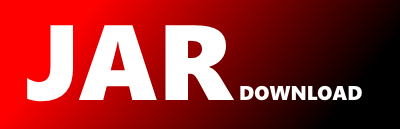
com.avos.avoscloud.Messages Maven / Gradle / Ivy
The newest version!
// Generated by the protocol buffer compiler. DO NOT EDIT!
// source: messages.proto
package com.avos.avoscloud;
public final class Messages {
private Messages() {}
public static void registerAllExtensions(
com.google.protobuf.ExtensionRegistryLite registry) {
}
public static void registerAllExtensions(
com.google.protobuf.ExtensionRegistry registry) {
registerAllExtensions(
(com.google.protobuf.ExtensionRegistryLite) registry);
}
/**
* Protobuf enum {@code com.avos.avoscloud.CommandType}
*/
public enum CommandType
implements com.google.protobuf.ProtocolMessageEnum {
/**
* session = 0;
*/
session(0),
/**
* conv = 1;
*/
conv(1),
/**
* direct = 2;
*/
direct(2),
/**
* ack = 3;
*/
ack(3),
/**
* rcp = 4;
*/
rcp(4),
/**
* unread = 5;
*/
unread(5),
/**
* logs = 6;
*/
logs(6),
/**
* error = 7;
*/
error(7),
/**
* login = 8;
*/
login(8),
/**
* data = 9;
*/
data(9),
/**
* room = 10;
*/
room(10),
/**
* read = 11;
*/
read(11),
/**
* presence = 12;
*/
presence(12),
/**
* report = 13;
*/
report(13),
/**
* echo = 14;
*/
echo(14),
/**
* loggedin = 15;
*/
loggedin(15),
/**
* logout = 16;
*/
logout(16),
/**
* loggedout = 17;
*/
loggedout(17),
/**
* patch = 18;
*/
patch(18),
/**
* pubsub = 19;
*/
pubsub(19),
/**
* blacklist = 20;
*/
blacklist(20),
/**
* goaway = 21;
*/
goaway(21),
;
/**
* session = 0;
*/
public static final int session_VALUE = 0;
/**
* conv = 1;
*/
public static final int conv_VALUE = 1;
/**
* direct = 2;
*/
public static final int direct_VALUE = 2;
/**
* ack = 3;
*/
public static final int ack_VALUE = 3;
/**
* rcp = 4;
*/
public static final int rcp_VALUE = 4;
/**
* unread = 5;
*/
public static final int unread_VALUE = 5;
/**
* logs = 6;
*/
public static final int logs_VALUE = 6;
/**
* error = 7;
*/
public static final int error_VALUE = 7;
/**
* login = 8;
*/
public static final int login_VALUE = 8;
/**
* data = 9;
*/
public static final int data_VALUE = 9;
/**
* room = 10;
*/
public static final int room_VALUE = 10;
/**
* read = 11;
*/
public static final int read_VALUE = 11;
/**
* presence = 12;
*/
public static final int presence_VALUE = 12;
/**
* report = 13;
*/
public static final int report_VALUE = 13;
/**
* echo = 14;
*/
public static final int echo_VALUE = 14;
/**
* loggedin = 15;
*/
public static final int loggedin_VALUE = 15;
/**
* logout = 16;
*/
public static final int logout_VALUE = 16;
/**
* loggedout = 17;
*/
public static final int loggedout_VALUE = 17;
/**
* patch = 18;
*/
public static final int patch_VALUE = 18;
/**
* pubsub = 19;
*/
public static final int pubsub_VALUE = 19;
/**
* blacklist = 20;
*/
public static final int blacklist_VALUE = 20;
/**
* goaway = 21;
*/
public static final int goaway_VALUE = 21;
public final int getNumber() {
return value;
}
/**
* @deprecated Use {@link #forNumber(int)} instead.
*/
@java.lang.Deprecated
public static CommandType valueOf(int value) {
return forNumber(value);
}
public static CommandType forNumber(int value) {
switch (value) {
case 0: return session;
case 1: return conv;
case 2: return direct;
case 3: return ack;
case 4: return rcp;
case 5: return unread;
case 6: return logs;
case 7: return error;
case 8: return login;
case 9: return data;
case 10: return room;
case 11: return read;
case 12: return presence;
case 13: return report;
case 14: return echo;
case 15: return loggedin;
case 16: return logout;
case 17: return loggedout;
case 18: return patch;
case 19: return pubsub;
case 20: return blacklist;
case 21: return goaway;
default: return null;
}
}
public static com.google.protobuf.Internal.EnumLiteMap
internalGetValueMap() {
return internalValueMap;
}
private static final com.google.protobuf.Internal.EnumLiteMap<
CommandType> internalValueMap =
new com.google.protobuf.Internal.EnumLiteMap() {
public CommandType findValueByNumber(int number) {
return CommandType.forNumber(number);
}
};
public final com.google.protobuf.Descriptors.EnumValueDescriptor
getValueDescriptor() {
return getDescriptor().getValues().get(ordinal());
}
public final com.google.protobuf.Descriptors.EnumDescriptor
getDescriptorForType() {
return getDescriptor();
}
public static final com.google.protobuf.Descriptors.EnumDescriptor
getDescriptor() {
return com.avos.avoscloud.Messages.getDescriptor().getEnumTypes().get(0);
}
private static final CommandType[] VALUES = values();
public static CommandType valueOf(
com.google.protobuf.Descriptors.EnumValueDescriptor desc) {
if (desc.getType() != getDescriptor()) {
throw new java.lang.IllegalArgumentException(
"EnumValueDescriptor is not for this type.");
}
return VALUES[desc.getIndex()];
}
private final int value;
private CommandType(int value) {
this.value = value;
}
// @@protoc_insertion_point(enum_scope:com.avos.avoscloud.CommandType)
}
/**
* Protobuf enum {@code com.avos.avoscloud.OpType}
*/
public enum OpType
implements com.google.protobuf.ProtocolMessageEnum {
/**
*
* session
*
*
* open = 1;
*/
open(1),
/**
* add = 2;
*/
add(2),
/**
* remove = 3;
*/
remove(3),
/**
* close = 4;
*/
close(4),
/**
* opened = 5;
*/
opened(5),
/**
* closed = 6;
*/
closed(6),
/**
* query = 7;
*/
query(7),
/**
* query_result = 8;
*/
query_result(8),
/**
* conflict = 9;
*/
conflict(9),
/**
* added = 10;
*/
added(10),
/**
* removed = 11;
*/
removed(11),
/**
* refresh = 12;
*/
refresh(12),
/**
* refreshed = 13;
*/
refreshed(13),
/**
*
* conv
*
*
* start = 30;
*/
start(30),
/**
* started = 31;
*/
started(31),
/**
* joined = 32;
*/
joined(32),
/**
* members_joined = 33;
*/
members_joined(33),
/**
*
* add = 34; reuse session.add
* added = 35; reuse session.added
* remove = 37; reuse session.remove
* removed = 38; reuse session.removed
*
*
* left = 39;
*/
left(39),
/**
* members_left = 40;
*/
members_left(40),
/**
*
* query = 41; reuse session.query
*
*
* results = 42;
*/
results(42),
/**
* count = 43;
*/
count(43),
/**
* result = 44;
*/
result(44),
/**
* update = 45;
*/
update(45),
/**
* updated = 46;
*/
updated(46),
/**
* mute = 47;
*/
mute(47),
/**
* unmute = 48;
*/
unmute(48),
/**
* status = 49;
*/
status(49),
/**
* members = 50;
*/
members(50),
/**
* max_read = 51;
*/
max_read(51),
/**
* is_member = 52;
*/
is_member(52),
/**
* member_info_update = 53;
*/
member_info_update(53),
/**
* member_info_updated = 54;
*/
member_info_updated(54),
/**
* member_info_changed = 55;
*/
member_info_changed(55),
/**
*
* room
*
*
* join = 80;
*/
join(80),
/**
* invite = 81;
*/
invite(81),
/**
* leave = 82;
*/
leave(82),
/**
* kick = 83;
*/
kick(83),
/**
* reject = 84;
*/
reject(84),
/**
* invited = 85;
*/
invited(85),
/**
*
* joined = 32; reuse the value in conv section
* left = 39; reuse the value in conv section
*
*
* kicked = 86;
*/
kicked(86),
/**
*
* report
*
*
* upload = 100;
*/
upload(100),
/**
* uploaded = 101;
*/
uploaded(101),
/**
*
* pubsub
*
*
* subscribe = 120;
*/
subscribe(120),
/**
* subscribed = 121;
*/
subscribed(121),
/**
* unsubscribe = 122;
*/
unsubscribe(122),
/**
* unsubscribed = 123;
*/
unsubscribed(123),
/**
* is_subscribed = 124;
*/
is_subscribed(124),
/**
*
* patch
*
*
* modify = 150;
*/
modify(150),
/**
* modified = 151;
*/
modified(151),
/**
*
* blacklist, query, query_result defined with 7, 8
*
*
* block = 170;
*/
block(170),
/**
* unblock = 171;
*/
unblock(171),
/**
* blocked = 172;
*/
blocked(172),
/**
* unblocked = 173;
*/
unblocked(173),
/**
* members_blocked = 174;
*/
members_blocked(174),
/**
* members_unblocked = 175;
*/
members_unblocked(175),
/**
* check_block = 176;
*/
check_block(176),
/**
* check_result = 177;
*/
check_result(177),
/**
* add_shutup = 180;
*/
add_shutup(180),
/**
* remove_shutup = 181;
*/
remove_shutup(181),
/**
* query_shutup = 182;
*/
query_shutup(182),
/**
* shutup_added = 183;
*/
shutup_added(183),
/**
* shutup_removed = 184;
*/
shutup_removed(184),
/**
* shutup_result = 185;
*/
shutup_result(185),
/**
* shutuped = 186;
*/
shutuped(186),
/**
* unshutuped = 187;
*/
unshutuped(187),
/**
* members_shutuped = 188;
*/
members_shutuped(188),
/**
* members_unshutuped = 189;
*/
members_unshutuped(189),
/**
*
* check_result define in 177
*
*
* check_shutup = 190;
*/
check_shutup(190),
;
/**
*
* session
*
*
* open = 1;
*/
public static final int open_VALUE = 1;
/**
* add = 2;
*/
public static final int add_VALUE = 2;
/**
* remove = 3;
*/
public static final int remove_VALUE = 3;
/**
* close = 4;
*/
public static final int close_VALUE = 4;
/**
* opened = 5;
*/
public static final int opened_VALUE = 5;
/**
* closed = 6;
*/
public static final int closed_VALUE = 6;
/**
* query = 7;
*/
public static final int query_VALUE = 7;
/**
* query_result = 8;
*/
public static final int query_result_VALUE = 8;
/**
* conflict = 9;
*/
public static final int conflict_VALUE = 9;
/**
* added = 10;
*/
public static final int added_VALUE = 10;
/**
* removed = 11;
*/
public static final int removed_VALUE = 11;
/**
* refresh = 12;
*/
public static final int refresh_VALUE = 12;
/**
* refreshed = 13;
*/
public static final int refreshed_VALUE = 13;
/**
*
* conv
*
*
* start = 30;
*/
public static final int start_VALUE = 30;
/**
* started = 31;
*/
public static final int started_VALUE = 31;
/**
* joined = 32;
*/
public static final int joined_VALUE = 32;
/**
* members_joined = 33;
*/
public static final int members_joined_VALUE = 33;
/**
*
* add = 34; reuse session.add
* added = 35; reuse session.added
* remove = 37; reuse session.remove
* removed = 38; reuse session.removed
*
*
* left = 39;
*/
public static final int left_VALUE = 39;
/**
* members_left = 40;
*/
public static final int members_left_VALUE = 40;
/**
*
* query = 41; reuse session.query
*
*
* results = 42;
*/
public static final int results_VALUE = 42;
/**
* count = 43;
*/
public static final int count_VALUE = 43;
/**
* result = 44;
*/
public static final int result_VALUE = 44;
/**
* update = 45;
*/
public static final int update_VALUE = 45;
/**
* updated = 46;
*/
public static final int updated_VALUE = 46;
/**
* mute = 47;
*/
public static final int mute_VALUE = 47;
/**
* unmute = 48;
*/
public static final int unmute_VALUE = 48;
/**
* status = 49;
*/
public static final int status_VALUE = 49;
/**
* members = 50;
*/
public static final int members_VALUE = 50;
/**
* max_read = 51;
*/
public static final int max_read_VALUE = 51;
/**
* is_member = 52;
*/
public static final int is_member_VALUE = 52;
/**
* member_info_update = 53;
*/
public static final int member_info_update_VALUE = 53;
/**
* member_info_updated = 54;
*/
public static final int member_info_updated_VALUE = 54;
/**
* member_info_changed = 55;
*/
public static final int member_info_changed_VALUE = 55;
/**
*
* room
*
*
* join = 80;
*/
public static final int join_VALUE = 80;
/**
* invite = 81;
*/
public static final int invite_VALUE = 81;
/**
* leave = 82;
*/
public static final int leave_VALUE = 82;
/**
* kick = 83;
*/
public static final int kick_VALUE = 83;
/**
* reject = 84;
*/
public static final int reject_VALUE = 84;
/**
* invited = 85;
*/
public static final int invited_VALUE = 85;
/**
*
* joined = 32; reuse the value in conv section
* left = 39; reuse the value in conv section
*
*
* kicked = 86;
*/
public static final int kicked_VALUE = 86;
/**
*
* report
*
*
* upload = 100;
*/
public static final int upload_VALUE = 100;
/**
* uploaded = 101;
*/
public static final int uploaded_VALUE = 101;
/**
*
* pubsub
*
*
* subscribe = 120;
*/
public static final int subscribe_VALUE = 120;
/**
* subscribed = 121;
*/
public static final int subscribed_VALUE = 121;
/**
* unsubscribe = 122;
*/
public static final int unsubscribe_VALUE = 122;
/**
* unsubscribed = 123;
*/
public static final int unsubscribed_VALUE = 123;
/**
* is_subscribed = 124;
*/
public static final int is_subscribed_VALUE = 124;
/**
*
* patch
*
*
* modify = 150;
*/
public static final int modify_VALUE = 150;
/**
* modified = 151;
*/
public static final int modified_VALUE = 151;
/**
*
* blacklist, query, query_result defined with 7, 8
*
*
* block = 170;
*/
public static final int block_VALUE = 170;
/**
* unblock = 171;
*/
public static final int unblock_VALUE = 171;
/**
* blocked = 172;
*/
public static final int blocked_VALUE = 172;
/**
* unblocked = 173;
*/
public static final int unblocked_VALUE = 173;
/**
* members_blocked = 174;
*/
public static final int members_blocked_VALUE = 174;
/**
* members_unblocked = 175;
*/
public static final int members_unblocked_VALUE = 175;
/**
* check_block = 176;
*/
public static final int check_block_VALUE = 176;
/**
* check_result = 177;
*/
public static final int check_result_VALUE = 177;
/**
* add_shutup = 180;
*/
public static final int add_shutup_VALUE = 180;
/**
* remove_shutup = 181;
*/
public static final int remove_shutup_VALUE = 181;
/**
* query_shutup = 182;
*/
public static final int query_shutup_VALUE = 182;
/**
* shutup_added = 183;
*/
public static final int shutup_added_VALUE = 183;
/**
* shutup_removed = 184;
*/
public static final int shutup_removed_VALUE = 184;
/**
* shutup_result = 185;
*/
public static final int shutup_result_VALUE = 185;
/**
* shutuped = 186;
*/
public static final int shutuped_VALUE = 186;
/**
* unshutuped = 187;
*/
public static final int unshutuped_VALUE = 187;
/**
* members_shutuped = 188;
*/
public static final int members_shutuped_VALUE = 188;
/**
* members_unshutuped = 189;
*/
public static final int members_unshutuped_VALUE = 189;
/**
*
* check_result define in 177
*
*
* check_shutup = 190;
*/
public static final int check_shutup_VALUE = 190;
public final int getNumber() {
return value;
}
/**
* @deprecated Use {@link #forNumber(int)} instead.
*/
@java.lang.Deprecated
public static OpType valueOf(int value) {
return forNumber(value);
}
public static OpType forNumber(int value) {
switch (value) {
case 1: return open;
case 2: return add;
case 3: return remove;
case 4: return close;
case 5: return opened;
case 6: return closed;
case 7: return query;
case 8: return query_result;
case 9: return conflict;
case 10: return added;
case 11: return removed;
case 12: return refresh;
case 13: return refreshed;
case 30: return start;
case 31: return started;
case 32: return joined;
case 33: return members_joined;
case 39: return left;
case 40: return members_left;
case 42: return results;
case 43: return count;
case 44: return result;
case 45: return update;
case 46: return updated;
case 47: return mute;
case 48: return unmute;
case 49: return status;
case 50: return members;
case 51: return max_read;
case 52: return is_member;
case 53: return member_info_update;
case 54: return member_info_updated;
case 55: return member_info_changed;
case 80: return join;
case 81: return invite;
case 82: return leave;
case 83: return kick;
case 84: return reject;
case 85: return invited;
case 86: return kicked;
case 100: return upload;
case 101: return uploaded;
case 120: return subscribe;
case 121: return subscribed;
case 122: return unsubscribe;
case 123: return unsubscribed;
case 124: return is_subscribed;
case 150: return modify;
case 151: return modified;
case 170: return block;
case 171: return unblock;
case 172: return blocked;
case 173: return unblocked;
case 174: return members_blocked;
case 175: return members_unblocked;
case 176: return check_block;
case 177: return check_result;
case 180: return add_shutup;
case 181: return remove_shutup;
case 182: return query_shutup;
case 183: return shutup_added;
case 184: return shutup_removed;
case 185: return shutup_result;
case 186: return shutuped;
case 187: return unshutuped;
case 188: return members_shutuped;
case 189: return members_unshutuped;
case 190: return check_shutup;
default: return null;
}
}
public static com.google.protobuf.Internal.EnumLiteMap
internalGetValueMap() {
return internalValueMap;
}
private static final com.google.protobuf.Internal.EnumLiteMap<
OpType> internalValueMap =
new com.google.protobuf.Internal.EnumLiteMap() {
public OpType findValueByNumber(int number) {
return OpType.forNumber(number);
}
};
public final com.google.protobuf.Descriptors.EnumValueDescriptor
getValueDescriptor() {
return getDescriptor().getValues().get(ordinal());
}
public final com.google.protobuf.Descriptors.EnumDescriptor
getDescriptorForType() {
return getDescriptor();
}
public static final com.google.protobuf.Descriptors.EnumDescriptor
getDescriptor() {
return com.avos.avoscloud.Messages.getDescriptor().getEnumTypes().get(1);
}
private static final OpType[] VALUES = values();
public static OpType valueOf(
com.google.protobuf.Descriptors.EnumValueDescriptor desc) {
if (desc.getType() != getDescriptor()) {
throw new java.lang.IllegalArgumentException(
"EnumValueDescriptor is not for this type.");
}
return VALUES[desc.getIndex()];
}
private final int value;
private OpType(int value) {
this.value = value;
}
// @@protoc_insertion_point(enum_scope:com.avos.avoscloud.OpType)
}
/**
* Protobuf enum {@code com.avos.avoscloud.StatusType}
*/
public enum StatusType
implements com.google.protobuf.ProtocolMessageEnum {
/**
* on = 1;
*/
on(1),
/**
* off = 2;
*/
off(2),
;
/**
* on = 1;
*/
public static final int on_VALUE = 1;
/**
* off = 2;
*/
public static final int off_VALUE = 2;
public final int getNumber() {
return value;
}
/**
* @deprecated Use {@link #forNumber(int)} instead.
*/
@java.lang.Deprecated
public static StatusType valueOf(int value) {
return forNumber(value);
}
public static StatusType forNumber(int value) {
switch (value) {
case 1: return on;
case 2: return off;
default: return null;
}
}
public static com.google.protobuf.Internal.EnumLiteMap
internalGetValueMap() {
return internalValueMap;
}
private static final com.google.protobuf.Internal.EnumLiteMap<
StatusType> internalValueMap =
new com.google.protobuf.Internal.EnumLiteMap() {
public StatusType findValueByNumber(int number) {
return StatusType.forNumber(number);
}
};
public final com.google.protobuf.Descriptors.EnumValueDescriptor
getValueDescriptor() {
return getDescriptor().getValues().get(ordinal());
}
public final com.google.protobuf.Descriptors.EnumDescriptor
getDescriptorForType() {
return getDescriptor();
}
public static final com.google.protobuf.Descriptors.EnumDescriptor
getDescriptor() {
return com.avos.avoscloud.Messages.getDescriptor().getEnumTypes().get(2);
}
private static final StatusType[] VALUES = values();
public static StatusType valueOf(
com.google.protobuf.Descriptors.EnumValueDescriptor desc) {
if (desc.getType() != getDescriptor()) {
throw new java.lang.IllegalArgumentException(
"EnumValueDescriptor is not for this type.");
}
return VALUES[desc.getIndex()];
}
private final int value;
private StatusType(int value) {
this.value = value;
}
// @@protoc_insertion_point(enum_scope:com.avos.avoscloud.StatusType)
}
public interface JsonObjectMessageOrBuilder extends
// @@protoc_insertion_point(interface_extends:com.avos.avoscloud.JsonObjectMessage)
com.google.protobuf.MessageOrBuilder {
/**
* required string data = 1;
*/
boolean hasData();
/**
* required string data = 1;
*/
java.lang.String getData();
/**
* required string data = 1;
*/
com.google.protobuf.ByteString
getDataBytes();
}
/**
* Protobuf type {@code com.avos.avoscloud.JsonObjectMessage}
*/
public static final class JsonObjectMessage extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:com.avos.avoscloud.JsonObjectMessage)
JsonObjectMessageOrBuilder {
private static final long serialVersionUID = 0L;
// Use JsonObjectMessage.newBuilder() to construct.
private JsonObjectMessage(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private JsonObjectMessage() {
data_ = "";
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private JsonObjectMessage(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!parseUnknownField(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
case 10: {
com.google.protobuf.ByteString bs = input.readBytes();
bitField0_ |= 0x00000001;
data_ = bs;
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.avos.avoscloud.Messages.internal_static_com_avos_avoscloud_JsonObjectMessage_descriptor;
}
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.avos.avoscloud.Messages.internal_static_com_avos_avoscloud_JsonObjectMessage_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.avos.avoscloud.Messages.JsonObjectMessage.class, com.avos.avoscloud.Messages.JsonObjectMessage.Builder.class);
}
private int bitField0_;
public static final int DATA_FIELD_NUMBER = 1;
private volatile java.lang.Object data_;
/**
* required string data = 1;
*/
public boolean hasData() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
* required string data = 1;
*/
public java.lang.String getData() {
java.lang.Object ref = data_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
data_ = s;
}
return s;
}
}
/**
* required string data = 1;
*/
public com.google.protobuf.ByteString
getDataBytes() {
java.lang.Object ref = data_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
data_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
private byte memoizedIsInitialized = -1;
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
if (!hasData()) {
memoizedIsInitialized = 0;
return false;
}
memoizedIsInitialized = 1;
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (((bitField0_ & 0x00000001) == 0x00000001)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 1, data_);
}
unknownFields.writeTo(output);
}
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (((bitField0_ & 0x00000001) == 0x00000001)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(1, data_);
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof com.avos.avoscloud.Messages.JsonObjectMessage)) {
return super.equals(obj);
}
com.avos.avoscloud.Messages.JsonObjectMessage other = (com.avos.avoscloud.Messages.JsonObjectMessage) obj;
boolean result = true;
result = result && (hasData() == other.hasData());
if (hasData()) {
result = result && getData()
.equals(other.getData());
}
result = result && unknownFields.equals(other.unknownFields);
return result;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (hasData()) {
hash = (37 * hash) + DATA_FIELD_NUMBER;
hash = (53 * hash) + getData().hashCode();
}
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static com.avos.avoscloud.Messages.JsonObjectMessage parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.avos.avoscloud.Messages.JsonObjectMessage parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.avos.avoscloud.Messages.JsonObjectMessage parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.avos.avoscloud.Messages.JsonObjectMessage parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.avos.avoscloud.Messages.JsonObjectMessage parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.avos.avoscloud.Messages.JsonObjectMessage parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.avos.avoscloud.Messages.JsonObjectMessage parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.avos.avoscloud.Messages.JsonObjectMessage parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static com.avos.avoscloud.Messages.JsonObjectMessage parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static com.avos.avoscloud.Messages.JsonObjectMessage parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static com.avos.avoscloud.Messages.JsonObjectMessage parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.avos.avoscloud.Messages.JsonObjectMessage parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(com.avos.avoscloud.Messages.JsonObjectMessage prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code com.avos.avoscloud.JsonObjectMessage}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:com.avos.avoscloud.JsonObjectMessage)
com.avos.avoscloud.Messages.JsonObjectMessageOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.avos.avoscloud.Messages.internal_static_com_avos_avoscloud_JsonObjectMessage_descriptor;
}
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.avos.avoscloud.Messages.internal_static_com_avos_avoscloud_JsonObjectMessage_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.avos.avoscloud.Messages.JsonObjectMessage.class, com.avos.avoscloud.Messages.JsonObjectMessage.Builder.class);
}
// Construct using com.avos.avoscloud.Messages.JsonObjectMessage.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
public Builder clear() {
super.clear();
data_ = "";
bitField0_ = (bitField0_ & ~0x00000001);
return this;
}
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return com.avos.avoscloud.Messages.internal_static_com_avos_avoscloud_JsonObjectMessage_descriptor;
}
public com.avos.avoscloud.Messages.JsonObjectMessage getDefaultInstanceForType() {
return com.avos.avoscloud.Messages.JsonObjectMessage.getDefaultInstance();
}
public com.avos.avoscloud.Messages.JsonObjectMessage build() {
com.avos.avoscloud.Messages.JsonObjectMessage result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
public com.avos.avoscloud.Messages.JsonObjectMessage buildPartial() {
com.avos.avoscloud.Messages.JsonObjectMessage result = new com.avos.avoscloud.Messages.JsonObjectMessage(this);
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (((from_bitField0_ & 0x00000001) == 0x00000001)) {
to_bitField0_ |= 0x00000001;
}
result.data_ = data_;
result.bitField0_ = to_bitField0_;
onBuilt();
return result;
}
public Builder clone() {
return (Builder) super.clone();
}
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.setField(field, value);
}
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return (Builder) super.clearField(field);
}
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return (Builder) super.clearOneof(oneof);
}
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return (Builder) super.setRepeatedField(field, index, value);
}
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.addRepeatedField(field, value);
}
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.avos.avoscloud.Messages.JsonObjectMessage) {
return mergeFrom((com.avos.avoscloud.Messages.JsonObjectMessage)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.avos.avoscloud.Messages.JsonObjectMessage other) {
if (other == com.avos.avoscloud.Messages.JsonObjectMessage.getDefaultInstance()) return this;
if (other.hasData()) {
bitField0_ |= 0x00000001;
data_ = other.data_;
onChanged();
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
public final boolean isInitialized() {
if (!hasData()) {
return false;
}
return true;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
com.avos.avoscloud.Messages.JsonObjectMessage parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (com.avos.avoscloud.Messages.JsonObjectMessage) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
private java.lang.Object data_ = "";
/**
* required string data = 1;
*/
public boolean hasData() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
* required string data = 1;
*/
public java.lang.String getData() {
java.lang.Object ref = data_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
data_ = s;
}
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* required string data = 1;
*/
public com.google.protobuf.ByteString
getDataBytes() {
java.lang.Object ref = data_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
data_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* required string data = 1;
*/
public Builder setData(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000001;
data_ = value;
onChanged();
return this;
}
/**
* required string data = 1;
*/
public Builder clearData() {
bitField0_ = (bitField0_ & ~0x00000001);
data_ = getDefaultInstance().getData();
onChanged();
return this;
}
/**
* required string data = 1;
*/
public Builder setDataBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000001;
data_ = value;
onChanged();
return this;
}
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:com.avos.avoscloud.JsonObjectMessage)
}
// @@protoc_insertion_point(class_scope:com.avos.avoscloud.JsonObjectMessage)
private static final com.avos.avoscloud.Messages.JsonObjectMessage DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new com.avos.avoscloud.Messages.JsonObjectMessage();
}
public static com.avos.avoscloud.Messages.JsonObjectMessage getDefaultInstance() {
return DEFAULT_INSTANCE;
}
@java.lang.Deprecated public static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
public JsonObjectMessage parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new JsonObjectMessage(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
public com.avos.avoscloud.Messages.JsonObjectMessage getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface UnreadTupleOrBuilder extends
// @@protoc_insertion_point(interface_extends:com.avos.avoscloud.UnreadTuple)
com.google.protobuf.MessageOrBuilder {
/**
* required string cid = 1;
*/
boolean hasCid();
/**
* required string cid = 1;
*/
java.lang.String getCid();
/**
* required string cid = 1;
*/
com.google.protobuf.ByteString
getCidBytes();
/**
* required int32 unread = 2;
*/
boolean hasUnread();
/**
* required int32 unread = 2;
*/
int getUnread();
/**
* optional string mid = 3;
*/
boolean hasMid();
/**
* optional string mid = 3;
*/
java.lang.String getMid();
/**
* optional string mid = 3;
*/
com.google.protobuf.ByteString
getMidBytes();
/**
* optional int64 timestamp = 4;
*/
boolean hasTimestamp();
/**
* optional int64 timestamp = 4;
*/
long getTimestamp();
/**
* optional string from = 5;
*/
boolean hasFrom();
/**
* optional string from = 5;
*/
java.lang.String getFrom();
/**
* optional string from = 5;
*/
com.google.protobuf.ByteString
getFromBytes();
/**
* optional string data = 6;
*/
boolean hasData();
/**
* optional string data = 6;
*/
java.lang.String getData();
/**
* optional string data = 6;
*/
com.google.protobuf.ByteString
getDataBytes();
/**
* optional int64 patchTimestamp = 7;
*/
boolean hasPatchTimestamp();
/**
* optional int64 patchTimestamp = 7;
*/
long getPatchTimestamp();
/**
* optional bool mentioned = 8;
*/
boolean hasMentioned();
/**
* optional bool mentioned = 8;
*/
boolean getMentioned();
/**
* optional bytes binaryMsg = 9;
*/
boolean hasBinaryMsg();
/**
* optional bytes binaryMsg = 9;
*/
com.google.protobuf.ByteString getBinaryMsg();
/**
* optional int32 convType = 10;
*/
boolean hasConvType();
/**
* optional int32 convType = 10;
*/
int getConvType();
}
/**
* Protobuf type {@code com.avos.avoscloud.UnreadTuple}
*/
public static final class UnreadTuple extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:com.avos.avoscloud.UnreadTuple)
UnreadTupleOrBuilder {
private static final long serialVersionUID = 0L;
// Use UnreadTuple.newBuilder() to construct.
private UnreadTuple(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private UnreadTuple() {
cid_ = "";
unread_ = 0;
mid_ = "";
timestamp_ = 0L;
from_ = "";
data_ = "";
patchTimestamp_ = 0L;
mentioned_ = false;
binaryMsg_ = com.google.protobuf.ByteString.EMPTY;
convType_ = 0;
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private UnreadTuple(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!parseUnknownField(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
case 10: {
com.google.protobuf.ByteString bs = input.readBytes();
bitField0_ |= 0x00000001;
cid_ = bs;
break;
}
case 16: {
bitField0_ |= 0x00000002;
unread_ = input.readInt32();
break;
}
case 26: {
com.google.protobuf.ByteString bs = input.readBytes();
bitField0_ |= 0x00000004;
mid_ = bs;
break;
}
case 32: {
bitField0_ |= 0x00000008;
timestamp_ = input.readInt64();
break;
}
case 42: {
com.google.protobuf.ByteString bs = input.readBytes();
bitField0_ |= 0x00000010;
from_ = bs;
break;
}
case 50: {
com.google.protobuf.ByteString bs = input.readBytes();
bitField0_ |= 0x00000020;
data_ = bs;
break;
}
case 56: {
bitField0_ |= 0x00000040;
patchTimestamp_ = input.readInt64();
break;
}
case 64: {
bitField0_ |= 0x00000080;
mentioned_ = input.readBool();
break;
}
case 74: {
bitField0_ |= 0x00000100;
binaryMsg_ = input.readBytes();
break;
}
case 80: {
bitField0_ |= 0x00000200;
convType_ = input.readInt32();
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.avos.avoscloud.Messages.internal_static_com_avos_avoscloud_UnreadTuple_descriptor;
}
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.avos.avoscloud.Messages.internal_static_com_avos_avoscloud_UnreadTuple_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.avos.avoscloud.Messages.UnreadTuple.class, com.avos.avoscloud.Messages.UnreadTuple.Builder.class);
}
private int bitField0_;
public static final int CID_FIELD_NUMBER = 1;
private volatile java.lang.Object cid_;
/**
* required string cid = 1;
*/
public boolean hasCid() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
* required string cid = 1;
*/
public java.lang.String getCid() {
java.lang.Object ref = cid_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
cid_ = s;
}
return s;
}
}
/**
* required string cid = 1;
*/
public com.google.protobuf.ByteString
getCidBytes() {
java.lang.Object ref = cid_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
cid_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int UNREAD_FIELD_NUMBER = 2;
private int unread_;
/**
* required int32 unread = 2;
*/
public boolean hasUnread() {
return ((bitField0_ & 0x00000002) == 0x00000002);
}
/**
* required int32 unread = 2;
*/
public int getUnread() {
return unread_;
}
public static final int MID_FIELD_NUMBER = 3;
private volatile java.lang.Object mid_;
/**
* optional string mid = 3;
*/
public boolean hasMid() {
return ((bitField0_ & 0x00000004) == 0x00000004);
}
/**
* optional string mid = 3;
*/
public java.lang.String getMid() {
java.lang.Object ref = mid_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
mid_ = s;
}
return s;
}
}
/**
* optional string mid = 3;
*/
public com.google.protobuf.ByteString
getMidBytes() {
java.lang.Object ref = mid_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
mid_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int TIMESTAMP_FIELD_NUMBER = 4;
private long timestamp_;
/**
* optional int64 timestamp = 4;
*/
public boolean hasTimestamp() {
return ((bitField0_ & 0x00000008) == 0x00000008);
}
/**
* optional int64 timestamp = 4;
*/
public long getTimestamp() {
return timestamp_;
}
public static final int FROM_FIELD_NUMBER = 5;
private volatile java.lang.Object from_;
/**
* optional string from = 5;
*/
public boolean hasFrom() {
return ((bitField0_ & 0x00000010) == 0x00000010);
}
/**
* optional string from = 5;
*/
public java.lang.String getFrom() {
java.lang.Object ref = from_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
from_ = s;
}
return s;
}
}
/**
* optional string from = 5;
*/
public com.google.protobuf.ByteString
getFromBytes() {
java.lang.Object ref = from_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
from_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int DATA_FIELD_NUMBER = 6;
private volatile java.lang.Object data_;
/**
* optional string data = 6;
*/
public boolean hasData() {
return ((bitField0_ & 0x00000020) == 0x00000020);
}
/**
* optional string data = 6;
*/
public java.lang.String getData() {
java.lang.Object ref = data_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
data_ = s;
}
return s;
}
}
/**
* optional string data = 6;
*/
public com.google.protobuf.ByteString
getDataBytes() {
java.lang.Object ref = data_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
data_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int PATCHTIMESTAMP_FIELD_NUMBER = 7;
private long patchTimestamp_;
/**
* optional int64 patchTimestamp = 7;
*/
public boolean hasPatchTimestamp() {
return ((bitField0_ & 0x00000040) == 0x00000040);
}
/**
* optional int64 patchTimestamp = 7;
*/
public long getPatchTimestamp() {
return patchTimestamp_;
}
public static final int MENTIONED_FIELD_NUMBER = 8;
private boolean mentioned_;
/**
* optional bool mentioned = 8;
*/
public boolean hasMentioned() {
return ((bitField0_ & 0x00000080) == 0x00000080);
}
/**
* optional bool mentioned = 8;
*/
public boolean getMentioned() {
return mentioned_;
}
public static final int BINARYMSG_FIELD_NUMBER = 9;
private com.google.protobuf.ByteString binaryMsg_;
/**
* optional bytes binaryMsg = 9;
*/
public boolean hasBinaryMsg() {
return ((bitField0_ & 0x00000100) == 0x00000100);
}
/**
* optional bytes binaryMsg = 9;
*/
public com.google.protobuf.ByteString getBinaryMsg() {
return binaryMsg_;
}
public static final int CONVTYPE_FIELD_NUMBER = 10;
private int convType_;
/**
* optional int32 convType = 10;
*/
public boolean hasConvType() {
return ((bitField0_ & 0x00000200) == 0x00000200);
}
/**
* optional int32 convType = 10;
*/
public int getConvType() {
return convType_;
}
private byte memoizedIsInitialized = -1;
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
if (!hasCid()) {
memoizedIsInitialized = 0;
return false;
}
if (!hasUnread()) {
memoizedIsInitialized = 0;
return false;
}
memoizedIsInitialized = 1;
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (((bitField0_ & 0x00000001) == 0x00000001)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 1, cid_);
}
if (((bitField0_ & 0x00000002) == 0x00000002)) {
output.writeInt32(2, unread_);
}
if (((bitField0_ & 0x00000004) == 0x00000004)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 3, mid_);
}
if (((bitField0_ & 0x00000008) == 0x00000008)) {
output.writeInt64(4, timestamp_);
}
if (((bitField0_ & 0x00000010) == 0x00000010)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 5, from_);
}
if (((bitField0_ & 0x00000020) == 0x00000020)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 6, data_);
}
if (((bitField0_ & 0x00000040) == 0x00000040)) {
output.writeInt64(7, patchTimestamp_);
}
if (((bitField0_ & 0x00000080) == 0x00000080)) {
output.writeBool(8, mentioned_);
}
if (((bitField0_ & 0x00000100) == 0x00000100)) {
output.writeBytes(9, binaryMsg_);
}
if (((bitField0_ & 0x00000200) == 0x00000200)) {
output.writeInt32(10, convType_);
}
unknownFields.writeTo(output);
}
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (((bitField0_ & 0x00000001) == 0x00000001)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(1, cid_);
}
if (((bitField0_ & 0x00000002) == 0x00000002)) {
size += com.google.protobuf.CodedOutputStream
.computeInt32Size(2, unread_);
}
if (((bitField0_ & 0x00000004) == 0x00000004)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(3, mid_);
}
if (((bitField0_ & 0x00000008) == 0x00000008)) {
size += com.google.protobuf.CodedOutputStream
.computeInt64Size(4, timestamp_);
}
if (((bitField0_ & 0x00000010) == 0x00000010)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(5, from_);
}
if (((bitField0_ & 0x00000020) == 0x00000020)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(6, data_);
}
if (((bitField0_ & 0x00000040) == 0x00000040)) {
size += com.google.protobuf.CodedOutputStream
.computeInt64Size(7, patchTimestamp_);
}
if (((bitField0_ & 0x00000080) == 0x00000080)) {
size += com.google.protobuf.CodedOutputStream
.computeBoolSize(8, mentioned_);
}
if (((bitField0_ & 0x00000100) == 0x00000100)) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(9, binaryMsg_);
}
if (((bitField0_ & 0x00000200) == 0x00000200)) {
size += com.google.protobuf.CodedOutputStream
.computeInt32Size(10, convType_);
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof com.avos.avoscloud.Messages.UnreadTuple)) {
return super.equals(obj);
}
com.avos.avoscloud.Messages.UnreadTuple other = (com.avos.avoscloud.Messages.UnreadTuple) obj;
boolean result = true;
result = result && (hasCid() == other.hasCid());
if (hasCid()) {
result = result && getCid()
.equals(other.getCid());
}
result = result && (hasUnread() == other.hasUnread());
if (hasUnread()) {
result = result && (getUnread()
== other.getUnread());
}
result = result && (hasMid() == other.hasMid());
if (hasMid()) {
result = result && getMid()
.equals(other.getMid());
}
result = result && (hasTimestamp() == other.hasTimestamp());
if (hasTimestamp()) {
result = result && (getTimestamp()
== other.getTimestamp());
}
result = result && (hasFrom() == other.hasFrom());
if (hasFrom()) {
result = result && getFrom()
.equals(other.getFrom());
}
result = result && (hasData() == other.hasData());
if (hasData()) {
result = result && getData()
.equals(other.getData());
}
result = result && (hasPatchTimestamp() == other.hasPatchTimestamp());
if (hasPatchTimestamp()) {
result = result && (getPatchTimestamp()
== other.getPatchTimestamp());
}
result = result && (hasMentioned() == other.hasMentioned());
if (hasMentioned()) {
result = result && (getMentioned()
== other.getMentioned());
}
result = result && (hasBinaryMsg() == other.hasBinaryMsg());
if (hasBinaryMsg()) {
result = result && getBinaryMsg()
.equals(other.getBinaryMsg());
}
result = result && (hasConvType() == other.hasConvType());
if (hasConvType()) {
result = result && (getConvType()
== other.getConvType());
}
result = result && unknownFields.equals(other.unknownFields);
return result;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (hasCid()) {
hash = (37 * hash) + CID_FIELD_NUMBER;
hash = (53 * hash) + getCid().hashCode();
}
if (hasUnread()) {
hash = (37 * hash) + UNREAD_FIELD_NUMBER;
hash = (53 * hash) + getUnread();
}
if (hasMid()) {
hash = (37 * hash) + MID_FIELD_NUMBER;
hash = (53 * hash) + getMid().hashCode();
}
if (hasTimestamp()) {
hash = (37 * hash) + TIMESTAMP_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getTimestamp());
}
if (hasFrom()) {
hash = (37 * hash) + FROM_FIELD_NUMBER;
hash = (53 * hash) + getFrom().hashCode();
}
if (hasData()) {
hash = (37 * hash) + DATA_FIELD_NUMBER;
hash = (53 * hash) + getData().hashCode();
}
if (hasPatchTimestamp()) {
hash = (37 * hash) + PATCHTIMESTAMP_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getPatchTimestamp());
}
if (hasMentioned()) {
hash = (37 * hash) + MENTIONED_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashBoolean(
getMentioned());
}
if (hasBinaryMsg()) {
hash = (37 * hash) + BINARYMSG_FIELD_NUMBER;
hash = (53 * hash) + getBinaryMsg().hashCode();
}
if (hasConvType()) {
hash = (37 * hash) + CONVTYPE_FIELD_NUMBER;
hash = (53 * hash) + getConvType();
}
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static com.avos.avoscloud.Messages.UnreadTuple parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.avos.avoscloud.Messages.UnreadTuple parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.avos.avoscloud.Messages.UnreadTuple parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.avos.avoscloud.Messages.UnreadTuple parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.avos.avoscloud.Messages.UnreadTuple parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.avos.avoscloud.Messages.UnreadTuple parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.avos.avoscloud.Messages.UnreadTuple parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.avos.avoscloud.Messages.UnreadTuple parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static com.avos.avoscloud.Messages.UnreadTuple parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static com.avos.avoscloud.Messages.UnreadTuple parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static com.avos.avoscloud.Messages.UnreadTuple parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.avos.avoscloud.Messages.UnreadTuple parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(com.avos.avoscloud.Messages.UnreadTuple prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code com.avos.avoscloud.UnreadTuple}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:com.avos.avoscloud.UnreadTuple)
com.avos.avoscloud.Messages.UnreadTupleOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.avos.avoscloud.Messages.internal_static_com_avos_avoscloud_UnreadTuple_descriptor;
}
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.avos.avoscloud.Messages.internal_static_com_avos_avoscloud_UnreadTuple_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.avos.avoscloud.Messages.UnreadTuple.class, com.avos.avoscloud.Messages.UnreadTuple.Builder.class);
}
// Construct using com.avos.avoscloud.Messages.UnreadTuple.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
public Builder clear() {
super.clear();
cid_ = "";
bitField0_ = (bitField0_ & ~0x00000001);
unread_ = 0;
bitField0_ = (bitField0_ & ~0x00000002);
mid_ = "";
bitField0_ = (bitField0_ & ~0x00000004);
timestamp_ = 0L;
bitField0_ = (bitField0_ & ~0x00000008);
from_ = "";
bitField0_ = (bitField0_ & ~0x00000010);
data_ = "";
bitField0_ = (bitField0_ & ~0x00000020);
patchTimestamp_ = 0L;
bitField0_ = (bitField0_ & ~0x00000040);
mentioned_ = false;
bitField0_ = (bitField0_ & ~0x00000080);
binaryMsg_ = com.google.protobuf.ByteString.EMPTY;
bitField0_ = (bitField0_ & ~0x00000100);
convType_ = 0;
bitField0_ = (bitField0_ & ~0x00000200);
return this;
}
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return com.avos.avoscloud.Messages.internal_static_com_avos_avoscloud_UnreadTuple_descriptor;
}
public com.avos.avoscloud.Messages.UnreadTuple getDefaultInstanceForType() {
return com.avos.avoscloud.Messages.UnreadTuple.getDefaultInstance();
}
public com.avos.avoscloud.Messages.UnreadTuple build() {
com.avos.avoscloud.Messages.UnreadTuple result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
public com.avos.avoscloud.Messages.UnreadTuple buildPartial() {
com.avos.avoscloud.Messages.UnreadTuple result = new com.avos.avoscloud.Messages.UnreadTuple(this);
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (((from_bitField0_ & 0x00000001) == 0x00000001)) {
to_bitField0_ |= 0x00000001;
}
result.cid_ = cid_;
if (((from_bitField0_ & 0x00000002) == 0x00000002)) {
to_bitField0_ |= 0x00000002;
}
result.unread_ = unread_;
if (((from_bitField0_ & 0x00000004) == 0x00000004)) {
to_bitField0_ |= 0x00000004;
}
result.mid_ = mid_;
if (((from_bitField0_ & 0x00000008) == 0x00000008)) {
to_bitField0_ |= 0x00000008;
}
result.timestamp_ = timestamp_;
if (((from_bitField0_ & 0x00000010) == 0x00000010)) {
to_bitField0_ |= 0x00000010;
}
result.from_ = from_;
if (((from_bitField0_ & 0x00000020) == 0x00000020)) {
to_bitField0_ |= 0x00000020;
}
result.data_ = data_;
if (((from_bitField0_ & 0x00000040) == 0x00000040)) {
to_bitField0_ |= 0x00000040;
}
result.patchTimestamp_ = patchTimestamp_;
if (((from_bitField0_ & 0x00000080) == 0x00000080)) {
to_bitField0_ |= 0x00000080;
}
result.mentioned_ = mentioned_;
if (((from_bitField0_ & 0x00000100) == 0x00000100)) {
to_bitField0_ |= 0x00000100;
}
result.binaryMsg_ = binaryMsg_;
if (((from_bitField0_ & 0x00000200) == 0x00000200)) {
to_bitField0_ |= 0x00000200;
}
result.convType_ = convType_;
result.bitField0_ = to_bitField0_;
onBuilt();
return result;
}
public Builder clone() {
return (Builder) super.clone();
}
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.setField(field, value);
}
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return (Builder) super.clearField(field);
}
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return (Builder) super.clearOneof(oneof);
}
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return (Builder) super.setRepeatedField(field, index, value);
}
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.addRepeatedField(field, value);
}
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.avos.avoscloud.Messages.UnreadTuple) {
return mergeFrom((com.avos.avoscloud.Messages.UnreadTuple)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.avos.avoscloud.Messages.UnreadTuple other) {
if (other == com.avos.avoscloud.Messages.UnreadTuple.getDefaultInstance()) return this;
if (other.hasCid()) {
bitField0_ |= 0x00000001;
cid_ = other.cid_;
onChanged();
}
if (other.hasUnread()) {
setUnread(other.getUnread());
}
if (other.hasMid()) {
bitField0_ |= 0x00000004;
mid_ = other.mid_;
onChanged();
}
if (other.hasTimestamp()) {
setTimestamp(other.getTimestamp());
}
if (other.hasFrom()) {
bitField0_ |= 0x00000010;
from_ = other.from_;
onChanged();
}
if (other.hasData()) {
bitField0_ |= 0x00000020;
data_ = other.data_;
onChanged();
}
if (other.hasPatchTimestamp()) {
setPatchTimestamp(other.getPatchTimestamp());
}
if (other.hasMentioned()) {
setMentioned(other.getMentioned());
}
if (other.hasBinaryMsg()) {
setBinaryMsg(other.getBinaryMsg());
}
if (other.hasConvType()) {
setConvType(other.getConvType());
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
public final boolean isInitialized() {
if (!hasCid()) {
return false;
}
if (!hasUnread()) {
return false;
}
return true;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
com.avos.avoscloud.Messages.UnreadTuple parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (com.avos.avoscloud.Messages.UnreadTuple) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
private java.lang.Object cid_ = "";
/**
* required string cid = 1;
*/
public boolean hasCid() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
* required string cid = 1;
*/
public java.lang.String getCid() {
java.lang.Object ref = cid_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
cid_ = s;
}
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* required string cid = 1;
*/
public com.google.protobuf.ByteString
getCidBytes() {
java.lang.Object ref = cid_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
cid_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* required string cid = 1;
*/
public Builder setCid(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000001;
cid_ = value;
onChanged();
return this;
}
/**
* required string cid = 1;
*/
public Builder clearCid() {
bitField0_ = (bitField0_ & ~0x00000001);
cid_ = getDefaultInstance().getCid();
onChanged();
return this;
}
/**
* required string cid = 1;
*/
public Builder setCidBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000001;
cid_ = value;
onChanged();
return this;
}
private int unread_ ;
/**
* required int32 unread = 2;
*/
public boolean hasUnread() {
return ((bitField0_ & 0x00000002) == 0x00000002);
}
/**
* required int32 unread = 2;
*/
public int getUnread() {
return unread_;
}
/**
* required int32 unread = 2;
*/
public Builder setUnread(int value) {
bitField0_ |= 0x00000002;
unread_ = value;
onChanged();
return this;
}
/**
* required int32 unread = 2;
*/
public Builder clearUnread() {
bitField0_ = (bitField0_ & ~0x00000002);
unread_ = 0;
onChanged();
return this;
}
private java.lang.Object mid_ = "";
/**
* optional string mid = 3;
*/
public boolean hasMid() {
return ((bitField0_ & 0x00000004) == 0x00000004);
}
/**
* optional string mid = 3;
*/
public java.lang.String getMid() {
java.lang.Object ref = mid_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
mid_ = s;
}
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* optional string mid = 3;
*/
public com.google.protobuf.ByteString
getMidBytes() {
java.lang.Object ref = mid_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
mid_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* optional string mid = 3;
*/
public Builder setMid(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000004;
mid_ = value;
onChanged();
return this;
}
/**
* optional string mid = 3;
*/
public Builder clearMid() {
bitField0_ = (bitField0_ & ~0x00000004);
mid_ = getDefaultInstance().getMid();
onChanged();
return this;
}
/**
* optional string mid = 3;
*/
public Builder setMidBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000004;
mid_ = value;
onChanged();
return this;
}
private long timestamp_ ;
/**
* optional int64 timestamp = 4;
*/
public boolean hasTimestamp() {
return ((bitField0_ & 0x00000008) == 0x00000008);
}
/**
* optional int64 timestamp = 4;
*/
public long getTimestamp() {
return timestamp_;
}
/**
* optional int64 timestamp = 4;
*/
public Builder setTimestamp(long value) {
bitField0_ |= 0x00000008;
timestamp_ = value;
onChanged();
return this;
}
/**
* optional int64 timestamp = 4;
*/
public Builder clearTimestamp() {
bitField0_ = (bitField0_ & ~0x00000008);
timestamp_ = 0L;
onChanged();
return this;
}
private java.lang.Object from_ = "";
/**
* optional string from = 5;
*/
public boolean hasFrom() {
return ((bitField0_ & 0x00000010) == 0x00000010);
}
/**
* optional string from = 5;
*/
public java.lang.String getFrom() {
java.lang.Object ref = from_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
from_ = s;
}
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* optional string from = 5;
*/
public com.google.protobuf.ByteString
getFromBytes() {
java.lang.Object ref = from_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
from_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* optional string from = 5;
*/
public Builder setFrom(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000010;
from_ = value;
onChanged();
return this;
}
/**
* optional string from = 5;
*/
public Builder clearFrom() {
bitField0_ = (bitField0_ & ~0x00000010);
from_ = getDefaultInstance().getFrom();
onChanged();
return this;
}
/**
* optional string from = 5;
*/
public Builder setFromBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000010;
from_ = value;
onChanged();
return this;
}
private java.lang.Object data_ = "";
/**
* optional string data = 6;
*/
public boolean hasData() {
return ((bitField0_ & 0x00000020) == 0x00000020);
}
/**
* optional string data = 6;
*/
public java.lang.String getData() {
java.lang.Object ref = data_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
data_ = s;
}
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* optional string data = 6;
*/
public com.google.protobuf.ByteString
getDataBytes() {
java.lang.Object ref = data_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
data_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* optional string data = 6;
*/
public Builder setData(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000020;
data_ = value;
onChanged();
return this;
}
/**
* optional string data = 6;
*/
public Builder clearData() {
bitField0_ = (bitField0_ & ~0x00000020);
data_ = getDefaultInstance().getData();
onChanged();
return this;
}
/**
* optional string data = 6;
*/
public Builder setDataBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000020;
data_ = value;
onChanged();
return this;
}
private long patchTimestamp_ ;
/**
* optional int64 patchTimestamp = 7;
*/
public boolean hasPatchTimestamp() {
return ((bitField0_ & 0x00000040) == 0x00000040);
}
/**
* optional int64 patchTimestamp = 7;
*/
public long getPatchTimestamp() {
return patchTimestamp_;
}
/**
* optional int64 patchTimestamp = 7;
*/
public Builder setPatchTimestamp(long value) {
bitField0_ |= 0x00000040;
patchTimestamp_ = value;
onChanged();
return this;
}
/**
* optional int64 patchTimestamp = 7;
*/
public Builder clearPatchTimestamp() {
bitField0_ = (bitField0_ & ~0x00000040);
patchTimestamp_ = 0L;
onChanged();
return this;
}
private boolean mentioned_ ;
/**
* optional bool mentioned = 8;
*/
public boolean hasMentioned() {
return ((bitField0_ & 0x00000080) == 0x00000080);
}
/**
* optional bool mentioned = 8;
*/
public boolean getMentioned() {
return mentioned_;
}
/**
* optional bool mentioned = 8;
*/
public Builder setMentioned(boolean value) {
bitField0_ |= 0x00000080;
mentioned_ = value;
onChanged();
return this;
}
/**
* optional bool mentioned = 8;
*/
public Builder clearMentioned() {
bitField0_ = (bitField0_ & ~0x00000080);
mentioned_ = false;
onChanged();
return this;
}
private com.google.protobuf.ByteString binaryMsg_ = com.google.protobuf.ByteString.EMPTY;
/**
* optional bytes binaryMsg = 9;
*/
public boolean hasBinaryMsg() {
return ((bitField0_ & 0x00000100) == 0x00000100);
}
/**
* optional bytes binaryMsg = 9;
*/
public com.google.protobuf.ByteString getBinaryMsg() {
return binaryMsg_;
}
/**
* optional bytes binaryMsg = 9;
*/
public Builder setBinaryMsg(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000100;
binaryMsg_ = value;
onChanged();
return this;
}
/**
* optional bytes binaryMsg = 9;
*/
public Builder clearBinaryMsg() {
bitField0_ = (bitField0_ & ~0x00000100);
binaryMsg_ = getDefaultInstance().getBinaryMsg();
onChanged();
return this;
}
private int convType_ ;
/**
* optional int32 convType = 10;
*/
public boolean hasConvType() {
return ((bitField0_ & 0x00000200) == 0x00000200);
}
/**
* optional int32 convType = 10;
*/
public int getConvType() {
return convType_;
}
/**
* optional int32 convType = 10;
*/
public Builder setConvType(int value) {
bitField0_ |= 0x00000200;
convType_ = value;
onChanged();
return this;
}
/**
* optional int32 convType = 10;
*/
public Builder clearConvType() {
bitField0_ = (bitField0_ & ~0x00000200);
convType_ = 0;
onChanged();
return this;
}
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:com.avos.avoscloud.UnreadTuple)
}
// @@protoc_insertion_point(class_scope:com.avos.avoscloud.UnreadTuple)
private static final com.avos.avoscloud.Messages.UnreadTuple DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new com.avos.avoscloud.Messages.UnreadTuple();
}
public static com.avos.avoscloud.Messages.UnreadTuple getDefaultInstance() {
return DEFAULT_INSTANCE;
}
@java.lang.Deprecated public static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
public UnreadTuple parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new UnreadTuple(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
public com.avos.avoscloud.Messages.UnreadTuple getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface LogItemOrBuilder extends
// @@protoc_insertion_point(interface_extends:com.avos.avoscloud.LogItem)
com.google.protobuf.MessageOrBuilder {
/**
* optional string from = 1;
*/
boolean hasFrom();
/**
* optional string from = 1;
*/
java.lang.String getFrom();
/**
* optional string from = 1;
*/
com.google.protobuf.ByteString
getFromBytes();
/**
* optional string data = 2;
*/
boolean hasData();
/**
* optional string data = 2;
*/
java.lang.String getData();
/**
* optional string data = 2;
*/
com.google.protobuf.ByteString
getDataBytes();
/**
* optional int64 timestamp = 3;
*/
boolean hasTimestamp();
/**
* optional int64 timestamp = 3;
*/
long getTimestamp();
/**
* optional string msgId = 4;
*/
boolean hasMsgId();
/**
* optional string msgId = 4;
*/
java.lang.String getMsgId();
/**
* optional string msgId = 4;
*/
com.google.protobuf.ByteString
getMsgIdBytes();
/**
* optional int64 ackAt = 5;
*/
boolean hasAckAt();
/**
* optional int64 ackAt = 5;
*/
long getAckAt();
/**
* optional int64 readAt = 6;
*/
boolean hasReadAt();
/**
* optional int64 readAt = 6;
*/
long getReadAt();
/**
* optional int64 patchTimestamp = 7;
*/
boolean hasPatchTimestamp();
/**
* optional int64 patchTimestamp = 7;
*/
long getPatchTimestamp();
/**
* optional bool mentionAll = 8;
*/
boolean hasMentionAll();
/**
* optional bool mentionAll = 8;
*/
boolean getMentionAll();
/**
* repeated string mentionPids = 9;
*/
java.util.List
getMentionPidsList();
/**
* repeated string mentionPids = 9;
*/
int getMentionPidsCount();
/**
* repeated string mentionPids = 9;
*/
java.lang.String getMentionPids(int index);
/**
* repeated string mentionPids = 9;
*/
com.google.protobuf.ByteString
getMentionPidsBytes(int index);
/**
* optional bool bin = 10;
*/
boolean hasBin();
/**
* optional bool bin = 10;
*/
boolean getBin();
/**
* optional int32 convType = 11;
*/
boolean hasConvType();
/**
* optional int32 convType = 11;
*/
int getConvType();
}
/**
* Protobuf type {@code com.avos.avoscloud.LogItem}
*/
public static final class LogItem extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:com.avos.avoscloud.LogItem)
LogItemOrBuilder {
private static final long serialVersionUID = 0L;
// Use LogItem.newBuilder() to construct.
private LogItem(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private LogItem() {
from_ = "";
data_ = "";
timestamp_ = 0L;
msgId_ = "";
ackAt_ = 0L;
readAt_ = 0L;
patchTimestamp_ = 0L;
mentionAll_ = false;
mentionPids_ = com.google.protobuf.LazyStringArrayList.EMPTY;
bin_ = false;
convType_ = 0;
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private LogItem(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!parseUnknownField(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
case 10: {
com.google.protobuf.ByteString bs = input.readBytes();
bitField0_ |= 0x00000001;
from_ = bs;
break;
}
case 18: {
com.google.protobuf.ByteString bs = input.readBytes();
bitField0_ |= 0x00000002;
data_ = bs;
break;
}
case 24: {
bitField0_ |= 0x00000004;
timestamp_ = input.readInt64();
break;
}
case 34: {
com.google.protobuf.ByteString bs = input.readBytes();
bitField0_ |= 0x00000008;
msgId_ = bs;
break;
}
case 40: {
bitField0_ |= 0x00000010;
ackAt_ = input.readInt64();
break;
}
case 48: {
bitField0_ |= 0x00000020;
readAt_ = input.readInt64();
break;
}
case 56: {
bitField0_ |= 0x00000040;
patchTimestamp_ = input.readInt64();
break;
}
case 64: {
bitField0_ |= 0x00000080;
mentionAll_ = input.readBool();
break;
}
case 74: {
com.google.protobuf.ByteString bs = input.readBytes();
if (!((mutable_bitField0_ & 0x00000100) == 0x00000100)) {
mentionPids_ = new com.google.protobuf.LazyStringArrayList();
mutable_bitField0_ |= 0x00000100;
}
mentionPids_.add(bs);
break;
}
case 80: {
bitField0_ |= 0x00000100;
bin_ = input.readBool();
break;
}
case 88: {
bitField0_ |= 0x00000200;
convType_ = input.readInt32();
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
if (((mutable_bitField0_ & 0x00000100) == 0x00000100)) {
mentionPids_ = mentionPids_.getUnmodifiableView();
}
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.avos.avoscloud.Messages.internal_static_com_avos_avoscloud_LogItem_descriptor;
}
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.avos.avoscloud.Messages.internal_static_com_avos_avoscloud_LogItem_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.avos.avoscloud.Messages.LogItem.class, com.avos.avoscloud.Messages.LogItem.Builder.class);
}
private int bitField0_;
public static final int FROM_FIELD_NUMBER = 1;
private volatile java.lang.Object from_;
/**
* optional string from = 1;
*/
public boolean hasFrom() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
* optional string from = 1;
*/
public java.lang.String getFrom() {
java.lang.Object ref = from_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
from_ = s;
}
return s;
}
}
/**
* optional string from = 1;
*/
public com.google.protobuf.ByteString
getFromBytes() {
java.lang.Object ref = from_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
from_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int DATA_FIELD_NUMBER = 2;
private volatile java.lang.Object data_;
/**
* optional string data = 2;
*/
public boolean hasData() {
return ((bitField0_ & 0x00000002) == 0x00000002);
}
/**
* optional string data = 2;
*/
public java.lang.String getData() {
java.lang.Object ref = data_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
data_ = s;
}
return s;
}
}
/**
* optional string data = 2;
*/
public com.google.protobuf.ByteString
getDataBytes() {
java.lang.Object ref = data_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
data_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int TIMESTAMP_FIELD_NUMBER = 3;
private long timestamp_;
/**
* optional int64 timestamp = 3;
*/
public boolean hasTimestamp() {
return ((bitField0_ & 0x00000004) == 0x00000004);
}
/**
* optional int64 timestamp = 3;
*/
public long getTimestamp() {
return timestamp_;
}
public static final int MSGID_FIELD_NUMBER = 4;
private volatile java.lang.Object msgId_;
/**
* optional string msgId = 4;
*/
public boolean hasMsgId() {
return ((bitField0_ & 0x00000008) == 0x00000008);
}
/**
* optional string msgId = 4;
*/
public java.lang.String getMsgId() {
java.lang.Object ref = msgId_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
msgId_ = s;
}
return s;
}
}
/**
* optional string msgId = 4;
*/
public com.google.protobuf.ByteString
getMsgIdBytes() {
java.lang.Object ref = msgId_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
msgId_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int ACKAT_FIELD_NUMBER = 5;
private long ackAt_;
/**
* optional int64 ackAt = 5;
*/
public boolean hasAckAt() {
return ((bitField0_ & 0x00000010) == 0x00000010);
}
/**
* optional int64 ackAt = 5;
*/
public long getAckAt() {
return ackAt_;
}
public static final int READAT_FIELD_NUMBER = 6;
private long readAt_;
/**
* optional int64 readAt = 6;
*/
public boolean hasReadAt() {
return ((bitField0_ & 0x00000020) == 0x00000020);
}
/**
* optional int64 readAt = 6;
*/
public long getReadAt() {
return readAt_;
}
public static final int PATCHTIMESTAMP_FIELD_NUMBER = 7;
private long patchTimestamp_;
/**
* optional int64 patchTimestamp = 7;
*/
public boolean hasPatchTimestamp() {
return ((bitField0_ & 0x00000040) == 0x00000040);
}
/**
* optional int64 patchTimestamp = 7;
*/
public long getPatchTimestamp() {
return patchTimestamp_;
}
public static final int MENTIONALL_FIELD_NUMBER = 8;
private boolean mentionAll_;
/**
* optional bool mentionAll = 8;
*/
public boolean hasMentionAll() {
return ((bitField0_ & 0x00000080) == 0x00000080);
}
/**
* optional bool mentionAll = 8;
*/
public boolean getMentionAll() {
return mentionAll_;
}
public static final int MENTIONPIDS_FIELD_NUMBER = 9;
private com.google.protobuf.LazyStringList mentionPids_;
/**
* repeated string mentionPids = 9;
*/
public com.google.protobuf.ProtocolStringList
getMentionPidsList() {
return mentionPids_;
}
/**
* repeated string mentionPids = 9;
*/
public int getMentionPidsCount() {
return mentionPids_.size();
}
/**
* repeated string mentionPids = 9;
*/
public java.lang.String getMentionPids(int index) {
return mentionPids_.get(index);
}
/**
* repeated string mentionPids = 9;
*/
public com.google.protobuf.ByteString
getMentionPidsBytes(int index) {
return mentionPids_.getByteString(index);
}
public static final int BIN_FIELD_NUMBER = 10;
private boolean bin_;
/**
* optional bool bin = 10;
*/
public boolean hasBin() {
return ((bitField0_ & 0x00000100) == 0x00000100);
}
/**
* optional bool bin = 10;
*/
public boolean getBin() {
return bin_;
}
public static final int CONVTYPE_FIELD_NUMBER = 11;
private int convType_;
/**
* optional int32 convType = 11;
*/
public boolean hasConvType() {
return ((bitField0_ & 0x00000200) == 0x00000200);
}
/**
* optional int32 convType = 11;
*/
public int getConvType() {
return convType_;
}
private byte memoizedIsInitialized = -1;
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (((bitField0_ & 0x00000001) == 0x00000001)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 1, from_);
}
if (((bitField0_ & 0x00000002) == 0x00000002)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 2, data_);
}
if (((bitField0_ & 0x00000004) == 0x00000004)) {
output.writeInt64(3, timestamp_);
}
if (((bitField0_ & 0x00000008) == 0x00000008)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 4, msgId_);
}
if (((bitField0_ & 0x00000010) == 0x00000010)) {
output.writeInt64(5, ackAt_);
}
if (((bitField0_ & 0x00000020) == 0x00000020)) {
output.writeInt64(6, readAt_);
}
if (((bitField0_ & 0x00000040) == 0x00000040)) {
output.writeInt64(7, patchTimestamp_);
}
if (((bitField0_ & 0x00000080) == 0x00000080)) {
output.writeBool(8, mentionAll_);
}
for (int i = 0; i < mentionPids_.size(); i++) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 9, mentionPids_.getRaw(i));
}
if (((bitField0_ & 0x00000100) == 0x00000100)) {
output.writeBool(10, bin_);
}
if (((bitField0_ & 0x00000200) == 0x00000200)) {
output.writeInt32(11, convType_);
}
unknownFields.writeTo(output);
}
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (((bitField0_ & 0x00000001) == 0x00000001)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(1, from_);
}
if (((bitField0_ & 0x00000002) == 0x00000002)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(2, data_);
}
if (((bitField0_ & 0x00000004) == 0x00000004)) {
size += com.google.protobuf.CodedOutputStream
.computeInt64Size(3, timestamp_);
}
if (((bitField0_ & 0x00000008) == 0x00000008)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(4, msgId_);
}
if (((bitField0_ & 0x00000010) == 0x00000010)) {
size += com.google.protobuf.CodedOutputStream
.computeInt64Size(5, ackAt_);
}
if (((bitField0_ & 0x00000020) == 0x00000020)) {
size += com.google.protobuf.CodedOutputStream
.computeInt64Size(6, readAt_);
}
if (((bitField0_ & 0x00000040) == 0x00000040)) {
size += com.google.protobuf.CodedOutputStream
.computeInt64Size(7, patchTimestamp_);
}
if (((bitField0_ & 0x00000080) == 0x00000080)) {
size += com.google.protobuf.CodedOutputStream
.computeBoolSize(8, mentionAll_);
}
{
int dataSize = 0;
for (int i = 0; i < mentionPids_.size(); i++) {
dataSize += computeStringSizeNoTag(mentionPids_.getRaw(i));
}
size += dataSize;
size += 1 * getMentionPidsList().size();
}
if (((bitField0_ & 0x00000100) == 0x00000100)) {
size += com.google.protobuf.CodedOutputStream
.computeBoolSize(10, bin_);
}
if (((bitField0_ & 0x00000200) == 0x00000200)) {
size += com.google.protobuf.CodedOutputStream
.computeInt32Size(11, convType_);
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof com.avos.avoscloud.Messages.LogItem)) {
return super.equals(obj);
}
com.avos.avoscloud.Messages.LogItem other = (com.avos.avoscloud.Messages.LogItem) obj;
boolean result = true;
result = result && (hasFrom() == other.hasFrom());
if (hasFrom()) {
result = result && getFrom()
.equals(other.getFrom());
}
result = result && (hasData() == other.hasData());
if (hasData()) {
result = result && getData()
.equals(other.getData());
}
result = result && (hasTimestamp() == other.hasTimestamp());
if (hasTimestamp()) {
result = result && (getTimestamp()
== other.getTimestamp());
}
result = result && (hasMsgId() == other.hasMsgId());
if (hasMsgId()) {
result = result && getMsgId()
.equals(other.getMsgId());
}
result = result && (hasAckAt() == other.hasAckAt());
if (hasAckAt()) {
result = result && (getAckAt()
== other.getAckAt());
}
result = result && (hasReadAt() == other.hasReadAt());
if (hasReadAt()) {
result = result && (getReadAt()
== other.getReadAt());
}
result = result && (hasPatchTimestamp() == other.hasPatchTimestamp());
if (hasPatchTimestamp()) {
result = result && (getPatchTimestamp()
== other.getPatchTimestamp());
}
result = result && (hasMentionAll() == other.hasMentionAll());
if (hasMentionAll()) {
result = result && (getMentionAll()
== other.getMentionAll());
}
result = result && getMentionPidsList()
.equals(other.getMentionPidsList());
result = result && (hasBin() == other.hasBin());
if (hasBin()) {
result = result && (getBin()
== other.getBin());
}
result = result && (hasConvType() == other.hasConvType());
if (hasConvType()) {
result = result && (getConvType()
== other.getConvType());
}
result = result && unknownFields.equals(other.unknownFields);
return result;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (hasFrom()) {
hash = (37 * hash) + FROM_FIELD_NUMBER;
hash = (53 * hash) + getFrom().hashCode();
}
if (hasData()) {
hash = (37 * hash) + DATA_FIELD_NUMBER;
hash = (53 * hash) + getData().hashCode();
}
if (hasTimestamp()) {
hash = (37 * hash) + TIMESTAMP_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getTimestamp());
}
if (hasMsgId()) {
hash = (37 * hash) + MSGID_FIELD_NUMBER;
hash = (53 * hash) + getMsgId().hashCode();
}
if (hasAckAt()) {
hash = (37 * hash) + ACKAT_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getAckAt());
}
if (hasReadAt()) {
hash = (37 * hash) + READAT_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getReadAt());
}
if (hasPatchTimestamp()) {
hash = (37 * hash) + PATCHTIMESTAMP_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getPatchTimestamp());
}
if (hasMentionAll()) {
hash = (37 * hash) + MENTIONALL_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashBoolean(
getMentionAll());
}
if (getMentionPidsCount() > 0) {
hash = (37 * hash) + MENTIONPIDS_FIELD_NUMBER;
hash = (53 * hash) + getMentionPidsList().hashCode();
}
if (hasBin()) {
hash = (37 * hash) + BIN_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashBoolean(
getBin());
}
if (hasConvType()) {
hash = (37 * hash) + CONVTYPE_FIELD_NUMBER;
hash = (53 * hash) + getConvType();
}
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static com.avos.avoscloud.Messages.LogItem parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.avos.avoscloud.Messages.LogItem parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.avos.avoscloud.Messages.LogItem parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.avos.avoscloud.Messages.LogItem parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.avos.avoscloud.Messages.LogItem parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.avos.avoscloud.Messages.LogItem parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.avos.avoscloud.Messages.LogItem parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.avos.avoscloud.Messages.LogItem parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static com.avos.avoscloud.Messages.LogItem parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static com.avos.avoscloud.Messages.LogItem parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static com.avos.avoscloud.Messages.LogItem parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.avos.avoscloud.Messages.LogItem parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(com.avos.avoscloud.Messages.LogItem prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code com.avos.avoscloud.LogItem}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:com.avos.avoscloud.LogItem)
com.avos.avoscloud.Messages.LogItemOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.avos.avoscloud.Messages.internal_static_com_avos_avoscloud_LogItem_descriptor;
}
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.avos.avoscloud.Messages.internal_static_com_avos_avoscloud_LogItem_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.avos.avoscloud.Messages.LogItem.class, com.avos.avoscloud.Messages.LogItem.Builder.class);
}
// Construct using com.avos.avoscloud.Messages.LogItem.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
public Builder clear() {
super.clear();
from_ = "";
bitField0_ = (bitField0_ & ~0x00000001);
data_ = "";
bitField0_ = (bitField0_ & ~0x00000002);
timestamp_ = 0L;
bitField0_ = (bitField0_ & ~0x00000004);
msgId_ = "";
bitField0_ = (bitField0_ & ~0x00000008);
ackAt_ = 0L;
bitField0_ = (bitField0_ & ~0x00000010);
readAt_ = 0L;
bitField0_ = (bitField0_ & ~0x00000020);
patchTimestamp_ = 0L;
bitField0_ = (bitField0_ & ~0x00000040);
mentionAll_ = false;
bitField0_ = (bitField0_ & ~0x00000080);
mentionPids_ = com.google.protobuf.LazyStringArrayList.EMPTY;
bitField0_ = (bitField0_ & ~0x00000100);
bin_ = false;
bitField0_ = (bitField0_ & ~0x00000200);
convType_ = 0;
bitField0_ = (bitField0_ & ~0x00000400);
return this;
}
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return com.avos.avoscloud.Messages.internal_static_com_avos_avoscloud_LogItem_descriptor;
}
public com.avos.avoscloud.Messages.LogItem getDefaultInstanceForType() {
return com.avos.avoscloud.Messages.LogItem.getDefaultInstance();
}
public com.avos.avoscloud.Messages.LogItem build() {
com.avos.avoscloud.Messages.LogItem result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
public com.avos.avoscloud.Messages.LogItem buildPartial() {
com.avos.avoscloud.Messages.LogItem result = new com.avos.avoscloud.Messages.LogItem(this);
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (((from_bitField0_ & 0x00000001) == 0x00000001)) {
to_bitField0_ |= 0x00000001;
}
result.from_ = from_;
if (((from_bitField0_ & 0x00000002) == 0x00000002)) {
to_bitField0_ |= 0x00000002;
}
result.data_ = data_;
if (((from_bitField0_ & 0x00000004) == 0x00000004)) {
to_bitField0_ |= 0x00000004;
}
result.timestamp_ = timestamp_;
if (((from_bitField0_ & 0x00000008) == 0x00000008)) {
to_bitField0_ |= 0x00000008;
}
result.msgId_ = msgId_;
if (((from_bitField0_ & 0x00000010) == 0x00000010)) {
to_bitField0_ |= 0x00000010;
}
result.ackAt_ = ackAt_;
if (((from_bitField0_ & 0x00000020) == 0x00000020)) {
to_bitField0_ |= 0x00000020;
}
result.readAt_ = readAt_;
if (((from_bitField0_ & 0x00000040) == 0x00000040)) {
to_bitField0_ |= 0x00000040;
}
result.patchTimestamp_ = patchTimestamp_;
if (((from_bitField0_ & 0x00000080) == 0x00000080)) {
to_bitField0_ |= 0x00000080;
}
result.mentionAll_ = mentionAll_;
if (((bitField0_ & 0x00000100) == 0x00000100)) {
mentionPids_ = mentionPids_.getUnmodifiableView();
bitField0_ = (bitField0_ & ~0x00000100);
}
result.mentionPids_ = mentionPids_;
if (((from_bitField0_ & 0x00000200) == 0x00000200)) {
to_bitField0_ |= 0x00000100;
}
result.bin_ = bin_;
if (((from_bitField0_ & 0x00000400) == 0x00000400)) {
to_bitField0_ |= 0x00000200;
}
result.convType_ = convType_;
result.bitField0_ = to_bitField0_;
onBuilt();
return result;
}
public Builder clone() {
return (Builder) super.clone();
}
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.setField(field, value);
}
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return (Builder) super.clearField(field);
}
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return (Builder) super.clearOneof(oneof);
}
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return (Builder) super.setRepeatedField(field, index, value);
}
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.addRepeatedField(field, value);
}
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.avos.avoscloud.Messages.LogItem) {
return mergeFrom((com.avos.avoscloud.Messages.LogItem)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.avos.avoscloud.Messages.LogItem other) {
if (other == com.avos.avoscloud.Messages.LogItem.getDefaultInstance()) return this;
if (other.hasFrom()) {
bitField0_ |= 0x00000001;
from_ = other.from_;
onChanged();
}
if (other.hasData()) {
bitField0_ |= 0x00000002;
data_ = other.data_;
onChanged();
}
if (other.hasTimestamp()) {
setTimestamp(other.getTimestamp());
}
if (other.hasMsgId()) {
bitField0_ |= 0x00000008;
msgId_ = other.msgId_;
onChanged();
}
if (other.hasAckAt()) {
setAckAt(other.getAckAt());
}
if (other.hasReadAt()) {
setReadAt(other.getReadAt());
}
if (other.hasPatchTimestamp()) {
setPatchTimestamp(other.getPatchTimestamp());
}
if (other.hasMentionAll()) {
setMentionAll(other.getMentionAll());
}
if (!other.mentionPids_.isEmpty()) {
if (mentionPids_.isEmpty()) {
mentionPids_ = other.mentionPids_;
bitField0_ = (bitField0_ & ~0x00000100);
} else {
ensureMentionPidsIsMutable();
mentionPids_.addAll(other.mentionPids_);
}
onChanged();
}
if (other.hasBin()) {
setBin(other.getBin());
}
if (other.hasConvType()) {
setConvType(other.getConvType());
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
public final boolean isInitialized() {
return true;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
com.avos.avoscloud.Messages.LogItem parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (com.avos.avoscloud.Messages.LogItem) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
private java.lang.Object from_ = "";
/**
* optional string from = 1;
*/
public boolean hasFrom() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
* optional string from = 1;
*/
public java.lang.String getFrom() {
java.lang.Object ref = from_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
from_ = s;
}
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* optional string from = 1;
*/
public com.google.protobuf.ByteString
getFromBytes() {
java.lang.Object ref = from_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
from_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* optional string from = 1;
*/
public Builder setFrom(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000001;
from_ = value;
onChanged();
return this;
}
/**
* optional string from = 1;
*/
public Builder clearFrom() {
bitField0_ = (bitField0_ & ~0x00000001);
from_ = getDefaultInstance().getFrom();
onChanged();
return this;
}
/**
* optional string from = 1;
*/
public Builder setFromBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000001;
from_ = value;
onChanged();
return this;
}
private java.lang.Object data_ = "";
/**
* optional string data = 2;
*/
public boolean hasData() {
return ((bitField0_ & 0x00000002) == 0x00000002);
}
/**
* optional string data = 2;
*/
public java.lang.String getData() {
java.lang.Object ref = data_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
data_ = s;
}
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* optional string data = 2;
*/
public com.google.protobuf.ByteString
getDataBytes() {
java.lang.Object ref = data_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
data_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* optional string data = 2;
*/
public Builder setData(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000002;
data_ = value;
onChanged();
return this;
}
/**
* optional string data = 2;
*/
public Builder clearData() {
bitField0_ = (bitField0_ & ~0x00000002);
data_ = getDefaultInstance().getData();
onChanged();
return this;
}
/**
* optional string data = 2;
*/
public Builder setDataBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000002;
data_ = value;
onChanged();
return this;
}
private long timestamp_ ;
/**
* optional int64 timestamp = 3;
*/
public boolean hasTimestamp() {
return ((bitField0_ & 0x00000004) == 0x00000004);
}
/**
* optional int64 timestamp = 3;
*/
public long getTimestamp() {
return timestamp_;
}
/**
* optional int64 timestamp = 3;
*/
public Builder setTimestamp(long value) {
bitField0_ |= 0x00000004;
timestamp_ = value;
onChanged();
return this;
}
/**
* optional int64 timestamp = 3;
*/
public Builder clearTimestamp() {
bitField0_ = (bitField0_ & ~0x00000004);
timestamp_ = 0L;
onChanged();
return this;
}
private java.lang.Object msgId_ = "";
/**
* optional string msgId = 4;
*/
public boolean hasMsgId() {
return ((bitField0_ & 0x00000008) == 0x00000008);
}
/**
* optional string msgId = 4;
*/
public java.lang.String getMsgId() {
java.lang.Object ref = msgId_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
msgId_ = s;
}
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* optional string msgId = 4;
*/
public com.google.protobuf.ByteString
getMsgIdBytes() {
java.lang.Object ref = msgId_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
msgId_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* optional string msgId = 4;
*/
public Builder setMsgId(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000008;
msgId_ = value;
onChanged();
return this;
}
/**
* optional string msgId = 4;
*/
public Builder clearMsgId() {
bitField0_ = (bitField0_ & ~0x00000008);
msgId_ = getDefaultInstance().getMsgId();
onChanged();
return this;
}
/**
* optional string msgId = 4;
*/
public Builder setMsgIdBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000008;
msgId_ = value;
onChanged();
return this;
}
private long ackAt_ ;
/**
* optional int64 ackAt = 5;
*/
public boolean hasAckAt() {
return ((bitField0_ & 0x00000010) == 0x00000010);
}
/**
* optional int64 ackAt = 5;
*/
public long getAckAt() {
return ackAt_;
}
/**
* optional int64 ackAt = 5;
*/
public Builder setAckAt(long value) {
bitField0_ |= 0x00000010;
ackAt_ = value;
onChanged();
return this;
}
/**
* optional int64 ackAt = 5;
*/
public Builder clearAckAt() {
bitField0_ = (bitField0_ & ~0x00000010);
ackAt_ = 0L;
onChanged();
return this;
}
private long readAt_ ;
/**
* optional int64 readAt = 6;
*/
public boolean hasReadAt() {
return ((bitField0_ & 0x00000020) == 0x00000020);
}
/**
* optional int64 readAt = 6;
*/
public long getReadAt() {
return readAt_;
}
/**
* optional int64 readAt = 6;
*/
public Builder setReadAt(long value) {
bitField0_ |= 0x00000020;
readAt_ = value;
onChanged();
return this;
}
/**
* optional int64 readAt = 6;
*/
public Builder clearReadAt() {
bitField0_ = (bitField0_ & ~0x00000020);
readAt_ = 0L;
onChanged();
return this;
}
private long patchTimestamp_ ;
/**
* optional int64 patchTimestamp = 7;
*/
public boolean hasPatchTimestamp() {
return ((bitField0_ & 0x00000040) == 0x00000040);
}
/**
* optional int64 patchTimestamp = 7;
*/
public long getPatchTimestamp() {
return patchTimestamp_;
}
/**
* optional int64 patchTimestamp = 7;
*/
public Builder setPatchTimestamp(long value) {
bitField0_ |= 0x00000040;
patchTimestamp_ = value;
onChanged();
return this;
}
/**
* optional int64 patchTimestamp = 7;
*/
public Builder clearPatchTimestamp() {
bitField0_ = (bitField0_ & ~0x00000040);
patchTimestamp_ = 0L;
onChanged();
return this;
}
private boolean mentionAll_ ;
/**
* optional bool mentionAll = 8;
*/
public boolean hasMentionAll() {
return ((bitField0_ & 0x00000080) == 0x00000080);
}
/**
* optional bool mentionAll = 8;
*/
public boolean getMentionAll() {
return mentionAll_;
}
/**
* optional bool mentionAll = 8;
*/
public Builder setMentionAll(boolean value) {
bitField0_ |= 0x00000080;
mentionAll_ = value;
onChanged();
return this;
}
/**
* optional bool mentionAll = 8;
*/
public Builder clearMentionAll() {
bitField0_ = (bitField0_ & ~0x00000080);
mentionAll_ = false;
onChanged();
return this;
}
private com.google.protobuf.LazyStringList mentionPids_ = com.google.protobuf.LazyStringArrayList.EMPTY;
private void ensureMentionPidsIsMutable() {
if (!((bitField0_ & 0x00000100) == 0x00000100)) {
mentionPids_ = new com.google.protobuf.LazyStringArrayList(mentionPids_);
bitField0_ |= 0x00000100;
}
}
/**
* repeated string mentionPids = 9;
*/
public com.google.protobuf.ProtocolStringList
getMentionPidsList() {
return mentionPids_.getUnmodifiableView();
}
/**
* repeated string mentionPids = 9;
*/
public int getMentionPidsCount() {
return mentionPids_.size();
}
/**
* repeated string mentionPids = 9;
*/
public java.lang.String getMentionPids(int index) {
return mentionPids_.get(index);
}
/**
* repeated string mentionPids = 9;
*/
public com.google.protobuf.ByteString
getMentionPidsBytes(int index) {
return mentionPids_.getByteString(index);
}
/**
* repeated string mentionPids = 9;
*/
public Builder setMentionPids(
int index, java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
ensureMentionPidsIsMutable();
mentionPids_.set(index, value);
onChanged();
return this;
}
/**
* repeated string mentionPids = 9;
*/
public Builder addMentionPids(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
ensureMentionPidsIsMutable();
mentionPids_.add(value);
onChanged();
return this;
}
/**
* repeated string mentionPids = 9;
*/
public Builder addAllMentionPids(
java.lang.Iterable values) {
ensureMentionPidsIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, mentionPids_);
onChanged();
return this;
}
/**
* repeated string mentionPids = 9;
*/
public Builder clearMentionPids() {
mentionPids_ = com.google.protobuf.LazyStringArrayList.EMPTY;
bitField0_ = (bitField0_ & ~0x00000100);
onChanged();
return this;
}
/**
* repeated string mentionPids = 9;
*/
public Builder addMentionPidsBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
ensureMentionPidsIsMutable();
mentionPids_.add(value);
onChanged();
return this;
}
private boolean bin_ ;
/**
* optional bool bin = 10;
*/
public boolean hasBin() {
return ((bitField0_ & 0x00000200) == 0x00000200);
}
/**
* optional bool bin = 10;
*/
public boolean getBin() {
return bin_;
}
/**
* optional bool bin = 10;
*/
public Builder setBin(boolean value) {
bitField0_ |= 0x00000200;
bin_ = value;
onChanged();
return this;
}
/**
* optional bool bin = 10;
*/
public Builder clearBin() {
bitField0_ = (bitField0_ & ~0x00000200);
bin_ = false;
onChanged();
return this;
}
private int convType_ ;
/**
* optional int32 convType = 11;
*/
public boolean hasConvType() {
return ((bitField0_ & 0x00000400) == 0x00000400);
}
/**
* optional int32 convType = 11;
*/
public int getConvType() {
return convType_;
}
/**
* optional int32 convType = 11;
*/
public Builder setConvType(int value) {
bitField0_ |= 0x00000400;
convType_ = value;
onChanged();
return this;
}
/**
* optional int32 convType = 11;
*/
public Builder clearConvType() {
bitField0_ = (bitField0_ & ~0x00000400);
convType_ = 0;
onChanged();
return this;
}
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:com.avos.avoscloud.LogItem)
}
// @@protoc_insertion_point(class_scope:com.avos.avoscloud.LogItem)
private static final com.avos.avoscloud.Messages.LogItem DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new com.avos.avoscloud.Messages.LogItem();
}
public static com.avos.avoscloud.Messages.LogItem getDefaultInstance() {
return DEFAULT_INSTANCE;
}
@java.lang.Deprecated public static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
public LogItem parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new LogItem(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
public com.avos.avoscloud.Messages.LogItem getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface ConvMemberInfoOrBuilder extends
// @@protoc_insertion_point(interface_extends:com.avos.avoscloud.ConvMemberInfo)
com.google.protobuf.MessageOrBuilder {
/**
* optional string pid = 1;
*/
boolean hasPid();
/**
* optional string pid = 1;
*/
java.lang.String getPid();
/**
* optional string pid = 1;
*/
com.google.protobuf.ByteString
getPidBytes();
/**
* optional string role = 2;
*/
boolean hasRole();
/**
* optional string role = 2;
*/
java.lang.String getRole();
/**
* optional string role = 2;
*/
com.google.protobuf.ByteString
getRoleBytes();
/**
* optional string infoId = 3;
*/
boolean hasInfoId();
/**
* optional string infoId = 3;
*/
java.lang.String getInfoId();
/**
* optional string infoId = 3;
*/
com.google.protobuf.ByteString
getInfoIdBytes();
}
/**
* Protobuf type {@code com.avos.avoscloud.ConvMemberInfo}
*/
public static final class ConvMemberInfo extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:com.avos.avoscloud.ConvMemberInfo)
ConvMemberInfoOrBuilder {
private static final long serialVersionUID = 0L;
// Use ConvMemberInfo.newBuilder() to construct.
private ConvMemberInfo(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private ConvMemberInfo() {
pid_ = "";
role_ = "";
infoId_ = "";
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private ConvMemberInfo(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!parseUnknownField(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
case 10: {
com.google.protobuf.ByteString bs = input.readBytes();
bitField0_ |= 0x00000001;
pid_ = bs;
break;
}
case 18: {
com.google.protobuf.ByteString bs = input.readBytes();
bitField0_ |= 0x00000002;
role_ = bs;
break;
}
case 26: {
com.google.protobuf.ByteString bs = input.readBytes();
bitField0_ |= 0x00000004;
infoId_ = bs;
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.avos.avoscloud.Messages.internal_static_com_avos_avoscloud_ConvMemberInfo_descriptor;
}
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.avos.avoscloud.Messages.internal_static_com_avos_avoscloud_ConvMemberInfo_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.avos.avoscloud.Messages.ConvMemberInfo.class, com.avos.avoscloud.Messages.ConvMemberInfo.Builder.class);
}
private int bitField0_;
public static final int PID_FIELD_NUMBER = 1;
private volatile java.lang.Object pid_;
/**
* optional string pid = 1;
*/
public boolean hasPid() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
* optional string pid = 1;
*/
public java.lang.String getPid() {
java.lang.Object ref = pid_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
pid_ = s;
}
return s;
}
}
/**
* optional string pid = 1;
*/
public com.google.protobuf.ByteString
getPidBytes() {
java.lang.Object ref = pid_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
pid_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int ROLE_FIELD_NUMBER = 2;
private volatile java.lang.Object role_;
/**
* optional string role = 2;
*/
public boolean hasRole() {
return ((bitField0_ & 0x00000002) == 0x00000002);
}
/**
* optional string role = 2;
*/
public java.lang.String getRole() {
java.lang.Object ref = role_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
role_ = s;
}
return s;
}
}
/**
* optional string role = 2;
*/
public com.google.protobuf.ByteString
getRoleBytes() {
java.lang.Object ref = role_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
role_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int INFOID_FIELD_NUMBER = 3;
private volatile java.lang.Object infoId_;
/**
* optional string infoId = 3;
*/
public boolean hasInfoId() {
return ((bitField0_ & 0x00000004) == 0x00000004);
}
/**
* optional string infoId = 3;
*/
public java.lang.String getInfoId() {
java.lang.Object ref = infoId_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
infoId_ = s;
}
return s;
}
}
/**
* optional string infoId = 3;
*/
public com.google.protobuf.ByteString
getInfoIdBytes() {
java.lang.Object ref = infoId_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
infoId_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
private byte memoizedIsInitialized = -1;
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (((bitField0_ & 0x00000001) == 0x00000001)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 1, pid_);
}
if (((bitField0_ & 0x00000002) == 0x00000002)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 2, role_);
}
if (((bitField0_ & 0x00000004) == 0x00000004)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 3, infoId_);
}
unknownFields.writeTo(output);
}
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (((bitField0_ & 0x00000001) == 0x00000001)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(1, pid_);
}
if (((bitField0_ & 0x00000002) == 0x00000002)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(2, role_);
}
if (((bitField0_ & 0x00000004) == 0x00000004)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(3, infoId_);
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof com.avos.avoscloud.Messages.ConvMemberInfo)) {
return super.equals(obj);
}
com.avos.avoscloud.Messages.ConvMemberInfo other = (com.avos.avoscloud.Messages.ConvMemberInfo) obj;
boolean result = true;
result = result && (hasPid() == other.hasPid());
if (hasPid()) {
result = result && getPid()
.equals(other.getPid());
}
result = result && (hasRole() == other.hasRole());
if (hasRole()) {
result = result && getRole()
.equals(other.getRole());
}
result = result && (hasInfoId() == other.hasInfoId());
if (hasInfoId()) {
result = result && getInfoId()
.equals(other.getInfoId());
}
result = result && unknownFields.equals(other.unknownFields);
return result;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (hasPid()) {
hash = (37 * hash) + PID_FIELD_NUMBER;
hash = (53 * hash) + getPid().hashCode();
}
if (hasRole()) {
hash = (37 * hash) + ROLE_FIELD_NUMBER;
hash = (53 * hash) + getRole().hashCode();
}
if (hasInfoId()) {
hash = (37 * hash) + INFOID_FIELD_NUMBER;
hash = (53 * hash) + getInfoId().hashCode();
}
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static com.avos.avoscloud.Messages.ConvMemberInfo parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.avos.avoscloud.Messages.ConvMemberInfo parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.avos.avoscloud.Messages.ConvMemberInfo parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.avos.avoscloud.Messages.ConvMemberInfo parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.avos.avoscloud.Messages.ConvMemberInfo parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.avos.avoscloud.Messages.ConvMemberInfo parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.avos.avoscloud.Messages.ConvMemberInfo parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.avos.avoscloud.Messages.ConvMemberInfo parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static com.avos.avoscloud.Messages.ConvMemberInfo parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static com.avos.avoscloud.Messages.ConvMemberInfo parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static com.avos.avoscloud.Messages.ConvMemberInfo parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.avos.avoscloud.Messages.ConvMemberInfo parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(com.avos.avoscloud.Messages.ConvMemberInfo prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code com.avos.avoscloud.ConvMemberInfo}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:com.avos.avoscloud.ConvMemberInfo)
com.avos.avoscloud.Messages.ConvMemberInfoOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.avos.avoscloud.Messages.internal_static_com_avos_avoscloud_ConvMemberInfo_descriptor;
}
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.avos.avoscloud.Messages.internal_static_com_avos_avoscloud_ConvMemberInfo_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.avos.avoscloud.Messages.ConvMemberInfo.class, com.avos.avoscloud.Messages.ConvMemberInfo.Builder.class);
}
// Construct using com.avos.avoscloud.Messages.ConvMemberInfo.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
public Builder clear() {
super.clear();
pid_ = "";
bitField0_ = (bitField0_ & ~0x00000001);
role_ = "";
bitField0_ = (bitField0_ & ~0x00000002);
infoId_ = "";
bitField0_ = (bitField0_ & ~0x00000004);
return this;
}
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return com.avos.avoscloud.Messages.internal_static_com_avos_avoscloud_ConvMemberInfo_descriptor;
}
public com.avos.avoscloud.Messages.ConvMemberInfo getDefaultInstanceForType() {
return com.avos.avoscloud.Messages.ConvMemberInfo.getDefaultInstance();
}
public com.avos.avoscloud.Messages.ConvMemberInfo build() {
com.avos.avoscloud.Messages.ConvMemberInfo result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
public com.avos.avoscloud.Messages.ConvMemberInfo buildPartial() {
com.avos.avoscloud.Messages.ConvMemberInfo result = new com.avos.avoscloud.Messages.ConvMemberInfo(this);
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (((from_bitField0_ & 0x00000001) == 0x00000001)) {
to_bitField0_ |= 0x00000001;
}
result.pid_ = pid_;
if (((from_bitField0_ & 0x00000002) == 0x00000002)) {
to_bitField0_ |= 0x00000002;
}
result.role_ = role_;
if (((from_bitField0_ & 0x00000004) == 0x00000004)) {
to_bitField0_ |= 0x00000004;
}
result.infoId_ = infoId_;
result.bitField0_ = to_bitField0_;
onBuilt();
return result;
}
public Builder clone() {
return (Builder) super.clone();
}
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.setField(field, value);
}
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return (Builder) super.clearField(field);
}
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return (Builder) super.clearOneof(oneof);
}
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return (Builder) super.setRepeatedField(field, index, value);
}
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.addRepeatedField(field, value);
}
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.avos.avoscloud.Messages.ConvMemberInfo) {
return mergeFrom((com.avos.avoscloud.Messages.ConvMemberInfo)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.avos.avoscloud.Messages.ConvMemberInfo other) {
if (other == com.avos.avoscloud.Messages.ConvMemberInfo.getDefaultInstance()) return this;
if (other.hasPid()) {
bitField0_ |= 0x00000001;
pid_ = other.pid_;
onChanged();
}
if (other.hasRole()) {
bitField0_ |= 0x00000002;
role_ = other.role_;
onChanged();
}
if (other.hasInfoId()) {
bitField0_ |= 0x00000004;
infoId_ = other.infoId_;
onChanged();
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
public final boolean isInitialized() {
return true;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
com.avos.avoscloud.Messages.ConvMemberInfo parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (com.avos.avoscloud.Messages.ConvMemberInfo) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
private java.lang.Object pid_ = "";
/**
* optional string pid = 1;
*/
public boolean hasPid() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
* optional string pid = 1;
*/
public java.lang.String getPid() {
java.lang.Object ref = pid_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
pid_ = s;
}
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* optional string pid = 1;
*/
public com.google.protobuf.ByteString
getPidBytes() {
java.lang.Object ref = pid_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
pid_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* optional string pid = 1;
*/
public Builder setPid(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000001;
pid_ = value;
onChanged();
return this;
}
/**
* optional string pid = 1;
*/
public Builder clearPid() {
bitField0_ = (bitField0_ & ~0x00000001);
pid_ = getDefaultInstance().getPid();
onChanged();
return this;
}
/**
* optional string pid = 1;
*/
public Builder setPidBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000001;
pid_ = value;
onChanged();
return this;
}
private java.lang.Object role_ = "";
/**
* optional string role = 2;
*/
public boolean hasRole() {
return ((bitField0_ & 0x00000002) == 0x00000002);
}
/**
* optional string role = 2;
*/
public java.lang.String getRole() {
java.lang.Object ref = role_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
role_ = s;
}
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* optional string role = 2;
*/
public com.google.protobuf.ByteString
getRoleBytes() {
java.lang.Object ref = role_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
role_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* optional string role = 2;
*/
public Builder setRole(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000002;
role_ = value;
onChanged();
return this;
}
/**
* optional string role = 2;
*/
public Builder clearRole() {
bitField0_ = (bitField0_ & ~0x00000002);
role_ = getDefaultInstance().getRole();
onChanged();
return this;
}
/**
* optional string role = 2;
*/
public Builder setRoleBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000002;
role_ = value;
onChanged();
return this;
}
private java.lang.Object infoId_ = "";
/**
* optional string infoId = 3;
*/
public boolean hasInfoId() {
return ((bitField0_ & 0x00000004) == 0x00000004);
}
/**
* optional string infoId = 3;
*/
public java.lang.String getInfoId() {
java.lang.Object ref = infoId_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
infoId_ = s;
}
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* optional string infoId = 3;
*/
public com.google.protobuf.ByteString
getInfoIdBytes() {
java.lang.Object ref = infoId_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
infoId_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* optional string infoId = 3;
*/
public Builder setInfoId(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000004;
infoId_ = value;
onChanged();
return this;
}
/**
* optional string infoId = 3;
*/
public Builder clearInfoId() {
bitField0_ = (bitField0_ & ~0x00000004);
infoId_ = getDefaultInstance().getInfoId();
onChanged();
return this;
}
/**
* optional string infoId = 3;
*/
public Builder setInfoIdBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000004;
infoId_ = value;
onChanged();
return this;
}
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:com.avos.avoscloud.ConvMemberInfo)
}
// @@protoc_insertion_point(class_scope:com.avos.avoscloud.ConvMemberInfo)
private static final com.avos.avoscloud.Messages.ConvMemberInfo DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new com.avos.avoscloud.Messages.ConvMemberInfo();
}
public static com.avos.avoscloud.Messages.ConvMemberInfo getDefaultInstance() {
return DEFAULT_INSTANCE;
}
@java.lang.Deprecated public static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
public ConvMemberInfo parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new ConvMemberInfo(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
public com.avos.avoscloud.Messages.ConvMemberInfo getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface DataCommandOrBuilder extends
// @@protoc_insertion_point(interface_extends:com.avos.avoscloud.DataCommand)
com.google.protobuf.MessageOrBuilder {
/**
* repeated string ids = 1;
*/
java.util.List
getIdsList();
/**
* repeated string ids = 1;
*/
int getIdsCount();
/**
* repeated string ids = 1;
*/
java.lang.String getIds(int index);
/**
* repeated string ids = 1;
*/
com.google.protobuf.ByteString
getIdsBytes(int index);
/**
* repeated .com.avos.avoscloud.JsonObjectMessage msg = 2;
*/
java.util.List
getMsgList();
/**
* repeated .com.avos.avoscloud.JsonObjectMessage msg = 2;
*/
com.avos.avoscloud.Messages.JsonObjectMessage getMsg(int index);
/**
* repeated .com.avos.avoscloud.JsonObjectMessage msg = 2;
*/
int getMsgCount();
/**
* repeated .com.avos.avoscloud.JsonObjectMessage msg = 2;
*/
java.util.List extends com.avos.avoscloud.Messages.JsonObjectMessageOrBuilder>
getMsgOrBuilderList();
/**
* repeated .com.avos.avoscloud.JsonObjectMessage msg = 2;
*/
com.avos.avoscloud.Messages.JsonObjectMessageOrBuilder getMsgOrBuilder(
int index);
/**
* optional bool offline = 3;
*/
boolean hasOffline();
/**
* optional bool offline = 3;
*/
boolean getOffline();
}
/**
* Protobuf type {@code com.avos.avoscloud.DataCommand}
*/
public static final class DataCommand extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:com.avos.avoscloud.DataCommand)
DataCommandOrBuilder {
private static final long serialVersionUID = 0L;
// Use DataCommand.newBuilder() to construct.
private DataCommand(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private DataCommand() {
ids_ = com.google.protobuf.LazyStringArrayList.EMPTY;
msg_ = java.util.Collections.emptyList();
offline_ = false;
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private DataCommand(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!parseUnknownField(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
case 10: {
com.google.protobuf.ByteString bs = input.readBytes();
if (!((mutable_bitField0_ & 0x00000001) == 0x00000001)) {
ids_ = new com.google.protobuf.LazyStringArrayList();
mutable_bitField0_ |= 0x00000001;
}
ids_.add(bs);
break;
}
case 18: {
if (!((mutable_bitField0_ & 0x00000002) == 0x00000002)) {
msg_ = new java.util.ArrayList();
mutable_bitField0_ |= 0x00000002;
}
msg_.add(
input.readMessage(com.avos.avoscloud.Messages.JsonObjectMessage.PARSER, extensionRegistry));
break;
}
case 24: {
bitField0_ |= 0x00000001;
offline_ = input.readBool();
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
if (((mutable_bitField0_ & 0x00000001) == 0x00000001)) {
ids_ = ids_.getUnmodifiableView();
}
if (((mutable_bitField0_ & 0x00000002) == 0x00000002)) {
msg_ = java.util.Collections.unmodifiableList(msg_);
}
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.avos.avoscloud.Messages.internal_static_com_avos_avoscloud_DataCommand_descriptor;
}
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.avos.avoscloud.Messages.internal_static_com_avos_avoscloud_DataCommand_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.avos.avoscloud.Messages.DataCommand.class, com.avos.avoscloud.Messages.DataCommand.Builder.class);
}
private int bitField0_;
public static final int IDS_FIELD_NUMBER = 1;
private com.google.protobuf.LazyStringList ids_;
/**
* repeated string ids = 1;
*/
public com.google.protobuf.ProtocolStringList
getIdsList() {
return ids_;
}
/**
* repeated string ids = 1;
*/
public int getIdsCount() {
return ids_.size();
}
/**
* repeated string ids = 1;
*/
public java.lang.String getIds(int index) {
return ids_.get(index);
}
/**
* repeated string ids = 1;
*/
public com.google.protobuf.ByteString
getIdsBytes(int index) {
return ids_.getByteString(index);
}
public static final int MSG_FIELD_NUMBER = 2;
private java.util.List msg_;
/**
* repeated .com.avos.avoscloud.JsonObjectMessage msg = 2;
*/
public java.util.List getMsgList() {
return msg_;
}
/**
* repeated .com.avos.avoscloud.JsonObjectMessage msg = 2;
*/
public java.util.List extends com.avos.avoscloud.Messages.JsonObjectMessageOrBuilder>
getMsgOrBuilderList() {
return msg_;
}
/**
* repeated .com.avos.avoscloud.JsonObjectMessage msg = 2;
*/
public int getMsgCount() {
return msg_.size();
}
/**
* repeated .com.avos.avoscloud.JsonObjectMessage msg = 2;
*/
public com.avos.avoscloud.Messages.JsonObjectMessage getMsg(int index) {
return msg_.get(index);
}
/**
* repeated .com.avos.avoscloud.JsonObjectMessage msg = 2;
*/
public com.avos.avoscloud.Messages.JsonObjectMessageOrBuilder getMsgOrBuilder(
int index) {
return msg_.get(index);
}
public static final int OFFLINE_FIELD_NUMBER = 3;
private boolean offline_;
/**
* optional bool offline = 3;
*/
public boolean hasOffline() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
* optional bool offline = 3;
*/
public boolean getOffline() {
return offline_;
}
private byte memoizedIsInitialized = -1;
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
for (int i = 0; i < getMsgCount(); i++) {
if (!getMsg(i).isInitialized()) {
memoizedIsInitialized = 0;
return false;
}
}
memoizedIsInitialized = 1;
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
for (int i = 0; i < ids_.size(); i++) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 1, ids_.getRaw(i));
}
for (int i = 0; i < msg_.size(); i++) {
output.writeMessage(2, msg_.get(i));
}
if (((bitField0_ & 0x00000001) == 0x00000001)) {
output.writeBool(3, offline_);
}
unknownFields.writeTo(output);
}
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
{
int dataSize = 0;
for (int i = 0; i < ids_.size(); i++) {
dataSize += computeStringSizeNoTag(ids_.getRaw(i));
}
size += dataSize;
size += 1 * getIdsList().size();
}
for (int i = 0; i < msg_.size(); i++) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(2, msg_.get(i));
}
if (((bitField0_ & 0x00000001) == 0x00000001)) {
size += com.google.protobuf.CodedOutputStream
.computeBoolSize(3, offline_);
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof com.avos.avoscloud.Messages.DataCommand)) {
return super.equals(obj);
}
com.avos.avoscloud.Messages.DataCommand other = (com.avos.avoscloud.Messages.DataCommand) obj;
boolean result = true;
result = result && getIdsList()
.equals(other.getIdsList());
result = result && getMsgList()
.equals(other.getMsgList());
result = result && (hasOffline() == other.hasOffline());
if (hasOffline()) {
result = result && (getOffline()
== other.getOffline());
}
result = result && unknownFields.equals(other.unknownFields);
return result;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (getIdsCount() > 0) {
hash = (37 * hash) + IDS_FIELD_NUMBER;
hash = (53 * hash) + getIdsList().hashCode();
}
if (getMsgCount() > 0) {
hash = (37 * hash) + MSG_FIELD_NUMBER;
hash = (53 * hash) + getMsgList().hashCode();
}
if (hasOffline()) {
hash = (37 * hash) + OFFLINE_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashBoolean(
getOffline());
}
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static com.avos.avoscloud.Messages.DataCommand parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.avos.avoscloud.Messages.DataCommand parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.avos.avoscloud.Messages.DataCommand parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.avos.avoscloud.Messages.DataCommand parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.avos.avoscloud.Messages.DataCommand parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.avos.avoscloud.Messages.DataCommand parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.avos.avoscloud.Messages.DataCommand parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.avos.avoscloud.Messages.DataCommand parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static com.avos.avoscloud.Messages.DataCommand parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static com.avos.avoscloud.Messages.DataCommand parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static com.avos.avoscloud.Messages.DataCommand parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.avos.avoscloud.Messages.DataCommand parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(com.avos.avoscloud.Messages.DataCommand prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code com.avos.avoscloud.DataCommand}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:com.avos.avoscloud.DataCommand)
com.avos.avoscloud.Messages.DataCommandOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.avos.avoscloud.Messages.internal_static_com_avos_avoscloud_DataCommand_descriptor;
}
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.avos.avoscloud.Messages.internal_static_com_avos_avoscloud_DataCommand_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.avos.avoscloud.Messages.DataCommand.class, com.avos.avoscloud.Messages.DataCommand.Builder.class);
}
// Construct using com.avos.avoscloud.Messages.DataCommand.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
getMsgFieldBuilder();
}
}
public Builder clear() {
super.clear();
ids_ = com.google.protobuf.LazyStringArrayList.EMPTY;
bitField0_ = (bitField0_ & ~0x00000001);
if (msgBuilder_ == null) {
msg_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000002);
} else {
msgBuilder_.clear();
}
offline_ = false;
bitField0_ = (bitField0_ & ~0x00000004);
return this;
}
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return com.avos.avoscloud.Messages.internal_static_com_avos_avoscloud_DataCommand_descriptor;
}
public com.avos.avoscloud.Messages.DataCommand getDefaultInstanceForType() {
return com.avos.avoscloud.Messages.DataCommand.getDefaultInstance();
}
public com.avos.avoscloud.Messages.DataCommand build() {
com.avos.avoscloud.Messages.DataCommand result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
public com.avos.avoscloud.Messages.DataCommand buildPartial() {
com.avos.avoscloud.Messages.DataCommand result = new com.avos.avoscloud.Messages.DataCommand(this);
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (((bitField0_ & 0x00000001) == 0x00000001)) {
ids_ = ids_.getUnmodifiableView();
bitField0_ = (bitField0_ & ~0x00000001);
}
result.ids_ = ids_;
if (msgBuilder_ == null) {
if (((bitField0_ & 0x00000002) == 0x00000002)) {
msg_ = java.util.Collections.unmodifiableList(msg_);
bitField0_ = (bitField0_ & ~0x00000002);
}
result.msg_ = msg_;
} else {
result.msg_ = msgBuilder_.build();
}
if (((from_bitField0_ & 0x00000004) == 0x00000004)) {
to_bitField0_ |= 0x00000001;
}
result.offline_ = offline_;
result.bitField0_ = to_bitField0_;
onBuilt();
return result;
}
public Builder clone() {
return (Builder) super.clone();
}
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.setField(field, value);
}
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return (Builder) super.clearField(field);
}
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return (Builder) super.clearOneof(oneof);
}
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return (Builder) super.setRepeatedField(field, index, value);
}
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.addRepeatedField(field, value);
}
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.avos.avoscloud.Messages.DataCommand) {
return mergeFrom((com.avos.avoscloud.Messages.DataCommand)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.avos.avoscloud.Messages.DataCommand other) {
if (other == com.avos.avoscloud.Messages.DataCommand.getDefaultInstance()) return this;
if (!other.ids_.isEmpty()) {
if (ids_.isEmpty()) {
ids_ = other.ids_;
bitField0_ = (bitField0_ & ~0x00000001);
} else {
ensureIdsIsMutable();
ids_.addAll(other.ids_);
}
onChanged();
}
if (msgBuilder_ == null) {
if (!other.msg_.isEmpty()) {
if (msg_.isEmpty()) {
msg_ = other.msg_;
bitField0_ = (bitField0_ & ~0x00000002);
} else {
ensureMsgIsMutable();
msg_.addAll(other.msg_);
}
onChanged();
}
} else {
if (!other.msg_.isEmpty()) {
if (msgBuilder_.isEmpty()) {
msgBuilder_.dispose();
msgBuilder_ = null;
msg_ = other.msg_;
bitField0_ = (bitField0_ & ~0x00000002);
msgBuilder_ =
com.google.protobuf.GeneratedMessageV3.alwaysUseFieldBuilders ?
getMsgFieldBuilder() : null;
} else {
msgBuilder_.addAllMessages(other.msg_);
}
}
}
if (other.hasOffline()) {
setOffline(other.getOffline());
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
public final boolean isInitialized() {
for (int i = 0; i < getMsgCount(); i++) {
if (!getMsg(i).isInitialized()) {
return false;
}
}
return true;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
com.avos.avoscloud.Messages.DataCommand parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (com.avos.avoscloud.Messages.DataCommand) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
private com.google.protobuf.LazyStringList ids_ = com.google.protobuf.LazyStringArrayList.EMPTY;
private void ensureIdsIsMutable() {
if (!((bitField0_ & 0x00000001) == 0x00000001)) {
ids_ = new com.google.protobuf.LazyStringArrayList(ids_);
bitField0_ |= 0x00000001;
}
}
/**
* repeated string ids = 1;
*/
public com.google.protobuf.ProtocolStringList
getIdsList() {
return ids_.getUnmodifiableView();
}
/**
* repeated string ids = 1;
*/
public int getIdsCount() {
return ids_.size();
}
/**
* repeated string ids = 1;
*/
public java.lang.String getIds(int index) {
return ids_.get(index);
}
/**
* repeated string ids = 1;
*/
public com.google.protobuf.ByteString
getIdsBytes(int index) {
return ids_.getByteString(index);
}
/**
* repeated string ids = 1;
*/
public Builder setIds(
int index, java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
ensureIdsIsMutable();
ids_.set(index, value);
onChanged();
return this;
}
/**
* repeated string ids = 1;
*/
public Builder addIds(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
ensureIdsIsMutable();
ids_.add(value);
onChanged();
return this;
}
/**
* repeated string ids = 1;
*/
public Builder addAllIds(
java.lang.Iterable values) {
ensureIdsIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, ids_);
onChanged();
return this;
}
/**
* repeated string ids = 1;
*/
public Builder clearIds() {
ids_ = com.google.protobuf.LazyStringArrayList.EMPTY;
bitField0_ = (bitField0_ & ~0x00000001);
onChanged();
return this;
}
/**
* repeated string ids = 1;
*/
public Builder addIdsBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
ensureIdsIsMutable();
ids_.add(value);
onChanged();
return this;
}
private java.util.List msg_ =
java.util.Collections.emptyList();
private void ensureMsgIsMutable() {
if (!((bitField0_ & 0x00000002) == 0x00000002)) {
msg_ = new java.util.ArrayList(msg_);
bitField0_ |= 0x00000002;
}
}
private com.google.protobuf.RepeatedFieldBuilderV3<
com.avos.avoscloud.Messages.JsonObjectMessage, com.avos.avoscloud.Messages.JsonObjectMessage.Builder, com.avos.avoscloud.Messages.JsonObjectMessageOrBuilder> msgBuilder_;
/**
* repeated .com.avos.avoscloud.JsonObjectMessage msg = 2;
*/
public java.util.List getMsgList() {
if (msgBuilder_ == null) {
return java.util.Collections.unmodifiableList(msg_);
} else {
return msgBuilder_.getMessageList();
}
}
/**
* repeated .com.avos.avoscloud.JsonObjectMessage msg = 2;
*/
public int getMsgCount() {
if (msgBuilder_ == null) {
return msg_.size();
} else {
return msgBuilder_.getCount();
}
}
/**
* repeated .com.avos.avoscloud.JsonObjectMessage msg = 2;
*/
public com.avos.avoscloud.Messages.JsonObjectMessage getMsg(int index) {
if (msgBuilder_ == null) {
return msg_.get(index);
} else {
return msgBuilder_.getMessage(index);
}
}
/**
* repeated .com.avos.avoscloud.JsonObjectMessage msg = 2;
*/
public Builder setMsg(
int index, com.avos.avoscloud.Messages.JsonObjectMessage value) {
if (msgBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureMsgIsMutable();
msg_.set(index, value);
onChanged();
} else {
msgBuilder_.setMessage(index, value);
}
return this;
}
/**
* repeated .com.avos.avoscloud.JsonObjectMessage msg = 2;
*/
public Builder setMsg(
int index, com.avos.avoscloud.Messages.JsonObjectMessage.Builder builderForValue) {
if (msgBuilder_ == null) {
ensureMsgIsMutable();
msg_.set(index, builderForValue.build());
onChanged();
} else {
msgBuilder_.setMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .com.avos.avoscloud.JsonObjectMessage msg = 2;
*/
public Builder addMsg(com.avos.avoscloud.Messages.JsonObjectMessage value) {
if (msgBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureMsgIsMutable();
msg_.add(value);
onChanged();
} else {
msgBuilder_.addMessage(value);
}
return this;
}
/**
* repeated .com.avos.avoscloud.JsonObjectMessage msg = 2;
*/
public Builder addMsg(
int index, com.avos.avoscloud.Messages.JsonObjectMessage value) {
if (msgBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureMsgIsMutable();
msg_.add(index, value);
onChanged();
} else {
msgBuilder_.addMessage(index, value);
}
return this;
}
/**
* repeated .com.avos.avoscloud.JsonObjectMessage msg = 2;
*/
public Builder addMsg(
com.avos.avoscloud.Messages.JsonObjectMessage.Builder builderForValue) {
if (msgBuilder_ == null) {
ensureMsgIsMutable();
msg_.add(builderForValue.build());
onChanged();
} else {
msgBuilder_.addMessage(builderForValue.build());
}
return this;
}
/**
* repeated .com.avos.avoscloud.JsonObjectMessage msg = 2;
*/
public Builder addMsg(
int index, com.avos.avoscloud.Messages.JsonObjectMessage.Builder builderForValue) {
if (msgBuilder_ == null) {
ensureMsgIsMutable();
msg_.add(index, builderForValue.build());
onChanged();
} else {
msgBuilder_.addMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .com.avos.avoscloud.JsonObjectMessage msg = 2;
*/
public Builder addAllMsg(
java.lang.Iterable extends com.avos.avoscloud.Messages.JsonObjectMessage> values) {
if (msgBuilder_ == null) {
ensureMsgIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, msg_);
onChanged();
} else {
msgBuilder_.addAllMessages(values);
}
return this;
}
/**
* repeated .com.avos.avoscloud.JsonObjectMessage msg = 2;
*/
public Builder clearMsg() {
if (msgBuilder_ == null) {
msg_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000002);
onChanged();
} else {
msgBuilder_.clear();
}
return this;
}
/**
* repeated .com.avos.avoscloud.JsonObjectMessage msg = 2;
*/
public Builder removeMsg(int index) {
if (msgBuilder_ == null) {
ensureMsgIsMutable();
msg_.remove(index);
onChanged();
} else {
msgBuilder_.remove(index);
}
return this;
}
/**
* repeated .com.avos.avoscloud.JsonObjectMessage msg = 2;
*/
public com.avos.avoscloud.Messages.JsonObjectMessage.Builder getMsgBuilder(
int index) {
return getMsgFieldBuilder().getBuilder(index);
}
/**
* repeated .com.avos.avoscloud.JsonObjectMessage msg = 2;
*/
public com.avos.avoscloud.Messages.JsonObjectMessageOrBuilder getMsgOrBuilder(
int index) {
if (msgBuilder_ == null) {
return msg_.get(index); } else {
return msgBuilder_.getMessageOrBuilder(index);
}
}
/**
* repeated .com.avos.avoscloud.JsonObjectMessage msg = 2;
*/
public java.util.List extends com.avos.avoscloud.Messages.JsonObjectMessageOrBuilder>
getMsgOrBuilderList() {
if (msgBuilder_ != null) {
return msgBuilder_.getMessageOrBuilderList();
} else {
return java.util.Collections.unmodifiableList(msg_);
}
}
/**
* repeated .com.avos.avoscloud.JsonObjectMessage msg = 2;
*/
public com.avos.avoscloud.Messages.JsonObjectMessage.Builder addMsgBuilder() {
return getMsgFieldBuilder().addBuilder(
com.avos.avoscloud.Messages.JsonObjectMessage.getDefaultInstance());
}
/**
* repeated .com.avos.avoscloud.JsonObjectMessage msg = 2;
*/
public com.avos.avoscloud.Messages.JsonObjectMessage.Builder addMsgBuilder(
int index) {
return getMsgFieldBuilder().addBuilder(
index, com.avos.avoscloud.Messages.JsonObjectMessage.getDefaultInstance());
}
/**
* repeated .com.avos.avoscloud.JsonObjectMessage msg = 2;
*/
public java.util.List
getMsgBuilderList() {
return getMsgFieldBuilder().getBuilderList();
}
private com.google.protobuf.RepeatedFieldBuilderV3<
com.avos.avoscloud.Messages.JsonObjectMessage, com.avos.avoscloud.Messages.JsonObjectMessage.Builder, com.avos.avoscloud.Messages.JsonObjectMessageOrBuilder>
getMsgFieldBuilder() {
if (msgBuilder_ == null) {
msgBuilder_ = new com.google.protobuf.RepeatedFieldBuilderV3<
com.avos.avoscloud.Messages.JsonObjectMessage, com.avos.avoscloud.Messages.JsonObjectMessage.Builder, com.avos.avoscloud.Messages.JsonObjectMessageOrBuilder>(
msg_,
((bitField0_ & 0x00000002) == 0x00000002),
getParentForChildren(),
isClean());
msg_ = null;
}
return msgBuilder_;
}
private boolean offline_ ;
/**
* optional bool offline = 3;
*/
public boolean hasOffline() {
return ((bitField0_ & 0x00000004) == 0x00000004);
}
/**
* optional bool offline = 3;
*/
public boolean getOffline() {
return offline_;
}
/**
* optional bool offline = 3;
*/
public Builder setOffline(boolean value) {
bitField0_ |= 0x00000004;
offline_ = value;
onChanged();
return this;
}
/**
* optional bool offline = 3;
*/
public Builder clearOffline() {
bitField0_ = (bitField0_ & ~0x00000004);
offline_ = false;
onChanged();
return this;
}
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:com.avos.avoscloud.DataCommand)
}
// @@protoc_insertion_point(class_scope:com.avos.avoscloud.DataCommand)
private static final com.avos.avoscloud.Messages.DataCommand DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new com.avos.avoscloud.Messages.DataCommand();
}
public static com.avos.avoscloud.Messages.DataCommand getDefaultInstance() {
return DEFAULT_INSTANCE;
}
@java.lang.Deprecated public static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
public DataCommand parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new DataCommand(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
public com.avos.avoscloud.Messages.DataCommand getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface SessionCommandOrBuilder extends
// @@protoc_insertion_point(interface_extends:com.avos.avoscloud.SessionCommand)
com.google.protobuf.MessageOrBuilder {
/**
* optional int64 t = 1;
*/
boolean hasT();
/**
* optional int64 t = 1;
*/
long getT();
/**
* optional string n = 2;
*/
boolean hasN();
/**
* optional string n = 2;
*/
java.lang.String getN();
/**
* optional string n = 2;
*/
com.google.protobuf.ByteString
getNBytes();
/**
* optional string s = 3;
*/
boolean hasS();
/**
* optional string s = 3;
*/
java.lang.String getS();
/**
* optional string s = 3;
*/
com.google.protobuf.ByteString
getSBytes();
/**
* optional string ua = 4;
*/
boolean hasUa();
/**
* optional string ua = 4;
*/
java.lang.String getUa();
/**
* optional string ua = 4;
*/
com.google.protobuf.ByteString
getUaBytes();
/**
* optional bool r = 5;
*/
boolean hasR();
/**
* optional bool r = 5;
*/
boolean getR();
/**
* optional string tag = 6;
*/
boolean hasTag();
/**
* optional string tag = 6;
*/
java.lang.String getTag();
/**
* optional string tag = 6;
*/
com.google.protobuf.ByteString
getTagBytes();
/**
* optional string deviceId = 7;
*/
boolean hasDeviceId();
/**
* optional string deviceId = 7;
*/
java.lang.String getDeviceId();
/**
* optional string deviceId = 7;
*/
com.google.protobuf.ByteString
getDeviceIdBytes();
/**
* repeated string sessionPeerIds = 8;
*/
java.util.List
getSessionPeerIdsList();
/**
* repeated string sessionPeerIds = 8;
*/
int getSessionPeerIdsCount();
/**
* repeated string sessionPeerIds = 8;
*/
java.lang.String getSessionPeerIds(int index);
/**
* repeated string sessionPeerIds = 8;
*/
com.google.protobuf.ByteString
getSessionPeerIdsBytes(int index);
/**
* repeated string onlineSessionPeerIds = 9;
*/
java.util.List
getOnlineSessionPeerIdsList();
/**
* repeated string onlineSessionPeerIds = 9;
*/
int getOnlineSessionPeerIdsCount();
/**
* repeated string onlineSessionPeerIds = 9;
*/
java.lang.String getOnlineSessionPeerIds(int index);
/**
* repeated string onlineSessionPeerIds = 9;
*/
com.google.protobuf.ByteString
getOnlineSessionPeerIdsBytes(int index);
/**
* optional string st = 10;
*/
boolean hasSt();
/**
* optional string st = 10;
*/
java.lang.String getSt();
/**
* optional string st = 10;
*/
com.google.protobuf.ByteString
getStBytes();
/**
* optional int32 stTtl = 11;
*/
boolean hasStTtl();
/**
* optional int32 stTtl = 11;
*/
int getStTtl();
/**
* optional int32 code = 12;
*/
boolean hasCode();
/**
* optional int32 code = 12;
*/
int getCode();
/**
* optional string reason = 13;
*/
boolean hasReason();
/**
* optional string reason = 13;
*/
java.lang.String getReason();
/**
* optional string reason = 13;
*/
com.google.protobuf.ByteString
getReasonBytes();
/**
* optional string deviceToken = 14;
*/
boolean hasDeviceToken();
/**
* optional string deviceToken = 14;
*/
java.lang.String getDeviceToken();
/**
* optional string deviceToken = 14;
*/
com.google.protobuf.ByteString
getDeviceTokenBytes();
/**
* optional bool sp = 15;
*/
boolean hasSp();
/**
* optional bool sp = 15;
*/
boolean getSp();
/**
* optional string detail = 16;
*/
boolean hasDetail();
/**
* optional string detail = 16;
*/
java.lang.String getDetail();
/**
* optional string detail = 16;
*/
com.google.protobuf.ByteString
getDetailBytes();
/**
* optional int64 lastUnreadNotifTime = 17;
*/
boolean hasLastUnreadNotifTime();
/**
* optional int64 lastUnreadNotifTime = 17;
*/
long getLastUnreadNotifTime();
/**
* optional int64 lastPatchTime = 18;
*/
boolean hasLastPatchTime();
/**
* optional int64 lastPatchTime = 18;
*/
long getLastPatchTime();
/**
* optional int64 configBitmap = 19;
*/
boolean hasConfigBitmap();
/**
* optional int64 configBitmap = 19;
*/
long getConfigBitmap();
}
/**
* Protobuf type {@code com.avos.avoscloud.SessionCommand}
*/
public static final class SessionCommand extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:com.avos.avoscloud.SessionCommand)
SessionCommandOrBuilder {
private static final long serialVersionUID = 0L;
// Use SessionCommand.newBuilder() to construct.
private SessionCommand(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private SessionCommand() {
t_ = 0L;
n_ = "";
s_ = "";
ua_ = "";
r_ = false;
tag_ = "";
deviceId_ = "";
sessionPeerIds_ = com.google.protobuf.LazyStringArrayList.EMPTY;
onlineSessionPeerIds_ = com.google.protobuf.LazyStringArrayList.EMPTY;
st_ = "";
stTtl_ = 0;
code_ = 0;
reason_ = "";
deviceToken_ = "";
sp_ = false;
detail_ = "";
lastUnreadNotifTime_ = 0L;
lastPatchTime_ = 0L;
configBitmap_ = 0L;
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private SessionCommand(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!parseUnknownField(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
case 8: {
bitField0_ |= 0x00000001;
t_ = input.readInt64();
break;
}
case 18: {
com.google.protobuf.ByteString bs = input.readBytes();
bitField0_ |= 0x00000002;
n_ = bs;
break;
}
case 26: {
com.google.protobuf.ByteString bs = input.readBytes();
bitField0_ |= 0x00000004;
s_ = bs;
break;
}
case 34: {
com.google.protobuf.ByteString bs = input.readBytes();
bitField0_ |= 0x00000008;
ua_ = bs;
break;
}
case 40: {
bitField0_ |= 0x00000010;
r_ = input.readBool();
break;
}
case 50: {
com.google.protobuf.ByteString bs = input.readBytes();
bitField0_ |= 0x00000020;
tag_ = bs;
break;
}
case 58: {
com.google.protobuf.ByteString bs = input.readBytes();
bitField0_ |= 0x00000040;
deviceId_ = bs;
break;
}
case 66: {
com.google.protobuf.ByteString bs = input.readBytes();
if (!((mutable_bitField0_ & 0x00000080) == 0x00000080)) {
sessionPeerIds_ = new com.google.protobuf.LazyStringArrayList();
mutable_bitField0_ |= 0x00000080;
}
sessionPeerIds_.add(bs);
break;
}
case 74: {
com.google.protobuf.ByteString bs = input.readBytes();
if (!((mutable_bitField0_ & 0x00000100) == 0x00000100)) {
onlineSessionPeerIds_ = new com.google.protobuf.LazyStringArrayList();
mutable_bitField0_ |= 0x00000100;
}
onlineSessionPeerIds_.add(bs);
break;
}
case 82: {
com.google.protobuf.ByteString bs = input.readBytes();
bitField0_ |= 0x00000080;
st_ = bs;
break;
}
case 88: {
bitField0_ |= 0x00000100;
stTtl_ = input.readInt32();
break;
}
case 96: {
bitField0_ |= 0x00000200;
code_ = input.readInt32();
break;
}
case 106: {
com.google.protobuf.ByteString bs = input.readBytes();
bitField0_ |= 0x00000400;
reason_ = bs;
break;
}
case 114: {
com.google.protobuf.ByteString bs = input.readBytes();
bitField0_ |= 0x00000800;
deviceToken_ = bs;
break;
}
case 120: {
bitField0_ |= 0x00001000;
sp_ = input.readBool();
break;
}
case 130: {
com.google.protobuf.ByteString bs = input.readBytes();
bitField0_ |= 0x00002000;
detail_ = bs;
break;
}
case 136: {
bitField0_ |= 0x00004000;
lastUnreadNotifTime_ = input.readInt64();
break;
}
case 144: {
bitField0_ |= 0x00008000;
lastPatchTime_ = input.readInt64();
break;
}
case 152: {
bitField0_ |= 0x00010000;
configBitmap_ = input.readInt64();
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
if (((mutable_bitField0_ & 0x00000080) == 0x00000080)) {
sessionPeerIds_ = sessionPeerIds_.getUnmodifiableView();
}
if (((mutable_bitField0_ & 0x00000100) == 0x00000100)) {
onlineSessionPeerIds_ = onlineSessionPeerIds_.getUnmodifiableView();
}
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.avos.avoscloud.Messages.internal_static_com_avos_avoscloud_SessionCommand_descriptor;
}
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.avos.avoscloud.Messages.internal_static_com_avos_avoscloud_SessionCommand_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.avos.avoscloud.Messages.SessionCommand.class, com.avos.avoscloud.Messages.SessionCommand.Builder.class);
}
private int bitField0_;
public static final int T_FIELD_NUMBER = 1;
private long t_;
/**
* optional int64 t = 1;
*/
public boolean hasT() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
* optional int64 t = 1;
*/
public long getT() {
return t_;
}
public static final int N_FIELD_NUMBER = 2;
private volatile java.lang.Object n_;
/**
* optional string n = 2;
*/
public boolean hasN() {
return ((bitField0_ & 0x00000002) == 0x00000002);
}
/**
* optional string n = 2;
*/
public java.lang.String getN() {
java.lang.Object ref = n_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
n_ = s;
}
return s;
}
}
/**
* optional string n = 2;
*/
public com.google.protobuf.ByteString
getNBytes() {
java.lang.Object ref = n_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
n_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int S_FIELD_NUMBER = 3;
private volatile java.lang.Object s_;
/**
* optional string s = 3;
*/
public boolean hasS() {
return ((bitField0_ & 0x00000004) == 0x00000004);
}
/**
* optional string s = 3;
*/
public java.lang.String getS() {
java.lang.Object ref = s_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
s_ = s;
}
return s;
}
}
/**
* optional string s = 3;
*/
public com.google.protobuf.ByteString
getSBytes() {
java.lang.Object ref = s_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
s_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int UA_FIELD_NUMBER = 4;
private volatile java.lang.Object ua_;
/**
* optional string ua = 4;
*/
public boolean hasUa() {
return ((bitField0_ & 0x00000008) == 0x00000008);
}
/**
* optional string ua = 4;
*/
public java.lang.String getUa() {
java.lang.Object ref = ua_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
ua_ = s;
}
return s;
}
}
/**
* optional string ua = 4;
*/
public com.google.protobuf.ByteString
getUaBytes() {
java.lang.Object ref = ua_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
ua_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int R_FIELD_NUMBER = 5;
private boolean r_;
/**
* optional bool r = 5;
*/
public boolean hasR() {
return ((bitField0_ & 0x00000010) == 0x00000010);
}
/**
* optional bool r = 5;
*/
public boolean getR() {
return r_;
}
public static final int TAG_FIELD_NUMBER = 6;
private volatile java.lang.Object tag_;
/**
* optional string tag = 6;
*/
public boolean hasTag() {
return ((bitField0_ & 0x00000020) == 0x00000020);
}
/**
* optional string tag = 6;
*/
public java.lang.String getTag() {
java.lang.Object ref = tag_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
tag_ = s;
}
return s;
}
}
/**
* optional string tag = 6;
*/
public com.google.protobuf.ByteString
getTagBytes() {
java.lang.Object ref = tag_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
tag_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int DEVICEID_FIELD_NUMBER = 7;
private volatile java.lang.Object deviceId_;
/**
* optional string deviceId = 7;
*/
public boolean hasDeviceId() {
return ((bitField0_ & 0x00000040) == 0x00000040);
}
/**
* optional string deviceId = 7;
*/
public java.lang.String getDeviceId() {
java.lang.Object ref = deviceId_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
deviceId_ = s;
}
return s;
}
}
/**
* optional string deviceId = 7;
*/
public com.google.protobuf.ByteString
getDeviceIdBytes() {
java.lang.Object ref = deviceId_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
deviceId_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int SESSIONPEERIDS_FIELD_NUMBER = 8;
private com.google.protobuf.LazyStringList sessionPeerIds_;
/**
* repeated string sessionPeerIds = 8;
*/
public com.google.protobuf.ProtocolStringList
getSessionPeerIdsList() {
return sessionPeerIds_;
}
/**
* repeated string sessionPeerIds = 8;
*/
public int getSessionPeerIdsCount() {
return sessionPeerIds_.size();
}
/**
* repeated string sessionPeerIds = 8;
*/
public java.lang.String getSessionPeerIds(int index) {
return sessionPeerIds_.get(index);
}
/**
* repeated string sessionPeerIds = 8;
*/
public com.google.protobuf.ByteString
getSessionPeerIdsBytes(int index) {
return sessionPeerIds_.getByteString(index);
}
public static final int ONLINESESSIONPEERIDS_FIELD_NUMBER = 9;
private com.google.protobuf.LazyStringList onlineSessionPeerIds_;
/**
* repeated string onlineSessionPeerIds = 9;
*/
public com.google.protobuf.ProtocolStringList
getOnlineSessionPeerIdsList() {
return onlineSessionPeerIds_;
}
/**
* repeated string onlineSessionPeerIds = 9;
*/
public int getOnlineSessionPeerIdsCount() {
return onlineSessionPeerIds_.size();
}
/**
* repeated string onlineSessionPeerIds = 9;
*/
public java.lang.String getOnlineSessionPeerIds(int index) {
return onlineSessionPeerIds_.get(index);
}
/**
* repeated string onlineSessionPeerIds = 9;
*/
public com.google.protobuf.ByteString
getOnlineSessionPeerIdsBytes(int index) {
return onlineSessionPeerIds_.getByteString(index);
}
public static final int ST_FIELD_NUMBER = 10;
private volatile java.lang.Object st_;
/**
* optional string st = 10;
*/
public boolean hasSt() {
return ((bitField0_ & 0x00000080) == 0x00000080);
}
/**
* optional string st = 10;
*/
public java.lang.String getSt() {
java.lang.Object ref = st_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
st_ = s;
}
return s;
}
}
/**
* optional string st = 10;
*/
public com.google.protobuf.ByteString
getStBytes() {
java.lang.Object ref = st_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
st_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int STTTL_FIELD_NUMBER = 11;
private int stTtl_;
/**
* optional int32 stTtl = 11;
*/
public boolean hasStTtl() {
return ((bitField0_ & 0x00000100) == 0x00000100);
}
/**
* optional int32 stTtl = 11;
*/
public int getStTtl() {
return stTtl_;
}
public static final int CODE_FIELD_NUMBER = 12;
private int code_;
/**
* optional int32 code = 12;
*/
public boolean hasCode() {
return ((bitField0_ & 0x00000200) == 0x00000200);
}
/**
* optional int32 code = 12;
*/
public int getCode() {
return code_;
}
public static final int REASON_FIELD_NUMBER = 13;
private volatile java.lang.Object reason_;
/**
* optional string reason = 13;
*/
public boolean hasReason() {
return ((bitField0_ & 0x00000400) == 0x00000400);
}
/**
* optional string reason = 13;
*/
public java.lang.String getReason() {
java.lang.Object ref = reason_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
reason_ = s;
}
return s;
}
}
/**
* optional string reason = 13;
*/
public com.google.protobuf.ByteString
getReasonBytes() {
java.lang.Object ref = reason_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
reason_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int DEVICETOKEN_FIELD_NUMBER = 14;
private volatile java.lang.Object deviceToken_;
/**
* optional string deviceToken = 14;
*/
public boolean hasDeviceToken() {
return ((bitField0_ & 0x00000800) == 0x00000800);
}
/**
* optional string deviceToken = 14;
*/
public java.lang.String getDeviceToken() {
java.lang.Object ref = deviceToken_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
deviceToken_ = s;
}
return s;
}
}
/**
* optional string deviceToken = 14;
*/
public com.google.protobuf.ByteString
getDeviceTokenBytes() {
java.lang.Object ref = deviceToken_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
deviceToken_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int SP_FIELD_NUMBER = 15;
private boolean sp_;
/**
* optional bool sp = 15;
*/
public boolean hasSp() {
return ((bitField0_ & 0x00001000) == 0x00001000);
}
/**
* optional bool sp = 15;
*/
public boolean getSp() {
return sp_;
}
public static final int DETAIL_FIELD_NUMBER = 16;
private volatile java.lang.Object detail_;
/**
* optional string detail = 16;
*/
public boolean hasDetail() {
return ((bitField0_ & 0x00002000) == 0x00002000);
}
/**
* optional string detail = 16;
*/
public java.lang.String getDetail() {
java.lang.Object ref = detail_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
detail_ = s;
}
return s;
}
}
/**
* optional string detail = 16;
*/
public com.google.protobuf.ByteString
getDetailBytes() {
java.lang.Object ref = detail_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
detail_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int LASTUNREADNOTIFTIME_FIELD_NUMBER = 17;
private long lastUnreadNotifTime_;
/**
* optional int64 lastUnreadNotifTime = 17;
*/
public boolean hasLastUnreadNotifTime() {
return ((bitField0_ & 0x00004000) == 0x00004000);
}
/**
* optional int64 lastUnreadNotifTime = 17;
*/
public long getLastUnreadNotifTime() {
return lastUnreadNotifTime_;
}
public static final int LASTPATCHTIME_FIELD_NUMBER = 18;
private long lastPatchTime_;
/**
* optional int64 lastPatchTime = 18;
*/
public boolean hasLastPatchTime() {
return ((bitField0_ & 0x00008000) == 0x00008000);
}
/**
* optional int64 lastPatchTime = 18;
*/
public long getLastPatchTime() {
return lastPatchTime_;
}
public static final int CONFIGBITMAP_FIELD_NUMBER = 19;
private long configBitmap_;
/**
* optional int64 configBitmap = 19;
*/
public boolean hasConfigBitmap() {
return ((bitField0_ & 0x00010000) == 0x00010000);
}
/**
* optional int64 configBitmap = 19;
*/
public long getConfigBitmap() {
return configBitmap_;
}
private byte memoizedIsInitialized = -1;
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (((bitField0_ & 0x00000001) == 0x00000001)) {
output.writeInt64(1, t_);
}
if (((bitField0_ & 0x00000002) == 0x00000002)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 2, n_);
}
if (((bitField0_ & 0x00000004) == 0x00000004)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 3, s_);
}
if (((bitField0_ & 0x00000008) == 0x00000008)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 4, ua_);
}
if (((bitField0_ & 0x00000010) == 0x00000010)) {
output.writeBool(5, r_);
}
if (((bitField0_ & 0x00000020) == 0x00000020)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 6, tag_);
}
if (((bitField0_ & 0x00000040) == 0x00000040)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 7, deviceId_);
}
for (int i = 0; i < sessionPeerIds_.size(); i++) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 8, sessionPeerIds_.getRaw(i));
}
for (int i = 0; i < onlineSessionPeerIds_.size(); i++) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 9, onlineSessionPeerIds_.getRaw(i));
}
if (((bitField0_ & 0x00000080) == 0x00000080)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 10, st_);
}
if (((bitField0_ & 0x00000100) == 0x00000100)) {
output.writeInt32(11, stTtl_);
}
if (((bitField0_ & 0x00000200) == 0x00000200)) {
output.writeInt32(12, code_);
}
if (((bitField0_ & 0x00000400) == 0x00000400)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 13, reason_);
}
if (((bitField0_ & 0x00000800) == 0x00000800)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 14, deviceToken_);
}
if (((bitField0_ & 0x00001000) == 0x00001000)) {
output.writeBool(15, sp_);
}
if (((bitField0_ & 0x00002000) == 0x00002000)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 16, detail_);
}
if (((bitField0_ & 0x00004000) == 0x00004000)) {
output.writeInt64(17, lastUnreadNotifTime_);
}
if (((bitField0_ & 0x00008000) == 0x00008000)) {
output.writeInt64(18, lastPatchTime_);
}
if (((bitField0_ & 0x00010000) == 0x00010000)) {
output.writeInt64(19, configBitmap_);
}
unknownFields.writeTo(output);
}
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (((bitField0_ & 0x00000001) == 0x00000001)) {
size += com.google.protobuf.CodedOutputStream
.computeInt64Size(1, t_);
}
if (((bitField0_ & 0x00000002) == 0x00000002)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(2, n_);
}
if (((bitField0_ & 0x00000004) == 0x00000004)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(3, s_);
}
if (((bitField0_ & 0x00000008) == 0x00000008)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(4, ua_);
}
if (((bitField0_ & 0x00000010) == 0x00000010)) {
size += com.google.protobuf.CodedOutputStream
.computeBoolSize(5, r_);
}
if (((bitField0_ & 0x00000020) == 0x00000020)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(6, tag_);
}
if (((bitField0_ & 0x00000040) == 0x00000040)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(7, deviceId_);
}
{
int dataSize = 0;
for (int i = 0; i < sessionPeerIds_.size(); i++) {
dataSize += computeStringSizeNoTag(sessionPeerIds_.getRaw(i));
}
size += dataSize;
size += 1 * getSessionPeerIdsList().size();
}
{
int dataSize = 0;
for (int i = 0; i < onlineSessionPeerIds_.size(); i++) {
dataSize += computeStringSizeNoTag(onlineSessionPeerIds_.getRaw(i));
}
size += dataSize;
size += 1 * getOnlineSessionPeerIdsList().size();
}
if (((bitField0_ & 0x00000080) == 0x00000080)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(10, st_);
}
if (((bitField0_ & 0x00000100) == 0x00000100)) {
size += com.google.protobuf.CodedOutputStream
.computeInt32Size(11, stTtl_);
}
if (((bitField0_ & 0x00000200) == 0x00000200)) {
size += com.google.protobuf.CodedOutputStream
.computeInt32Size(12, code_);
}
if (((bitField0_ & 0x00000400) == 0x00000400)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(13, reason_);
}
if (((bitField0_ & 0x00000800) == 0x00000800)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(14, deviceToken_);
}
if (((bitField0_ & 0x00001000) == 0x00001000)) {
size += com.google.protobuf.CodedOutputStream
.computeBoolSize(15, sp_);
}
if (((bitField0_ & 0x00002000) == 0x00002000)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(16, detail_);
}
if (((bitField0_ & 0x00004000) == 0x00004000)) {
size += com.google.protobuf.CodedOutputStream
.computeInt64Size(17, lastUnreadNotifTime_);
}
if (((bitField0_ & 0x00008000) == 0x00008000)) {
size += com.google.protobuf.CodedOutputStream
.computeInt64Size(18, lastPatchTime_);
}
if (((bitField0_ & 0x00010000) == 0x00010000)) {
size += com.google.protobuf.CodedOutputStream
.computeInt64Size(19, configBitmap_);
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof com.avos.avoscloud.Messages.SessionCommand)) {
return super.equals(obj);
}
com.avos.avoscloud.Messages.SessionCommand other = (com.avos.avoscloud.Messages.SessionCommand) obj;
boolean result = true;
result = result && (hasT() == other.hasT());
if (hasT()) {
result = result && (getT()
== other.getT());
}
result = result && (hasN() == other.hasN());
if (hasN()) {
result = result && getN()
.equals(other.getN());
}
result = result && (hasS() == other.hasS());
if (hasS()) {
result = result && getS()
.equals(other.getS());
}
result = result && (hasUa() == other.hasUa());
if (hasUa()) {
result = result && getUa()
.equals(other.getUa());
}
result = result && (hasR() == other.hasR());
if (hasR()) {
result = result && (getR()
== other.getR());
}
result = result && (hasTag() == other.hasTag());
if (hasTag()) {
result = result && getTag()
.equals(other.getTag());
}
result = result && (hasDeviceId() == other.hasDeviceId());
if (hasDeviceId()) {
result = result && getDeviceId()
.equals(other.getDeviceId());
}
result = result && getSessionPeerIdsList()
.equals(other.getSessionPeerIdsList());
result = result && getOnlineSessionPeerIdsList()
.equals(other.getOnlineSessionPeerIdsList());
result = result && (hasSt() == other.hasSt());
if (hasSt()) {
result = result && getSt()
.equals(other.getSt());
}
result = result && (hasStTtl() == other.hasStTtl());
if (hasStTtl()) {
result = result && (getStTtl()
== other.getStTtl());
}
result = result && (hasCode() == other.hasCode());
if (hasCode()) {
result = result && (getCode()
== other.getCode());
}
result = result && (hasReason() == other.hasReason());
if (hasReason()) {
result = result && getReason()
.equals(other.getReason());
}
result = result && (hasDeviceToken() == other.hasDeviceToken());
if (hasDeviceToken()) {
result = result && getDeviceToken()
.equals(other.getDeviceToken());
}
result = result && (hasSp() == other.hasSp());
if (hasSp()) {
result = result && (getSp()
== other.getSp());
}
result = result && (hasDetail() == other.hasDetail());
if (hasDetail()) {
result = result && getDetail()
.equals(other.getDetail());
}
result = result && (hasLastUnreadNotifTime() == other.hasLastUnreadNotifTime());
if (hasLastUnreadNotifTime()) {
result = result && (getLastUnreadNotifTime()
== other.getLastUnreadNotifTime());
}
result = result && (hasLastPatchTime() == other.hasLastPatchTime());
if (hasLastPatchTime()) {
result = result && (getLastPatchTime()
== other.getLastPatchTime());
}
result = result && (hasConfigBitmap() == other.hasConfigBitmap());
if (hasConfigBitmap()) {
result = result && (getConfigBitmap()
== other.getConfigBitmap());
}
result = result && unknownFields.equals(other.unknownFields);
return result;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (hasT()) {
hash = (37 * hash) + T_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getT());
}
if (hasN()) {
hash = (37 * hash) + N_FIELD_NUMBER;
hash = (53 * hash) + getN().hashCode();
}
if (hasS()) {
hash = (37 * hash) + S_FIELD_NUMBER;
hash = (53 * hash) + getS().hashCode();
}
if (hasUa()) {
hash = (37 * hash) + UA_FIELD_NUMBER;
hash = (53 * hash) + getUa().hashCode();
}
if (hasR()) {
hash = (37 * hash) + R_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashBoolean(
getR());
}
if (hasTag()) {
hash = (37 * hash) + TAG_FIELD_NUMBER;
hash = (53 * hash) + getTag().hashCode();
}
if (hasDeviceId()) {
hash = (37 * hash) + DEVICEID_FIELD_NUMBER;
hash = (53 * hash) + getDeviceId().hashCode();
}
if (getSessionPeerIdsCount() > 0) {
hash = (37 * hash) + SESSIONPEERIDS_FIELD_NUMBER;
hash = (53 * hash) + getSessionPeerIdsList().hashCode();
}
if (getOnlineSessionPeerIdsCount() > 0) {
hash = (37 * hash) + ONLINESESSIONPEERIDS_FIELD_NUMBER;
hash = (53 * hash) + getOnlineSessionPeerIdsList().hashCode();
}
if (hasSt()) {
hash = (37 * hash) + ST_FIELD_NUMBER;
hash = (53 * hash) + getSt().hashCode();
}
if (hasStTtl()) {
hash = (37 * hash) + STTTL_FIELD_NUMBER;
hash = (53 * hash) + getStTtl();
}
if (hasCode()) {
hash = (37 * hash) + CODE_FIELD_NUMBER;
hash = (53 * hash) + getCode();
}
if (hasReason()) {
hash = (37 * hash) + REASON_FIELD_NUMBER;
hash = (53 * hash) + getReason().hashCode();
}
if (hasDeviceToken()) {
hash = (37 * hash) + DEVICETOKEN_FIELD_NUMBER;
hash = (53 * hash) + getDeviceToken().hashCode();
}
if (hasSp()) {
hash = (37 * hash) + SP_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashBoolean(
getSp());
}
if (hasDetail()) {
hash = (37 * hash) + DETAIL_FIELD_NUMBER;
hash = (53 * hash) + getDetail().hashCode();
}
if (hasLastUnreadNotifTime()) {
hash = (37 * hash) + LASTUNREADNOTIFTIME_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getLastUnreadNotifTime());
}
if (hasLastPatchTime()) {
hash = (37 * hash) + LASTPATCHTIME_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getLastPatchTime());
}
if (hasConfigBitmap()) {
hash = (37 * hash) + CONFIGBITMAP_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getConfigBitmap());
}
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static com.avos.avoscloud.Messages.SessionCommand parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.avos.avoscloud.Messages.SessionCommand parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.avos.avoscloud.Messages.SessionCommand parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.avos.avoscloud.Messages.SessionCommand parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.avos.avoscloud.Messages.SessionCommand parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.avos.avoscloud.Messages.SessionCommand parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.avos.avoscloud.Messages.SessionCommand parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.avos.avoscloud.Messages.SessionCommand parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static com.avos.avoscloud.Messages.SessionCommand parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static com.avos.avoscloud.Messages.SessionCommand parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static com.avos.avoscloud.Messages.SessionCommand parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.avos.avoscloud.Messages.SessionCommand parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(com.avos.avoscloud.Messages.SessionCommand prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code com.avos.avoscloud.SessionCommand}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:com.avos.avoscloud.SessionCommand)
com.avos.avoscloud.Messages.SessionCommandOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.avos.avoscloud.Messages.internal_static_com_avos_avoscloud_SessionCommand_descriptor;
}
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.avos.avoscloud.Messages.internal_static_com_avos_avoscloud_SessionCommand_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.avos.avoscloud.Messages.SessionCommand.class, com.avos.avoscloud.Messages.SessionCommand.Builder.class);
}
// Construct using com.avos.avoscloud.Messages.SessionCommand.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
public Builder clear() {
super.clear();
t_ = 0L;
bitField0_ = (bitField0_ & ~0x00000001);
n_ = "";
bitField0_ = (bitField0_ & ~0x00000002);
s_ = "";
bitField0_ = (bitField0_ & ~0x00000004);
ua_ = "";
bitField0_ = (bitField0_ & ~0x00000008);
r_ = false;
bitField0_ = (bitField0_ & ~0x00000010);
tag_ = "";
bitField0_ = (bitField0_ & ~0x00000020);
deviceId_ = "";
bitField0_ = (bitField0_ & ~0x00000040);
sessionPeerIds_ = com.google.protobuf.LazyStringArrayList.EMPTY;
bitField0_ = (bitField0_ & ~0x00000080);
onlineSessionPeerIds_ = com.google.protobuf.LazyStringArrayList.EMPTY;
bitField0_ = (bitField0_ & ~0x00000100);
st_ = "";
bitField0_ = (bitField0_ & ~0x00000200);
stTtl_ = 0;
bitField0_ = (bitField0_ & ~0x00000400);
code_ = 0;
bitField0_ = (bitField0_ & ~0x00000800);
reason_ = "";
bitField0_ = (bitField0_ & ~0x00001000);
deviceToken_ = "";
bitField0_ = (bitField0_ & ~0x00002000);
sp_ = false;
bitField0_ = (bitField0_ & ~0x00004000);
detail_ = "";
bitField0_ = (bitField0_ & ~0x00008000);
lastUnreadNotifTime_ = 0L;
bitField0_ = (bitField0_ & ~0x00010000);
lastPatchTime_ = 0L;
bitField0_ = (bitField0_ & ~0x00020000);
configBitmap_ = 0L;
bitField0_ = (bitField0_ & ~0x00040000);
return this;
}
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return com.avos.avoscloud.Messages.internal_static_com_avos_avoscloud_SessionCommand_descriptor;
}
public com.avos.avoscloud.Messages.SessionCommand getDefaultInstanceForType() {
return com.avos.avoscloud.Messages.SessionCommand.getDefaultInstance();
}
public com.avos.avoscloud.Messages.SessionCommand build() {
com.avos.avoscloud.Messages.SessionCommand result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
public com.avos.avoscloud.Messages.SessionCommand buildPartial() {
com.avos.avoscloud.Messages.SessionCommand result = new com.avos.avoscloud.Messages.SessionCommand(this);
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (((from_bitField0_ & 0x00000001) == 0x00000001)) {
to_bitField0_ |= 0x00000001;
}
result.t_ = t_;
if (((from_bitField0_ & 0x00000002) == 0x00000002)) {
to_bitField0_ |= 0x00000002;
}
result.n_ = n_;
if (((from_bitField0_ & 0x00000004) == 0x00000004)) {
to_bitField0_ |= 0x00000004;
}
result.s_ = s_;
if (((from_bitField0_ & 0x00000008) == 0x00000008)) {
to_bitField0_ |= 0x00000008;
}
result.ua_ = ua_;
if (((from_bitField0_ & 0x00000010) == 0x00000010)) {
to_bitField0_ |= 0x00000010;
}
result.r_ = r_;
if (((from_bitField0_ & 0x00000020) == 0x00000020)) {
to_bitField0_ |= 0x00000020;
}
result.tag_ = tag_;
if (((from_bitField0_ & 0x00000040) == 0x00000040)) {
to_bitField0_ |= 0x00000040;
}
result.deviceId_ = deviceId_;
if (((bitField0_ & 0x00000080) == 0x00000080)) {
sessionPeerIds_ = sessionPeerIds_.getUnmodifiableView();
bitField0_ = (bitField0_ & ~0x00000080);
}
result.sessionPeerIds_ = sessionPeerIds_;
if (((bitField0_ & 0x00000100) == 0x00000100)) {
onlineSessionPeerIds_ = onlineSessionPeerIds_.getUnmodifiableView();
bitField0_ = (bitField0_ & ~0x00000100);
}
result.onlineSessionPeerIds_ = onlineSessionPeerIds_;
if (((from_bitField0_ & 0x00000200) == 0x00000200)) {
to_bitField0_ |= 0x00000080;
}
result.st_ = st_;
if (((from_bitField0_ & 0x00000400) == 0x00000400)) {
to_bitField0_ |= 0x00000100;
}
result.stTtl_ = stTtl_;
if (((from_bitField0_ & 0x00000800) == 0x00000800)) {
to_bitField0_ |= 0x00000200;
}
result.code_ = code_;
if (((from_bitField0_ & 0x00001000) == 0x00001000)) {
to_bitField0_ |= 0x00000400;
}
result.reason_ = reason_;
if (((from_bitField0_ & 0x00002000) == 0x00002000)) {
to_bitField0_ |= 0x00000800;
}
result.deviceToken_ = deviceToken_;
if (((from_bitField0_ & 0x00004000) == 0x00004000)) {
to_bitField0_ |= 0x00001000;
}
result.sp_ = sp_;
if (((from_bitField0_ & 0x00008000) == 0x00008000)) {
to_bitField0_ |= 0x00002000;
}
result.detail_ = detail_;
if (((from_bitField0_ & 0x00010000) == 0x00010000)) {
to_bitField0_ |= 0x00004000;
}
result.lastUnreadNotifTime_ = lastUnreadNotifTime_;
if (((from_bitField0_ & 0x00020000) == 0x00020000)) {
to_bitField0_ |= 0x00008000;
}
result.lastPatchTime_ = lastPatchTime_;
if (((from_bitField0_ & 0x00040000) == 0x00040000)) {
to_bitField0_ |= 0x00010000;
}
result.configBitmap_ = configBitmap_;
result.bitField0_ = to_bitField0_;
onBuilt();
return result;
}
public Builder clone() {
return (Builder) super.clone();
}
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.setField(field, value);
}
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return (Builder) super.clearField(field);
}
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return (Builder) super.clearOneof(oneof);
}
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return (Builder) super.setRepeatedField(field, index, value);
}
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.addRepeatedField(field, value);
}
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.avos.avoscloud.Messages.SessionCommand) {
return mergeFrom((com.avos.avoscloud.Messages.SessionCommand)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.avos.avoscloud.Messages.SessionCommand other) {
if (other == com.avos.avoscloud.Messages.SessionCommand.getDefaultInstance()) return this;
if (other.hasT()) {
setT(other.getT());
}
if (other.hasN()) {
bitField0_ |= 0x00000002;
n_ = other.n_;
onChanged();
}
if (other.hasS()) {
bitField0_ |= 0x00000004;
s_ = other.s_;
onChanged();
}
if (other.hasUa()) {
bitField0_ |= 0x00000008;
ua_ = other.ua_;
onChanged();
}
if (other.hasR()) {
setR(other.getR());
}
if (other.hasTag()) {
bitField0_ |= 0x00000020;
tag_ = other.tag_;
onChanged();
}
if (other.hasDeviceId()) {
bitField0_ |= 0x00000040;
deviceId_ = other.deviceId_;
onChanged();
}
if (!other.sessionPeerIds_.isEmpty()) {
if (sessionPeerIds_.isEmpty()) {
sessionPeerIds_ = other.sessionPeerIds_;
bitField0_ = (bitField0_ & ~0x00000080);
} else {
ensureSessionPeerIdsIsMutable();
sessionPeerIds_.addAll(other.sessionPeerIds_);
}
onChanged();
}
if (!other.onlineSessionPeerIds_.isEmpty()) {
if (onlineSessionPeerIds_.isEmpty()) {
onlineSessionPeerIds_ = other.onlineSessionPeerIds_;
bitField0_ = (bitField0_ & ~0x00000100);
} else {
ensureOnlineSessionPeerIdsIsMutable();
onlineSessionPeerIds_.addAll(other.onlineSessionPeerIds_);
}
onChanged();
}
if (other.hasSt()) {
bitField0_ |= 0x00000200;
st_ = other.st_;
onChanged();
}
if (other.hasStTtl()) {
setStTtl(other.getStTtl());
}
if (other.hasCode()) {
setCode(other.getCode());
}
if (other.hasReason()) {
bitField0_ |= 0x00001000;
reason_ = other.reason_;
onChanged();
}
if (other.hasDeviceToken()) {
bitField0_ |= 0x00002000;
deviceToken_ = other.deviceToken_;
onChanged();
}
if (other.hasSp()) {
setSp(other.getSp());
}
if (other.hasDetail()) {
bitField0_ |= 0x00008000;
detail_ = other.detail_;
onChanged();
}
if (other.hasLastUnreadNotifTime()) {
setLastUnreadNotifTime(other.getLastUnreadNotifTime());
}
if (other.hasLastPatchTime()) {
setLastPatchTime(other.getLastPatchTime());
}
if (other.hasConfigBitmap()) {
setConfigBitmap(other.getConfigBitmap());
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
public final boolean isInitialized() {
return true;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
com.avos.avoscloud.Messages.SessionCommand parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (com.avos.avoscloud.Messages.SessionCommand) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
private long t_ ;
/**
* optional int64 t = 1;
*/
public boolean hasT() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
* optional int64 t = 1;
*/
public long getT() {
return t_;
}
/**
* optional int64 t = 1;
*/
public Builder setT(long value) {
bitField0_ |= 0x00000001;
t_ = value;
onChanged();
return this;
}
/**
* optional int64 t = 1;
*/
public Builder clearT() {
bitField0_ = (bitField0_ & ~0x00000001);
t_ = 0L;
onChanged();
return this;
}
private java.lang.Object n_ = "";
/**
* optional string n = 2;
*/
public boolean hasN() {
return ((bitField0_ & 0x00000002) == 0x00000002);
}
/**
* optional string n = 2;
*/
public java.lang.String getN() {
java.lang.Object ref = n_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
n_ = s;
}
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* optional string n = 2;
*/
public com.google.protobuf.ByteString
getNBytes() {
java.lang.Object ref = n_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
n_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* optional string n = 2;
*/
public Builder setN(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000002;
n_ = value;
onChanged();
return this;
}
/**
* optional string n = 2;
*/
public Builder clearN() {
bitField0_ = (bitField0_ & ~0x00000002);
n_ = getDefaultInstance().getN();
onChanged();
return this;
}
/**
* optional string n = 2;
*/
public Builder setNBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000002;
n_ = value;
onChanged();
return this;
}
private java.lang.Object s_ = "";
/**
* optional string s = 3;
*/
public boolean hasS() {
return ((bitField0_ & 0x00000004) == 0x00000004);
}
/**
* optional string s = 3;
*/
public java.lang.String getS() {
java.lang.Object ref = s_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
s_ = s;
}
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* optional string s = 3;
*/
public com.google.protobuf.ByteString
getSBytes() {
java.lang.Object ref = s_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
s_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* optional string s = 3;
*/
public Builder setS(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000004;
s_ = value;
onChanged();
return this;
}
/**
* optional string s = 3;
*/
public Builder clearS() {
bitField0_ = (bitField0_ & ~0x00000004);
s_ = getDefaultInstance().getS();
onChanged();
return this;
}
/**
* optional string s = 3;
*/
public Builder setSBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000004;
s_ = value;
onChanged();
return this;
}
private java.lang.Object ua_ = "";
/**
* optional string ua = 4;
*/
public boolean hasUa() {
return ((bitField0_ & 0x00000008) == 0x00000008);
}
/**
* optional string ua = 4;
*/
public java.lang.String getUa() {
java.lang.Object ref = ua_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
ua_ = s;
}
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* optional string ua = 4;
*/
public com.google.protobuf.ByteString
getUaBytes() {
java.lang.Object ref = ua_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
ua_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* optional string ua = 4;
*/
public Builder setUa(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000008;
ua_ = value;
onChanged();
return this;
}
/**
* optional string ua = 4;
*/
public Builder clearUa() {
bitField0_ = (bitField0_ & ~0x00000008);
ua_ = getDefaultInstance().getUa();
onChanged();
return this;
}
/**
* optional string ua = 4;
*/
public Builder setUaBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000008;
ua_ = value;
onChanged();
return this;
}
private boolean r_ ;
/**
* optional bool r = 5;
*/
public boolean hasR() {
return ((bitField0_ & 0x00000010) == 0x00000010);
}
/**
* optional bool r = 5;
*/
public boolean getR() {
return r_;
}
/**
* optional bool r = 5;
*/
public Builder setR(boolean value) {
bitField0_ |= 0x00000010;
r_ = value;
onChanged();
return this;
}
/**
* optional bool r = 5;
*/
public Builder clearR() {
bitField0_ = (bitField0_ & ~0x00000010);
r_ = false;
onChanged();
return this;
}
private java.lang.Object tag_ = "";
/**
* optional string tag = 6;
*/
public boolean hasTag() {
return ((bitField0_ & 0x00000020) == 0x00000020);
}
/**
* optional string tag = 6;
*/
public java.lang.String getTag() {
java.lang.Object ref = tag_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
tag_ = s;
}
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* optional string tag = 6;
*/
public com.google.protobuf.ByteString
getTagBytes() {
java.lang.Object ref = tag_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
tag_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* optional string tag = 6;
*/
public Builder setTag(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000020;
tag_ = value;
onChanged();
return this;
}
/**
* optional string tag = 6;
*/
public Builder clearTag() {
bitField0_ = (bitField0_ & ~0x00000020);
tag_ = getDefaultInstance().getTag();
onChanged();
return this;
}
/**
* optional string tag = 6;
*/
public Builder setTagBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000020;
tag_ = value;
onChanged();
return this;
}
private java.lang.Object deviceId_ = "";
/**
* optional string deviceId = 7;
*/
public boolean hasDeviceId() {
return ((bitField0_ & 0x00000040) == 0x00000040);
}
/**
* optional string deviceId = 7;
*/
public java.lang.String getDeviceId() {
java.lang.Object ref = deviceId_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
deviceId_ = s;
}
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* optional string deviceId = 7;
*/
public com.google.protobuf.ByteString
getDeviceIdBytes() {
java.lang.Object ref = deviceId_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
deviceId_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* optional string deviceId = 7;
*/
public Builder setDeviceId(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000040;
deviceId_ = value;
onChanged();
return this;
}
/**
* optional string deviceId = 7;
*/
public Builder clearDeviceId() {
bitField0_ = (bitField0_ & ~0x00000040);
deviceId_ = getDefaultInstance().getDeviceId();
onChanged();
return this;
}
/**
* optional string deviceId = 7;
*/
public Builder setDeviceIdBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000040;
deviceId_ = value;
onChanged();
return this;
}
private com.google.protobuf.LazyStringList sessionPeerIds_ = com.google.protobuf.LazyStringArrayList.EMPTY;
private void ensureSessionPeerIdsIsMutable() {
if (!((bitField0_ & 0x00000080) == 0x00000080)) {
sessionPeerIds_ = new com.google.protobuf.LazyStringArrayList(sessionPeerIds_);
bitField0_ |= 0x00000080;
}
}
/**
* repeated string sessionPeerIds = 8;
*/
public com.google.protobuf.ProtocolStringList
getSessionPeerIdsList() {
return sessionPeerIds_.getUnmodifiableView();
}
/**
* repeated string sessionPeerIds = 8;
*/
public int getSessionPeerIdsCount() {
return sessionPeerIds_.size();
}
/**
* repeated string sessionPeerIds = 8;
*/
public java.lang.String getSessionPeerIds(int index) {
return sessionPeerIds_.get(index);
}
/**
* repeated string sessionPeerIds = 8;
*/
public com.google.protobuf.ByteString
getSessionPeerIdsBytes(int index) {
return sessionPeerIds_.getByteString(index);
}
/**
* repeated string sessionPeerIds = 8;
*/
public Builder setSessionPeerIds(
int index, java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
ensureSessionPeerIdsIsMutable();
sessionPeerIds_.set(index, value);
onChanged();
return this;
}
/**
* repeated string sessionPeerIds = 8;
*/
public Builder addSessionPeerIds(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
ensureSessionPeerIdsIsMutable();
sessionPeerIds_.add(value);
onChanged();
return this;
}
/**
* repeated string sessionPeerIds = 8;
*/
public Builder addAllSessionPeerIds(
java.lang.Iterable values) {
ensureSessionPeerIdsIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, sessionPeerIds_);
onChanged();
return this;
}
/**
* repeated string sessionPeerIds = 8;
*/
public Builder clearSessionPeerIds() {
sessionPeerIds_ = com.google.protobuf.LazyStringArrayList.EMPTY;
bitField0_ = (bitField0_ & ~0x00000080);
onChanged();
return this;
}
/**
* repeated string sessionPeerIds = 8;
*/
public Builder addSessionPeerIdsBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
ensureSessionPeerIdsIsMutable();
sessionPeerIds_.add(value);
onChanged();
return this;
}
private com.google.protobuf.LazyStringList onlineSessionPeerIds_ = com.google.protobuf.LazyStringArrayList.EMPTY;
private void ensureOnlineSessionPeerIdsIsMutable() {
if (!((bitField0_ & 0x00000100) == 0x00000100)) {
onlineSessionPeerIds_ = new com.google.protobuf.LazyStringArrayList(onlineSessionPeerIds_);
bitField0_ |= 0x00000100;
}
}
/**
* repeated string onlineSessionPeerIds = 9;
*/
public com.google.protobuf.ProtocolStringList
getOnlineSessionPeerIdsList() {
return onlineSessionPeerIds_.getUnmodifiableView();
}
/**
* repeated string onlineSessionPeerIds = 9;
*/
public int getOnlineSessionPeerIdsCount() {
return onlineSessionPeerIds_.size();
}
/**
* repeated string onlineSessionPeerIds = 9;
*/
public java.lang.String getOnlineSessionPeerIds(int index) {
return onlineSessionPeerIds_.get(index);
}
/**
* repeated string onlineSessionPeerIds = 9;
*/
public com.google.protobuf.ByteString
getOnlineSessionPeerIdsBytes(int index) {
return onlineSessionPeerIds_.getByteString(index);
}
/**
* repeated string onlineSessionPeerIds = 9;
*/
public Builder setOnlineSessionPeerIds(
int index, java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
ensureOnlineSessionPeerIdsIsMutable();
onlineSessionPeerIds_.set(index, value);
onChanged();
return this;
}
/**
* repeated string onlineSessionPeerIds = 9;
*/
public Builder addOnlineSessionPeerIds(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
ensureOnlineSessionPeerIdsIsMutable();
onlineSessionPeerIds_.add(value);
onChanged();
return this;
}
/**
* repeated string onlineSessionPeerIds = 9;
*/
public Builder addAllOnlineSessionPeerIds(
java.lang.Iterable values) {
ensureOnlineSessionPeerIdsIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, onlineSessionPeerIds_);
onChanged();
return this;
}
/**
* repeated string onlineSessionPeerIds = 9;
*/
public Builder clearOnlineSessionPeerIds() {
onlineSessionPeerIds_ = com.google.protobuf.LazyStringArrayList.EMPTY;
bitField0_ = (bitField0_ & ~0x00000100);
onChanged();
return this;
}
/**
* repeated string onlineSessionPeerIds = 9;
*/
public Builder addOnlineSessionPeerIdsBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
ensureOnlineSessionPeerIdsIsMutable();
onlineSessionPeerIds_.add(value);
onChanged();
return this;
}
private java.lang.Object st_ = "";
/**
* optional string st = 10;
*/
public boolean hasSt() {
return ((bitField0_ & 0x00000200) == 0x00000200);
}
/**
* optional string st = 10;
*/
public java.lang.String getSt() {
java.lang.Object ref = st_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
st_ = s;
}
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* optional string st = 10;
*/
public com.google.protobuf.ByteString
getStBytes() {
java.lang.Object ref = st_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
st_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* optional string st = 10;
*/
public Builder setSt(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000200;
st_ = value;
onChanged();
return this;
}
/**
* optional string st = 10;
*/
public Builder clearSt() {
bitField0_ = (bitField0_ & ~0x00000200);
st_ = getDefaultInstance().getSt();
onChanged();
return this;
}
/**
* optional string st = 10;
*/
public Builder setStBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000200;
st_ = value;
onChanged();
return this;
}
private int stTtl_ ;
/**
* optional int32 stTtl = 11;
*/
public boolean hasStTtl() {
return ((bitField0_ & 0x00000400) == 0x00000400);
}
/**
* optional int32 stTtl = 11;
*/
public int getStTtl() {
return stTtl_;
}
/**
* optional int32 stTtl = 11;
*/
public Builder setStTtl(int value) {
bitField0_ |= 0x00000400;
stTtl_ = value;
onChanged();
return this;
}
/**
* optional int32 stTtl = 11;
*/
public Builder clearStTtl() {
bitField0_ = (bitField0_ & ~0x00000400);
stTtl_ = 0;
onChanged();
return this;
}
private int code_ ;
/**
* optional int32 code = 12;
*/
public boolean hasCode() {
return ((bitField0_ & 0x00000800) == 0x00000800);
}
/**
* optional int32 code = 12;
*/
public int getCode() {
return code_;
}
/**
* optional int32 code = 12;
*/
public Builder setCode(int value) {
bitField0_ |= 0x00000800;
code_ = value;
onChanged();
return this;
}
/**
* optional int32 code = 12;
*/
public Builder clearCode() {
bitField0_ = (bitField0_ & ~0x00000800);
code_ = 0;
onChanged();
return this;
}
private java.lang.Object reason_ = "";
/**
* optional string reason = 13;
*/
public boolean hasReason() {
return ((bitField0_ & 0x00001000) == 0x00001000);
}
/**
* optional string reason = 13;
*/
public java.lang.String getReason() {
java.lang.Object ref = reason_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
reason_ = s;
}
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* optional string reason = 13;
*/
public com.google.protobuf.ByteString
getReasonBytes() {
java.lang.Object ref = reason_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
reason_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* optional string reason = 13;
*/
public Builder setReason(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00001000;
reason_ = value;
onChanged();
return this;
}
/**
* optional string reason = 13;
*/
public Builder clearReason() {
bitField0_ = (bitField0_ & ~0x00001000);
reason_ = getDefaultInstance().getReason();
onChanged();
return this;
}
/**
* optional string reason = 13;
*/
public Builder setReasonBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00001000;
reason_ = value;
onChanged();
return this;
}
private java.lang.Object deviceToken_ = "";
/**
* optional string deviceToken = 14;
*/
public boolean hasDeviceToken() {
return ((bitField0_ & 0x00002000) == 0x00002000);
}
/**
* optional string deviceToken = 14;
*/
public java.lang.String getDeviceToken() {
java.lang.Object ref = deviceToken_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
deviceToken_ = s;
}
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* optional string deviceToken = 14;
*/
public com.google.protobuf.ByteString
getDeviceTokenBytes() {
java.lang.Object ref = deviceToken_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
deviceToken_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* optional string deviceToken = 14;
*/
public Builder setDeviceToken(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00002000;
deviceToken_ = value;
onChanged();
return this;
}
/**
* optional string deviceToken = 14;
*/
public Builder clearDeviceToken() {
bitField0_ = (bitField0_ & ~0x00002000);
deviceToken_ = getDefaultInstance().getDeviceToken();
onChanged();
return this;
}
/**
* optional string deviceToken = 14;
*/
public Builder setDeviceTokenBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00002000;
deviceToken_ = value;
onChanged();
return this;
}
private boolean sp_ ;
/**
* optional bool sp = 15;
*/
public boolean hasSp() {
return ((bitField0_ & 0x00004000) == 0x00004000);
}
/**
* optional bool sp = 15;
*/
public boolean getSp() {
return sp_;
}
/**
* optional bool sp = 15;
*/
public Builder setSp(boolean value) {
bitField0_ |= 0x00004000;
sp_ = value;
onChanged();
return this;
}
/**
* optional bool sp = 15;
*/
public Builder clearSp() {
bitField0_ = (bitField0_ & ~0x00004000);
sp_ = false;
onChanged();
return this;
}
private java.lang.Object detail_ = "";
/**
* optional string detail = 16;
*/
public boolean hasDetail() {
return ((bitField0_ & 0x00008000) == 0x00008000);
}
/**
* optional string detail = 16;
*/
public java.lang.String getDetail() {
java.lang.Object ref = detail_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
detail_ = s;
}
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* optional string detail = 16;
*/
public com.google.protobuf.ByteString
getDetailBytes() {
java.lang.Object ref = detail_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
detail_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* optional string detail = 16;
*/
public Builder setDetail(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00008000;
detail_ = value;
onChanged();
return this;
}
/**
* optional string detail = 16;
*/
public Builder clearDetail() {
bitField0_ = (bitField0_ & ~0x00008000);
detail_ = getDefaultInstance().getDetail();
onChanged();
return this;
}
/**
* optional string detail = 16;
*/
public Builder setDetailBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00008000;
detail_ = value;
onChanged();
return this;
}
private long lastUnreadNotifTime_ ;
/**
* optional int64 lastUnreadNotifTime = 17;
*/
public boolean hasLastUnreadNotifTime() {
return ((bitField0_ & 0x00010000) == 0x00010000);
}
/**
* optional int64 lastUnreadNotifTime = 17;
*/
public long getLastUnreadNotifTime() {
return lastUnreadNotifTime_;
}
/**
* optional int64 lastUnreadNotifTime = 17;
*/
public Builder setLastUnreadNotifTime(long value) {
bitField0_ |= 0x00010000;
lastUnreadNotifTime_ = value;
onChanged();
return this;
}
/**
* optional int64 lastUnreadNotifTime = 17;
*/
public Builder clearLastUnreadNotifTime() {
bitField0_ = (bitField0_ & ~0x00010000);
lastUnreadNotifTime_ = 0L;
onChanged();
return this;
}
private long lastPatchTime_ ;
/**
* optional int64 lastPatchTime = 18;
*/
public boolean hasLastPatchTime() {
return ((bitField0_ & 0x00020000) == 0x00020000);
}
/**
* optional int64 lastPatchTime = 18;
*/
public long getLastPatchTime() {
return lastPatchTime_;
}
/**
* optional int64 lastPatchTime = 18;
*/
public Builder setLastPatchTime(long value) {
bitField0_ |= 0x00020000;
lastPatchTime_ = value;
onChanged();
return this;
}
/**
* optional int64 lastPatchTime = 18;
*/
public Builder clearLastPatchTime() {
bitField0_ = (bitField0_ & ~0x00020000);
lastPatchTime_ = 0L;
onChanged();
return this;
}
private long configBitmap_ ;
/**
* optional int64 configBitmap = 19;
*/
public boolean hasConfigBitmap() {
return ((bitField0_ & 0x00040000) == 0x00040000);
}
/**
* optional int64 configBitmap = 19;
*/
public long getConfigBitmap() {
return configBitmap_;
}
/**
* optional int64 configBitmap = 19;
*/
public Builder setConfigBitmap(long value) {
bitField0_ |= 0x00040000;
configBitmap_ = value;
onChanged();
return this;
}
/**
* optional int64 configBitmap = 19;
*/
public Builder clearConfigBitmap() {
bitField0_ = (bitField0_ & ~0x00040000);
configBitmap_ = 0L;
onChanged();
return this;
}
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:com.avos.avoscloud.SessionCommand)
}
// @@protoc_insertion_point(class_scope:com.avos.avoscloud.SessionCommand)
private static final com.avos.avoscloud.Messages.SessionCommand DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new com.avos.avoscloud.Messages.SessionCommand();
}
public static com.avos.avoscloud.Messages.SessionCommand getDefaultInstance() {
return DEFAULT_INSTANCE;
}
@java.lang.Deprecated public static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
public SessionCommand parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new SessionCommand(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
public com.avos.avoscloud.Messages.SessionCommand getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface ErrorCommandOrBuilder extends
// @@protoc_insertion_point(interface_extends:com.avos.avoscloud.ErrorCommand)
com.google.protobuf.MessageOrBuilder {
/**
* required int32 code = 1;
*/
boolean hasCode();
/**
* required int32 code = 1;
*/
int getCode();
/**
* required string reason = 2;
*/
boolean hasReason();
/**
* required string reason = 2;
*/
java.lang.String getReason();
/**
* required string reason = 2;
*/
com.google.protobuf.ByteString
getReasonBytes();
/**
* optional int32 appCode = 3;
*/
boolean hasAppCode();
/**
* optional int32 appCode = 3;
*/
int getAppCode();
/**
* optional string detail = 4;
*/
boolean hasDetail();
/**
* optional string detail = 4;
*/
java.lang.String getDetail();
/**
* optional string detail = 4;
*/
com.google.protobuf.ByteString
getDetailBytes();
/**
* repeated string pids = 5;
*/
java.util.List
getPidsList();
/**
* repeated string pids = 5;
*/
int getPidsCount();
/**
* repeated string pids = 5;
*/
java.lang.String getPids(int index);
/**
* repeated string pids = 5;
*/
com.google.protobuf.ByteString
getPidsBytes(int index);
/**
* optional string appMsg = 6;
*/
boolean hasAppMsg();
/**
* optional string appMsg = 6;
*/
java.lang.String getAppMsg();
/**
* optional string appMsg = 6;
*/
com.google.protobuf.ByteString
getAppMsgBytes();
}
/**
* Protobuf type {@code com.avos.avoscloud.ErrorCommand}
*/
public static final class ErrorCommand extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:com.avos.avoscloud.ErrorCommand)
ErrorCommandOrBuilder {
private static final long serialVersionUID = 0L;
// Use ErrorCommand.newBuilder() to construct.
private ErrorCommand(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private ErrorCommand() {
code_ = 0;
reason_ = "";
appCode_ = 0;
detail_ = "";
pids_ = com.google.protobuf.LazyStringArrayList.EMPTY;
appMsg_ = "";
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private ErrorCommand(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!parseUnknownField(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
case 8: {
bitField0_ |= 0x00000001;
code_ = input.readInt32();
break;
}
case 18: {
com.google.protobuf.ByteString bs = input.readBytes();
bitField0_ |= 0x00000002;
reason_ = bs;
break;
}
case 24: {
bitField0_ |= 0x00000004;
appCode_ = input.readInt32();
break;
}
case 34: {
com.google.protobuf.ByteString bs = input.readBytes();
bitField0_ |= 0x00000008;
detail_ = bs;
break;
}
case 42: {
com.google.protobuf.ByteString bs = input.readBytes();
if (!((mutable_bitField0_ & 0x00000010) == 0x00000010)) {
pids_ = new com.google.protobuf.LazyStringArrayList();
mutable_bitField0_ |= 0x00000010;
}
pids_.add(bs);
break;
}
case 50: {
com.google.protobuf.ByteString bs = input.readBytes();
bitField0_ |= 0x00000010;
appMsg_ = bs;
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
if (((mutable_bitField0_ & 0x00000010) == 0x00000010)) {
pids_ = pids_.getUnmodifiableView();
}
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.avos.avoscloud.Messages.internal_static_com_avos_avoscloud_ErrorCommand_descriptor;
}
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.avos.avoscloud.Messages.internal_static_com_avos_avoscloud_ErrorCommand_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.avos.avoscloud.Messages.ErrorCommand.class, com.avos.avoscloud.Messages.ErrorCommand.Builder.class);
}
private int bitField0_;
public static final int CODE_FIELD_NUMBER = 1;
private int code_;
/**
* required int32 code = 1;
*/
public boolean hasCode() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
* required int32 code = 1;
*/
public int getCode() {
return code_;
}
public static final int REASON_FIELD_NUMBER = 2;
private volatile java.lang.Object reason_;
/**
* required string reason = 2;
*/
public boolean hasReason() {
return ((bitField0_ & 0x00000002) == 0x00000002);
}
/**
* required string reason = 2;
*/
public java.lang.String getReason() {
java.lang.Object ref = reason_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
reason_ = s;
}
return s;
}
}
/**
* required string reason = 2;
*/
public com.google.protobuf.ByteString
getReasonBytes() {
java.lang.Object ref = reason_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
reason_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int APPCODE_FIELD_NUMBER = 3;
private int appCode_;
/**
* optional int32 appCode = 3;
*/
public boolean hasAppCode() {
return ((bitField0_ & 0x00000004) == 0x00000004);
}
/**
* optional int32 appCode = 3;
*/
public int getAppCode() {
return appCode_;
}
public static final int DETAIL_FIELD_NUMBER = 4;
private volatile java.lang.Object detail_;
/**
* optional string detail = 4;
*/
public boolean hasDetail() {
return ((bitField0_ & 0x00000008) == 0x00000008);
}
/**
* optional string detail = 4;
*/
public java.lang.String getDetail() {
java.lang.Object ref = detail_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
detail_ = s;
}
return s;
}
}
/**
* optional string detail = 4;
*/
public com.google.protobuf.ByteString
getDetailBytes() {
java.lang.Object ref = detail_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
detail_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int PIDS_FIELD_NUMBER = 5;
private com.google.protobuf.LazyStringList pids_;
/**
* repeated string pids = 5;
*/
public com.google.protobuf.ProtocolStringList
getPidsList() {
return pids_;
}
/**
* repeated string pids = 5;
*/
public int getPidsCount() {
return pids_.size();
}
/**
* repeated string pids = 5;
*/
public java.lang.String getPids(int index) {
return pids_.get(index);
}
/**
* repeated string pids = 5;
*/
public com.google.protobuf.ByteString
getPidsBytes(int index) {
return pids_.getByteString(index);
}
public static final int APPMSG_FIELD_NUMBER = 6;
private volatile java.lang.Object appMsg_;
/**
* optional string appMsg = 6;
*/
public boolean hasAppMsg() {
return ((bitField0_ & 0x00000010) == 0x00000010);
}
/**
* optional string appMsg = 6;
*/
public java.lang.String getAppMsg() {
java.lang.Object ref = appMsg_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
appMsg_ = s;
}
return s;
}
}
/**
* optional string appMsg = 6;
*/
public com.google.protobuf.ByteString
getAppMsgBytes() {
java.lang.Object ref = appMsg_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
appMsg_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
private byte memoizedIsInitialized = -1;
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
if (!hasCode()) {
memoizedIsInitialized = 0;
return false;
}
if (!hasReason()) {
memoizedIsInitialized = 0;
return false;
}
memoizedIsInitialized = 1;
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (((bitField0_ & 0x00000001) == 0x00000001)) {
output.writeInt32(1, code_);
}
if (((bitField0_ & 0x00000002) == 0x00000002)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 2, reason_);
}
if (((bitField0_ & 0x00000004) == 0x00000004)) {
output.writeInt32(3, appCode_);
}
if (((bitField0_ & 0x00000008) == 0x00000008)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 4, detail_);
}
for (int i = 0; i < pids_.size(); i++) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 5, pids_.getRaw(i));
}
if (((bitField0_ & 0x00000010) == 0x00000010)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 6, appMsg_);
}
unknownFields.writeTo(output);
}
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (((bitField0_ & 0x00000001) == 0x00000001)) {
size += com.google.protobuf.CodedOutputStream
.computeInt32Size(1, code_);
}
if (((bitField0_ & 0x00000002) == 0x00000002)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(2, reason_);
}
if (((bitField0_ & 0x00000004) == 0x00000004)) {
size += com.google.protobuf.CodedOutputStream
.computeInt32Size(3, appCode_);
}
if (((bitField0_ & 0x00000008) == 0x00000008)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(4, detail_);
}
{
int dataSize = 0;
for (int i = 0; i < pids_.size(); i++) {
dataSize += computeStringSizeNoTag(pids_.getRaw(i));
}
size += dataSize;
size += 1 * getPidsList().size();
}
if (((bitField0_ & 0x00000010) == 0x00000010)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(6, appMsg_);
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof com.avos.avoscloud.Messages.ErrorCommand)) {
return super.equals(obj);
}
com.avos.avoscloud.Messages.ErrorCommand other = (com.avos.avoscloud.Messages.ErrorCommand) obj;
boolean result = true;
result = result && (hasCode() == other.hasCode());
if (hasCode()) {
result = result && (getCode()
== other.getCode());
}
result = result && (hasReason() == other.hasReason());
if (hasReason()) {
result = result && getReason()
.equals(other.getReason());
}
result = result && (hasAppCode() == other.hasAppCode());
if (hasAppCode()) {
result = result && (getAppCode()
== other.getAppCode());
}
result = result && (hasDetail() == other.hasDetail());
if (hasDetail()) {
result = result && getDetail()
.equals(other.getDetail());
}
result = result && getPidsList()
.equals(other.getPidsList());
result = result && (hasAppMsg() == other.hasAppMsg());
if (hasAppMsg()) {
result = result && getAppMsg()
.equals(other.getAppMsg());
}
result = result && unknownFields.equals(other.unknownFields);
return result;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (hasCode()) {
hash = (37 * hash) + CODE_FIELD_NUMBER;
hash = (53 * hash) + getCode();
}
if (hasReason()) {
hash = (37 * hash) + REASON_FIELD_NUMBER;
hash = (53 * hash) + getReason().hashCode();
}
if (hasAppCode()) {
hash = (37 * hash) + APPCODE_FIELD_NUMBER;
hash = (53 * hash) + getAppCode();
}
if (hasDetail()) {
hash = (37 * hash) + DETAIL_FIELD_NUMBER;
hash = (53 * hash) + getDetail().hashCode();
}
if (getPidsCount() > 0) {
hash = (37 * hash) + PIDS_FIELD_NUMBER;
hash = (53 * hash) + getPidsList().hashCode();
}
if (hasAppMsg()) {
hash = (37 * hash) + APPMSG_FIELD_NUMBER;
hash = (53 * hash) + getAppMsg().hashCode();
}
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static com.avos.avoscloud.Messages.ErrorCommand parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.avos.avoscloud.Messages.ErrorCommand parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.avos.avoscloud.Messages.ErrorCommand parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.avos.avoscloud.Messages.ErrorCommand parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.avos.avoscloud.Messages.ErrorCommand parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.avos.avoscloud.Messages.ErrorCommand parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.avos.avoscloud.Messages.ErrorCommand parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.avos.avoscloud.Messages.ErrorCommand parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static com.avos.avoscloud.Messages.ErrorCommand parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static com.avos.avoscloud.Messages.ErrorCommand parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static com.avos.avoscloud.Messages.ErrorCommand parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.avos.avoscloud.Messages.ErrorCommand parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(com.avos.avoscloud.Messages.ErrorCommand prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code com.avos.avoscloud.ErrorCommand}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:com.avos.avoscloud.ErrorCommand)
com.avos.avoscloud.Messages.ErrorCommandOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.avos.avoscloud.Messages.internal_static_com_avos_avoscloud_ErrorCommand_descriptor;
}
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.avos.avoscloud.Messages.internal_static_com_avos_avoscloud_ErrorCommand_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.avos.avoscloud.Messages.ErrorCommand.class, com.avos.avoscloud.Messages.ErrorCommand.Builder.class);
}
// Construct using com.avos.avoscloud.Messages.ErrorCommand.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
public Builder clear() {
super.clear();
code_ = 0;
bitField0_ = (bitField0_ & ~0x00000001);
reason_ = "";
bitField0_ = (bitField0_ & ~0x00000002);
appCode_ = 0;
bitField0_ = (bitField0_ & ~0x00000004);
detail_ = "";
bitField0_ = (bitField0_ & ~0x00000008);
pids_ = com.google.protobuf.LazyStringArrayList.EMPTY;
bitField0_ = (bitField0_ & ~0x00000010);
appMsg_ = "";
bitField0_ = (bitField0_ & ~0x00000020);
return this;
}
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return com.avos.avoscloud.Messages.internal_static_com_avos_avoscloud_ErrorCommand_descriptor;
}
public com.avos.avoscloud.Messages.ErrorCommand getDefaultInstanceForType() {
return com.avos.avoscloud.Messages.ErrorCommand.getDefaultInstance();
}
public com.avos.avoscloud.Messages.ErrorCommand build() {
com.avos.avoscloud.Messages.ErrorCommand result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
public com.avos.avoscloud.Messages.ErrorCommand buildPartial() {
com.avos.avoscloud.Messages.ErrorCommand result = new com.avos.avoscloud.Messages.ErrorCommand(this);
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (((from_bitField0_ & 0x00000001) == 0x00000001)) {
to_bitField0_ |= 0x00000001;
}
result.code_ = code_;
if (((from_bitField0_ & 0x00000002) == 0x00000002)) {
to_bitField0_ |= 0x00000002;
}
result.reason_ = reason_;
if (((from_bitField0_ & 0x00000004) == 0x00000004)) {
to_bitField0_ |= 0x00000004;
}
result.appCode_ = appCode_;
if (((from_bitField0_ & 0x00000008) == 0x00000008)) {
to_bitField0_ |= 0x00000008;
}
result.detail_ = detail_;
if (((bitField0_ & 0x00000010) == 0x00000010)) {
pids_ = pids_.getUnmodifiableView();
bitField0_ = (bitField0_ & ~0x00000010);
}
result.pids_ = pids_;
if (((from_bitField0_ & 0x00000020) == 0x00000020)) {
to_bitField0_ |= 0x00000010;
}
result.appMsg_ = appMsg_;
result.bitField0_ = to_bitField0_;
onBuilt();
return result;
}
public Builder clone() {
return (Builder) super.clone();
}
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.setField(field, value);
}
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return (Builder) super.clearField(field);
}
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return (Builder) super.clearOneof(oneof);
}
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return (Builder) super.setRepeatedField(field, index, value);
}
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.addRepeatedField(field, value);
}
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.avos.avoscloud.Messages.ErrorCommand) {
return mergeFrom((com.avos.avoscloud.Messages.ErrorCommand)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.avos.avoscloud.Messages.ErrorCommand other) {
if (other == com.avos.avoscloud.Messages.ErrorCommand.getDefaultInstance()) return this;
if (other.hasCode()) {
setCode(other.getCode());
}
if (other.hasReason()) {
bitField0_ |= 0x00000002;
reason_ = other.reason_;
onChanged();
}
if (other.hasAppCode()) {
setAppCode(other.getAppCode());
}
if (other.hasDetail()) {
bitField0_ |= 0x00000008;
detail_ = other.detail_;
onChanged();
}
if (!other.pids_.isEmpty()) {
if (pids_.isEmpty()) {
pids_ = other.pids_;
bitField0_ = (bitField0_ & ~0x00000010);
} else {
ensurePidsIsMutable();
pids_.addAll(other.pids_);
}
onChanged();
}
if (other.hasAppMsg()) {
bitField0_ |= 0x00000020;
appMsg_ = other.appMsg_;
onChanged();
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
public final boolean isInitialized() {
if (!hasCode()) {
return false;
}
if (!hasReason()) {
return false;
}
return true;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
com.avos.avoscloud.Messages.ErrorCommand parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (com.avos.avoscloud.Messages.ErrorCommand) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
private int code_ ;
/**
* required int32 code = 1;
*/
public boolean hasCode() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
* required int32 code = 1;
*/
public int getCode() {
return code_;
}
/**
* required int32 code = 1;
*/
public Builder setCode(int value) {
bitField0_ |= 0x00000001;
code_ = value;
onChanged();
return this;
}
/**
* required int32 code = 1;
*/
public Builder clearCode() {
bitField0_ = (bitField0_ & ~0x00000001);
code_ = 0;
onChanged();
return this;
}
private java.lang.Object reason_ = "";
/**
* required string reason = 2;
*/
public boolean hasReason() {
return ((bitField0_ & 0x00000002) == 0x00000002);
}
/**
* required string reason = 2;
*/
public java.lang.String getReason() {
java.lang.Object ref = reason_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
reason_ = s;
}
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* required string reason = 2;
*/
public com.google.protobuf.ByteString
getReasonBytes() {
java.lang.Object ref = reason_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
reason_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* required string reason = 2;
*/
public Builder setReason(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000002;
reason_ = value;
onChanged();
return this;
}
/**
* required string reason = 2;
*/
public Builder clearReason() {
bitField0_ = (bitField0_ & ~0x00000002);
reason_ = getDefaultInstance().getReason();
onChanged();
return this;
}
/**
* required string reason = 2;
*/
public Builder setReasonBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000002;
reason_ = value;
onChanged();
return this;
}
private int appCode_ ;
/**
* optional int32 appCode = 3;
*/
public boolean hasAppCode() {
return ((bitField0_ & 0x00000004) == 0x00000004);
}
/**
* optional int32 appCode = 3;
*/
public int getAppCode() {
return appCode_;
}
/**
* optional int32 appCode = 3;
*/
public Builder setAppCode(int value) {
bitField0_ |= 0x00000004;
appCode_ = value;
onChanged();
return this;
}
/**
* optional int32 appCode = 3;
*/
public Builder clearAppCode() {
bitField0_ = (bitField0_ & ~0x00000004);
appCode_ = 0;
onChanged();
return this;
}
private java.lang.Object detail_ = "";
/**
* optional string detail = 4;
*/
public boolean hasDetail() {
return ((bitField0_ & 0x00000008) == 0x00000008);
}
/**
* optional string detail = 4;
*/
public java.lang.String getDetail() {
java.lang.Object ref = detail_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
detail_ = s;
}
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* optional string detail = 4;
*/
public com.google.protobuf.ByteString
getDetailBytes() {
java.lang.Object ref = detail_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
detail_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* optional string detail = 4;
*/
public Builder setDetail(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000008;
detail_ = value;
onChanged();
return this;
}
/**
* optional string detail = 4;
*/
public Builder clearDetail() {
bitField0_ = (bitField0_ & ~0x00000008);
detail_ = getDefaultInstance().getDetail();
onChanged();
return this;
}
/**
* optional string detail = 4;
*/
public Builder setDetailBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000008;
detail_ = value;
onChanged();
return this;
}
private com.google.protobuf.LazyStringList pids_ = com.google.protobuf.LazyStringArrayList.EMPTY;
private void ensurePidsIsMutable() {
if (!((bitField0_ & 0x00000010) == 0x00000010)) {
pids_ = new com.google.protobuf.LazyStringArrayList(pids_);
bitField0_ |= 0x00000010;
}
}
/**
* repeated string pids = 5;
*/
public com.google.protobuf.ProtocolStringList
getPidsList() {
return pids_.getUnmodifiableView();
}
/**
* repeated string pids = 5;
*/
public int getPidsCount() {
return pids_.size();
}
/**
* repeated string pids = 5;
*/
public java.lang.String getPids(int index) {
return pids_.get(index);
}
/**
* repeated string pids = 5;
*/
public com.google.protobuf.ByteString
getPidsBytes(int index) {
return pids_.getByteString(index);
}
/**
* repeated string pids = 5;
*/
public Builder setPids(
int index, java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
ensurePidsIsMutable();
pids_.set(index, value);
onChanged();
return this;
}
/**
* repeated string pids = 5;
*/
public Builder addPids(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
ensurePidsIsMutable();
pids_.add(value);
onChanged();
return this;
}
/**
* repeated string pids = 5;
*/
public Builder addAllPids(
java.lang.Iterable values) {
ensurePidsIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, pids_);
onChanged();
return this;
}
/**
* repeated string pids = 5;
*/
public Builder clearPids() {
pids_ = com.google.protobuf.LazyStringArrayList.EMPTY;
bitField0_ = (bitField0_ & ~0x00000010);
onChanged();
return this;
}
/**
* repeated string pids = 5;
*/
public Builder addPidsBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
ensurePidsIsMutable();
pids_.add(value);
onChanged();
return this;
}
private java.lang.Object appMsg_ = "";
/**
* optional string appMsg = 6;
*/
public boolean hasAppMsg() {
return ((bitField0_ & 0x00000020) == 0x00000020);
}
/**
* optional string appMsg = 6;
*/
public java.lang.String getAppMsg() {
java.lang.Object ref = appMsg_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
appMsg_ = s;
}
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* optional string appMsg = 6;
*/
public com.google.protobuf.ByteString
getAppMsgBytes() {
java.lang.Object ref = appMsg_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
appMsg_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* optional string appMsg = 6;
*/
public Builder setAppMsg(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000020;
appMsg_ = value;
onChanged();
return this;
}
/**
* optional string appMsg = 6;
*/
public Builder clearAppMsg() {
bitField0_ = (bitField0_ & ~0x00000020);
appMsg_ = getDefaultInstance().getAppMsg();
onChanged();
return this;
}
/**
* optional string appMsg = 6;
*/
public Builder setAppMsgBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000020;
appMsg_ = value;
onChanged();
return this;
}
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:com.avos.avoscloud.ErrorCommand)
}
// @@protoc_insertion_point(class_scope:com.avos.avoscloud.ErrorCommand)
private static final com.avos.avoscloud.Messages.ErrorCommand DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new com.avos.avoscloud.Messages.ErrorCommand();
}
public static com.avos.avoscloud.Messages.ErrorCommand getDefaultInstance() {
return DEFAULT_INSTANCE;
}
@java.lang.Deprecated public static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
public ErrorCommand parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new ErrorCommand(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
public com.avos.avoscloud.Messages.ErrorCommand getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface DirectCommandOrBuilder extends
// @@protoc_insertion_point(interface_extends:com.avos.avoscloud.DirectCommand)
com.google.protobuf.MessageOrBuilder {
/**
* optional string msg = 1;
*/
boolean hasMsg();
/**
* optional string msg = 1;
*/
java.lang.String getMsg();
/**
* optional string msg = 1;
*/
com.google.protobuf.ByteString
getMsgBytes();
/**
* optional string uid = 2;
*/
boolean hasUid();
/**
* optional string uid = 2;
*/
java.lang.String getUid();
/**
* optional string uid = 2;
*/
com.google.protobuf.ByteString
getUidBytes();
/**
* optional string fromPeerId = 3;
*/
boolean hasFromPeerId();
/**
* optional string fromPeerId = 3;
*/
java.lang.String getFromPeerId();
/**
* optional string fromPeerId = 3;
*/
com.google.protobuf.ByteString
getFromPeerIdBytes();
/**
* optional int64 timestamp = 4;
*/
boolean hasTimestamp();
/**
* optional int64 timestamp = 4;
*/
long getTimestamp();
/**
* optional bool offline = 5;
*/
boolean hasOffline();
/**
* optional bool offline = 5;
*/
boolean getOffline();
/**
* optional bool hasMore = 6;
*/
boolean hasHasMore();
/**
* optional bool hasMore = 6;
*/
boolean getHasMore();
/**
* repeated string toPeerIds = 7;
*/
java.util.List
getToPeerIdsList();
/**
* repeated string toPeerIds = 7;
*/
int getToPeerIdsCount();
/**
* repeated string toPeerIds = 7;
*/
java.lang.String getToPeerIds(int index);
/**
* repeated string toPeerIds = 7;
*/
com.google.protobuf.ByteString
getToPeerIdsBytes(int index);
/**
* optional bool r = 10;
*/
boolean hasR();
/**
* optional bool r = 10;
*/
boolean getR();
/**
* optional string cid = 11;
*/
boolean hasCid();
/**
* optional string cid = 11;
*/
java.lang.String getCid();
/**
* optional string cid = 11;
*/
com.google.protobuf.ByteString
getCidBytes();
/**
* optional string id = 12;
*/
boolean hasId();
/**
* optional string id = 12;
*/
java.lang.String getId();
/**
* optional string id = 12;
*/
com.google.protobuf.ByteString
getIdBytes();
/**
* optional bool transient = 13;
*/
boolean hasTransient();
/**
* optional bool transient = 13;
*/
boolean getTransient();
/**
* optional string dt = 14;
*/
boolean hasDt();
/**
* optional string dt = 14;
*/
java.lang.String getDt();
/**
* optional string dt = 14;
*/
com.google.protobuf.ByteString
getDtBytes();
/**
* optional string roomId = 15;
*/
boolean hasRoomId();
/**
* optional string roomId = 15;
*/
java.lang.String getRoomId();
/**
* optional string roomId = 15;
*/
com.google.protobuf.ByteString
getRoomIdBytes();
/**
* optional string pushData = 16;
*/
boolean hasPushData();
/**
* optional string pushData = 16;
*/
java.lang.String getPushData();
/**
* optional string pushData = 16;
*/
com.google.protobuf.ByteString
getPushDataBytes();
/**
* optional bool will = 17;
*/
boolean hasWill();
/**
* optional bool will = 17;
*/
boolean getWill();
/**
* optional int64 patchTimestamp = 18;
*/
boolean hasPatchTimestamp();
/**
* optional int64 patchTimestamp = 18;
*/
long getPatchTimestamp();
/**
* optional bytes binaryMsg = 19;
*/
boolean hasBinaryMsg();
/**
* optional bytes binaryMsg = 19;
*/
com.google.protobuf.ByteString getBinaryMsg();
/**
* repeated string mentionPids = 20;
*/
java.util.List
getMentionPidsList();
/**
* repeated string mentionPids = 20;
*/
int getMentionPidsCount();
/**
* repeated string mentionPids = 20;
*/
java.lang.String getMentionPids(int index);
/**
* repeated string mentionPids = 20;
*/
com.google.protobuf.ByteString
getMentionPidsBytes(int index);
/**
* optional bool mentionAll = 21;
*/
boolean hasMentionAll();
/**
* optional bool mentionAll = 21;
*/
boolean getMentionAll();
/**
* optional int32 convType = 22;
*/
boolean hasConvType();
/**
* optional int32 convType = 22;
*/
int getConvType();
}
/**
* Protobuf type {@code com.avos.avoscloud.DirectCommand}
*/
public static final class DirectCommand extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:com.avos.avoscloud.DirectCommand)
DirectCommandOrBuilder {
private static final long serialVersionUID = 0L;
// Use DirectCommand.newBuilder() to construct.
private DirectCommand(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private DirectCommand() {
msg_ = "";
uid_ = "";
fromPeerId_ = "";
timestamp_ = 0L;
offline_ = false;
hasMore_ = false;
toPeerIds_ = com.google.protobuf.LazyStringArrayList.EMPTY;
r_ = false;
cid_ = "";
id_ = "";
transient_ = false;
dt_ = "";
roomId_ = "";
pushData_ = "";
will_ = false;
patchTimestamp_ = 0L;
binaryMsg_ = com.google.protobuf.ByteString.EMPTY;
mentionPids_ = com.google.protobuf.LazyStringArrayList.EMPTY;
mentionAll_ = false;
convType_ = 0;
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private DirectCommand(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!parseUnknownField(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
case 10: {
com.google.protobuf.ByteString bs = input.readBytes();
bitField0_ |= 0x00000001;
msg_ = bs;
break;
}
case 18: {
com.google.protobuf.ByteString bs = input.readBytes();
bitField0_ |= 0x00000002;
uid_ = bs;
break;
}
case 26: {
com.google.protobuf.ByteString bs = input.readBytes();
bitField0_ |= 0x00000004;
fromPeerId_ = bs;
break;
}
case 32: {
bitField0_ |= 0x00000008;
timestamp_ = input.readInt64();
break;
}
case 40: {
bitField0_ |= 0x00000010;
offline_ = input.readBool();
break;
}
case 48: {
bitField0_ |= 0x00000020;
hasMore_ = input.readBool();
break;
}
case 58: {
com.google.protobuf.ByteString bs = input.readBytes();
if (!((mutable_bitField0_ & 0x00000040) == 0x00000040)) {
toPeerIds_ = new com.google.protobuf.LazyStringArrayList();
mutable_bitField0_ |= 0x00000040;
}
toPeerIds_.add(bs);
break;
}
case 80: {
bitField0_ |= 0x00000040;
r_ = input.readBool();
break;
}
case 90: {
com.google.protobuf.ByteString bs = input.readBytes();
bitField0_ |= 0x00000080;
cid_ = bs;
break;
}
case 98: {
com.google.protobuf.ByteString bs = input.readBytes();
bitField0_ |= 0x00000100;
id_ = bs;
break;
}
case 104: {
bitField0_ |= 0x00000200;
transient_ = input.readBool();
break;
}
case 114: {
com.google.protobuf.ByteString bs = input.readBytes();
bitField0_ |= 0x00000400;
dt_ = bs;
break;
}
case 122: {
com.google.protobuf.ByteString bs = input.readBytes();
bitField0_ |= 0x00000800;
roomId_ = bs;
break;
}
case 130: {
com.google.protobuf.ByteString bs = input.readBytes();
bitField0_ |= 0x00001000;
pushData_ = bs;
break;
}
case 136: {
bitField0_ |= 0x00002000;
will_ = input.readBool();
break;
}
case 144: {
bitField0_ |= 0x00004000;
patchTimestamp_ = input.readInt64();
break;
}
case 154: {
bitField0_ |= 0x00008000;
binaryMsg_ = input.readBytes();
break;
}
case 162: {
com.google.protobuf.ByteString bs = input.readBytes();
if (!((mutable_bitField0_ & 0x00020000) == 0x00020000)) {
mentionPids_ = new com.google.protobuf.LazyStringArrayList();
mutable_bitField0_ |= 0x00020000;
}
mentionPids_.add(bs);
break;
}
case 168: {
bitField0_ |= 0x00010000;
mentionAll_ = input.readBool();
break;
}
case 176: {
bitField0_ |= 0x00020000;
convType_ = input.readInt32();
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
if (((mutable_bitField0_ & 0x00000040) == 0x00000040)) {
toPeerIds_ = toPeerIds_.getUnmodifiableView();
}
if (((mutable_bitField0_ & 0x00020000) == 0x00020000)) {
mentionPids_ = mentionPids_.getUnmodifiableView();
}
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.avos.avoscloud.Messages.internal_static_com_avos_avoscloud_DirectCommand_descriptor;
}
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.avos.avoscloud.Messages.internal_static_com_avos_avoscloud_DirectCommand_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.avos.avoscloud.Messages.DirectCommand.class, com.avos.avoscloud.Messages.DirectCommand.Builder.class);
}
private int bitField0_;
public static final int MSG_FIELD_NUMBER = 1;
private volatile java.lang.Object msg_;
/**
* optional string msg = 1;
*/
public boolean hasMsg() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
* optional string msg = 1;
*/
public java.lang.String getMsg() {
java.lang.Object ref = msg_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
msg_ = s;
}
return s;
}
}
/**
* optional string msg = 1;
*/
public com.google.protobuf.ByteString
getMsgBytes() {
java.lang.Object ref = msg_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
msg_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int UID_FIELD_NUMBER = 2;
private volatile java.lang.Object uid_;
/**
* optional string uid = 2;
*/
public boolean hasUid() {
return ((bitField0_ & 0x00000002) == 0x00000002);
}
/**
* optional string uid = 2;
*/
public java.lang.String getUid() {
java.lang.Object ref = uid_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
uid_ = s;
}
return s;
}
}
/**
* optional string uid = 2;
*/
public com.google.protobuf.ByteString
getUidBytes() {
java.lang.Object ref = uid_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
uid_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int FROMPEERID_FIELD_NUMBER = 3;
private volatile java.lang.Object fromPeerId_;
/**
* optional string fromPeerId = 3;
*/
public boolean hasFromPeerId() {
return ((bitField0_ & 0x00000004) == 0x00000004);
}
/**
* optional string fromPeerId = 3;
*/
public java.lang.String getFromPeerId() {
java.lang.Object ref = fromPeerId_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
fromPeerId_ = s;
}
return s;
}
}
/**
* optional string fromPeerId = 3;
*/
public com.google.protobuf.ByteString
getFromPeerIdBytes() {
java.lang.Object ref = fromPeerId_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
fromPeerId_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int TIMESTAMP_FIELD_NUMBER = 4;
private long timestamp_;
/**
* optional int64 timestamp = 4;
*/
public boolean hasTimestamp() {
return ((bitField0_ & 0x00000008) == 0x00000008);
}
/**
* optional int64 timestamp = 4;
*/
public long getTimestamp() {
return timestamp_;
}
public static final int OFFLINE_FIELD_NUMBER = 5;
private boolean offline_;
/**
* optional bool offline = 5;
*/
public boolean hasOffline() {
return ((bitField0_ & 0x00000010) == 0x00000010);
}
/**
* optional bool offline = 5;
*/
public boolean getOffline() {
return offline_;
}
public static final int HASMORE_FIELD_NUMBER = 6;
private boolean hasMore_;
/**
* optional bool hasMore = 6;
*/
public boolean hasHasMore() {
return ((bitField0_ & 0x00000020) == 0x00000020);
}
/**
* optional bool hasMore = 6;
*/
public boolean getHasMore() {
return hasMore_;
}
public static final int TOPEERIDS_FIELD_NUMBER = 7;
private com.google.protobuf.LazyStringList toPeerIds_;
/**
* repeated string toPeerIds = 7;
*/
public com.google.protobuf.ProtocolStringList
getToPeerIdsList() {
return toPeerIds_;
}
/**
* repeated string toPeerIds = 7;
*/
public int getToPeerIdsCount() {
return toPeerIds_.size();
}
/**
* repeated string toPeerIds = 7;
*/
public java.lang.String getToPeerIds(int index) {
return toPeerIds_.get(index);
}
/**
* repeated string toPeerIds = 7;
*/
public com.google.protobuf.ByteString
getToPeerIdsBytes(int index) {
return toPeerIds_.getByteString(index);
}
public static final int R_FIELD_NUMBER = 10;
private boolean r_;
/**
* optional bool r = 10;
*/
public boolean hasR() {
return ((bitField0_ & 0x00000040) == 0x00000040);
}
/**
* optional bool r = 10;
*/
public boolean getR() {
return r_;
}
public static final int CID_FIELD_NUMBER = 11;
private volatile java.lang.Object cid_;
/**
* optional string cid = 11;
*/
public boolean hasCid() {
return ((bitField0_ & 0x00000080) == 0x00000080);
}
/**
* optional string cid = 11;
*/
public java.lang.String getCid() {
java.lang.Object ref = cid_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
cid_ = s;
}
return s;
}
}
/**
* optional string cid = 11;
*/
public com.google.protobuf.ByteString
getCidBytes() {
java.lang.Object ref = cid_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
cid_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int ID_FIELD_NUMBER = 12;
private volatile java.lang.Object id_;
/**
* optional string id = 12;
*/
public boolean hasId() {
return ((bitField0_ & 0x00000100) == 0x00000100);
}
/**
* optional string id = 12;
*/
public java.lang.String getId() {
java.lang.Object ref = id_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
id_ = s;
}
return s;
}
}
/**
* optional string id = 12;
*/
public com.google.protobuf.ByteString
getIdBytes() {
java.lang.Object ref = id_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
id_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int TRANSIENT_FIELD_NUMBER = 13;
private boolean transient_;
/**
* optional bool transient = 13;
*/
public boolean hasTransient() {
return ((bitField0_ & 0x00000200) == 0x00000200);
}
/**
* optional bool transient = 13;
*/
public boolean getTransient() {
return transient_;
}
public static final int DT_FIELD_NUMBER = 14;
private volatile java.lang.Object dt_;
/**
* optional string dt = 14;
*/
public boolean hasDt() {
return ((bitField0_ & 0x00000400) == 0x00000400);
}
/**
* optional string dt = 14;
*/
public java.lang.String getDt() {
java.lang.Object ref = dt_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
dt_ = s;
}
return s;
}
}
/**
* optional string dt = 14;
*/
public com.google.protobuf.ByteString
getDtBytes() {
java.lang.Object ref = dt_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
dt_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int ROOMID_FIELD_NUMBER = 15;
private volatile java.lang.Object roomId_;
/**
* optional string roomId = 15;
*/
public boolean hasRoomId() {
return ((bitField0_ & 0x00000800) == 0x00000800);
}
/**
* optional string roomId = 15;
*/
public java.lang.String getRoomId() {
java.lang.Object ref = roomId_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
roomId_ = s;
}
return s;
}
}
/**
* optional string roomId = 15;
*/
public com.google.protobuf.ByteString
getRoomIdBytes() {
java.lang.Object ref = roomId_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
roomId_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int PUSHDATA_FIELD_NUMBER = 16;
private volatile java.lang.Object pushData_;
/**
* optional string pushData = 16;
*/
public boolean hasPushData() {
return ((bitField0_ & 0x00001000) == 0x00001000);
}
/**
* optional string pushData = 16;
*/
public java.lang.String getPushData() {
java.lang.Object ref = pushData_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
pushData_ = s;
}
return s;
}
}
/**
* optional string pushData = 16;
*/
public com.google.protobuf.ByteString
getPushDataBytes() {
java.lang.Object ref = pushData_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
pushData_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int WILL_FIELD_NUMBER = 17;
private boolean will_;
/**
* optional bool will = 17;
*/
public boolean hasWill() {
return ((bitField0_ & 0x00002000) == 0x00002000);
}
/**
* optional bool will = 17;
*/
public boolean getWill() {
return will_;
}
public static final int PATCHTIMESTAMP_FIELD_NUMBER = 18;
private long patchTimestamp_;
/**
* optional int64 patchTimestamp = 18;
*/
public boolean hasPatchTimestamp() {
return ((bitField0_ & 0x00004000) == 0x00004000);
}
/**
* optional int64 patchTimestamp = 18;
*/
public long getPatchTimestamp() {
return patchTimestamp_;
}
public static final int BINARYMSG_FIELD_NUMBER = 19;
private com.google.protobuf.ByteString binaryMsg_;
/**
* optional bytes binaryMsg = 19;
*/
public boolean hasBinaryMsg() {
return ((bitField0_ & 0x00008000) == 0x00008000);
}
/**
* optional bytes binaryMsg = 19;
*/
public com.google.protobuf.ByteString getBinaryMsg() {
return binaryMsg_;
}
public static final int MENTIONPIDS_FIELD_NUMBER = 20;
private com.google.protobuf.LazyStringList mentionPids_;
/**
* repeated string mentionPids = 20;
*/
public com.google.protobuf.ProtocolStringList
getMentionPidsList() {
return mentionPids_;
}
/**
* repeated string mentionPids = 20;
*/
public int getMentionPidsCount() {
return mentionPids_.size();
}
/**
* repeated string mentionPids = 20;
*/
public java.lang.String getMentionPids(int index) {
return mentionPids_.get(index);
}
/**
* repeated string mentionPids = 20;
*/
public com.google.protobuf.ByteString
getMentionPidsBytes(int index) {
return mentionPids_.getByteString(index);
}
public static final int MENTIONALL_FIELD_NUMBER = 21;
private boolean mentionAll_;
/**
* optional bool mentionAll = 21;
*/
public boolean hasMentionAll() {
return ((bitField0_ & 0x00010000) == 0x00010000);
}
/**
* optional bool mentionAll = 21;
*/
public boolean getMentionAll() {
return mentionAll_;
}
public static final int CONVTYPE_FIELD_NUMBER = 22;
private int convType_;
/**
* optional int32 convType = 22;
*/
public boolean hasConvType() {
return ((bitField0_ & 0x00020000) == 0x00020000);
}
/**
* optional int32 convType = 22;
*/
public int getConvType() {
return convType_;
}
private byte memoizedIsInitialized = -1;
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (((bitField0_ & 0x00000001) == 0x00000001)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 1, msg_);
}
if (((bitField0_ & 0x00000002) == 0x00000002)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 2, uid_);
}
if (((bitField0_ & 0x00000004) == 0x00000004)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 3, fromPeerId_);
}
if (((bitField0_ & 0x00000008) == 0x00000008)) {
output.writeInt64(4, timestamp_);
}
if (((bitField0_ & 0x00000010) == 0x00000010)) {
output.writeBool(5, offline_);
}
if (((bitField0_ & 0x00000020) == 0x00000020)) {
output.writeBool(6, hasMore_);
}
for (int i = 0; i < toPeerIds_.size(); i++) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 7, toPeerIds_.getRaw(i));
}
if (((bitField0_ & 0x00000040) == 0x00000040)) {
output.writeBool(10, r_);
}
if (((bitField0_ & 0x00000080) == 0x00000080)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 11, cid_);
}
if (((bitField0_ & 0x00000100) == 0x00000100)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 12, id_);
}
if (((bitField0_ & 0x00000200) == 0x00000200)) {
output.writeBool(13, transient_);
}
if (((bitField0_ & 0x00000400) == 0x00000400)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 14, dt_);
}
if (((bitField0_ & 0x00000800) == 0x00000800)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 15, roomId_);
}
if (((bitField0_ & 0x00001000) == 0x00001000)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 16, pushData_);
}
if (((bitField0_ & 0x00002000) == 0x00002000)) {
output.writeBool(17, will_);
}
if (((bitField0_ & 0x00004000) == 0x00004000)) {
output.writeInt64(18, patchTimestamp_);
}
if (((bitField0_ & 0x00008000) == 0x00008000)) {
output.writeBytes(19, binaryMsg_);
}
for (int i = 0; i < mentionPids_.size(); i++) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 20, mentionPids_.getRaw(i));
}
if (((bitField0_ & 0x00010000) == 0x00010000)) {
output.writeBool(21, mentionAll_);
}
if (((bitField0_ & 0x00020000) == 0x00020000)) {
output.writeInt32(22, convType_);
}
unknownFields.writeTo(output);
}
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (((bitField0_ & 0x00000001) == 0x00000001)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(1, msg_);
}
if (((bitField0_ & 0x00000002) == 0x00000002)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(2, uid_);
}
if (((bitField0_ & 0x00000004) == 0x00000004)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(3, fromPeerId_);
}
if (((bitField0_ & 0x00000008) == 0x00000008)) {
size += com.google.protobuf.CodedOutputStream
.computeInt64Size(4, timestamp_);
}
if (((bitField0_ & 0x00000010) == 0x00000010)) {
size += com.google.protobuf.CodedOutputStream
.computeBoolSize(5, offline_);
}
if (((bitField0_ & 0x00000020) == 0x00000020)) {
size += com.google.protobuf.CodedOutputStream
.computeBoolSize(6, hasMore_);
}
{
int dataSize = 0;
for (int i = 0; i < toPeerIds_.size(); i++) {
dataSize += computeStringSizeNoTag(toPeerIds_.getRaw(i));
}
size += dataSize;
size += 1 * getToPeerIdsList().size();
}
if (((bitField0_ & 0x00000040) == 0x00000040)) {
size += com.google.protobuf.CodedOutputStream
.computeBoolSize(10, r_);
}
if (((bitField0_ & 0x00000080) == 0x00000080)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(11, cid_);
}
if (((bitField0_ & 0x00000100) == 0x00000100)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(12, id_);
}
if (((bitField0_ & 0x00000200) == 0x00000200)) {
size += com.google.protobuf.CodedOutputStream
.computeBoolSize(13, transient_);
}
if (((bitField0_ & 0x00000400) == 0x00000400)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(14, dt_);
}
if (((bitField0_ & 0x00000800) == 0x00000800)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(15, roomId_);
}
if (((bitField0_ & 0x00001000) == 0x00001000)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(16, pushData_);
}
if (((bitField0_ & 0x00002000) == 0x00002000)) {
size += com.google.protobuf.CodedOutputStream
.computeBoolSize(17, will_);
}
if (((bitField0_ & 0x00004000) == 0x00004000)) {
size += com.google.protobuf.CodedOutputStream
.computeInt64Size(18, patchTimestamp_);
}
if (((bitField0_ & 0x00008000) == 0x00008000)) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(19, binaryMsg_);
}
{
int dataSize = 0;
for (int i = 0; i < mentionPids_.size(); i++) {
dataSize += computeStringSizeNoTag(mentionPids_.getRaw(i));
}
size += dataSize;
size += 2 * getMentionPidsList().size();
}
if (((bitField0_ & 0x00010000) == 0x00010000)) {
size += com.google.protobuf.CodedOutputStream
.computeBoolSize(21, mentionAll_);
}
if (((bitField0_ & 0x00020000) == 0x00020000)) {
size += com.google.protobuf.CodedOutputStream
.computeInt32Size(22, convType_);
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof com.avos.avoscloud.Messages.DirectCommand)) {
return super.equals(obj);
}
com.avos.avoscloud.Messages.DirectCommand other = (com.avos.avoscloud.Messages.DirectCommand) obj;
boolean result = true;
result = result && (hasMsg() == other.hasMsg());
if (hasMsg()) {
result = result && getMsg()
.equals(other.getMsg());
}
result = result && (hasUid() == other.hasUid());
if (hasUid()) {
result = result && getUid()
.equals(other.getUid());
}
result = result && (hasFromPeerId() == other.hasFromPeerId());
if (hasFromPeerId()) {
result = result && getFromPeerId()
.equals(other.getFromPeerId());
}
result = result && (hasTimestamp() == other.hasTimestamp());
if (hasTimestamp()) {
result = result && (getTimestamp()
== other.getTimestamp());
}
result = result && (hasOffline() == other.hasOffline());
if (hasOffline()) {
result = result && (getOffline()
== other.getOffline());
}
result = result && (hasHasMore() == other.hasHasMore());
if (hasHasMore()) {
result = result && (getHasMore()
== other.getHasMore());
}
result = result && getToPeerIdsList()
.equals(other.getToPeerIdsList());
result = result && (hasR() == other.hasR());
if (hasR()) {
result = result && (getR()
== other.getR());
}
result = result && (hasCid() == other.hasCid());
if (hasCid()) {
result = result && getCid()
.equals(other.getCid());
}
result = result && (hasId() == other.hasId());
if (hasId()) {
result = result && getId()
.equals(other.getId());
}
result = result && (hasTransient() == other.hasTransient());
if (hasTransient()) {
result = result && (getTransient()
== other.getTransient());
}
result = result && (hasDt() == other.hasDt());
if (hasDt()) {
result = result && getDt()
.equals(other.getDt());
}
result = result && (hasRoomId() == other.hasRoomId());
if (hasRoomId()) {
result = result && getRoomId()
.equals(other.getRoomId());
}
result = result && (hasPushData() == other.hasPushData());
if (hasPushData()) {
result = result && getPushData()
.equals(other.getPushData());
}
result = result && (hasWill() == other.hasWill());
if (hasWill()) {
result = result && (getWill()
== other.getWill());
}
result = result && (hasPatchTimestamp() == other.hasPatchTimestamp());
if (hasPatchTimestamp()) {
result = result && (getPatchTimestamp()
== other.getPatchTimestamp());
}
result = result && (hasBinaryMsg() == other.hasBinaryMsg());
if (hasBinaryMsg()) {
result = result && getBinaryMsg()
.equals(other.getBinaryMsg());
}
result = result && getMentionPidsList()
.equals(other.getMentionPidsList());
result = result && (hasMentionAll() == other.hasMentionAll());
if (hasMentionAll()) {
result = result && (getMentionAll()
== other.getMentionAll());
}
result = result && (hasConvType() == other.hasConvType());
if (hasConvType()) {
result = result && (getConvType()
== other.getConvType());
}
result = result && unknownFields.equals(other.unknownFields);
return result;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (hasMsg()) {
hash = (37 * hash) + MSG_FIELD_NUMBER;
hash = (53 * hash) + getMsg().hashCode();
}
if (hasUid()) {
hash = (37 * hash) + UID_FIELD_NUMBER;
hash = (53 * hash) + getUid().hashCode();
}
if (hasFromPeerId()) {
hash = (37 * hash) + FROMPEERID_FIELD_NUMBER;
hash = (53 * hash) + getFromPeerId().hashCode();
}
if (hasTimestamp()) {
hash = (37 * hash) + TIMESTAMP_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getTimestamp());
}
if (hasOffline()) {
hash = (37 * hash) + OFFLINE_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashBoolean(
getOffline());
}
if (hasHasMore()) {
hash = (37 * hash) + HASMORE_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashBoolean(
getHasMore());
}
if (getToPeerIdsCount() > 0) {
hash = (37 * hash) + TOPEERIDS_FIELD_NUMBER;
hash = (53 * hash) + getToPeerIdsList().hashCode();
}
if (hasR()) {
hash = (37 * hash) + R_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashBoolean(
getR());
}
if (hasCid()) {
hash = (37 * hash) + CID_FIELD_NUMBER;
hash = (53 * hash) + getCid().hashCode();
}
if (hasId()) {
hash = (37 * hash) + ID_FIELD_NUMBER;
hash = (53 * hash) + getId().hashCode();
}
if (hasTransient()) {
hash = (37 * hash) + TRANSIENT_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashBoolean(
getTransient());
}
if (hasDt()) {
hash = (37 * hash) + DT_FIELD_NUMBER;
hash = (53 * hash) + getDt().hashCode();
}
if (hasRoomId()) {
hash = (37 * hash) + ROOMID_FIELD_NUMBER;
hash = (53 * hash) + getRoomId().hashCode();
}
if (hasPushData()) {
hash = (37 * hash) + PUSHDATA_FIELD_NUMBER;
hash = (53 * hash) + getPushData().hashCode();
}
if (hasWill()) {
hash = (37 * hash) + WILL_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashBoolean(
getWill());
}
if (hasPatchTimestamp()) {
hash = (37 * hash) + PATCHTIMESTAMP_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getPatchTimestamp());
}
if (hasBinaryMsg()) {
hash = (37 * hash) + BINARYMSG_FIELD_NUMBER;
hash = (53 * hash) + getBinaryMsg().hashCode();
}
if (getMentionPidsCount() > 0) {
hash = (37 * hash) + MENTIONPIDS_FIELD_NUMBER;
hash = (53 * hash) + getMentionPidsList().hashCode();
}
if (hasMentionAll()) {
hash = (37 * hash) + MENTIONALL_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashBoolean(
getMentionAll());
}
if (hasConvType()) {
hash = (37 * hash) + CONVTYPE_FIELD_NUMBER;
hash = (53 * hash) + getConvType();
}
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static com.avos.avoscloud.Messages.DirectCommand parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.avos.avoscloud.Messages.DirectCommand parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.avos.avoscloud.Messages.DirectCommand parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.avos.avoscloud.Messages.DirectCommand parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.avos.avoscloud.Messages.DirectCommand parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.avos.avoscloud.Messages.DirectCommand parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.avos.avoscloud.Messages.DirectCommand parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.avos.avoscloud.Messages.DirectCommand parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static com.avos.avoscloud.Messages.DirectCommand parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static com.avos.avoscloud.Messages.DirectCommand parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static com.avos.avoscloud.Messages.DirectCommand parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.avos.avoscloud.Messages.DirectCommand parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(com.avos.avoscloud.Messages.DirectCommand prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code com.avos.avoscloud.DirectCommand}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:com.avos.avoscloud.DirectCommand)
com.avos.avoscloud.Messages.DirectCommandOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.avos.avoscloud.Messages.internal_static_com_avos_avoscloud_DirectCommand_descriptor;
}
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.avos.avoscloud.Messages.internal_static_com_avos_avoscloud_DirectCommand_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.avos.avoscloud.Messages.DirectCommand.class, com.avos.avoscloud.Messages.DirectCommand.Builder.class);
}
// Construct using com.avos.avoscloud.Messages.DirectCommand.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
public Builder clear() {
super.clear();
msg_ = "";
bitField0_ = (bitField0_ & ~0x00000001);
uid_ = "";
bitField0_ = (bitField0_ & ~0x00000002);
fromPeerId_ = "";
bitField0_ = (bitField0_ & ~0x00000004);
timestamp_ = 0L;
bitField0_ = (bitField0_ & ~0x00000008);
offline_ = false;
bitField0_ = (bitField0_ & ~0x00000010);
hasMore_ = false;
bitField0_ = (bitField0_ & ~0x00000020);
toPeerIds_ = com.google.protobuf.LazyStringArrayList.EMPTY;
bitField0_ = (bitField0_ & ~0x00000040);
r_ = false;
bitField0_ = (bitField0_ & ~0x00000080);
cid_ = "";
bitField0_ = (bitField0_ & ~0x00000100);
id_ = "";
bitField0_ = (bitField0_ & ~0x00000200);
transient_ = false;
bitField0_ = (bitField0_ & ~0x00000400);
dt_ = "";
bitField0_ = (bitField0_ & ~0x00000800);
roomId_ = "";
bitField0_ = (bitField0_ & ~0x00001000);
pushData_ = "";
bitField0_ = (bitField0_ & ~0x00002000);
will_ = false;
bitField0_ = (bitField0_ & ~0x00004000);
patchTimestamp_ = 0L;
bitField0_ = (bitField0_ & ~0x00008000);
binaryMsg_ = com.google.protobuf.ByteString.EMPTY;
bitField0_ = (bitField0_ & ~0x00010000);
mentionPids_ = com.google.protobuf.LazyStringArrayList.EMPTY;
bitField0_ = (bitField0_ & ~0x00020000);
mentionAll_ = false;
bitField0_ = (bitField0_ & ~0x00040000);
convType_ = 0;
bitField0_ = (bitField0_ & ~0x00080000);
return this;
}
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return com.avos.avoscloud.Messages.internal_static_com_avos_avoscloud_DirectCommand_descriptor;
}
public com.avos.avoscloud.Messages.DirectCommand getDefaultInstanceForType() {
return com.avos.avoscloud.Messages.DirectCommand.getDefaultInstance();
}
public com.avos.avoscloud.Messages.DirectCommand build() {
com.avos.avoscloud.Messages.DirectCommand result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
public com.avos.avoscloud.Messages.DirectCommand buildPartial() {
com.avos.avoscloud.Messages.DirectCommand result = new com.avos.avoscloud.Messages.DirectCommand(this);
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (((from_bitField0_ & 0x00000001) == 0x00000001)) {
to_bitField0_ |= 0x00000001;
}
result.msg_ = msg_;
if (((from_bitField0_ & 0x00000002) == 0x00000002)) {
to_bitField0_ |= 0x00000002;
}
result.uid_ = uid_;
if (((from_bitField0_ & 0x00000004) == 0x00000004)) {
to_bitField0_ |= 0x00000004;
}
result.fromPeerId_ = fromPeerId_;
if (((from_bitField0_ & 0x00000008) == 0x00000008)) {
to_bitField0_ |= 0x00000008;
}
result.timestamp_ = timestamp_;
if (((from_bitField0_ & 0x00000010) == 0x00000010)) {
to_bitField0_ |= 0x00000010;
}
result.offline_ = offline_;
if (((from_bitField0_ & 0x00000020) == 0x00000020)) {
to_bitField0_ |= 0x00000020;
}
result.hasMore_ = hasMore_;
if (((bitField0_ & 0x00000040) == 0x00000040)) {
toPeerIds_ = toPeerIds_.getUnmodifiableView();
bitField0_ = (bitField0_ & ~0x00000040);
}
result.toPeerIds_ = toPeerIds_;
if (((from_bitField0_ & 0x00000080) == 0x00000080)) {
to_bitField0_ |= 0x00000040;
}
result.r_ = r_;
if (((from_bitField0_ & 0x00000100) == 0x00000100)) {
to_bitField0_ |= 0x00000080;
}
result.cid_ = cid_;
if (((from_bitField0_ & 0x00000200) == 0x00000200)) {
to_bitField0_ |= 0x00000100;
}
result.id_ = id_;
if (((from_bitField0_ & 0x00000400) == 0x00000400)) {
to_bitField0_ |= 0x00000200;
}
result.transient_ = transient_;
if (((from_bitField0_ & 0x00000800) == 0x00000800)) {
to_bitField0_ |= 0x00000400;
}
result.dt_ = dt_;
if (((from_bitField0_ & 0x00001000) == 0x00001000)) {
to_bitField0_ |= 0x00000800;
}
result.roomId_ = roomId_;
if (((from_bitField0_ & 0x00002000) == 0x00002000)) {
to_bitField0_ |= 0x00001000;
}
result.pushData_ = pushData_;
if (((from_bitField0_ & 0x00004000) == 0x00004000)) {
to_bitField0_ |= 0x00002000;
}
result.will_ = will_;
if (((from_bitField0_ & 0x00008000) == 0x00008000)) {
to_bitField0_ |= 0x00004000;
}
result.patchTimestamp_ = patchTimestamp_;
if (((from_bitField0_ & 0x00010000) == 0x00010000)) {
to_bitField0_ |= 0x00008000;
}
result.binaryMsg_ = binaryMsg_;
if (((bitField0_ & 0x00020000) == 0x00020000)) {
mentionPids_ = mentionPids_.getUnmodifiableView();
bitField0_ = (bitField0_ & ~0x00020000);
}
result.mentionPids_ = mentionPids_;
if (((from_bitField0_ & 0x00040000) == 0x00040000)) {
to_bitField0_ |= 0x00010000;
}
result.mentionAll_ = mentionAll_;
if (((from_bitField0_ & 0x00080000) == 0x00080000)) {
to_bitField0_ |= 0x00020000;
}
result.convType_ = convType_;
result.bitField0_ = to_bitField0_;
onBuilt();
return result;
}
public Builder clone() {
return (Builder) super.clone();
}
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.setField(field, value);
}
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return (Builder) super.clearField(field);
}
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return (Builder) super.clearOneof(oneof);
}
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return (Builder) super.setRepeatedField(field, index, value);
}
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.addRepeatedField(field, value);
}
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.avos.avoscloud.Messages.DirectCommand) {
return mergeFrom((com.avos.avoscloud.Messages.DirectCommand)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.avos.avoscloud.Messages.DirectCommand other) {
if (other == com.avos.avoscloud.Messages.DirectCommand.getDefaultInstance()) return this;
if (other.hasMsg()) {
bitField0_ |= 0x00000001;
msg_ = other.msg_;
onChanged();
}
if (other.hasUid()) {
bitField0_ |= 0x00000002;
uid_ = other.uid_;
onChanged();
}
if (other.hasFromPeerId()) {
bitField0_ |= 0x00000004;
fromPeerId_ = other.fromPeerId_;
onChanged();
}
if (other.hasTimestamp()) {
setTimestamp(other.getTimestamp());
}
if (other.hasOffline()) {
setOffline(other.getOffline());
}
if (other.hasHasMore()) {
setHasMore(other.getHasMore());
}
if (!other.toPeerIds_.isEmpty()) {
if (toPeerIds_.isEmpty()) {
toPeerIds_ = other.toPeerIds_;
bitField0_ = (bitField0_ & ~0x00000040);
} else {
ensureToPeerIdsIsMutable();
toPeerIds_.addAll(other.toPeerIds_);
}
onChanged();
}
if (other.hasR()) {
setR(other.getR());
}
if (other.hasCid()) {
bitField0_ |= 0x00000100;
cid_ = other.cid_;
onChanged();
}
if (other.hasId()) {
bitField0_ |= 0x00000200;
id_ = other.id_;
onChanged();
}
if (other.hasTransient()) {
setTransient(other.getTransient());
}
if (other.hasDt()) {
bitField0_ |= 0x00000800;
dt_ = other.dt_;
onChanged();
}
if (other.hasRoomId()) {
bitField0_ |= 0x00001000;
roomId_ = other.roomId_;
onChanged();
}
if (other.hasPushData()) {
bitField0_ |= 0x00002000;
pushData_ = other.pushData_;
onChanged();
}
if (other.hasWill()) {
setWill(other.getWill());
}
if (other.hasPatchTimestamp()) {
setPatchTimestamp(other.getPatchTimestamp());
}
if (other.hasBinaryMsg()) {
setBinaryMsg(other.getBinaryMsg());
}
if (!other.mentionPids_.isEmpty()) {
if (mentionPids_.isEmpty()) {
mentionPids_ = other.mentionPids_;
bitField0_ = (bitField0_ & ~0x00020000);
} else {
ensureMentionPidsIsMutable();
mentionPids_.addAll(other.mentionPids_);
}
onChanged();
}
if (other.hasMentionAll()) {
setMentionAll(other.getMentionAll());
}
if (other.hasConvType()) {
setConvType(other.getConvType());
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
public final boolean isInitialized() {
return true;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
com.avos.avoscloud.Messages.DirectCommand parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (com.avos.avoscloud.Messages.DirectCommand) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
private java.lang.Object msg_ = "";
/**
* optional string msg = 1;
*/
public boolean hasMsg() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
* optional string msg = 1;
*/
public java.lang.String getMsg() {
java.lang.Object ref = msg_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
msg_ = s;
}
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* optional string msg = 1;
*/
public com.google.protobuf.ByteString
getMsgBytes() {
java.lang.Object ref = msg_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
msg_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* optional string msg = 1;
*/
public Builder setMsg(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000001;
msg_ = value;
onChanged();
return this;
}
/**
* optional string msg = 1;
*/
public Builder clearMsg() {
bitField0_ = (bitField0_ & ~0x00000001);
msg_ = getDefaultInstance().getMsg();
onChanged();
return this;
}
/**
* optional string msg = 1;
*/
public Builder setMsgBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000001;
msg_ = value;
onChanged();
return this;
}
private java.lang.Object uid_ = "";
/**
* optional string uid = 2;
*/
public boolean hasUid() {
return ((bitField0_ & 0x00000002) == 0x00000002);
}
/**
* optional string uid = 2;
*/
public java.lang.String getUid() {
java.lang.Object ref = uid_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
uid_ = s;
}
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* optional string uid = 2;
*/
public com.google.protobuf.ByteString
getUidBytes() {
java.lang.Object ref = uid_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
uid_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* optional string uid = 2;
*/
public Builder setUid(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000002;
uid_ = value;
onChanged();
return this;
}
/**
* optional string uid = 2;
*/
public Builder clearUid() {
bitField0_ = (bitField0_ & ~0x00000002);
uid_ = getDefaultInstance().getUid();
onChanged();
return this;
}
/**
* optional string uid = 2;
*/
public Builder setUidBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000002;
uid_ = value;
onChanged();
return this;
}
private java.lang.Object fromPeerId_ = "";
/**
* optional string fromPeerId = 3;
*/
public boolean hasFromPeerId() {
return ((bitField0_ & 0x00000004) == 0x00000004);
}
/**
* optional string fromPeerId = 3;
*/
public java.lang.String getFromPeerId() {
java.lang.Object ref = fromPeerId_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
fromPeerId_ = s;
}
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* optional string fromPeerId = 3;
*/
public com.google.protobuf.ByteString
getFromPeerIdBytes() {
java.lang.Object ref = fromPeerId_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
fromPeerId_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* optional string fromPeerId = 3;
*/
public Builder setFromPeerId(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000004;
fromPeerId_ = value;
onChanged();
return this;
}
/**
* optional string fromPeerId = 3;
*/
public Builder clearFromPeerId() {
bitField0_ = (bitField0_ & ~0x00000004);
fromPeerId_ = getDefaultInstance().getFromPeerId();
onChanged();
return this;
}
/**
* optional string fromPeerId = 3;
*/
public Builder setFromPeerIdBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000004;
fromPeerId_ = value;
onChanged();
return this;
}
private long timestamp_ ;
/**
* optional int64 timestamp = 4;
*/
public boolean hasTimestamp() {
return ((bitField0_ & 0x00000008) == 0x00000008);
}
/**
* optional int64 timestamp = 4;
*/
public long getTimestamp() {
return timestamp_;
}
/**
* optional int64 timestamp = 4;
*/
public Builder setTimestamp(long value) {
bitField0_ |= 0x00000008;
timestamp_ = value;
onChanged();
return this;
}
/**
* optional int64 timestamp = 4;
*/
public Builder clearTimestamp() {
bitField0_ = (bitField0_ & ~0x00000008);
timestamp_ = 0L;
onChanged();
return this;
}
private boolean offline_ ;
/**
* optional bool offline = 5;
*/
public boolean hasOffline() {
return ((bitField0_ & 0x00000010) == 0x00000010);
}
/**
* optional bool offline = 5;
*/
public boolean getOffline() {
return offline_;
}
/**
* optional bool offline = 5;
*/
public Builder setOffline(boolean value) {
bitField0_ |= 0x00000010;
offline_ = value;
onChanged();
return this;
}
/**
* optional bool offline = 5;
*/
public Builder clearOffline() {
bitField0_ = (bitField0_ & ~0x00000010);
offline_ = false;
onChanged();
return this;
}
private boolean hasMore_ ;
/**
* optional bool hasMore = 6;
*/
public boolean hasHasMore() {
return ((bitField0_ & 0x00000020) == 0x00000020);
}
/**
* optional bool hasMore = 6;
*/
public boolean getHasMore() {
return hasMore_;
}
/**
* optional bool hasMore = 6;
*/
public Builder setHasMore(boolean value) {
bitField0_ |= 0x00000020;
hasMore_ = value;
onChanged();
return this;
}
/**
* optional bool hasMore = 6;
*/
public Builder clearHasMore() {
bitField0_ = (bitField0_ & ~0x00000020);
hasMore_ = false;
onChanged();
return this;
}
private com.google.protobuf.LazyStringList toPeerIds_ = com.google.protobuf.LazyStringArrayList.EMPTY;
private void ensureToPeerIdsIsMutable() {
if (!((bitField0_ & 0x00000040) == 0x00000040)) {
toPeerIds_ = new com.google.protobuf.LazyStringArrayList(toPeerIds_);
bitField0_ |= 0x00000040;
}
}
/**
* repeated string toPeerIds = 7;
*/
public com.google.protobuf.ProtocolStringList
getToPeerIdsList() {
return toPeerIds_.getUnmodifiableView();
}
/**
* repeated string toPeerIds = 7;
*/
public int getToPeerIdsCount() {
return toPeerIds_.size();
}
/**
* repeated string toPeerIds = 7;
*/
public java.lang.String getToPeerIds(int index) {
return toPeerIds_.get(index);
}
/**
* repeated string toPeerIds = 7;
*/
public com.google.protobuf.ByteString
getToPeerIdsBytes(int index) {
return toPeerIds_.getByteString(index);
}
/**
* repeated string toPeerIds = 7;
*/
public Builder setToPeerIds(
int index, java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
ensureToPeerIdsIsMutable();
toPeerIds_.set(index, value);
onChanged();
return this;
}
/**
* repeated string toPeerIds = 7;
*/
public Builder addToPeerIds(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
ensureToPeerIdsIsMutable();
toPeerIds_.add(value);
onChanged();
return this;
}
/**
* repeated string toPeerIds = 7;
*/
public Builder addAllToPeerIds(
java.lang.Iterable values) {
ensureToPeerIdsIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, toPeerIds_);
onChanged();
return this;
}
/**
* repeated string toPeerIds = 7;
*/
public Builder clearToPeerIds() {
toPeerIds_ = com.google.protobuf.LazyStringArrayList.EMPTY;
bitField0_ = (bitField0_ & ~0x00000040);
onChanged();
return this;
}
/**
* repeated string toPeerIds = 7;
*/
public Builder addToPeerIdsBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
ensureToPeerIdsIsMutable();
toPeerIds_.add(value);
onChanged();
return this;
}
private boolean r_ ;
/**
* optional bool r = 10;
*/
public boolean hasR() {
return ((bitField0_ & 0x00000080) == 0x00000080);
}
/**
* optional bool r = 10;
*/
public boolean getR() {
return r_;
}
/**
* optional bool r = 10;
*/
public Builder setR(boolean value) {
bitField0_ |= 0x00000080;
r_ = value;
onChanged();
return this;
}
/**
* optional bool r = 10;
*/
public Builder clearR() {
bitField0_ = (bitField0_ & ~0x00000080);
r_ = false;
onChanged();
return this;
}
private java.lang.Object cid_ = "";
/**
* optional string cid = 11;
*/
public boolean hasCid() {
return ((bitField0_ & 0x00000100) == 0x00000100);
}
/**
* optional string cid = 11;
*/
public java.lang.String getCid() {
java.lang.Object ref = cid_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
cid_ = s;
}
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* optional string cid = 11;
*/
public com.google.protobuf.ByteString
getCidBytes() {
java.lang.Object ref = cid_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
cid_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* optional string cid = 11;
*/
public Builder setCid(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000100;
cid_ = value;
onChanged();
return this;
}
/**
* optional string cid = 11;
*/
public Builder clearCid() {
bitField0_ = (bitField0_ & ~0x00000100);
cid_ = getDefaultInstance().getCid();
onChanged();
return this;
}
/**
* optional string cid = 11;
*/
public Builder setCidBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000100;
cid_ = value;
onChanged();
return this;
}
private java.lang.Object id_ = "";
/**
* optional string id = 12;
*/
public boolean hasId() {
return ((bitField0_ & 0x00000200) == 0x00000200);
}
/**
* optional string id = 12;
*/
public java.lang.String getId() {
java.lang.Object ref = id_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
id_ = s;
}
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* optional string id = 12;
*/
public com.google.protobuf.ByteString
getIdBytes() {
java.lang.Object ref = id_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
id_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* optional string id = 12;
*/
public Builder setId(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000200;
id_ = value;
onChanged();
return this;
}
/**
* optional string id = 12;
*/
public Builder clearId() {
bitField0_ = (bitField0_ & ~0x00000200);
id_ = getDefaultInstance().getId();
onChanged();
return this;
}
/**
* optional string id = 12;
*/
public Builder setIdBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000200;
id_ = value;
onChanged();
return this;
}
private boolean transient_ ;
/**
* optional bool transient = 13;
*/
public boolean hasTransient() {
return ((bitField0_ & 0x00000400) == 0x00000400);
}
/**
* optional bool transient = 13;
*/
public boolean getTransient() {
return transient_;
}
/**
* optional bool transient = 13;
*/
public Builder setTransient(boolean value) {
bitField0_ |= 0x00000400;
transient_ = value;
onChanged();
return this;
}
/**
* optional bool transient = 13;
*/
public Builder clearTransient() {
bitField0_ = (bitField0_ & ~0x00000400);
transient_ = false;
onChanged();
return this;
}
private java.lang.Object dt_ = "";
/**
* optional string dt = 14;
*/
public boolean hasDt() {
return ((bitField0_ & 0x00000800) == 0x00000800);
}
/**
* optional string dt = 14;
*/
public java.lang.String getDt() {
java.lang.Object ref = dt_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
dt_ = s;
}
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* optional string dt = 14;
*/
public com.google.protobuf.ByteString
getDtBytes() {
java.lang.Object ref = dt_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
dt_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* optional string dt = 14;
*/
public Builder setDt(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000800;
dt_ = value;
onChanged();
return this;
}
/**
* optional string dt = 14;
*/
public Builder clearDt() {
bitField0_ = (bitField0_ & ~0x00000800);
dt_ = getDefaultInstance().getDt();
onChanged();
return this;
}
/**
* optional string dt = 14;
*/
public Builder setDtBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000800;
dt_ = value;
onChanged();
return this;
}
private java.lang.Object roomId_ = "";
/**
* optional string roomId = 15;
*/
public boolean hasRoomId() {
return ((bitField0_ & 0x00001000) == 0x00001000);
}
/**
* optional string roomId = 15;
*/
public java.lang.String getRoomId() {
java.lang.Object ref = roomId_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
roomId_ = s;
}
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* optional string roomId = 15;
*/
public com.google.protobuf.ByteString
getRoomIdBytes() {
java.lang.Object ref = roomId_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
roomId_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* optional string roomId = 15;
*/
public Builder setRoomId(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00001000;
roomId_ = value;
onChanged();
return this;
}
/**
* optional string roomId = 15;
*/
public Builder clearRoomId() {
bitField0_ = (bitField0_ & ~0x00001000);
roomId_ = getDefaultInstance().getRoomId();
onChanged();
return this;
}
/**
* optional string roomId = 15;
*/
public Builder setRoomIdBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00001000;
roomId_ = value;
onChanged();
return this;
}
private java.lang.Object pushData_ = "";
/**
* optional string pushData = 16;
*/
public boolean hasPushData() {
return ((bitField0_ & 0x00002000) == 0x00002000);
}
/**
* optional string pushData = 16;
*/
public java.lang.String getPushData() {
java.lang.Object ref = pushData_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
pushData_ = s;
}
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* optional string pushData = 16;
*/
public com.google.protobuf.ByteString
getPushDataBytes() {
java.lang.Object ref = pushData_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
pushData_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* optional string pushData = 16;
*/
public Builder setPushData(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00002000;
pushData_ = value;
onChanged();
return this;
}
/**
* optional string pushData = 16;
*/
public Builder clearPushData() {
bitField0_ = (bitField0_ & ~0x00002000);
pushData_ = getDefaultInstance().getPushData();
onChanged();
return this;
}
/**
* optional string pushData = 16;
*/
public Builder setPushDataBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00002000;
pushData_ = value;
onChanged();
return this;
}
private boolean will_ ;
/**
* optional bool will = 17;
*/
public boolean hasWill() {
return ((bitField0_ & 0x00004000) == 0x00004000);
}
/**
* optional bool will = 17;
*/
public boolean getWill() {
return will_;
}
/**
* optional bool will = 17;
*/
public Builder setWill(boolean value) {
bitField0_ |= 0x00004000;
will_ = value;
onChanged();
return this;
}
/**
* optional bool will = 17;
*/
public Builder clearWill() {
bitField0_ = (bitField0_ & ~0x00004000);
will_ = false;
onChanged();
return this;
}
private long patchTimestamp_ ;
/**
* optional int64 patchTimestamp = 18;
*/
public boolean hasPatchTimestamp() {
return ((bitField0_ & 0x00008000) == 0x00008000);
}
/**
* optional int64 patchTimestamp = 18;
*/
public long getPatchTimestamp() {
return patchTimestamp_;
}
/**
* optional int64 patchTimestamp = 18;
*/
public Builder setPatchTimestamp(long value) {
bitField0_ |= 0x00008000;
patchTimestamp_ = value;
onChanged();
return this;
}
/**
* optional int64 patchTimestamp = 18;
*/
public Builder clearPatchTimestamp() {
bitField0_ = (bitField0_ & ~0x00008000);
patchTimestamp_ = 0L;
onChanged();
return this;
}
private com.google.protobuf.ByteString binaryMsg_ = com.google.protobuf.ByteString.EMPTY;
/**
* optional bytes binaryMsg = 19;
*/
public boolean hasBinaryMsg() {
return ((bitField0_ & 0x00010000) == 0x00010000);
}
/**
* optional bytes binaryMsg = 19;
*/
public com.google.protobuf.ByteString getBinaryMsg() {
return binaryMsg_;
}
/**
* optional bytes binaryMsg = 19;
*/
public Builder setBinaryMsg(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00010000;
binaryMsg_ = value;
onChanged();
return this;
}
/**
* optional bytes binaryMsg = 19;
*/
public Builder clearBinaryMsg() {
bitField0_ = (bitField0_ & ~0x00010000);
binaryMsg_ = getDefaultInstance().getBinaryMsg();
onChanged();
return this;
}
private com.google.protobuf.LazyStringList mentionPids_ = com.google.protobuf.LazyStringArrayList.EMPTY;
private void ensureMentionPidsIsMutable() {
if (!((bitField0_ & 0x00020000) == 0x00020000)) {
mentionPids_ = new com.google.protobuf.LazyStringArrayList(mentionPids_);
bitField0_ |= 0x00020000;
}
}
/**
* repeated string mentionPids = 20;
*/
public com.google.protobuf.ProtocolStringList
getMentionPidsList() {
return mentionPids_.getUnmodifiableView();
}
/**
* repeated string mentionPids = 20;
*/
public int getMentionPidsCount() {
return mentionPids_.size();
}
/**
* repeated string mentionPids = 20;
*/
public java.lang.String getMentionPids(int index) {
return mentionPids_.get(index);
}
/**
* repeated string mentionPids = 20;
*/
public com.google.protobuf.ByteString
getMentionPidsBytes(int index) {
return mentionPids_.getByteString(index);
}
/**
* repeated string mentionPids = 20;
*/
public Builder setMentionPids(
int index, java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
ensureMentionPidsIsMutable();
mentionPids_.set(index, value);
onChanged();
return this;
}
/**
* repeated string mentionPids = 20;
*/
public Builder addMentionPids(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
ensureMentionPidsIsMutable();
mentionPids_.add(value);
onChanged();
return this;
}
/**
* repeated string mentionPids = 20;
*/
public Builder addAllMentionPids(
java.lang.Iterable values) {
ensureMentionPidsIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, mentionPids_);
onChanged();
return this;
}
/**
* repeated string mentionPids = 20;
*/
public Builder clearMentionPids() {
mentionPids_ = com.google.protobuf.LazyStringArrayList.EMPTY;
bitField0_ = (bitField0_ & ~0x00020000);
onChanged();
return this;
}
/**
* repeated string mentionPids = 20;
*/
public Builder addMentionPidsBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
ensureMentionPidsIsMutable();
mentionPids_.add(value);
onChanged();
return this;
}
private boolean mentionAll_ ;
/**
* optional bool mentionAll = 21;
*/
public boolean hasMentionAll() {
return ((bitField0_ & 0x00040000) == 0x00040000);
}
/**
* optional bool mentionAll = 21;
*/
public boolean getMentionAll() {
return mentionAll_;
}
/**
* optional bool mentionAll = 21;
*/
public Builder setMentionAll(boolean value) {
bitField0_ |= 0x00040000;
mentionAll_ = value;
onChanged();
return this;
}
/**
* optional bool mentionAll = 21;
*/
public Builder clearMentionAll() {
bitField0_ = (bitField0_ & ~0x00040000);
mentionAll_ = false;
onChanged();
return this;
}
private int convType_ ;
/**
* optional int32 convType = 22;
*/
public boolean hasConvType() {
return ((bitField0_ & 0x00080000) == 0x00080000);
}
/**
* optional int32 convType = 22;
*/
public int getConvType() {
return convType_;
}
/**
* optional int32 convType = 22;
*/
public Builder setConvType(int value) {
bitField0_ |= 0x00080000;
convType_ = value;
onChanged();
return this;
}
/**
* optional int32 convType = 22;
*/
public Builder clearConvType() {
bitField0_ = (bitField0_ & ~0x00080000);
convType_ = 0;
onChanged();
return this;
}
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:com.avos.avoscloud.DirectCommand)
}
// @@protoc_insertion_point(class_scope:com.avos.avoscloud.DirectCommand)
private static final com.avos.avoscloud.Messages.DirectCommand DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new com.avos.avoscloud.Messages.DirectCommand();
}
public static com.avos.avoscloud.Messages.DirectCommand getDefaultInstance() {
return DEFAULT_INSTANCE;
}
@java.lang.Deprecated public static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
public DirectCommand parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new DirectCommand(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
public com.avos.avoscloud.Messages.DirectCommand getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface AckCommandOrBuilder extends
// @@protoc_insertion_point(interface_extends:com.avos.avoscloud.AckCommand)
com.google.protobuf.MessageOrBuilder {
/**
* optional int32 code = 1;
*/
boolean hasCode();
/**
* optional int32 code = 1;
*/
int getCode();
/**
* optional string reason = 2;
*/
boolean hasReason();
/**
* optional string reason = 2;
*/
java.lang.String getReason();
/**
* optional string reason = 2;
*/
com.google.protobuf.ByteString
getReasonBytes();
/**
* optional string mid = 3;
*/
boolean hasMid();
/**
* optional string mid = 3;
*/
java.lang.String getMid();
/**
* optional string mid = 3;
*/
com.google.protobuf.ByteString
getMidBytes();
/**
* optional string cid = 4;
*/
boolean hasCid();
/**
* optional string cid = 4;
*/
java.lang.String getCid();
/**
* optional string cid = 4;
*/
com.google.protobuf.ByteString
getCidBytes();
/**
* optional int64 t = 5;
*/
boolean hasT();
/**
* optional int64 t = 5;
*/
long getT();
/**
* optional string uid = 6;
*/
boolean hasUid();
/**
* optional string uid = 6;
*/
java.lang.String getUid();
/**
* optional string uid = 6;
*/
com.google.protobuf.ByteString
getUidBytes();
/**
* optional int64 fromts = 7;
*/
boolean hasFromts();
/**
* optional int64 fromts = 7;
*/
long getFromts();
/**
* optional int64 tots = 8;
*/
boolean hasTots();
/**
* optional int64 tots = 8;
*/
long getTots();
/**
* optional string type = 9;
*/
boolean hasType();
/**
* optional string type = 9;
*/
java.lang.String getType();
/**
* optional string type = 9;
*/
com.google.protobuf.ByteString
getTypeBytes();
/**
* repeated string ids = 10;
*/
java.util.List
getIdsList();
/**
* repeated string ids = 10;
*/
int getIdsCount();
/**
* repeated string ids = 10;
*/
java.lang.String getIds(int index);
/**
* repeated string ids = 10;
*/
com.google.protobuf.ByteString
getIdsBytes(int index);
/**
* optional int32 appCode = 11;
*/
boolean hasAppCode();
/**
* optional int32 appCode = 11;
*/
int getAppCode();
/**
* optional string appMsg = 12;
*/
boolean hasAppMsg();
/**
* optional string appMsg = 12;
*/
java.lang.String getAppMsg();
/**
* optional string appMsg = 12;
*/
com.google.protobuf.ByteString
getAppMsgBytes();
}
/**
* Protobuf type {@code com.avos.avoscloud.AckCommand}
*/
public static final class AckCommand extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:com.avos.avoscloud.AckCommand)
AckCommandOrBuilder {
private static final long serialVersionUID = 0L;
// Use AckCommand.newBuilder() to construct.
private AckCommand(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private AckCommand() {
code_ = 0;
reason_ = "";
mid_ = "";
cid_ = "";
t_ = 0L;
uid_ = "";
fromts_ = 0L;
tots_ = 0L;
type_ = "";
ids_ = com.google.protobuf.LazyStringArrayList.EMPTY;
appCode_ = 0;
appMsg_ = "";
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private AckCommand(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!parseUnknownField(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
case 8: {
bitField0_ |= 0x00000001;
code_ = input.readInt32();
break;
}
case 18: {
com.google.protobuf.ByteString bs = input.readBytes();
bitField0_ |= 0x00000002;
reason_ = bs;
break;
}
case 26: {
com.google.protobuf.ByteString bs = input.readBytes();
bitField0_ |= 0x00000004;
mid_ = bs;
break;
}
case 34: {
com.google.protobuf.ByteString bs = input.readBytes();
bitField0_ |= 0x00000008;
cid_ = bs;
break;
}
case 40: {
bitField0_ |= 0x00000010;
t_ = input.readInt64();
break;
}
case 50: {
com.google.protobuf.ByteString bs = input.readBytes();
bitField0_ |= 0x00000020;
uid_ = bs;
break;
}
case 56: {
bitField0_ |= 0x00000040;
fromts_ = input.readInt64();
break;
}
case 64: {
bitField0_ |= 0x00000080;
tots_ = input.readInt64();
break;
}
case 74: {
com.google.protobuf.ByteString bs = input.readBytes();
bitField0_ |= 0x00000100;
type_ = bs;
break;
}
case 82: {
com.google.protobuf.ByteString bs = input.readBytes();
if (!((mutable_bitField0_ & 0x00000200) == 0x00000200)) {
ids_ = new com.google.protobuf.LazyStringArrayList();
mutable_bitField0_ |= 0x00000200;
}
ids_.add(bs);
break;
}
case 88: {
bitField0_ |= 0x00000200;
appCode_ = input.readInt32();
break;
}
case 98: {
com.google.protobuf.ByteString bs = input.readBytes();
bitField0_ |= 0x00000400;
appMsg_ = bs;
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
if (((mutable_bitField0_ & 0x00000200) == 0x00000200)) {
ids_ = ids_.getUnmodifiableView();
}
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.avos.avoscloud.Messages.internal_static_com_avos_avoscloud_AckCommand_descriptor;
}
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.avos.avoscloud.Messages.internal_static_com_avos_avoscloud_AckCommand_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.avos.avoscloud.Messages.AckCommand.class, com.avos.avoscloud.Messages.AckCommand.Builder.class);
}
private int bitField0_;
public static final int CODE_FIELD_NUMBER = 1;
private int code_;
/**
* optional int32 code = 1;
*/
public boolean hasCode() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
* optional int32 code = 1;
*/
public int getCode() {
return code_;
}
public static final int REASON_FIELD_NUMBER = 2;
private volatile java.lang.Object reason_;
/**
* optional string reason = 2;
*/
public boolean hasReason() {
return ((bitField0_ & 0x00000002) == 0x00000002);
}
/**
* optional string reason = 2;
*/
public java.lang.String getReason() {
java.lang.Object ref = reason_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
reason_ = s;
}
return s;
}
}
/**
* optional string reason = 2;
*/
public com.google.protobuf.ByteString
getReasonBytes() {
java.lang.Object ref = reason_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
reason_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int MID_FIELD_NUMBER = 3;
private volatile java.lang.Object mid_;
/**
* optional string mid = 3;
*/
public boolean hasMid() {
return ((bitField0_ & 0x00000004) == 0x00000004);
}
/**
* optional string mid = 3;
*/
public java.lang.String getMid() {
java.lang.Object ref = mid_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
mid_ = s;
}
return s;
}
}
/**
* optional string mid = 3;
*/
public com.google.protobuf.ByteString
getMidBytes() {
java.lang.Object ref = mid_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
mid_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int CID_FIELD_NUMBER = 4;
private volatile java.lang.Object cid_;
/**
* optional string cid = 4;
*/
public boolean hasCid() {
return ((bitField0_ & 0x00000008) == 0x00000008);
}
/**
* optional string cid = 4;
*/
public java.lang.String getCid() {
java.lang.Object ref = cid_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
cid_ = s;
}
return s;
}
}
/**
* optional string cid = 4;
*/
public com.google.protobuf.ByteString
getCidBytes() {
java.lang.Object ref = cid_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
cid_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int T_FIELD_NUMBER = 5;
private long t_;
/**
* optional int64 t = 5;
*/
public boolean hasT() {
return ((bitField0_ & 0x00000010) == 0x00000010);
}
/**
* optional int64 t = 5;
*/
public long getT() {
return t_;
}
public static final int UID_FIELD_NUMBER = 6;
private volatile java.lang.Object uid_;
/**
* optional string uid = 6;
*/
public boolean hasUid() {
return ((bitField0_ & 0x00000020) == 0x00000020);
}
/**
* optional string uid = 6;
*/
public java.lang.String getUid() {
java.lang.Object ref = uid_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
uid_ = s;
}
return s;
}
}
/**
* optional string uid = 6;
*/
public com.google.protobuf.ByteString
getUidBytes() {
java.lang.Object ref = uid_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
uid_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int FROMTS_FIELD_NUMBER = 7;
private long fromts_;
/**
* optional int64 fromts = 7;
*/
public boolean hasFromts() {
return ((bitField0_ & 0x00000040) == 0x00000040);
}
/**
* optional int64 fromts = 7;
*/
public long getFromts() {
return fromts_;
}
public static final int TOTS_FIELD_NUMBER = 8;
private long tots_;
/**
* optional int64 tots = 8;
*/
public boolean hasTots() {
return ((bitField0_ & 0x00000080) == 0x00000080);
}
/**
* optional int64 tots = 8;
*/
public long getTots() {
return tots_;
}
public static final int TYPE_FIELD_NUMBER = 9;
private volatile java.lang.Object type_;
/**
* optional string type = 9;
*/
public boolean hasType() {
return ((bitField0_ & 0x00000100) == 0x00000100);
}
/**
* optional string type = 9;
*/
public java.lang.String getType() {
java.lang.Object ref = type_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
type_ = s;
}
return s;
}
}
/**
* optional string type = 9;
*/
public com.google.protobuf.ByteString
getTypeBytes() {
java.lang.Object ref = type_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
type_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int IDS_FIELD_NUMBER = 10;
private com.google.protobuf.LazyStringList ids_;
/**
* repeated string ids = 10;
*/
public com.google.protobuf.ProtocolStringList
getIdsList() {
return ids_;
}
/**
* repeated string ids = 10;
*/
public int getIdsCount() {
return ids_.size();
}
/**
* repeated string ids = 10;
*/
public java.lang.String getIds(int index) {
return ids_.get(index);
}
/**
* repeated string ids = 10;
*/
public com.google.protobuf.ByteString
getIdsBytes(int index) {
return ids_.getByteString(index);
}
public static final int APPCODE_FIELD_NUMBER = 11;
private int appCode_;
/**
* optional int32 appCode = 11;
*/
public boolean hasAppCode() {
return ((bitField0_ & 0x00000200) == 0x00000200);
}
/**
* optional int32 appCode = 11;
*/
public int getAppCode() {
return appCode_;
}
public static final int APPMSG_FIELD_NUMBER = 12;
private volatile java.lang.Object appMsg_;
/**
* optional string appMsg = 12;
*/
public boolean hasAppMsg() {
return ((bitField0_ & 0x00000400) == 0x00000400);
}
/**
* optional string appMsg = 12;
*/
public java.lang.String getAppMsg() {
java.lang.Object ref = appMsg_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
appMsg_ = s;
}
return s;
}
}
/**
* optional string appMsg = 12;
*/
public com.google.protobuf.ByteString
getAppMsgBytes() {
java.lang.Object ref = appMsg_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
appMsg_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
private byte memoizedIsInitialized = -1;
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (((bitField0_ & 0x00000001) == 0x00000001)) {
output.writeInt32(1, code_);
}
if (((bitField0_ & 0x00000002) == 0x00000002)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 2, reason_);
}
if (((bitField0_ & 0x00000004) == 0x00000004)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 3, mid_);
}
if (((bitField0_ & 0x00000008) == 0x00000008)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 4, cid_);
}
if (((bitField0_ & 0x00000010) == 0x00000010)) {
output.writeInt64(5, t_);
}
if (((bitField0_ & 0x00000020) == 0x00000020)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 6, uid_);
}
if (((bitField0_ & 0x00000040) == 0x00000040)) {
output.writeInt64(7, fromts_);
}
if (((bitField0_ & 0x00000080) == 0x00000080)) {
output.writeInt64(8, tots_);
}
if (((bitField0_ & 0x00000100) == 0x00000100)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 9, type_);
}
for (int i = 0; i < ids_.size(); i++) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 10, ids_.getRaw(i));
}
if (((bitField0_ & 0x00000200) == 0x00000200)) {
output.writeInt32(11, appCode_);
}
if (((bitField0_ & 0x00000400) == 0x00000400)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 12, appMsg_);
}
unknownFields.writeTo(output);
}
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (((bitField0_ & 0x00000001) == 0x00000001)) {
size += com.google.protobuf.CodedOutputStream
.computeInt32Size(1, code_);
}
if (((bitField0_ & 0x00000002) == 0x00000002)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(2, reason_);
}
if (((bitField0_ & 0x00000004) == 0x00000004)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(3, mid_);
}
if (((bitField0_ & 0x00000008) == 0x00000008)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(4, cid_);
}
if (((bitField0_ & 0x00000010) == 0x00000010)) {
size += com.google.protobuf.CodedOutputStream
.computeInt64Size(5, t_);
}
if (((bitField0_ & 0x00000020) == 0x00000020)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(6, uid_);
}
if (((bitField0_ & 0x00000040) == 0x00000040)) {
size += com.google.protobuf.CodedOutputStream
.computeInt64Size(7, fromts_);
}
if (((bitField0_ & 0x00000080) == 0x00000080)) {
size += com.google.protobuf.CodedOutputStream
.computeInt64Size(8, tots_);
}
if (((bitField0_ & 0x00000100) == 0x00000100)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(9, type_);
}
{
int dataSize = 0;
for (int i = 0; i < ids_.size(); i++) {
dataSize += computeStringSizeNoTag(ids_.getRaw(i));
}
size += dataSize;
size += 1 * getIdsList().size();
}
if (((bitField0_ & 0x00000200) == 0x00000200)) {
size += com.google.protobuf.CodedOutputStream
.computeInt32Size(11, appCode_);
}
if (((bitField0_ & 0x00000400) == 0x00000400)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(12, appMsg_);
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof com.avos.avoscloud.Messages.AckCommand)) {
return super.equals(obj);
}
com.avos.avoscloud.Messages.AckCommand other = (com.avos.avoscloud.Messages.AckCommand) obj;
boolean result = true;
result = result && (hasCode() == other.hasCode());
if (hasCode()) {
result = result && (getCode()
== other.getCode());
}
result = result && (hasReason() == other.hasReason());
if (hasReason()) {
result = result && getReason()
.equals(other.getReason());
}
result = result && (hasMid() == other.hasMid());
if (hasMid()) {
result = result && getMid()
.equals(other.getMid());
}
result = result && (hasCid() == other.hasCid());
if (hasCid()) {
result = result && getCid()
.equals(other.getCid());
}
result = result && (hasT() == other.hasT());
if (hasT()) {
result = result && (getT()
== other.getT());
}
result = result && (hasUid() == other.hasUid());
if (hasUid()) {
result = result && getUid()
.equals(other.getUid());
}
result = result && (hasFromts() == other.hasFromts());
if (hasFromts()) {
result = result && (getFromts()
== other.getFromts());
}
result = result && (hasTots() == other.hasTots());
if (hasTots()) {
result = result && (getTots()
== other.getTots());
}
result = result && (hasType() == other.hasType());
if (hasType()) {
result = result && getType()
.equals(other.getType());
}
result = result && getIdsList()
.equals(other.getIdsList());
result = result && (hasAppCode() == other.hasAppCode());
if (hasAppCode()) {
result = result && (getAppCode()
== other.getAppCode());
}
result = result && (hasAppMsg() == other.hasAppMsg());
if (hasAppMsg()) {
result = result && getAppMsg()
.equals(other.getAppMsg());
}
result = result && unknownFields.equals(other.unknownFields);
return result;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (hasCode()) {
hash = (37 * hash) + CODE_FIELD_NUMBER;
hash = (53 * hash) + getCode();
}
if (hasReason()) {
hash = (37 * hash) + REASON_FIELD_NUMBER;
hash = (53 * hash) + getReason().hashCode();
}
if (hasMid()) {
hash = (37 * hash) + MID_FIELD_NUMBER;
hash = (53 * hash) + getMid().hashCode();
}
if (hasCid()) {
hash = (37 * hash) + CID_FIELD_NUMBER;
hash = (53 * hash) + getCid().hashCode();
}
if (hasT()) {
hash = (37 * hash) + T_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getT());
}
if (hasUid()) {
hash = (37 * hash) + UID_FIELD_NUMBER;
hash = (53 * hash) + getUid().hashCode();
}
if (hasFromts()) {
hash = (37 * hash) + FROMTS_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getFromts());
}
if (hasTots()) {
hash = (37 * hash) + TOTS_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getTots());
}
if (hasType()) {
hash = (37 * hash) + TYPE_FIELD_NUMBER;
hash = (53 * hash) + getType().hashCode();
}
if (getIdsCount() > 0) {
hash = (37 * hash) + IDS_FIELD_NUMBER;
hash = (53 * hash) + getIdsList().hashCode();
}
if (hasAppCode()) {
hash = (37 * hash) + APPCODE_FIELD_NUMBER;
hash = (53 * hash) + getAppCode();
}
if (hasAppMsg()) {
hash = (37 * hash) + APPMSG_FIELD_NUMBER;
hash = (53 * hash) + getAppMsg().hashCode();
}
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static com.avos.avoscloud.Messages.AckCommand parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.avos.avoscloud.Messages.AckCommand parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.avos.avoscloud.Messages.AckCommand parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.avos.avoscloud.Messages.AckCommand parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.avos.avoscloud.Messages.AckCommand parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.avos.avoscloud.Messages.AckCommand parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.avos.avoscloud.Messages.AckCommand parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.avos.avoscloud.Messages.AckCommand parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static com.avos.avoscloud.Messages.AckCommand parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static com.avos.avoscloud.Messages.AckCommand parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static com.avos.avoscloud.Messages.AckCommand parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.avos.avoscloud.Messages.AckCommand parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(com.avos.avoscloud.Messages.AckCommand prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code com.avos.avoscloud.AckCommand}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:com.avos.avoscloud.AckCommand)
com.avos.avoscloud.Messages.AckCommandOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.avos.avoscloud.Messages.internal_static_com_avos_avoscloud_AckCommand_descriptor;
}
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.avos.avoscloud.Messages.internal_static_com_avos_avoscloud_AckCommand_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.avos.avoscloud.Messages.AckCommand.class, com.avos.avoscloud.Messages.AckCommand.Builder.class);
}
// Construct using com.avos.avoscloud.Messages.AckCommand.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
public Builder clear() {
super.clear();
code_ = 0;
bitField0_ = (bitField0_ & ~0x00000001);
reason_ = "";
bitField0_ = (bitField0_ & ~0x00000002);
mid_ = "";
bitField0_ = (bitField0_ & ~0x00000004);
cid_ = "";
bitField0_ = (bitField0_ & ~0x00000008);
t_ = 0L;
bitField0_ = (bitField0_ & ~0x00000010);
uid_ = "";
bitField0_ = (bitField0_ & ~0x00000020);
fromts_ = 0L;
bitField0_ = (bitField0_ & ~0x00000040);
tots_ = 0L;
bitField0_ = (bitField0_ & ~0x00000080);
type_ = "";
bitField0_ = (bitField0_ & ~0x00000100);
ids_ = com.google.protobuf.LazyStringArrayList.EMPTY;
bitField0_ = (bitField0_ & ~0x00000200);
appCode_ = 0;
bitField0_ = (bitField0_ & ~0x00000400);
appMsg_ = "";
bitField0_ = (bitField0_ & ~0x00000800);
return this;
}
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return com.avos.avoscloud.Messages.internal_static_com_avos_avoscloud_AckCommand_descriptor;
}
public com.avos.avoscloud.Messages.AckCommand getDefaultInstanceForType() {
return com.avos.avoscloud.Messages.AckCommand.getDefaultInstance();
}
public com.avos.avoscloud.Messages.AckCommand build() {
com.avos.avoscloud.Messages.AckCommand result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
public com.avos.avoscloud.Messages.AckCommand buildPartial() {
com.avos.avoscloud.Messages.AckCommand result = new com.avos.avoscloud.Messages.AckCommand(this);
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (((from_bitField0_ & 0x00000001) == 0x00000001)) {
to_bitField0_ |= 0x00000001;
}
result.code_ = code_;
if (((from_bitField0_ & 0x00000002) == 0x00000002)) {
to_bitField0_ |= 0x00000002;
}
result.reason_ = reason_;
if (((from_bitField0_ & 0x00000004) == 0x00000004)) {
to_bitField0_ |= 0x00000004;
}
result.mid_ = mid_;
if (((from_bitField0_ & 0x00000008) == 0x00000008)) {
to_bitField0_ |= 0x00000008;
}
result.cid_ = cid_;
if (((from_bitField0_ & 0x00000010) == 0x00000010)) {
to_bitField0_ |= 0x00000010;
}
result.t_ = t_;
if (((from_bitField0_ & 0x00000020) == 0x00000020)) {
to_bitField0_ |= 0x00000020;
}
result.uid_ = uid_;
if (((from_bitField0_ & 0x00000040) == 0x00000040)) {
to_bitField0_ |= 0x00000040;
}
result.fromts_ = fromts_;
if (((from_bitField0_ & 0x00000080) == 0x00000080)) {
to_bitField0_ |= 0x00000080;
}
result.tots_ = tots_;
if (((from_bitField0_ & 0x00000100) == 0x00000100)) {
to_bitField0_ |= 0x00000100;
}
result.type_ = type_;
if (((bitField0_ & 0x00000200) == 0x00000200)) {
ids_ = ids_.getUnmodifiableView();
bitField0_ = (bitField0_ & ~0x00000200);
}
result.ids_ = ids_;
if (((from_bitField0_ & 0x00000400) == 0x00000400)) {
to_bitField0_ |= 0x00000200;
}
result.appCode_ = appCode_;
if (((from_bitField0_ & 0x00000800) == 0x00000800)) {
to_bitField0_ |= 0x00000400;
}
result.appMsg_ = appMsg_;
result.bitField0_ = to_bitField0_;
onBuilt();
return result;
}
public Builder clone() {
return (Builder) super.clone();
}
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.setField(field, value);
}
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return (Builder) super.clearField(field);
}
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return (Builder) super.clearOneof(oneof);
}
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return (Builder) super.setRepeatedField(field, index, value);
}
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.addRepeatedField(field, value);
}
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.avos.avoscloud.Messages.AckCommand) {
return mergeFrom((com.avos.avoscloud.Messages.AckCommand)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.avos.avoscloud.Messages.AckCommand other) {
if (other == com.avos.avoscloud.Messages.AckCommand.getDefaultInstance()) return this;
if (other.hasCode()) {
setCode(other.getCode());
}
if (other.hasReason()) {
bitField0_ |= 0x00000002;
reason_ = other.reason_;
onChanged();
}
if (other.hasMid()) {
bitField0_ |= 0x00000004;
mid_ = other.mid_;
onChanged();
}
if (other.hasCid()) {
bitField0_ |= 0x00000008;
cid_ = other.cid_;
onChanged();
}
if (other.hasT()) {
setT(other.getT());
}
if (other.hasUid()) {
bitField0_ |= 0x00000020;
uid_ = other.uid_;
onChanged();
}
if (other.hasFromts()) {
setFromts(other.getFromts());
}
if (other.hasTots()) {
setTots(other.getTots());
}
if (other.hasType()) {
bitField0_ |= 0x00000100;
type_ = other.type_;
onChanged();
}
if (!other.ids_.isEmpty()) {
if (ids_.isEmpty()) {
ids_ = other.ids_;
bitField0_ = (bitField0_ & ~0x00000200);
} else {
ensureIdsIsMutable();
ids_.addAll(other.ids_);
}
onChanged();
}
if (other.hasAppCode()) {
setAppCode(other.getAppCode());
}
if (other.hasAppMsg()) {
bitField0_ |= 0x00000800;
appMsg_ = other.appMsg_;
onChanged();
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
public final boolean isInitialized() {
return true;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
com.avos.avoscloud.Messages.AckCommand parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (com.avos.avoscloud.Messages.AckCommand) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
private int code_ ;
/**
* optional int32 code = 1;
*/
public boolean hasCode() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
* optional int32 code = 1;
*/
public int getCode() {
return code_;
}
/**
* optional int32 code = 1;
*/
public Builder setCode(int value) {
bitField0_ |= 0x00000001;
code_ = value;
onChanged();
return this;
}
/**
* optional int32 code = 1;
*/
public Builder clearCode() {
bitField0_ = (bitField0_ & ~0x00000001);
code_ = 0;
onChanged();
return this;
}
private java.lang.Object reason_ = "";
/**
* optional string reason = 2;
*/
public boolean hasReason() {
return ((bitField0_ & 0x00000002) == 0x00000002);
}
/**
* optional string reason = 2;
*/
public java.lang.String getReason() {
java.lang.Object ref = reason_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
reason_ = s;
}
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* optional string reason = 2;
*/
public com.google.protobuf.ByteString
getReasonBytes() {
java.lang.Object ref = reason_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
reason_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* optional string reason = 2;
*/
public Builder setReason(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000002;
reason_ = value;
onChanged();
return this;
}
/**
* optional string reason = 2;
*/
public Builder clearReason() {
bitField0_ = (bitField0_ & ~0x00000002);
reason_ = getDefaultInstance().getReason();
onChanged();
return this;
}
/**
* optional string reason = 2;
*/
public Builder setReasonBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000002;
reason_ = value;
onChanged();
return this;
}
private java.lang.Object mid_ = "";
/**
* optional string mid = 3;
*/
public boolean hasMid() {
return ((bitField0_ & 0x00000004) == 0x00000004);
}
/**
* optional string mid = 3;
*/
public java.lang.String getMid() {
java.lang.Object ref = mid_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
mid_ = s;
}
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* optional string mid = 3;
*/
public com.google.protobuf.ByteString
getMidBytes() {
java.lang.Object ref = mid_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
mid_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* optional string mid = 3;
*/
public Builder setMid(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000004;
mid_ = value;
onChanged();
return this;
}
/**
* optional string mid = 3;
*/
public Builder clearMid() {
bitField0_ = (bitField0_ & ~0x00000004);
mid_ = getDefaultInstance().getMid();
onChanged();
return this;
}
/**
* optional string mid = 3;
*/
public Builder setMidBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000004;
mid_ = value;
onChanged();
return this;
}
private java.lang.Object cid_ = "";
/**
* optional string cid = 4;
*/
public boolean hasCid() {
return ((bitField0_ & 0x00000008) == 0x00000008);
}
/**
* optional string cid = 4;
*/
public java.lang.String getCid() {
java.lang.Object ref = cid_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
cid_ = s;
}
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* optional string cid = 4;
*/
public com.google.protobuf.ByteString
getCidBytes() {
java.lang.Object ref = cid_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
cid_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* optional string cid = 4;
*/
public Builder setCid(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000008;
cid_ = value;
onChanged();
return this;
}
/**
* optional string cid = 4;
*/
public Builder clearCid() {
bitField0_ = (bitField0_ & ~0x00000008);
cid_ = getDefaultInstance().getCid();
onChanged();
return this;
}
/**
* optional string cid = 4;
*/
public Builder setCidBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000008;
cid_ = value;
onChanged();
return this;
}
private long t_ ;
/**
* optional int64 t = 5;
*/
public boolean hasT() {
return ((bitField0_ & 0x00000010) == 0x00000010);
}
/**
* optional int64 t = 5;
*/
public long getT() {
return t_;
}
/**
* optional int64 t = 5;
*/
public Builder setT(long value) {
bitField0_ |= 0x00000010;
t_ = value;
onChanged();
return this;
}
/**
* optional int64 t = 5;
*/
public Builder clearT() {
bitField0_ = (bitField0_ & ~0x00000010);
t_ = 0L;
onChanged();
return this;
}
private java.lang.Object uid_ = "";
/**
* optional string uid = 6;
*/
public boolean hasUid() {
return ((bitField0_ & 0x00000020) == 0x00000020);
}
/**
* optional string uid = 6;
*/
public java.lang.String getUid() {
java.lang.Object ref = uid_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
uid_ = s;
}
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* optional string uid = 6;
*/
public com.google.protobuf.ByteString
getUidBytes() {
java.lang.Object ref = uid_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
uid_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* optional string uid = 6;
*/
public Builder setUid(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000020;
uid_ = value;
onChanged();
return this;
}
/**
* optional string uid = 6;
*/
public Builder clearUid() {
bitField0_ = (bitField0_ & ~0x00000020);
uid_ = getDefaultInstance().getUid();
onChanged();
return this;
}
/**
* optional string uid = 6;
*/
public Builder setUidBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000020;
uid_ = value;
onChanged();
return this;
}
private long fromts_ ;
/**
* optional int64 fromts = 7;
*/
public boolean hasFromts() {
return ((bitField0_ & 0x00000040) == 0x00000040);
}
/**
* optional int64 fromts = 7;
*/
public long getFromts() {
return fromts_;
}
/**
* optional int64 fromts = 7;
*/
public Builder setFromts(long value) {
bitField0_ |= 0x00000040;
fromts_ = value;
onChanged();
return this;
}
/**
* optional int64 fromts = 7;
*/
public Builder clearFromts() {
bitField0_ = (bitField0_ & ~0x00000040);
fromts_ = 0L;
onChanged();
return this;
}
private long tots_ ;
/**
* optional int64 tots = 8;
*/
public boolean hasTots() {
return ((bitField0_ & 0x00000080) == 0x00000080);
}
/**
* optional int64 tots = 8;
*/
public long getTots() {
return tots_;
}
/**
* optional int64 tots = 8;
*/
public Builder setTots(long value) {
bitField0_ |= 0x00000080;
tots_ = value;
onChanged();
return this;
}
/**
* optional int64 tots = 8;
*/
public Builder clearTots() {
bitField0_ = (bitField0_ & ~0x00000080);
tots_ = 0L;
onChanged();
return this;
}
private java.lang.Object type_ = "";
/**
* optional string type = 9;
*/
public boolean hasType() {
return ((bitField0_ & 0x00000100) == 0x00000100);
}
/**
* optional string type = 9;
*/
public java.lang.String getType() {
java.lang.Object ref = type_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
type_ = s;
}
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* optional string type = 9;
*/
public com.google.protobuf.ByteString
getTypeBytes() {
java.lang.Object ref = type_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
type_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* optional string type = 9;
*/
public Builder setType(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000100;
type_ = value;
onChanged();
return this;
}
/**
* optional string type = 9;
*/
public Builder clearType() {
bitField0_ = (bitField0_ & ~0x00000100);
type_ = getDefaultInstance().getType();
onChanged();
return this;
}
/**
* optional string type = 9;
*/
public Builder setTypeBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000100;
type_ = value;
onChanged();
return this;
}
private com.google.protobuf.LazyStringList ids_ = com.google.protobuf.LazyStringArrayList.EMPTY;
private void ensureIdsIsMutable() {
if (!((bitField0_ & 0x00000200) == 0x00000200)) {
ids_ = new com.google.protobuf.LazyStringArrayList(ids_);
bitField0_ |= 0x00000200;
}
}
/**
* repeated string ids = 10;
*/
public com.google.protobuf.ProtocolStringList
getIdsList() {
return ids_.getUnmodifiableView();
}
/**
* repeated string ids = 10;
*/
public int getIdsCount() {
return ids_.size();
}
/**
* repeated string ids = 10;
*/
public java.lang.String getIds(int index) {
return ids_.get(index);
}
/**
* repeated string ids = 10;
*/
public com.google.protobuf.ByteString
getIdsBytes(int index) {
return ids_.getByteString(index);
}
/**
* repeated string ids = 10;
*/
public Builder setIds(
int index, java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
ensureIdsIsMutable();
ids_.set(index, value);
onChanged();
return this;
}
/**
* repeated string ids = 10;
*/
public Builder addIds(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
ensureIdsIsMutable();
ids_.add(value);
onChanged();
return this;
}
/**
* repeated string ids = 10;
*/
public Builder addAllIds(
java.lang.Iterable values) {
ensureIdsIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, ids_);
onChanged();
return this;
}
/**
* repeated string ids = 10;
*/
public Builder clearIds() {
ids_ = com.google.protobuf.LazyStringArrayList.EMPTY;
bitField0_ = (bitField0_ & ~0x00000200);
onChanged();
return this;
}
/**
* repeated string ids = 10;
*/
public Builder addIdsBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
ensureIdsIsMutable();
ids_.add(value);
onChanged();
return this;
}
private int appCode_ ;
/**
* optional int32 appCode = 11;
*/
public boolean hasAppCode() {
return ((bitField0_ & 0x00000400) == 0x00000400);
}
/**
* optional int32 appCode = 11;
*/
public int getAppCode() {
return appCode_;
}
/**
* optional int32 appCode = 11;
*/
public Builder setAppCode(int value) {
bitField0_ |= 0x00000400;
appCode_ = value;
onChanged();
return this;
}
/**
* optional int32 appCode = 11;
*/
public Builder clearAppCode() {
bitField0_ = (bitField0_ & ~0x00000400);
appCode_ = 0;
onChanged();
return this;
}
private java.lang.Object appMsg_ = "";
/**
* optional string appMsg = 12;
*/
public boolean hasAppMsg() {
return ((bitField0_ & 0x00000800) == 0x00000800);
}
/**
* optional string appMsg = 12;
*/
public java.lang.String getAppMsg() {
java.lang.Object ref = appMsg_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
appMsg_ = s;
}
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* optional string appMsg = 12;
*/
public com.google.protobuf.ByteString
getAppMsgBytes() {
java.lang.Object ref = appMsg_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
appMsg_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* optional string appMsg = 12;
*/
public Builder setAppMsg(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000800;
appMsg_ = value;
onChanged();
return this;
}
/**
* optional string appMsg = 12;
*/
public Builder clearAppMsg() {
bitField0_ = (bitField0_ & ~0x00000800);
appMsg_ = getDefaultInstance().getAppMsg();
onChanged();
return this;
}
/**
* optional string appMsg = 12;
*/
public Builder setAppMsgBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000800;
appMsg_ = value;
onChanged();
return this;
}
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:com.avos.avoscloud.AckCommand)
}
// @@protoc_insertion_point(class_scope:com.avos.avoscloud.AckCommand)
private static final com.avos.avoscloud.Messages.AckCommand DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new com.avos.avoscloud.Messages.AckCommand();
}
public static com.avos.avoscloud.Messages.AckCommand getDefaultInstance() {
return DEFAULT_INSTANCE;
}
@java.lang.Deprecated public static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
public AckCommand parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new AckCommand(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
public com.avos.avoscloud.Messages.AckCommand getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface UnreadCommandOrBuilder extends
// @@protoc_insertion_point(interface_extends:com.avos.avoscloud.UnreadCommand)
com.google.protobuf.MessageOrBuilder {
/**
* repeated .com.avos.avoscloud.UnreadTuple convs = 1;
*/
java.util.List
getConvsList();
/**
* repeated .com.avos.avoscloud.UnreadTuple convs = 1;
*/
com.avos.avoscloud.Messages.UnreadTuple getConvs(int index);
/**
* repeated .com.avos.avoscloud.UnreadTuple convs = 1;
*/
int getConvsCount();
/**
* repeated .com.avos.avoscloud.UnreadTuple convs = 1;
*/
java.util.List extends com.avos.avoscloud.Messages.UnreadTupleOrBuilder>
getConvsOrBuilderList();
/**
* repeated .com.avos.avoscloud.UnreadTuple convs = 1;
*/
com.avos.avoscloud.Messages.UnreadTupleOrBuilder getConvsOrBuilder(
int index);
/**
* optional int64 notifTime = 2;
*/
boolean hasNotifTime();
/**
* optional int64 notifTime = 2;
*/
long getNotifTime();
}
/**
* Protobuf type {@code com.avos.avoscloud.UnreadCommand}
*/
public static final class UnreadCommand extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:com.avos.avoscloud.UnreadCommand)
UnreadCommandOrBuilder {
private static final long serialVersionUID = 0L;
// Use UnreadCommand.newBuilder() to construct.
private UnreadCommand(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private UnreadCommand() {
convs_ = java.util.Collections.emptyList();
notifTime_ = 0L;
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private UnreadCommand(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!parseUnknownField(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
case 10: {
if (!((mutable_bitField0_ & 0x00000001) == 0x00000001)) {
convs_ = new java.util.ArrayList();
mutable_bitField0_ |= 0x00000001;
}
convs_.add(
input.readMessage(com.avos.avoscloud.Messages.UnreadTuple.PARSER, extensionRegistry));
break;
}
case 16: {
bitField0_ |= 0x00000001;
notifTime_ = input.readInt64();
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
if (((mutable_bitField0_ & 0x00000001) == 0x00000001)) {
convs_ = java.util.Collections.unmodifiableList(convs_);
}
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.avos.avoscloud.Messages.internal_static_com_avos_avoscloud_UnreadCommand_descriptor;
}
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.avos.avoscloud.Messages.internal_static_com_avos_avoscloud_UnreadCommand_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.avos.avoscloud.Messages.UnreadCommand.class, com.avos.avoscloud.Messages.UnreadCommand.Builder.class);
}
private int bitField0_;
public static final int CONVS_FIELD_NUMBER = 1;
private java.util.List convs_;
/**
* repeated .com.avos.avoscloud.UnreadTuple convs = 1;
*/
public java.util.List getConvsList() {
return convs_;
}
/**
* repeated .com.avos.avoscloud.UnreadTuple convs = 1;
*/
public java.util.List extends com.avos.avoscloud.Messages.UnreadTupleOrBuilder>
getConvsOrBuilderList() {
return convs_;
}
/**
* repeated .com.avos.avoscloud.UnreadTuple convs = 1;
*/
public int getConvsCount() {
return convs_.size();
}
/**
* repeated .com.avos.avoscloud.UnreadTuple convs = 1;
*/
public com.avos.avoscloud.Messages.UnreadTuple getConvs(int index) {
return convs_.get(index);
}
/**
* repeated .com.avos.avoscloud.UnreadTuple convs = 1;
*/
public com.avos.avoscloud.Messages.UnreadTupleOrBuilder getConvsOrBuilder(
int index) {
return convs_.get(index);
}
public static final int NOTIFTIME_FIELD_NUMBER = 2;
private long notifTime_;
/**
* optional int64 notifTime = 2;
*/
public boolean hasNotifTime() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
* optional int64 notifTime = 2;
*/
public long getNotifTime() {
return notifTime_;
}
private byte memoizedIsInitialized = -1;
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
for (int i = 0; i < getConvsCount(); i++) {
if (!getConvs(i).isInitialized()) {
memoizedIsInitialized = 0;
return false;
}
}
memoizedIsInitialized = 1;
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
for (int i = 0; i < convs_.size(); i++) {
output.writeMessage(1, convs_.get(i));
}
if (((bitField0_ & 0x00000001) == 0x00000001)) {
output.writeInt64(2, notifTime_);
}
unknownFields.writeTo(output);
}
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
for (int i = 0; i < convs_.size(); i++) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(1, convs_.get(i));
}
if (((bitField0_ & 0x00000001) == 0x00000001)) {
size += com.google.protobuf.CodedOutputStream
.computeInt64Size(2, notifTime_);
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof com.avos.avoscloud.Messages.UnreadCommand)) {
return super.equals(obj);
}
com.avos.avoscloud.Messages.UnreadCommand other = (com.avos.avoscloud.Messages.UnreadCommand) obj;
boolean result = true;
result = result && getConvsList()
.equals(other.getConvsList());
result = result && (hasNotifTime() == other.hasNotifTime());
if (hasNotifTime()) {
result = result && (getNotifTime()
== other.getNotifTime());
}
result = result && unknownFields.equals(other.unknownFields);
return result;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (getConvsCount() > 0) {
hash = (37 * hash) + CONVS_FIELD_NUMBER;
hash = (53 * hash) + getConvsList().hashCode();
}
if (hasNotifTime()) {
hash = (37 * hash) + NOTIFTIME_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getNotifTime());
}
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static com.avos.avoscloud.Messages.UnreadCommand parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.avos.avoscloud.Messages.UnreadCommand parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.avos.avoscloud.Messages.UnreadCommand parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.avos.avoscloud.Messages.UnreadCommand parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.avos.avoscloud.Messages.UnreadCommand parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.avos.avoscloud.Messages.UnreadCommand parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.avos.avoscloud.Messages.UnreadCommand parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.avos.avoscloud.Messages.UnreadCommand parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static com.avos.avoscloud.Messages.UnreadCommand parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static com.avos.avoscloud.Messages.UnreadCommand parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static com.avos.avoscloud.Messages.UnreadCommand parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.avos.avoscloud.Messages.UnreadCommand parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(com.avos.avoscloud.Messages.UnreadCommand prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code com.avos.avoscloud.UnreadCommand}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:com.avos.avoscloud.UnreadCommand)
com.avos.avoscloud.Messages.UnreadCommandOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.avos.avoscloud.Messages.internal_static_com_avos_avoscloud_UnreadCommand_descriptor;
}
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.avos.avoscloud.Messages.internal_static_com_avos_avoscloud_UnreadCommand_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.avos.avoscloud.Messages.UnreadCommand.class, com.avos.avoscloud.Messages.UnreadCommand.Builder.class);
}
// Construct using com.avos.avoscloud.Messages.UnreadCommand.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
getConvsFieldBuilder();
}
}
public Builder clear() {
super.clear();
if (convsBuilder_ == null) {
convs_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000001);
} else {
convsBuilder_.clear();
}
notifTime_ = 0L;
bitField0_ = (bitField0_ & ~0x00000002);
return this;
}
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return com.avos.avoscloud.Messages.internal_static_com_avos_avoscloud_UnreadCommand_descriptor;
}
public com.avos.avoscloud.Messages.UnreadCommand getDefaultInstanceForType() {
return com.avos.avoscloud.Messages.UnreadCommand.getDefaultInstance();
}
public com.avos.avoscloud.Messages.UnreadCommand build() {
com.avos.avoscloud.Messages.UnreadCommand result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
public com.avos.avoscloud.Messages.UnreadCommand buildPartial() {
com.avos.avoscloud.Messages.UnreadCommand result = new com.avos.avoscloud.Messages.UnreadCommand(this);
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (convsBuilder_ == null) {
if (((bitField0_ & 0x00000001) == 0x00000001)) {
convs_ = java.util.Collections.unmodifiableList(convs_);
bitField0_ = (bitField0_ & ~0x00000001);
}
result.convs_ = convs_;
} else {
result.convs_ = convsBuilder_.build();
}
if (((from_bitField0_ & 0x00000002) == 0x00000002)) {
to_bitField0_ |= 0x00000001;
}
result.notifTime_ = notifTime_;
result.bitField0_ = to_bitField0_;
onBuilt();
return result;
}
public Builder clone() {
return (Builder) super.clone();
}
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.setField(field, value);
}
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return (Builder) super.clearField(field);
}
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return (Builder) super.clearOneof(oneof);
}
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return (Builder) super.setRepeatedField(field, index, value);
}
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.addRepeatedField(field, value);
}
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.avos.avoscloud.Messages.UnreadCommand) {
return mergeFrom((com.avos.avoscloud.Messages.UnreadCommand)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.avos.avoscloud.Messages.UnreadCommand other) {
if (other == com.avos.avoscloud.Messages.UnreadCommand.getDefaultInstance()) return this;
if (convsBuilder_ == null) {
if (!other.convs_.isEmpty()) {
if (convs_.isEmpty()) {
convs_ = other.convs_;
bitField0_ = (bitField0_ & ~0x00000001);
} else {
ensureConvsIsMutable();
convs_.addAll(other.convs_);
}
onChanged();
}
} else {
if (!other.convs_.isEmpty()) {
if (convsBuilder_.isEmpty()) {
convsBuilder_.dispose();
convsBuilder_ = null;
convs_ = other.convs_;
bitField0_ = (bitField0_ & ~0x00000001);
convsBuilder_ =
com.google.protobuf.GeneratedMessageV3.alwaysUseFieldBuilders ?
getConvsFieldBuilder() : null;
} else {
convsBuilder_.addAllMessages(other.convs_);
}
}
}
if (other.hasNotifTime()) {
setNotifTime(other.getNotifTime());
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
public final boolean isInitialized() {
for (int i = 0; i < getConvsCount(); i++) {
if (!getConvs(i).isInitialized()) {
return false;
}
}
return true;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
com.avos.avoscloud.Messages.UnreadCommand parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (com.avos.avoscloud.Messages.UnreadCommand) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
private java.util.List convs_ =
java.util.Collections.emptyList();
private void ensureConvsIsMutable() {
if (!((bitField0_ & 0x00000001) == 0x00000001)) {
convs_ = new java.util.ArrayList(convs_);
bitField0_ |= 0x00000001;
}
}
private com.google.protobuf.RepeatedFieldBuilderV3<
com.avos.avoscloud.Messages.UnreadTuple, com.avos.avoscloud.Messages.UnreadTuple.Builder, com.avos.avoscloud.Messages.UnreadTupleOrBuilder> convsBuilder_;
/**
* repeated .com.avos.avoscloud.UnreadTuple convs = 1;
*/
public java.util.List getConvsList() {
if (convsBuilder_ == null) {
return java.util.Collections.unmodifiableList(convs_);
} else {
return convsBuilder_.getMessageList();
}
}
/**
* repeated .com.avos.avoscloud.UnreadTuple convs = 1;
*/
public int getConvsCount() {
if (convsBuilder_ == null) {
return convs_.size();
} else {
return convsBuilder_.getCount();
}
}
/**
* repeated .com.avos.avoscloud.UnreadTuple convs = 1;
*/
public com.avos.avoscloud.Messages.UnreadTuple getConvs(int index) {
if (convsBuilder_ == null) {
return convs_.get(index);
} else {
return convsBuilder_.getMessage(index);
}
}
/**
* repeated .com.avos.avoscloud.UnreadTuple convs = 1;
*/
public Builder setConvs(
int index, com.avos.avoscloud.Messages.UnreadTuple value) {
if (convsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureConvsIsMutable();
convs_.set(index, value);
onChanged();
} else {
convsBuilder_.setMessage(index, value);
}
return this;
}
/**
* repeated .com.avos.avoscloud.UnreadTuple convs = 1;
*/
public Builder setConvs(
int index, com.avos.avoscloud.Messages.UnreadTuple.Builder builderForValue) {
if (convsBuilder_ == null) {
ensureConvsIsMutable();
convs_.set(index, builderForValue.build());
onChanged();
} else {
convsBuilder_.setMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .com.avos.avoscloud.UnreadTuple convs = 1;
*/
public Builder addConvs(com.avos.avoscloud.Messages.UnreadTuple value) {
if (convsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureConvsIsMutable();
convs_.add(value);
onChanged();
} else {
convsBuilder_.addMessage(value);
}
return this;
}
/**
* repeated .com.avos.avoscloud.UnreadTuple convs = 1;
*/
public Builder addConvs(
int index, com.avos.avoscloud.Messages.UnreadTuple value) {
if (convsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureConvsIsMutable();
convs_.add(index, value);
onChanged();
} else {
convsBuilder_.addMessage(index, value);
}
return this;
}
/**
* repeated .com.avos.avoscloud.UnreadTuple convs = 1;
*/
public Builder addConvs(
com.avos.avoscloud.Messages.UnreadTuple.Builder builderForValue) {
if (convsBuilder_ == null) {
ensureConvsIsMutable();
convs_.add(builderForValue.build());
onChanged();
} else {
convsBuilder_.addMessage(builderForValue.build());
}
return this;
}
/**
* repeated .com.avos.avoscloud.UnreadTuple convs = 1;
*/
public Builder addConvs(
int index, com.avos.avoscloud.Messages.UnreadTuple.Builder builderForValue) {
if (convsBuilder_ == null) {
ensureConvsIsMutable();
convs_.add(index, builderForValue.build());
onChanged();
} else {
convsBuilder_.addMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .com.avos.avoscloud.UnreadTuple convs = 1;
*/
public Builder addAllConvs(
java.lang.Iterable extends com.avos.avoscloud.Messages.UnreadTuple> values) {
if (convsBuilder_ == null) {
ensureConvsIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, convs_);
onChanged();
} else {
convsBuilder_.addAllMessages(values);
}
return this;
}
/**
* repeated .com.avos.avoscloud.UnreadTuple convs = 1;
*/
public Builder clearConvs() {
if (convsBuilder_ == null) {
convs_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000001);
onChanged();
} else {
convsBuilder_.clear();
}
return this;
}
/**
* repeated .com.avos.avoscloud.UnreadTuple convs = 1;
*/
public Builder removeConvs(int index) {
if (convsBuilder_ == null) {
ensureConvsIsMutable();
convs_.remove(index);
onChanged();
} else {
convsBuilder_.remove(index);
}
return this;
}
/**
* repeated .com.avos.avoscloud.UnreadTuple convs = 1;
*/
public com.avos.avoscloud.Messages.UnreadTuple.Builder getConvsBuilder(
int index) {
return getConvsFieldBuilder().getBuilder(index);
}
/**
* repeated .com.avos.avoscloud.UnreadTuple convs = 1;
*/
public com.avos.avoscloud.Messages.UnreadTupleOrBuilder getConvsOrBuilder(
int index) {
if (convsBuilder_ == null) {
return convs_.get(index); } else {
return convsBuilder_.getMessageOrBuilder(index);
}
}
/**
* repeated .com.avos.avoscloud.UnreadTuple convs = 1;
*/
public java.util.List extends com.avos.avoscloud.Messages.UnreadTupleOrBuilder>
getConvsOrBuilderList() {
if (convsBuilder_ != null) {
return convsBuilder_.getMessageOrBuilderList();
} else {
return java.util.Collections.unmodifiableList(convs_);
}
}
/**
* repeated .com.avos.avoscloud.UnreadTuple convs = 1;
*/
public com.avos.avoscloud.Messages.UnreadTuple.Builder addConvsBuilder() {
return getConvsFieldBuilder().addBuilder(
com.avos.avoscloud.Messages.UnreadTuple.getDefaultInstance());
}
/**
* repeated .com.avos.avoscloud.UnreadTuple convs = 1;
*/
public com.avos.avoscloud.Messages.UnreadTuple.Builder addConvsBuilder(
int index) {
return getConvsFieldBuilder().addBuilder(
index, com.avos.avoscloud.Messages.UnreadTuple.getDefaultInstance());
}
/**
* repeated .com.avos.avoscloud.UnreadTuple convs = 1;
*/
public java.util.List
getConvsBuilderList() {
return getConvsFieldBuilder().getBuilderList();
}
private com.google.protobuf.RepeatedFieldBuilderV3<
com.avos.avoscloud.Messages.UnreadTuple, com.avos.avoscloud.Messages.UnreadTuple.Builder, com.avos.avoscloud.Messages.UnreadTupleOrBuilder>
getConvsFieldBuilder() {
if (convsBuilder_ == null) {
convsBuilder_ = new com.google.protobuf.RepeatedFieldBuilderV3<
com.avos.avoscloud.Messages.UnreadTuple, com.avos.avoscloud.Messages.UnreadTuple.Builder, com.avos.avoscloud.Messages.UnreadTupleOrBuilder>(
convs_,
((bitField0_ & 0x00000001) == 0x00000001),
getParentForChildren(),
isClean());
convs_ = null;
}
return convsBuilder_;
}
private long notifTime_ ;
/**
* optional int64 notifTime = 2;
*/
public boolean hasNotifTime() {
return ((bitField0_ & 0x00000002) == 0x00000002);
}
/**
* optional int64 notifTime = 2;
*/
public long getNotifTime() {
return notifTime_;
}
/**
* optional int64 notifTime = 2;
*/
public Builder setNotifTime(long value) {
bitField0_ |= 0x00000002;
notifTime_ = value;
onChanged();
return this;
}
/**
* optional int64 notifTime = 2;
*/
public Builder clearNotifTime() {
bitField0_ = (bitField0_ & ~0x00000002);
notifTime_ = 0L;
onChanged();
return this;
}
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:com.avos.avoscloud.UnreadCommand)
}
// @@protoc_insertion_point(class_scope:com.avos.avoscloud.UnreadCommand)
private static final com.avos.avoscloud.Messages.UnreadCommand DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new com.avos.avoscloud.Messages.UnreadCommand();
}
public static com.avos.avoscloud.Messages.UnreadCommand getDefaultInstance() {
return DEFAULT_INSTANCE;
}
@java.lang.Deprecated public static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
public UnreadCommand parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new UnreadCommand(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
public com.avos.avoscloud.Messages.UnreadCommand getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface ConvCommandOrBuilder extends
// @@protoc_insertion_point(interface_extends:com.avos.avoscloud.ConvCommand)
com.google.protobuf.MessageOrBuilder {
/**
* repeated string m = 1;
*/
java.util.List
getMList();
/**
* repeated string m = 1;
*/
int getMCount();
/**
* repeated string m = 1;
*/
java.lang.String getM(int index);
/**
* repeated string m = 1;
*/
com.google.protobuf.ByteString
getMBytes(int index);
/**
* optional bool transient = 2;
*/
boolean hasTransient();
/**
* optional bool transient = 2;
*/
boolean getTransient();
/**
* optional bool unique = 3;
*/
boolean hasUnique();
/**
* optional bool unique = 3;
*/
boolean getUnique();
/**
* optional string cid = 4;
*/
boolean hasCid();
/**
* optional string cid = 4;
*/
java.lang.String getCid();
/**
* optional string cid = 4;
*/
com.google.protobuf.ByteString
getCidBytes();
/**
* optional string cdate = 5;
*/
boolean hasCdate();
/**
* optional string cdate = 5;
*/
java.lang.String getCdate();
/**
* optional string cdate = 5;
*/
com.google.protobuf.ByteString
getCdateBytes();
/**
* optional string initBy = 6;
*/
boolean hasInitBy();
/**
* optional string initBy = 6;
*/
java.lang.String getInitBy();
/**
* optional string initBy = 6;
*/
com.google.protobuf.ByteString
getInitByBytes();
/**
* optional string sort = 7;
*/
boolean hasSort();
/**
* optional string sort = 7;
*/
java.lang.String getSort();
/**
* optional string sort = 7;
*/
com.google.protobuf.ByteString
getSortBytes();
/**
* optional int32 limit = 8;
*/
boolean hasLimit();
/**
* optional int32 limit = 8;
*/
int getLimit();
/**
* optional int32 skip = 9;
*/
boolean hasSkip();
/**
* optional int32 skip = 9;
*/
int getSkip();
/**
* optional int32 flag = 10;
*/
boolean hasFlag();
/**
* optional int32 flag = 10;
*/
int getFlag();
/**
* optional int32 count = 11;
*/
boolean hasCount();
/**
* optional int32 count = 11;
*/
int getCount();
/**
* optional string udate = 12;
*/
boolean hasUdate();
/**
* optional string udate = 12;
*/
java.lang.String getUdate();
/**
* optional string udate = 12;
*/
com.google.protobuf.ByteString
getUdateBytes();
/**
* optional int64 t = 13;
*/
boolean hasT();
/**
* optional int64 t = 13;
*/
long getT();
/**
* optional string n = 14;
*/
boolean hasN();
/**
* optional string n = 14;
*/
java.lang.String getN();
/**
* optional string n = 14;
*/
com.google.protobuf.ByteString
getNBytes();
/**
* optional string s = 15;
*/
boolean hasS();
/**
* optional string s = 15;
*/
java.lang.String getS();
/**
* optional string s = 15;
*/
com.google.protobuf.ByteString
getSBytes();
/**
* optional bool statusSub = 16;
*/
boolean hasStatusSub();
/**
* optional bool statusSub = 16;
*/
boolean getStatusSub();
/**
* optional bool statusPub = 17;
*/
boolean hasStatusPub();
/**
* optional bool statusPub = 17;
*/
boolean getStatusPub();
/**
* optional int32 statusTTL = 18;
*/
boolean hasStatusTTL();
/**
* optional int32 statusTTL = 18;
*/
int getStatusTTL();
/**
* optional string uniqueId = 19;
*/
boolean hasUniqueId();
/**
* optional string uniqueId = 19;
*/
java.lang.String getUniqueId();
/**
* optional string uniqueId = 19;
*/
com.google.protobuf.ByteString
getUniqueIdBytes();
/**
* optional string targetClientId = 20;
*/
boolean hasTargetClientId();
/**
* optional string targetClientId = 20;
*/
java.lang.String getTargetClientId();
/**
* optional string targetClientId = 20;
*/
com.google.protobuf.ByteString
getTargetClientIdBytes();
/**
* optional int64 maxReadTimestamp = 21;
*/
boolean hasMaxReadTimestamp();
/**
* optional int64 maxReadTimestamp = 21;
*/
long getMaxReadTimestamp();
/**
* optional int64 maxAckTimestamp = 22;
*/
boolean hasMaxAckTimestamp();
/**
* optional int64 maxAckTimestamp = 22;
*/
long getMaxAckTimestamp();
/**
* optional bool queryAllMembers = 23;
*/
boolean hasQueryAllMembers();
/**
* optional bool queryAllMembers = 23;
*/
boolean getQueryAllMembers();
/**
* repeated .com.avos.avoscloud.MaxReadTuple maxReadTuples = 24;
*/
java.util.List
getMaxReadTuplesList();
/**
* repeated .com.avos.avoscloud.MaxReadTuple maxReadTuples = 24;
*/
com.avos.avoscloud.Messages.MaxReadTuple getMaxReadTuples(int index);
/**
* repeated .com.avos.avoscloud.MaxReadTuple maxReadTuples = 24;
*/
int getMaxReadTuplesCount();
/**
* repeated .com.avos.avoscloud.MaxReadTuple maxReadTuples = 24;
*/
java.util.List extends com.avos.avoscloud.Messages.MaxReadTupleOrBuilder>
getMaxReadTuplesOrBuilderList();
/**
* repeated .com.avos.avoscloud.MaxReadTuple maxReadTuples = 24;
*/
com.avos.avoscloud.Messages.MaxReadTupleOrBuilder getMaxReadTuplesOrBuilder(
int index);
/**
* repeated string cids = 25;
*/
java.util.List
getCidsList();
/**
* repeated string cids = 25;
*/
int getCidsCount();
/**
* repeated string cids = 25;
*/
java.lang.String getCids(int index);
/**
* repeated string cids = 25;
*/
com.google.protobuf.ByteString
getCidsBytes(int index);
/**
* optional .com.avos.avoscloud.ConvMemberInfo info = 26;
*/
boolean hasInfo();
/**
* optional .com.avos.avoscloud.ConvMemberInfo info = 26;
*/
com.avos.avoscloud.Messages.ConvMemberInfo getInfo();
/**
* optional .com.avos.avoscloud.ConvMemberInfo info = 26;
*/
com.avos.avoscloud.Messages.ConvMemberInfoOrBuilder getInfoOrBuilder();
/**
* optional bool tempConv = 27;
*/
boolean hasTempConv();
/**
* optional bool tempConv = 27;
*/
boolean getTempConv();
/**
* optional int32 tempConvTTL = 28;
*/
boolean hasTempConvTTL();
/**
* optional int32 tempConvTTL = 28;
*/
int getTempConvTTL();
/**
* repeated string tempConvIds = 29;
*/
java.util.List
getTempConvIdsList();
/**
* repeated string tempConvIds = 29;
*/
int getTempConvIdsCount();
/**
* repeated string tempConvIds = 29;
*/
java.lang.String getTempConvIds(int index);
/**
* repeated string tempConvIds = 29;
*/
com.google.protobuf.ByteString
getTempConvIdsBytes(int index);
/**
* repeated string allowedPids = 30;
*/
java.util.List
getAllowedPidsList();
/**
* repeated string allowedPids = 30;
*/
int getAllowedPidsCount();
/**
* repeated string allowedPids = 30;
*/
java.lang.String getAllowedPids(int index);
/**
* repeated string allowedPids = 30;
*/
com.google.protobuf.ByteString
getAllowedPidsBytes(int index);
/**
* repeated .com.avos.avoscloud.ErrorCommand failedPids = 31;
*/
java.util.List
getFailedPidsList();
/**
* repeated .com.avos.avoscloud.ErrorCommand failedPids = 31;
*/
com.avos.avoscloud.Messages.ErrorCommand getFailedPids(int index);
/**
* repeated .com.avos.avoscloud.ErrorCommand failedPids = 31;
*/
int getFailedPidsCount();
/**
* repeated .com.avos.avoscloud.ErrorCommand failedPids = 31;
*/
java.util.List extends com.avos.avoscloud.Messages.ErrorCommandOrBuilder>
getFailedPidsOrBuilderList();
/**
* repeated .com.avos.avoscloud.ErrorCommand failedPids = 31;
*/
com.avos.avoscloud.Messages.ErrorCommandOrBuilder getFailedPidsOrBuilder(
int index);
/**
*
* used in shutup query
*
*
* optional string next = 40;
*/
boolean hasNext();
/**
*
* used in shutup query
*
*
* optional string next = 40;
*/
java.lang.String getNext();
/**
*
* used in shutup query
*
*
* optional string next = 40;
*/
com.google.protobuf.ByteString
getNextBytes();
/**
* optional .com.avos.avoscloud.JsonObjectMessage results = 100;
*/
boolean hasResults();
/**
* optional .com.avos.avoscloud.JsonObjectMessage results = 100;
*/
com.avos.avoscloud.Messages.JsonObjectMessage getResults();
/**
* optional .com.avos.avoscloud.JsonObjectMessage results = 100;
*/
com.avos.avoscloud.Messages.JsonObjectMessageOrBuilder getResultsOrBuilder();
/**
* optional .com.avos.avoscloud.JsonObjectMessage where = 101;
*/
boolean hasWhere();
/**
* optional .com.avos.avoscloud.JsonObjectMessage where = 101;
*/
com.avos.avoscloud.Messages.JsonObjectMessage getWhere();
/**
* optional .com.avos.avoscloud.JsonObjectMessage where = 101;
*/
com.avos.avoscloud.Messages.JsonObjectMessageOrBuilder getWhereOrBuilder();
/**
* optional .com.avos.avoscloud.JsonObjectMessage attr = 103;
*/
boolean hasAttr();
/**
* optional .com.avos.avoscloud.JsonObjectMessage attr = 103;
*/
com.avos.avoscloud.Messages.JsonObjectMessage getAttr();
/**
* optional .com.avos.avoscloud.JsonObjectMessage attr = 103;
*/
com.avos.avoscloud.Messages.JsonObjectMessageOrBuilder getAttrOrBuilder();
/**
* optional .com.avos.avoscloud.JsonObjectMessage attrModified = 104;
*/
boolean hasAttrModified();
/**
* optional .com.avos.avoscloud.JsonObjectMessage attrModified = 104;
*/
com.avos.avoscloud.Messages.JsonObjectMessage getAttrModified();
/**
* optional .com.avos.avoscloud.JsonObjectMessage attrModified = 104;
*/
com.avos.avoscloud.Messages.JsonObjectMessageOrBuilder getAttrModifiedOrBuilder();
}
/**
* Protobuf type {@code com.avos.avoscloud.ConvCommand}
*/
public static final class ConvCommand extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:com.avos.avoscloud.ConvCommand)
ConvCommandOrBuilder {
private static final long serialVersionUID = 0L;
// Use ConvCommand.newBuilder() to construct.
private ConvCommand(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private ConvCommand() {
m_ = com.google.protobuf.LazyStringArrayList.EMPTY;
transient_ = false;
unique_ = false;
cid_ = "";
cdate_ = "";
initBy_ = "";
sort_ = "";
limit_ = 0;
skip_ = 0;
flag_ = 0;
count_ = 0;
udate_ = "";
t_ = 0L;
n_ = "";
s_ = "";
statusSub_ = false;
statusPub_ = false;
statusTTL_ = 0;
uniqueId_ = "";
targetClientId_ = "";
maxReadTimestamp_ = 0L;
maxAckTimestamp_ = 0L;
queryAllMembers_ = false;
maxReadTuples_ = java.util.Collections.emptyList();
cids_ = com.google.protobuf.LazyStringArrayList.EMPTY;
tempConv_ = false;
tempConvTTL_ = 0;
tempConvIds_ = com.google.protobuf.LazyStringArrayList.EMPTY;
allowedPids_ = com.google.protobuf.LazyStringArrayList.EMPTY;
failedPids_ = java.util.Collections.emptyList();
next_ = "";
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private ConvCommand(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
int mutable_bitField0_ = 0;
int mutable_bitField1_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!parseUnknownField(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
case 10: {
com.google.protobuf.ByteString bs = input.readBytes();
if (!((mutable_bitField0_ & 0x00000001) == 0x00000001)) {
m_ = new com.google.protobuf.LazyStringArrayList();
mutable_bitField0_ |= 0x00000001;
}
m_.add(bs);
break;
}
case 16: {
bitField0_ |= 0x00000001;
transient_ = input.readBool();
break;
}
case 24: {
bitField0_ |= 0x00000002;
unique_ = input.readBool();
break;
}
case 34: {
com.google.protobuf.ByteString bs = input.readBytes();
bitField0_ |= 0x00000004;
cid_ = bs;
break;
}
case 42: {
com.google.protobuf.ByteString bs = input.readBytes();
bitField0_ |= 0x00000008;
cdate_ = bs;
break;
}
case 50: {
com.google.protobuf.ByteString bs = input.readBytes();
bitField0_ |= 0x00000010;
initBy_ = bs;
break;
}
case 58: {
com.google.protobuf.ByteString bs = input.readBytes();
bitField0_ |= 0x00000020;
sort_ = bs;
break;
}
case 64: {
bitField0_ |= 0x00000040;
limit_ = input.readInt32();
break;
}
case 72: {
bitField0_ |= 0x00000080;
skip_ = input.readInt32();
break;
}
case 80: {
bitField0_ |= 0x00000100;
flag_ = input.readInt32();
break;
}
case 88: {
bitField0_ |= 0x00000200;
count_ = input.readInt32();
break;
}
case 98: {
com.google.protobuf.ByteString bs = input.readBytes();
bitField0_ |= 0x00000400;
udate_ = bs;
break;
}
case 104: {
bitField0_ |= 0x00000800;
t_ = input.readInt64();
break;
}
case 114: {
com.google.protobuf.ByteString bs = input.readBytes();
bitField0_ |= 0x00001000;
n_ = bs;
break;
}
case 122: {
com.google.protobuf.ByteString bs = input.readBytes();
bitField0_ |= 0x00002000;
s_ = bs;
break;
}
case 128: {
bitField0_ |= 0x00004000;
statusSub_ = input.readBool();
break;
}
case 136: {
bitField0_ |= 0x00008000;
statusPub_ = input.readBool();
break;
}
case 144: {
bitField0_ |= 0x00010000;
statusTTL_ = input.readInt32();
break;
}
case 154: {
com.google.protobuf.ByteString bs = input.readBytes();
bitField0_ |= 0x00020000;
uniqueId_ = bs;
break;
}
case 162: {
com.google.protobuf.ByteString bs = input.readBytes();
bitField0_ |= 0x00040000;
targetClientId_ = bs;
break;
}
case 168: {
bitField0_ |= 0x00080000;
maxReadTimestamp_ = input.readInt64();
break;
}
case 176: {
bitField0_ |= 0x00100000;
maxAckTimestamp_ = input.readInt64();
break;
}
case 184: {
bitField0_ |= 0x00200000;
queryAllMembers_ = input.readBool();
break;
}
case 194: {
if (!((mutable_bitField0_ & 0x00800000) == 0x00800000)) {
maxReadTuples_ = new java.util.ArrayList();
mutable_bitField0_ |= 0x00800000;
}
maxReadTuples_.add(
input.readMessage(com.avos.avoscloud.Messages.MaxReadTuple.PARSER, extensionRegistry));
break;
}
case 202: {
com.google.protobuf.ByteString bs = input.readBytes();
if (!((mutable_bitField0_ & 0x01000000) == 0x01000000)) {
cids_ = new com.google.protobuf.LazyStringArrayList();
mutable_bitField0_ |= 0x01000000;
}
cids_.add(bs);
break;
}
case 210: {
com.avos.avoscloud.Messages.ConvMemberInfo.Builder subBuilder = null;
if (((bitField0_ & 0x00400000) == 0x00400000)) {
subBuilder = info_.toBuilder();
}
info_ = input.readMessage(com.avos.avoscloud.Messages.ConvMemberInfo.PARSER, extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(info_);
info_ = subBuilder.buildPartial();
}
bitField0_ |= 0x00400000;
break;
}
case 216: {
bitField0_ |= 0x00800000;
tempConv_ = input.readBool();
break;
}
case 224: {
bitField0_ |= 0x01000000;
tempConvTTL_ = input.readInt32();
break;
}
case 234: {
com.google.protobuf.ByteString bs = input.readBytes();
if (!((mutable_bitField0_ & 0x10000000) == 0x10000000)) {
tempConvIds_ = new com.google.protobuf.LazyStringArrayList();
mutable_bitField0_ |= 0x10000000;
}
tempConvIds_.add(bs);
break;
}
case 242: {
com.google.protobuf.ByteString bs = input.readBytes();
if (!((mutable_bitField0_ & 0x20000000) == 0x20000000)) {
allowedPids_ = new com.google.protobuf.LazyStringArrayList();
mutable_bitField0_ |= 0x20000000;
}
allowedPids_.add(bs);
break;
}
case 250: {
if (!((mutable_bitField0_ & 0x40000000) == 0x40000000)) {
failedPids_ = new java.util.ArrayList();
mutable_bitField0_ |= 0x40000000;
}
failedPids_.add(
input.readMessage(com.avos.avoscloud.Messages.ErrorCommand.PARSER, extensionRegistry));
break;
}
case 322: {
com.google.protobuf.ByteString bs = input.readBytes();
bitField0_ |= 0x02000000;
next_ = bs;
break;
}
case 802: {
com.avos.avoscloud.Messages.JsonObjectMessage.Builder subBuilder = null;
if (((bitField0_ & 0x04000000) == 0x04000000)) {
subBuilder = results_.toBuilder();
}
results_ = input.readMessage(com.avos.avoscloud.Messages.JsonObjectMessage.PARSER, extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(results_);
results_ = subBuilder.buildPartial();
}
bitField0_ |= 0x04000000;
break;
}
case 810: {
com.avos.avoscloud.Messages.JsonObjectMessage.Builder subBuilder = null;
if (((bitField0_ & 0x08000000) == 0x08000000)) {
subBuilder = where_.toBuilder();
}
where_ = input.readMessage(com.avos.avoscloud.Messages.JsonObjectMessage.PARSER, extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(where_);
where_ = subBuilder.buildPartial();
}
bitField0_ |= 0x08000000;
break;
}
case 826: {
com.avos.avoscloud.Messages.JsonObjectMessage.Builder subBuilder = null;
if (((bitField0_ & 0x10000000) == 0x10000000)) {
subBuilder = attr_.toBuilder();
}
attr_ = input.readMessage(com.avos.avoscloud.Messages.JsonObjectMessage.PARSER, extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(attr_);
attr_ = subBuilder.buildPartial();
}
bitField0_ |= 0x10000000;
break;
}
case 834: {
com.avos.avoscloud.Messages.JsonObjectMessage.Builder subBuilder = null;
if (((bitField0_ & 0x20000000) == 0x20000000)) {
subBuilder = attrModified_.toBuilder();
}
attrModified_ = input.readMessage(com.avos.avoscloud.Messages.JsonObjectMessage.PARSER, extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(attrModified_);
attrModified_ = subBuilder.buildPartial();
}
bitField0_ |= 0x20000000;
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
if (((mutable_bitField0_ & 0x00000001) == 0x00000001)) {
m_ = m_.getUnmodifiableView();
}
if (((mutable_bitField0_ & 0x00800000) == 0x00800000)) {
maxReadTuples_ = java.util.Collections.unmodifiableList(maxReadTuples_);
}
if (((mutable_bitField0_ & 0x01000000) == 0x01000000)) {
cids_ = cids_.getUnmodifiableView();
}
if (((mutable_bitField0_ & 0x10000000) == 0x10000000)) {
tempConvIds_ = tempConvIds_.getUnmodifiableView();
}
if (((mutable_bitField0_ & 0x20000000) == 0x20000000)) {
allowedPids_ = allowedPids_.getUnmodifiableView();
}
if (((mutable_bitField0_ & 0x40000000) == 0x40000000)) {
failedPids_ = java.util.Collections.unmodifiableList(failedPids_);
}
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.avos.avoscloud.Messages.internal_static_com_avos_avoscloud_ConvCommand_descriptor;
}
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.avos.avoscloud.Messages.internal_static_com_avos_avoscloud_ConvCommand_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.avos.avoscloud.Messages.ConvCommand.class, com.avos.avoscloud.Messages.ConvCommand.Builder.class);
}
private int bitField0_;
public static final int M_FIELD_NUMBER = 1;
private com.google.protobuf.LazyStringList m_;
/**
* repeated string m = 1;
*/
public com.google.protobuf.ProtocolStringList
getMList() {
return m_;
}
/**
* repeated string m = 1;
*/
public int getMCount() {
return m_.size();
}
/**
* repeated string m = 1;
*/
public java.lang.String getM(int index) {
return m_.get(index);
}
/**
* repeated string m = 1;
*/
public com.google.protobuf.ByteString
getMBytes(int index) {
return m_.getByteString(index);
}
public static final int TRANSIENT_FIELD_NUMBER = 2;
private boolean transient_;
/**
* optional bool transient = 2;
*/
public boolean hasTransient() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
* optional bool transient = 2;
*/
public boolean getTransient() {
return transient_;
}
public static final int UNIQUE_FIELD_NUMBER = 3;
private boolean unique_;
/**
* optional bool unique = 3;
*/
public boolean hasUnique() {
return ((bitField0_ & 0x00000002) == 0x00000002);
}
/**
* optional bool unique = 3;
*/
public boolean getUnique() {
return unique_;
}
public static final int CID_FIELD_NUMBER = 4;
private volatile java.lang.Object cid_;
/**
* optional string cid = 4;
*/
public boolean hasCid() {
return ((bitField0_ & 0x00000004) == 0x00000004);
}
/**
* optional string cid = 4;
*/
public java.lang.String getCid() {
java.lang.Object ref = cid_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
cid_ = s;
}
return s;
}
}
/**
* optional string cid = 4;
*/
public com.google.protobuf.ByteString
getCidBytes() {
java.lang.Object ref = cid_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
cid_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int CDATE_FIELD_NUMBER = 5;
private volatile java.lang.Object cdate_;
/**
* optional string cdate = 5;
*/
public boolean hasCdate() {
return ((bitField0_ & 0x00000008) == 0x00000008);
}
/**
* optional string cdate = 5;
*/
public java.lang.String getCdate() {
java.lang.Object ref = cdate_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
cdate_ = s;
}
return s;
}
}
/**
* optional string cdate = 5;
*/
public com.google.protobuf.ByteString
getCdateBytes() {
java.lang.Object ref = cdate_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
cdate_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int INITBY_FIELD_NUMBER = 6;
private volatile java.lang.Object initBy_;
/**
* optional string initBy = 6;
*/
public boolean hasInitBy() {
return ((bitField0_ & 0x00000010) == 0x00000010);
}
/**
* optional string initBy = 6;
*/
public java.lang.String getInitBy() {
java.lang.Object ref = initBy_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
initBy_ = s;
}
return s;
}
}
/**
* optional string initBy = 6;
*/
public com.google.protobuf.ByteString
getInitByBytes() {
java.lang.Object ref = initBy_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
initBy_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int SORT_FIELD_NUMBER = 7;
private volatile java.lang.Object sort_;
/**
* optional string sort = 7;
*/
public boolean hasSort() {
return ((bitField0_ & 0x00000020) == 0x00000020);
}
/**
* optional string sort = 7;
*/
public java.lang.String getSort() {
java.lang.Object ref = sort_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
sort_ = s;
}
return s;
}
}
/**
* optional string sort = 7;
*/
public com.google.protobuf.ByteString
getSortBytes() {
java.lang.Object ref = sort_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
sort_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int LIMIT_FIELD_NUMBER = 8;
private int limit_;
/**
* optional int32 limit = 8;
*/
public boolean hasLimit() {
return ((bitField0_ & 0x00000040) == 0x00000040);
}
/**
* optional int32 limit = 8;
*/
public int getLimit() {
return limit_;
}
public static final int SKIP_FIELD_NUMBER = 9;
private int skip_;
/**
* optional int32 skip = 9;
*/
public boolean hasSkip() {
return ((bitField0_ & 0x00000080) == 0x00000080);
}
/**
* optional int32 skip = 9;
*/
public int getSkip() {
return skip_;
}
public static final int FLAG_FIELD_NUMBER = 10;
private int flag_;
/**
* optional int32 flag = 10;
*/
public boolean hasFlag() {
return ((bitField0_ & 0x00000100) == 0x00000100);
}
/**
* optional int32 flag = 10;
*/
public int getFlag() {
return flag_;
}
public static final int COUNT_FIELD_NUMBER = 11;
private int count_;
/**
* optional int32 count = 11;
*/
public boolean hasCount() {
return ((bitField0_ & 0x00000200) == 0x00000200);
}
/**
* optional int32 count = 11;
*/
public int getCount() {
return count_;
}
public static final int UDATE_FIELD_NUMBER = 12;
private volatile java.lang.Object udate_;
/**
* optional string udate = 12;
*/
public boolean hasUdate() {
return ((bitField0_ & 0x00000400) == 0x00000400);
}
/**
* optional string udate = 12;
*/
public java.lang.String getUdate() {
java.lang.Object ref = udate_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
udate_ = s;
}
return s;
}
}
/**
* optional string udate = 12;
*/
public com.google.protobuf.ByteString
getUdateBytes() {
java.lang.Object ref = udate_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
udate_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int T_FIELD_NUMBER = 13;
private long t_;
/**
* optional int64 t = 13;
*/
public boolean hasT() {
return ((bitField0_ & 0x00000800) == 0x00000800);
}
/**
* optional int64 t = 13;
*/
public long getT() {
return t_;
}
public static final int N_FIELD_NUMBER = 14;
private volatile java.lang.Object n_;
/**
* optional string n = 14;
*/
public boolean hasN() {
return ((bitField0_ & 0x00001000) == 0x00001000);
}
/**
* optional string n = 14;
*/
public java.lang.String getN() {
java.lang.Object ref = n_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
n_ = s;
}
return s;
}
}
/**
* optional string n = 14;
*/
public com.google.protobuf.ByteString
getNBytes() {
java.lang.Object ref = n_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
n_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int S_FIELD_NUMBER = 15;
private volatile java.lang.Object s_;
/**
* optional string s = 15;
*/
public boolean hasS() {
return ((bitField0_ & 0x00002000) == 0x00002000);
}
/**
* optional string s = 15;
*/
public java.lang.String getS() {
java.lang.Object ref = s_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
s_ = s;
}
return s;
}
}
/**
* optional string s = 15;
*/
public com.google.protobuf.ByteString
getSBytes() {
java.lang.Object ref = s_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
s_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int STATUSSUB_FIELD_NUMBER = 16;
private boolean statusSub_;
/**
* optional bool statusSub = 16;
*/
public boolean hasStatusSub() {
return ((bitField0_ & 0x00004000) == 0x00004000);
}
/**
* optional bool statusSub = 16;
*/
public boolean getStatusSub() {
return statusSub_;
}
public static final int STATUSPUB_FIELD_NUMBER = 17;
private boolean statusPub_;
/**
* optional bool statusPub = 17;
*/
public boolean hasStatusPub() {
return ((bitField0_ & 0x00008000) == 0x00008000);
}
/**
* optional bool statusPub = 17;
*/
public boolean getStatusPub() {
return statusPub_;
}
public static final int STATUSTTL_FIELD_NUMBER = 18;
private int statusTTL_;
/**
* optional int32 statusTTL = 18;
*/
public boolean hasStatusTTL() {
return ((bitField0_ & 0x00010000) == 0x00010000);
}
/**
* optional int32 statusTTL = 18;
*/
public int getStatusTTL() {
return statusTTL_;
}
public static final int UNIQUEID_FIELD_NUMBER = 19;
private volatile java.lang.Object uniqueId_;
/**
* optional string uniqueId = 19;
*/
public boolean hasUniqueId() {
return ((bitField0_ & 0x00020000) == 0x00020000);
}
/**
* optional string uniqueId = 19;
*/
public java.lang.String getUniqueId() {
java.lang.Object ref = uniqueId_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
uniqueId_ = s;
}
return s;
}
}
/**
* optional string uniqueId = 19;
*/
public com.google.protobuf.ByteString
getUniqueIdBytes() {
java.lang.Object ref = uniqueId_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
uniqueId_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int TARGETCLIENTID_FIELD_NUMBER = 20;
private volatile java.lang.Object targetClientId_;
/**
* optional string targetClientId = 20;
*/
public boolean hasTargetClientId() {
return ((bitField0_ & 0x00040000) == 0x00040000);
}
/**
* optional string targetClientId = 20;
*/
public java.lang.String getTargetClientId() {
java.lang.Object ref = targetClientId_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
targetClientId_ = s;
}
return s;
}
}
/**
* optional string targetClientId = 20;
*/
public com.google.protobuf.ByteString
getTargetClientIdBytes() {
java.lang.Object ref = targetClientId_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
targetClientId_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int MAXREADTIMESTAMP_FIELD_NUMBER = 21;
private long maxReadTimestamp_;
/**
* optional int64 maxReadTimestamp = 21;
*/
public boolean hasMaxReadTimestamp() {
return ((bitField0_ & 0x00080000) == 0x00080000);
}
/**
* optional int64 maxReadTimestamp = 21;
*/
public long getMaxReadTimestamp() {
return maxReadTimestamp_;
}
public static final int MAXACKTIMESTAMP_FIELD_NUMBER = 22;
private long maxAckTimestamp_;
/**
* optional int64 maxAckTimestamp = 22;
*/
public boolean hasMaxAckTimestamp() {
return ((bitField0_ & 0x00100000) == 0x00100000);
}
/**
* optional int64 maxAckTimestamp = 22;
*/
public long getMaxAckTimestamp() {
return maxAckTimestamp_;
}
public static final int QUERYALLMEMBERS_FIELD_NUMBER = 23;
private boolean queryAllMembers_;
/**
* optional bool queryAllMembers = 23;
*/
public boolean hasQueryAllMembers() {
return ((bitField0_ & 0x00200000) == 0x00200000);
}
/**
* optional bool queryAllMembers = 23;
*/
public boolean getQueryAllMembers() {
return queryAllMembers_;
}
public static final int MAXREADTUPLES_FIELD_NUMBER = 24;
private java.util.List maxReadTuples_;
/**
* repeated .com.avos.avoscloud.MaxReadTuple maxReadTuples = 24;
*/
public java.util.List getMaxReadTuplesList() {
return maxReadTuples_;
}
/**
* repeated .com.avos.avoscloud.MaxReadTuple maxReadTuples = 24;
*/
public java.util.List extends com.avos.avoscloud.Messages.MaxReadTupleOrBuilder>
getMaxReadTuplesOrBuilderList() {
return maxReadTuples_;
}
/**
* repeated .com.avos.avoscloud.MaxReadTuple maxReadTuples = 24;
*/
public int getMaxReadTuplesCount() {
return maxReadTuples_.size();
}
/**
* repeated .com.avos.avoscloud.MaxReadTuple maxReadTuples = 24;
*/
public com.avos.avoscloud.Messages.MaxReadTuple getMaxReadTuples(int index) {
return maxReadTuples_.get(index);
}
/**
* repeated .com.avos.avoscloud.MaxReadTuple maxReadTuples = 24;
*/
public com.avos.avoscloud.Messages.MaxReadTupleOrBuilder getMaxReadTuplesOrBuilder(
int index) {
return maxReadTuples_.get(index);
}
public static final int CIDS_FIELD_NUMBER = 25;
private com.google.protobuf.LazyStringList cids_;
/**
* repeated string cids = 25;
*/
public com.google.protobuf.ProtocolStringList
getCidsList() {
return cids_;
}
/**
* repeated string cids = 25;
*/
public int getCidsCount() {
return cids_.size();
}
/**
* repeated string cids = 25;
*/
public java.lang.String getCids(int index) {
return cids_.get(index);
}
/**
* repeated string cids = 25;
*/
public com.google.protobuf.ByteString
getCidsBytes(int index) {
return cids_.getByteString(index);
}
public static final int INFO_FIELD_NUMBER = 26;
private com.avos.avoscloud.Messages.ConvMemberInfo info_;
/**
* optional .com.avos.avoscloud.ConvMemberInfo info = 26;
*/
public boolean hasInfo() {
return ((bitField0_ & 0x00400000) == 0x00400000);
}
/**
* optional .com.avos.avoscloud.ConvMemberInfo info = 26;
*/
public com.avos.avoscloud.Messages.ConvMemberInfo getInfo() {
return info_ == null ? com.avos.avoscloud.Messages.ConvMemberInfo.getDefaultInstance() : info_;
}
/**
* optional .com.avos.avoscloud.ConvMemberInfo info = 26;
*/
public com.avos.avoscloud.Messages.ConvMemberInfoOrBuilder getInfoOrBuilder() {
return info_ == null ? com.avos.avoscloud.Messages.ConvMemberInfo.getDefaultInstance() : info_;
}
public static final int TEMPCONV_FIELD_NUMBER = 27;
private boolean tempConv_;
/**
* optional bool tempConv = 27;
*/
public boolean hasTempConv() {
return ((bitField0_ & 0x00800000) == 0x00800000);
}
/**
* optional bool tempConv = 27;
*/
public boolean getTempConv() {
return tempConv_;
}
public static final int TEMPCONVTTL_FIELD_NUMBER = 28;
private int tempConvTTL_;
/**
* optional int32 tempConvTTL = 28;
*/
public boolean hasTempConvTTL() {
return ((bitField0_ & 0x01000000) == 0x01000000);
}
/**
* optional int32 tempConvTTL = 28;
*/
public int getTempConvTTL() {
return tempConvTTL_;
}
public static final int TEMPCONVIDS_FIELD_NUMBER = 29;
private com.google.protobuf.LazyStringList tempConvIds_;
/**
* repeated string tempConvIds = 29;
*/
public com.google.protobuf.ProtocolStringList
getTempConvIdsList() {
return tempConvIds_;
}
/**
* repeated string tempConvIds = 29;
*/
public int getTempConvIdsCount() {
return tempConvIds_.size();
}
/**
* repeated string tempConvIds = 29;
*/
public java.lang.String getTempConvIds(int index) {
return tempConvIds_.get(index);
}
/**
* repeated string tempConvIds = 29;
*/
public com.google.protobuf.ByteString
getTempConvIdsBytes(int index) {
return tempConvIds_.getByteString(index);
}
public static final int ALLOWEDPIDS_FIELD_NUMBER = 30;
private com.google.protobuf.LazyStringList allowedPids_;
/**
* repeated string allowedPids = 30;
*/
public com.google.protobuf.ProtocolStringList
getAllowedPidsList() {
return allowedPids_;
}
/**
* repeated string allowedPids = 30;
*/
public int getAllowedPidsCount() {
return allowedPids_.size();
}
/**
* repeated string allowedPids = 30;
*/
public java.lang.String getAllowedPids(int index) {
return allowedPids_.get(index);
}
/**
* repeated string allowedPids = 30;
*/
public com.google.protobuf.ByteString
getAllowedPidsBytes(int index) {
return allowedPids_.getByteString(index);
}
public static final int FAILEDPIDS_FIELD_NUMBER = 31;
private java.util.List failedPids_;
/**
* repeated .com.avos.avoscloud.ErrorCommand failedPids = 31;
*/
public java.util.List getFailedPidsList() {
return failedPids_;
}
/**
* repeated .com.avos.avoscloud.ErrorCommand failedPids = 31;
*/
public java.util.List extends com.avos.avoscloud.Messages.ErrorCommandOrBuilder>
getFailedPidsOrBuilderList() {
return failedPids_;
}
/**
* repeated .com.avos.avoscloud.ErrorCommand failedPids = 31;
*/
public int getFailedPidsCount() {
return failedPids_.size();
}
/**
* repeated .com.avos.avoscloud.ErrorCommand failedPids = 31;
*/
public com.avos.avoscloud.Messages.ErrorCommand getFailedPids(int index) {
return failedPids_.get(index);
}
/**
* repeated .com.avos.avoscloud.ErrorCommand failedPids = 31;
*/
public com.avos.avoscloud.Messages.ErrorCommandOrBuilder getFailedPidsOrBuilder(
int index) {
return failedPids_.get(index);
}
public static final int NEXT_FIELD_NUMBER = 40;
private volatile java.lang.Object next_;
/**
*
* used in shutup query
*
*
* optional string next = 40;
*/
public boolean hasNext() {
return ((bitField0_ & 0x02000000) == 0x02000000);
}
/**
*
* used in shutup query
*
*
* optional string next = 40;
*/
public java.lang.String getNext() {
java.lang.Object ref = next_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
next_ = s;
}
return s;
}
}
/**
*
* used in shutup query
*
*
* optional string next = 40;
*/
public com.google.protobuf.ByteString
getNextBytes() {
java.lang.Object ref = next_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
next_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int RESULTS_FIELD_NUMBER = 100;
private com.avos.avoscloud.Messages.JsonObjectMessage results_;
/**
* optional .com.avos.avoscloud.JsonObjectMessage results = 100;
*/
public boolean hasResults() {
return ((bitField0_ & 0x04000000) == 0x04000000);
}
/**
* optional .com.avos.avoscloud.JsonObjectMessage results = 100;
*/
public com.avos.avoscloud.Messages.JsonObjectMessage getResults() {
return results_ == null ? com.avos.avoscloud.Messages.JsonObjectMessage.getDefaultInstance() : results_;
}
/**
* optional .com.avos.avoscloud.JsonObjectMessage results = 100;
*/
public com.avos.avoscloud.Messages.JsonObjectMessageOrBuilder getResultsOrBuilder() {
return results_ == null ? com.avos.avoscloud.Messages.JsonObjectMessage.getDefaultInstance() : results_;
}
public static final int WHERE_FIELD_NUMBER = 101;
private com.avos.avoscloud.Messages.JsonObjectMessage where_;
/**
* optional .com.avos.avoscloud.JsonObjectMessage where = 101;
*/
public boolean hasWhere() {
return ((bitField0_ & 0x08000000) == 0x08000000);
}
/**
* optional .com.avos.avoscloud.JsonObjectMessage where = 101;
*/
public com.avos.avoscloud.Messages.JsonObjectMessage getWhere() {
return where_ == null ? com.avos.avoscloud.Messages.JsonObjectMessage.getDefaultInstance() : where_;
}
/**
* optional .com.avos.avoscloud.JsonObjectMessage where = 101;
*/
public com.avos.avoscloud.Messages.JsonObjectMessageOrBuilder getWhereOrBuilder() {
return where_ == null ? com.avos.avoscloud.Messages.JsonObjectMessage.getDefaultInstance() : where_;
}
public static final int ATTR_FIELD_NUMBER = 103;
private com.avos.avoscloud.Messages.JsonObjectMessage attr_;
/**
* optional .com.avos.avoscloud.JsonObjectMessage attr = 103;
*/
public boolean hasAttr() {
return ((bitField0_ & 0x10000000) == 0x10000000);
}
/**
* optional .com.avos.avoscloud.JsonObjectMessage attr = 103;
*/
public com.avos.avoscloud.Messages.JsonObjectMessage getAttr() {
return attr_ == null ? com.avos.avoscloud.Messages.JsonObjectMessage.getDefaultInstance() : attr_;
}
/**
* optional .com.avos.avoscloud.JsonObjectMessage attr = 103;
*/
public com.avos.avoscloud.Messages.JsonObjectMessageOrBuilder getAttrOrBuilder() {
return attr_ == null ? com.avos.avoscloud.Messages.JsonObjectMessage.getDefaultInstance() : attr_;
}
public static final int ATTRMODIFIED_FIELD_NUMBER = 104;
private com.avos.avoscloud.Messages.JsonObjectMessage attrModified_;
/**
* optional .com.avos.avoscloud.JsonObjectMessage attrModified = 104;
*/
public boolean hasAttrModified() {
return ((bitField0_ & 0x20000000) == 0x20000000);
}
/**
* optional .com.avos.avoscloud.JsonObjectMessage attrModified = 104;
*/
public com.avos.avoscloud.Messages.JsonObjectMessage getAttrModified() {
return attrModified_ == null ? com.avos.avoscloud.Messages.JsonObjectMessage.getDefaultInstance() : attrModified_;
}
/**
* optional .com.avos.avoscloud.JsonObjectMessage attrModified = 104;
*/
public com.avos.avoscloud.Messages.JsonObjectMessageOrBuilder getAttrModifiedOrBuilder() {
return attrModified_ == null ? com.avos.avoscloud.Messages.JsonObjectMessage.getDefaultInstance() : attrModified_;
}
private byte memoizedIsInitialized = -1;
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
for (int i = 0; i < getFailedPidsCount(); i++) {
if (!getFailedPids(i).isInitialized()) {
memoizedIsInitialized = 0;
return false;
}
}
if (hasResults()) {
if (!getResults().isInitialized()) {
memoizedIsInitialized = 0;
return false;
}
}
if (hasWhere()) {
if (!getWhere().isInitialized()) {
memoizedIsInitialized = 0;
return false;
}
}
if (hasAttr()) {
if (!getAttr().isInitialized()) {
memoizedIsInitialized = 0;
return false;
}
}
if (hasAttrModified()) {
if (!getAttrModified().isInitialized()) {
memoizedIsInitialized = 0;
return false;
}
}
memoizedIsInitialized = 1;
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
for (int i = 0; i < m_.size(); i++) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 1, m_.getRaw(i));
}
if (((bitField0_ & 0x00000001) == 0x00000001)) {
output.writeBool(2, transient_);
}
if (((bitField0_ & 0x00000002) == 0x00000002)) {
output.writeBool(3, unique_);
}
if (((bitField0_ & 0x00000004) == 0x00000004)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 4, cid_);
}
if (((bitField0_ & 0x00000008) == 0x00000008)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 5, cdate_);
}
if (((bitField0_ & 0x00000010) == 0x00000010)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 6, initBy_);
}
if (((bitField0_ & 0x00000020) == 0x00000020)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 7, sort_);
}
if (((bitField0_ & 0x00000040) == 0x00000040)) {
output.writeInt32(8, limit_);
}
if (((bitField0_ & 0x00000080) == 0x00000080)) {
output.writeInt32(9, skip_);
}
if (((bitField0_ & 0x00000100) == 0x00000100)) {
output.writeInt32(10, flag_);
}
if (((bitField0_ & 0x00000200) == 0x00000200)) {
output.writeInt32(11, count_);
}
if (((bitField0_ & 0x00000400) == 0x00000400)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 12, udate_);
}
if (((bitField0_ & 0x00000800) == 0x00000800)) {
output.writeInt64(13, t_);
}
if (((bitField0_ & 0x00001000) == 0x00001000)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 14, n_);
}
if (((bitField0_ & 0x00002000) == 0x00002000)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 15, s_);
}
if (((bitField0_ & 0x00004000) == 0x00004000)) {
output.writeBool(16, statusSub_);
}
if (((bitField0_ & 0x00008000) == 0x00008000)) {
output.writeBool(17, statusPub_);
}
if (((bitField0_ & 0x00010000) == 0x00010000)) {
output.writeInt32(18, statusTTL_);
}
if (((bitField0_ & 0x00020000) == 0x00020000)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 19, uniqueId_);
}
if (((bitField0_ & 0x00040000) == 0x00040000)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 20, targetClientId_);
}
if (((bitField0_ & 0x00080000) == 0x00080000)) {
output.writeInt64(21, maxReadTimestamp_);
}
if (((bitField0_ & 0x00100000) == 0x00100000)) {
output.writeInt64(22, maxAckTimestamp_);
}
if (((bitField0_ & 0x00200000) == 0x00200000)) {
output.writeBool(23, queryAllMembers_);
}
for (int i = 0; i < maxReadTuples_.size(); i++) {
output.writeMessage(24, maxReadTuples_.get(i));
}
for (int i = 0; i < cids_.size(); i++) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 25, cids_.getRaw(i));
}
if (((bitField0_ & 0x00400000) == 0x00400000)) {
output.writeMessage(26, getInfo());
}
if (((bitField0_ & 0x00800000) == 0x00800000)) {
output.writeBool(27, tempConv_);
}
if (((bitField0_ & 0x01000000) == 0x01000000)) {
output.writeInt32(28, tempConvTTL_);
}
for (int i = 0; i < tempConvIds_.size(); i++) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 29, tempConvIds_.getRaw(i));
}
for (int i = 0; i < allowedPids_.size(); i++) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 30, allowedPids_.getRaw(i));
}
for (int i = 0; i < failedPids_.size(); i++) {
output.writeMessage(31, failedPids_.get(i));
}
if (((bitField0_ & 0x02000000) == 0x02000000)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 40, next_);
}
if (((bitField0_ & 0x04000000) == 0x04000000)) {
output.writeMessage(100, getResults());
}
if (((bitField0_ & 0x08000000) == 0x08000000)) {
output.writeMessage(101, getWhere());
}
if (((bitField0_ & 0x10000000) == 0x10000000)) {
output.writeMessage(103, getAttr());
}
if (((bitField0_ & 0x20000000) == 0x20000000)) {
output.writeMessage(104, getAttrModified());
}
unknownFields.writeTo(output);
}
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
{
int dataSize = 0;
for (int i = 0; i < m_.size(); i++) {
dataSize += computeStringSizeNoTag(m_.getRaw(i));
}
size += dataSize;
size += 1 * getMList().size();
}
if (((bitField0_ & 0x00000001) == 0x00000001)) {
size += com.google.protobuf.CodedOutputStream
.computeBoolSize(2, transient_);
}
if (((bitField0_ & 0x00000002) == 0x00000002)) {
size += com.google.protobuf.CodedOutputStream
.computeBoolSize(3, unique_);
}
if (((bitField0_ & 0x00000004) == 0x00000004)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(4, cid_);
}
if (((bitField0_ & 0x00000008) == 0x00000008)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(5, cdate_);
}
if (((bitField0_ & 0x00000010) == 0x00000010)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(6, initBy_);
}
if (((bitField0_ & 0x00000020) == 0x00000020)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(7, sort_);
}
if (((bitField0_ & 0x00000040) == 0x00000040)) {
size += com.google.protobuf.CodedOutputStream
.computeInt32Size(8, limit_);
}
if (((bitField0_ & 0x00000080) == 0x00000080)) {
size += com.google.protobuf.CodedOutputStream
.computeInt32Size(9, skip_);
}
if (((bitField0_ & 0x00000100) == 0x00000100)) {
size += com.google.protobuf.CodedOutputStream
.computeInt32Size(10, flag_);
}
if (((bitField0_ & 0x00000200) == 0x00000200)) {
size += com.google.protobuf.CodedOutputStream
.computeInt32Size(11, count_);
}
if (((bitField0_ & 0x00000400) == 0x00000400)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(12, udate_);
}
if (((bitField0_ & 0x00000800) == 0x00000800)) {
size += com.google.protobuf.CodedOutputStream
.computeInt64Size(13, t_);
}
if (((bitField0_ & 0x00001000) == 0x00001000)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(14, n_);
}
if (((bitField0_ & 0x00002000) == 0x00002000)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(15, s_);
}
if (((bitField0_ & 0x00004000) == 0x00004000)) {
size += com.google.protobuf.CodedOutputStream
.computeBoolSize(16, statusSub_);
}
if (((bitField0_ & 0x00008000) == 0x00008000)) {
size += com.google.protobuf.CodedOutputStream
.computeBoolSize(17, statusPub_);
}
if (((bitField0_ & 0x00010000) == 0x00010000)) {
size += com.google.protobuf.CodedOutputStream
.computeInt32Size(18, statusTTL_);
}
if (((bitField0_ & 0x00020000) == 0x00020000)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(19, uniqueId_);
}
if (((bitField0_ & 0x00040000) == 0x00040000)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(20, targetClientId_);
}
if (((bitField0_ & 0x00080000) == 0x00080000)) {
size += com.google.protobuf.CodedOutputStream
.computeInt64Size(21, maxReadTimestamp_);
}
if (((bitField0_ & 0x00100000) == 0x00100000)) {
size += com.google.protobuf.CodedOutputStream
.computeInt64Size(22, maxAckTimestamp_);
}
if (((bitField0_ & 0x00200000) == 0x00200000)) {
size += com.google.protobuf.CodedOutputStream
.computeBoolSize(23, queryAllMembers_);
}
for (int i = 0; i < maxReadTuples_.size(); i++) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(24, maxReadTuples_.get(i));
}
{
int dataSize = 0;
for (int i = 0; i < cids_.size(); i++) {
dataSize += computeStringSizeNoTag(cids_.getRaw(i));
}
size += dataSize;
size += 2 * getCidsList().size();
}
if (((bitField0_ & 0x00400000) == 0x00400000)) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(26, getInfo());
}
if (((bitField0_ & 0x00800000) == 0x00800000)) {
size += com.google.protobuf.CodedOutputStream
.computeBoolSize(27, tempConv_);
}
if (((bitField0_ & 0x01000000) == 0x01000000)) {
size += com.google.protobuf.CodedOutputStream
.computeInt32Size(28, tempConvTTL_);
}
{
int dataSize = 0;
for (int i = 0; i < tempConvIds_.size(); i++) {
dataSize += computeStringSizeNoTag(tempConvIds_.getRaw(i));
}
size += dataSize;
size += 2 * getTempConvIdsList().size();
}
{
int dataSize = 0;
for (int i = 0; i < allowedPids_.size(); i++) {
dataSize += computeStringSizeNoTag(allowedPids_.getRaw(i));
}
size += dataSize;
size += 2 * getAllowedPidsList().size();
}
for (int i = 0; i < failedPids_.size(); i++) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(31, failedPids_.get(i));
}
if (((bitField0_ & 0x02000000) == 0x02000000)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(40, next_);
}
if (((bitField0_ & 0x04000000) == 0x04000000)) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(100, getResults());
}
if (((bitField0_ & 0x08000000) == 0x08000000)) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(101, getWhere());
}
if (((bitField0_ & 0x10000000) == 0x10000000)) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(103, getAttr());
}
if (((bitField0_ & 0x20000000) == 0x20000000)) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(104, getAttrModified());
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof com.avos.avoscloud.Messages.ConvCommand)) {
return super.equals(obj);
}
com.avos.avoscloud.Messages.ConvCommand other = (com.avos.avoscloud.Messages.ConvCommand) obj;
boolean result = true;
result = result && getMList()
.equals(other.getMList());
result = result && (hasTransient() == other.hasTransient());
if (hasTransient()) {
result = result && (getTransient()
== other.getTransient());
}
result = result && (hasUnique() == other.hasUnique());
if (hasUnique()) {
result = result && (getUnique()
== other.getUnique());
}
result = result && (hasCid() == other.hasCid());
if (hasCid()) {
result = result && getCid()
.equals(other.getCid());
}
result = result && (hasCdate() == other.hasCdate());
if (hasCdate()) {
result = result && getCdate()
.equals(other.getCdate());
}
result = result && (hasInitBy() == other.hasInitBy());
if (hasInitBy()) {
result = result && getInitBy()
.equals(other.getInitBy());
}
result = result && (hasSort() == other.hasSort());
if (hasSort()) {
result = result && getSort()
.equals(other.getSort());
}
result = result && (hasLimit() == other.hasLimit());
if (hasLimit()) {
result = result && (getLimit()
== other.getLimit());
}
result = result && (hasSkip() == other.hasSkip());
if (hasSkip()) {
result = result && (getSkip()
== other.getSkip());
}
result = result && (hasFlag() == other.hasFlag());
if (hasFlag()) {
result = result && (getFlag()
== other.getFlag());
}
result = result && (hasCount() == other.hasCount());
if (hasCount()) {
result = result && (getCount()
== other.getCount());
}
result = result && (hasUdate() == other.hasUdate());
if (hasUdate()) {
result = result && getUdate()
.equals(other.getUdate());
}
result = result && (hasT() == other.hasT());
if (hasT()) {
result = result && (getT()
== other.getT());
}
result = result && (hasN() == other.hasN());
if (hasN()) {
result = result && getN()
.equals(other.getN());
}
result = result && (hasS() == other.hasS());
if (hasS()) {
result = result && getS()
.equals(other.getS());
}
result = result && (hasStatusSub() == other.hasStatusSub());
if (hasStatusSub()) {
result = result && (getStatusSub()
== other.getStatusSub());
}
result = result && (hasStatusPub() == other.hasStatusPub());
if (hasStatusPub()) {
result = result && (getStatusPub()
== other.getStatusPub());
}
result = result && (hasStatusTTL() == other.hasStatusTTL());
if (hasStatusTTL()) {
result = result && (getStatusTTL()
== other.getStatusTTL());
}
result = result && (hasUniqueId() == other.hasUniqueId());
if (hasUniqueId()) {
result = result && getUniqueId()
.equals(other.getUniqueId());
}
result = result && (hasTargetClientId() == other.hasTargetClientId());
if (hasTargetClientId()) {
result = result && getTargetClientId()
.equals(other.getTargetClientId());
}
result = result && (hasMaxReadTimestamp() == other.hasMaxReadTimestamp());
if (hasMaxReadTimestamp()) {
result = result && (getMaxReadTimestamp()
== other.getMaxReadTimestamp());
}
result = result && (hasMaxAckTimestamp() == other.hasMaxAckTimestamp());
if (hasMaxAckTimestamp()) {
result = result && (getMaxAckTimestamp()
== other.getMaxAckTimestamp());
}
result = result && (hasQueryAllMembers() == other.hasQueryAllMembers());
if (hasQueryAllMembers()) {
result = result && (getQueryAllMembers()
== other.getQueryAllMembers());
}
result = result && getMaxReadTuplesList()
.equals(other.getMaxReadTuplesList());
result = result && getCidsList()
.equals(other.getCidsList());
result = result && (hasInfo() == other.hasInfo());
if (hasInfo()) {
result = result && getInfo()
.equals(other.getInfo());
}
result = result && (hasTempConv() == other.hasTempConv());
if (hasTempConv()) {
result = result && (getTempConv()
== other.getTempConv());
}
result = result && (hasTempConvTTL() == other.hasTempConvTTL());
if (hasTempConvTTL()) {
result = result && (getTempConvTTL()
== other.getTempConvTTL());
}
result = result && getTempConvIdsList()
.equals(other.getTempConvIdsList());
result = result && getAllowedPidsList()
.equals(other.getAllowedPidsList());
result = result && getFailedPidsList()
.equals(other.getFailedPidsList());
result = result && (hasNext() == other.hasNext());
if (hasNext()) {
result = result && getNext()
.equals(other.getNext());
}
result = result && (hasResults() == other.hasResults());
if (hasResults()) {
result = result && getResults()
.equals(other.getResults());
}
result = result && (hasWhere() == other.hasWhere());
if (hasWhere()) {
result = result && getWhere()
.equals(other.getWhere());
}
result = result && (hasAttr() == other.hasAttr());
if (hasAttr()) {
result = result && getAttr()
.equals(other.getAttr());
}
result = result && (hasAttrModified() == other.hasAttrModified());
if (hasAttrModified()) {
result = result && getAttrModified()
.equals(other.getAttrModified());
}
result = result && unknownFields.equals(other.unknownFields);
return result;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (getMCount() > 0) {
hash = (37 * hash) + M_FIELD_NUMBER;
hash = (53 * hash) + getMList().hashCode();
}
if (hasTransient()) {
hash = (37 * hash) + TRANSIENT_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashBoolean(
getTransient());
}
if (hasUnique()) {
hash = (37 * hash) + UNIQUE_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashBoolean(
getUnique());
}
if (hasCid()) {
hash = (37 * hash) + CID_FIELD_NUMBER;
hash = (53 * hash) + getCid().hashCode();
}
if (hasCdate()) {
hash = (37 * hash) + CDATE_FIELD_NUMBER;
hash = (53 * hash) + getCdate().hashCode();
}
if (hasInitBy()) {
hash = (37 * hash) + INITBY_FIELD_NUMBER;
hash = (53 * hash) + getInitBy().hashCode();
}
if (hasSort()) {
hash = (37 * hash) + SORT_FIELD_NUMBER;
hash = (53 * hash) + getSort().hashCode();
}
if (hasLimit()) {
hash = (37 * hash) + LIMIT_FIELD_NUMBER;
hash = (53 * hash) + getLimit();
}
if (hasSkip()) {
hash = (37 * hash) + SKIP_FIELD_NUMBER;
hash = (53 * hash) + getSkip();
}
if (hasFlag()) {
hash = (37 * hash) + FLAG_FIELD_NUMBER;
hash = (53 * hash) + getFlag();
}
if (hasCount()) {
hash = (37 * hash) + COUNT_FIELD_NUMBER;
hash = (53 * hash) + getCount();
}
if (hasUdate()) {
hash = (37 * hash) + UDATE_FIELD_NUMBER;
hash = (53 * hash) + getUdate().hashCode();
}
if (hasT()) {
hash = (37 * hash) + T_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getT());
}
if (hasN()) {
hash = (37 * hash) + N_FIELD_NUMBER;
hash = (53 * hash) + getN().hashCode();
}
if (hasS()) {
hash = (37 * hash) + S_FIELD_NUMBER;
hash = (53 * hash) + getS().hashCode();
}
if (hasStatusSub()) {
hash = (37 * hash) + STATUSSUB_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashBoolean(
getStatusSub());
}
if (hasStatusPub()) {
hash = (37 * hash) + STATUSPUB_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashBoolean(
getStatusPub());
}
if (hasStatusTTL()) {
hash = (37 * hash) + STATUSTTL_FIELD_NUMBER;
hash = (53 * hash) + getStatusTTL();
}
if (hasUniqueId()) {
hash = (37 * hash) + UNIQUEID_FIELD_NUMBER;
hash = (53 * hash) + getUniqueId().hashCode();
}
if (hasTargetClientId()) {
hash = (37 * hash) + TARGETCLIENTID_FIELD_NUMBER;
hash = (53 * hash) + getTargetClientId().hashCode();
}
if (hasMaxReadTimestamp()) {
hash = (37 * hash) + MAXREADTIMESTAMP_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getMaxReadTimestamp());
}
if (hasMaxAckTimestamp()) {
hash = (37 * hash) + MAXACKTIMESTAMP_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getMaxAckTimestamp());
}
if (hasQueryAllMembers()) {
hash = (37 * hash) + QUERYALLMEMBERS_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashBoolean(
getQueryAllMembers());
}
if (getMaxReadTuplesCount() > 0) {
hash = (37 * hash) + MAXREADTUPLES_FIELD_NUMBER;
hash = (53 * hash) + getMaxReadTuplesList().hashCode();
}
if (getCidsCount() > 0) {
hash = (37 * hash) + CIDS_FIELD_NUMBER;
hash = (53 * hash) + getCidsList().hashCode();
}
if (hasInfo()) {
hash = (37 * hash) + INFO_FIELD_NUMBER;
hash = (53 * hash) + getInfo().hashCode();
}
if (hasTempConv()) {
hash = (37 * hash) + TEMPCONV_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashBoolean(
getTempConv());
}
if (hasTempConvTTL()) {
hash = (37 * hash) + TEMPCONVTTL_FIELD_NUMBER;
hash = (53 * hash) + getTempConvTTL();
}
if (getTempConvIdsCount() > 0) {
hash = (37 * hash) + TEMPCONVIDS_FIELD_NUMBER;
hash = (53 * hash) + getTempConvIdsList().hashCode();
}
if (getAllowedPidsCount() > 0) {
hash = (37 * hash) + ALLOWEDPIDS_FIELD_NUMBER;
hash = (53 * hash) + getAllowedPidsList().hashCode();
}
if (getFailedPidsCount() > 0) {
hash = (37 * hash) + FAILEDPIDS_FIELD_NUMBER;
hash = (53 * hash) + getFailedPidsList().hashCode();
}
if (hasNext()) {
hash = (37 * hash) + NEXT_FIELD_NUMBER;
hash = (53 * hash) + getNext().hashCode();
}
if (hasResults()) {
hash = (37 * hash) + RESULTS_FIELD_NUMBER;
hash = (53 * hash) + getResults().hashCode();
}
if (hasWhere()) {
hash = (37 * hash) + WHERE_FIELD_NUMBER;
hash = (53 * hash) + getWhere().hashCode();
}
if (hasAttr()) {
hash = (37 * hash) + ATTR_FIELD_NUMBER;
hash = (53 * hash) + getAttr().hashCode();
}
if (hasAttrModified()) {
hash = (37 * hash) + ATTRMODIFIED_FIELD_NUMBER;
hash = (53 * hash) + getAttrModified().hashCode();
}
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static com.avos.avoscloud.Messages.ConvCommand parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.avos.avoscloud.Messages.ConvCommand parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.avos.avoscloud.Messages.ConvCommand parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.avos.avoscloud.Messages.ConvCommand parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.avos.avoscloud.Messages.ConvCommand parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.avos.avoscloud.Messages.ConvCommand parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.avos.avoscloud.Messages.ConvCommand parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.avos.avoscloud.Messages.ConvCommand parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static com.avos.avoscloud.Messages.ConvCommand parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static com.avos.avoscloud.Messages.ConvCommand parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static com.avos.avoscloud.Messages.ConvCommand parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.avos.avoscloud.Messages.ConvCommand parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(com.avos.avoscloud.Messages.ConvCommand prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code com.avos.avoscloud.ConvCommand}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:com.avos.avoscloud.ConvCommand)
com.avos.avoscloud.Messages.ConvCommandOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.avos.avoscloud.Messages.internal_static_com_avos_avoscloud_ConvCommand_descriptor;
}
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.avos.avoscloud.Messages.internal_static_com_avos_avoscloud_ConvCommand_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.avos.avoscloud.Messages.ConvCommand.class, com.avos.avoscloud.Messages.ConvCommand.Builder.class);
}
// Construct using com.avos.avoscloud.Messages.ConvCommand.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
getMaxReadTuplesFieldBuilder();
getInfoFieldBuilder();
getFailedPidsFieldBuilder();
getResultsFieldBuilder();
getWhereFieldBuilder();
getAttrFieldBuilder();
getAttrModifiedFieldBuilder();
}
}
public Builder clear() {
super.clear();
m_ = com.google.protobuf.LazyStringArrayList.EMPTY;
bitField0_ = (bitField0_ & ~0x00000001);
transient_ = false;
bitField0_ = (bitField0_ & ~0x00000002);
unique_ = false;
bitField0_ = (bitField0_ & ~0x00000004);
cid_ = "";
bitField0_ = (bitField0_ & ~0x00000008);
cdate_ = "";
bitField0_ = (bitField0_ & ~0x00000010);
initBy_ = "";
bitField0_ = (bitField0_ & ~0x00000020);
sort_ = "";
bitField0_ = (bitField0_ & ~0x00000040);
limit_ = 0;
bitField0_ = (bitField0_ & ~0x00000080);
skip_ = 0;
bitField0_ = (bitField0_ & ~0x00000100);
flag_ = 0;
bitField0_ = (bitField0_ & ~0x00000200);
count_ = 0;
bitField0_ = (bitField0_ & ~0x00000400);
udate_ = "";
bitField0_ = (bitField0_ & ~0x00000800);
t_ = 0L;
bitField0_ = (bitField0_ & ~0x00001000);
n_ = "";
bitField0_ = (bitField0_ & ~0x00002000);
s_ = "";
bitField0_ = (bitField0_ & ~0x00004000);
statusSub_ = false;
bitField0_ = (bitField0_ & ~0x00008000);
statusPub_ = false;
bitField0_ = (bitField0_ & ~0x00010000);
statusTTL_ = 0;
bitField0_ = (bitField0_ & ~0x00020000);
uniqueId_ = "";
bitField0_ = (bitField0_ & ~0x00040000);
targetClientId_ = "";
bitField0_ = (bitField0_ & ~0x00080000);
maxReadTimestamp_ = 0L;
bitField0_ = (bitField0_ & ~0x00100000);
maxAckTimestamp_ = 0L;
bitField0_ = (bitField0_ & ~0x00200000);
queryAllMembers_ = false;
bitField0_ = (bitField0_ & ~0x00400000);
if (maxReadTuplesBuilder_ == null) {
maxReadTuples_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00800000);
} else {
maxReadTuplesBuilder_.clear();
}
cids_ = com.google.protobuf.LazyStringArrayList.EMPTY;
bitField0_ = (bitField0_ & ~0x01000000);
if (infoBuilder_ == null) {
info_ = null;
} else {
infoBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x02000000);
tempConv_ = false;
bitField0_ = (bitField0_ & ~0x04000000);
tempConvTTL_ = 0;
bitField0_ = (bitField0_ & ~0x08000000);
tempConvIds_ = com.google.protobuf.LazyStringArrayList.EMPTY;
bitField0_ = (bitField0_ & ~0x10000000);
allowedPids_ = com.google.protobuf.LazyStringArrayList.EMPTY;
bitField0_ = (bitField0_ & ~0x20000000);
if (failedPidsBuilder_ == null) {
failedPids_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x40000000);
} else {
failedPidsBuilder_.clear();
}
next_ = "";
bitField0_ = (bitField0_ & ~0x80000000);
if (resultsBuilder_ == null) {
results_ = null;
} else {
resultsBuilder_.clear();
}
bitField1_ = (bitField1_ & ~0x00000001);
if (whereBuilder_ == null) {
where_ = null;
} else {
whereBuilder_.clear();
}
bitField1_ = (bitField1_ & ~0x00000002);
if (attrBuilder_ == null) {
attr_ = null;
} else {
attrBuilder_.clear();
}
bitField1_ = (bitField1_ & ~0x00000004);
if (attrModifiedBuilder_ == null) {
attrModified_ = null;
} else {
attrModifiedBuilder_.clear();
}
bitField1_ = (bitField1_ & ~0x00000008);
return this;
}
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return com.avos.avoscloud.Messages.internal_static_com_avos_avoscloud_ConvCommand_descriptor;
}
public com.avos.avoscloud.Messages.ConvCommand getDefaultInstanceForType() {
return com.avos.avoscloud.Messages.ConvCommand.getDefaultInstance();
}
public com.avos.avoscloud.Messages.ConvCommand build() {
com.avos.avoscloud.Messages.ConvCommand result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
public com.avos.avoscloud.Messages.ConvCommand buildPartial() {
com.avos.avoscloud.Messages.ConvCommand result = new com.avos.avoscloud.Messages.ConvCommand(this);
int from_bitField0_ = bitField0_;
int from_bitField1_ = bitField1_;
int to_bitField0_ = 0;
if (((bitField0_ & 0x00000001) == 0x00000001)) {
m_ = m_.getUnmodifiableView();
bitField0_ = (bitField0_ & ~0x00000001);
}
result.m_ = m_;
if (((from_bitField0_ & 0x00000002) == 0x00000002)) {
to_bitField0_ |= 0x00000001;
}
result.transient_ = transient_;
if (((from_bitField0_ & 0x00000004) == 0x00000004)) {
to_bitField0_ |= 0x00000002;
}
result.unique_ = unique_;
if (((from_bitField0_ & 0x00000008) == 0x00000008)) {
to_bitField0_ |= 0x00000004;
}
result.cid_ = cid_;
if (((from_bitField0_ & 0x00000010) == 0x00000010)) {
to_bitField0_ |= 0x00000008;
}
result.cdate_ = cdate_;
if (((from_bitField0_ & 0x00000020) == 0x00000020)) {
to_bitField0_ |= 0x00000010;
}
result.initBy_ = initBy_;
if (((from_bitField0_ & 0x00000040) == 0x00000040)) {
to_bitField0_ |= 0x00000020;
}
result.sort_ = sort_;
if (((from_bitField0_ & 0x00000080) == 0x00000080)) {
to_bitField0_ |= 0x00000040;
}
result.limit_ = limit_;
if (((from_bitField0_ & 0x00000100) == 0x00000100)) {
to_bitField0_ |= 0x00000080;
}
result.skip_ = skip_;
if (((from_bitField0_ & 0x00000200) == 0x00000200)) {
to_bitField0_ |= 0x00000100;
}
result.flag_ = flag_;
if (((from_bitField0_ & 0x00000400) == 0x00000400)) {
to_bitField0_ |= 0x00000200;
}
result.count_ = count_;
if (((from_bitField0_ & 0x00000800) == 0x00000800)) {
to_bitField0_ |= 0x00000400;
}
result.udate_ = udate_;
if (((from_bitField0_ & 0x00001000) == 0x00001000)) {
to_bitField0_ |= 0x00000800;
}
result.t_ = t_;
if (((from_bitField0_ & 0x00002000) == 0x00002000)) {
to_bitField0_ |= 0x00001000;
}
result.n_ = n_;
if (((from_bitField0_ & 0x00004000) == 0x00004000)) {
to_bitField0_ |= 0x00002000;
}
result.s_ = s_;
if (((from_bitField0_ & 0x00008000) == 0x00008000)) {
to_bitField0_ |= 0x00004000;
}
result.statusSub_ = statusSub_;
if (((from_bitField0_ & 0x00010000) == 0x00010000)) {
to_bitField0_ |= 0x00008000;
}
result.statusPub_ = statusPub_;
if (((from_bitField0_ & 0x00020000) == 0x00020000)) {
to_bitField0_ |= 0x00010000;
}
result.statusTTL_ = statusTTL_;
if (((from_bitField0_ & 0x00040000) == 0x00040000)) {
to_bitField0_ |= 0x00020000;
}
result.uniqueId_ = uniqueId_;
if (((from_bitField0_ & 0x00080000) == 0x00080000)) {
to_bitField0_ |= 0x00040000;
}
result.targetClientId_ = targetClientId_;
if (((from_bitField0_ & 0x00100000) == 0x00100000)) {
to_bitField0_ |= 0x00080000;
}
result.maxReadTimestamp_ = maxReadTimestamp_;
if (((from_bitField0_ & 0x00200000) == 0x00200000)) {
to_bitField0_ |= 0x00100000;
}
result.maxAckTimestamp_ = maxAckTimestamp_;
if (((from_bitField0_ & 0x00400000) == 0x00400000)) {
to_bitField0_ |= 0x00200000;
}
result.queryAllMembers_ = queryAllMembers_;
if (maxReadTuplesBuilder_ == null) {
if (((bitField0_ & 0x00800000) == 0x00800000)) {
maxReadTuples_ = java.util.Collections.unmodifiableList(maxReadTuples_);
bitField0_ = (bitField0_ & ~0x00800000);
}
result.maxReadTuples_ = maxReadTuples_;
} else {
result.maxReadTuples_ = maxReadTuplesBuilder_.build();
}
if (((bitField0_ & 0x01000000) == 0x01000000)) {
cids_ = cids_.getUnmodifiableView();
bitField0_ = (bitField0_ & ~0x01000000);
}
result.cids_ = cids_;
if (((from_bitField0_ & 0x02000000) == 0x02000000)) {
to_bitField0_ |= 0x00400000;
}
if (infoBuilder_ == null) {
result.info_ = info_;
} else {
result.info_ = infoBuilder_.build();
}
if (((from_bitField0_ & 0x04000000) == 0x04000000)) {
to_bitField0_ |= 0x00800000;
}
result.tempConv_ = tempConv_;
if (((from_bitField0_ & 0x08000000) == 0x08000000)) {
to_bitField0_ |= 0x01000000;
}
result.tempConvTTL_ = tempConvTTL_;
if (((bitField0_ & 0x10000000) == 0x10000000)) {
tempConvIds_ = tempConvIds_.getUnmodifiableView();
bitField0_ = (bitField0_ & ~0x10000000);
}
result.tempConvIds_ = tempConvIds_;
if (((bitField0_ & 0x20000000) == 0x20000000)) {
allowedPids_ = allowedPids_.getUnmodifiableView();
bitField0_ = (bitField0_ & ~0x20000000);
}
result.allowedPids_ = allowedPids_;
if (failedPidsBuilder_ == null) {
if (((bitField0_ & 0x40000000) == 0x40000000)) {
failedPids_ = java.util.Collections.unmodifiableList(failedPids_);
bitField0_ = (bitField0_ & ~0x40000000);
}
result.failedPids_ = failedPids_;
} else {
result.failedPids_ = failedPidsBuilder_.build();
}
if (((from_bitField0_ & 0x80000000) == 0x80000000)) {
to_bitField0_ |= 0x02000000;
}
result.next_ = next_;
if (((from_bitField1_ & 0x00000001) == 0x00000001)) {
to_bitField0_ |= 0x04000000;
}
if (resultsBuilder_ == null) {
result.results_ = results_;
} else {
result.results_ = resultsBuilder_.build();
}
if (((from_bitField1_ & 0x00000002) == 0x00000002)) {
to_bitField0_ |= 0x08000000;
}
if (whereBuilder_ == null) {
result.where_ = where_;
} else {
result.where_ = whereBuilder_.build();
}
if (((from_bitField1_ & 0x00000004) == 0x00000004)) {
to_bitField0_ |= 0x10000000;
}
if (attrBuilder_ == null) {
result.attr_ = attr_;
} else {
result.attr_ = attrBuilder_.build();
}
if (((from_bitField1_ & 0x00000008) == 0x00000008)) {
to_bitField0_ |= 0x20000000;
}
if (attrModifiedBuilder_ == null) {
result.attrModified_ = attrModified_;
} else {
result.attrModified_ = attrModifiedBuilder_.build();
}
result.bitField0_ = to_bitField0_;
onBuilt();
return result;
}
public Builder clone() {
return (Builder) super.clone();
}
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.setField(field, value);
}
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return (Builder) super.clearField(field);
}
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return (Builder) super.clearOneof(oneof);
}
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return (Builder) super.setRepeatedField(field, index, value);
}
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.addRepeatedField(field, value);
}
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.avos.avoscloud.Messages.ConvCommand) {
return mergeFrom((com.avos.avoscloud.Messages.ConvCommand)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.avos.avoscloud.Messages.ConvCommand other) {
if (other == com.avos.avoscloud.Messages.ConvCommand.getDefaultInstance()) return this;
if (!other.m_.isEmpty()) {
if (m_.isEmpty()) {
m_ = other.m_;
bitField0_ = (bitField0_ & ~0x00000001);
} else {
ensureMIsMutable();
m_.addAll(other.m_);
}
onChanged();
}
if (other.hasTransient()) {
setTransient(other.getTransient());
}
if (other.hasUnique()) {
setUnique(other.getUnique());
}
if (other.hasCid()) {
bitField0_ |= 0x00000008;
cid_ = other.cid_;
onChanged();
}
if (other.hasCdate()) {
bitField0_ |= 0x00000010;
cdate_ = other.cdate_;
onChanged();
}
if (other.hasInitBy()) {
bitField0_ |= 0x00000020;
initBy_ = other.initBy_;
onChanged();
}
if (other.hasSort()) {
bitField0_ |= 0x00000040;
sort_ = other.sort_;
onChanged();
}
if (other.hasLimit()) {
setLimit(other.getLimit());
}
if (other.hasSkip()) {
setSkip(other.getSkip());
}
if (other.hasFlag()) {
setFlag(other.getFlag());
}
if (other.hasCount()) {
setCount(other.getCount());
}
if (other.hasUdate()) {
bitField0_ |= 0x00000800;
udate_ = other.udate_;
onChanged();
}
if (other.hasT()) {
setT(other.getT());
}
if (other.hasN()) {
bitField0_ |= 0x00002000;
n_ = other.n_;
onChanged();
}
if (other.hasS()) {
bitField0_ |= 0x00004000;
s_ = other.s_;
onChanged();
}
if (other.hasStatusSub()) {
setStatusSub(other.getStatusSub());
}
if (other.hasStatusPub()) {
setStatusPub(other.getStatusPub());
}
if (other.hasStatusTTL()) {
setStatusTTL(other.getStatusTTL());
}
if (other.hasUniqueId()) {
bitField0_ |= 0x00040000;
uniqueId_ = other.uniqueId_;
onChanged();
}
if (other.hasTargetClientId()) {
bitField0_ |= 0x00080000;
targetClientId_ = other.targetClientId_;
onChanged();
}
if (other.hasMaxReadTimestamp()) {
setMaxReadTimestamp(other.getMaxReadTimestamp());
}
if (other.hasMaxAckTimestamp()) {
setMaxAckTimestamp(other.getMaxAckTimestamp());
}
if (other.hasQueryAllMembers()) {
setQueryAllMembers(other.getQueryAllMembers());
}
if (maxReadTuplesBuilder_ == null) {
if (!other.maxReadTuples_.isEmpty()) {
if (maxReadTuples_.isEmpty()) {
maxReadTuples_ = other.maxReadTuples_;
bitField0_ = (bitField0_ & ~0x00800000);
} else {
ensureMaxReadTuplesIsMutable();
maxReadTuples_.addAll(other.maxReadTuples_);
}
onChanged();
}
} else {
if (!other.maxReadTuples_.isEmpty()) {
if (maxReadTuplesBuilder_.isEmpty()) {
maxReadTuplesBuilder_.dispose();
maxReadTuplesBuilder_ = null;
maxReadTuples_ = other.maxReadTuples_;
bitField0_ = (bitField0_ & ~0x00800000);
maxReadTuplesBuilder_ =
com.google.protobuf.GeneratedMessageV3.alwaysUseFieldBuilders ?
getMaxReadTuplesFieldBuilder() : null;
} else {
maxReadTuplesBuilder_.addAllMessages(other.maxReadTuples_);
}
}
}
if (!other.cids_.isEmpty()) {
if (cids_.isEmpty()) {
cids_ = other.cids_;
bitField0_ = (bitField0_ & ~0x01000000);
} else {
ensureCidsIsMutable();
cids_.addAll(other.cids_);
}
onChanged();
}
if (other.hasInfo()) {
mergeInfo(other.getInfo());
}
if (other.hasTempConv()) {
setTempConv(other.getTempConv());
}
if (other.hasTempConvTTL()) {
setTempConvTTL(other.getTempConvTTL());
}
if (!other.tempConvIds_.isEmpty()) {
if (tempConvIds_.isEmpty()) {
tempConvIds_ = other.tempConvIds_;
bitField0_ = (bitField0_ & ~0x10000000);
} else {
ensureTempConvIdsIsMutable();
tempConvIds_.addAll(other.tempConvIds_);
}
onChanged();
}
if (!other.allowedPids_.isEmpty()) {
if (allowedPids_.isEmpty()) {
allowedPids_ = other.allowedPids_;
bitField0_ = (bitField0_ & ~0x20000000);
} else {
ensureAllowedPidsIsMutable();
allowedPids_.addAll(other.allowedPids_);
}
onChanged();
}
if (failedPidsBuilder_ == null) {
if (!other.failedPids_.isEmpty()) {
if (failedPids_.isEmpty()) {
failedPids_ = other.failedPids_;
bitField0_ = (bitField0_ & ~0x40000000);
} else {
ensureFailedPidsIsMutable();
failedPids_.addAll(other.failedPids_);
}
onChanged();
}
} else {
if (!other.failedPids_.isEmpty()) {
if (failedPidsBuilder_.isEmpty()) {
failedPidsBuilder_.dispose();
failedPidsBuilder_ = null;
failedPids_ = other.failedPids_;
bitField0_ = (bitField0_ & ~0x40000000);
failedPidsBuilder_ =
com.google.protobuf.GeneratedMessageV3.alwaysUseFieldBuilders ?
getFailedPidsFieldBuilder() : null;
} else {
failedPidsBuilder_.addAllMessages(other.failedPids_);
}
}
}
if (other.hasNext()) {
bitField0_ |= 0x80000000;
next_ = other.next_;
onChanged();
}
if (other.hasResults()) {
mergeResults(other.getResults());
}
if (other.hasWhere()) {
mergeWhere(other.getWhere());
}
if (other.hasAttr()) {
mergeAttr(other.getAttr());
}
if (other.hasAttrModified()) {
mergeAttrModified(other.getAttrModified());
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
public final boolean isInitialized() {
for (int i = 0; i < getFailedPidsCount(); i++) {
if (!getFailedPids(i).isInitialized()) {
return false;
}
}
if (hasResults()) {
if (!getResults().isInitialized()) {
return false;
}
}
if (hasWhere()) {
if (!getWhere().isInitialized()) {
return false;
}
}
if (hasAttr()) {
if (!getAttr().isInitialized()) {
return false;
}
}
if (hasAttrModified()) {
if (!getAttrModified().isInitialized()) {
return false;
}
}
return true;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
com.avos.avoscloud.Messages.ConvCommand parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (com.avos.avoscloud.Messages.ConvCommand) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
private int bitField1_;
private com.google.protobuf.LazyStringList m_ = com.google.protobuf.LazyStringArrayList.EMPTY;
private void ensureMIsMutable() {
if (!((bitField0_ & 0x00000001) == 0x00000001)) {
m_ = new com.google.protobuf.LazyStringArrayList(m_);
bitField0_ |= 0x00000001;
}
}
/**
* repeated string m = 1;
*/
public com.google.protobuf.ProtocolStringList
getMList() {
return m_.getUnmodifiableView();
}
/**
* repeated string m = 1;
*/
public int getMCount() {
return m_.size();
}
/**
* repeated string m = 1;
*/
public java.lang.String getM(int index) {
return m_.get(index);
}
/**
* repeated string m = 1;
*/
public com.google.protobuf.ByteString
getMBytes(int index) {
return m_.getByteString(index);
}
/**
* repeated string m = 1;
*/
public Builder setM(
int index, java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
ensureMIsMutable();
m_.set(index, value);
onChanged();
return this;
}
/**
* repeated string m = 1;
*/
public Builder addM(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
ensureMIsMutable();
m_.add(value);
onChanged();
return this;
}
/**
* repeated string m = 1;
*/
public Builder addAllM(
java.lang.Iterable values) {
ensureMIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, m_);
onChanged();
return this;
}
/**
* repeated string m = 1;
*/
public Builder clearM() {
m_ = com.google.protobuf.LazyStringArrayList.EMPTY;
bitField0_ = (bitField0_ & ~0x00000001);
onChanged();
return this;
}
/**
* repeated string m = 1;
*/
public Builder addMBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
ensureMIsMutable();
m_.add(value);
onChanged();
return this;
}
private boolean transient_ ;
/**
* optional bool transient = 2;
*/
public boolean hasTransient() {
return ((bitField0_ & 0x00000002) == 0x00000002);
}
/**
* optional bool transient = 2;
*/
public boolean getTransient() {
return transient_;
}
/**
* optional bool transient = 2;
*/
public Builder setTransient(boolean value) {
bitField0_ |= 0x00000002;
transient_ = value;
onChanged();
return this;
}
/**
* optional bool transient = 2;
*/
public Builder clearTransient() {
bitField0_ = (bitField0_ & ~0x00000002);
transient_ = false;
onChanged();
return this;
}
private boolean unique_ ;
/**
* optional bool unique = 3;
*/
public boolean hasUnique() {
return ((bitField0_ & 0x00000004) == 0x00000004);
}
/**
* optional bool unique = 3;
*/
public boolean getUnique() {
return unique_;
}
/**
* optional bool unique = 3;
*/
public Builder setUnique(boolean value) {
bitField0_ |= 0x00000004;
unique_ = value;
onChanged();
return this;
}
/**
* optional bool unique = 3;
*/
public Builder clearUnique() {
bitField0_ = (bitField0_ & ~0x00000004);
unique_ = false;
onChanged();
return this;
}
private java.lang.Object cid_ = "";
/**
* optional string cid = 4;
*/
public boolean hasCid() {
return ((bitField0_ & 0x00000008) == 0x00000008);
}
/**
* optional string cid = 4;
*/
public java.lang.String getCid() {
java.lang.Object ref = cid_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
cid_ = s;
}
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* optional string cid = 4;
*/
public com.google.protobuf.ByteString
getCidBytes() {
java.lang.Object ref = cid_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
cid_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* optional string cid = 4;
*/
public Builder setCid(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000008;
cid_ = value;
onChanged();
return this;
}
/**
* optional string cid = 4;
*/
public Builder clearCid() {
bitField0_ = (bitField0_ & ~0x00000008);
cid_ = getDefaultInstance().getCid();
onChanged();
return this;
}
/**
* optional string cid = 4;
*/
public Builder setCidBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000008;
cid_ = value;
onChanged();
return this;
}
private java.lang.Object cdate_ = "";
/**
* optional string cdate = 5;
*/
public boolean hasCdate() {
return ((bitField0_ & 0x00000010) == 0x00000010);
}
/**
* optional string cdate = 5;
*/
public java.lang.String getCdate() {
java.lang.Object ref = cdate_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
cdate_ = s;
}
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* optional string cdate = 5;
*/
public com.google.protobuf.ByteString
getCdateBytes() {
java.lang.Object ref = cdate_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
cdate_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* optional string cdate = 5;
*/
public Builder setCdate(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000010;
cdate_ = value;
onChanged();
return this;
}
/**
* optional string cdate = 5;
*/
public Builder clearCdate() {
bitField0_ = (bitField0_ & ~0x00000010);
cdate_ = getDefaultInstance().getCdate();
onChanged();
return this;
}
/**
* optional string cdate = 5;
*/
public Builder setCdateBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000010;
cdate_ = value;
onChanged();
return this;
}
private java.lang.Object initBy_ = "";
/**
* optional string initBy = 6;
*/
public boolean hasInitBy() {
return ((bitField0_ & 0x00000020) == 0x00000020);
}
/**
* optional string initBy = 6;
*/
public java.lang.String getInitBy() {
java.lang.Object ref = initBy_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
initBy_ = s;
}
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* optional string initBy = 6;
*/
public com.google.protobuf.ByteString
getInitByBytes() {
java.lang.Object ref = initBy_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
initBy_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* optional string initBy = 6;
*/
public Builder setInitBy(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000020;
initBy_ = value;
onChanged();
return this;
}
/**
* optional string initBy = 6;
*/
public Builder clearInitBy() {
bitField0_ = (bitField0_ & ~0x00000020);
initBy_ = getDefaultInstance().getInitBy();
onChanged();
return this;
}
/**
* optional string initBy = 6;
*/
public Builder setInitByBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000020;
initBy_ = value;
onChanged();
return this;
}
private java.lang.Object sort_ = "";
/**
* optional string sort = 7;
*/
public boolean hasSort() {
return ((bitField0_ & 0x00000040) == 0x00000040);
}
/**
* optional string sort = 7;
*/
public java.lang.String getSort() {
java.lang.Object ref = sort_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
sort_ = s;
}
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* optional string sort = 7;
*/
public com.google.protobuf.ByteString
getSortBytes() {
java.lang.Object ref = sort_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
sort_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* optional string sort = 7;
*/
public Builder setSort(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000040;
sort_ = value;
onChanged();
return this;
}
/**
* optional string sort = 7;
*/
public Builder clearSort() {
bitField0_ = (bitField0_ & ~0x00000040);
sort_ = getDefaultInstance().getSort();
onChanged();
return this;
}
/**
* optional string sort = 7;
*/
public Builder setSortBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000040;
sort_ = value;
onChanged();
return this;
}
private int limit_ ;
/**
* optional int32 limit = 8;
*/
public boolean hasLimit() {
return ((bitField0_ & 0x00000080) == 0x00000080);
}
/**
* optional int32 limit = 8;
*/
public int getLimit() {
return limit_;
}
/**
* optional int32 limit = 8;
*/
public Builder setLimit(int value) {
bitField0_ |= 0x00000080;
limit_ = value;
onChanged();
return this;
}
/**
* optional int32 limit = 8;
*/
public Builder clearLimit() {
bitField0_ = (bitField0_ & ~0x00000080);
limit_ = 0;
onChanged();
return this;
}
private int skip_ ;
/**
* optional int32 skip = 9;
*/
public boolean hasSkip() {
return ((bitField0_ & 0x00000100) == 0x00000100);
}
/**
* optional int32 skip = 9;
*/
public int getSkip() {
return skip_;
}
/**
* optional int32 skip = 9;
*/
public Builder setSkip(int value) {
bitField0_ |= 0x00000100;
skip_ = value;
onChanged();
return this;
}
/**
* optional int32 skip = 9;
*/
public Builder clearSkip() {
bitField0_ = (bitField0_ & ~0x00000100);
skip_ = 0;
onChanged();
return this;
}
private int flag_ ;
/**
* optional int32 flag = 10;
*/
public boolean hasFlag() {
return ((bitField0_ & 0x00000200) == 0x00000200);
}
/**
* optional int32 flag = 10;
*/
public int getFlag() {
return flag_;
}
/**
* optional int32 flag = 10;
*/
public Builder setFlag(int value) {
bitField0_ |= 0x00000200;
flag_ = value;
onChanged();
return this;
}
/**
* optional int32 flag = 10;
*/
public Builder clearFlag() {
bitField0_ = (bitField0_ & ~0x00000200);
flag_ = 0;
onChanged();
return this;
}
private int count_ ;
/**
* optional int32 count = 11;
*/
public boolean hasCount() {
return ((bitField0_ & 0x00000400) == 0x00000400);
}
/**
* optional int32 count = 11;
*/
public int getCount() {
return count_;
}
/**
* optional int32 count = 11;
*/
public Builder setCount(int value) {
bitField0_ |= 0x00000400;
count_ = value;
onChanged();
return this;
}
/**
* optional int32 count = 11;
*/
public Builder clearCount() {
bitField0_ = (bitField0_ & ~0x00000400);
count_ = 0;
onChanged();
return this;
}
private java.lang.Object udate_ = "";
/**
* optional string udate = 12;
*/
public boolean hasUdate() {
return ((bitField0_ & 0x00000800) == 0x00000800);
}
/**
* optional string udate = 12;
*/
public java.lang.String getUdate() {
java.lang.Object ref = udate_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
udate_ = s;
}
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* optional string udate = 12;
*/
public com.google.protobuf.ByteString
getUdateBytes() {
java.lang.Object ref = udate_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
udate_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* optional string udate = 12;
*/
public Builder setUdate(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000800;
udate_ = value;
onChanged();
return this;
}
/**
* optional string udate = 12;
*/
public Builder clearUdate() {
bitField0_ = (bitField0_ & ~0x00000800);
udate_ = getDefaultInstance().getUdate();
onChanged();
return this;
}
/**
* optional string udate = 12;
*/
public Builder setUdateBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000800;
udate_ = value;
onChanged();
return this;
}
private long t_ ;
/**
* optional int64 t = 13;
*/
public boolean hasT() {
return ((bitField0_ & 0x00001000) == 0x00001000);
}
/**
* optional int64 t = 13;
*/
public long getT() {
return t_;
}
/**
* optional int64 t = 13;
*/
public Builder setT(long value) {
bitField0_ |= 0x00001000;
t_ = value;
onChanged();
return this;
}
/**
* optional int64 t = 13;
*/
public Builder clearT() {
bitField0_ = (bitField0_ & ~0x00001000);
t_ = 0L;
onChanged();
return this;
}
private java.lang.Object n_ = "";
/**
* optional string n = 14;
*/
public boolean hasN() {
return ((bitField0_ & 0x00002000) == 0x00002000);
}
/**
* optional string n = 14;
*/
public java.lang.String getN() {
java.lang.Object ref = n_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
n_ = s;
}
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* optional string n = 14;
*/
public com.google.protobuf.ByteString
getNBytes() {
java.lang.Object ref = n_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
n_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* optional string n = 14;
*/
public Builder setN(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00002000;
n_ = value;
onChanged();
return this;
}
/**
* optional string n = 14;
*/
public Builder clearN() {
bitField0_ = (bitField0_ & ~0x00002000);
n_ = getDefaultInstance().getN();
onChanged();
return this;
}
/**
* optional string n = 14;
*/
public Builder setNBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00002000;
n_ = value;
onChanged();
return this;
}
private java.lang.Object s_ = "";
/**
* optional string s = 15;
*/
public boolean hasS() {
return ((bitField0_ & 0x00004000) == 0x00004000);
}
/**
* optional string s = 15;
*/
public java.lang.String getS() {
java.lang.Object ref = s_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
s_ = s;
}
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* optional string s = 15;
*/
public com.google.protobuf.ByteString
getSBytes() {
java.lang.Object ref = s_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
s_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* optional string s = 15;
*/
public Builder setS(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00004000;
s_ = value;
onChanged();
return this;
}
/**
* optional string s = 15;
*/
public Builder clearS() {
bitField0_ = (bitField0_ & ~0x00004000);
s_ = getDefaultInstance().getS();
onChanged();
return this;
}
/**
* optional string s = 15;
*/
public Builder setSBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00004000;
s_ = value;
onChanged();
return this;
}
private boolean statusSub_ ;
/**
* optional bool statusSub = 16;
*/
public boolean hasStatusSub() {
return ((bitField0_ & 0x00008000) == 0x00008000);
}
/**
* optional bool statusSub = 16;
*/
public boolean getStatusSub() {
return statusSub_;
}
/**
* optional bool statusSub = 16;
*/
public Builder setStatusSub(boolean value) {
bitField0_ |= 0x00008000;
statusSub_ = value;
onChanged();
return this;
}
/**
* optional bool statusSub = 16;
*/
public Builder clearStatusSub() {
bitField0_ = (bitField0_ & ~0x00008000);
statusSub_ = false;
onChanged();
return this;
}
private boolean statusPub_ ;
/**
* optional bool statusPub = 17;
*/
public boolean hasStatusPub() {
return ((bitField0_ & 0x00010000) == 0x00010000);
}
/**
* optional bool statusPub = 17;
*/
public boolean getStatusPub() {
return statusPub_;
}
/**
* optional bool statusPub = 17;
*/
public Builder setStatusPub(boolean value) {
bitField0_ |= 0x00010000;
statusPub_ = value;
onChanged();
return this;
}
/**
* optional bool statusPub = 17;
*/
public Builder clearStatusPub() {
bitField0_ = (bitField0_ & ~0x00010000);
statusPub_ = false;
onChanged();
return this;
}
private int statusTTL_ ;
/**
* optional int32 statusTTL = 18;
*/
public boolean hasStatusTTL() {
return ((bitField0_ & 0x00020000) == 0x00020000);
}
/**
* optional int32 statusTTL = 18;
*/
public int getStatusTTL() {
return statusTTL_;
}
/**
* optional int32 statusTTL = 18;
*/
public Builder setStatusTTL(int value) {
bitField0_ |= 0x00020000;
statusTTL_ = value;
onChanged();
return this;
}
/**
* optional int32 statusTTL = 18;
*/
public Builder clearStatusTTL() {
bitField0_ = (bitField0_ & ~0x00020000);
statusTTL_ = 0;
onChanged();
return this;
}
private java.lang.Object uniqueId_ = "";
/**
* optional string uniqueId = 19;
*/
public boolean hasUniqueId() {
return ((bitField0_ & 0x00040000) == 0x00040000);
}
/**
* optional string uniqueId = 19;
*/
public java.lang.String getUniqueId() {
java.lang.Object ref = uniqueId_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
uniqueId_ = s;
}
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* optional string uniqueId = 19;
*/
public com.google.protobuf.ByteString
getUniqueIdBytes() {
java.lang.Object ref = uniqueId_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
uniqueId_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* optional string uniqueId = 19;
*/
public Builder setUniqueId(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00040000;
uniqueId_ = value;
onChanged();
return this;
}
/**
* optional string uniqueId = 19;
*/
public Builder clearUniqueId() {
bitField0_ = (bitField0_ & ~0x00040000);
uniqueId_ = getDefaultInstance().getUniqueId();
onChanged();
return this;
}
/**
* optional string uniqueId = 19;
*/
public Builder setUniqueIdBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00040000;
uniqueId_ = value;
onChanged();
return this;
}
private java.lang.Object targetClientId_ = "";
/**
* optional string targetClientId = 20;
*/
public boolean hasTargetClientId() {
return ((bitField0_ & 0x00080000) == 0x00080000);
}
/**
* optional string targetClientId = 20;
*/
public java.lang.String getTargetClientId() {
java.lang.Object ref = targetClientId_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
targetClientId_ = s;
}
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* optional string targetClientId = 20;
*/
public com.google.protobuf.ByteString
getTargetClientIdBytes() {
java.lang.Object ref = targetClientId_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
targetClientId_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* optional string targetClientId = 20;
*/
public Builder setTargetClientId(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00080000;
targetClientId_ = value;
onChanged();
return this;
}
/**
* optional string targetClientId = 20;
*/
public Builder clearTargetClientId() {
bitField0_ = (bitField0_ & ~0x00080000);
targetClientId_ = getDefaultInstance().getTargetClientId();
onChanged();
return this;
}
/**
* optional string targetClientId = 20;
*/
public Builder setTargetClientIdBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00080000;
targetClientId_ = value;
onChanged();
return this;
}
private long maxReadTimestamp_ ;
/**
* optional int64 maxReadTimestamp = 21;
*/
public boolean hasMaxReadTimestamp() {
return ((bitField0_ & 0x00100000) == 0x00100000);
}
/**
* optional int64 maxReadTimestamp = 21;
*/
public long getMaxReadTimestamp() {
return maxReadTimestamp_;
}
/**
* optional int64 maxReadTimestamp = 21;
*/
public Builder setMaxReadTimestamp(long value) {
bitField0_ |= 0x00100000;
maxReadTimestamp_ = value;
onChanged();
return this;
}
/**
* optional int64 maxReadTimestamp = 21;
*/
public Builder clearMaxReadTimestamp() {
bitField0_ = (bitField0_ & ~0x00100000);
maxReadTimestamp_ = 0L;
onChanged();
return this;
}
private long maxAckTimestamp_ ;
/**
* optional int64 maxAckTimestamp = 22;
*/
public boolean hasMaxAckTimestamp() {
return ((bitField0_ & 0x00200000) == 0x00200000);
}
/**
* optional int64 maxAckTimestamp = 22;
*/
public long getMaxAckTimestamp() {
return maxAckTimestamp_;
}
/**
* optional int64 maxAckTimestamp = 22;
*/
public Builder setMaxAckTimestamp(long value) {
bitField0_ |= 0x00200000;
maxAckTimestamp_ = value;
onChanged();
return this;
}
/**
* optional int64 maxAckTimestamp = 22;
*/
public Builder clearMaxAckTimestamp() {
bitField0_ = (bitField0_ & ~0x00200000);
maxAckTimestamp_ = 0L;
onChanged();
return this;
}
private boolean queryAllMembers_ ;
/**
* optional bool queryAllMembers = 23;
*/
public boolean hasQueryAllMembers() {
return ((bitField0_ & 0x00400000) == 0x00400000);
}
/**
* optional bool queryAllMembers = 23;
*/
public boolean getQueryAllMembers() {
return queryAllMembers_;
}
/**
* optional bool queryAllMembers = 23;
*/
public Builder setQueryAllMembers(boolean value) {
bitField0_ |= 0x00400000;
queryAllMembers_ = value;
onChanged();
return this;
}
/**
* optional bool queryAllMembers = 23;
*/
public Builder clearQueryAllMembers() {
bitField0_ = (bitField0_ & ~0x00400000);
queryAllMembers_ = false;
onChanged();
return this;
}
private java.util.List maxReadTuples_ =
java.util.Collections.emptyList();
private void ensureMaxReadTuplesIsMutable() {
if (!((bitField0_ & 0x00800000) == 0x00800000)) {
maxReadTuples_ = new java.util.ArrayList(maxReadTuples_);
bitField0_ |= 0x00800000;
}
}
private com.google.protobuf.RepeatedFieldBuilderV3<
com.avos.avoscloud.Messages.MaxReadTuple, com.avos.avoscloud.Messages.MaxReadTuple.Builder, com.avos.avoscloud.Messages.MaxReadTupleOrBuilder> maxReadTuplesBuilder_;
/**
* repeated .com.avos.avoscloud.MaxReadTuple maxReadTuples = 24;
*/
public java.util.List getMaxReadTuplesList() {
if (maxReadTuplesBuilder_ == null) {
return java.util.Collections.unmodifiableList(maxReadTuples_);
} else {
return maxReadTuplesBuilder_.getMessageList();
}
}
/**
* repeated .com.avos.avoscloud.MaxReadTuple maxReadTuples = 24;
*/
public int getMaxReadTuplesCount() {
if (maxReadTuplesBuilder_ == null) {
return maxReadTuples_.size();
} else {
return maxReadTuplesBuilder_.getCount();
}
}
/**
* repeated .com.avos.avoscloud.MaxReadTuple maxReadTuples = 24;
*/
public com.avos.avoscloud.Messages.MaxReadTuple getMaxReadTuples(int index) {
if (maxReadTuplesBuilder_ == null) {
return maxReadTuples_.get(index);
} else {
return maxReadTuplesBuilder_.getMessage(index);
}
}
/**
* repeated .com.avos.avoscloud.MaxReadTuple maxReadTuples = 24;
*/
public Builder setMaxReadTuples(
int index, com.avos.avoscloud.Messages.MaxReadTuple value) {
if (maxReadTuplesBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureMaxReadTuplesIsMutable();
maxReadTuples_.set(index, value);
onChanged();
} else {
maxReadTuplesBuilder_.setMessage(index, value);
}
return this;
}
/**
* repeated .com.avos.avoscloud.MaxReadTuple maxReadTuples = 24;
*/
public Builder setMaxReadTuples(
int index, com.avos.avoscloud.Messages.MaxReadTuple.Builder builderForValue) {
if (maxReadTuplesBuilder_ == null) {
ensureMaxReadTuplesIsMutable();
maxReadTuples_.set(index, builderForValue.build());
onChanged();
} else {
maxReadTuplesBuilder_.setMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .com.avos.avoscloud.MaxReadTuple maxReadTuples = 24;
*/
public Builder addMaxReadTuples(com.avos.avoscloud.Messages.MaxReadTuple value) {
if (maxReadTuplesBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureMaxReadTuplesIsMutable();
maxReadTuples_.add(value);
onChanged();
} else {
maxReadTuplesBuilder_.addMessage(value);
}
return this;
}
/**
* repeated .com.avos.avoscloud.MaxReadTuple maxReadTuples = 24;
*/
public Builder addMaxReadTuples(
int index, com.avos.avoscloud.Messages.MaxReadTuple value) {
if (maxReadTuplesBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureMaxReadTuplesIsMutable();
maxReadTuples_.add(index, value);
onChanged();
} else {
maxReadTuplesBuilder_.addMessage(index, value);
}
return this;
}
/**
* repeated .com.avos.avoscloud.MaxReadTuple maxReadTuples = 24;
*/
public Builder addMaxReadTuples(
com.avos.avoscloud.Messages.MaxReadTuple.Builder builderForValue) {
if (maxReadTuplesBuilder_ == null) {
ensureMaxReadTuplesIsMutable();
maxReadTuples_.add(builderForValue.build());
onChanged();
} else {
maxReadTuplesBuilder_.addMessage(builderForValue.build());
}
return this;
}
/**
* repeated .com.avos.avoscloud.MaxReadTuple maxReadTuples = 24;
*/
public Builder addMaxReadTuples(
int index, com.avos.avoscloud.Messages.MaxReadTuple.Builder builderForValue) {
if (maxReadTuplesBuilder_ == null) {
ensureMaxReadTuplesIsMutable();
maxReadTuples_.add(index, builderForValue.build());
onChanged();
} else {
maxReadTuplesBuilder_.addMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .com.avos.avoscloud.MaxReadTuple maxReadTuples = 24;
*/
public Builder addAllMaxReadTuples(
java.lang.Iterable extends com.avos.avoscloud.Messages.MaxReadTuple> values) {
if (maxReadTuplesBuilder_ == null) {
ensureMaxReadTuplesIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, maxReadTuples_);
onChanged();
} else {
maxReadTuplesBuilder_.addAllMessages(values);
}
return this;
}
/**
* repeated .com.avos.avoscloud.MaxReadTuple maxReadTuples = 24;
*/
public Builder clearMaxReadTuples() {
if (maxReadTuplesBuilder_ == null) {
maxReadTuples_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00800000);
onChanged();
} else {
maxReadTuplesBuilder_.clear();
}
return this;
}
/**
* repeated .com.avos.avoscloud.MaxReadTuple maxReadTuples = 24;
*/
public Builder removeMaxReadTuples(int index) {
if (maxReadTuplesBuilder_ == null) {
ensureMaxReadTuplesIsMutable();
maxReadTuples_.remove(index);
onChanged();
} else {
maxReadTuplesBuilder_.remove(index);
}
return this;
}
/**
* repeated .com.avos.avoscloud.MaxReadTuple maxReadTuples = 24;
*/
public com.avos.avoscloud.Messages.MaxReadTuple.Builder getMaxReadTuplesBuilder(
int index) {
return getMaxReadTuplesFieldBuilder().getBuilder(index);
}
/**
* repeated .com.avos.avoscloud.MaxReadTuple maxReadTuples = 24;
*/
public com.avos.avoscloud.Messages.MaxReadTupleOrBuilder getMaxReadTuplesOrBuilder(
int index) {
if (maxReadTuplesBuilder_ == null) {
return maxReadTuples_.get(index); } else {
return maxReadTuplesBuilder_.getMessageOrBuilder(index);
}
}
/**
* repeated .com.avos.avoscloud.MaxReadTuple maxReadTuples = 24;
*/
public java.util.List extends com.avos.avoscloud.Messages.MaxReadTupleOrBuilder>
getMaxReadTuplesOrBuilderList() {
if (maxReadTuplesBuilder_ != null) {
return maxReadTuplesBuilder_.getMessageOrBuilderList();
} else {
return java.util.Collections.unmodifiableList(maxReadTuples_);
}
}
/**
* repeated .com.avos.avoscloud.MaxReadTuple maxReadTuples = 24;
*/
public com.avos.avoscloud.Messages.MaxReadTuple.Builder addMaxReadTuplesBuilder() {
return getMaxReadTuplesFieldBuilder().addBuilder(
com.avos.avoscloud.Messages.MaxReadTuple.getDefaultInstance());
}
/**
* repeated .com.avos.avoscloud.MaxReadTuple maxReadTuples = 24;
*/
public com.avos.avoscloud.Messages.MaxReadTuple.Builder addMaxReadTuplesBuilder(
int index) {
return getMaxReadTuplesFieldBuilder().addBuilder(
index, com.avos.avoscloud.Messages.MaxReadTuple.getDefaultInstance());
}
/**
* repeated .com.avos.avoscloud.MaxReadTuple maxReadTuples = 24;
*/
public java.util.List
getMaxReadTuplesBuilderList() {
return getMaxReadTuplesFieldBuilder().getBuilderList();
}
private com.google.protobuf.RepeatedFieldBuilderV3<
com.avos.avoscloud.Messages.MaxReadTuple, com.avos.avoscloud.Messages.MaxReadTuple.Builder, com.avos.avoscloud.Messages.MaxReadTupleOrBuilder>
getMaxReadTuplesFieldBuilder() {
if (maxReadTuplesBuilder_ == null) {
maxReadTuplesBuilder_ = new com.google.protobuf.RepeatedFieldBuilderV3<
com.avos.avoscloud.Messages.MaxReadTuple, com.avos.avoscloud.Messages.MaxReadTuple.Builder, com.avos.avoscloud.Messages.MaxReadTupleOrBuilder>(
maxReadTuples_,
((bitField0_ & 0x00800000) == 0x00800000),
getParentForChildren(),
isClean());
maxReadTuples_ = null;
}
return maxReadTuplesBuilder_;
}
private com.google.protobuf.LazyStringList cids_ = com.google.protobuf.LazyStringArrayList.EMPTY;
private void ensureCidsIsMutable() {
if (!((bitField0_ & 0x01000000) == 0x01000000)) {
cids_ = new com.google.protobuf.LazyStringArrayList(cids_);
bitField0_ |= 0x01000000;
}
}
/**
* repeated string cids = 25;
*/
public com.google.protobuf.ProtocolStringList
getCidsList() {
return cids_.getUnmodifiableView();
}
/**
* repeated string cids = 25;
*/
public int getCidsCount() {
return cids_.size();
}
/**
* repeated string cids = 25;
*/
public java.lang.String getCids(int index) {
return cids_.get(index);
}
/**
* repeated string cids = 25;
*/
public com.google.protobuf.ByteString
getCidsBytes(int index) {
return cids_.getByteString(index);
}
/**
* repeated string cids = 25;
*/
public Builder setCids(
int index, java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
ensureCidsIsMutable();
cids_.set(index, value);
onChanged();
return this;
}
/**
* repeated string cids = 25;
*/
public Builder addCids(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
ensureCidsIsMutable();
cids_.add(value);
onChanged();
return this;
}
/**
* repeated string cids = 25;
*/
public Builder addAllCids(
java.lang.Iterable values) {
ensureCidsIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, cids_);
onChanged();
return this;
}
/**
* repeated string cids = 25;
*/
public Builder clearCids() {
cids_ = com.google.protobuf.LazyStringArrayList.EMPTY;
bitField0_ = (bitField0_ & ~0x01000000);
onChanged();
return this;
}
/**
* repeated string cids = 25;
*/
public Builder addCidsBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
ensureCidsIsMutable();
cids_.add(value);
onChanged();
return this;
}
private com.avos.avoscloud.Messages.ConvMemberInfo info_ = null;
private com.google.protobuf.SingleFieldBuilderV3<
com.avos.avoscloud.Messages.ConvMemberInfo, com.avos.avoscloud.Messages.ConvMemberInfo.Builder, com.avos.avoscloud.Messages.ConvMemberInfoOrBuilder> infoBuilder_;
/**
* optional .com.avos.avoscloud.ConvMemberInfo info = 26;
*/
public boolean hasInfo() {
return ((bitField0_ & 0x02000000) == 0x02000000);
}
/**
* optional .com.avos.avoscloud.ConvMemberInfo info = 26;
*/
public com.avos.avoscloud.Messages.ConvMemberInfo getInfo() {
if (infoBuilder_ == null) {
return info_ == null ? com.avos.avoscloud.Messages.ConvMemberInfo.getDefaultInstance() : info_;
} else {
return infoBuilder_.getMessage();
}
}
/**
* optional .com.avos.avoscloud.ConvMemberInfo info = 26;
*/
public Builder setInfo(com.avos.avoscloud.Messages.ConvMemberInfo value) {
if (infoBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
info_ = value;
onChanged();
} else {
infoBuilder_.setMessage(value);
}
bitField0_ |= 0x02000000;
return this;
}
/**
* optional .com.avos.avoscloud.ConvMemberInfo info = 26;
*/
public Builder setInfo(
com.avos.avoscloud.Messages.ConvMemberInfo.Builder builderForValue) {
if (infoBuilder_ == null) {
info_ = builderForValue.build();
onChanged();
} else {
infoBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x02000000;
return this;
}
/**
* optional .com.avos.avoscloud.ConvMemberInfo info = 26;
*/
public Builder mergeInfo(com.avos.avoscloud.Messages.ConvMemberInfo value) {
if (infoBuilder_ == null) {
if (((bitField0_ & 0x02000000) == 0x02000000) &&
info_ != null &&
info_ != com.avos.avoscloud.Messages.ConvMemberInfo.getDefaultInstance()) {
info_ =
com.avos.avoscloud.Messages.ConvMemberInfo.newBuilder(info_).mergeFrom(value).buildPartial();
} else {
info_ = value;
}
onChanged();
} else {
infoBuilder_.mergeFrom(value);
}
bitField0_ |= 0x02000000;
return this;
}
/**
* optional .com.avos.avoscloud.ConvMemberInfo info = 26;
*/
public Builder clearInfo() {
if (infoBuilder_ == null) {
info_ = null;
onChanged();
} else {
infoBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x02000000);
return this;
}
/**
* optional .com.avos.avoscloud.ConvMemberInfo info = 26;
*/
public com.avos.avoscloud.Messages.ConvMemberInfo.Builder getInfoBuilder() {
bitField0_ |= 0x02000000;
onChanged();
return getInfoFieldBuilder().getBuilder();
}
/**
* optional .com.avos.avoscloud.ConvMemberInfo info = 26;
*/
public com.avos.avoscloud.Messages.ConvMemberInfoOrBuilder getInfoOrBuilder() {
if (infoBuilder_ != null) {
return infoBuilder_.getMessageOrBuilder();
} else {
return info_ == null ?
com.avos.avoscloud.Messages.ConvMemberInfo.getDefaultInstance() : info_;
}
}
/**
* optional .com.avos.avoscloud.ConvMemberInfo info = 26;
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.avos.avoscloud.Messages.ConvMemberInfo, com.avos.avoscloud.Messages.ConvMemberInfo.Builder, com.avos.avoscloud.Messages.ConvMemberInfoOrBuilder>
getInfoFieldBuilder() {
if (infoBuilder_ == null) {
infoBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
com.avos.avoscloud.Messages.ConvMemberInfo, com.avos.avoscloud.Messages.ConvMemberInfo.Builder, com.avos.avoscloud.Messages.ConvMemberInfoOrBuilder>(
getInfo(),
getParentForChildren(),
isClean());
info_ = null;
}
return infoBuilder_;
}
private boolean tempConv_ ;
/**
* optional bool tempConv = 27;
*/
public boolean hasTempConv() {
return ((bitField0_ & 0x04000000) == 0x04000000);
}
/**
* optional bool tempConv = 27;
*/
public boolean getTempConv() {
return tempConv_;
}
/**
* optional bool tempConv = 27;
*/
public Builder setTempConv(boolean value) {
bitField0_ |= 0x04000000;
tempConv_ = value;
onChanged();
return this;
}
/**
* optional bool tempConv = 27;
*/
public Builder clearTempConv() {
bitField0_ = (bitField0_ & ~0x04000000);
tempConv_ = false;
onChanged();
return this;
}
private int tempConvTTL_ ;
/**
* optional int32 tempConvTTL = 28;
*/
public boolean hasTempConvTTL() {
return ((bitField0_ & 0x08000000) == 0x08000000);
}
/**
* optional int32 tempConvTTL = 28;
*/
public int getTempConvTTL() {
return tempConvTTL_;
}
/**
* optional int32 tempConvTTL = 28;
*/
public Builder setTempConvTTL(int value) {
bitField0_ |= 0x08000000;
tempConvTTL_ = value;
onChanged();
return this;
}
/**
* optional int32 tempConvTTL = 28;
*/
public Builder clearTempConvTTL() {
bitField0_ = (bitField0_ & ~0x08000000);
tempConvTTL_ = 0;
onChanged();
return this;
}
private com.google.protobuf.LazyStringList tempConvIds_ = com.google.protobuf.LazyStringArrayList.EMPTY;
private void ensureTempConvIdsIsMutable() {
if (!((bitField0_ & 0x10000000) == 0x10000000)) {
tempConvIds_ = new com.google.protobuf.LazyStringArrayList(tempConvIds_);
bitField0_ |= 0x10000000;
}
}
/**
* repeated string tempConvIds = 29;
*/
public com.google.protobuf.ProtocolStringList
getTempConvIdsList() {
return tempConvIds_.getUnmodifiableView();
}
/**
* repeated string tempConvIds = 29;
*/
public int getTempConvIdsCount() {
return tempConvIds_.size();
}
/**
* repeated string tempConvIds = 29;
*/
public java.lang.String getTempConvIds(int index) {
return tempConvIds_.get(index);
}
/**
* repeated string tempConvIds = 29;
*/
public com.google.protobuf.ByteString
getTempConvIdsBytes(int index) {
return tempConvIds_.getByteString(index);
}
/**
* repeated string tempConvIds = 29;
*/
public Builder setTempConvIds(
int index, java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
ensureTempConvIdsIsMutable();
tempConvIds_.set(index, value);
onChanged();
return this;
}
/**
* repeated string tempConvIds = 29;
*/
public Builder addTempConvIds(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
ensureTempConvIdsIsMutable();
tempConvIds_.add(value);
onChanged();
return this;
}
/**
* repeated string tempConvIds = 29;
*/
public Builder addAllTempConvIds(
java.lang.Iterable values) {
ensureTempConvIdsIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, tempConvIds_);
onChanged();
return this;
}
/**
* repeated string tempConvIds = 29;
*/
public Builder clearTempConvIds() {
tempConvIds_ = com.google.protobuf.LazyStringArrayList.EMPTY;
bitField0_ = (bitField0_ & ~0x10000000);
onChanged();
return this;
}
/**
* repeated string tempConvIds = 29;
*/
public Builder addTempConvIdsBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
ensureTempConvIdsIsMutable();
tempConvIds_.add(value);
onChanged();
return this;
}
private com.google.protobuf.LazyStringList allowedPids_ = com.google.protobuf.LazyStringArrayList.EMPTY;
private void ensureAllowedPidsIsMutable() {
if (!((bitField0_ & 0x20000000) == 0x20000000)) {
allowedPids_ = new com.google.protobuf.LazyStringArrayList(allowedPids_);
bitField0_ |= 0x20000000;
}
}
/**
* repeated string allowedPids = 30;
*/
public com.google.protobuf.ProtocolStringList
getAllowedPidsList() {
return allowedPids_.getUnmodifiableView();
}
/**
* repeated string allowedPids = 30;
*/
public int getAllowedPidsCount() {
return allowedPids_.size();
}
/**
* repeated string allowedPids = 30;
*/
public java.lang.String getAllowedPids(int index) {
return allowedPids_.get(index);
}
/**
* repeated string allowedPids = 30;
*/
public com.google.protobuf.ByteString
getAllowedPidsBytes(int index) {
return allowedPids_.getByteString(index);
}
/**
* repeated string allowedPids = 30;
*/
public Builder setAllowedPids(
int index, java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
ensureAllowedPidsIsMutable();
allowedPids_.set(index, value);
onChanged();
return this;
}
/**
* repeated string allowedPids = 30;
*/
public Builder addAllowedPids(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
ensureAllowedPidsIsMutable();
allowedPids_.add(value);
onChanged();
return this;
}
/**
* repeated string allowedPids = 30;
*/
public Builder addAllAllowedPids(
java.lang.Iterable values) {
ensureAllowedPidsIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, allowedPids_);
onChanged();
return this;
}
/**
* repeated string allowedPids = 30;
*/
public Builder clearAllowedPids() {
allowedPids_ = com.google.protobuf.LazyStringArrayList.EMPTY;
bitField0_ = (bitField0_ & ~0x20000000);
onChanged();
return this;
}
/**
* repeated string allowedPids = 30;
*/
public Builder addAllowedPidsBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
ensureAllowedPidsIsMutable();
allowedPids_.add(value);
onChanged();
return this;
}
private java.util.List failedPids_ =
java.util.Collections.emptyList();
private void ensureFailedPidsIsMutable() {
if (!((bitField0_ & 0x40000000) == 0x40000000)) {
failedPids_ = new java.util.ArrayList(failedPids_);
bitField0_ |= 0x40000000;
}
}
private com.google.protobuf.RepeatedFieldBuilderV3<
com.avos.avoscloud.Messages.ErrorCommand, com.avos.avoscloud.Messages.ErrorCommand.Builder, com.avos.avoscloud.Messages.ErrorCommandOrBuilder> failedPidsBuilder_;
/**
* repeated .com.avos.avoscloud.ErrorCommand failedPids = 31;
*/
public java.util.List getFailedPidsList() {
if (failedPidsBuilder_ == null) {
return java.util.Collections.unmodifiableList(failedPids_);
} else {
return failedPidsBuilder_.getMessageList();
}
}
/**
* repeated .com.avos.avoscloud.ErrorCommand failedPids = 31;
*/
public int getFailedPidsCount() {
if (failedPidsBuilder_ == null) {
return failedPids_.size();
} else {
return failedPidsBuilder_.getCount();
}
}
/**
* repeated .com.avos.avoscloud.ErrorCommand failedPids = 31;
*/
public com.avos.avoscloud.Messages.ErrorCommand getFailedPids(int index) {
if (failedPidsBuilder_ == null) {
return failedPids_.get(index);
} else {
return failedPidsBuilder_.getMessage(index);
}
}
/**
* repeated .com.avos.avoscloud.ErrorCommand failedPids = 31;
*/
public Builder setFailedPids(
int index, com.avos.avoscloud.Messages.ErrorCommand value) {
if (failedPidsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureFailedPidsIsMutable();
failedPids_.set(index, value);
onChanged();
} else {
failedPidsBuilder_.setMessage(index, value);
}
return this;
}
/**
* repeated .com.avos.avoscloud.ErrorCommand failedPids = 31;
*/
public Builder setFailedPids(
int index, com.avos.avoscloud.Messages.ErrorCommand.Builder builderForValue) {
if (failedPidsBuilder_ == null) {
ensureFailedPidsIsMutable();
failedPids_.set(index, builderForValue.build());
onChanged();
} else {
failedPidsBuilder_.setMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .com.avos.avoscloud.ErrorCommand failedPids = 31;
*/
public Builder addFailedPids(com.avos.avoscloud.Messages.ErrorCommand value) {
if (failedPidsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureFailedPidsIsMutable();
failedPids_.add(value);
onChanged();
} else {
failedPidsBuilder_.addMessage(value);
}
return this;
}
/**
* repeated .com.avos.avoscloud.ErrorCommand failedPids = 31;
*/
public Builder addFailedPids(
int index, com.avos.avoscloud.Messages.ErrorCommand value) {
if (failedPidsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureFailedPidsIsMutable();
failedPids_.add(index, value);
onChanged();
} else {
failedPidsBuilder_.addMessage(index, value);
}
return this;
}
/**
* repeated .com.avos.avoscloud.ErrorCommand failedPids = 31;
*/
public Builder addFailedPids(
com.avos.avoscloud.Messages.ErrorCommand.Builder builderForValue) {
if (failedPidsBuilder_ == null) {
ensureFailedPidsIsMutable();
failedPids_.add(builderForValue.build());
onChanged();
} else {
failedPidsBuilder_.addMessage(builderForValue.build());
}
return this;
}
/**
* repeated .com.avos.avoscloud.ErrorCommand failedPids = 31;
*/
public Builder addFailedPids(
int index, com.avos.avoscloud.Messages.ErrorCommand.Builder builderForValue) {
if (failedPidsBuilder_ == null) {
ensureFailedPidsIsMutable();
failedPids_.add(index, builderForValue.build());
onChanged();
} else {
failedPidsBuilder_.addMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .com.avos.avoscloud.ErrorCommand failedPids = 31;
*/
public Builder addAllFailedPids(
java.lang.Iterable extends com.avos.avoscloud.Messages.ErrorCommand> values) {
if (failedPidsBuilder_ == null) {
ensureFailedPidsIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, failedPids_);
onChanged();
} else {
failedPidsBuilder_.addAllMessages(values);
}
return this;
}
/**
* repeated .com.avos.avoscloud.ErrorCommand failedPids = 31;
*/
public Builder clearFailedPids() {
if (failedPidsBuilder_ == null) {
failedPids_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x40000000);
onChanged();
} else {
failedPidsBuilder_.clear();
}
return this;
}
/**
* repeated .com.avos.avoscloud.ErrorCommand failedPids = 31;
*/
public Builder removeFailedPids(int index) {
if (failedPidsBuilder_ == null) {
ensureFailedPidsIsMutable();
failedPids_.remove(index);
onChanged();
} else {
failedPidsBuilder_.remove(index);
}
return this;
}
/**
* repeated .com.avos.avoscloud.ErrorCommand failedPids = 31;
*/
public com.avos.avoscloud.Messages.ErrorCommand.Builder getFailedPidsBuilder(
int index) {
return getFailedPidsFieldBuilder().getBuilder(index);
}
/**
* repeated .com.avos.avoscloud.ErrorCommand failedPids = 31;
*/
public com.avos.avoscloud.Messages.ErrorCommandOrBuilder getFailedPidsOrBuilder(
int index) {
if (failedPidsBuilder_ == null) {
return failedPids_.get(index); } else {
return failedPidsBuilder_.getMessageOrBuilder(index);
}
}
/**
* repeated .com.avos.avoscloud.ErrorCommand failedPids = 31;
*/
public java.util.List extends com.avos.avoscloud.Messages.ErrorCommandOrBuilder>
getFailedPidsOrBuilderList() {
if (failedPidsBuilder_ != null) {
return failedPidsBuilder_.getMessageOrBuilderList();
} else {
return java.util.Collections.unmodifiableList(failedPids_);
}
}
/**
* repeated .com.avos.avoscloud.ErrorCommand failedPids = 31;
*/
public com.avos.avoscloud.Messages.ErrorCommand.Builder addFailedPidsBuilder() {
return getFailedPidsFieldBuilder().addBuilder(
com.avos.avoscloud.Messages.ErrorCommand.getDefaultInstance());
}
/**
* repeated .com.avos.avoscloud.ErrorCommand failedPids = 31;
*/
public com.avos.avoscloud.Messages.ErrorCommand.Builder addFailedPidsBuilder(
int index) {
return getFailedPidsFieldBuilder().addBuilder(
index, com.avos.avoscloud.Messages.ErrorCommand.getDefaultInstance());
}
/**
* repeated .com.avos.avoscloud.ErrorCommand failedPids = 31;
*/
public java.util.List
getFailedPidsBuilderList() {
return getFailedPidsFieldBuilder().getBuilderList();
}
private com.google.protobuf.RepeatedFieldBuilderV3<
com.avos.avoscloud.Messages.ErrorCommand, com.avos.avoscloud.Messages.ErrorCommand.Builder, com.avos.avoscloud.Messages.ErrorCommandOrBuilder>
getFailedPidsFieldBuilder() {
if (failedPidsBuilder_ == null) {
failedPidsBuilder_ = new com.google.protobuf.RepeatedFieldBuilderV3<
com.avos.avoscloud.Messages.ErrorCommand, com.avos.avoscloud.Messages.ErrorCommand.Builder, com.avos.avoscloud.Messages.ErrorCommandOrBuilder>(
failedPids_,
((bitField0_ & 0x40000000) == 0x40000000),
getParentForChildren(),
isClean());
failedPids_ = null;
}
return failedPidsBuilder_;
}
private java.lang.Object next_ = "";
/**
*
* used in shutup query
*
*
* optional string next = 40;
*/
public boolean hasNext() {
return ((bitField0_ & 0x80000000) == 0x80000000);
}
/**
*
* used in shutup query
*
*
* optional string next = 40;
*/
public java.lang.String getNext() {
java.lang.Object ref = next_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
next_ = s;
}
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* used in shutup query
*
*
* optional string next = 40;
*/
public com.google.protobuf.ByteString
getNextBytes() {
java.lang.Object ref = next_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
next_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* used in shutup query
*
*
* optional string next = 40;
*/
public Builder setNext(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x80000000;
next_ = value;
onChanged();
return this;
}
/**
*
* used in shutup query
*
*
* optional string next = 40;
*/
public Builder clearNext() {
bitField0_ = (bitField0_ & ~0x80000000);
next_ = getDefaultInstance().getNext();
onChanged();
return this;
}
/**
*
* used in shutup query
*
*
* optional string next = 40;
*/
public Builder setNextBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x80000000;
next_ = value;
onChanged();
return this;
}
private com.avos.avoscloud.Messages.JsonObjectMessage results_ = null;
private com.google.protobuf.SingleFieldBuilderV3<
com.avos.avoscloud.Messages.JsonObjectMessage, com.avos.avoscloud.Messages.JsonObjectMessage.Builder, com.avos.avoscloud.Messages.JsonObjectMessageOrBuilder> resultsBuilder_;
/**
* optional .com.avos.avoscloud.JsonObjectMessage results = 100;
*/
public boolean hasResults() {
return ((bitField1_ & 0x00000001) == 0x00000001);
}
/**
* optional .com.avos.avoscloud.JsonObjectMessage results = 100;
*/
public com.avos.avoscloud.Messages.JsonObjectMessage getResults() {
if (resultsBuilder_ == null) {
return results_ == null ? com.avos.avoscloud.Messages.JsonObjectMessage.getDefaultInstance() : results_;
} else {
return resultsBuilder_.getMessage();
}
}
/**
* optional .com.avos.avoscloud.JsonObjectMessage results = 100;
*/
public Builder setResults(com.avos.avoscloud.Messages.JsonObjectMessage value) {
if (resultsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
results_ = value;
onChanged();
} else {
resultsBuilder_.setMessage(value);
}
bitField1_ |= 0x00000001;
return this;
}
/**
* optional .com.avos.avoscloud.JsonObjectMessage results = 100;
*/
public Builder setResults(
com.avos.avoscloud.Messages.JsonObjectMessage.Builder builderForValue) {
if (resultsBuilder_ == null) {
results_ = builderForValue.build();
onChanged();
} else {
resultsBuilder_.setMessage(builderForValue.build());
}
bitField1_ |= 0x00000001;
return this;
}
/**
* optional .com.avos.avoscloud.JsonObjectMessage results = 100;
*/
public Builder mergeResults(com.avos.avoscloud.Messages.JsonObjectMessage value) {
if (resultsBuilder_ == null) {
if (((bitField1_ & 0x00000001) == 0x00000001) &&
results_ != null &&
results_ != com.avos.avoscloud.Messages.JsonObjectMessage.getDefaultInstance()) {
results_ =
com.avos.avoscloud.Messages.JsonObjectMessage.newBuilder(results_).mergeFrom(value).buildPartial();
} else {
results_ = value;
}
onChanged();
} else {
resultsBuilder_.mergeFrom(value);
}
bitField1_ |= 0x00000001;
return this;
}
/**
* optional .com.avos.avoscloud.JsonObjectMessage results = 100;
*/
public Builder clearResults() {
if (resultsBuilder_ == null) {
results_ = null;
onChanged();
} else {
resultsBuilder_.clear();
}
bitField1_ = (bitField1_ & ~0x00000001);
return this;
}
/**
* optional .com.avos.avoscloud.JsonObjectMessage results = 100;
*/
public com.avos.avoscloud.Messages.JsonObjectMessage.Builder getResultsBuilder() {
bitField1_ |= 0x00000001;
onChanged();
return getResultsFieldBuilder().getBuilder();
}
/**
* optional .com.avos.avoscloud.JsonObjectMessage results = 100;
*/
public com.avos.avoscloud.Messages.JsonObjectMessageOrBuilder getResultsOrBuilder() {
if (resultsBuilder_ != null) {
return resultsBuilder_.getMessageOrBuilder();
} else {
return results_ == null ?
com.avos.avoscloud.Messages.JsonObjectMessage.getDefaultInstance() : results_;
}
}
/**
* optional .com.avos.avoscloud.JsonObjectMessage results = 100;
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.avos.avoscloud.Messages.JsonObjectMessage, com.avos.avoscloud.Messages.JsonObjectMessage.Builder, com.avos.avoscloud.Messages.JsonObjectMessageOrBuilder>
getResultsFieldBuilder() {
if (resultsBuilder_ == null) {
resultsBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
com.avos.avoscloud.Messages.JsonObjectMessage, com.avos.avoscloud.Messages.JsonObjectMessage.Builder, com.avos.avoscloud.Messages.JsonObjectMessageOrBuilder>(
getResults(),
getParentForChildren(),
isClean());
results_ = null;
}
return resultsBuilder_;
}
private com.avos.avoscloud.Messages.JsonObjectMessage where_ = null;
private com.google.protobuf.SingleFieldBuilderV3<
com.avos.avoscloud.Messages.JsonObjectMessage, com.avos.avoscloud.Messages.JsonObjectMessage.Builder, com.avos.avoscloud.Messages.JsonObjectMessageOrBuilder> whereBuilder_;
/**
* optional .com.avos.avoscloud.JsonObjectMessage where = 101;
*/
public boolean hasWhere() {
return ((bitField1_ & 0x00000002) == 0x00000002);
}
/**
* optional .com.avos.avoscloud.JsonObjectMessage where = 101;
*/
public com.avos.avoscloud.Messages.JsonObjectMessage getWhere() {
if (whereBuilder_ == null) {
return where_ == null ? com.avos.avoscloud.Messages.JsonObjectMessage.getDefaultInstance() : where_;
} else {
return whereBuilder_.getMessage();
}
}
/**
* optional .com.avos.avoscloud.JsonObjectMessage where = 101;
*/
public Builder setWhere(com.avos.avoscloud.Messages.JsonObjectMessage value) {
if (whereBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
where_ = value;
onChanged();
} else {
whereBuilder_.setMessage(value);
}
bitField1_ |= 0x00000002;
return this;
}
/**
* optional .com.avos.avoscloud.JsonObjectMessage where = 101;
*/
public Builder setWhere(
com.avos.avoscloud.Messages.JsonObjectMessage.Builder builderForValue) {
if (whereBuilder_ == null) {
where_ = builderForValue.build();
onChanged();
} else {
whereBuilder_.setMessage(builderForValue.build());
}
bitField1_ |= 0x00000002;
return this;
}
/**
* optional .com.avos.avoscloud.JsonObjectMessage where = 101;
*/
public Builder mergeWhere(com.avos.avoscloud.Messages.JsonObjectMessage value) {
if (whereBuilder_ == null) {
if (((bitField1_ & 0x00000002) == 0x00000002) &&
where_ != null &&
where_ != com.avos.avoscloud.Messages.JsonObjectMessage.getDefaultInstance()) {
where_ =
com.avos.avoscloud.Messages.JsonObjectMessage.newBuilder(where_).mergeFrom(value).buildPartial();
} else {
where_ = value;
}
onChanged();
} else {
whereBuilder_.mergeFrom(value);
}
bitField1_ |= 0x00000002;
return this;
}
/**
* optional .com.avos.avoscloud.JsonObjectMessage where = 101;
*/
public Builder clearWhere() {
if (whereBuilder_ == null) {
where_ = null;
onChanged();
} else {
whereBuilder_.clear();
}
bitField1_ = (bitField1_ & ~0x00000002);
return this;
}
/**
* optional .com.avos.avoscloud.JsonObjectMessage where = 101;
*/
public com.avos.avoscloud.Messages.JsonObjectMessage.Builder getWhereBuilder() {
bitField1_ |= 0x00000002;
onChanged();
return getWhereFieldBuilder().getBuilder();
}
/**
* optional .com.avos.avoscloud.JsonObjectMessage where = 101;
*/
public com.avos.avoscloud.Messages.JsonObjectMessageOrBuilder getWhereOrBuilder() {
if (whereBuilder_ != null) {
return whereBuilder_.getMessageOrBuilder();
} else {
return where_ == null ?
com.avos.avoscloud.Messages.JsonObjectMessage.getDefaultInstance() : where_;
}
}
/**
* optional .com.avos.avoscloud.JsonObjectMessage where = 101;
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.avos.avoscloud.Messages.JsonObjectMessage, com.avos.avoscloud.Messages.JsonObjectMessage.Builder, com.avos.avoscloud.Messages.JsonObjectMessageOrBuilder>
getWhereFieldBuilder() {
if (whereBuilder_ == null) {
whereBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
com.avos.avoscloud.Messages.JsonObjectMessage, com.avos.avoscloud.Messages.JsonObjectMessage.Builder, com.avos.avoscloud.Messages.JsonObjectMessageOrBuilder>(
getWhere(),
getParentForChildren(),
isClean());
where_ = null;
}
return whereBuilder_;
}
private com.avos.avoscloud.Messages.JsonObjectMessage attr_ = null;
private com.google.protobuf.SingleFieldBuilderV3<
com.avos.avoscloud.Messages.JsonObjectMessage, com.avos.avoscloud.Messages.JsonObjectMessage.Builder, com.avos.avoscloud.Messages.JsonObjectMessageOrBuilder> attrBuilder_;
/**
* optional .com.avos.avoscloud.JsonObjectMessage attr = 103;
*/
public boolean hasAttr() {
return ((bitField1_ & 0x00000004) == 0x00000004);
}
/**
* optional .com.avos.avoscloud.JsonObjectMessage attr = 103;
*/
public com.avos.avoscloud.Messages.JsonObjectMessage getAttr() {
if (attrBuilder_ == null) {
return attr_ == null ? com.avos.avoscloud.Messages.JsonObjectMessage.getDefaultInstance() : attr_;
} else {
return attrBuilder_.getMessage();
}
}
/**
* optional .com.avos.avoscloud.JsonObjectMessage attr = 103;
*/
public Builder setAttr(com.avos.avoscloud.Messages.JsonObjectMessage value) {
if (attrBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
attr_ = value;
onChanged();
} else {
attrBuilder_.setMessage(value);
}
bitField1_ |= 0x00000004;
return this;
}
/**
* optional .com.avos.avoscloud.JsonObjectMessage attr = 103;
*/
public Builder setAttr(
com.avos.avoscloud.Messages.JsonObjectMessage.Builder builderForValue) {
if (attrBuilder_ == null) {
attr_ = builderForValue.build();
onChanged();
} else {
attrBuilder_.setMessage(builderForValue.build());
}
bitField1_ |= 0x00000004;
return this;
}
/**
* optional .com.avos.avoscloud.JsonObjectMessage attr = 103;
*/
public Builder mergeAttr(com.avos.avoscloud.Messages.JsonObjectMessage value) {
if (attrBuilder_ == null) {
if (((bitField1_ & 0x00000004) == 0x00000004) &&
attr_ != null &&
attr_ != com.avos.avoscloud.Messages.JsonObjectMessage.getDefaultInstance()) {
attr_ =
com.avos.avoscloud.Messages.JsonObjectMessage.newBuilder(attr_).mergeFrom(value).buildPartial();
} else {
attr_ = value;
}
onChanged();
} else {
attrBuilder_.mergeFrom(value);
}
bitField1_ |= 0x00000004;
return this;
}
/**
* optional .com.avos.avoscloud.JsonObjectMessage attr = 103;
*/
public Builder clearAttr() {
if (attrBuilder_ == null) {
attr_ = null;
onChanged();
} else {
attrBuilder_.clear();
}
bitField1_ = (bitField1_ & ~0x00000004);
return this;
}
/**
* optional .com.avos.avoscloud.JsonObjectMessage attr = 103;
*/
public com.avos.avoscloud.Messages.JsonObjectMessage.Builder getAttrBuilder() {
bitField1_ |= 0x00000004;
onChanged();
return getAttrFieldBuilder().getBuilder();
}
/**
* optional .com.avos.avoscloud.JsonObjectMessage attr = 103;
*/
public com.avos.avoscloud.Messages.JsonObjectMessageOrBuilder getAttrOrBuilder() {
if (attrBuilder_ != null) {
return attrBuilder_.getMessageOrBuilder();
} else {
return attr_ == null ?
com.avos.avoscloud.Messages.JsonObjectMessage.getDefaultInstance() : attr_;
}
}
/**
* optional .com.avos.avoscloud.JsonObjectMessage attr = 103;
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.avos.avoscloud.Messages.JsonObjectMessage, com.avos.avoscloud.Messages.JsonObjectMessage.Builder, com.avos.avoscloud.Messages.JsonObjectMessageOrBuilder>
getAttrFieldBuilder() {
if (attrBuilder_ == null) {
attrBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
com.avos.avoscloud.Messages.JsonObjectMessage, com.avos.avoscloud.Messages.JsonObjectMessage.Builder, com.avos.avoscloud.Messages.JsonObjectMessageOrBuilder>(
getAttr(),
getParentForChildren(),
isClean());
attr_ = null;
}
return attrBuilder_;
}
private com.avos.avoscloud.Messages.JsonObjectMessage attrModified_ = null;
private com.google.protobuf.SingleFieldBuilderV3<
com.avos.avoscloud.Messages.JsonObjectMessage, com.avos.avoscloud.Messages.JsonObjectMessage.Builder, com.avos.avoscloud.Messages.JsonObjectMessageOrBuilder> attrModifiedBuilder_;
/**
* optional .com.avos.avoscloud.JsonObjectMessage attrModified = 104;
*/
public boolean hasAttrModified() {
return ((bitField1_ & 0x00000008) == 0x00000008);
}
/**
* optional .com.avos.avoscloud.JsonObjectMessage attrModified = 104;
*/
public com.avos.avoscloud.Messages.JsonObjectMessage getAttrModified() {
if (attrModifiedBuilder_ == null) {
return attrModified_ == null ? com.avos.avoscloud.Messages.JsonObjectMessage.getDefaultInstance() : attrModified_;
} else {
return attrModifiedBuilder_.getMessage();
}
}
/**
* optional .com.avos.avoscloud.JsonObjectMessage attrModified = 104;
*/
public Builder setAttrModified(com.avos.avoscloud.Messages.JsonObjectMessage value) {
if (attrModifiedBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
attrModified_ = value;
onChanged();
} else {
attrModifiedBuilder_.setMessage(value);
}
bitField1_ |= 0x00000008;
return this;
}
/**
* optional .com.avos.avoscloud.JsonObjectMessage attrModified = 104;
*/
public Builder setAttrModified(
com.avos.avoscloud.Messages.JsonObjectMessage.Builder builderForValue) {
if (attrModifiedBuilder_ == null) {
attrModified_ = builderForValue.build();
onChanged();
} else {
attrModifiedBuilder_.setMessage(builderForValue.build());
}
bitField1_ |= 0x00000008;
return this;
}
/**
* optional .com.avos.avoscloud.JsonObjectMessage attrModified = 104;
*/
public Builder mergeAttrModified(com.avos.avoscloud.Messages.JsonObjectMessage value) {
if (attrModifiedBuilder_ == null) {
if (((bitField1_ & 0x00000008) == 0x00000008) &&
attrModified_ != null &&
attrModified_ != com.avos.avoscloud.Messages.JsonObjectMessage.getDefaultInstance()) {
attrModified_ =
com.avos.avoscloud.Messages.JsonObjectMessage.newBuilder(attrModified_).mergeFrom(value).buildPartial();
} else {
attrModified_ = value;
}
onChanged();
} else {
attrModifiedBuilder_.mergeFrom(value);
}
bitField1_ |= 0x00000008;
return this;
}
/**
* optional .com.avos.avoscloud.JsonObjectMessage attrModified = 104;
*/
public Builder clearAttrModified() {
if (attrModifiedBuilder_ == null) {
attrModified_ = null;
onChanged();
} else {
attrModifiedBuilder_.clear();
}
bitField1_ = (bitField1_ & ~0x00000008);
return this;
}
/**
* optional .com.avos.avoscloud.JsonObjectMessage attrModified = 104;
*/
public com.avos.avoscloud.Messages.JsonObjectMessage.Builder getAttrModifiedBuilder() {
bitField1_ |= 0x00000008;
onChanged();
return getAttrModifiedFieldBuilder().getBuilder();
}
/**
* optional .com.avos.avoscloud.JsonObjectMessage attrModified = 104;
*/
public com.avos.avoscloud.Messages.JsonObjectMessageOrBuilder getAttrModifiedOrBuilder() {
if (attrModifiedBuilder_ != null) {
return attrModifiedBuilder_.getMessageOrBuilder();
} else {
return attrModified_ == null ?
com.avos.avoscloud.Messages.JsonObjectMessage.getDefaultInstance() : attrModified_;
}
}
/**
* optional .com.avos.avoscloud.JsonObjectMessage attrModified = 104;
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.avos.avoscloud.Messages.JsonObjectMessage, com.avos.avoscloud.Messages.JsonObjectMessage.Builder, com.avos.avoscloud.Messages.JsonObjectMessageOrBuilder>
getAttrModifiedFieldBuilder() {
if (attrModifiedBuilder_ == null) {
attrModifiedBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
com.avos.avoscloud.Messages.JsonObjectMessage, com.avos.avoscloud.Messages.JsonObjectMessage.Builder, com.avos.avoscloud.Messages.JsonObjectMessageOrBuilder>(
getAttrModified(),
getParentForChildren(),
isClean());
attrModified_ = null;
}
return attrModifiedBuilder_;
}
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:com.avos.avoscloud.ConvCommand)
}
// @@protoc_insertion_point(class_scope:com.avos.avoscloud.ConvCommand)
private static final com.avos.avoscloud.Messages.ConvCommand DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new com.avos.avoscloud.Messages.ConvCommand();
}
public static com.avos.avoscloud.Messages.ConvCommand getDefaultInstance() {
return DEFAULT_INSTANCE;
}
@java.lang.Deprecated public static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
public ConvCommand parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new ConvCommand(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
public com.avos.avoscloud.Messages.ConvCommand getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface RoomCommandOrBuilder extends
// @@protoc_insertion_point(interface_extends:com.avos.avoscloud.RoomCommand)
com.google.protobuf.MessageOrBuilder {
/**
* optional string roomId = 1;
*/
boolean hasRoomId();
/**
* optional string roomId = 1;
*/
java.lang.String getRoomId();
/**
* optional string roomId = 1;
*/
com.google.protobuf.ByteString
getRoomIdBytes();
/**
* optional string s = 2;
*/
boolean hasS();
/**
* optional string s = 2;
*/
java.lang.String getS();
/**
* optional string s = 2;
*/
com.google.protobuf.ByteString
getSBytes();
/**
* optional int64 t = 3;
*/
boolean hasT();
/**
* optional int64 t = 3;
*/
long getT();
/**
* optional string n = 4;
*/
boolean hasN();
/**
* optional string n = 4;
*/
java.lang.String getN();
/**
* optional string n = 4;
*/
com.google.protobuf.ByteString
getNBytes();
/**
* optional bool transient = 5;
*/
boolean hasTransient();
/**
* optional bool transient = 5;
*/
boolean getTransient();
/**
* repeated string roomPeerIds = 6;
*/
java.util.List
getRoomPeerIdsList();
/**
* repeated string roomPeerIds = 6;
*/
int getRoomPeerIdsCount();
/**
* repeated string roomPeerIds = 6;
*/
java.lang.String getRoomPeerIds(int index);
/**
* repeated string roomPeerIds = 6;
*/
com.google.protobuf.ByteString
getRoomPeerIdsBytes(int index);
/**
* optional string byPeerId = 7;
*/
boolean hasByPeerId();
/**
* optional string byPeerId = 7;
*/
java.lang.String getByPeerId();
/**
* optional string byPeerId = 7;
*/
com.google.protobuf.ByteString
getByPeerIdBytes();
}
/**
* Protobuf type {@code com.avos.avoscloud.RoomCommand}
*/
public static final class RoomCommand extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:com.avos.avoscloud.RoomCommand)
RoomCommandOrBuilder {
private static final long serialVersionUID = 0L;
// Use RoomCommand.newBuilder() to construct.
private RoomCommand(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private RoomCommand() {
roomId_ = "";
s_ = "";
t_ = 0L;
n_ = "";
transient_ = false;
roomPeerIds_ = com.google.protobuf.LazyStringArrayList.EMPTY;
byPeerId_ = "";
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private RoomCommand(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!parseUnknownField(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
case 10: {
com.google.protobuf.ByteString bs = input.readBytes();
bitField0_ |= 0x00000001;
roomId_ = bs;
break;
}
case 18: {
com.google.protobuf.ByteString bs = input.readBytes();
bitField0_ |= 0x00000002;
s_ = bs;
break;
}
case 24: {
bitField0_ |= 0x00000004;
t_ = input.readInt64();
break;
}
case 34: {
com.google.protobuf.ByteString bs = input.readBytes();
bitField0_ |= 0x00000008;
n_ = bs;
break;
}
case 40: {
bitField0_ |= 0x00000010;
transient_ = input.readBool();
break;
}
case 50: {
com.google.protobuf.ByteString bs = input.readBytes();
if (!((mutable_bitField0_ & 0x00000020) == 0x00000020)) {
roomPeerIds_ = new com.google.protobuf.LazyStringArrayList();
mutable_bitField0_ |= 0x00000020;
}
roomPeerIds_.add(bs);
break;
}
case 58: {
com.google.protobuf.ByteString bs = input.readBytes();
bitField0_ |= 0x00000020;
byPeerId_ = bs;
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
if (((mutable_bitField0_ & 0x00000020) == 0x00000020)) {
roomPeerIds_ = roomPeerIds_.getUnmodifiableView();
}
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.avos.avoscloud.Messages.internal_static_com_avos_avoscloud_RoomCommand_descriptor;
}
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.avos.avoscloud.Messages.internal_static_com_avos_avoscloud_RoomCommand_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.avos.avoscloud.Messages.RoomCommand.class, com.avos.avoscloud.Messages.RoomCommand.Builder.class);
}
private int bitField0_;
public static final int ROOMID_FIELD_NUMBER = 1;
private volatile java.lang.Object roomId_;
/**
* optional string roomId = 1;
*/
public boolean hasRoomId() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
* optional string roomId = 1;
*/
public java.lang.String getRoomId() {
java.lang.Object ref = roomId_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
roomId_ = s;
}
return s;
}
}
/**
* optional string roomId = 1;
*/
public com.google.protobuf.ByteString
getRoomIdBytes() {
java.lang.Object ref = roomId_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
roomId_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int S_FIELD_NUMBER = 2;
private volatile java.lang.Object s_;
/**
* optional string s = 2;
*/
public boolean hasS() {
return ((bitField0_ & 0x00000002) == 0x00000002);
}
/**
* optional string s = 2;
*/
public java.lang.String getS() {
java.lang.Object ref = s_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
s_ = s;
}
return s;
}
}
/**
* optional string s = 2;
*/
public com.google.protobuf.ByteString
getSBytes() {
java.lang.Object ref = s_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
s_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int T_FIELD_NUMBER = 3;
private long t_;
/**
* optional int64 t = 3;
*/
public boolean hasT() {
return ((bitField0_ & 0x00000004) == 0x00000004);
}
/**
* optional int64 t = 3;
*/
public long getT() {
return t_;
}
public static final int N_FIELD_NUMBER = 4;
private volatile java.lang.Object n_;
/**
* optional string n = 4;
*/
public boolean hasN() {
return ((bitField0_ & 0x00000008) == 0x00000008);
}
/**
* optional string n = 4;
*/
public java.lang.String getN() {
java.lang.Object ref = n_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
n_ = s;
}
return s;
}
}
/**
* optional string n = 4;
*/
public com.google.protobuf.ByteString
getNBytes() {
java.lang.Object ref = n_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
n_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int TRANSIENT_FIELD_NUMBER = 5;
private boolean transient_;
/**
* optional bool transient = 5;
*/
public boolean hasTransient() {
return ((bitField0_ & 0x00000010) == 0x00000010);
}
/**
* optional bool transient = 5;
*/
public boolean getTransient() {
return transient_;
}
public static final int ROOMPEERIDS_FIELD_NUMBER = 6;
private com.google.protobuf.LazyStringList roomPeerIds_;
/**
* repeated string roomPeerIds = 6;
*/
public com.google.protobuf.ProtocolStringList
getRoomPeerIdsList() {
return roomPeerIds_;
}
/**
* repeated string roomPeerIds = 6;
*/
public int getRoomPeerIdsCount() {
return roomPeerIds_.size();
}
/**
* repeated string roomPeerIds = 6;
*/
public java.lang.String getRoomPeerIds(int index) {
return roomPeerIds_.get(index);
}
/**
* repeated string roomPeerIds = 6;
*/
public com.google.protobuf.ByteString
getRoomPeerIdsBytes(int index) {
return roomPeerIds_.getByteString(index);
}
public static final int BYPEERID_FIELD_NUMBER = 7;
private volatile java.lang.Object byPeerId_;
/**
* optional string byPeerId = 7;
*/
public boolean hasByPeerId() {
return ((bitField0_ & 0x00000020) == 0x00000020);
}
/**
* optional string byPeerId = 7;
*/
public java.lang.String getByPeerId() {
java.lang.Object ref = byPeerId_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
byPeerId_ = s;
}
return s;
}
}
/**
* optional string byPeerId = 7;
*/
public com.google.protobuf.ByteString
getByPeerIdBytes() {
java.lang.Object ref = byPeerId_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
byPeerId_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
private byte memoizedIsInitialized = -1;
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (((bitField0_ & 0x00000001) == 0x00000001)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 1, roomId_);
}
if (((bitField0_ & 0x00000002) == 0x00000002)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 2, s_);
}
if (((bitField0_ & 0x00000004) == 0x00000004)) {
output.writeInt64(3, t_);
}
if (((bitField0_ & 0x00000008) == 0x00000008)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 4, n_);
}
if (((bitField0_ & 0x00000010) == 0x00000010)) {
output.writeBool(5, transient_);
}
for (int i = 0; i < roomPeerIds_.size(); i++) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 6, roomPeerIds_.getRaw(i));
}
if (((bitField0_ & 0x00000020) == 0x00000020)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 7, byPeerId_);
}
unknownFields.writeTo(output);
}
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (((bitField0_ & 0x00000001) == 0x00000001)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(1, roomId_);
}
if (((bitField0_ & 0x00000002) == 0x00000002)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(2, s_);
}
if (((bitField0_ & 0x00000004) == 0x00000004)) {
size += com.google.protobuf.CodedOutputStream
.computeInt64Size(3, t_);
}
if (((bitField0_ & 0x00000008) == 0x00000008)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(4, n_);
}
if (((bitField0_ & 0x00000010) == 0x00000010)) {
size += com.google.protobuf.CodedOutputStream
.computeBoolSize(5, transient_);
}
{
int dataSize = 0;
for (int i = 0; i < roomPeerIds_.size(); i++) {
dataSize += computeStringSizeNoTag(roomPeerIds_.getRaw(i));
}
size += dataSize;
size += 1 * getRoomPeerIdsList().size();
}
if (((bitField0_ & 0x00000020) == 0x00000020)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(7, byPeerId_);
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof com.avos.avoscloud.Messages.RoomCommand)) {
return super.equals(obj);
}
com.avos.avoscloud.Messages.RoomCommand other = (com.avos.avoscloud.Messages.RoomCommand) obj;
boolean result = true;
result = result && (hasRoomId() == other.hasRoomId());
if (hasRoomId()) {
result = result && getRoomId()
.equals(other.getRoomId());
}
result = result && (hasS() == other.hasS());
if (hasS()) {
result = result && getS()
.equals(other.getS());
}
result = result && (hasT() == other.hasT());
if (hasT()) {
result = result && (getT()
== other.getT());
}
result = result && (hasN() == other.hasN());
if (hasN()) {
result = result && getN()
.equals(other.getN());
}
result = result && (hasTransient() == other.hasTransient());
if (hasTransient()) {
result = result && (getTransient()
== other.getTransient());
}
result = result && getRoomPeerIdsList()
.equals(other.getRoomPeerIdsList());
result = result && (hasByPeerId() == other.hasByPeerId());
if (hasByPeerId()) {
result = result && getByPeerId()
.equals(other.getByPeerId());
}
result = result && unknownFields.equals(other.unknownFields);
return result;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (hasRoomId()) {
hash = (37 * hash) + ROOMID_FIELD_NUMBER;
hash = (53 * hash) + getRoomId().hashCode();
}
if (hasS()) {
hash = (37 * hash) + S_FIELD_NUMBER;
hash = (53 * hash) + getS().hashCode();
}
if (hasT()) {
hash = (37 * hash) + T_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getT());
}
if (hasN()) {
hash = (37 * hash) + N_FIELD_NUMBER;
hash = (53 * hash) + getN().hashCode();
}
if (hasTransient()) {
hash = (37 * hash) + TRANSIENT_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashBoolean(
getTransient());
}
if (getRoomPeerIdsCount() > 0) {
hash = (37 * hash) + ROOMPEERIDS_FIELD_NUMBER;
hash = (53 * hash) + getRoomPeerIdsList().hashCode();
}
if (hasByPeerId()) {
hash = (37 * hash) + BYPEERID_FIELD_NUMBER;
hash = (53 * hash) + getByPeerId().hashCode();
}
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static com.avos.avoscloud.Messages.RoomCommand parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.avos.avoscloud.Messages.RoomCommand parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.avos.avoscloud.Messages.RoomCommand parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.avos.avoscloud.Messages.RoomCommand parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.avos.avoscloud.Messages.RoomCommand parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.avos.avoscloud.Messages.RoomCommand parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.avos.avoscloud.Messages.RoomCommand parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.avos.avoscloud.Messages.RoomCommand parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static com.avos.avoscloud.Messages.RoomCommand parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static com.avos.avoscloud.Messages.RoomCommand parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static com.avos.avoscloud.Messages.RoomCommand parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.avos.avoscloud.Messages.RoomCommand parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(com.avos.avoscloud.Messages.RoomCommand prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code com.avos.avoscloud.RoomCommand}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:com.avos.avoscloud.RoomCommand)
com.avos.avoscloud.Messages.RoomCommandOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.avos.avoscloud.Messages.internal_static_com_avos_avoscloud_RoomCommand_descriptor;
}
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.avos.avoscloud.Messages.internal_static_com_avos_avoscloud_RoomCommand_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.avos.avoscloud.Messages.RoomCommand.class, com.avos.avoscloud.Messages.RoomCommand.Builder.class);
}
// Construct using com.avos.avoscloud.Messages.RoomCommand.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
public Builder clear() {
super.clear();
roomId_ = "";
bitField0_ = (bitField0_ & ~0x00000001);
s_ = "";
bitField0_ = (bitField0_ & ~0x00000002);
t_ = 0L;
bitField0_ = (bitField0_ & ~0x00000004);
n_ = "";
bitField0_ = (bitField0_ & ~0x00000008);
transient_ = false;
bitField0_ = (bitField0_ & ~0x00000010);
roomPeerIds_ = com.google.protobuf.LazyStringArrayList.EMPTY;
bitField0_ = (bitField0_ & ~0x00000020);
byPeerId_ = "";
bitField0_ = (bitField0_ & ~0x00000040);
return this;
}
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return com.avos.avoscloud.Messages.internal_static_com_avos_avoscloud_RoomCommand_descriptor;
}
public com.avos.avoscloud.Messages.RoomCommand getDefaultInstanceForType() {
return com.avos.avoscloud.Messages.RoomCommand.getDefaultInstance();
}
public com.avos.avoscloud.Messages.RoomCommand build() {
com.avos.avoscloud.Messages.RoomCommand result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
public com.avos.avoscloud.Messages.RoomCommand buildPartial() {
com.avos.avoscloud.Messages.RoomCommand result = new com.avos.avoscloud.Messages.RoomCommand(this);
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (((from_bitField0_ & 0x00000001) == 0x00000001)) {
to_bitField0_ |= 0x00000001;
}
result.roomId_ = roomId_;
if (((from_bitField0_ & 0x00000002) == 0x00000002)) {
to_bitField0_ |= 0x00000002;
}
result.s_ = s_;
if (((from_bitField0_ & 0x00000004) == 0x00000004)) {
to_bitField0_ |= 0x00000004;
}
result.t_ = t_;
if (((from_bitField0_ & 0x00000008) == 0x00000008)) {
to_bitField0_ |= 0x00000008;
}
result.n_ = n_;
if (((from_bitField0_ & 0x00000010) == 0x00000010)) {
to_bitField0_ |= 0x00000010;
}
result.transient_ = transient_;
if (((bitField0_ & 0x00000020) == 0x00000020)) {
roomPeerIds_ = roomPeerIds_.getUnmodifiableView();
bitField0_ = (bitField0_ & ~0x00000020);
}
result.roomPeerIds_ = roomPeerIds_;
if (((from_bitField0_ & 0x00000040) == 0x00000040)) {
to_bitField0_ |= 0x00000020;
}
result.byPeerId_ = byPeerId_;
result.bitField0_ = to_bitField0_;
onBuilt();
return result;
}
public Builder clone() {
return (Builder) super.clone();
}
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.setField(field, value);
}
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return (Builder) super.clearField(field);
}
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return (Builder) super.clearOneof(oneof);
}
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return (Builder) super.setRepeatedField(field, index, value);
}
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.addRepeatedField(field, value);
}
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.avos.avoscloud.Messages.RoomCommand) {
return mergeFrom((com.avos.avoscloud.Messages.RoomCommand)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.avos.avoscloud.Messages.RoomCommand other) {
if (other == com.avos.avoscloud.Messages.RoomCommand.getDefaultInstance()) return this;
if (other.hasRoomId()) {
bitField0_ |= 0x00000001;
roomId_ = other.roomId_;
onChanged();
}
if (other.hasS()) {
bitField0_ |= 0x00000002;
s_ = other.s_;
onChanged();
}
if (other.hasT()) {
setT(other.getT());
}
if (other.hasN()) {
bitField0_ |= 0x00000008;
n_ = other.n_;
onChanged();
}
if (other.hasTransient()) {
setTransient(other.getTransient());
}
if (!other.roomPeerIds_.isEmpty()) {
if (roomPeerIds_.isEmpty()) {
roomPeerIds_ = other.roomPeerIds_;
bitField0_ = (bitField0_ & ~0x00000020);
} else {
ensureRoomPeerIdsIsMutable();
roomPeerIds_.addAll(other.roomPeerIds_);
}
onChanged();
}
if (other.hasByPeerId()) {
bitField0_ |= 0x00000040;
byPeerId_ = other.byPeerId_;
onChanged();
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
public final boolean isInitialized() {
return true;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
com.avos.avoscloud.Messages.RoomCommand parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (com.avos.avoscloud.Messages.RoomCommand) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
private java.lang.Object roomId_ = "";
/**
* optional string roomId = 1;
*/
public boolean hasRoomId() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
* optional string roomId = 1;
*/
public java.lang.String getRoomId() {
java.lang.Object ref = roomId_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
roomId_ = s;
}
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* optional string roomId = 1;
*/
public com.google.protobuf.ByteString
getRoomIdBytes() {
java.lang.Object ref = roomId_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
roomId_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* optional string roomId = 1;
*/
public Builder setRoomId(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000001;
roomId_ = value;
onChanged();
return this;
}
/**
* optional string roomId = 1;
*/
public Builder clearRoomId() {
bitField0_ = (bitField0_ & ~0x00000001);
roomId_ = getDefaultInstance().getRoomId();
onChanged();
return this;
}
/**
* optional string roomId = 1;
*/
public Builder setRoomIdBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000001;
roomId_ = value;
onChanged();
return this;
}
private java.lang.Object s_ = "";
/**
* optional string s = 2;
*/
public boolean hasS() {
return ((bitField0_ & 0x00000002) == 0x00000002);
}
/**
* optional string s = 2;
*/
public java.lang.String getS() {
java.lang.Object ref = s_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
s_ = s;
}
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* optional string s = 2;
*/
public com.google.protobuf.ByteString
getSBytes() {
java.lang.Object ref = s_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
s_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* optional string s = 2;
*/
public Builder setS(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000002;
s_ = value;
onChanged();
return this;
}
/**
* optional string s = 2;
*/
public Builder clearS() {
bitField0_ = (bitField0_ & ~0x00000002);
s_ = getDefaultInstance().getS();
onChanged();
return this;
}
/**
* optional string s = 2;
*/
public Builder setSBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000002;
s_ = value;
onChanged();
return this;
}
private long t_ ;
/**
* optional int64 t = 3;
*/
public boolean hasT() {
return ((bitField0_ & 0x00000004) == 0x00000004);
}
/**
* optional int64 t = 3;
*/
public long getT() {
return t_;
}
/**
* optional int64 t = 3;
*/
public Builder setT(long value) {
bitField0_ |= 0x00000004;
t_ = value;
onChanged();
return this;
}
/**
* optional int64 t = 3;
*/
public Builder clearT() {
bitField0_ = (bitField0_ & ~0x00000004);
t_ = 0L;
onChanged();
return this;
}
private java.lang.Object n_ = "";
/**
* optional string n = 4;
*/
public boolean hasN() {
return ((bitField0_ & 0x00000008) == 0x00000008);
}
/**
* optional string n = 4;
*/
public java.lang.String getN() {
java.lang.Object ref = n_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
n_ = s;
}
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* optional string n = 4;
*/
public com.google.protobuf.ByteString
getNBytes() {
java.lang.Object ref = n_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
n_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* optional string n = 4;
*/
public Builder setN(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000008;
n_ = value;
onChanged();
return this;
}
/**
* optional string n = 4;
*/
public Builder clearN() {
bitField0_ = (bitField0_ & ~0x00000008);
n_ = getDefaultInstance().getN();
onChanged();
return this;
}
/**
* optional string n = 4;
*/
public Builder setNBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000008;
n_ = value;
onChanged();
return this;
}
private boolean transient_ ;
/**
* optional bool transient = 5;
*/
public boolean hasTransient() {
return ((bitField0_ & 0x00000010) == 0x00000010);
}
/**
* optional bool transient = 5;
*/
public boolean getTransient() {
return transient_;
}
/**
* optional bool transient = 5;
*/
public Builder setTransient(boolean value) {
bitField0_ |= 0x00000010;
transient_ = value;
onChanged();
return this;
}
/**
* optional bool transient = 5;
*/
public Builder clearTransient() {
bitField0_ = (bitField0_ & ~0x00000010);
transient_ = false;
onChanged();
return this;
}
private com.google.protobuf.LazyStringList roomPeerIds_ = com.google.protobuf.LazyStringArrayList.EMPTY;
private void ensureRoomPeerIdsIsMutable() {
if (!((bitField0_ & 0x00000020) == 0x00000020)) {
roomPeerIds_ = new com.google.protobuf.LazyStringArrayList(roomPeerIds_);
bitField0_ |= 0x00000020;
}
}
/**
* repeated string roomPeerIds = 6;
*/
public com.google.protobuf.ProtocolStringList
getRoomPeerIdsList() {
return roomPeerIds_.getUnmodifiableView();
}
/**
* repeated string roomPeerIds = 6;
*/
public int getRoomPeerIdsCount() {
return roomPeerIds_.size();
}
/**
* repeated string roomPeerIds = 6;
*/
public java.lang.String getRoomPeerIds(int index) {
return roomPeerIds_.get(index);
}
/**
* repeated string roomPeerIds = 6;
*/
public com.google.protobuf.ByteString
getRoomPeerIdsBytes(int index) {
return roomPeerIds_.getByteString(index);
}
/**
* repeated string roomPeerIds = 6;
*/
public Builder setRoomPeerIds(
int index, java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
ensureRoomPeerIdsIsMutable();
roomPeerIds_.set(index, value);
onChanged();
return this;
}
/**
* repeated string roomPeerIds = 6;
*/
public Builder addRoomPeerIds(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
ensureRoomPeerIdsIsMutable();
roomPeerIds_.add(value);
onChanged();
return this;
}
/**
* repeated string roomPeerIds = 6;
*/
public Builder addAllRoomPeerIds(
java.lang.Iterable values) {
ensureRoomPeerIdsIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, roomPeerIds_);
onChanged();
return this;
}
/**
* repeated string roomPeerIds = 6;
*/
public Builder clearRoomPeerIds() {
roomPeerIds_ = com.google.protobuf.LazyStringArrayList.EMPTY;
bitField0_ = (bitField0_ & ~0x00000020);
onChanged();
return this;
}
/**
* repeated string roomPeerIds = 6;
*/
public Builder addRoomPeerIdsBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
ensureRoomPeerIdsIsMutable();
roomPeerIds_.add(value);
onChanged();
return this;
}
private java.lang.Object byPeerId_ = "";
/**
* optional string byPeerId = 7;
*/
public boolean hasByPeerId() {
return ((bitField0_ & 0x00000040) == 0x00000040);
}
/**
* optional string byPeerId = 7;
*/
public java.lang.String getByPeerId() {
java.lang.Object ref = byPeerId_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
byPeerId_ = s;
}
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* optional string byPeerId = 7;
*/
public com.google.protobuf.ByteString
getByPeerIdBytes() {
java.lang.Object ref = byPeerId_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
byPeerId_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* optional string byPeerId = 7;
*/
public Builder setByPeerId(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000040;
byPeerId_ = value;
onChanged();
return this;
}
/**
* optional string byPeerId = 7;
*/
public Builder clearByPeerId() {
bitField0_ = (bitField0_ & ~0x00000040);
byPeerId_ = getDefaultInstance().getByPeerId();
onChanged();
return this;
}
/**
* optional string byPeerId = 7;
*/
public Builder setByPeerIdBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000040;
byPeerId_ = value;
onChanged();
return this;
}
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:com.avos.avoscloud.RoomCommand)
}
// @@protoc_insertion_point(class_scope:com.avos.avoscloud.RoomCommand)
private static final com.avos.avoscloud.Messages.RoomCommand DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new com.avos.avoscloud.Messages.RoomCommand();
}
public static com.avos.avoscloud.Messages.RoomCommand getDefaultInstance() {
return DEFAULT_INSTANCE;
}
@java.lang.Deprecated public static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
public RoomCommand parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new RoomCommand(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
public com.avos.avoscloud.Messages.RoomCommand getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface LogsCommandOrBuilder extends
// @@protoc_insertion_point(interface_extends:com.avos.avoscloud.LogsCommand)
com.google.protobuf.MessageOrBuilder {
/**
* optional string cid = 1;
*/
boolean hasCid();
/**
* optional string cid = 1;
*/
java.lang.String getCid();
/**
* optional string cid = 1;
*/
com.google.protobuf.ByteString
getCidBytes();
/**
* optional int32 l = 2;
*/
boolean hasL();
/**
* optional int32 l = 2;
*/
int getL();
/**
* optional int32 limit = 3;
*/
boolean hasLimit();
/**
* optional int32 limit = 3;
*/
int getLimit();
/**
* optional int64 t = 4;
*/
boolean hasT();
/**
* optional int64 t = 4;
*/
long getT();
/**
* optional int64 tt = 5;
*/
boolean hasTt();
/**
* optional int64 tt = 5;
*/
long getTt();
/**
* optional string tmid = 6;
*/
boolean hasTmid();
/**
* optional string tmid = 6;
*/
java.lang.String getTmid();
/**
* optional string tmid = 6;
*/
com.google.protobuf.ByteString
getTmidBytes();
/**
* optional string mid = 7;
*/
boolean hasMid();
/**
* optional string mid = 7;
*/
java.lang.String getMid();
/**
* optional string mid = 7;
*/
com.google.protobuf.ByteString
getMidBytes();
/**
* optional string checksum = 8;
*/
boolean hasChecksum();
/**
* optional string checksum = 8;
*/
java.lang.String getChecksum();
/**
* optional string checksum = 8;
*/
com.google.protobuf.ByteString
getChecksumBytes();
/**
* optional bool stored = 9;
*/
boolean hasStored();
/**
* optional bool stored = 9;
*/
boolean getStored();
/**
* optional .com.avos.avoscloud.LogsCommand.QueryDirection direction = 10 [default = OLD];
*/
boolean hasDirection();
/**
* optional .com.avos.avoscloud.LogsCommand.QueryDirection direction = 10 [default = OLD];
*/
com.avos.avoscloud.Messages.LogsCommand.QueryDirection getDirection();
/**
* optional bool tIncluded = 11;
*/
boolean hasTIncluded();
/**
* optional bool tIncluded = 11;
*/
boolean getTIncluded();
/**
* optional bool ttIncluded = 12;
*/
boolean hasTtIncluded();
/**
* optional bool ttIncluded = 12;
*/
boolean getTtIncluded();
/**
* optional int32 lctype = 13;
*/
boolean hasLctype();
/**
* optional int32 lctype = 13;
*/
int getLctype();
/**
* repeated .com.avos.avoscloud.LogItem logs = 105;
*/
java.util.List
getLogsList();
/**
* repeated .com.avos.avoscloud.LogItem logs = 105;
*/
com.avos.avoscloud.Messages.LogItem getLogs(int index);
/**
* repeated .com.avos.avoscloud.LogItem logs = 105;
*/
int getLogsCount();
/**
* repeated .com.avos.avoscloud.LogItem logs = 105;
*/
java.util.List extends com.avos.avoscloud.Messages.LogItemOrBuilder>
getLogsOrBuilderList();
/**
* repeated .com.avos.avoscloud.LogItem logs = 105;
*/
com.avos.avoscloud.Messages.LogItemOrBuilder getLogsOrBuilder(
int index);
}
/**
* Protobuf type {@code com.avos.avoscloud.LogsCommand}
*/
public static final class LogsCommand extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:com.avos.avoscloud.LogsCommand)
LogsCommandOrBuilder {
private static final long serialVersionUID = 0L;
// Use LogsCommand.newBuilder() to construct.
private LogsCommand(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private LogsCommand() {
cid_ = "";
l_ = 0;
limit_ = 0;
t_ = 0L;
tt_ = 0L;
tmid_ = "";
mid_ = "";
checksum_ = "";
stored_ = false;
direction_ = 1;
tIncluded_ = false;
ttIncluded_ = false;
lctype_ = 0;
logs_ = java.util.Collections.emptyList();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private LogsCommand(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!parseUnknownField(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
case 10: {
com.google.protobuf.ByteString bs = input.readBytes();
bitField0_ |= 0x00000001;
cid_ = bs;
break;
}
case 16: {
bitField0_ |= 0x00000002;
l_ = input.readInt32();
break;
}
case 24: {
bitField0_ |= 0x00000004;
limit_ = input.readInt32();
break;
}
case 32: {
bitField0_ |= 0x00000008;
t_ = input.readInt64();
break;
}
case 40: {
bitField0_ |= 0x00000010;
tt_ = input.readInt64();
break;
}
case 50: {
com.google.protobuf.ByteString bs = input.readBytes();
bitField0_ |= 0x00000020;
tmid_ = bs;
break;
}
case 58: {
com.google.protobuf.ByteString bs = input.readBytes();
bitField0_ |= 0x00000040;
mid_ = bs;
break;
}
case 66: {
com.google.protobuf.ByteString bs = input.readBytes();
bitField0_ |= 0x00000080;
checksum_ = bs;
break;
}
case 72: {
bitField0_ |= 0x00000100;
stored_ = input.readBool();
break;
}
case 80: {
int rawValue = input.readEnum();
com.avos.avoscloud.Messages.LogsCommand.QueryDirection value = com.avos.avoscloud.Messages.LogsCommand.QueryDirection.valueOf(rawValue);
if (value == null) {
unknownFields.mergeVarintField(10, rawValue);
} else {
bitField0_ |= 0x00000200;
direction_ = rawValue;
}
break;
}
case 88: {
bitField0_ |= 0x00000400;
tIncluded_ = input.readBool();
break;
}
case 96: {
bitField0_ |= 0x00000800;
ttIncluded_ = input.readBool();
break;
}
case 104: {
bitField0_ |= 0x00001000;
lctype_ = input.readInt32();
break;
}
case 842: {
if (!((mutable_bitField0_ & 0x00002000) == 0x00002000)) {
logs_ = new java.util.ArrayList();
mutable_bitField0_ |= 0x00002000;
}
logs_.add(
input.readMessage(com.avos.avoscloud.Messages.LogItem.PARSER, extensionRegistry));
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
if (((mutable_bitField0_ & 0x00002000) == 0x00002000)) {
logs_ = java.util.Collections.unmodifiableList(logs_);
}
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.avos.avoscloud.Messages.internal_static_com_avos_avoscloud_LogsCommand_descriptor;
}
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.avos.avoscloud.Messages.internal_static_com_avos_avoscloud_LogsCommand_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.avos.avoscloud.Messages.LogsCommand.class, com.avos.avoscloud.Messages.LogsCommand.Builder.class);
}
/**
* Protobuf enum {@code com.avos.avoscloud.LogsCommand.QueryDirection}
*/
public enum QueryDirection
implements com.google.protobuf.ProtocolMessageEnum {
/**
* OLD = 1;
*/
OLD(1),
/**
* NEW = 2;
*/
NEW(2),
;
/**
* OLD = 1;
*/
public static final int OLD_VALUE = 1;
/**
* NEW = 2;
*/
public static final int NEW_VALUE = 2;
public final int getNumber() {
return value;
}
/**
* @deprecated Use {@link #forNumber(int)} instead.
*/
@java.lang.Deprecated
public static QueryDirection valueOf(int value) {
return forNumber(value);
}
public static QueryDirection forNumber(int value) {
switch (value) {
case 1: return OLD;
case 2: return NEW;
default: return null;
}
}
public static com.google.protobuf.Internal.EnumLiteMap
internalGetValueMap() {
return internalValueMap;
}
private static final com.google.protobuf.Internal.EnumLiteMap<
QueryDirection> internalValueMap =
new com.google.protobuf.Internal.EnumLiteMap() {
public QueryDirection findValueByNumber(int number) {
return QueryDirection.forNumber(number);
}
};
public final com.google.protobuf.Descriptors.EnumValueDescriptor
getValueDescriptor() {
return getDescriptor().getValues().get(ordinal());
}
public final com.google.protobuf.Descriptors.EnumDescriptor
getDescriptorForType() {
return getDescriptor();
}
public static final com.google.protobuf.Descriptors.EnumDescriptor
getDescriptor() {
return com.avos.avoscloud.Messages.LogsCommand.getDescriptor().getEnumTypes().get(0);
}
private static final QueryDirection[] VALUES = values();
public static QueryDirection valueOf(
com.google.protobuf.Descriptors.EnumValueDescriptor desc) {
if (desc.getType() != getDescriptor()) {
throw new java.lang.IllegalArgumentException(
"EnumValueDescriptor is not for this type.");
}
return VALUES[desc.getIndex()];
}
private final int value;
private QueryDirection(int value) {
this.value = value;
}
// @@protoc_insertion_point(enum_scope:com.avos.avoscloud.LogsCommand.QueryDirection)
}
private int bitField0_;
public static final int CID_FIELD_NUMBER = 1;
private volatile java.lang.Object cid_;
/**
* optional string cid = 1;
*/
public boolean hasCid() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
* optional string cid = 1;
*/
public java.lang.String getCid() {
java.lang.Object ref = cid_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
cid_ = s;
}
return s;
}
}
/**
* optional string cid = 1;
*/
public com.google.protobuf.ByteString
getCidBytes() {
java.lang.Object ref = cid_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
cid_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int L_FIELD_NUMBER = 2;
private int l_;
/**
* optional int32 l = 2;
*/
public boolean hasL() {
return ((bitField0_ & 0x00000002) == 0x00000002);
}
/**
* optional int32 l = 2;
*/
public int getL() {
return l_;
}
public static final int LIMIT_FIELD_NUMBER = 3;
private int limit_;
/**
* optional int32 limit = 3;
*/
public boolean hasLimit() {
return ((bitField0_ & 0x00000004) == 0x00000004);
}
/**
* optional int32 limit = 3;
*/
public int getLimit() {
return limit_;
}
public static final int T_FIELD_NUMBER = 4;
private long t_;
/**
* optional int64 t = 4;
*/
public boolean hasT() {
return ((bitField0_ & 0x00000008) == 0x00000008);
}
/**
* optional int64 t = 4;
*/
public long getT() {
return t_;
}
public static final int TT_FIELD_NUMBER = 5;
private long tt_;
/**
* optional int64 tt = 5;
*/
public boolean hasTt() {
return ((bitField0_ & 0x00000010) == 0x00000010);
}
/**
* optional int64 tt = 5;
*/
public long getTt() {
return tt_;
}
public static final int TMID_FIELD_NUMBER = 6;
private volatile java.lang.Object tmid_;
/**
* optional string tmid = 6;
*/
public boolean hasTmid() {
return ((bitField0_ & 0x00000020) == 0x00000020);
}
/**
* optional string tmid = 6;
*/
public java.lang.String getTmid() {
java.lang.Object ref = tmid_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
tmid_ = s;
}
return s;
}
}
/**
* optional string tmid = 6;
*/
public com.google.protobuf.ByteString
getTmidBytes() {
java.lang.Object ref = tmid_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
tmid_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int MID_FIELD_NUMBER = 7;
private volatile java.lang.Object mid_;
/**
* optional string mid = 7;
*/
public boolean hasMid() {
return ((bitField0_ & 0x00000040) == 0x00000040);
}
/**
* optional string mid = 7;
*/
public java.lang.String getMid() {
java.lang.Object ref = mid_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
mid_ = s;
}
return s;
}
}
/**
* optional string mid = 7;
*/
public com.google.protobuf.ByteString
getMidBytes() {
java.lang.Object ref = mid_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
mid_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int CHECKSUM_FIELD_NUMBER = 8;
private volatile java.lang.Object checksum_;
/**
* optional string checksum = 8;
*/
public boolean hasChecksum() {
return ((bitField0_ & 0x00000080) == 0x00000080);
}
/**
* optional string checksum = 8;
*/
public java.lang.String getChecksum() {
java.lang.Object ref = checksum_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
checksum_ = s;
}
return s;
}
}
/**
* optional string checksum = 8;
*/
public com.google.protobuf.ByteString
getChecksumBytes() {
java.lang.Object ref = checksum_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
checksum_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int STORED_FIELD_NUMBER = 9;
private boolean stored_;
/**
* optional bool stored = 9;
*/
public boolean hasStored() {
return ((bitField0_ & 0x00000100) == 0x00000100);
}
/**
* optional bool stored = 9;
*/
public boolean getStored() {
return stored_;
}
public static final int DIRECTION_FIELD_NUMBER = 10;
private int direction_;
/**
* optional .com.avos.avoscloud.LogsCommand.QueryDirection direction = 10 [default = OLD];
*/
public boolean hasDirection() {
return ((bitField0_ & 0x00000200) == 0x00000200);
}
/**
* optional .com.avos.avoscloud.LogsCommand.QueryDirection direction = 10 [default = OLD];
*/
public com.avos.avoscloud.Messages.LogsCommand.QueryDirection getDirection() {
com.avos.avoscloud.Messages.LogsCommand.QueryDirection result = com.avos.avoscloud.Messages.LogsCommand.QueryDirection.valueOf(direction_);
return result == null ? com.avos.avoscloud.Messages.LogsCommand.QueryDirection.OLD : result;
}
public static final int TINCLUDED_FIELD_NUMBER = 11;
private boolean tIncluded_;
/**
* optional bool tIncluded = 11;
*/
public boolean hasTIncluded() {
return ((bitField0_ & 0x00000400) == 0x00000400);
}
/**
* optional bool tIncluded = 11;
*/
public boolean getTIncluded() {
return tIncluded_;
}
public static final int TTINCLUDED_FIELD_NUMBER = 12;
private boolean ttIncluded_;
/**
* optional bool ttIncluded = 12;
*/
public boolean hasTtIncluded() {
return ((bitField0_ & 0x00000800) == 0x00000800);
}
/**
* optional bool ttIncluded = 12;
*/
public boolean getTtIncluded() {
return ttIncluded_;
}
public static final int LCTYPE_FIELD_NUMBER = 13;
private int lctype_;
/**
* optional int32 lctype = 13;
*/
public boolean hasLctype() {
return ((bitField0_ & 0x00001000) == 0x00001000);
}
/**
* optional int32 lctype = 13;
*/
public int getLctype() {
return lctype_;
}
public static final int LOGS_FIELD_NUMBER = 105;
private java.util.List logs_;
/**
* repeated .com.avos.avoscloud.LogItem logs = 105;
*/
public java.util.List getLogsList() {
return logs_;
}
/**
* repeated .com.avos.avoscloud.LogItem logs = 105;
*/
public java.util.List extends com.avos.avoscloud.Messages.LogItemOrBuilder>
getLogsOrBuilderList() {
return logs_;
}
/**
* repeated .com.avos.avoscloud.LogItem logs = 105;
*/
public int getLogsCount() {
return logs_.size();
}
/**
* repeated .com.avos.avoscloud.LogItem logs = 105;
*/
public com.avos.avoscloud.Messages.LogItem getLogs(int index) {
return logs_.get(index);
}
/**
* repeated .com.avos.avoscloud.LogItem logs = 105;
*/
public com.avos.avoscloud.Messages.LogItemOrBuilder getLogsOrBuilder(
int index) {
return logs_.get(index);
}
private byte memoizedIsInitialized = -1;
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (((bitField0_ & 0x00000001) == 0x00000001)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 1, cid_);
}
if (((bitField0_ & 0x00000002) == 0x00000002)) {
output.writeInt32(2, l_);
}
if (((bitField0_ & 0x00000004) == 0x00000004)) {
output.writeInt32(3, limit_);
}
if (((bitField0_ & 0x00000008) == 0x00000008)) {
output.writeInt64(4, t_);
}
if (((bitField0_ & 0x00000010) == 0x00000010)) {
output.writeInt64(5, tt_);
}
if (((bitField0_ & 0x00000020) == 0x00000020)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 6, tmid_);
}
if (((bitField0_ & 0x00000040) == 0x00000040)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 7, mid_);
}
if (((bitField0_ & 0x00000080) == 0x00000080)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 8, checksum_);
}
if (((bitField0_ & 0x00000100) == 0x00000100)) {
output.writeBool(9, stored_);
}
if (((bitField0_ & 0x00000200) == 0x00000200)) {
output.writeEnum(10, direction_);
}
if (((bitField0_ & 0x00000400) == 0x00000400)) {
output.writeBool(11, tIncluded_);
}
if (((bitField0_ & 0x00000800) == 0x00000800)) {
output.writeBool(12, ttIncluded_);
}
if (((bitField0_ & 0x00001000) == 0x00001000)) {
output.writeInt32(13, lctype_);
}
for (int i = 0; i < logs_.size(); i++) {
output.writeMessage(105, logs_.get(i));
}
unknownFields.writeTo(output);
}
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (((bitField0_ & 0x00000001) == 0x00000001)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(1, cid_);
}
if (((bitField0_ & 0x00000002) == 0x00000002)) {
size += com.google.protobuf.CodedOutputStream
.computeInt32Size(2, l_);
}
if (((bitField0_ & 0x00000004) == 0x00000004)) {
size += com.google.protobuf.CodedOutputStream
.computeInt32Size(3, limit_);
}
if (((bitField0_ & 0x00000008) == 0x00000008)) {
size += com.google.protobuf.CodedOutputStream
.computeInt64Size(4, t_);
}
if (((bitField0_ & 0x00000010) == 0x00000010)) {
size += com.google.protobuf.CodedOutputStream
.computeInt64Size(5, tt_);
}
if (((bitField0_ & 0x00000020) == 0x00000020)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(6, tmid_);
}
if (((bitField0_ & 0x00000040) == 0x00000040)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(7, mid_);
}
if (((bitField0_ & 0x00000080) == 0x00000080)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(8, checksum_);
}
if (((bitField0_ & 0x00000100) == 0x00000100)) {
size += com.google.protobuf.CodedOutputStream
.computeBoolSize(9, stored_);
}
if (((bitField0_ & 0x00000200) == 0x00000200)) {
size += com.google.protobuf.CodedOutputStream
.computeEnumSize(10, direction_);
}
if (((bitField0_ & 0x00000400) == 0x00000400)) {
size += com.google.protobuf.CodedOutputStream
.computeBoolSize(11, tIncluded_);
}
if (((bitField0_ & 0x00000800) == 0x00000800)) {
size += com.google.protobuf.CodedOutputStream
.computeBoolSize(12, ttIncluded_);
}
if (((bitField0_ & 0x00001000) == 0x00001000)) {
size += com.google.protobuf.CodedOutputStream
.computeInt32Size(13, lctype_);
}
for (int i = 0; i < logs_.size(); i++) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(105, logs_.get(i));
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof com.avos.avoscloud.Messages.LogsCommand)) {
return super.equals(obj);
}
com.avos.avoscloud.Messages.LogsCommand other = (com.avos.avoscloud.Messages.LogsCommand) obj;
boolean result = true;
result = result && (hasCid() == other.hasCid());
if (hasCid()) {
result = result && getCid()
.equals(other.getCid());
}
result = result && (hasL() == other.hasL());
if (hasL()) {
result = result && (getL()
== other.getL());
}
result = result && (hasLimit() == other.hasLimit());
if (hasLimit()) {
result = result && (getLimit()
== other.getLimit());
}
result = result && (hasT() == other.hasT());
if (hasT()) {
result = result && (getT()
== other.getT());
}
result = result && (hasTt() == other.hasTt());
if (hasTt()) {
result = result && (getTt()
== other.getTt());
}
result = result && (hasTmid() == other.hasTmid());
if (hasTmid()) {
result = result && getTmid()
.equals(other.getTmid());
}
result = result && (hasMid() == other.hasMid());
if (hasMid()) {
result = result && getMid()
.equals(other.getMid());
}
result = result && (hasChecksum() == other.hasChecksum());
if (hasChecksum()) {
result = result && getChecksum()
.equals(other.getChecksum());
}
result = result && (hasStored() == other.hasStored());
if (hasStored()) {
result = result && (getStored()
== other.getStored());
}
result = result && (hasDirection() == other.hasDirection());
if (hasDirection()) {
result = result && direction_ == other.direction_;
}
result = result && (hasTIncluded() == other.hasTIncluded());
if (hasTIncluded()) {
result = result && (getTIncluded()
== other.getTIncluded());
}
result = result && (hasTtIncluded() == other.hasTtIncluded());
if (hasTtIncluded()) {
result = result && (getTtIncluded()
== other.getTtIncluded());
}
result = result && (hasLctype() == other.hasLctype());
if (hasLctype()) {
result = result && (getLctype()
== other.getLctype());
}
result = result && getLogsList()
.equals(other.getLogsList());
result = result && unknownFields.equals(other.unknownFields);
return result;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (hasCid()) {
hash = (37 * hash) + CID_FIELD_NUMBER;
hash = (53 * hash) + getCid().hashCode();
}
if (hasL()) {
hash = (37 * hash) + L_FIELD_NUMBER;
hash = (53 * hash) + getL();
}
if (hasLimit()) {
hash = (37 * hash) + LIMIT_FIELD_NUMBER;
hash = (53 * hash) + getLimit();
}
if (hasT()) {
hash = (37 * hash) + T_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getT());
}
if (hasTt()) {
hash = (37 * hash) + TT_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getTt());
}
if (hasTmid()) {
hash = (37 * hash) + TMID_FIELD_NUMBER;
hash = (53 * hash) + getTmid().hashCode();
}
if (hasMid()) {
hash = (37 * hash) + MID_FIELD_NUMBER;
hash = (53 * hash) + getMid().hashCode();
}
if (hasChecksum()) {
hash = (37 * hash) + CHECKSUM_FIELD_NUMBER;
hash = (53 * hash) + getChecksum().hashCode();
}
if (hasStored()) {
hash = (37 * hash) + STORED_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashBoolean(
getStored());
}
if (hasDirection()) {
hash = (37 * hash) + DIRECTION_FIELD_NUMBER;
hash = (53 * hash) + direction_;
}
if (hasTIncluded()) {
hash = (37 * hash) + TINCLUDED_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashBoolean(
getTIncluded());
}
if (hasTtIncluded()) {
hash = (37 * hash) + TTINCLUDED_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashBoolean(
getTtIncluded());
}
if (hasLctype()) {
hash = (37 * hash) + LCTYPE_FIELD_NUMBER;
hash = (53 * hash) + getLctype();
}
if (getLogsCount() > 0) {
hash = (37 * hash) + LOGS_FIELD_NUMBER;
hash = (53 * hash) + getLogsList().hashCode();
}
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static com.avos.avoscloud.Messages.LogsCommand parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.avos.avoscloud.Messages.LogsCommand parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.avos.avoscloud.Messages.LogsCommand parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.avos.avoscloud.Messages.LogsCommand parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.avos.avoscloud.Messages.LogsCommand parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.avos.avoscloud.Messages.LogsCommand parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.avos.avoscloud.Messages.LogsCommand parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.avos.avoscloud.Messages.LogsCommand parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static com.avos.avoscloud.Messages.LogsCommand parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static com.avos.avoscloud.Messages.LogsCommand parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static com.avos.avoscloud.Messages.LogsCommand parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.avos.avoscloud.Messages.LogsCommand parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(com.avos.avoscloud.Messages.LogsCommand prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code com.avos.avoscloud.LogsCommand}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:com.avos.avoscloud.LogsCommand)
com.avos.avoscloud.Messages.LogsCommandOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.avos.avoscloud.Messages.internal_static_com_avos_avoscloud_LogsCommand_descriptor;
}
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.avos.avoscloud.Messages.internal_static_com_avos_avoscloud_LogsCommand_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.avos.avoscloud.Messages.LogsCommand.class, com.avos.avoscloud.Messages.LogsCommand.Builder.class);
}
// Construct using com.avos.avoscloud.Messages.LogsCommand.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
getLogsFieldBuilder();
}
}
public Builder clear() {
super.clear();
cid_ = "";
bitField0_ = (bitField0_ & ~0x00000001);
l_ = 0;
bitField0_ = (bitField0_ & ~0x00000002);
limit_ = 0;
bitField0_ = (bitField0_ & ~0x00000004);
t_ = 0L;
bitField0_ = (bitField0_ & ~0x00000008);
tt_ = 0L;
bitField0_ = (bitField0_ & ~0x00000010);
tmid_ = "";
bitField0_ = (bitField0_ & ~0x00000020);
mid_ = "";
bitField0_ = (bitField0_ & ~0x00000040);
checksum_ = "";
bitField0_ = (bitField0_ & ~0x00000080);
stored_ = false;
bitField0_ = (bitField0_ & ~0x00000100);
direction_ = 1;
bitField0_ = (bitField0_ & ~0x00000200);
tIncluded_ = false;
bitField0_ = (bitField0_ & ~0x00000400);
ttIncluded_ = false;
bitField0_ = (bitField0_ & ~0x00000800);
lctype_ = 0;
bitField0_ = (bitField0_ & ~0x00001000);
if (logsBuilder_ == null) {
logs_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00002000);
} else {
logsBuilder_.clear();
}
return this;
}
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return com.avos.avoscloud.Messages.internal_static_com_avos_avoscloud_LogsCommand_descriptor;
}
public com.avos.avoscloud.Messages.LogsCommand getDefaultInstanceForType() {
return com.avos.avoscloud.Messages.LogsCommand.getDefaultInstance();
}
public com.avos.avoscloud.Messages.LogsCommand build() {
com.avos.avoscloud.Messages.LogsCommand result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
public com.avos.avoscloud.Messages.LogsCommand buildPartial() {
com.avos.avoscloud.Messages.LogsCommand result = new com.avos.avoscloud.Messages.LogsCommand(this);
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (((from_bitField0_ & 0x00000001) == 0x00000001)) {
to_bitField0_ |= 0x00000001;
}
result.cid_ = cid_;
if (((from_bitField0_ & 0x00000002) == 0x00000002)) {
to_bitField0_ |= 0x00000002;
}
result.l_ = l_;
if (((from_bitField0_ & 0x00000004) == 0x00000004)) {
to_bitField0_ |= 0x00000004;
}
result.limit_ = limit_;
if (((from_bitField0_ & 0x00000008) == 0x00000008)) {
to_bitField0_ |= 0x00000008;
}
result.t_ = t_;
if (((from_bitField0_ & 0x00000010) == 0x00000010)) {
to_bitField0_ |= 0x00000010;
}
result.tt_ = tt_;
if (((from_bitField0_ & 0x00000020) == 0x00000020)) {
to_bitField0_ |= 0x00000020;
}
result.tmid_ = tmid_;
if (((from_bitField0_ & 0x00000040) == 0x00000040)) {
to_bitField0_ |= 0x00000040;
}
result.mid_ = mid_;
if (((from_bitField0_ & 0x00000080) == 0x00000080)) {
to_bitField0_ |= 0x00000080;
}
result.checksum_ = checksum_;
if (((from_bitField0_ & 0x00000100) == 0x00000100)) {
to_bitField0_ |= 0x00000100;
}
result.stored_ = stored_;
if (((from_bitField0_ & 0x00000200) == 0x00000200)) {
to_bitField0_ |= 0x00000200;
}
result.direction_ = direction_;
if (((from_bitField0_ & 0x00000400) == 0x00000400)) {
to_bitField0_ |= 0x00000400;
}
result.tIncluded_ = tIncluded_;
if (((from_bitField0_ & 0x00000800) == 0x00000800)) {
to_bitField0_ |= 0x00000800;
}
result.ttIncluded_ = ttIncluded_;
if (((from_bitField0_ & 0x00001000) == 0x00001000)) {
to_bitField0_ |= 0x00001000;
}
result.lctype_ = lctype_;
if (logsBuilder_ == null) {
if (((bitField0_ & 0x00002000) == 0x00002000)) {
logs_ = java.util.Collections.unmodifiableList(logs_);
bitField0_ = (bitField0_ & ~0x00002000);
}
result.logs_ = logs_;
} else {
result.logs_ = logsBuilder_.build();
}
result.bitField0_ = to_bitField0_;
onBuilt();
return result;
}
public Builder clone() {
return (Builder) super.clone();
}
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.setField(field, value);
}
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return (Builder) super.clearField(field);
}
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return (Builder) super.clearOneof(oneof);
}
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return (Builder) super.setRepeatedField(field, index, value);
}
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.addRepeatedField(field, value);
}
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.avos.avoscloud.Messages.LogsCommand) {
return mergeFrom((com.avos.avoscloud.Messages.LogsCommand)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.avos.avoscloud.Messages.LogsCommand other) {
if (other == com.avos.avoscloud.Messages.LogsCommand.getDefaultInstance()) return this;
if (other.hasCid()) {
bitField0_ |= 0x00000001;
cid_ = other.cid_;
onChanged();
}
if (other.hasL()) {
setL(other.getL());
}
if (other.hasLimit()) {
setLimit(other.getLimit());
}
if (other.hasT()) {
setT(other.getT());
}
if (other.hasTt()) {
setTt(other.getTt());
}
if (other.hasTmid()) {
bitField0_ |= 0x00000020;
tmid_ = other.tmid_;
onChanged();
}
if (other.hasMid()) {
bitField0_ |= 0x00000040;
mid_ = other.mid_;
onChanged();
}
if (other.hasChecksum()) {
bitField0_ |= 0x00000080;
checksum_ = other.checksum_;
onChanged();
}
if (other.hasStored()) {
setStored(other.getStored());
}
if (other.hasDirection()) {
setDirection(other.getDirection());
}
if (other.hasTIncluded()) {
setTIncluded(other.getTIncluded());
}
if (other.hasTtIncluded()) {
setTtIncluded(other.getTtIncluded());
}
if (other.hasLctype()) {
setLctype(other.getLctype());
}
if (logsBuilder_ == null) {
if (!other.logs_.isEmpty()) {
if (logs_.isEmpty()) {
logs_ = other.logs_;
bitField0_ = (bitField0_ & ~0x00002000);
} else {
ensureLogsIsMutable();
logs_.addAll(other.logs_);
}
onChanged();
}
} else {
if (!other.logs_.isEmpty()) {
if (logsBuilder_.isEmpty()) {
logsBuilder_.dispose();
logsBuilder_ = null;
logs_ = other.logs_;
bitField0_ = (bitField0_ & ~0x00002000);
logsBuilder_ =
com.google.protobuf.GeneratedMessageV3.alwaysUseFieldBuilders ?
getLogsFieldBuilder() : null;
} else {
logsBuilder_.addAllMessages(other.logs_);
}
}
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
public final boolean isInitialized() {
return true;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
com.avos.avoscloud.Messages.LogsCommand parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (com.avos.avoscloud.Messages.LogsCommand) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
private java.lang.Object cid_ = "";
/**
* optional string cid = 1;
*/
public boolean hasCid() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
* optional string cid = 1;
*/
public java.lang.String getCid() {
java.lang.Object ref = cid_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
cid_ = s;
}
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* optional string cid = 1;
*/
public com.google.protobuf.ByteString
getCidBytes() {
java.lang.Object ref = cid_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
cid_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* optional string cid = 1;
*/
public Builder setCid(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000001;
cid_ = value;
onChanged();
return this;
}
/**
* optional string cid = 1;
*/
public Builder clearCid() {
bitField0_ = (bitField0_ & ~0x00000001);
cid_ = getDefaultInstance().getCid();
onChanged();
return this;
}
/**
* optional string cid = 1;
*/
public Builder setCidBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000001;
cid_ = value;
onChanged();
return this;
}
private int l_ ;
/**
* optional int32 l = 2;
*/
public boolean hasL() {
return ((bitField0_ & 0x00000002) == 0x00000002);
}
/**
* optional int32 l = 2;
*/
public int getL() {
return l_;
}
/**
* optional int32 l = 2;
*/
public Builder setL(int value) {
bitField0_ |= 0x00000002;
l_ = value;
onChanged();
return this;
}
/**
* optional int32 l = 2;
*/
public Builder clearL() {
bitField0_ = (bitField0_ & ~0x00000002);
l_ = 0;
onChanged();
return this;
}
private int limit_ ;
/**
* optional int32 limit = 3;
*/
public boolean hasLimit() {
return ((bitField0_ & 0x00000004) == 0x00000004);
}
/**
* optional int32 limit = 3;
*/
public int getLimit() {
return limit_;
}
/**
* optional int32 limit = 3;
*/
public Builder setLimit(int value) {
bitField0_ |= 0x00000004;
limit_ = value;
onChanged();
return this;
}
/**
* optional int32 limit = 3;
*/
public Builder clearLimit() {
bitField0_ = (bitField0_ & ~0x00000004);
limit_ = 0;
onChanged();
return this;
}
private long t_ ;
/**
* optional int64 t = 4;
*/
public boolean hasT() {
return ((bitField0_ & 0x00000008) == 0x00000008);
}
/**
* optional int64 t = 4;
*/
public long getT() {
return t_;
}
/**
* optional int64 t = 4;
*/
public Builder setT(long value) {
bitField0_ |= 0x00000008;
t_ = value;
onChanged();
return this;
}
/**
* optional int64 t = 4;
*/
public Builder clearT() {
bitField0_ = (bitField0_ & ~0x00000008);
t_ = 0L;
onChanged();
return this;
}
private long tt_ ;
/**
* optional int64 tt = 5;
*/
public boolean hasTt() {
return ((bitField0_ & 0x00000010) == 0x00000010);
}
/**
* optional int64 tt = 5;
*/
public long getTt() {
return tt_;
}
/**
* optional int64 tt = 5;
*/
public Builder setTt(long value) {
bitField0_ |= 0x00000010;
tt_ = value;
onChanged();
return this;
}
/**
* optional int64 tt = 5;
*/
public Builder clearTt() {
bitField0_ = (bitField0_ & ~0x00000010);
tt_ = 0L;
onChanged();
return this;
}
private java.lang.Object tmid_ = "";
/**
* optional string tmid = 6;
*/
public boolean hasTmid() {
return ((bitField0_ & 0x00000020) == 0x00000020);
}
/**
* optional string tmid = 6;
*/
public java.lang.String getTmid() {
java.lang.Object ref = tmid_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
tmid_ = s;
}
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* optional string tmid = 6;
*/
public com.google.protobuf.ByteString
getTmidBytes() {
java.lang.Object ref = tmid_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
tmid_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* optional string tmid = 6;
*/
public Builder setTmid(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000020;
tmid_ = value;
onChanged();
return this;
}
/**
* optional string tmid = 6;
*/
public Builder clearTmid() {
bitField0_ = (bitField0_ & ~0x00000020);
tmid_ = getDefaultInstance().getTmid();
onChanged();
return this;
}
/**
* optional string tmid = 6;
*/
public Builder setTmidBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000020;
tmid_ = value;
onChanged();
return this;
}
private java.lang.Object mid_ = "";
/**
* optional string mid = 7;
*/
public boolean hasMid() {
return ((bitField0_ & 0x00000040) == 0x00000040);
}
/**
* optional string mid = 7;
*/
public java.lang.String getMid() {
java.lang.Object ref = mid_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
mid_ = s;
}
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* optional string mid = 7;
*/
public com.google.protobuf.ByteString
getMidBytes() {
java.lang.Object ref = mid_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
mid_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* optional string mid = 7;
*/
public Builder setMid(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000040;
mid_ = value;
onChanged();
return this;
}
/**
* optional string mid = 7;
*/
public Builder clearMid() {
bitField0_ = (bitField0_ & ~0x00000040);
mid_ = getDefaultInstance().getMid();
onChanged();
return this;
}
/**
* optional string mid = 7;
*/
public Builder setMidBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000040;
mid_ = value;
onChanged();
return this;
}
private java.lang.Object checksum_ = "";
/**
* optional string checksum = 8;
*/
public boolean hasChecksum() {
return ((bitField0_ & 0x00000080) == 0x00000080);
}
/**
* optional string checksum = 8;
*/
public java.lang.String getChecksum() {
java.lang.Object ref = checksum_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
checksum_ = s;
}
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* optional string checksum = 8;
*/
public com.google.protobuf.ByteString
getChecksumBytes() {
java.lang.Object ref = checksum_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
checksum_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* optional string checksum = 8;
*/
public Builder setChecksum(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000080;
checksum_ = value;
onChanged();
return this;
}
/**
* optional string checksum = 8;
*/
public Builder clearChecksum() {
bitField0_ = (bitField0_ & ~0x00000080);
checksum_ = getDefaultInstance().getChecksum();
onChanged();
return this;
}
/**
* optional string checksum = 8;
*/
public Builder setChecksumBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000080;
checksum_ = value;
onChanged();
return this;
}
private boolean stored_ ;
/**
* optional bool stored = 9;
*/
public boolean hasStored() {
return ((bitField0_ & 0x00000100) == 0x00000100);
}
/**
* optional bool stored = 9;
*/
public boolean getStored() {
return stored_;
}
/**
* optional bool stored = 9;
*/
public Builder setStored(boolean value) {
bitField0_ |= 0x00000100;
stored_ = value;
onChanged();
return this;
}
/**
* optional bool stored = 9;
*/
public Builder clearStored() {
bitField0_ = (bitField0_ & ~0x00000100);
stored_ = false;
onChanged();
return this;
}
private int direction_ = 1;
/**
* optional .com.avos.avoscloud.LogsCommand.QueryDirection direction = 10 [default = OLD];
*/
public boolean hasDirection() {
return ((bitField0_ & 0x00000200) == 0x00000200);
}
/**
* optional .com.avos.avoscloud.LogsCommand.QueryDirection direction = 10 [default = OLD];
*/
public com.avos.avoscloud.Messages.LogsCommand.QueryDirection getDirection() {
com.avos.avoscloud.Messages.LogsCommand.QueryDirection result = com.avos.avoscloud.Messages.LogsCommand.QueryDirection.valueOf(direction_);
return result == null ? com.avos.avoscloud.Messages.LogsCommand.QueryDirection.OLD : result;
}
/**
* optional .com.avos.avoscloud.LogsCommand.QueryDirection direction = 10 [default = OLD];
*/
public Builder setDirection(com.avos.avoscloud.Messages.LogsCommand.QueryDirection value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000200;
direction_ = value.getNumber();
onChanged();
return this;
}
/**
* optional .com.avos.avoscloud.LogsCommand.QueryDirection direction = 10 [default = OLD];
*/
public Builder clearDirection() {
bitField0_ = (bitField0_ & ~0x00000200);
direction_ = 1;
onChanged();
return this;
}
private boolean tIncluded_ ;
/**
* optional bool tIncluded = 11;
*/
public boolean hasTIncluded() {
return ((bitField0_ & 0x00000400) == 0x00000400);
}
/**
* optional bool tIncluded = 11;
*/
public boolean getTIncluded() {
return tIncluded_;
}
/**
* optional bool tIncluded = 11;
*/
public Builder setTIncluded(boolean value) {
bitField0_ |= 0x00000400;
tIncluded_ = value;
onChanged();
return this;
}
/**
* optional bool tIncluded = 11;
*/
public Builder clearTIncluded() {
bitField0_ = (bitField0_ & ~0x00000400);
tIncluded_ = false;
onChanged();
return this;
}
private boolean ttIncluded_ ;
/**
* optional bool ttIncluded = 12;
*/
public boolean hasTtIncluded() {
return ((bitField0_ & 0x00000800) == 0x00000800);
}
/**
* optional bool ttIncluded = 12;
*/
public boolean getTtIncluded() {
return ttIncluded_;
}
/**
* optional bool ttIncluded = 12;
*/
public Builder setTtIncluded(boolean value) {
bitField0_ |= 0x00000800;
ttIncluded_ = value;
onChanged();
return this;
}
/**
* optional bool ttIncluded = 12;
*/
public Builder clearTtIncluded() {
bitField0_ = (bitField0_ & ~0x00000800);
ttIncluded_ = false;
onChanged();
return this;
}
private int lctype_ ;
/**
* optional int32 lctype = 13;
*/
public boolean hasLctype() {
return ((bitField0_ & 0x00001000) == 0x00001000);
}
/**
* optional int32 lctype = 13;
*/
public int getLctype() {
return lctype_;
}
/**
* optional int32 lctype = 13;
*/
public Builder setLctype(int value) {
bitField0_ |= 0x00001000;
lctype_ = value;
onChanged();
return this;
}
/**
* optional int32 lctype = 13;
*/
public Builder clearLctype() {
bitField0_ = (bitField0_ & ~0x00001000);
lctype_ = 0;
onChanged();
return this;
}
private java.util.List logs_ =
java.util.Collections.emptyList();
private void ensureLogsIsMutable() {
if (!((bitField0_ & 0x00002000) == 0x00002000)) {
logs_ = new java.util.ArrayList(logs_);
bitField0_ |= 0x00002000;
}
}
private com.google.protobuf.RepeatedFieldBuilderV3<
com.avos.avoscloud.Messages.LogItem, com.avos.avoscloud.Messages.LogItem.Builder, com.avos.avoscloud.Messages.LogItemOrBuilder> logsBuilder_;
/**
* repeated .com.avos.avoscloud.LogItem logs = 105;
*/
public java.util.List getLogsList() {
if (logsBuilder_ == null) {
return java.util.Collections.unmodifiableList(logs_);
} else {
return logsBuilder_.getMessageList();
}
}
/**
* repeated .com.avos.avoscloud.LogItem logs = 105;
*/
public int getLogsCount() {
if (logsBuilder_ == null) {
return logs_.size();
} else {
return logsBuilder_.getCount();
}
}
/**
* repeated .com.avos.avoscloud.LogItem logs = 105;
*/
public com.avos.avoscloud.Messages.LogItem getLogs(int index) {
if (logsBuilder_ == null) {
return logs_.get(index);
} else {
return logsBuilder_.getMessage(index);
}
}
/**
* repeated .com.avos.avoscloud.LogItem logs = 105;
*/
public Builder setLogs(
int index, com.avos.avoscloud.Messages.LogItem value) {
if (logsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureLogsIsMutable();
logs_.set(index, value);
onChanged();
} else {
logsBuilder_.setMessage(index, value);
}
return this;
}
/**
* repeated .com.avos.avoscloud.LogItem logs = 105;
*/
public Builder setLogs(
int index, com.avos.avoscloud.Messages.LogItem.Builder builderForValue) {
if (logsBuilder_ == null) {
ensureLogsIsMutable();
logs_.set(index, builderForValue.build());
onChanged();
} else {
logsBuilder_.setMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .com.avos.avoscloud.LogItem logs = 105;
*/
public Builder addLogs(com.avos.avoscloud.Messages.LogItem value) {
if (logsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureLogsIsMutable();
logs_.add(value);
onChanged();
} else {
logsBuilder_.addMessage(value);
}
return this;
}
/**
* repeated .com.avos.avoscloud.LogItem logs = 105;
*/
public Builder addLogs(
int index, com.avos.avoscloud.Messages.LogItem value) {
if (logsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureLogsIsMutable();
logs_.add(index, value);
onChanged();
} else {
logsBuilder_.addMessage(index, value);
}
return this;
}
/**
* repeated .com.avos.avoscloud.LogItem logs = 105;
*/
public Builder addLogs(
com.avos.avoscloud.Messages.LogItem.Builder builderForValue) {
if (logsBuilder_ == null) {
ensureLogsIsMutable();
logs_.add(builderForValue.build());
onChanged();
} else {
logsBuilder_.addMessage(builderForValue.build());
}
return this;
}
/**
* repeated .com.avos.avoscloud.LogItem logs = 105;
*/
public Builder addLogs(
int index, com.avos.avoscloud.Messages.LogItem.Builder builderForValue) {
if (logsBuilder_ == null) {
ensureLogsIsMutable();
logs_.add(index, builderForValue.build());
onChanged();
} else {
logsBuilder_.addMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .com.avos.avoscloud.LogItem logs = 105;
*/
public Builder addAllLogs(
java.lang.Iterable extends com.avos.avoscloud.Messages.LogItem> values) {
if (logsBuilder_ == null) {
ensureLogsIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, logs_);
onChanged();
} else {
logsBuilder_.addAllMessages(values);
}
return this;
}
/**
* repeated .com.avos.avoscloud.LogItem logs = 105;
*/
public Builder clearLogs() {
if (logsBuilder_ == null) {
logs_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00002000);
onChanged();
} else {
logsBuilder_.clear();
}
return this;
}
/**
* repeated .com.avos.avoscloud.LogItem logs = 105;
*/
public Builder removeLogs(int index) {
if (logsBuilder_ == null) {
ensureLogsIsMutable();
logs_.remove(index);
onChanged();
} else {
logsBuilder_.remove(index);
}
return this;
}
/**
* repeated .com.avos.avoscloud.LogItem logs = 105;
*/
public com.avos.avoscloud.Messages.LogItem.Builder getLogsBuilder(
int index) {
return getLogsFieldBuilder().getBuilder(index);
}
/**
* repeated .com.avos.avoscloud.LogItem logs = 105;
*/
public com.avos.avoscloud.Messages.LogItemOrBuilder getLogsOrBuilder(
int index) {
if (logsBuilder_ == null) {
return logs_.get(index); } else {
return logsBuilder_.getMessageOrBuilder(index);
}
}
/**
* repeated .com.avos.avoscloud.LogItem logs = 105;
*/
public java.util.List extends com.avos.avoscloud.Messages.LogItemOrBuilder>
getLogsOrBuilderList() {
if (logsBuilder_ != null) {
return logsBuilder_.getMessageOrBuilderList();
} else {
return java.util.Collections.unmodifiableList(logs_);
}
}
/**
* repeated .com.avos.avoscloud.LogItem logs = 105;
*/
public com.avos.avoscloud.Messages.LogItem.Builder addLogsBuilder() {
return getLogsFieldBuilder().addBuilder(
com.avos.avoscloud.Messages.LogItem.getDefaultInstance());
}
/**
* repeated .com.avos.avoscloud.LogItem logs = 105;
*/
public com.avos.avoscloud.Messages.LogItem.Builder addLogsBuilder(
int index) {
return getLogsFieldBuilder().addBuilder(
index, com.avos.avoscloud.Messages.LogItem.getDefaultInstance());
}
/**
* repeated .com.avos.avoscloud.LogItem logs = 105;
*/
public java.util.List
getLogsBuilderList() {
return getLogsFieldBuilder().getBuilderList();
}
private com.google.protobuf.RepeatedFieldBuilderV3<
com.avos.avoscloud.Messages.LogItem, com.avos.avoscloud.Messages.LogItem.Builder, com.avos.avoscloud.Messages.LogItemOrBuilder>
getLogsFieldBuilder() {
if (logsBuilder_ == null) {
logsBuilder_ = new com.google.protobuf.RepeatedFieldBuilderV3<
com.avos.avoscloud.Messages.LogItem, com.avos.avoscloud.Messages.LogItem.Builder, com.avos.avoscloud.Messages.LogItemOrBuilder>(
logs_,
((bitField0_ & 0x00002000) == 0x00002000),
getParentForChildren(),
isClean());
logs_ = null;
}
return logsBuilder_;
}
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:com.avos.avoscloud.LogsCommand)
}
// @@protoc_insertion_point(class_scope:com.avos.avoscloud.LogsCommand)
private static final com.avos.avoscloud.Messages.LogsCommand DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new com.avos.avoscloud.Messages.LogsCommand();
}
public static com.avos.avoscloud.Messages.LogsCommand getDefaultInstance() {
return DEFAULT_INSTANCE;
}
@java.lang.Deprecated public static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
public LogsCommand parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new LogsCommand(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
public com.avos.avoscloud.Messages.LogsCommand getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface RcpCommandOrBuilder extends
// @@protoc_insertion_point(interface_extends:com.avos.avoscloud.RcpCommand)
com.google.protobuf.MessageOrBuilder {
/**
* optional string id = 1;
*/
boolean hasId();
/**
* optional string id = 1;
*/
java.lang.String getId();
/**
* optional string id = 1;
*/
com.google.protobuf.ByteString
getIdBytes();
/**
* optional string cid = 2;
*/
boolean hasCid();
/**
* optional string cid = 2;
*/
java.lang.String getCid();
/**
* optional string cid = 2;
*/
com.google.protobuf.ByteString
getCidBytes();
/**
* optional int64 t = 3;
*/
boolean hasT();
/**
* optional int64 t = 3;
*/
long getT();
/**
* optional bool read = 4;
*/
boolean hasRead();
/**
* optional bool read = 4;
*/
boolean getRead();
/**
* optional string from = 5;
*/
boolean hasFrom();
/**
* optional string from = 5;
*/
java.lang.String getFrom();
/**
* optional string from = 5;
*/
com.google.protobuf.ByteString
getFromBytes();
}
/**
* Protobuf type {@code com.avos.avoscloud.RcpCommand}
*/
public static final class RcpCommand extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:com.avos.avoscloud.RcpCommand)
RcpCommandOrBuilder {
private static final long serialVersionUID = 0L;
// Use RcpCommand.newBuilder() to construct.
private RcpCommand(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private RcpCommand() {
id_ = "";
cid_ = "";
t_ = 0L;
read_ = false;
from_ = "";
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private RcpCommand(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!parseUnknownField(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
case 10: {
com.google.protobuf.ByteString bs = input.readBytes();
bitField0_ |= 0x00000001;
id_ = bs;
break;
}
case 18: {
com.google.protobuf.ByteString bs = input.readBytes();
bitField0_ |= 0x00000002;
cid_ = bs;
break;
}
case 24: {
bitField0_ |= 0x00000004;
t_ = input.readInt64();
break;
}
case 32: {
bitField0_ |= 0x00000008;
read_ = input.readBool();
break;
}
case 42: {
com.google.protobuf.ByteString bs = input.readBytes();
bitField0_ |= 0x00000010;
from_ = bs;
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.avos.avoscloud.Messages.internal_static_com_avos_avoscloud_RcpCommand_descriptor;
}
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.avos.avoscloud.Messages.internal_static_com_avos_avoscloud_RcpCommand_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.avos.avoscloud.Messages.RcpCommand.class, com.avos.avoscloud.Messages.RcpCommand.Builder.class);
}
private int bitField0_;
public static final int ID_FIELD_NUMBER = 1;
private volatile java.lang.Object id_;
/**
* optional string id = 1;
*/
public boolean hasId() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
* optional string id = 1;
*/
public java.lang.String getId() {
java.lang.Object ref = id_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
id_ = s;
}
return s;
}
}
/**
* optional string id = 1;
*/
public com.google.protobuf.ByteString
getIdBytes() {
java.lang.Object ref = id_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
id_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int CID_FIELD_NUMBER = 2;
private volatile java.lang.Object cid_;
/**
* optional string cid = 2;
*/
public boolean hasCid() {
return ((bitField0_ & 0x00000002) == 0x00000002);
}
/**
* optional string cid = 2;
*/
public java.lang.String getCid() {
java.lang.Object ref = cid_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
cid_ = s;
}
return s;
}
}
/**
* optional string cid = 2;
*/
public com.google.protobuf.ByteString
getCidBytes() {
java.lang.Object ref = cid_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
cid_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int T_FIELD_NUMBER = 3;
private long t_;
/**
* optional int64 t = 3;
*/
public boolean hasT() {
return ((bitField0_ & 0x00000004) == 0x00000004);
}
/**
* optional int64 t = 3;
*/
public long getT() {
return t_;
}
public static final int READ_FIELD_NUMBER = 4;
private boolean read_;
/**
* optional bool read = 4;
*/
public boolean hasRead() {
return ((bitField0_ & 0x00000008) == 0x00000008);
}
/**
* optional bool read = 4;
*/
public boolean getRead() {
return read_;
}
public static final int FROM_FIELD_NUMBER = 5;
private volatile java.lang.Object from_;
/**
* optional string from = 5;
*/
public boolean hasFrom() {
return ((bitField0_ & 0x00000010) == 0x00000010);
}
/**
* optional string from = 5;
*/
public java.lang.String getFrom() {
java.lang.Object ref = from_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
from_ = s;
}
return s;
}
}
/**
* optional string from = 5;
*/
public com.google.protobuf.ByteString
getFromBytes() {
java.lang.Object ref = from_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
from_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
private byte memoizedIsInitialized = -1;
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (((bitField0_ & 0x00000001) == 0x00000001)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 1, id_);
}
if (((bitField0_ & 0x00000002) == 0x00000002)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 2, cid_);
}
if (((bitField0_ & 0x00000004) == 0x00000004)) {
output.writeInt64(3, t_);
}
if (((bitField0_ & 0x00000008) == 0x00000008)) {
output.writeBool(4, read_);
}
if (((bitField0_ & 0x00000010) == 0x00000010)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 5, from_);
}
unknownFields.writeTo(output);
}
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (((bitField0_ & 0x00000001) == 0x00000001)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(1, id_);
}
if (((bitField0_ & 0x00000002) == 0x00000002)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(2, cid_);
}
if (((bitField0_ & 0x00000004) == 0x00000004)) {
size += com.google.protobuf.CodedOutputStream
.computeInt64Size(3, t_);
}
if (((bitField0_ & 0x00000008) == 0x00000008)) {
size += com.google.protobuf.CodedOutputStream
.computeBoolSize(4, read_);
}
if (((bitField0_ & 0x00000010) == 0x00000010)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(5, from_);
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof com.avos.avoscloud.Messages.RcpCommand)) {
return super.equals(obj);
}
com.avos.avoscloud.Messages.RcpCommand other = (com.avos.avoscloud.Messages.RcpCommand) obj;
boolean result = true;
result = result && (hasId() == other.hasId());
if (hasId()) {
result = result && getId()
.equals(other.getId());
}
result = result && (hasCid() == other.hasCid());
if (hasCid()) {
result = result && getCid()
.equals(other.getCid());
}
result = result && (hasT() == other.hasT());
if (hasT()) {
result = result && (getT()
== other.getT());
}
result = result && (hasRead() == other.hasRead());
if (hasRead()) {
result = result && (getRead()
== other.getRead());
}
result = result && (hasFrom() == other.hasFrom());
if (hasFrom()) {
result = result && getFrom()
.equals(other.getFrom());
}
result = result && unknownFields.equals(other.unknownFields);
return result;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (hasId()) {
hash = (37 * hash) + ID_FIELD_NUMBER;
hash = (53 * hash) + getId().hashCode();
}
if (hasCid()) {
hash = (37 * hash) + CID_FIELD_NUMBER;
hash = (53 * hash) + getCid().hashCode();
}
if (hasT()) {
hash = (37 * hash) + T_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getT());
}
if (hasRead()) {
hash = (37 * hash) + READ_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashBoolean(
getRead());
}
if (hasFrom()) {
hash = (37 * hash) + FROM_FIELD_NUMBER;
hash = (53 * hash) + getFrom().hashCode();
}
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static com.avos.avoscloud.Messages.RcpCommand parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.avos.avoscloud.Messages.RcpCommand parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.avos.avoscloud.Messages.RcpCommand parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.avos.avoscloud.Messages.RcpCommand parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.avos.avoscloud.Messages.RcpCommand parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.avos.avoscloud.Messages.RcpCommand parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.avos.avoscloud.Messages.RcpCommand parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.avos.avoscloud.Messages.RcpCommand parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static com.avos.avoscloud.Messages.RcpCommand parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static com.avos.avoscloud.Messages.RcpCommand parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static com.avos.avoscloud.Messages.RcpCommand parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.avos.avoscloud.Messages.RcpCommand parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(com.avos.avoscloud.Messages.RcpCommand prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code com.avos.avoscloud.RcpCommand}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:com.avos.avoscloud.RcpCommand)
com.avos.avoscloud.Messages.RcpCommandOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.avos.avoscloud.Messages.internal_static_com_avos_avoscloud_RcpCommand_descriptor;
}
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.avos.avoscloud.Messages.internal_static_com_avos_avoscloud_RcpCommand_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.avos.avoscloud.Messages.RcpCommand.class, com.avos.avoscloud.Messages.RcpCommand.Builder.class);
}
// Construct using com.avos.avoscloud.Messages.RcpCommand.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
public Builder clear() {
super.clear();
id_ = "";
bitField0_ = (bitField0_ & ~0x00000001);
cid_ = "";
bitField0_ = (bitField0_ & ~0x00000002);
t_ = 0L;
bitField0_ = (bitField0_ & ~0x00000004);
read_ = false;
bitField0_ = (bitField0_ & ~0x00000008);
from_ = "";
bitField0_ = (bitField0_ & ~0x00000010);
return this;
}
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return com.avos.avoscloud.Messages.internal_static_com_avos_avoscloud_RcpCommand_descriptor;
}
public com.avos.avoscloud.Messages.RcpCommand getDefaultInstanceForType() {
return com.avos.avoscloud.Messages.RcpCommand.getDefaultInstance();
}
public com.avos.avoscloud.Messages.RcpCommand build() {
com.avos.avoscloud.Messages.RcpCommand result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
public com.avos.avoscloud.Messages.RcpCommand buildPartial() {
com.avos.avoscloud.Messages.RcpCommand result = new com.avos.avoscloud.Messages.RcpCommand(this);
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (((from_bitField0_ & 0x00000001) == 0x00000001)) {
to_bitField0_ |= 0x00000001;
}
result.id_ = id_;
if (((from_bitField0_ & 0x00000002) == 0x00000002)) {
to_bitField0_ |= 0x00000002;
}
result.cid_ = cid_;
if (((from_bitField0_ & 0x00000004) == 0x00000004)) {
to_bitField0_ |= 0x00000004;
}
result.t_ = t_;
if (((from_bitField0_ & 0x00000008) == 0x00000008)) {
to_bitField0_ |= 0x00000008;
}
result.read_ = read_;
if (((from_bitField0_ & 0x00000010) == 0x00000010)) {
to_bitField0_ |= 0x00000010;
}
result.from_ = from_;
result.bitField0_ = to_bitField0_;
onBuilt();
return result;
}
public Builder clone() {
return (Builder) super.clone();
}
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.setField(field, value);
}
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return (Builder) super.clearField(field);
}
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return (Builder) super.clearOneof(oneof);
}
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return (Builder) super.setRepeatedField(field, index, value);
}
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.addRepeatedField(field, value);
}
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.avos.avoscloud.Messages.RcpCommand) {
return mergeFrom((com.avos.avoscloud.Messages.RcpCommand)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.avos.avoscloud.Messages.RcpCommand other) {
if (other == com.avos.avoscloud.Messages.RcpCommand.getDefaultInstance()) return this;
if (other.hasId()) {
bitField0_ |= 0x00000001;
id_ = other.id_;
onChanged();
}
if (other.hasCid()) {
bitField0_ |= 0x00000002;
cid_ = other.cid_;
onChanged();
}
if (other.hasT()) {
setT(other.getT());
}
if (other.hasRead()) {
setRead(other.getRead());
}
if (other.hasFrom()) {
bitField0_ |= 0x00000010;
from_ = other.from_;
onChanged();
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
public final boolean isInitialized() {
return true;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
com.avos.avoscloud.Messages.RcpCommand parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (com.avos.avoscloud.Messages.RcpCommand) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
private java.lang.Object id_ = "";
/**
* optional string id = 1;
*/
public boolean hasId() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
* optional string id = 1;
*/
public java.lang.String getId() {
java.lang.Object ref = id_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
id_ = s;
}
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* optional string id = 1;
*/
public com.google.protobuf.ByteString
getIdBytes() {
java.lang.Object ref = id_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
id_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* optional string id = 1;
*/
public Builder setId(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000001;
id_ = value;
onChanged();
return this;
}
/**
* optional string id = 1;
*/
public Builder clearId() {
bitField0_ = (bitField0_ & ~0x00000001);
id_ = getDefaultInstance().getId();
onChanged();
return this;
}
/**
* optional string id = 1;
*/
public Builder setIdBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000001;
id_ = value;
onChanged();
return this;
}
private java.lang.Object cid_ = "";
/**
* optional string cid = 2;
*/
public boolean hasCid() {
return ((bitField0_ & 0x00000002) == 0x00000002);
}
/**
* optional string cid = 2;
*/
public java.lang.String getCid() {
java.lang.Object ref = cid_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
cid_ = s;
}
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* optional string cid = 2;
*/
public com.google.protobuf.ByteString
getCidBytes() {
java.lang.Object ref = cid_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
cid_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* optional string cid = 2;
*/
public Builder setCid(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000002;
cid_ = value;
onChanged();
return this;
}
/**
* optional string cid = 2;
*/
public Builder clearCid() {
bitField0_ = (bitField0_ & ~0x00000002);
cid_ = getDefaultInstance().getCid();
onChanged();
return this;
}
/**
* optional string cid = 2;
*/
public Builder setCidBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000002;
cid_ = value;
onChanged();
return this;
}
private long t_ ;
/**
* optional int64 t = 3;
*/
public boolean hasT() {
return ((bitField0_ & 0x00000004) == 0x00000004);
}
/**
* optional int64 t = 3;
*/
public long getT() {
return t_;
}
/**
* optional int64 t = 3;
*/
public Builder setT(long value) {
bitField0_ |= 0x00000004;
t_ = value;
onChanged();
return this;
}
/**
* optional int64 t = 3;
*/
public Builder clearT() {
bitField0_ = (bitField0_ & ~0x00000004);
t_ = 0L;
onChanged();
return this;
}
private boolean read_ ;
/**
* optional bool read = 4;
*/
public boolean hasRead() {
return ((bitField0_ & 0x00000008) == 0x00000008);
}
/**
* optional bool read = 4;
*/
public boolean getRead() {
return read_;
}
/**
* optional bool read = 4;
*/
public Builder setRead(boolean value) {
bitField0_ |= 0x00000008;
read_ = value;
onChanged();
return this;
}
/**
* optional bool read = 4;
*/
public Builder clearRead() {
bitField0_ = (bitField0_ & ~0x00000008);
read_ = false;
onChanged();
return this;
}
private java.lang.Object from_ = "";
/**
* optional string from = 5;
*/
public boolean hasFrom() {
return ((bitField0_ & 0x00000010) == 0x00000010);
}
/**
* optional string from = 5;
*/
public java.lang.String getFrom() {
java.lang.Object ref = from_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
from_ = s;
}
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* optional string from = 5;
*/
public com.google.protobuf.ByteString
getFromBytes() {
java.lang.Object ref = from_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
from_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* optional string from = 5;
*/
public Builder setFrom(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000010;
from_ = value;
onChanged();
return this;
}
/**
* optional string from = 5;
*/
public Builder clearFrom() {
bitField0_ = (bitField0_ & ~0x00000010);
from_ = getDefaultInstance().getFrom();
onChanged();
return this;
}
/**
* optional string from = 5;
*/
public Builder setFromBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000010;
from_ = value;
onChanged();
return this;
}
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:com.avos.avoscloud.RcpCommand)
}
// @@protoc_insertion_point(class_scope:com.avos.avoscloud.RcpCommand)
private static final com.avos.avoscloud.Messages.RcpCommand DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new com.avos.avoscloud.Messages.RcpCommand();
}
public static com.avos.avoscloud.Messages.RcpCommand getDefaultInstance() {
return DEFAULT_INSTANCE;
}
@java.lang.Deprecated public static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
public RcpCommand parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new RcpCommand(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
public com.avos.avoscloud.Messages.RcpCommand getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface ReadTupleOrBuilder extends
// @@protoc_insertion_point(interface_extends:com.avos.avoscloud.ReadTuple)
com.google.protobuf.MessageOrBuilder {
/**
* required string cid = 1;
*/
boolean hasCid();
/**
* required string cid = 1;
*/
java.lang.String getCid();
/**
* required string cid = 1;
*/
com.google.protobuf.ByteString
getCidBytes();
/**
* optional int64 timestamp = 2;
*/
boolean hasTimestamp();
/**
* optional int64 timestamp = 2;
*/
long getTimestamp();
/**
* optional string mid = 3;
*/
boolean hasMid();
/**
* optional string mid = 3;
*/
java.lang.String getMid();
/**
* optional string mid = 3;
*/
com.google.protobuf.ByteString
getMidBytes();
}
/**
* Protobuf type {@code com.avos.avoscloud.ReadTuple}
*/
public static final class ReadTuple extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:com.avos.avoscloud.ReadTuple)
ReadTupleOrBuilder {
private static final long serialVersionUID = 0L;
// Use ReadTuple.newBuilder() to construct.
private ReadTuple(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private ReadTuple() {
cid_ = "";
timestamp_ = 0L;
mid_ = "";
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private ReadTuple(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!parseUnknownField(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
case 10: {
com.google.protobuf.ByteString bs = input.readBytes();
bitField0_ |= 0x00000001;
cid_ = bs;
break;
}
case 16: {
bitField0_ |= 0x00000002;
timestamp_ = input.readInt64();
break;
}
case 26: {
com.google.protobuf.ByteString bs = input.readBytes();
bitField0_ |= 0x00000004;
mid_ = bs;
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.avos.avoscloud.Messages.internal_static_com_avos_avoscloud_ReadTuple_descriptor;
}
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.avos.avoscloud.Messages.internal_static_com_avos_avoscloud_ReadTuple_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.avos.avoscloud.Messages.ReadTuple.class, com.avos.avoscloud.Messages.ReadTuple.Builder.class);
}
private int bitField0_;
public static final int CID_FIELD_NUMBER = 1;
private volatile java.lang.Object cid_;
/**
* required string cid = 1;
*/
public boolean hasCid() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
* required string cid = 1;
*/
public java.lang.String getCid() {
java.lang.Object ref = cid_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
cid_ = s;
}
return s;
}
}
/**
* required string cid = 1;
*/
public com.google.protobuf.ByteString
getCidBytes() {
java.lang.Object ref = cid_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
cid_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int TIMESTAMP_FIELD_NUMBER = 2;
private long timestamp_;
/**
* optional int64 timestamp = 2;
*/
public boolean hasTimestamp() {
return ((bitField0_ & 0x00000002) == 0x00000002);
}
/**
* optional int64 timestamp = 2;
*/
public long getTimestamp() {
return timestamp_;
}
public static final int MID_FIELD_NUMBER = 3;
private volatile java.lang.Object mid_;
/**
* optional string mid = 3;
*/
public boolean hasMid() {
return ((bitField0_ & 0x00000004) == 0x00000004);
}
/**
* optional string mid = 3;
*/
public java.lang.String getMid() {
java.lang.Object ref = mid_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
mid_ = s;
}
return s;
}
}
/**
* optional string mid = 3;
*/
public com.google.protobuf.ByteString
getMidBytes() {
java.lang.Object ref = mid_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
mid_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
private byte memoizedIsInitialized = -1;
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
if (!hasCid()) {
memoizedIsInitialized = 0;
return false;
}
memoizedIsInitialized = 1;
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (((bitField0_ & 0x00000001) == 0x00000001)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 1, cid_);
}
if (((bitField0_ & 0x00000002) == 0x00000002)) {
output.writeInt64(2, timestamp_);
}
if (((bitField0_ & 0x00000004) == 0x00000004)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 3, mid_);
}
unknownFields.writeTo(output);
}
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (((bitField0_ & 0x00000001) == 0x00000001)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(1, cid_);
}
if (((bitField0_ & 0x00000002) == 0x00000002)) {
size += com.google.protobuf.CodedOutputStream
.computeInt64Size(2, timestamp_);
}
if (((bitField0_ & 0x00000004) == 0x00000004)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(3, mid_);
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof com.avos.avoscloud.Messages.ReadTuple)) {
return super.equals(obj);
}
com.avos.avoscloud.Messages.ReadTuple other = (com.avos.avoscloud.Messages.ReadTuple) obj;
boolean result = true;
result = result && (hasCid() == other.hasCid());
if (hasCid()) {
result = result && getCid()
.equals(other.getCid());
}
result = result && (hasTimestamp() == other.hasTimestamp());
if (hasTimestamp()) {
result = result && (getTimestamp()
== other.getTimestamp());
}
result = result && (hasMid() == other.hasMid());
if (hasMid()) {
result = result && getMid()
.equals(other.getMid());
}
result = result && unknownFields.equals(other.unknownFields);
return result;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (hasCid()) {
hash = (37 * hash) + CID_FIELD_NUMBER;
hash = (53 * hash) + getCid().hashCode();
}
if (hasTimestamp()) {
hash = (37 * hash) + TIMESTAMP_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getTimestamp());
}
if (hasMid()) {
hash = (37 * hash) + MID_FIELD_NUMBER;
hash = (53 * hash) + getMid().hashCode();
}
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static com.avos.avoscloud.Messages.ReadTuple parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.avos.avoscloud.Messages.ReadTuple parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.avos.avoscloud.Messages.ReadTuple parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.avos.avoscloud.Messages.ReadTuple parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.avos.avoscloud.Messages.ReadTuple parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.avos.avoscloud.Messages.ReadTuple parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.avos.avoscloud.Messages.ReadTuple parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.avos.avoscloud.Messages.ReadTuple parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static com.avos.avoscloud.Messages.ReadTuple parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static com.avos.avoscloud.Messages.ReadTuple parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static com.avos.avoscloud.Messages.ReadTuple parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.avos.avoscloud.Messages.ReadTuple parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(com.avos.avoscloud.Messages.ReadTuple prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code com.avos.avoscloud.ReadTuple}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:com.avos.avoscloud.ReadTuple)
com.avos.avoscloud.Messages.ReadTupleOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.avos.avoscloud.Messages.internal_static_com_avos_avoscloud_ReadTuple_descriptor;
}
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.avos.avoscloud.Messages.internal_static_com_avos_avoscloud_ReadTuple_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.avos.avoscloud.Messages.ReadTuple.class, com.avos.avoscloud.Messages.ReadTuple.Builder.class);
}
// Construct using com.avos.avoscloud.Messages.ReadTuple.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
public Builder clear() {
super.clear();
cid_ = "";
bitField0_ = (bitField0_ & ~0x00000001);
timestamp_ = 0L;
bitField0_ = (bitField0_ & ~0x00000002);
mid_ = "";
bitField0_ = (bitField0_ & ~0x00000004);
return this;
}
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return com.avos.avoscloud.Messages.internal_static_com_avos_avoscloud_ReadTuple_descriptor;
}
public com.avos.avoscloud.Messages.ReadTuple getDefaultInstanceForType() {
return com.avos.avoscloud.Messages.ReadTuple.getDefaultInstance();
}
public com.avos.avoscloud.Messages.ReadTuple build() {
com.avos.avoscloud.Messages.ReadTuple result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
public com.avos.avoscloud.Messages.ReadTuple buildPartial() {
com.avos.avoscloud.Messages.ReadTuple result = new com.avos.avoscloud.Messages.ReadTuple(this);
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (((from_bitField0_ & 0x00000001) == 0x00000001)) {
to_bitField0_ |= 0x00000001;
}
result.cid_ = cid_;
if (((from_bitField0_ & 0x00000002) == 0x00000002)) {
to_bitField0_ |= 0x00000002;
}
result.timestamp_ = timestamp_;
if (((from_bitField0_ & 0x00000004) == 0x00000004)) {
to_bitField0_ |= 0x00000004;
}
result.mid_ = mid_;
result.bitField0_ = to_bitField0_;
onBuilt();
return result;
}
public Builder clone() {
return (Builder) super.clone();
}
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.setField(field, value);
}
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return (Builder) super.clearField(field);
}
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return (Builder) super.clearOneof(oneof);
}
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return (Builder) super.setRepeatedField(field, index, value);
}
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.addRepeatedField(field, value);
}
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.avos.avoscloud.Messages.ReadTuple) {
return mergeFrom((com.avos.avoscloud.Messages.ReadTuple)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.avos.avoscloud.Messages.ReadTuple other) {
if (other == com.avos.avoscloud.Messages.ReadTuple.getDefaultInstance()) return this;
if (other.hasCid()) {
bitField0_ |= 0x00000001;
cid_ = other.cid_;
onChanged();
}
if (other.hasTimestamp()) {
setTimestamp(other.getTimestamp());
}
if (other.hasMid()) {
bitField0_ |= 0x00000004;
mid_ = other.mid_;
onChanged();
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
public final boolean isInitialized() {
if (!hasCid()) {
return false;
}
return true;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
com.avos.avoscloud.Messages.ReadTuple parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (com.avos.avoscloud.Messages.ReadTuple) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
private java.lang.Object cid_ = "";
/**
* required string cid = 1;
*/
public boolean hasCid() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
* required string cid = 1;
*/
public java.lang.String getCid() {
java.lang.Object ref = cid_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
cid_ = s;
}
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* required string cid = 1;
*/
public com.google.protobuf.ByteString
getCidBytes() {
java.lang.Object ref = cid_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
cid_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* required string cid = 1;
*/
public Builder setCid(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000001;
cid_ = value;
onChanged();
return this;
}
/**
* required string cid = 1;
*/
public Builder clearCid() {
bitField0_ = (bitField0_ & ~0x00000001);
cid_ = getDefaultInstance().getCid();
onChanged();
return this;
}
/**
* required string cid = 1;
*/
public Builder setCidBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000001;
cid_ = value;
onChanged();
return this;
}
private long timestamp_ ;
/**
* optional int64 timestamp = 2;
*/
public boolean hasTimestamp() {
return ((bitField0_ & 0x00000002) == 0x00000002);
}
/**
* optional int64 timestamp = 2;
*/
public long getTimestamp() {
return timestamp_;
}
/**
* optional int64 timestamp = 2;
*/
public Builder setTimestamp(long value) {
bitField0_ |= 0x00000002;
timestamp_ = value;
onChanged();
return this;
}
/**
* optional int64 timestamp = 2;
*/
public Builder clearTimestamp() {
bitField0_ = (bitField0_ & ~0x00000002);
timestamp_ = 0L;
onChanged();
return this;
}
private java.lang.Object mid_ = "";
/**
* optional string mid = 3;
*/
public boolean hasMid() {
return ((bitField0_ & 0x00000004) == 0x00000004);
}
/**
* optional string mid = 3;
*/
public java.lang.String getMid() {
java.lang.Object ref = mid_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
mid_ = s;
}
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* optional string mid = 3;
*/
public com.google.protobuf.ByteString
getMidBytes() {
java.lang.Object ref = mid_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
mid_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* optional string mid = 3;
*/
public Builder setMid(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000004;
mid_ = value;
onChanged();
return this;
}
/**
* optional string mid = 3;
*/
public Builder clearMid() {
bitField0_ = (bitField0_ & ~0x00000004);
mid_ = getDefaultInstance().getMid();
onChanged();
return this;
}
/**
* optional string mid = 3;
*/
public Builder setMidBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000004;
mid_ = value;
onChanged();
return this;
}
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:com.avos.avoscloud.ReadTuple)
}
// @@protoc_insertion_point(class_scope:com.avos.avoscloud.ReadTuple)
private static final com.avos.avoscloud.Messages.ReadTuple DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new com.avos.avoscloud.Messages.ReadTuple();
}
public static com.avos.avoscloud.Messages.ReadTuple getDefaultInstance() {
return DEFAULT_INSTANCE;
}
@java.lang.Deprecated public static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
public ReadTuple parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new ReadTuple(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
public com.avos.avoscloud.Messages.ReadTuple getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface MaxReadTupleOrBuilder extends
// @@protoc_insertion_point(interface_extends:com.avos.avoscloud.MaxReadTuple)
com.google.protobuf.MessageOrBuilder {
/**
* optional string pid = 1;
*/
boolean hasPid();
/**
* optional string pid = 1;
*/
java.lang.String getPid();
/**
* optional string pid = 1;
*/
com.google.protobuf.ByteString
getPidBytes();
/**
* optional int64 maxAckTimestamp = 2;
*/
boolean hasMaxAckTimestamp();
/**
* optional int64 maxAckTimestamp = 2;
*/
long getMaxAckTimestamp();
/**
* optional int64 maxReadTimestamp = 3;
*/
boolean hasMaxReadTimestamp();
/**
* optional int64 maxReadTimestamp = 3;
*/
long getMaxReadTimestamp();
}
/**
* Protobuf type {@code com.avos.avoscloud.MaxReadTuple}
*/
public static final class MaxReadTuple extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:com.avos.avoscloud.MaxReadTuple)
MaxReadTupleOrBuilder {
private static final long serialVersionUID = 0L;
// Use MaxReadTuple.newBuilder() to construct.
private MaxReadTuple(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private MaxReadTuple() {
pid_ = "";
maxAckTimestamp_ = 0L;
maxReadTimestamp_ = 0L;
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private MaxReadTuple(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!parseUnknownField(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
case 10: {
com.google.protobuf.ByteString bs = input.readBytes();
bitField0_ |= 0x00000001;
pid_ = bs;
break;
}
case 16: {
bitField0_ |= 0x00000002;
maxAckTimestamp_ = input.readInt64();
break;
}
case 24: {
bitField0_ |= 0x00000004;
maxReadTimestamp_ = input.readInt64();
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.avos.avoscloud.Messages.internal_static_com_avos_avoscloud_MaxReadTuple_descriptor;
}
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.avos.avoscloud.Messages.internal_static_com_avos_avoscloud_MaxReadTuple_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.avos.avoscloud.Messages.MaxReadTuple.class, com.avos.avoscloud.Messages.MaxReadTuple.Builder.class);
}
private int bitField0_;
public static final int PID_FIELD_NUMBER = 1;
private volatile java.lang.Object pid_;
/**
* optional string pid = 1;
*/
public boolean hasPid() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
* optional string pid = 1;
*/
public java.lang.String getPid() {
java.lang.Object ref = pid_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
pid_ = s;
}
return s;
}
}
/**
* optional string pid = 1;
*/
public com.google.protobuf.ByteString
getPidBytes() {
java.lang.Object ref = pid_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
pid_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int MAXACKTIMESTAMP_FIELD_NUMBER = 2;
private long maxAckTimestamp_;
/**
* optional int64 maxAckTimestamp = 2;
*/
public boolean hasMaxAckTimestamp() {
return ((bitField0_ & 0x00000002) == 0x00000002);
}
/**
* optional int64 maxAckTimestamp = 2;
*/
public long getMaxAckTimestamp() {
return maxAckTimestamp_;
}
public static final int MAXREADTIMESTAMP_FIELD_NUMBER = 3;
private long maxReadTimestamp_;
/**
* optional int64 maxReadTimestamp = 3;
*/
public boolean hasMaxReadTimestamp() {
return ((bitField0_ & 0x00000004) == 0x00000004);
}
/**
* optional int64 maxReadTimestamp = 3;
*/
public long getMaxReadTimestamp() {
return maxReadTimestamp_;
}
private byte memoizedIsInitialized = -1;
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (((bitField0_ & 0x00000001) == 0x00000001)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 1, pid_);
}
if (((bitField0_ & 0x00000002) == 0x00000002)) {
output.writeInt64(2, maxAckTimestamp_);
}
if (((bitField0_ & 0x00000004) == 0x00000004)) {
output.writeInt64(3, maxReadTimestamp_);
}
unknownFields.writeTo(output);
}
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (((bitField0_ & 0x00000001) == 0x00000001)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(1, pid_);
}
if (((bitField0_ & 0x00000002) == 0x00000002)) {
size += com.google.protobuf.CodedOutputStream
.computeInt64Size(2, maxAckTimestamp_);
}
if (((bitField0_ & 0x00000004) == 0x00000004)) {
size += com.google.protobuf.CodedOutputStream
.computeInt64Size(3, maxReadTimestamp_);
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof com.avos.avoscloud.Messages.MaxReadTuple)) {
return super.equals(obj);
}
com.avos.avoscloud.Messages.MaxReadTuple other = (com.avos.avoscloud.Messages.MaxReadTuple) obj;
boolean result = true;
result = result && (hasPid() == other.hasPid());
if (hasPid()) {
result = result && getPid()
.equals(other.getPid());
}
result = result && (hasMaxAckTimestamp() == other.hasMaxAckTimestamp());
if (hasMaxAckTimestamp()) {
result = result && (getMaxAckTimestamp()
== other.getMaxAckTimestamp());
}
result = result && (hasMaxReadTimestamp() == other.hasMaxReadTimestamp());
if (hasMaxReadTimestamp()) {
result = result && (getMaxReadTimestamp()
== other.getMaxReadTimestamp());
}
result = result && unknownFields.equals(other.unknownFields);
return result;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (hasPid()) {
hash = (37 * hash) + PID_FIELD_NUMBER;
hash = (53 * hash) + getPid().hashCode();
}
if (hasMaxAckTimestamp()) {
hash = (37 * hash) + MAXACKTIMESTAMP_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getMaxAckTimestamp());
}
if (hasMaxReadTimestamp()) {
hash = (37 * hash) + MAXREADTIMESTAMP_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getMaxReadTimestamp());
}
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static com.avos.avoscloud.Messages.MaxReadTuple parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.avos.avoscloud.Messages.MaxReadTuple parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.avos.avoscloud.Messages.MaxReadTuple parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.avos.avoscloud.Messages.MaxReadTuple parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.avos.avoscloud.Messages.MaxReadTuple parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.avos.avoscloud.Messages.MaxReadTuple parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.avos.avoscloud.Messages.MaxReadTuple parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.avos.avoscloud.Messages.MaxReadTuple parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static com.avos.avoscloud.Messages.MaxReadTuple parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static com.avos.avoscloud.Messages.MaxReadTuple parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static com.avos.avoscloud.Messages.MaxReadTuple parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.avos.avoscloud.Messages.MaxReadTuple parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(com.avos.avoscloud.Messages.MaxReadTuple prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code com.avos.avoscloud.MaxReadTuple}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:com.avos.avoscloud.MaxReadTuple)
com.avos.avoscloud.Messages.MaxReadTupleOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.avos.avoscloud.Messages.internal_static_com_avos_avoscloud_MaxReadTuple_descriptor;
}
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.avos.avoscloud.Messages.internal_static_com_avos_avoscloud_MaxReadTuple_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.avos.avoscloud.Messages.MaxReadTuple.class, com.avos.avoscloud.Messages.MaxReadTuple.Builder.class);
}
// Construct using com.avos.avoscloud.Messages.MaxReadTuple.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
public Builder clear() {
super.clear();
pid_ = "";
bitField0_ = (bitField0_ & ~0x00000001);
maxAckTimestamp_ = 0L;
bitField0_ = (bitField0_ & ~0x00000002);
maxReadTimestamp_ = 0L;
bitField0_ = (bitField0_ & ~0x00000004);
return this;
}
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return com.avos.avoscloud.Messages.internal_static_com_avos_avoscloud_MaxReadTuple_descriptor;
}
public com.avos.avoscloud.Messages.MaxReadTuple getDefaultInstanceForType() {
return com.avos.avoscloud.Messages.MaxReadTuple.getDefaultInstance();
}
public com.avos.avoscloud.Messages.MaxReadTuple build() {
com.avos.avoscloud.Messages.MaxReadTuple result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
public com.avos.avoscloud.Messages.MaxReadTuple buildPartial() {
com.avos.avoscloud.Messages.MaxReadTuple result = new com.avos.avoscloud.Messages.MaxReadTuple(this);
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (((from_bitField0_ & 0x00000001) == 0x00000001)) {
to_bitField0_ |= 0x00000001;
}
result.pid_ = pid_;
if (((from_bitField0_ & 0x00000002) == 0x00000002)) {
to_bitField0_ |= 0x00000002;
}
result.maxAckTimestamp_ = maxAckTimestamp_;
if (((from_bitField0_ & 0x00000004) == 0x00000004)) {
to_bitField0_ |= 0x00000004;
}
result.maxReadTimestamp_ = maxReadTimestamp_;
result.bitField0_ = to_bitField0_;
onBuilt();
return result;
}
public Builder clone() {
return (Builder) super.clone();
}
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.setField(field, value);
}
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return (Builder) super.clearField(field);
}
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return (Builder) super.clearOneof(oneof);
}
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return (Builder) super.setRepeatedField(field, index, value);
}
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.addRepeatedField(field, value);
}
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.avos.avoscloud.Messages.MaxReadTuple) {
return mergeFrom((com.avos.avoscloud.Messages.MaxReadTuple)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.avos.avoscloud.Messages.MaxReadTuple other) {
if (other == com.avos.avoscloud.Messages.MaxReadTuple.getDefaultInstance()) return this;
if (other.hasPid()) {
bitField0_ |= 0x00000001;
pid_ = other.pid_;
onChanged();
}
if (other.hasMaxAckTimestamp()) {
setMaxAckTimestamp(other.getMaxAckTimestamp());
}
if (other.hasMaxReadTimestamp()) {
setMaxReadTimestamp(other.getMaxReadTimestamp());
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
public final boolean isInitialized() {
return true;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
com.avos.avoscloud.Messages.MaxReadTuple parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (com.avos.avoscloud.Messages.MaxReadTuple) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
private java.lang.Object pid_ = "";
/**
* optional string pid = 1;
*/
public boolean hasPid() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
* optional string pid = 1;
*/
public java.lang.String getPid() {
java.lang.Object ref = pid_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
pid_ = s;
}
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* optional string pid = 1;
*/
public com.google.protobuf.ByteString
getPidBytes() {
java.lang.Object ref = pid_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
pid_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* optional string pid = 1;
*/
public Builder setPid(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000001;
pid_ = value;
onChanged();
return this;
}
/**
* optional string pid = 1;
*/
public Builder clearPid() {
bitField0_ = (bitField0_ & ~0x00000001);
pid_ = getDefaultInstance().getPid();
onChanged();
return this;
}
/**
* optional string pid = 1;
*/
public Builder setPidBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000001;
pid_ = value;
onChanged();
return this;
}
private long maxAckTimestamp_ ;
/**
* optional int64 maxAckTimestamp = 2;
*/
public boolean hasMaxAckTimestamp() {
return ((bitField0_ & 0x00000002) == 0x00000002);
}
/**
* optional int64 maxAckTimestamp = 2;
*/
public long getMaxAckTimestamp() {
return maxAckTimestamp_;
}
/**
* optional int64 maxAckTimestamp = 2;
*/
public Builder setMaxAckTimestamp(long value) {
bitField0_ |= 0x00000002;
maxAckTimestamp_ = value;
onChanged();
return this;
}
/**
* optional int64 maxAckTimestamp = 2;
*/
public Builder clearMaxAckTimestamp() {
bitField0_ = (bitField0_ & ~0x00000002);
maxAckTimestamp_ = 0L;
onChanged();
return this;
}
private long maxReadTimestamp_ ;
/**
* optional int64 maxReadTimestamp = 3;
*/
public boolean hasMaxReadTimestamp() {
return ((bitField0_ & 0x00000004) == 0x00000004);
}
/**
* optional int64 maxReadTimestamp = 3;
*/
public long getMaxReadTimestamp() {
return maxReadTimestamp_;
}
/**
* optional int64 maxReadTimestamp = 3;
*/
public Builder setMaxReadTimestamp(long value) {
bitField0_ |= 0x00000004;
maxReadTimestamp_ = value;
onChanged();
return this;
}
/**
* optional int64 maxReadTimestamp = 3;
*/
public Builder clearMaxReadTimestamp() {
bitField0_ = (bitField0_ & ~0x00000004);
maxReadTimestamp_ = 0L;
onChanged();
return this;
}
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:com.avos.avoscloud.MaxReadTuple)
}
// @@protoc_insertion_point(class_scope:com.avos.avoscloud.MaxReadTuple)
private static final com.avos.avoscloud.Messages.MaxReadTuple DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new com.avos.avoscloud.Messages.MaxReadTuple();
}
public static com.avos.avoscloud.Messages.MaxReadTuple getDefaultInstance() {
return DEFAULT_INSTANCE;
}
@java.lang.Deprecated public static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
public MaxReadTuple parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new MaxReadTuple(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
public com.avos.avoscloud.Messages.MaxReadTuple getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface ReadCommandOrBuilder extends
// @@protoc_insertion_point(interface_extends:com.avos.avoscloud.ReadCommand)
com.google.protobuf.MessageOrBuilder {
/**
* optional string cid = 1;
*/
boolean hasCid();
/**
* optional string cid = 1;
*/
java.lang.String getCid();
/**
* optional string cid = 1;
*/
com.google.protobuf.ByteString
getCidBytes();
/**
* repeated string cids = 2;
*/
java.util.List
getCidsList();
/**
* repeated string cids = 2;
*/
int getCidsCount();
/**
* repeated string cids = 2;
*/
java.lang.String getCids(int index);
/**
* repeated string cids = 2;
*/
com.google.protobuf.ByteString
getCidsBytes(int index);
/**
* repeated .com.avos.avoscloud.ReadTuple convs = 3;
*/
java.util.List
getConvsList();
/**
* repeated .com.avos.avoscloud.ReadTuple convs = 3;
*/
com.avos.avoscloud.Messages.ReadTuple getConvs(int index);
/**
* repeated .com.avos.avoscloud.ReadTuple convs = 3;
*/
int getConvsCount();
/**
* repeated .com.avos.avoscloud.ReadTuple convs = 3;
*/
java.util.List extends com.avos.avoscloud.Messages.ReadTupleOrBuilder>
getConvsOrBuilderList();
/**
* repeated .com.avos.avoscloud.ReadTuple convs = 3;
*/
com.avos.avoscloud.Messages.ReadTupleOrBuilder getConvsOrBuilder(
int index);
}
/**
* Protobuf type {@code com.avos.avoscloud.ReadCommand}
*/
public static final class ReadCommand extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:com.avos.avoscloud.ReadCommand)
ReadCommandOrBuilder {
private static final long serialVersionUID = 0L;
// Use ReadCommand.newBuilder() to construct.
private ReadCommand(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private ReadCommand() {
cid_ = "";
cids_ = com.google.protobuf.LazyStringArrayList.EMPTY;
convs_ = java.util.Collections.emptyList();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private ReadCommand(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!parseUnknownField(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
case 10: {
com.google.protobuf.ByteString bs = input.readBytes();
bitField0_ |= 0x00000001;
cid_ = bs;
break;
}
case 18: {
com.google.protobuf.ByteString bs = input.readBytes();
if (!((mutable_bitField0_ & 0x00000002) == 0x00000002)) {
cids_ = new com.google.protobuf.LazyStringArrayList();
mutable_bitField0_ |= 0x00000002;
}
cids_.add(bs);
break;
}
case 26: {
if (!((mutable_bitField0_ & 0x00000004) == 0x00000004)) {
convs_ = new java.util.ArrayList();
mutable_bitField0_ |= 0x00000004;
}
convs_.add(
input.readMessage(com.avos.avoscloud.Messages.ReadTuple.PARSER, extensionRegistry));
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
if (((mutable_bitField0_ & 0x00000002) == 0x00000002)) {
cids_ = cids_.getUnmodifiableView();
}
if (((mutable_bitField0_ & 0x00000004) == 0x00000004)) {
convs_ = java.util.Collections.unmodifiableList(convs_);
}
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.avos.avoscloud.Messages.internal_static_com_avos_avoscloud_ReadCommand_descriptor;
}
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.avos.avoscloud.Messages.internal_static_com_avos_avoscloud_ReadCommand_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.avos.avoscloud.Messages.ReadCommand.class, com.avos.avoscloud.Messages.ReadCommand.Builder.class);
}
private int bitField0_;
public static final int CID_FIELD_NUMBER = 1;
private volatile java.lang.Object cid_;
/**
* optional string cid = 1;
*/
public boolean hasCid() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
* optional string cid = 1;
*/
public java.lang.String getCid() {
java.lang.Object ref = cid_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
cid_ = s;
}
return s;
}
}
/**
* optional string cid = 1;
*/
public com.google.protobuf.ByteString
getCidBytes() {
java.lang.Object ref = cid_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
cid_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int CIDS_FIELD_NUMBER = 2;
private com.google.protobuf.LazyStringList cids_;
/**
* repeated string cids = 2;
*/
public com.google.protobuf.ProtocolStringList
getCidsList() {
return cids_;
}
/**
* repeated string cids = 2;
*/
public int getCidsCount() {
return cids_.size();
}
/**
* repeated string cids = 2;
*/
public java.lang.String getCids(int index) {
return cids_.get(index);
}
/**
* repeated string cids = 2;
*/
public com.google.protobuf.ByteString
getCidsBytes(int index) {
return cids_.getByteString(index);
}
public static final int CONVS_FIELD_NUMBER = 3;
private java.util.List convs_;
/**
* repeated .com.avos.avoscloud.ReadTuple convs = 3;
*/
public java.util.List getConvsList() {
return convs_;
}
/**
* repeated .com.avos.avoscloud.ReadTuple convs = 3;
*/
public java.util.List extends com.avos.avoscloud.Messages.ReadTupleOrBuilder>
getConvsOrBuilderList() {
return convs_;
}
/**
* repeated .com.avos.avoscloud.ReadTuple convs = 3;
*/
public int getConvsCount() {
return convs_.size();
}
/**
* repeated .com.avos.avoscloud.ReadTuple convs = 3;
*/
public com.avos.avoscloud.Messages.ReadTuple getConvs(int index) {
return convs_.get(index);
}
/**
* repeated .com.avos.avoscloud.ReadTuple convs = 3;
*/
public com.avos.avoscloud.Messages.ReadTupleOrBuilder getConvsOrBuilder(
int index) {
return convs_.get(index);
}
private byte memoizedIsInitialized = -1;
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
for (int i = 0; i < getConvsCount(); i++) {
if (!getConvs(i).isInitialized()) {
memoizedIsInitialized = 0;
return false;
}
}
memoizedIsInitialized = 1;
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (((bitField0_ & 0x00000001) == 0x00000001)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 1, cid_);
}
for (int i = 0; i < cids_.size(); i++) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 2, cids_.getRaw(i));
}
for (int i = 0; i < convs_.size(); i++) {
output.writeMessage(3, convs_.get(i));
}
unknownFields.writeTo(output);
}
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (((bitField0_ & 0x00000001) == 0x00000001)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(1, cid_);
}
{
int dataSize = 0;
for (int i = 0; i < cids_.size(); i++) {
dataSize += computeStringSizeNoTag(cids_.getRaw(i));
}
size += dataSize;
size += 1 * getCidsList().size();
}
for (int i = 0; i < convs_.size(); i++) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(3, convs_.get(i));
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof com.avos.avoscloud.Messages.ReadCommand)) {
return super.equals(obj);
}
com.avos.avoscloud.Messages.ReadCommand other = (com.avos.avoscloud.Messages.ReadCommand) obj;
boolean result = true;
result = result && (hasCid() == other.hasCid());
if (hasCid()) {
result = result && getCid()
.equals(other.getCid());
}
result = result && getCidsList()
.equals(other.getCidsList());
result = result && getConvsList()
.equals(other.getConvsList());
result = result && unknownFields.equals(other.unknownFields);
return result;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (hasCid()) {
hash = (37 * hash) + CID_FIELD_NUMBER;
hash = (53 * hash) + getCid().hashCode();
}
if (getCidsCount() > 0) {
hash = (37 * hash) + CIDS_FIELD_NUMBER;
hash = (53 * hash) + getCidsList().hashCode();
}
if (getConvsCount() > 0) {
hash = (37 * hash) + CONVS_FIELD_NUMBER;
hash = (53 * hash) + getConvsList().hashCode();
}
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static com.avos.avoscloud.Messages.ReadCommand parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.avos.avoscloud.Messages.ReadCommand parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.avos.avoscloud.Messages.ReadCommand parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.avos.avoscloud.Messages.ReadCommand parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.avos.avoscloud.Messages.ReadCommand parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.avos.avoscloud.Messages.ReadCommand parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.avos.avoscloud.Messages.ReadCommand parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.avos.avoscloud.Messages.ReadCommand parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static com.avos.avoscloud.Messages.ReadCommand parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static com.avos.avoscloud.Messages.ReadCommand parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static com.avos.avoscloud.Messages.ReadCommand parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.avos.avoscloud.Messages.ReadCommand parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(com.avos.avoscloud.Messages.ReadCommand prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code com.avos.avoscloud.ReadCommand}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:com.avos.avoscloud.ReadCommand)
com.avos.avoscloud.Messages.ReadCommandOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.avos.avoscloud.Messages.internal_static_com_avos_avoscloud_ReadCommand_descriptor;
}
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.avos.avoscloud.Messages.internal_static_com_avos_avoscloud_ReadCommand_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.avos.avoscloud.Messages.ReadCommand.class, com.avos.avoscloud.Messages.ReadCommand.Builder.class);
}
// Construct using com.avos.avoscloud.Messages.ReadCommand.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
getConvsFieldBuilder();
}
}
public Builder clear() {
super.clear();
cid_ = "";
bitField0_ = (bitField0_ & ~0x00000001);
cids_ = com.google.protobuf.LazyStringArrayList.EMPTY;
bitField0_ = (bitField0_ & ~0x00000002);
if (convsBuilder_ == null) {
convs_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000004);
} else {
convsBuilder_.clear();
}
return this;
}
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return com.avos.avoscloud.Messages.internal_static_com_avos_avoscloud_ReadCommand_descriptor;
}
public com.avos.avoscloud.Messages.ReadCommand getDefaultInstanceForType() {
return com.avos.avoscloud.Messages.ReadCommand.getDefaultInstance();
}
public com.avos.avoscloud.Messages.ReadCommand build() {
com.avos.avoscloud.Messages.ReadCommand result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
public com.avos.avoscloud.Messages.ReadCommand buildPartial() {
com.avos.avoscloud.Messages.ReadCommand result = new com.avos.avoscloud.Messages.ReadCommand(this);
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (((from_bitField0_ & 0x00000001) == 0x00000001)) {
to_bitField0_ |= 0x00000001;
}
result.cid_ = cid_;
if (((bitField0_ & 0x00000002) == 0x00000002)) {
cids_ = cids_.getUnmodifiableView();
bitField0_ = (bitField0_ & ~0x00000002);
}
result.cids_ = cids_;
if (convsBuilder_ == null) {
if (((bitField0_ & 0x00000004) == 0x00000004)) {
convs_ = java.util.Collections.unmodifiableList(convs_);
bitField0_ = (bitField0_ & ~0x00000004);
}
result.convs_ = convs_;
} else {
result.convs_ = convsBuilder_.build();
}
result.bitField0_ = to_bitField0_;
onBuilt();
return result;
}
public Builder clone() {
return (Builder) super.clone();
}
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.setField(field, value);
}
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return (Builder) super.clearField(field);
}
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return (Builder) super.clearOneof(oneof);
}
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return (Builder) super.setRepeatedField(field, index, value);
}
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.addRepeatedField(field, value);
}
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.avos.avoscloud.Messages.ReadCommand) {
return mergeFrom((com.avos.avoscloud.Messages.ReadCommand)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.avos.avoscloud.Messages.ReadCommand other) {
if (other == com.avos.avoscloud.Messages.ReadCommand.getDefaultInstance()) return this;
if (other.hasCid()) {
bitField0_ |= 0x00000001;
cid_ = other.cid_;
onChanged();
}
if (!other.cids_.isEmpty()) {
if (cids_.isEmpty()) {
cids_ = other.cids_;
bitField0_ = (bitField0_ & ~0x00000002);
} else {
ensureCidsIsMutable();
cids_.addAll(other.cids_);
}
onChanged();
}
if (convsBuilder_ == null) {
if (!other.convs_.isEmpty()) {
if (convs_.isEmpty()) {
convs_ = other.convs_;
bitField0_ = (bitField0_ & ~0x00000004);
} else {
ensureConvsIsMutable();
convs_.addAll(other.convs_);
}
onChanged();
}
} else {
if (!other.convs_.isEmpty()) {
if (convsBuilder_.isEmpty()) {
convsBuilder_.dispose();
convsBuilder_ = null;
convs_ = other.convs_;
bitField0_ = (bitField0_ & ~0x00000004);
convsBuilder_ =
com.google.protobuf.GeneratedMessageV3.alwaysUseFieldBuilders ?
getConvsFieldBuilder() : null;
} else {
convsBuilder_.addAllMessages(other.convs_);
}
}
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
public final boolean isInitialized() {
for (int i = 0; i < getConvsCount(); i++) {
if (!getConvs(i).isInitialized()) {
return false;
}
}
return true;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
com.avos.avoscloud.Messages.ReadCommand parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (com.avos.avoscloud.Messages.ReadCommand) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
private java.lang.Object cid_ = "";
/**
* optional string cid = 1;
*/
public boolean hasCid() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
* optional string cid = 1;
*/
public java.lang.String getCid() {
java.lang.Object ref = cid_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
cid_ = s;
}
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* optional string cid = 1;
*/
public com.google.protobuf.ByteString
getCidBytes() {
java.lang.Object ref = cid_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
cid_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* optional string cid = 1;
*/
public Builder setCid(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000001;
cid_ = value;
onChanged();
return this;
}
/**
* optional string cid = 1;
*/
public Builder clearCid() {
bitField0_ = (bitField0_ & ~0x00000001);
cid_ = getDefaultInstance().getCid();
onChanged();
return this;
}
/**
* optional string cid = 1;
*/
public Builder setCidBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000001;
cid_ = value;
onChanged();
return this;
}
private com.google.protobuf.LazyStringList cids_ = com.google.protobuf.LazyStringArrayList.EMPTY;
private void ensureCidsIsMutable() {
if (!((bitField0_ & 0x00000002) == 0x00000002)) {
cids_ = new com.google.protobuf.LazyStringArrayList(cids_);
bitField0_ |= 0x00000002;
}
}
/**
* repeated string cids = 2;
*/
public com.google.protobuf.ProtocolStringList
getCidsList() {
return cids_.getUnmodifiableView();
}
/**
* repeated string cids = 2;
*/
public int getCidsCount() {
return cids_.size();
}
/**
* repeated string cids = 2;
*/
public java.lang.String getCids(int index) {
return cids_.get(index);
}
/**
* repeated string cids = 2;
*/
public com.google.protobuf.ByteString
getCidsBytes(int index) {
return cids_.getByteString(index);
}
/**
* repeated string cids = 2;
*/
public Builder setCids(
int index, java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
ensureCidsIsMutable();
cids_.set(index, value);
onChanged();
return this;
}
/**
* repeated string cids = 2;
*/
public Builder addCids(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
ensureCidsIsMutable();
cids_.add(value);
onChanged();
return this;
}
/**
* repeated string cids = 2;
*/
public Builder addAllCids(
java.lang.Iterable values) {
ensureCidsIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, cids_);
onChanged();
return this;
}
/**
* repeated string cids = 2;
*/
public Builder clearCids() {
cids_ = com.google.protobuf.LazyStringArrayList.EMPTY;
bitField0_ = (bitField0_ & ~0x00000002);
onChanged();
return this;
}
/**
* repeated string cids = 2;
*/
public Builder addCidsBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
ensureCidsIsMutable();
cids_.add(value);
onChanged();
return this;
}
private java.util.List convs_ =
java.util.Collections.emptyList();
private void ensureConvsIsMutable() {
if (!((bitField0_ & 0x00000004) == 0x00000004)) {
convs_ = new java.util.ArrayList(convs_);
bitField0_ |= 0x00000004;
}
}
private com.google.protobuf.RepeatedFieldBuilderV3<
com.avos.avoscloud.Messages.ReadTuple, com.avos.avoscloud.Messages.ReadTuple.Builder, com.avos.avoscloud.Messages.ReadTupleOrBuilder> convsBuilder_;
/**
* repeated .com.avos.avoscloud.ReadTuple convs = 3;
*/
public java.util.List getConvsList() {
if (convsBuilder_ == null) {
return java.util.Collections.unmodifiableList(convs_);
} else {
return convsBuilder_.getMessageList();
}
}
/**
* repeated .com.avos.avoscloud.ReadTuple convs = 3;
*/
public int getConvsCount() {
if (convsBuilder_ == null) {
return convs_.size();
} else {
return convsBuilder_.getCount();
}
}
/**
* repeated .com.avos.avoscloud.ReadTuple convs = 3;
*/
public com.avos.avoscloud.Messages.ReadTuple getConvs(int index) {
if (convsBuilder_ == null) {
return convs_.get(index);
} else {
return convsBuilder_.getMessage(index);
}
}
/**
* repeated .com.avos.avoscloud.ReadTuple convs = 3;
*/
public Builder setConvs(
int index, com.avos.avoscloud.Messages.ReadTuple value) {
if (convsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureConvsIsMutable();
convs_.set(index, value);
onChanged();
} else {
convsBuilder_.setMessage(index, value);
}
return this;
}
/**
* repeated .com.avos.avoscloud.ReadTuple convs = 3;
*/
public Builder setConvs(
int index, com.avos.avoscloud.Messages.ReadTuple.Builder builderForValue) {
if (convsBuilder_ == null) {
ensureConvsIsMutable();
convs_.set(index, builderForValue.build());
onChanged();
} else {
convsBuilder_.setMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .com.avos.avoscloud.ReadTuple convs = 3;
*/
public Builder addConvs(com.avos.avoscloud.Messages.ReadTuple value) {
if (convsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureConvsIsMutable();
convs_.add(value);
onChanged();
} else {
convsBuilder_.addMessage(value);
}
return this;
}
/**
* repeated .com.avos.avoscloud.ReadTuple convs = 3;
*/
public Builder addConvs(
int index, com.avos.avoscloud.Messages.ReadTuple value) {
if (convsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureConvsIsMutable();
convs_.add(index, value);
onChanged();
} else {
convsBuilder_.addMessage(index, value);
}
return this;
}
/**
* repeated .com.avos.avoscloud.ReadTuple convs = 3;
*/
public Builder addConvs(
com.avos.avoscloud.Messages.ReadTuple.Builder builderForValue) {
if (convsBuilder_ == null) {
ensureConvsIsMutable();
convs_.add(builderForValue.build());
onChanged();
} else {
convsBuilder_.addMessage(builderForValue.build());
}
return this;
}
/**
* repeated .com.avos.avoscloud.ReadTuple convs = 3;
*/
public Builder addConvs(
int index, com.avos.avoscloud.Messages.ReadTuple.Builder builderForValue) {
if (convsBuilder_ == null) {
ensureConvsIsMutable();
convs_.add(index, builderForValue.build());
onChanged();
} else {
convsBuilder_.addMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .com.avos.avoscloud.ReadTuple convs = 3;
*/
public Builder addAllConvs(
java.lang.Iterable extends com.avos.avoscloud.Messages.ReadTuple> values) {
if (convsBuilder_ == null) {
ensureConvsIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, convs_);
onChanged();
} else {
convsBuilder_.addAllMessages(values);
}
return this;
}
/**
* repeated .com.avos.avoscloud.ReadTuple convs = 3;
*/
public Builder clearConvs() {
if (convsBuilder_ == null) {
convs_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000004);
onChanged();
} else {
convsBuilder_.clear();
}
return this;
}
/**
* repeated .com.avos.avoscloud.ReadTuple convs = 3;
*/
public Builder removeConvs(int index) {
if (convsBuilder_ == null) {
ensureConvsIsMutable();
convs_.remove(index);
onChanged();
} else {
convsBuilder_.remove(index);
}
return this;
}
/**
* repeated .com.avos.avoscloud.ReadTuple convs = 3;
*/
public com.avos.avoscloud.Messages.ReadTuple.Builder getConvsBuilder(
int index) {
return getConvsFieldBuilder().getBuilder(index);
}
/**
* repeated .com.avos.avoscloud.ReadTuple convs = 3;
*/
public com.avos.avoscloud.Messages.ReadTupleOrBuilder getConvsOrBuilder(
int index) {
if (convsBuilder_ == null) {
return convs_.get(index); } else {
return convsBuilder_.getMessageOrBuilder(index);
}
}
/**
* repeated .com.avos.avoscloud.ReadTuple convs = 3;
*/
public java.util.List extends com.avos.avoscloud.Messages.ReadTupleOrBuilder>
getConvsOrBuilderList() {
if (convsBuilder_ != null) {
return convsBuilder_.getMessageOrBuilderList();
} else {
return java.util.Collections.unmodifiableList(convs_);
}
}
/**
* repeated .com.avos.avoscloud.ReadTuple convs = 3;
*/
public com.avos.avoscloud.Messages.ReadTuple.Builder addConvsBuilder() {
return getConvsFieldBuilder().addBuilder(
com.avos.avoscloud.Messages.ReadTuple.getDefaultInstance());
}
/**
* repeated .com.avos.avoscloud.ReadTuple convs = 3;
*/
public com.avos.avoscloud.Messages.ReadTuple.Builder addConvsBuilder(
int index) {
return getConvsFieldBuilder().addBuilder(
index, com.avos.avoscloud.Messages.ReadTuple.getDefaultInstance());
}
/**
* repeated .com.avos.avoscloud.ReadTuple convs = 3;
*/
public java.util.List
getConvsBuilderList() {
return getConvsFieldBuilder().getBuilderList();
}
private com.google.protobuf.RepeatedFieldBuilderV3<
com.avos.avoscloud.Messages.ReadTuple, com.avos.avoscloud.Messages.ReadTuple.Builder, com.avos.avoscloud.Messages.ReadTupleOrBuilder>
getConvsFieldBuilder() {
if (convsBuilder_ == null) {
convsBuilder_ = new com.google.protobuf.RepeatedFieldBuilderV3<
com.avos.avoscloud.Messages.ReadTuple, com.avos.avoscloud.Messages.ReadTuple.Builder, com.avos.avoscloud.Messages.ReadTupleOrBuilder>(
convs_,
((bitField0_ & 0x00000004) == 0x00000004),
getParentForChildren(),
isClean());
convs_ = null;
}
return convsBuilder_;
}
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:com.avos.avoscloud.ReadCommand)
}
// @@protoc_insertion_point(class_scope:com.avos.avoscloud.ReadCommand)
private static final com.avos.avoscloud.Messages.ReadCommand DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new com.avos.avoscloud.Messages.ReadCommand();
}
public static com.avos.avoscloud.Messages.ReadCommand getDefaultInstance() {
return DEFAULT_INSTANCE;
}
@java.lang.Deprecated public static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
public ReadCommand parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new ReadCommand(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
public com.avos.avoscloud.Messages.ReadCommand getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface PresenceCommandOrBuilder extends
// @@protoc_insertion_point(interface_extends:com.avos.avoscloud.PresenceCommand)
com.google.protobuf.MessageOrBuilder {
/**
* optional .com.avos.avoscloud.StatusType status = 1;
*/
boolean hasStatus();
/**
* optional .com.avos.avoscloud.StatusType status = 1;
*/
com.avos.avoscloud.Messages.StatusType getStatus();
/**
* repeated string sessionPeerIds = 2;
*/
java.util.List
getSessionPeerIdsList();
/**
* repeated string sessionPeerIds = 2;
*/
int getSessionPeerIdsCount();
/**
* repeated string sessionPeerIds = 2;
*/
java.lang.String getSessionPeerIds(int index);
/**
* repeated string sessionPeerIds = 2;
*/
com.google.protobuf.ByteString
getSessionPeerIdsBytes(int index);
/**
* optional string cid = 3;
*/
boolean hasCid();
/**
* optional string cid = 3;
*/
java.lang.String getCid();
/**
* optional string cid = 3;
*/
com.google.protobuf.ByteString
getCidBytes();
}
/**
* Protobuf type {@code com.avos.avoscloud.PresenceCommand}
*/
public static final class PresenceCommand extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:com.avos.avoscloud.PresenceCommand)
PresenceCommandOrBuilder {
private static final long serialVersionUID = 0L;
// Use PresenceCommand.newBuilder() to construct.
private PresenceCommand(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private PresenceCommand() {
status_ = 1;
sessionPeerIds_ = com.google.protobuf.LazyStringArrayList.EMPTY;
cid_ = "";
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private PresenceCommand(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!parseUnknownField(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
case 8: {
int rawValue = input.readEnum();
com.avos.avoscloud.Messages.StatusType value = com.avos.avoscloud.Messages.StatusType.valueOf(rawValue);
if (value == null) {
unknownFields.mergeVarintField(1, rawValue);
} else {
bitField0_ |= 0x00000001;
status_ = rawValue;
}
break;
}
case 18: {
com.google.protobuf.ByteString bs = input.readBytes();
if (!((mutable_bitField0_ & 0x00000002) == 0x00000002)) {
sessionPeerIds_ = new com.google.protobuf.LazyStringArrayList();
mutable_bitField0_ |= 0x00000002;
}
sessionPeerIds_.add(bs);
break;
}
case 26: {
com.google.protobuf.ByteString bs = input.readBytes();
bitField0_ |= 0x00000002;
cid_ = bs;
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
if (((mutable_bitField0_ & 0x00000002) == 0x00000002)) {
sessionPeerIds_ = sessionPeerIds_.getUnmodifiableView();
}
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.avos.avoscloud.Messages.internal_static_com_avos_avoscloud_PresenceCommand_descriptor;
}
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.avos.avoscloud.Messages.internal_static_com_avos_avoscloud_PresenceCommand_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.avos.avoscloud.Messages.PresenceCommand.class, com.avos.avoscloud.Messages.PresenceCommand.Builder.class);
}
private int bitField0_;
public static final int STATUS_FIELD_NUMBER = 1;
private int status_;
/**
* optional .com.avos.avoscloud.StatusType status = 1;
*/
public boolean hasStatus() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
* optional .com.avos.avoscloud.StatusType status = 1;
*/
public com.avos.avoscloud.Messages.StatusType getStatus() {
com.avos.avoscloud.Messages.StatusType result = com.avos.avoscloud.Messages.StatusType.valueOf(status_);
return result == null ? com.avos.avoscloud.Messages.StatusType.on : result;
}
public static final int SESSIONPEERIDS_FIELD_NUMBER = 2;
private com.google.protobuf.LazyStringList sessionPeerIds_;
/**
* repeated string sessionPeerIds = 2;
*/
public com.google.protobuf.ProtocolStringList
getSessionPeerIdsList() {
return sessionPeerIds_;
}
/**
* repeated string sessionPeerIds = 2;
*/
public int getSessionPeerIdsCount() {
return sessionPeerIds_.size();
}
/**
* repeated string sessionPeerIds = 2;
*/
public java.lang.String getSessionPeerIds(int index) {
return sessionPeerIds_.get(index);
}
/**
* repeated string sessionPeerIds = 2;
*/
public com.google.protobuf.ByteString
getSessionPeerIdsBytes(int index) {
return sessionPeerIds_.getByteString(index);
}
public static final int CID_FIELD_NUMBER = 3;
private volatile java.lang.Object cid_;
/**
* optional string cid = 3;
*/
public boolean hasCid() {
return ((bitField0_ & 0x00000002) == 0x00000002);
}
/**
* optional string cid = 3;
*/
public java.lang.String getCid() {
java.lang.Object ref = cid_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
cid_ = s;
}
return s;
}
}
/**
* optional string cid = 3;
*/
public com.google.protobuf.ByteString
getCidBytes() {
java.lang.Object ref = cid_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
cid_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
private byte memoizedIsInitialized = -1;
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (((bitField0_ & 0x00000001) == 0x00000001)) {
output.writeEnum(1, status_);
}
for (int i = 0; i < sessionPeerIds_.size(); i++) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 2, sessionPeerIds_.getRaw(i));
}
if (((bitField0_ & 0x00000002) == 0x00000002)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 3, cid_);
}
unknownFields.writeTo(output);
}
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (((bitField0_ & 0x00000001) == 0x00000001)) {
size += com.google.protobuf.CodedOutputStream
.computeEnumSize(1, status_);
}
{
int dataSize = 0;
for (int i = 0; i < sessionPeerIds_.size(); i++) {
dataSize += computeStringSizeNoTag(sessionPeerIds_.getRaw(i));
}
size += dataSize;
size += 1 * getSessionPeerIdsList().size();
}
if (((bitField0_ & 0x00000002) == 0x00000002)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(3, cid_);
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof com.avos.avoscloud.Messages.PresenceCommand)) {
return super.equals(obj);
}
com.avos.avoscloud.Messages.PresenceCommand other = (com.avos.avoscloud.Messages.PresenceCommand) obj;
boolean result = true;
result = result && (hasStatus() == other.hasStatus());
if (hasStatus()) {
result = result && status_ == other.status_;
}
result = result && getSessionPeerIdsList()
.equals(other.getSessionPeerIdsList());
result = result && (hasCid() == other.hasCid());
if (hasCid()) {
result = result && getCid()
.equals(other.getCid());
}
result = result && unknownFields.equals(other.unknownFields);
return result;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (hasStatus()) {
hash = (37 * hash) + STATUS_FIELD_NUMBER;
hash = (53 * hash) + status_;
}
if (getSessionPeerIdsCount() > 0) {
hash = (37 * hash) + SESSIONPEERIDS_FIELD_NUMBER;
hash = (53 * hash) + getSessionPeerIdsList().hashCode();
}
if (hasCid()) {
hash = (37 * hash) + CID_FIELD_NUMBER;
hash = (53 * hash) + getCid().hashCode();
}
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static com.avos.avoscloud.Messages.PresenceCommand parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.avos.avoscloud.Messages.PresenceCommand parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.avos.avoscloud.Messages.PresenceCommand parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.avos.avoscloud.Messages.PresenceCommand parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.avos.avoscloud.Messages.PresenceCommand parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.avos.avoscloud.Messages.PresenceCommand parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.avos.avoscloud.Messages.PresenceCommand parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.avos.avoscloud.Messages.PresenceCommand parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static com.avos.avoscloud.Messages.PresenceCommand parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static com.avos.avoscloud.Messages.PresenceCommand parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static com.avos.avoscloud.Messages.PresenceCommand parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.avos.avoscloud.Messages.PresenceCommand parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(com.avos.avoscloud.Messages.PresenceCommand prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code com.avos.avoscloud.PresenceCommand}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:com.avos.avoscloud.PresenceCommand)
com.avos.avoscloud.Messages.PresenceCommandOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.avos.avoscloud.Messages.internal_static_com_avos_avoscloud_PresenceCommand_descriptor;
}
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.avos.avoscloud.Messages.internal_static_com_avos_avoscloud_PresenceCommand_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.avos.avoscloud.Messages.PresenceCommand.class, com.avos.avoscloud.Messages.PresenceCommand.Builder.class);
}
// Construct using com.avos.avoscloud.Messages.PresenceCommand.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
public Builder clear() {
super.clear();
status_ = 1;
bitField0_ = (bitField0_ & ~0x00000001);
sessionPeerIds_ = com.google.protobuf.LazyStringArrayList.EMPTY;
bitField0_ = (bitField0_ & ~0x00000002);
cid_ = "";
bitField0_ = (bitField0_ & ~0x00000004);
return this;
}
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return com.avos.avoscloud.Messages.internal_static_com_avos_avoscloud_PresenceCommand_descriptor;
}
public com.avos.avoscloud.Messages.PresenceCommand getDefaultInstanceForType() {
return com.avos.avoscloud.Messages.PresenceCommand.getDefaultInstance();
}
public com.avos.avoscloud.Messages.PresenceCommand build() {
com.avos.avoscloud.Messages.PresenceCommand result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
public com.avos.avoscloud.Messages.PresenceCommand buildPartial() {
com.avos.avoscloud.Messages.PresenceCommand result = new com.avos.avoscloud.Messages.PresenceCommand(this);
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (((from_bitField0_ & 0x00000001) == 0x00000001)) {
to_bitField0_ |= 0x00000001;
}
result.status_ = status_;
if (((bitField0_ & 0x00000002) == 0x00000002)) {
sessionPeerIds_ = sessionPeerIds_.getUnmodifiableView();
bitField0_ = (bitField0_ & ~0x00000002);
}
result.sessionPeerIds_ = sessionPeerIds_;
if (((from_bitField0_ & 0x00000004) == 0x00000004)) {
to_bitField0_ |= 0x00000002;
}
result.cid_ = cid_;
result.bitField0_ = to_bitField0_;
onBuilt();
return result;
}
public Builder clone() {
return (Builder) super.clone();
}
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.setField(field, value);
}
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return (Builder) super.clearField(field);
}
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return (Builder) super.clearOneof(oneof);
}
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return (Builder) super.setRepeatedField(field, index, value);
}
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.addRepeatedField(field, value);
}
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.avos.avoscloud.Messages.PresenceCommand) {
return mergeFrom((com.avos.avoscloud.Messages.PresenceCommand)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.avos.avoscloud.Messages.PresenceCommand other) {
if (other == com.avos.avoscloud.Messages.PresenceCommand.getDefaultInstance()) return this;
if (other.hasStatus()) {
setStatus(other.getStatus());
}
if (!other.sessionPeerIds_.isEmpty()) {
if (sessionPeerIds_.isEmpty()) {
sessionPeerIds_ = other.sessionPeerIds_;
bitField0_ = (bitField0_ & ~0x00000002);
} else {
ensureSessionPeerIdsIsMutable();
sessionPeerIds_.addAll(other.sessionPeerIds_);
}
onChanged();
}
if (other.hasCid()) {
bitField0_ |= 0x00000004;
cid_ = other.cid_;
onChanged();
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
public final boolean isInitialized() {
return true;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
com.avos.avoscloud.Messages.PresenceCommand parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (com.avos.avoscloud.Messages.PresenceCommand) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
private int status_ = 1;
/**
* optional .com.avos.avoscloud.StatusType status = 1;
*/
public boolean hasStatus() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
* optional .com.avos.avoscloud.StatusType status = 1;
*/
public com.avos.avoscloud.Messages.StatusType getStatus() {
com.avos.avoscloud.Messages.StatusType result = com.avos.avoscloud.Messages.StatusType.valueOf(status_);
return result == null ? com.avos.avoscloud.Messages.StatusType.on : result;
}
/**
* optional .com.avos.avoscloud.StatusType status = 1;
*/
public Builder setStatus(com.avos.avoscloud.Messages.StatusType value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000001;
status_ = value.getNumber();
onChanged();
return this;
}
/**
* optional .com.avos.avoscloud.StatusType status = 1;
*/
public Builder clearStatus() {
bitField0_ = (bitField0_ & ~0x00000001);
status_ = 1;
onChanged();
return this;
}
private com.google.protobuf.LazyStringList sessionPeerIds_ = com.google.protobuf.LazyStringArrayList.EMPTY;
private void ensureSessionPeerIdsIsMutable() {
if (!((bitField0_ & 0x00000002) == 0x00000002)) {
sessionPeerIds_ = new com.google.protobuf.LazyStringArrayList(sessionPeerIds_);
bitField0_ |= 0x00000002;
}
}
/**
* repeated string sessionPeerIds = 2;
*/
public com.google.protobuf.ProtocolStringList
getSessionPeerIdsList() {
return sessionPeerIds_.getUnmodifiableView();
}
/**
* repeated string sessionPeerIds = 2;
*/
public int getSessionPeerIdsCount() {
return sessionPeerIds_.size();
}
/**
* repeated string sessionPeerIds = 2;
*/
public java.lang.String getSessionPeerIds(int index) {
return sessionPeerIds_.get(index);
}
/**
* repeated string sessionPeerIds = 2;
*/
public com.google.protobuf.ByteString
getSessionPeerIdsBytes(int index) {
return sessionPeerIds_.getByteString(index);
}
/**
* repeated string sessionPeerIds = 2;
*/
public Builder setSessionPeerIds(
int index, java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
ensureSessionPeerIdsIsMutable();
sessionPeerIds_.set(index, value);
onChanged();
return this;
}
/**
* repeated string sessionPeerIds = 2;
*/
public Builder addSessionPeerIds(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
ensureSessionPeerIdsIsMutable();
sessionPeerIds_.add(value);
onChanged();
return this;
}
/**
* repeated string sessionPeerIds = 2;
*/
public Builder addAllSessionPeerIds(
java.lang.Iterable values) {
ensureSessionPeerIdsIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, sessionPeerIds_);
onChanged();
return this;
}
/**
* repeated string sessionPeerIds = 2;
*/
public Builder clearSessionPeerIds() {
sessionPeerIds_ = com.google.protobuf.LazyStringArrayList.EMPTY;
bitField0_ = (bitField0_ & ~0x00000002);
onChanged();
return this;
}
/**
* repeated string sessionPeerIds = 2;
*/
public Builder addSessionPeerIdsBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
ensureSessionPeerIdsIsMutable();
sessionPeerIds_.add(value);
onChanged();
return this;
}
private java.lang.Object cid_ = "";
/**
* optional string cid = 3;
*/
public boolean hasCid() {
return ((bitField0_ & 0x00000004) == 0x00000004);
}
/**
* optional string cid = 3;
*/
public java.lang.String getCid() {
java.lang.Object ref = cid_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
cid_ = s;
}
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* optional string cid = 3;
*/
public com.google.protobuf.ByteString
getCidBytes() {
java.lang.Object ref = cid_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
cid_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* optional string cid = 3;
*/
public Builder setCid(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000004;
cid_ = value;
onChanged();
return this;
}
/**
* optional string cid = 3;
*/
public Builder clearCid() {
bitField0_ = (bitField0_ & ~0x00000004);
cid_ = getDefaultInstance().getCid();
onChanged();
return this;
}
/**
* optional string cid = 3;
*/
public Builder setCidBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000004;
cid_ = value;
onChanged();
return this;
}
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:com.avos.avoscloud.PresenceCommand)
}
// @@protoc_insertion_point(class_scope:com.avos.avoscloud.PresenceCommand)
private static final com.avos.avoscloud.Messages.PresenceCommand DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new com.avos.avoscloud.Messages.PresenceCommand();
}
public static com.avos.avoscloud.Messages.PresenceCommand getDefaultInstance() {
return DEFAULT_INSTANCE;
}
@java.lang.Deprecated public static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
public PresenceCommand parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new PresenceCommand(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
public com.avos.avoscloud.Messages.PresenceCommand getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface ReportCommandOrBuilder extends
// @@protoc_insertion_point(interface_extends:com.avos.avoscloud.ReportCommand)
com.google.protobuf.MessageOrBuilder {
/**
* optional bool initiative = 1;
*/
boolean hasInitiative();
/**
* optional bool initiative = 1;
*/
boolean getInitiative();
/**
* optional string type = 2;
*/
boolean hasType();
/**
* optional string type = 2;
*/
java.lang.String getType();
/**
* optional string type = 2;
*/
com.google.protobuf.ByteString
getTypeBytes();
/**
* optional string data = 3;
*/
boolean hasData();
/**
* optional string data = 3;
*/
java.lang.String getData();
/**
* optional string data = 3;
*/
com.google.protobuf.ByteString
getDataBytes();
}
/**
* Protobuf type {@code com.avos.avoscloud.ReportCommand}
*/
public static final class ReportCommand extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:com.avos.avoscloud.ReportCommand)
ReportCommandOrBuilder {
private static final long serialVersionUID = 0L;
// Use ReportCommand.newBuilder() to construct.
