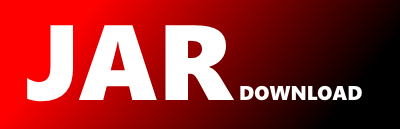
cn.jiangzeyin.database.base.ReadBase Maven / Gradle / Ivy
package cn.jiangzeyin.database.base;
import cn.jiangzeyin.StringUtil;
import cn.jiangzeyin.database.config.SystemColumn;
import com.alibaba.druid.util.StringUtils;
import java.util.ArrayList;
import java.util.Collections;
import java.util.LinkedList;
import java.util.List;
/**
* 读取数据
*
* @author jiangzeyin
*/
@SuppressWarnings("unchecked")
public abstract class ReadBase extends Base {
/**
* 返回值类型
*
* @author jiangzeyin
*/
public enum Result {
/**
* 数组
*/
JsonArray,
/**
* 返回单个实体 json 一行
*/
JsonObject,
/**
* 返回实体新
*/
Entity,
/**
* 原数据格式
*/
ListMap,
/**
* 支持取一行数据
*
* columns 确定取值的列名
*
* 默认第一行第一列
*/
String,
/**
* 支持取一行数据
*
* columns 确定取值的列名
*
* 默认第一行第一列
*/
Integer,
/**
* 分页信息查询
*/
PageResultType;
}
protected ReadBase() {
setThrows(true);
}
/**
* 查询哪些列
*/
protected String columns;
/**
* 查询索引
*/
private String index;
/**
* 参数
*/
private List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy