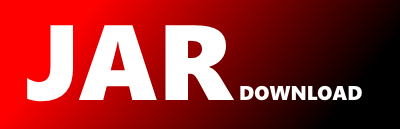
cn.jiangzeyin.database.run.write.Remove Maven / Gradle / Ivy
package cn.jiangzeyin.database.run.write;
import cn.jiangzeyin.database.DbWriteService;
import cn.jiangzeyin.database.base.WriteBase;
import cn.jiangzeyin.database.config.DatabaseContextHolder;
import cn.jiangzeyin.database.config.SystemColumn;
import cn.jiangzeyin.database.util.SqlUtil;
import cn.jiangzeyin.system.DBExecutorService;
import cn.jiangzeyin.system.DbLog;
import com.alibaba.druid.util.JdbcUtils;
import javax.sql.DataSource;
import java.sql.Connection;
import java.util.*;
/**
* 移除数据 即更改isDelete 状态
*
* @author jiangzeyin
*/
public class Remove extends WriteBase {
public enum Type {
/**
* 物理删除
*/
delete,
/**
* 撤销清除
*/
recovery,
/**
* 清除
*/
remove
}
private String ids;
private String where;
private List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy