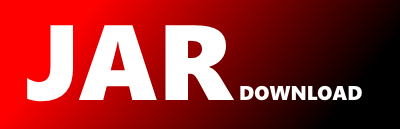
cn.jiangzeyin.system.SystemThreadFactory Maven / Gradle / Ivy
package cn.jiangzeyin.system;
import com.alibaba.druid.util.StringUtils;
import java.util.concurrent.ThreadFactory;
import java.util.concurrent.atomic.AtomicInteger;
/**
* 线程池工厂
*
* @author jiangzeyin
*/
public class SystemThreadFactory implements ThreadFactory {
private static final AtomicInteger poolNumber = new AtomicInteger(1);
private final ThreadGroup group;
private final AtomicInteger threadNumber = new AtomicInteger(1);
private final String namePrefix;
/**
* @return the threadNumber
*/
public int getThreadNumber() {
return threadNumber.get();
}
SystemThreadFactory(String poolName) {
if (StringUtils.isEmpty(poolName))
poolName = "pool";
SecurityManager s = System.getSecurityManager();
group = (s != null) ? s.getThreadGroup() : Thread.currentThread().getThreadGroup();
namePrefix = poolName + "-" + poolNumber.getAndIncrement() + "-thread-";
}
@Override
public Thread newThread(Runnable r) {
Thread t = new Thread(group, r, namePrefix + threadNumber.getAndIncrement(), 0);
if (t.isDaemon())
t.setDaemon(false);
if (t.getPriority() != Thread.NORM_PRIORITY)
t.setPriority(Thread.NORM_PRIORITY);
return t;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy