnings("rawtypes")
private List query(String dictionaryType) {
List> enumItems = dictionaryEnumStore.listAllAsDictionaryEnum(dictionaryType);
return enumItems.stream()
.map(e -> (DictionaryItemEnum) e)
.map(d -> conversionService.convert(d, DictionaryItemDTO.class))
.collect(Collectors.toList());
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy