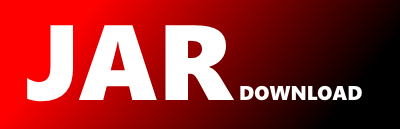
cn.jiangzeyin.database.config.DataSourceConfig Maven / Gradle / Ivy
package cn.jiangzeyin.database.config;
import cn.jiangzeyin.StringUtil;
import cn.jiangzeyin.des.SystemKey;
import cn.jiangzeyin.system.DbLog;
import cn.jiangzeyin.util.PropertiesParser;
import cn.jiangzeyin.util.ResourceUtil;
import com.alibaba.druid.pool.DruidDataSourceFactory;
import javax.sql.DataSource;
import java.io.IOException;
import java.io.InputStream;
import java.net.InetSocketAddress;
import java.net.Socket;
import java.util.*;
/**
* 数据源配置信息
*
* @author jiangzeyin
*/
public final class DataSourceConfig {
private static boolean active;
private static PropertiesParser systemPropertiesParser;
public static boolean isActive() {
return active;
}
private DataSourceConfig() {
}
/**
* 配置对象初始化
*
* @param props props
* @throws Exception e
*/
public static void init(Properties props) throws Exception {
Objects.requireNonNull(props);
systemPropertiesParser = new PropertiesParser(props);
String active = systemPropertiesParser.getStringProperty(ConfigProperties.ACTIVE, "dev");
DataSourceConfig.active = "prod".equals(active);
String[] sourceTags = systemPropertiesParser.getStringArrayProperty(ConfigProperties.PROP_SOURCE_TAG);
Objects.requireNonNull(sourceTags, "sourceTag is blank");
if (sourceTags.length < 1) {
throw new IllegalArgumentException("sourceTag is blank");
}
String[] configPaths = systemPropertiesParser.getStringArrayProperty(ConfigProperties.PROP_CONFIG_PATH);
Objects.requireNonNull(configPaths, "configPath is blank");
if (configPaths.length < 1) {
throw new IllegalArgumentException("configPath is blank");
}
dataSource(sourceTags, configPaths);
//
ModifyUser.initModify(systemPropertiesParser.getPropertyGroup(ConfigProperties.PROP_LAST_MODIFY));
//
ModifyUser.initCreate(systemPropertiesParser.getPropertyGroup(ConfigProperties.PROP_CREATE));
//
SystemColumn.init(systemPropertiesParser.getPropertyGroup(ConfigProperties.PROP_SYSTEM_COLUMN));
}
/**
* 指定文件初始化
*
* @param propertyPath 路径
* @throws Exception e
*/
public static void init(String propertyPath) throws Exception {
if (StringUtil.isEmpty(propertyPath)) {
throw new IllegalArgumentException("propertyPath is null ");
}
InputStream inputStream = ResourceUtil.getResource(propertyPath);
Properties props = new Properties();
props.load(inputStream);
init(props);
}
private static void dataSource(String[] sourceTags, String[] configPaths) throws Exception {
DbLog.getInstance().info("初始化连接数据库");
if (configPaths.length == 1) {
Map concurrentHashMap = initConfigPath(sourceTags, configPaths[0]);
DatabaseContextHolder.init(concurrentHashMap, configPaths[0]);
} else {
List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy