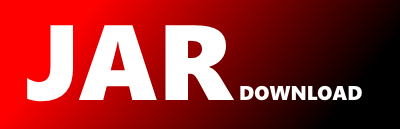
cn.jiangzeyin.database.config.DatabaseContextHolder Maven / Gradle / Ivy
package cn.jiangzeyin.database.config;
import cn.jiangzeyin.system.SystemDbLog;
import cn.jiangzeyin.util.Assert;
import javax.sql.DataSource;
import java.sql.Connection;
import java.sql.SQLException;
import java.util.Map;
import java.util.Random;
/**
* Created by jiangzeyin on 2017/1/6.
*/
public final class DatabaseContextHolder {
private DatabaseContextHolder() {
}
private static final ThreadLocal threadLocal = new ThreadLocal<>();
private static final Random random = new Random();
private static Map[] MAPS;
private static String[] tagNames;
private static DatabaseOptType databaseOptType = DatabaseOptType.One;
private static Map targetDataSourcesMap;
public enum DatabaseOptType {
One, Two, More
}
public static String getConnectionTagName() {
return threadLocal.get();
}
static void init(Map[] maps, String[] tagName) {
Assert.notNull(maps);
if (maps.length == 0)
throw new IllegalArgumentException("数据库连接信息不能为空");
DatabaseContextHolder.MAPS = maps;
DatabaseContextHolder.tagNames = tagName;
if (maps.length == 1)
DatabaseContextHolder.databaseOptType = DatabaseOptType.One;
else if (maps.length == 2)
DatabaseContextHolder.databaseOptType = DatabaseOptType.Two;
else
DatabaseContextHolder.databaseOptType = DatabaseOptType.More;
DatabaseContextHolder.targetDataSourcesMap = MAPS[0];
SystemDbLog.getInstance().info(" 数据库操作:" + databaseOptType.toString());
}
static void init(Map map) {
Assert.notNull(map);
if (map.size() < 1)
throw new RuntimeException("数据库连接加载为空");
DatabaseContextHolder.targetDataSourcesMap = map;
SystemDbLog.getInstance().info(" 数据库操作:" + databaseOptType.toString());
}
// public static void setThreadLocal(String tag) {
// threadLocal.set(tag);
// }
// public void setTargetDataSources(Map
© 2015 - 2025 Weber Informatics LLC | Privacy Policy