private ReportCommand(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private ReportCommand() {
initiative_ = false;
type_ = "";
data_ = "";
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private ReportCommand(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!parseUnknownField(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
case 8: {
bitField0_ |= 0x00000001;
initiative_ = input.readBool();
break;
}
case 18: {
com.google.protobuf.ByteString bs = input.readBytes();
bitField0_ |= 0x00000002;
type_ = bs;
break;
}
case 26: {
com.google.protobuf.ByteString bs = input.readBytes();
bitField0_ |= 0x00000004;
data_ = bs;
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.avos.avoscloud.Messages.internal_static_com_avos_avoscloud_ReportCommand_descriptor;
}
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.avos.avoscloud.Messages.internal_static_com_avos_avoscloud_ReportCommand_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.avos.avoscloud.Messages.ReportCommand.class, com.avos.avoscloud.Messages.ReportCommand.Builder.class);
}
private int bitField0_;
public static final int INITIATIVE_FIELD_NUMBER = 1;
private boolean initiative_;
/**
* optional bool initiative = 1;
*/
public boolean hasInitiative() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
* optional bool initiative = 1;
*/
public boolean getInitiative() {
return initiative_;
}
public static final int TYPE_FIELD_NUMBER = 2;
private volatile java.lang.Object type_;
/**
* optional string type = 2;
*/
public boolean hasType() {
return ((bitField0_ & 0x00000002) == 0x00000002);
}
/**
* optional string type = 2;
*/
public java.lang.String getType() {
java.lang.Object ref = type_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
type_ = s;
}
return s;
}
}
/**
* optional string type = 2;
*/
public com.google.protobuf.ByteString
getTypeBytes() {
java.lang.Object ref = type_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
type_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int DATA_FIELD_NUMBER = 3;
private volatile java.lang.Object data_;
/**
* optional string data = 3;
*/
public boolean hasData() {
return ((bitField0_ & 0x00000004) == 0x00000004);
}
/**
* optional string data = 3;
*/
public java.lang.String getData() {
java.lang.Object ref = data_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
data_ = s;
}
return s;
}
}
/**
* optional string data = 3;
*/
public com.google.protobuf.ByteString
getDataBytes() {
java.lang.Object ref = data_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
data_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
private byte memoizedIsInitialized = -1;
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (((bitField0_ & 0x00000001) == 0x00000001)) {
output.writeBool(1, initiative_);
}
if (((bitField0_ & 0x00000002) == 0x00000002)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 2, type_);
}
if (((bitField0_ & 0x00000004) == 0x00000004)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 3, data_);
}
unknownFields.writeTo(output);
}
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (((bitField0_ & 0x00000001) == 0x00000001)) {
size += com.google.protobuf.CodedOutputStream
.computeBoolSize(1, initiative_);
}
if (((bitField0_ & 0x00000002) == 0x00000002)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(2, type_);
}
if (((bitField0_ & 0x00000004) == 0x00000004)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(3, data_);
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof com.avos.avoscloud.Messages.ReportCommand)) {
return super.equals(obj);
}
com.avos.avoscloud.Messages.ReportCommand other = (com.avos.avoscloud.Messages.ReportCommand) obj;
boolean result = true;
result = result && (hasInitiative() == other.hasInitiative());
if (hasInitiative()) {
result = result && (getInitiative()
== other.getInitiative());
}
result = result && (hasType() == other.hasType());
if (hasType()) {
result = result && getType()
.equals(other.getType());
}
result = result && (hasData() == other.hasData());
if (hasData()) {
result = result && getData()
.equals(other.getData());
}
result = result && unknownFields.equals(other.unknownFields);
return result;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (hasInitiative()) {
hash = (37 * hash) + INITIATIVE_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashBoolean(
getInitiative());
}
if (hasType()) {
hash = (37 * hash) + TYPE_FIELD_NUMBER;
hash = (53 * hash) + getType().hashCode();
}
if (hasData()) {
hash = (37 * hash) + DATA_FIELD_NUMBER;
hash = (53 * hash) + getData().hashCode();
}
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static com.avos.avoscloud.Messages.ReportCommand parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.avos.avoscloud.Messages.ReportCommand parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.avos.avoscloud.Messages.ReportCommand parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.avos.avoscloud.Messages.ReportCommand parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.avos.avoscloud.Messages.ReportCommand parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.avos.avoscloud.Messages.ReportCommand parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.avos.avoscloud.Messages.ReportCommand parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.avos.avoscloud.Messages.ReportCommand parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static com.avos.avoscloud.Messages.ReportCommand parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static com.avos.avoscloud.Messages.ReportCommand parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static com.avos.avoscloud.Messages.ReportCommand parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.avos.avoscloud.Messages.ReportCommand parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(com.avos.avoscloud.Messages.ReportCommand prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code com.avos.avoscloud.ReportCommand}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:com.avos.avoscloud.ReportCommand)
com.avos.avoscloud.Messages.ReportCommandOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.avos.avoscloud.Messages.internal_static_com_avos_avoscloud_ReportCommand_descriptor;
}
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.avos.avoscloud.Messages.internal_static_com_avos_avoscloud_ReportCommand_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.avos.avoscloud.Messages.ReportCommand.class, com.avos.avoscloud.Messages.ReportCommand.Builder.class);
}
// Construct using com.avos.avoscloud.Messages.ReportCommand.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
public Builder clear() {
super.clear();
initiative_ = false;
bitField0_ = (bitField0_ & ~0x00000001);
type_ = "";
bitField0_ = (bitField0_ & ~0x00000002);
data_ = "";
bitField0_ = (bitField0_ & ~0x00000004);
return this;
}
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return com.avos.avoscloud.Messages.internal_static_com_avos_avoscloud_ReportCommand_descriptor;
}
public com.avos.avoscloud.Messages.ReportCommand getDefaultInstanceForType() {
return com.avos.avoscloud.Messages.ReportCommand.getDefaultInstance();
}
public com.avos.avoscloud.Messages.ReportCommand build() {
com.avos.avoscloud.Messages.ReportCommand result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
public com.avos.avoscloud.Messages.ReportCommand buildPartial() {
com.avos.avoscloud.Messages.ReportCommand result = new com.avos.avoscloud.Messages.ReportCommand(this);
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (((from_bitField0_ & 0x00000001) == 0x00000001)) {
to_bitField0_ |= 0x00000001;
}
result.initiative_ = initiative_;
if (((from_bitField0_ & 0x00000002) == 0x00000002)) {
to_bitField0_ |= 0x00000002;
}
result.type_ = type_;
if (((from_bitField0_ & 0x00000004) == 0x00000004)) {
to_bitField0_ |= 0x00000004;
}
result.data_ = data_;
result.bitField0_ = to_bitField0_;
onBuilt();
return result;
}
public Builder clone() {
return (Builder) super.clone();
}
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.setField(field, value);
}
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return (Builder) super.clearField(field);
}
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return (Builder) super.clearOneof(oneof);
}
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return (Builder) super.setRepeatedField(field, index, value);
}
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.addRepeatedField(field, value);
}
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.avos.avoscloud.Messages.ReportCommand) {
return mergeFrom((com.avos.avoscloud.Messages.ReportCommand)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.avos.avoscloud.Messages.ReportCommand other) {
if (other == com.avos.avoscloud.Messages.ReportCommand.getDefaultInstance()) return this;
if (other.hasInitiative()) {
setInitiative(other.getInitiative());
}
if (other.hasType()) {
bitField0_ |= 0x00000002;
type_ = other.type_;
onChanged();
}
if (other.hasData()) {
bitField0_ |= 0x00000004;
data_ = other.data_;
onChanged();
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
public final boolean isInitialized() {
return true;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
com.avos.avoscloud.Messages.ReportCommand parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (com.avos.avoscloud.Messages.ReportCommand) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
private boolean initiative_ ;
/**
* optional bool initiative = 1;
*/
public boolean hasInitiative() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
* optional bool initiative = 1;
*/
public boolean getInitiative() {
return initiative_;
}
/**
* optional bool initiative = 1;
*/
public Builder setInitiative(boolean value) {
bitField0_ |= 0x00000001;
initiative_ = value;
onChanged();
return this;
}
/**
* optional bool initiative = 1;
*/
public Builder clearInitiative() {
bitField0_ = (bitField0_ & ~0x00000001);
initiative_ = false;
onChanged();
return this;
}
private java.lang.Object type_ = "";
/**
* optional string type = 2;
*/
public boolean hasType() {
return ((bitField0_ & 0x00000002) == 0x00000002);
}
/**
* optional string type = 2;
*/
public java.lang.String getType() {
java.lang.Object ref = type_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
type_ = s;
}
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* optional string type = 2;
*/
public com.google.protobuf.ByteString
getTypeBytes() {
java.lang.Object ref = type_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
type_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* optional string type = 2;
*/
public Builder setType(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000002;
type_ = value;
onChanged();
return this;
}
/**
* optional string type = 2;
*/
public Builder clearType() {
bitField0_ = (bitField0_ & ~0x00000002);
type_ = getDefaultInstance().getType();
onChanged();
return this;
}
/**
* optional string type = 2;
*/
public Builder setTypeBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000002;
type_ = value;
onChanged();
return this;
}
private java.lang.Object data_ = "";
/**
* optional string data = 3;
*/
public boolean hasData() {
return ((bitField0_ & 0x00000004) == 0x00000004);
}
/**
* optional string data = 3;
*/
public java.lang.String getData() {
java.lang.Object ref = data_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
data_ = s;
}
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* optional string data = 3;
*/
public com.google.protobuf.ByteString
getDataBytes() {
java.lang.Object ref = data_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
data_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* optional string data = 3;
*/
public Builder setData(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000004;
data_ = value;
onChanged();
return this;
}
/**
* optional string data = 3;
*/
public Builder clearData() {
bitField0_ = (bitField0_ & ~0x00000004);
data_ = getDefaultInstance().getData();
onChanged();
return this;
}
/**
* optional string data = 3;
*/
public Builder setDataBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000004;
data_ = value;
onChanged();
return this;
}
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:com.avos.avoscloud.ReportCommand)
}
// @@protoc_insertion_point(class_scope:com.avos.avoscloud.ReportCommand)
private static final com.avos.avoscloud.Messages.ReportCommand DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new com.avos.avoscloud.Messages.ReportCommand();
}
public static com.avos.avoscloud.Messages.ReportCommand getDefaultInstance() {
return DEFAULT_INSTANCE;
}
@java.lang.Deprecated public static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
public ReportCommand parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new ReportCommand(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
public com.avos.avoscloud.Messages.ReportCommand getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface PatchItemOrBuilder extends
// @@protoc_insertion_point(interface_extends:com.avos.avoscloud.PatchItem)
com.google.protobuf.MessageOrBuilder {
/**
* optional string cid = 1;
*/
boolean hasCid();
/**
* optional string cid = 1;
*/
java.lang.String getCid();
/**
* optional string cid = 1;
*/
com.google.protobuf.ByteString
getCidBytes();
/**
* optional string mid = 2;
*/
boolean hasMid();
/**
* optional string mid = 2;
*/
java.lang.String getMid();
/**
* optional string mid = 2;
*/
com.google.protobuf.ByteString
getMidBytes();
/**
* optional int64 timestamp = 3;
*/
boolean hasTimestamp();
/**
* optional int64 timestamp = 3;
*/
long getTimestamp();
/**
* optional bool recall = 4;
*/
boolean hasRecall();
/**
* optional bool recall = 4;
*/
boolean getRecall();
/**
* optional string data = 5;
*/
boolean hasData();
/**
* optional string data = 5;
*/
java.lang.String getData();
/**
* optional string data = 5;
*/
com.google.protobuf.ByteString
getDataBytes();
/**
* optional int64 patchTimestamp = 6;
*/
boolean hasPatchTimestamp();
/**
* optional int64 patchTimestamp = 6;
*/
long getPatchTimestamp();
/**
* optional string from = 7;
*/
boolean hasFrom();
/**
* optional string from = 7;
*/
java.lang.String getFrom();
/**
* optional string from = 7;
*/
com.google.protobuf.ByteString
getFromBytes();
/**
* optional bytes binaryMsg = 8;
*/
boolean hasBinaryMsg();
/**
* optional bytes binaryMsg = 8;
*/
com.google.protobuf.ByteString getBinaryMsg();
/**
* optional bool mentionAll = 9;
*/
boolean hasMentionAll();
/**
* optional bool mentionAll = 9;
*/
boolean getMentionAll();
/**
* repeated string mentionPids = 10;
*/
java.util.List
getMentionPidsList();
/**
* repeated string mentionPids = 10;
*/
int getMentionPidsCount();
/**
* repeated string mentionPids = 10;
*/
java.lang.String getMentionPids(int index);
/**
* repeated string mentionPids = 10;
*/
com.google.protobuf.ByteString
getMentionPidsBytes(int index);
/**
* optional int64 patchCode = 11;
*/
boolean hasPatchCode();
/**
* optional int64 patchCode = 11;
*/
long getPatchCode();
/**
* optional string patchReason = 12;
*/
boolean hasPatchReason();
/**
* optional string patchReason = 12;
*/
java.lang.String getPatchReason();
/**
* optional string patchReason = 12;
*/
com.google.protobuf.ByteString
getPatchReasonBytes();
}
/**
* Protobuf type {@code com.avos.avoscloud.PatchItem}
*/
public static final class PatchItem extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:com.avos.avoscloud.PatchItem)
PatchItemOrBuilder {
private static final long serialVersionUID = 0L;
// Use PatchItem.newBuilder() to construct.
private PatchItem(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private PatchItem() {
cid_ = "";
mid_ = "";
timestamp_ = 0L;
recall_ = false;
data_ = "";
patchTimestamp_ = 0L;
from_ = "";
binaryMsg_ = com.google.protobuf.ByteString.EMPTY;
mentionAll_ = false;
mentionPids_ = com.google.protobuf.LazyStringArrayList.EMPTY;
patchCode_ = 0L;
patchReason_ = "";
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private PatchItem(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!parseUnknownField(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
case 10: {
com.google.protobuf.ByteString bs = input.readBytes();
bitField0_ |= 0x00000001;
cid_ = bs;
break;
}
case 18: {
com.google.protobuf.ByteString bs = input.readBytes();
bitField0_ |= 0x00000002;
mid_ = bs;
break;
}
case 24: {
bitField0_ |= 0x00000004;
timestamp_ = input.readInt64();
break;
}
case 32: {
bitField0_ |= 0x00000008;
recall_ = input.readBool();
break;
}
case 42: {
com.google.protobuf.ByteString bs = input.readBytes();
bitField0_ |= 0x00000010;
data_ = bs;
break;
}
case 48: {
bitField0_ |= 0x00000020;
patchTimestamp_ = input.readInt64();
break;
}
case 58: {
com.google.protobuf.ByteString bs = input.readBytes();
bitField0_ |= 0x00000040;
from_ = bs;
break;
}
case 66: {
bitField0_ |= 0x00000080;
binaryMsg_ = input.readBytes();
break;
}
case 72: {
bitField0_ |= 0x00000100;
mentionAll_ = input.readBool();
break;
}
case 82: {
com.google.protobuf.ByteString bs = input.readBytes();
if (!((mutable_bitField0_ & 0x00000200) == 0x00000200)) {
mentionPids_ = new com.google.protobuf.LazyStringArrayList();
mutable_bitField0_ |= 0x00000200;
}
mentionPids_.add(bs);
break;
}
case 88: {
bitField0_ |= 0x00000200;
patchCode_ = input.readInt64();
break;
}
case 98: {
com.google.protobuf.ByteString bs = input.readBytes();
bitField0_ |= 0x00000400;
patchReason_ = bs;
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
if (((mutable_bitField0_ & 0x00000200) == 0x00000200)) {
mentionPids_ = mentionPids_.getUnmodifiableView();
}
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.avos.avoscloud.Messages.internal_static_com_avos_avoscloud_PatchItem_descriptor;
}
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.avos.avoscloud.Messages.internal_static_com_avos_avoscloud_PatchItem_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.avos.avoscloud.Messages.PatchItem.class, com.avos.avoscloud.Messages.PatchItem.Builder.class);
}
private int bitField0_;
public static final int CID_FIELD_NUMBER = 1;
private volatile java.lang.Object cid_;
/**
* optional string cid = 1;
*/
public boolean hasCid() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
* optional string cid = 1;
*/
public java.lang.String getCid() {
java.lang.Object ref = cid_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
cid_ = s;
}
return s;
}
}
/**
* optional string cid = 1;
*/
public com.google.protobuf.ByteString
getCidBytes() {
java.lang.Object ref = cid_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
cid_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int MID_FIELD_NUMBER = 2;
private volatile java.lang.Object mid_;
/**
* optional string mid = 2;
*/
public boolean hasMid() {
return ((bitField0_ & 0x00000002) == 0x00000002);
}
/**
* optional string mid = 2;
*/
public java.lang.String getMid() {
java.lang.Object ref = mid_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
mid_ = s;
}
return s;
}
}
/**
* optional string mid = 2;
*/
public com.google.protobuf.ByteString
getMidBytes() {
java.lang.Object ref = mid_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
mid_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int TIMESTAMP_FIELD_NUMBER = 3;
private long timestamp_;
/**
* optional int64 timestamp = 3;
*/
public boolean hasTimestamp() {
return ((bitField0_ & 0x00000004) == 0x00000004);
}
/**
* optional int64 timestamp = 3;
*/
public long getTimestamp() {
return timestamp_;
}
public static final int RECALL_FIELD_NUMBER = 4;
private boolean recall_;
/**
* optional bool recall = 4;
*/
public boolean hasRecall() {
return ((bitField0_ & 0x00000008) == 0x00000008);
}
/**
* optional bool recall = 4;
*/
public boolean getRecall() {
return recall_;
}
public static final int DATA_FIELD_NUMBER = 5;
private volatile java.lang.Object data_;
/**
* optional string data = 5;
*/
public boolean hasData() {
return ((bitField0_ & 0x00000010) == 0x00000010);
}
/**
* optional string data = 5;
*/
public java.lang.String getData() {
java.lang.Object ref = data_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
data_ = s;
}
return s;
}
}
/**
* optional string data = 5;
*/
public com.google.protobuf.ByteString
getDataBytes() {
java.lang.Object ref = data_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
data_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int PATCHTIMESTAMP_FIELD_NUMBER = 6;
private long patchTimestamp_;
/**
* optional int64 patchTimestamp = 6;
*/
public boolean hasPatchTimestamp() {
return ((bitField0_ & 0x00000020) == 0x00000020);
}
/**
* optional int64 patchTimestamp = 6;
*/
public long getPatchTimestamp() {
return patchTimestamp_;
}
public static final int FROM_FIELD_NUMBER = 7;
private volatile java.lang.Object from_;
/**
* optional string from = 7;
*/
public boolean hasFrom() {
return ((bitField0_ & 0x00000040) == 0x00000040);
}
/**
* optional string from = 7;
*/
public java.lang.String getFrom() {
java.lang.Object ref = from_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
from_ = s;
}
return s;
}
}
/**
* optional string from = 7;
*/
public com.google.protobuf.ByteString
getFromBytes() {
java.lang.Object ref = from_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
from_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int BINARYMSG_FIELD_NUMBER = 8;
private com.google.protobuf.ByteString binaryMsg_;
/**
* optional bytes binaryMsg = 8;
*/
public boolean hasBinaryMsg() {
return ((bitField0_ & 0x00000080) == 0x00000080);
}
/**
* optional bytes binaryMsg = 8;
*/
public com.google.protobuf.ByteString getBinaryMsg() {
return binaryMsg_;
}
public static final int MENTIONALL_FIELD_NUMBER = 9;
private boolean mentionAll_;
/**
* optional bool mentionAll = 9;
*/
public boolean hasMentionAll() {
return ((bitField0_ & 0x00000100) == 0x00000100);
}
/**
* optional bool mentionAll = 9;
*/
public boolean getMentionAll() {
return mentionAll_;
}
public static final int MENTIONPIDS_FIELD_NUMBER = 10;
private com.google.protobuf.LazyStringList mentionPids_;
/**
* repeated string mentionPids = 10;
*/
public com.google.protobuf.ProtocolStringList
getMentionPidsList() {
return mentionPids_;
}
/**
* repeated string mentionPids = 10;
*/
public int getMentionPidsCount() {
return mentionPids_.size();
}
/**
* repeated string mentionPids = 10;
*/
public java.lang.String getMentionPids(int index) {
return mentionPids_.get(index);
}
/**
* repeated string mentionPids = 10;
*/
public com.google.protobuf.ByteString
getMentionPidsBytes(int index) {
return mentionPids_.getByteString(index);
}
public static final int PATCHCODE_FIELD_NUMBER = 11;
private long patchCode_;
/**
* optional int64 patchCode = 11;
*/
public boolean hasPatchCode() {
return ((bitField0_ & 0x00000200) == 0x00000200);
}
/**
* optional int64 patchCode = 11;
*/
public long getPatchCode() {
return patchCode_;
}
public static final int PATCHREASON_FIELD_NUMBER = 12;
private volatile java.lang.Object patchReason_;
/**
* optional string patchReason = 12;
*/
public boolean hasPatchReason() {
return ((bitField0_ & 0x00000400) == 0x00000400);
}
/**
* optional string patchReason = 12;
*/
public java.lang.String getPatchReason() {
java.lang.Object ref = patchReason_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
patchReason_ = s;
}
return s;
}
}
/**
* optional string patchReason = 12;
*/
public com.google.protobuf.ByteString
getPatchReasonBytes() {
java.lang.Object ref = patchReason_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
patchReason_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
private byte memoizedIsInitialized = -1;
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (((bitField0_ & 0x00000001) == 0x00000001)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 1, cid_);
}
if (((bitField0_ & 0x00000002) == 0x00000002)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 2, mid_);
}
if (((bitField0_ & 0x00000004) == 0x00000004)) {
output.writeInt64(3, timestamp_);
}
if (((bitField0_ & 0x00000008) == 0x00000008)) {
output.writeBool(4, recall_);
}
if (((bitField0_ & 0x00000010) == 0x00000010)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 5, data_);
}
if (((bitField0_ & 0x00000020) == 0x00000020)) {
output.writeInt64(6, patchTimestamp_);
}
if (((bitField0_ & 0x00000040) == 0x00000040)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 7, from_);
}
if (((bitField0_ & 0x00000080) == 0x00000080)) {
output.writeBytes(8, binaryMsg_);
}
if (((bitField0_ & 0x00000100) == 0x00000100)) {
output.writeBool(9, mentionAll_);
}
for (int i = 0; i < mentionPids_.size(); i++) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 10, mentionPids_.getRaw(i));
}
if (((bitField0_ & 0x00000200) == 0x00000200)) {
output.writeInt64(11, patchCode_);
}
if (((bitField0_ & 0x00000400) == 0x00000400)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 12, patchReason_);
}
unknownFields.writeTo(output);
}
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (((bitField0_ & 0x00000001) == 0x00000001)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(1, cid_);
}
if (((bitField0_ & 0x00000002) == 0x00000002)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(2, mid_);
}
if (((bitField0_ & 0x00000004) == 0x00000004)) {
size += com.google.protobuf.CodedOutputStream
.computeInt64Size(3, timestamp_);
}
if (((bitField0_ & 0x00000008) == 0x00000008)) {
size += com.google.protobuf.CodedOutputStream
.computeBoolSize(4, recall_);
}
if (((bitField0_ & 0x00000010) == 0x00000010)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(5, data_);
}
if (((bitField0_ & 0x00000020) == 0x00000020)) {
size += com.google.protobuf.CodedOutputStream
.computeInt64Size(6, patchTimestamp_);
}
if (((bitField0_ & 0x00000040) == 0x00000040)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(7, from_);
}
if (((bitField0_ & 0x00000080) == 0x00000080)) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(8, binaryMsg_);
}
if (((bitField0_ & 0x00000100) == 0x00000100)) {
size += com.google.protobuf.CodedOutputStream
.computeBoolSize(9, mentionAll_);
}
{
int dataSize = 0;
for (int i = 0; i < mentionPids_.size(); i++) {
dataSize += computeStringSizeNoTag(mentionPids_.getRaw(i));
}
size += dataSize;
size += 1 * getMentionPidsList().size();
}
if (((bitField0_ & 0x00000200) == 0x00000200)) {
size += com.google.protobuf.CodedOutputStream
.computeInt64Size(11, patchCode_);
}
if (((bitField0_ & 0x00000400) == 0x00000400)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(12, patchReason_);
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof com.avos.avoscloud.Messages.PatchItem)) {
return super.equals(obj);
}
com.avos.avoscloud.Messages.PatchItem other = (com.avos.avoscloud.Messages.PatchItem) obj;
boolean result = true;
result = result && (hasCid() == other.hasCid());
if (hasCid()) {
result = result && getCid()
.equals(other.getCid());
}
result = result && (hasMid() == other.hasMid());
if (hasMid()) {
result = result && getMid()
.equals(other.getMid());
}
result = result && (hasTimestamp() == other.hasTimestamp());
if (hasTimestamp()) {
result = result && (getTimestamp()
== other.getTimestamp());
}
result = result && (hasRecall() == other.hasRecall());
if (hasRecall()) {
result = result && (getRecall()
== other.getRecall());
}
result = result && (hasData() == other.hasData());
if (hasData()) {
result = result && getData()
.equals(other.getData());
}
result = result && (hasPatchTimestamp() == other.hasPatchTimestamp());
if (hasPatchTimestamp()) {
result = result && (getPatchTimestamp()
== other.getPatchTimestamp());
}
result = result && (hasFrom() == other.hasFrom());
if (hasFrom()) {
result = result && getFrom()
.equals(other.getFrom());
}
result = result && (hasBinaryMsg() == other.hasBinaryMsg());
if (hasBinaryMsg()) {
result = result && getBinaryMsg()
.equals(other.getBinaryMsg());
}
result = result && (hasMentionAll() == other.hasMentionAll());
if (hasMentionAll()) {
result = result && (getMentionAll()
== other.getMentionAll());
}
result = result && getMentionPidsList()
.equals(other.getMentionPidsList());
result = result && (hasPatchCode() == other.hasPatchCode());
if (hasPatchCode()) {
result = result && (getPatchCode()
== other.getPatchCode());
}
result = result && (hasPatchReason() == other.hasPatchReason());
if (hasPatchReason()) {
result = result && getPatchReason()
.equals(other.getPatchReason());
}
result = result && unknownFields.equals(other.unknownFields);
return result;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (hasCid()) {
hash = (37 * hash) + CID_FIELD_NUMBER;
hash = (53 * hash) + getCid().hashCode();
}
if (hasMid()) {
hash = (37 * hash) + MID_FIELD_NUMBER;
hash = (53 * hash) + getMid().hashCode();
}
if (hasTimestamp()) {
hash = (37 * hash) + TIMESTAMP_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getTimestamp());
}
if (hasRecall()) {
hash = (37 * hash) + RECALL_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashBoolean(
getRecall());
}
if (hasData()) {
hash = (37 * hash) + DATA_FIELD_NUMBER;
hash = (53 * hash) + getData().hashCode();
}
if (hasPatchTimestamp()) {
hash = (37 * hash) + PATCHTIMESTAMP_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getPatchTimestamp());
}
if (hasFrom()) {
hash = (37 * hash) + FROM_FIELD_NUMBER;
hash = (53 * hash) + getFrom().hashCode();
}
if (hasBinaryMsg()) {
hash = (37 * hash) + BINARYMSG_FIELD_NUMBER;
hash = (53 * hash) + getBinaryMsg().hashCode();
}
if (hasMentionAll()) {
hash = (37 * hash) + MENTIONALL_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashBoolean(
getMentionAll());
}
if (getMentionPidsCount() > 0) {
hash = (37 * hash) + MENTIONPIDS_FIELD_NUMBER;
hash = (53 * hash) + getMentionPidsList().hashCode();
}
if (hasPatchCode()) {
hash = (37 * hash) + PATCHCODE_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getPatchCode());
}
if (hasPatchReason()) {
hash = (37 * hash) + PATCHREASON_FIELD_NUMBER;
hash = (53 * hash) + getPatchReason().hashCode();
}
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static com.avos.avoscloud.Messages.PatchItem parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.avos.avoscloud.Messages.PatchItem parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.avos.avoscloud.Messages.PatchItem parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.avos.avoscloud.Messages.PatchItem parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.avos.avoscloud.Messages.PatchItem parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.avos.avoscloud.Messages.PatchItem parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.avos.avoscloud.Messages.PatchItem parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.avos.avoscloud.Messages.PatchItem parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static com.avos.avoscloud.Messages.PatchItem parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static com.avos.avoscloud.Messages.PatchItem parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static com.avos.avoscloud.Messages.PatchItem parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.avos.avoscloud.Messages.PatchItem parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(com.avos.avoscloud.Messages.PatchItem prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code com.avos.avoscloud.PatchItem}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:com.avos.avoscloud.PatchItem)
com.avos.avoscloud.Messages.PatchItemOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.avos.avoscloud.Messages.internal_static_com_avos_avoscloud_PatchItem_descriptor;
}
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.avos.avoscloud.Messages.internal_static_com_avos_avoscloud_PatchItem_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.avos.avoscloud.Messages.PatchItem.class, com.avos.avoscloud.Messages.PatchItem.Builder.class);
}
// Construct using com.avos.avoscloud.Messages.PatchItem.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
public Builder clear() {
super.clear();
cid_ = "";
bitField0_ = (bitField0_ & ~0x00000001);
mid_ = "";
bitField0_ = (bitField0_ & ~0x00000002);
timestamp_ = 0L;
bitField0_ = (bitField0_ & ~0x00000004);
recall_ = false;
bitField0_ = (bitField0_ & ~0x00000008);
data_ = "";
bitField0_ = (bitField0_ & ~0x00000010);
patchTimestamp_ = 0L;
bitField0_ = (bitField0_ & ~0x00000020);
from_ = "";
bitField0_ = (bitField0_ & ~0x00000040);
binaryMsg_ = com.google.protobuf.ByteString.EMPTY;
bitField0_ = (bitField0_ & ~0x00000080);
mentionAll_ = false;
bitField0_ = (bitField0_ & ~0x00000100);
mentionPids_ = com.google.protobuf.LazyStringArrayList.EMPTY;
bitField0_ = (bitField0_ & ~0x00000200);
patchCode_ = 0L;
bitField0_ = (bitField0_ & ~0x00000400);
patchReason_ = "";
bitField0_ = (bitField0_ & ~0x00000800);
return this;
}
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return com.avos.avoscloud.Messages.internal_static_com_avos_avoscloud_PatchItem_descriptor;
}
public com.avos.avoscloud.Messages.PatchItem getDefaultInstanceForType() {
return com.avos.avoscloud.Messages.PatchItem.getDefaultInstance();
}
public com.avos.avoscloud.Messages.PatchItem build() {
com.avos.avoscloud.Messages.PatchItem result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
public com.avos.avoscloud.Messages.PatchItem buildPartial() {
com.avos.avoscloud.Messages.PatchItem result = new com.avos.avoscloud.Messages.PatchItem(this);
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (((from_bitField0_ & 0x00000001) == 0x00000001)) {
to_bitField0_ |= 0x00000001;
}
result.cid_ = cid_;
if (((from_bitField0_ & 0x00000002) == 0x00000002)) {
to_bitField0_ |= 0x00000002;
}
result.mid_ = mid_;
if (((from_bitField0_ & 0x00000004) == 0x00000004)) {
to_bitField0_ |= 0x00000004;
}
result.timestamp_ = timestamp_;
if (((from_bitField0_ & 0x00000008) == 0x00000008)) {
to_bitField0_ |= 0x00000008;
}
result.recall_ = recall_;
if (((from_bitField0_ & 0x00000010) == 0x00000010)) {
to_bitField0_ |= 0x00000010;
}
result.data_ = data_;
if (((from_bitField0_ & 0x00000020) == 0x00000020)) {
to_bitField0_ |= 0x00000020;
}
result.patchTimestamp_ = patchTimestamp_;
if (((from_bitField0_ & 0x00000040) == 0x00000040)) {
to_bitField0_ |= 0x00000040;
}
result.from_ = from_;
if (((from_bitField0_ & 0x00000080) == 0x00000080)) {
to_bitField0_ |= 0x00000080;
}
result.binaryMsg_ = binaryMsg_;
if (((from_bitField0_ & 0x00000100) == 0x00000100)) {
to_bitField0_ |= 0x00000100;
}
result.mentionAll_ = mentionAll_;
if (((bitField0_ & 0x00000200) == 0x00000200)) {
mentionPids_ = mentionPids_.getUnmodifiableView();
bitField0_ = (bitField0_ & ~0x00000200);
}
result.mentionPids_ = mentionPids_;
if (((from_bitField0_ & 0x00000400) == 0x00000400)) {
to_bitField0_ |= 0x00000200;
}
result.patchCode_ = patchCode_;
if (((from_bitField0_ & 0x00000800) == 0x00000800)) {
to_bitField0_ |= 0x00000400;
}
result.patchReason_ = patchReason_;
result.bitField0_ = to_bitField0_;
onBuilt();
return result;
}
public Builder clone() {
return (Builder) super.clone();
}
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.setField(field, value);
}
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return (Builder) super.clearField(field);
}
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return (Builder) super.clearOneof(oneof);
}
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return (Builder) super.setRepeatedField(field, index, value);
}
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.addRepeatedField(field, value);
}
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.avos.avoscloud.Messages.PatchItem) {
return mergeFrom((com.avos.avoscloud.Messages.PatchItem)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.avos.avoscloud.Messages.PatchItem other) {
if (other == com.avos.avoscloud.Messages.PatchItem.getDefaultInstance()) return this;
if (other.hasCid()) {
bitField0_ |= 0x00000001;
cid_ = other.cid_;
onChanged();
}
if (other.hasMid()) {
bitField0_ |= 0x00000002;
mid_ = other.mid_;
onChanged();
}
if (other.hasTimestamp()) {
setTimestamp(other.getTimestamp());
}
if (other.hasRecall()) {
setRecall(other.getRecall());
}
if (other.hasData()) {
bitField0_ |= 0x00000010;
data_ = other.data_;
onChanged();
}
if (other.hasPatchTimestamp()) {
setPatchTimestamp(other.getPatchTimestamp());
}
if (other.hasFrom()) {
bitField0_ |= 0x00000040;
from_ = other.from_;
onChanged();
}
if (other.hasBinaryMsg()) {
setBinaryMsg(other.getBinaryMsg());
}
if (other.hasMentionAll()) {
setMentionAll(other.getMentionAll());
}
if (!other.mentionPids_.isEmpty()) {
if (mentionPids_.isEmpty()) {
mentionPids_ = other.mentionPids_;
bitField0_ = (bitField0_ & ~0x00000200);
} else {
ensureMentionPidsIsMutable();
mentionPids_.addAll(other.mentionPids_);
}
onChanged();
}
if (other.hasPatchCode()) {
setPatchCode(other.getPatchCode());
}
if (other.hasPatchReason()) {
bitField0_ |= 0x00000800;
patchReason_ = other.patchReason_;
onChanged();
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
public final boolean isInitialized() {
return true;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
com.avos.avoscloud.Messages.PatchItem parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (com.avos.avoscloud.Messages.PatchItem) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
private java.lang.Object cid_ = "";
/**
* optional string cid = 1;
*/
public boolean hasCid() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
* optional string cid = 1;
*/
public java.lang.String getCid() {
java.lang.Object ref = cid_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
cid_ = s;
}
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* optional string cid = 1;
*/
public com.google.protobuf.ByteString
getCidBytes() {
java.lang.Object ref = cid_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
cid_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* optional string cid = 1;
*/
public Builder setCid(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000001;
cid_ = value;
onChanged();
return this;
}
/**
* optional string cid = 1;
*/
public Builder clearCid() {
bitField0_ = (bitField0_ & ~0x00000001);
cid_ = getDefaultInstance().getCid();
onChanged();
return this;
}
/**
* optional string cid = 1;
*/
public Builder setCidBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000001;
cid_ = value;
onChanged();
return this;
}
private java.lang.Object mid_ = "";
/**
* optional string mid = 2;
*/
public boolean hasMid() {
return ((bitField0_ & 0x00000002) == 0x00000002);
}
/**
* optional string mid = 2;
*/
public java.lang.String getMid() {
java.lang.Object ref = mid_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
mid_ = s;
}
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* optional string mid = 2;
*/
public com.google.protobuf.ByteString
getMidBytes() {
java.lang.Object ref = mid_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
mid_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* optional string mid = 2;
*/
public Builder setMid(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000002;
mid_ = value;
onChanged();
return this;
}
/**
* optional string mid = 2;
*/
public Builder clearMid() {
bitField0_ = (bitField0_ & ~0x00000002);
mid_ = getDefaultInstance().getMid();
onChanged();
return this;
}
/**
* optional string mid = 2;
*/
public Builder setMidBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000002;
mid_ = value;
onChanged();
return this;
}
private long timestamp_ ;
/**
* optional int64 timestamp = 3;
*/
public boolean hasTimestamp() {
return ((bitField0_ & 0x00000004) == 0x00000004);
}
/**
* optional int64 timestamp = 3;
*/
public long getTimestamp() {
return timestamp_;
}
/**
* optional int64 timestamp = 3;
*/
public Builder setTimestamp(long value) {
bitField0_ |= 0x00000004;
timestamp_ = value;
onChanged();
return this;
}
/**
* optional int64 timestamp = 3;
*/
public Builder clearTimestamp() {
bitField0_ = (bitField0_ & ~0x00000004);
timestamp_ = 0L;
onChanged();
return this;
}
private boolean recall_ ;
/**
* optional bool recall = 4;
*/
public boolean hasRecall() {
return ((bitField0_ & 0x00000008) == 0x00000008);
}
/**
* optional bool recall = 4;
*/
public boolean getRecall() {
return recall_;
}
/**
* optional bool recall = 4;
*/
public Builder setRecall(boolean value) {
bitField0_ |= 0x00000008;
recall_ = value;
onChanged();
return this;
}
/**
* optional bool recall = 4;
*/
public Builder clearRecall() {
bitField0_ = (bitField0_ & ~0x00000008);
recall_ = false;
onChanged();
return this;
}
private java.lang.Object data_ = "";
/**
* optional string data = 5;
*/
public boolean hasData() {
return ((bitField0_ & 0x00000010) == 0x00000010);
}
/**
* optional string data = 5;
*/
public java.lang.String getData() {
java.lang.Object ref = data_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
data_ = s;
}
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* optional string data = 5;
*/
public com.google.protobuf.ByteString
getDataBytes() {
java.lang.Object ref = data_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
data_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* optional string data = 5;
*/
public Builder setData(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000010;
data_ = value;
onChanged();
return this;
}
/**
* optional string data = 5;
*/
public Builder clearData() {
bitField0_ = (bitField0_ & ~0x00000010);
data_ = getDefaultInstance().getData();
onChanged();
return this;
}
/**
* optional string data = 5;
*/
public Builder setDataBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000010;
data_ = value;
onChanged();
return this;
}
private long patchTimestamp_ ;
/**
* optional int64 patchTimestamp = 6;
*/
public boolean hasPatchTimestamp() {
return ((bitField0_ & 0x00000020) == 0x00000020);
}
/**
* optional int64 patchTimestamp = 6;
*/
public long getPatchTimestamp() {
return patchTimestamp_;
}
/**
* optional int64 patchTimestamp = 6;
*/
public Builder setPatchTimestamp(long value) {
bitField0_ |= 0x00000020;
patchTimestamp_ = value;
onChanged();
return this;
}
/**
* optional int64 patchTimestamp = 6;
*/
public Builder clearPatchTimestamp() {
bitField0_ = (bitField0_ & ~0x00000020);
patchTimestamp_ = 0L;
onChanged();
return this;
}
private java.lang.Object from_ = "";
/**
* optional string from = 7;
*/
public boolean hasFrom() {
return ((bitField0_ & 0x00000040) == 0x00000040);
}
/**
* optional string from = 7;
*/
public java.lang.String getFrom() {
java.lang.Object ref = from_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
from_ = s;
}
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* optional string from = 7;
*/
public com.google.protobuf.ByteString
getFromBytes() {
java.lang.Object ref = from_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
from_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* optional string from = 7;
*/
public Builder setFrom(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000040;
from_ = value;
onChanged();
return this;
}
/**
* optional string from = 7;
*/
public Builder clearFrom() {
bitField0_ = (bitField0_ & ~0x00000040);
from_ = getDefaultInstance().getFrom();
onChanged();
return this;
}
/**
* optional string from = 7;
*/
public Builder setFromBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000040;
from_ = value;
onChanged();
return this;
}
private com.google.protobuf.ByteString binaryMsg_ = com.google.protobuf.ByteString.EMPTY;
/**
* optional bytes binaryMsg = 8;
*/
public boolean hasBinaryMsg() {
return ((bitField0_ & 0x00000080) == 0x00000080);
}
/**
* optional bytes binaryMsg = 8;
*/
public com.google.protobuf.ByteString getBinaryMsg() {
return binaryMsg_;
}
/**
* optional bytes binaryMsg = 8;
*/
public Builder setBinaryMsg(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000080;
binaryMsg_ = value;
onChanged();
return this;
}
/**
* optional bytes binaryMsg = 8;
*/
public Builder clearBinaryMsg() {
bitField0_ = (bitField0_ & ~0x00000080);
binaryMsg_ = getDefaultInstance().getBinaryMsg();
onChanged();
return this;
}
private boolean mentionAll_ ;
/**
* optional bool mentionAll = 9;
*/
public boolean hasMentionAll() {
return ((bitField0_ & 0x00000100) == 0x00000100);
}
/**
* optional bool mentionAll = 9;
*/
public boolean getMentionAll() {
return mentionAll_;
}
/**
* optional bool mentionAll = 9;
*/
public Builder setMentionAll(boolean value) {
bitField0_ |= 0x00000100;
mentionAll_ = value;
onChanged();
return this;
}
/**
* optional bool mentionAll = 9;
*/
public Builder clearMentionAll() {
bitField0_ = (bitField0_ & ~0x00000100);
mentionAll_ = false;
onChanged();
return this;
}
private com.google.protobuf.LazyStringList mentionPids_ = com.google.protobuf.LazyStringArrayList.EMPTY;
private void ensureMentionPidsIsMutable() {
if (!((bitField0_ & 0x00000200) == 0x00000200)) {
mentionPids_ = new com.google.protobuf.LazyStringArrayList(mentionPids_);
bitField0_ |= 0x00000200;
}
}
/**
* repeated string mentionPids = 10;
*/
public com.google.protobuf.ProtocolStringList
getMentionPidsList() {
return mentionPids_.getUnmodifiableView();
}
/**
* repeated string mentionPids = 10;
*/
public int getMentionPidsCount() {
return mentionPids_.size();
}
/**
* repeated string mentionPids = 10;
*/
public java.lang.String getMentionPids(int index) {
return mentionPids_.get(index);
}
/**
* repeated string mentionPids = 10;
*/
public com.google.protobuf.ByteString
getMentionPidsBytes(int index) {
return mentionPids_.getByteString(index);
}
/**
* repeated string mentionPids = 10;
*/
public Builder setMentionPids(
int index, java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
ensureMentionPidsIsMutable();
mentionPids_.set(index, value);
onChanged();
return this;
}
/**
* repeated string mentionPids = 10;
*/
public Builder addMentionPids(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
ensureMentionPidsIsMutable();
mentionPids_.add(value);
onChanged();
return this;
}
/**
* repeated string mentionPids = 10;
*/
public Builder addAllMentionPids(
java.lang.Iterable values) {
ensureMentionPidsIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, mentionPids_);
onChanged();
return this;
}
/**
* repeated string mentionPids = 10;
*/
public Builder clearMentionPids() {
mentionPids_ = com.google.protobuf.LazyStringArrayList.EMPTY;
bitField0_ = (bitField0_ & ~0x00000200);
onChanged();
return this;
}
/**
* repeated string mentionPids = 10;
*/
public Builder addMentionPidsBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
ensureMentionPidsIsMutable();
mentionPids_.add(value);
onChanged();
return this;
}
private long patchCode_ ;
/**
* optional int64 patchCode = 11;
*/
public boolean hasPatchCode() {
return ((bitField0_ & 0x00000400) == 0x00000400);
}
/**
* optional int64 patchCode = 11;
*/
public long getPatchCode() {
return patchCode_;
}
/**
* optional int64 patchCode = 11;
*/
public Builder setPatchCode(long value) {
bitField0_ |= 0x00000400;
patchCode_ = value;
onChanged();
return this;
}
/**
* optional int64 patchCode = 11;
*/
public Builder clearPatchCode() {
bitField0_ = (bitField0_ & ~0x00000400);
patchCode_ = 0L;
onChanged();
return this;
}
private java.lang.Object patchReason_ = "";
/**
* optional string patchReason = 12;
*/
public boolean hasPatchReason() {
return ((bitField0_ & 0x00000800) == 0x00000800);
}
/**
* optional string patchReason = 12;
*/
public java.lang.String getPatchReason() {
java.lang.Object ref = patchReason_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
patchReason_ = s;
}
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* optional string patchReason = 12;
*/
public com.google.protobuf.ByteString
getPatchReasonBytes() {
java.lang.Object ref = patchReason_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
patchReason_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* optional string patchReason = 12;
*/
public Builder setPatchReason(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000800;
patchReason_ = value;
onChanged();
return this;
}
/**
* optional string patchReason = 12;
*/
public Builder clearPatchReason() {
bitField0_ = (bitField0_ & ~0x00000800);
patchReason_ = getDefaultInstance().getPatchReason();
onChanged();
return this;
}
/**
* optional string patchReason = 12;
*/
public Builder setPatchReasonBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000800;
patchReason_ = value;
onChanged();
return this;
}
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:com.avos.avoscloud.PatchItem)
}
// @@protoc_insertion_point(class_scope:com.avos.avoscloud.PatchItem)
private static final com.avos.avoscloud.Messages.PatchItem DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new com.avos.avoscloud.Messages.PatchItem();
}
public static com.avos.avoscloud.Messages.PatchItem getDefaultInstance() {
return DEFAULT_INSTANCE;
}
@java.lang.Deprecated public static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
public PatchItem parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new PatchItem(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
public com.avos.avoscloud.Messages.PatchItem getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface PatchCommandOrBuilder extends
// @@protoc_insertion_point(interface_extends:com.avos.avoscloud.PatchCommand)
com.google.protobuf.MessageOrBuilder {
/**
* repeated .com.avos.avoscloud.PatchItem patches = 1;
*/
java.util.List
getPatchesList();
/**
* repeated .com.avos.avoscloud.PatchItem patches = 1;
*/
com.avos.avoscloud.Messages.PatchItem getPatches(int index);
/**
* repeated .com.avos.avoscloud.PatchItem patches = 1;
*/
int getPatchesCount();
/**
* repeated .com.avos.avoscloud.PatchItem patches = 1;
*/
java.util.List extends com.avos.avoscloud.Messages.PatchItemOrBuilder>
getPatchesOrBuilderList();
/**
* repeated .com.avos.avoscloud.PatchItem patches = 1;
*/
com.avos.avoscloud.Messages.PatchItemOrBuilder getPatchesOrBuilder(
int index);
/**
* optional int64 lastPatchTime = 2;
*/
boolean hasLastPatchTime();
/**
* optional int64 lastPatchTime = 2;
*/
long getLastPatchTime();
}
/**
* Protobuf type {@code com.avos.avoscloud.PatchCommand}
*/
public static final class PatchCommand extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:com.avos.avoscloud.PatchCommand)
PatchCommandOrBuilder {
private static final long serialVersionUID = 0L;
// Use PatchCommand.newBuilder() to construct.
private PatchCommand(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private PatchCommand() {
patches_ = java.util.Collections.emptyList();
lastPatchTime_ = 0L;
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private PatchCommand(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!parseUnknownField(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
case 10: {
if (!((mutable_bitField0_ & 0x00000001) == 0x00000001)) {
patches_ = new java.util.ArrayList();
mutable_bitField0_ |= 0x00000001;
}
patches_.add(
input.readMessage(com.avos.avoscloud.Messages.PatchItem.PARSER, extensionRegistry));
break;
}
case 16: {
bitField0_ |= 0x00000001;
lastPatchTime_ = input.readInt64();
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
if (((mutable_bitField0_ & 0x00000001) == 0x00000001)) {
patches_ = java.util.Collections.unmodifiableList(patches_);
}
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.avos.avoscloud.Messages.internal_static_com_avos_avoscloud_PatchCommand_descriptor;
}
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.avos.avoscloud.Messages.internal_static_com_avos_avoscloud_PatchCommand_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.avos.avoscloud.Messages.PatchCommand.class, com.avos.avoscloud.Messages.PatchCommand.Builder.class);
}
private int bitField0_;
public static final int PATCHES_FIELD_NUMBER = 1;
private java.util.List patches_;
/**
* repeated .com.avos.avoscloud.PatchItem patches = 1;
*/
public java.util.List getPatchesList() {
return patches_;
}
/**
* repeated .com.avos.avoscloud.PatchItem patches = 1;
*/
public java.util.List extends com.avos.avoscloud.Messages.PatchItemOrBuilder>
getPatchesOrBuilderList() {
return patches_;
}
/**
* repeated .com.avos.avoscloud.PatchItem patches = 1;
*/
public int getPatchesCount() {
return patches_.size();
}
/**
* repeated .com.avos.avoscloud.PatchItem patches = 1;
*/
public com.avos.avoscloud.Messages.PatchItem getPatches(int index) {
return patches_.get(index);
}
/**
* repeated .com.avos.avoscloud.PatchItem patches = 1;
*/
public com.avos.avoscloud.Messages.PatchItemOrBuilder getPatchesOrBuilder(
int index) {
return patches_.get(index);
}
public static final int LASTPATCHTIME_FIELD_NUMBER = 2;
private long lastPatchTime_;
/**
* optional int64 lastPatchTime = 2;
*/
public boolean hasLastPatchTime() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
* optional int64 lastPatchTime = 2;
*/
public long getLastPatchTime() {
return lastPatchTime_;
}
private byte memoizedIsInitialized = -1;
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
for (int i = 0; i < patches_.size(); i++) {
output.writeMessage(1, patches_.get(i));
}
if (((bitField0_ & 0x00000001) == 0x00000001)) {
output.writeInt64(2, lastPatchTime_);
}
unknownFields.writeTo(output);
}
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
for (int i = 0; i < patches_.size(); i++) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(1, patches_.get(i));
}
if (((bitField0_ & 0x00000001) == 0x00000001)) {
size += com.google.protobuf.CodedOutputStream
.computeInt64Size(2, lastPatchTime_);
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof com.avos.avoscloud.Messages.PatchCommand)) {
return super.equals(obj);
}
com.avos.avoscloud.Messages.PatchCommand other = (com.avos.avoscloud.Messages.PatchCommand) obj;
boolean result = true;
result = result && getPatchesList()
.equals(other.getPatchesList());
result = result && (hasLastPatchTime() == other.hasLastPatchTime());
if (hasLastPatchTime()) {
result = result && (getLastPatchTime()
== other.getLastPatchTime());
}
result = result && unknownFields.equals(other.unknownFields);
return result;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (getPatchesCount() > 0) {
hash = (37 * hash) + PATCHES_FIELD_NUMBER;
hash = (53 * hash) + getPatchesList().hashCode();
}
if (hasLastPatchTime()) {
hash = (37 * hash) + LASTPATCHTIME_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getLastPatchTime());
}
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static com.avos.avoscloud.Messages.PatchCommand parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.avos.avoscloud.Messages.PatchCommand parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.avos.avoscloud.Messages.PatchCommand parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.avos.avoscloud.Messages.PatchCommand parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.avos.avoscloud.Messages.PatchCommand parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.avos.avoscloud.Messages.PatchCommand parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.avos.avoscloud.Messages.PatchCommand parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.avos.avoscloud.Messages.PatchCommand parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static com.avos.avoscloud.Messages.PatchCommand parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static com.avos.avoscloud.Messages.PatchCommand parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static com.avos.avoscloud.Messages.PatchCommand parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.avos.avoscloud.Messages.PatchCommand parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(com.avos.avoscloud.Messages.PatchCommand prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code com.avos.avoscloud.PatchCommand}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:com.avos.avoscloud.PatchCommand)
com.avos.avoscloud.Messages.PatchCommandOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.avos.avoscloud.Messages.internal_static_com_avos_avoscloud_PatchCommand_descriptor;
}
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.avos.avoscloud.Messages.internal_static_com_avos_avoscloud_PatchCommand_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.avos.avoscloud.Messages.PatchCommand.class, com.avos.avoscloud.Messages.PatchCommand.Builder.class);
}
// Construct using com.avos.avoscloud.Messages.PatchCommand.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
getPatchesFieldBuilder();
}
}
public Builder clear() {
super.clear();
if (patchesBuilder_ == null) {
patches_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000001);
} else {
patchesBuilder_.clear();
}
lastPatchTime_ = 0L;
bitField0_ = (bitField0_ & ~0x00000002);
return this;
}
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return com.avos.avoscloud.Messages.internal_static_com_avos_avoscloud_PatchCommand_descriptor;
}
public com.avos.avoscloud.Messages.PatchCommand getDefaultInstanceForType() {
return com.avos.avoscloud.Messages.PatchCommand.getDefaultInstance();
}
public com.avos.avoscloud.Messages.PatchCommand build() {
com.avos.avoscloud.Messages.PatchCommand result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
public com.avos.avoscloud.Messages.PatchCommand buildPartial() {
com.avos.avoscloud.Messages.PatchCommand result = new com.avos.avoscloud.Messages.PatchCommand(this);
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (patchesBuilder_ == null) {
if (((bitField0_ & 0x00000001) == 0x00000001)) {
patches_ = java.util.Collections.unmodifiableList(patches_);
bitField0_ = (bitField0_ & ~0x00000001);
}
result.patches_ = patches_;
} else {
result.patches_ = patchesBuilder_.build();
}
if (((from_bitField0_ & 0x00000002) == 0x00000002)) {
to_bitField0_ |= 0x00000001;
}
result.lastPatchTime_ = lastPatchTime_;
result.bitField0_ = to_bitField0_;
onBuilt();
return result;
}
public Builder clone() {
return (Builder) super.clone();
}
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.setField(field, value);
}
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return (Builder) super.clearField(field);
}
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return (Builder) super.clearOneof(oneof);
}
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return (Builder) super.setRepeatedField(field, index, value);
}
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.addRepeatedField(field, value);
}
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.avos.avoscloud.Messages.PatchCommand) {
return mergeFrom((com.avos.avoscloud.Messages.PatchCommand)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.avos.avoscloud.Messages.PatchCommand other) {
if (other == com.avos.avoscloud.Messages.PatchCommand.getDefaultInstance()) return this;
if (patchesBuilder_ == null) {
if (!other.patches_.isEmpty()) {
if (patches_.isEmpty()) {
patches_ = other.patches_;
bitField0_ = (bitField0_ & ~0x00000001);
} else {
ensurePatchesIsMutable();
patches_.addAll(other.patches_);
}
onChanged();
}
} else {
if (!other.patches_.isEmpty()) {
if (patchesBuilder_.isEmpty()) {
patchesBuilder_.dispose();
patchesBuilder_ = null;
patches_ = other.patches_;
bitField0_ = (bitField0_ & ~0x00000001);
patchesBuilder_ =
com.google.protobuf.GeneratedMessageV3.alwaysUseFieldBuilders ?
getPatchesFieldBuilder() : null;
} else {
patchesBuilder_.addAllMessages(other.patches_);
}
}
}
if (other.hasLastPatchTime()) {
setLastPatchTime(other.getLastPatchTime());
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
public final boolean isInitialized() {
return true;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
com.avos.avoscloud.Messages.PatchCommand parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (com.avos.avoscloud.Messages.PatchCommand) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
private java.util.List patches_ =
java.util.Collections.emptyList();
private void ensurePatchesIsMutable() {
if (!((bitField0_ & 0x00000001) == 0x00000001)) {
patches_ = new java.util.ArrayList(patches_);
bitField0_ |= 0x00000001;
}
}
private com.google.protobuf.RepeatedFieldBuilderV3<
com.avos.avoscloud.Messages.PatchItem, com.avos.avoscloud.Messages.PatchItem.Builder, com.avos.avoscloud.Messages.PatchItemOrBuilder> patchesBuilder_;
/**
* repeated .com.avos.avoscloud.PatchItem patches = 1;
*/
public java.util.List getPatchesList() {
if (patchesBuilder_ == null) {
return java.util.Collections.unmodifiableList(patches_);
} else {
return patchesBuilder_.getMessageList();
}
}
/**
* repeated .com.avos.avoscloud.PatchItem patches = 1;
*/
public int getPatchesCount() {
if (patchesBuilder_ == null) {
return patches_.size();
} else {
return patchesBuilder_.getCount();
}
}
/**
* repeated .com.avos.avoscloud.PatchItem patches = 1;
*/
public com.avos.avoscloud.Messages.PatchItem getPatches(int index) {
if (patchesBuilder_ == null) {
return patches_.get(index);
} else {
return patchesBuilder_.getMessage(index);
}
}
/**
* repeated .com.avos.avoscloud.PatchItem patches = 1;
*/
public Builder setPatches(
int index, com.avos.avoscloud.Messages.PatchItem value) {
if (patchesBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensurePatchesIsMutable();
patches_.set(index, value);
onChanged();
} else {
patchesBuilder_.setMessage(index, value);
}
return this;
}
/**
* repeated .com.avos.avoscloud.PatchItem patches = 1;
*/
public Builder setPatches(
int index, com.avos.avoscloud.Messages.PatchItem.Builder builderForValue) {
if (patchesBuilder_ == null) {
ensurePatchesIsMutable();
patches_.set(index, builderForValue.build());
onChanged();
} else {
patchesBuilder_.setMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .com.avos.avoscloud.PatchItem patches = 1;
*/
public Builder addPatches(com.avos.avoscloud.Messages.PatchItem value) {
if (patchesBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensurePatchesIsMutable();
patches_.add(value);
onChanged();
} else {
patchesBuilder_.addMessage(value);
}
return this;
}
/**
* repeated .com.avos.avoscloud.PatchItem patches = 1;
*/
public Builder addPatches(
int index, com.avos.avoscloud.Messages.PatchItem value) {
if (patchesBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensurePatchesIsMutable();
patches_.add(index, value);
onChanged();
} else {
patchesBuilder_.addMessage(index, value);
}
return this;
}
/**
* repeated .com.avos.avoscloud.PatchItem patches = 1;
*/
public Builder addPatches(
com.avos.avoscloud.Messages.PatchItem.Builder builderForValue) {
if (patchesBuilder_ == null) {
ensurePatchesIsMutable();
patches_.add(builderForValue.build());
onChanged();
} else {
patchesBuilder_.addMessage(builderForValue.build());
}
return this;
}
/**
* repeated .com.avos.avoscloud.PatchItem patches = 1;
*/
public Builder addPatches(
int index, com.avos.avoscloud.Messages.PatchItem.Builder builderForValue) {
if (patchesBuilder_ == null) {
ensurePatchesIsMutable();
patches_.add(index, builderForValue.build());
onChanged();
} else {
patchesBuilder_.addMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .com.avos.avoscloud.PatchItem patches = 1;
*/
public Builder addAllPatches(
java.lang.Iterable extends com.avos.avoscloud.Messages.PatchItem> values) {
if (patchesBuilder_ == null) {
ensurePatchesIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, patches_);
onChanged();
} else {
patchesBuilder_.addAllMessages(values);
}
return this;
}
/**
* repeated .com.avos.avoscloud.PatchItem patches = 1;
*/
public Builder clearPatches() {
if (patchesBuilder_ == null) {
patches_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000001);
onChanged();
} else {
patchesBuilder_.clear();
}
return this;
}
/**
* repeated .com.avos.avoscloud.PatchItem patches = 1;
*/
public Builder removePatches(int index) {
if (patchesBuilder_ == null) {
ensurePatchesIsMutable();
patches_.remove(index);
onChanged();
} else {
patchesBuilder_.remove(index);
}
return this;
}
/**
* repeated .com.avos.avoscloud.PatchItem patches = 1;
*/
public com.avos.avoscloud.Messages.PatchItem.Builder getPatchesBuilder(
int index) {
return getPatchesFieldBuilder().getBuilder(index);
}
/**
* repeated .com.avos.avoscloud.PatchItem patches = 1;
*/
public com.avos.avoscloud.Messages.PatchItemOrBuilder getPatchesOrBuilder(
int index) {
if (patchesBuilder_ == null) {
return patches_.get(index); } else {
return patchesBuilder_.getMessageOrBuilder(index);
}
}
/**
* repeated .com.avos.avoscloud.PatchItem patches = 1;
*/
public java.util.List extends com.avos.avoscloud.Messages.PatchItemOrBuilder>
getPatchesOrBuilderList() {
if (patchesBuilder_ != null) {
return patchesBuilder_.getMessageOrBuilderList();
} else {
return java.util.Collections.unmodifiableList(patches_);
}
}
/**
* repeated .com.avos.avoscloud.PatchItem patches = 1;
*/
public com.avos.avoscloud.Messages.PatchItem.Builder addPatchesBuilder() {
return getPatchesFieldBuilder().addBuilder(
com.avos.avoscloud.Messages.PatchItem.getDefaultInstance());
}
/**
* repeated .com.avos.avoscloud.PatchItem patches = 1;
*/
public com.avos.avoscloud.Messages.PatchItem.Builder addPatchesBuilder(
int index) {
return getPatchesFieldBuilder().addBuilder(
index, com.avos.avoscloud.Messages.PatchItem.getDefaultInstance());
}
/**
* repeated .com.avos.avoscloud.PatchItem patches = 1;
*/
public java.util.List
getPatchesBuilderList() {
return getPatchesFieldBuilder().getBuilderList();
}
private com.google.protobuf.RepeatedFieldBuilderV3<
com.avos.avoscloud.Messages.PatchItem, com.avos.avoscloud.Messages.PatchItem.Builder, com.avos.avoscloud.Messages.PatchItemOrBuilder>
getPatchesFieldBuilder() {
if (patchesBuilder_ == null) {
patchesBuilder_ = new com.google.protobuf.RepeatedFieldBuilderV3<
com.avos.avoscloud.Messages.PatchItem, com.avos.avoscloud.Messages.PatchItem.Builder, com.avos.avoscloud.Messages.PatchItemOrBuilder>(
patches_,
((bitField0_ & 0x00000001) == 0x00000001),
getParentForChildren(),
isClean());
patches_ = null;
}
return patchesBuilder_;
}
private long lastPatchTime_ ;
/**
* optional int64 lastPatchTime = 2;
*/
public boolean hasLastPatchTime() {
return ((bitField0_ & 0x00000002) == 0x00000002);
}
/**
* optional int64 lastPatchTime = 2;
*/
public long getLastPatchTime() {
return lastPatchTime_;
}
/**
* optional int64 lastPatchTime = 2;
*/
public Builder setLastPatchTime(long value) {
bitField0_ |= 0x00000002;
lastPatchTime_ = value;
onChanged();
return this;
}
/**
* optional int64 lastPatchTime = 2;
*/
public Builder clearLastPatchTime() {
bitField0_ = (bitField0_ & ~0x00000002);
lastPatchTime_ = 0L;
onChanged();
return this;
}
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:com.avos.avoscloud.PatchCommand)
}
// @@protoc_insertion_point(class_scope:com.avos.avoscloud.PatchCommand)
private static final com.avos.avoscloud.Messages.PatchCommand DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new com.avos.avoscloud.Messages.PatchCommand();
}
public static com.avos.avoscloud.Messages.PatchCommand getDefaultInstance() {
return DEFAULT_INSTANCE;
}
@java.lang.Deprecated public static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
public PatchCommand parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new PatchCommand(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
public com.avos.avoscloud.Messages.PatchCommand getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface PubsubCommandOrBuilder extends
// @@protoc_insertion_point(interface_extends:com.avos.avoscloud.PubsubCommand)
com.google.protobuf.MessageOrBuilder {
/**
* optional string cid = 1;
*/
boolean hasCid();
/**
* optional string cid = 1;
*/
java.lang.String getCid();
/**
* optional string cid = 1;
*/
com.google.protobuf.ByteString
getCidBytes();
/**
* repeated string cids = 2;
*/
java.util.List
getCidsList();
/**
* repeated string cids = 2;
*/
int getCidsCount();
/**
* repeated string cids = 2;
*/
java.lang.String getCids(int index);
/**
* repeated string cids = 2;
*/
com.google.protobuf.ByteString
getCidsBytes(int index);
/**
* optional string topic = 3;
*/
boolean hasTopic();
/**
* optional string topic = 3;
*/
java.lang.String getTopic();
/**
* optional string topic = 3;
*/
com.google.protobuf.ByteString
getTopicBytes();
/**
* optional string subtopic = 4;
*/
boolean hasSubtopic();
/**
* optional string subtopic = 4;
*/
java.lang.String getSubtopic();
/**
* optional string subtopic = 4;
*/
com.google.protobuf.ByteString
getSubtopicBytes();
/**
* repeated string topics = 5;
*/
java.util.List
getTopicsList();
/**
* repeated string topics = 5;
*/
int getTopicsCount();
/**
* repeated string topics = 5;
*/
java.lang.String getTopics(int index);
/**
* repeated string topics = 5;
*/
com.google.protobuf.ByteString
getTopicsBytes(int index);
/**
* repeated string subtopics = 6;
*/
java.util.List
getSubtopicsList();
/**
* repeated string subtopics = 6;
*/
int getSubtopicsCount();
/**
* repeated string subtopics = 6;
*/
java.lang.String getSubtopics(int index);
/**
* repeated string subtopics = 6;
*/
com.google.protobuf.ByteString
getSubtopicsBytes(int index);
/**
* optional .com.avos.avoscloud.JsonObjectMessage results = 7;
*/
boolean hasResults();
/**
* optional .com.avos.avoscloud.JsonObjectMessage results = 7;
*/
com.avos.avoscloud.Messages.JsonObjectMessage getResults();
/**
* optional .com.avos.avoscloud.JsonObjectMessage results = 7;
*/
com.avos.avoscloud.Messages.JsonObjectMessageOrBuilder getResultsOrBuilder();
}
/**
* Protobuf type {@code com.avos.avoscloud.PubsubCommand}
*/
public static final class PubsubCommand extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:com.avos.avoscloud.PubsubCommand)
PubsubCommandOrBuilder {
private static final long serialVersionUID = 0L;
// Use PubsubCommand.newBuilder() to construct.
private PubsubCommand(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private PubsubCommand() {
cid_ = "";
cids_ = com.google.protobuf.LazyStringArrayList.EMPTY;
topic_ = "";
subtopic_ = "";
topics_ = com.google.protobuf.LazyStringArrayList.EMPTY;
subtopics_ = com.google.protobuf.LazyStringArrayList.EMPTY;
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private PubsubCommand(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!parseUnknownField(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
case 10: {
com.google.protobuf.ByteString bs = input.readBytes();
bitField0_ |= 0x00000001;
cid_ = bs;
break;
}
case 18: {
com.google.protobuf.ByteString bs = input.readBytes();
if (!((mutable_bitField0_ & 0x00000002) == 0x00000002)) {
cids_ = new com.google.protobuf.LazyStringArrayList();
mutable_bitField0_ |= 0x00000002;
}
cids_.add(bs);
break;
}
case 26: {
com.google.protobuf.ByteString bs = input.readBytes();
bitField0_ |= 0x00000002;
topic_ = bs;
break;
}
case 34: {
com.google.protobuf.ByteString bs = input.readBytes();
bitField0_ |= 0x00000004;
subtopic_ = bs;
break;
}
case 42: {
com.google.protobuf.ByteString bs = input.readBytes();
if (!((mutable_bitField0_ & 0x00000010) == 0x00000010)) {
topics_ = new com.google.protobuf.LazyStringArrayList();
mutable_bitField0_ |= 0x00000010;
}
topics_.add(bs);
break;
}
case 50: {
com.google.protobuf.ByteString bs = input.readBytes();
if (!((mutable_bitField0_ & 0x00000020) == 0x00000020)) {
subtopics_ = new com.google.protobuf.LazyStringArrayList();
mutable_bitField0_ |= 0x00000020;
}
subtopics_.add(bs);
break;
}
case 58: {
com.avos.avoscloud.Messages.JsonObjectMessage.Builder subBuilder = null;
if (((bitField0_ & 0x00000008) == 0x00000008)) {
subBuilder = results_.toBuilder();
}
results_ = input.readMessage(com.avos.avoscloud.Messages.JsonObjectMessage.PARSER, extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(results_);
results_ = subBuilder.buildPartial();
}
bitField0_ |= 0x00000008;
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
if (((mutable_bitField0_ & 0x00000002) == 0x00000002)) {
cids_ = cids_.getUnmodifiableView();
}
if (((mutable_bitField0_ & 0x00000010) == 0x00000010)) {
topics_ = topics_.getUnmodifiableView();
}
if (((mutable_bitField0_ & 0x00000020) == 0x00000020)) {
subtopics_ = subtopics_.getUnmodifiableView();
}
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.avos.avoscloud.Messages.internal_static_com_avos_avoscloud_PubsubCommand_descriptor;
}
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.avos.avoscloud.Messages.internal_static_com_avos_avoscloud_PubsubCommand_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.avos.avoscloud.Messages.PubsubCommand.class, com.avos.avoscloud.Messages.PubsubCommand.Builder.class);
}
private int bitField0_;
public static final int CID_FIELD_NUMBER = 1;
private volatile java.lang.Object cid_;
/**
* optional string cid = 1;
*/
public boolean hasCid() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
* optional string cid = 1;
*/
public java.lang.String getCid() {
java.lang.Object ref = cid_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
cid_ = s;
}
return s;
}
}
/**
* optional string cid = 1;
*/
public com.google.protobuf.ByteString
getCidBytes() {
java.lang.Object ref = cid_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
cid_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int CIDS_FIELD_NUMBER = 2;
private com.google.protobuf.LazyStringList cids_;
/**
* repeated string cids = 2;
*/
public com.google.protobuf.ProtocolStringList
getCidsList() {
return cids_;
}
/**
* repeated string cids = 2;
*/
public int getCidsCount() {
return cids_.size();
}
/**
* repeated string cids = 2;
*/
public java.lang.String getCids(int index) {
return cids_.get(index);
}
/**
* repeated string cids = 2;
*/
public com.google.protobuf.ByteString
getCidsBytes(int index) {
return cids_.getByteString(index);
}
public static final int TOPIC_FIELD_NUMBER = 3;
private volatile java.lang.Object topic_;
/**
* optional string topic = 3;
*/
public boolean hasTopic() {
return ((bitField0_ & 0x00000002) == 0x00000002);
}
/**
* optional string topic = 3;
*/
public java.lang.String getTopic() {
java.lang.Object ref = topic_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
topic_ = s;
}
return s;
}
}
/**
* optional string topic = 3;
*/
public com.google.protobuf.ByteString
getTopicBytes() {
java.lang.Object ref = topic_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
topic_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int SUBTOPIC_FIELD_NUMBER = 4;
private volatile java.lang.Object subtopic_;
/**
* optional string subtopic = 4;
*/
public boolean hasSubtopic() {
return ((bitField0_ & 0x00000004) == 0x00000004);
}
/**
* optional string subtopic = 4;
*/
public java.lang.String getSubtopic() {
java.lang.Object ref = subtopic_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
subtopic_ = s;
}
return s;
}
}
/**
* optional string subtopic = 4;
*/
public com.google.protobuf.ByteString
getSubtopicBytes() {
java.lang.Object ref = subtopic_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
subtopic_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int TOPICS_FIELD_NUMBER = 5;
private com.google.protobuf.LazyStringList topics_;
/**
* repeated string topics = 5;
*/
public com.google.protobuf.ProtocolStringList
getTopicsList() {
return topics_;
}
/**
* repeated string topics = 5;
*/
public int getTopicsCount() {
return topics_.size();
}
/**
* repeated string topics = 5;
*/
public java.lang.String getTopics(int index) {
return topics_.get(index);
}
/**
* repeated string topics = 5;
*/
public com.google.protobuf.ByteString
getTopicsBytes(int index) {
return topics_.getByteString(index);
}
public static final int SUBTOPICS_FIELD_NUMBER = 6;
private com.google.protobuf.LazyStringList subtopics_;
/**
* repeated string subtopics = 6;
*/
public com.google.protobuf.ProtocolStringList
getSubtopicsList() {
return subtopics_;
}
/**
* repeated string subtopics = 6;
*/
public int getSubtopicsCount() {
return subtopics_.size();
}
/**
* repeated string subtopics = 6;
*/
public java.lang.String getSubtopics(int index) {
return subtopics_.get(index);
}
/**
* repeated string subtopics = 6;
*/
public com.google.protobuf.ByteString
getSubtopicsBytes(int index) {
return subtopics_.getByteString(index);
}
public static final int RESULTS_FIELD_NUMBER = 7;
private com.avos.avoscloud.Messages.JsonObjectMessage results_;
/**
* optional .com.avos.avoscloud.JsonObjectMessage results = 7;
*/
public boolean hasResults() {
return ((bitField0_ & 0x00000008) == 0x00000008);
}
/**
* optional .com.avos.avoscloud.JsonObjectMessage results = 7;
*/
public com.avos.avoscloud.Messages.JsonObjectMessage getResults() {
return results_ == null ? com.avos.avoscloud.Messages.JsonObjectMessage.getDefaultInstance() : results_;
}
/**
* optional .com.avos.avoscloud.JsonObjectMessage results = 7;
*/
public com.avos.avoscloud.Messages.JsonObjectMessageOrBuilder getResultsOrBuilder() {
return results_ == null ? com.avos.avoscloud.Messages.JsonObjectMessage.getDefaultInstance() : results_;
}
private byte memoizedIsInitialized = -1;
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
if (hasResults()) {
if (!getResults().isInitialized()) {
memoizedIsInitialized = 0;
return false;
}
}
memoizedIsInitialized = 1;
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (((bitField0_ & 0x00000001) == 0x00000001)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 1, cid_);
}
for (int i = 0; i < cids_.size(); i++) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 2, cids_.getRaw(i));
}
if (((bitField0_ & 0x00000002) == 0x00000002)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 3, topic_);
}
if (((bitField0_ & 0x00000004) == 0x00000004)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 4, subtopic_);
}
for (int i = 0; i < topics_.size(); i++) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 5, topics_.getRaw(i));
}
for (int i = 0; i < subtopics_.size(); i++) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 6, subtopics_.getRaw(i));
}
if (((bitField0_ & 0x00000008) == 0x00000008)) {
output.writeMessage(7, getResults());
}
unknownFields.writeTo(output);
}
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (((bitField0_ & 0x00000001) == 0x00000001)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(1, cid_);
}
{
int dataSize = 0;
for (int i = 0; i < cids_.size(); i++) {
dataSize += computeStringSizeNoTag(cids_.getRaw(i));
}
size += dataSize;
size += 1 * getCidsList().size();
}
if (((bitField0_ & 0x00000002) == 0x00000002)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(3, topic_);
}
if (((bitField0_ & 0x00000004) == 0x00000004)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(4, subtopic_);
}
{
int dataSize = 0;
for (int i = 0; i < topics_.size(); i++) {
dataSize += computeStringSizeNoTag(topics_.getRaw(i));
}
size += dataSize;
size += 1 * getTopicsList().size();
}
{
int dataSize = 0;
for (int i = 0; i < subtopics_.size(); i++) {
dataSize += computeStringSizeNoTag(subtopics_.getRaw(i));
}
size += dataSize;
size += 1 * getSubtopicsList().size();
}
if (((bitField0_ & 0x00000008) == 0x00000008)) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(7, getResults());
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof com.avos.avoscloud.Messages.PubsubCommand)) {
return super.equals(obj);
}
com.avos.avoscloud.Messages.PubsubCommand other = (com.avos.avoscloud.Messages.PubsubCommand) obj;
boolean result = true;
result = result && (hasCid() == other.hasCid());
if (hasCid()) {
result = result && getCid()
.equals(other.getCid());
}
result = result && getCidsList()
.equals(other.getCidsList());
result = result && (hasTopic() == other.hasTopic());
if (hasTopic()) {
result = result && getTopic()
.equals(other.getTopic());
}
result = result && (hasSubtopic() == other.hasSubtopic());
if (hasSubtopic()) {
result = result && getSubtopic()
.equals(other.getSubtopic());
}
result = result && getTopicsList()
.equals(other.getTopicsList());
result = result && getSubtopicsList()
.equals(other.getSubtopicsList());
result = result && (hasResults() == other.hasResults());
if (hasResults()) {
result = result && getResults()
.equals(other.getResults());
}
result = result && unknownFields.equals(other.unknownFields);
return result;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (hasCid()) {
hash = (37 * hash) + CID_FIELD_NUMBER;
hash = (53 * hash) + getCid().hashCode();
}
if (getCidsCount() > 0) {
hash = (37 * hash) + CIDS_FIELD_NUMBER;
hash = (53 * hash) + getCidsList().hashCode();
}
if (hasTopic()) {
hash = (37 * hash) + TOPIC_FIELD_NUMBER;
hash = (53 * hash) + getTopic().hashCode();
}
if (hasSubtopic()) {
hash = (37 * hash) + SUBTOPIC_FIELD_NUMBER;
hash = (53 * hash) + getSubtopic().hashCode();
}
if (getTopicsCount() > 0) {
hash = (37 * hash) + TOPICS_FIELD_NUMBER;
hash = (53 * hash) + getTopicsList().hashCode();
}
if (getSubtopicsCount() > 0) {
hash = (37 * hash) + SUBTOPICS_FIELD_NUMBER;
hash = (53 * hash) + getSubtopicsList().hashCode();
}
if (hasResults()) {
hash = (37 * hash) + RESULTS_FIELD_NUMBER;
hash = (53 * hash) + getResults().hashCode();
}
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static com.avos.avoscloud.Messages.PubsubCommand parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.avos.avoscloud.Messages.PubsubCommand parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.avos.avoscloud.Messages.PubsubCommand parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.avos.avoscloud.Messages.PubsubCommand parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.avos.avoscloud.Messages.PubsubCommand parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.avos.avoscloud.Messages.PubsubCommand parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.avos.avoscloud.Messages.PubsubCommand parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.avos.avoscloud.Messages.PubsubCommand parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static com.avos.avoscloud.Messages.PubsubCommand parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static com.avos.avoscloud.Messages.PubsubCommand parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static com.avos.avoscloud.Messages.PubsubCommand parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.avos.avoscloud.Messages.PubsubCommand parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(com.avos.avoscloud.Messages.PubsubCommand prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code com.avos.avoscloud.PubsubCommand}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:com.avos.avoscloud.PubsubCommand)
com.avos.avoscloud.Messages.PubsubCommandOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.avos.avoscloud.Messages.internal_static_com_avos_avoscloud_PubsubCommand_descriptor;
}
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.avos.avoscloud.Messages.internal_static_com_avos_avoscloud_PubsubCommand_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.avos.avoscloud.Messages.PubsubCommand.class, com.avos.avoscloud.Messages.PubsubCommand.Builder.class);
}
// Construct using com.avos.avoscloud.Messages.PubsubCommand.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
getResultsFieldBuilder();
}
}
public Builder clear() {
super.clear();
cid_ = "";
bitField0_ = (bitField0_ & ~0x00000001);
cids_ = com.google.protobuf.LazyStringArrayList.EMPTY;
bitField0_ = (bitField0_ & ~0x00000002);
topic_ = "";
bitField0_ = (bitField0_ & ~0x00000004);
subtopic_ = "";
bitField0_ = (bitField0_ & ~0x00000008);
topics_ = com.google.protobuf.LazyStringArrayList.EMPTY;
bitField0_ = (bitField0_ & ~0x00000010);
subtopics_ = com.google.protobuf.LazyStringArrayList.EMPTY;
bitField0_ = (bitField0_ & ~0x00000020);
if (resultsBuilder_ == null) {
results_ = null;
} else {
resultsBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000040);
return this;
}
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return com.avos.avoscloud.Messages.internal_static_com_avos_avoscloud_PubsubCommand_descriptor;
}
public com.avos.avoscloud.Messages.PubsubCommand getDefaultInstanceForType() {
return com.avos.avoscloud.Messages.PubsubCommand.getDefaultInstance();
}
public com.avos.avoscloud.Messages.PubsubCommand build() {
com.avos.avoscloud.Messages.PubsubCommand result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
public com.avos.avoscloud.Messages.PubsubCommand buildPartial() {
com.avos.avoscloud.Messages.PubsubCommand result = new com.avos.avoscloud.Messages.PubsubCommand(this);
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (((from_bitField0_ & 0x00000001) == 0x00000001)) {
to_bitField0_ |= 0x00000001;
}
result.cid_ = cid_;
if (((bitField0_ & 0x00000002) == 0x00000002)) {
cids_ = cids_.getUnmodifiableView();
bitField0_ = (bitField0_ & ~0x00000002);
}
result.cids_ = cids_;
if (((from_bitField0_ & 0x00000004) == 0x00000004)) {
to_bitField0_ |= 0x00000002;
}
result.topic_ = topic_;
if (((from_bitField0_ & 0x00000008) == 0x00000008)) {
to_bitField0_ |= 0x00000004;
}
result.subtopic_ = subtopic_;
if (((bitField0_ & 0x00000010) == 0x00000010)) {
topics_ = topics_.getUnmodifiableView();
bitField0_ = (bitField0_ & ~0x00000010);
}
result.topics_ = topics_;
if (((bitField0_ & 0x00000020) == 0x00000020)) {
subtopics_ = subtopics_.getUnmodifiableView();
bitField0_ = (bitField0_ & ~0x00000020);
}
result.subtopics_ = subtopics_;
if (((from_bitField0_ & 0x00000040) == 0x00000040)) {
to_bitField0_ |= 0x00000008;
}
if (resultsBuilder_ == null) {
result.results_ = results_;
} else {
result.results_ = resultsBuilder_.build();
}
result.bitField0_ = to_bitField0_;
onBuilt();
return result;
}
public Builder clone() {
return (Builder) super.clone();
}
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.setField(field, value);
}
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return (Builder) super.clearField(field);
}
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return (Builder) super.clearOneof(oneof);
}
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return (Builder) super.setRepeatedField(field, index, value);
}
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.addRepeatedField(field, value);
}
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.avos.avoscloud.Messages.PubsubCommand) {
return mergeFrom((com.avos.avoscloud.Messages.PubsubCommand)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.avos.avoscloud.Messages.PubsubCommand other) {
if (other == com.avos.avoscloud.Messages.PubsubCommand.getDefaultInstance()) return this;
if (other.hasCid()) {
bitField0_ |= 0x00000001;
cid_ = other.cid_;
onChanged();
}
if (!other.cids_.isEmpty()) {
if (cids_.isEmpty()) {
cids_ = other.cids_;
bitField0_ = (bitField0_ & ~0x00000002);
} else {
ensureCidsIsMutable();
cids_.addAll(other.cids_);
}
onChanged();
}
if (other.hasTopic()) {
bitField0_ |= 0x00000004;
topic_ = other.topic_;
onChanged();
}
if (other.hasSubtopic()) {
bitField0_ |= 0x00000008;
subtopic_ = other.subtopic_;
onChanged();
}
if (!other.topics_.isEmpty()) {
if (topics_.isEmpty()) {
topics_ = other.topics_;
bitField0_ = (bitField0_ & ~0x00000010);
} else {
ensureTopicsIsMutable();
topics_.addAll(other.topics_);
}
onChanged();
}
if (!other.subtopics_.isEmpty()) {
if (subtopics_.isEmpty()) {
subtopics_ = other.subtopics_;
bitField0_ = (bitField0_ & ~0x00000020);
} else {
ensureSubtopicsIsMutable();
subtopics_.addAll(other.subtopics_);
}
onChanged();
}
if (other.hasResults()) {
mergeResults(other.getResults());
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
public final boolean isInitialized() {
if (hasResults()) {
if (!getResults().isInitialized()) {
return false;
}
}
return true;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
com.avos.avoscloud.Messages.PubsubCommand parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (com.avos.avoscloud.Messages.PubsubCommand) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
private java.lang.Object cid_ = "";
/**
* optional string cid = 1;
*/
public boolean hasCid() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
* optional string cid = 1;
*/
public java.lang.String getCid() {
java.lang.Object ref = cid_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
cid_ = s;
}
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* optional string cid = 1;
*/
public com.google.protobuf.ByteString
getCidBytes() {
java.lang.Object ref = cid_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
cid_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* optional string cid = 1;
*/
public Builder setCid(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000001;
cid_ = value;
onChanged();
return this;
}
/**
* optional string cid = 1;
*/
public Builder clearCid() {
bitField0_ = (bitField0_ & ~0x00000001);
cid_ = getDefaultInstance().getCid();
onChanged();
return this;
}
/**
* optional string cid = 1;
*/
public Builder setCidBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000001;
cid_ = value;
onChanged();
return this;
}
private com.google.protobuf.LazyStringList cids_ = com.google.protobuf.LazyStringArrayList.EMPTY;
private void ensureCidsIsMutable() {
if (!((bitField0_ & 0x00000002) == 0x00000002)) {
cids_ = new com.google.protobuf.LazyStringArrayList(cids_);
bitField0_ |= 0x00000002;
}
}
/**
* repeated string cids = 2;
*/
public com.google.protobuf.ProtocolStringList
getCidsList() {
return cids_.getUnmodifiableView();
}
/**
* repeated string cids = 2;
*/
public int getCidsCount() {
return cids_.size();
}
/**
* repeated string cids = 2;
*/
public java.lang.String getCids(int index) {
return cids_.get(index);
}
/**
* repeated string cids = 2;
*/
public com.google.protobuf.ByteString
getCidsBytes(int index) {
return cids_.getByteString(index);
}
/**
* repeated string cids = 2;
*/
public Builder setCids(
int index, java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
ensureCidsIsMutable();
cids_.set(index, value);
onChanged();
return this;
}
/**
* repeated string cids = 2;
*/
public Builder addCids(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
ensureCidsIsMutable();
cids_.add(value);
onChanged();
return this;
}
/**
* repeated string cids = 2;
*/
public Builder addAllCids(
java.lang.Iterable values) {
ensureCidsIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, cids_);
onChanged();
return this;
}
/**
* repeated string cids = 2;
*/
public Builder clearCids() {
cids_ = com.google.protobuf.LazyStringArrayList.EMPTY;
bitField0_ = (bitField0_ & ~0x00000002);
onChanged();
return this;
}
/**
* repeated string cids = 2;
*/
public Builder addCidsBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
ensureCidsIsMutable();
cids_.add(value);
onChanged();
return this;
}
private java.lang.Object topic_ = "";
/**
* optional string topic = 3;
*/
public boolean hasTopic() {
return ((bitField0_ & 0x00000004) == 0x00000004);
}
/**
* optional string topic = 3;
*/
public java.lang.String getTopic() {
java.lang.Object ref = topic_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
topic_ = s;
}
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* optional string topic = 3;
*/
public com.google.protobuf.ByteString
getTopicBytes() {
java.lang.Object ref = topic_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
topic_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* optional string topic = 3;
*/
public Builder setTopic(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000004;
topic_ = value;
onChanged();
return this;
}
/**
* optional string topic = 3;
*/
public Builder clearTopic() {
bitField0_ = (bitField0_ & ~0x00000004);
topic_ = getDefaultInstance().getTopic();
onChanged();
return this;
}
/**
* optional string topic = 3;
*/
public Builder setTopicBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000004;
topic_ = value;
onChanged();
return this;
}
private java.lang.Object subtopic_ = "";
/**
* optional string subtopic = 4;
*/
public boolean hasSubtopic() {
return ((bitField0_ & 0x00000008) == 0x00000008);
}
/**
* optional string subtopic = 4;
*/
public java.lang.String getSubtopic() {
java.lang.Object ref = subtopic_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
subtopic_ = s;
}
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* optional string subtopic = 4;
*/
public com.google.protobuf.ByteString
getSubtopicBytes() {
java.lang.Object ref = subtopic_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
subtopic_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* optional string subtopic = 4;
*/
public Builder setSubtopic(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000008;
subtopic_ = value;
onChanged();
return this;
}
/**
* optional string subtopic = 4;
*/
public Builder clearSubtopic() {
bitField0_ = (bitField0_ & ~0x00000008);
subtopic_ = getDefaultInstance().getSubtopic();
onChanged();
return this;
}
/**
* optional string subtopic = 4;
*/
public Builder setSubtopicBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000008;
subtopic_ = value;
onChanged();
return this;
}
private com.google.protobuf.LazyStringList topics_ = com.google.protobuf.LazyStringArrayList.EMPTY;
private void ensureTopicsIsMutable() {
if (!((bitField0_ & 0x00000010) == 0x00000010)) {
topics_ = new com.google.protobuf.LazyStringArrayList(topics_);
bitField0_ |= 0x00000010;
}
}
/**
* repeated string topics = 5;
*/
public com.google.protobuf.ProtocolStringList
getTopicsList() {
return topics_.getUnmodifiableView();
}
/**
* repeated string topics = 5;
*/
public int getTopicsCount() {
return topics_.size();
}
/**
* repeated string topics = 5;
*/
public java.lang.String getTopics(int index) {
return topics_.get(index);
}
/**
* repeated string topics = 5;
*/
public com.google.protobuf.ByteString
getTopicsBytes(int index) {
return topics_.getByteString(index);
}
/**
* repeated string topics = 5;
*/
public Builder setTopics(
int index, java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
ensureTopicsIsMutable();
topics_.set(index, value);
onChanged();
return this;
}
/**
* repeated string topics = 5;
*/
public Builder addTopics(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
ensureTopicsIsMutable();
topics_.add(value);
onChanged();
return this;
}
/**
* repeated string topics = 5;
*/
public Builder addAllTopics(
java.lang.Iterable values) {
ensureTopicsIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, topics_);
onChanged();
return this;
}
/**
* repeated string topics = 5;
*/
public Builder clearTopics() {
topics_ = com.google.protobuf.LazyStringArrayList.EMPTY;
bitField0_ = (bitField0_ & ~0x00000010);
onChanged();
return this;
}
/**
* repeated string topics = 5;
*/
public Builder addTopicsBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
ensureTopicsIsMutable();
topics_.add(value);
onChanged();
return this;
}
private com.google.protobuf.LazyStringList subtopics_ = com.google.protobuf.LazyStringArrayList.EMPTY;
private void ensureSubtopicsIsMutable() {
if (!((bitField0_ & 0x00000020) == 0x00000020)) {
subtopics_ = new com.google.protobuf.LazyStringArrayList(subtopics_);
bitField0_ |= 0x00000020;
}
}
/**
* repeated string subtopics = 6;
*/
public com.google.protobuf.ProtocolStringList
getSubtopicsList() {
return subtopics_.getUnmodifiableView();
}
/**
* repeated string subtopics = 6;
*/
public int getSubtopicsCount() {
return subtopics_.size();
}
/**
* repeated string subtopics = 6;
*/
public java.lang.String getSubtopics(int index) {
return subtopics_.get(index);
}
/**
* repeated string subtopics = 6;
*/
public com.google.protobuf.ByteString
getSubtopicsBytes(int index) {
return subtopics_.getByteString(index);
}
/**
* repeated string subtopics = 6;
*/
public Builder setSubtopics(
int index, java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
ensureSubtopicsIsMutable();
subtopics_.set(index, value);
onChanged();
return this;
}
/**
* repeated string subtopics = 6;
*/
public Builder addSubtopics(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
ensureSubtopicsIsMutable();
subtopics_.add(value);
onChanged();
return this;
}
/**
* repeated string subtopics = 6;
*/
public Builder addAllSubtopics(
java.lang.Iterable values) {
ensureSubtopicsIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, subtopics_);
onChanged();
return this;
}
/**
* repeated string subtopics = 6;
*/
public Builder clearSubtopics() {
subtopics_ = com.google.protobuf.LazyStringArrayList.EMPTY;
bitField0_ = (bitField0_ & ~0x00000020);
onChanged();
return this;
}
/**
* repeated string subtopics = 6;
*/
public Builder addSubtopicsBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
ensureSubtopicsIsMutable();
subtopics_.add(value);
onChanged();
return this;
}
private com.avos.avoscloud.Messages.JsonObjectMessage results_ = null;
private com.google.protobuf.SingleFieldBuilderV3<
com.avos.avoscloud.Messages.JsonObjectMessage, com.avos.avoscloud.Messages.JsonObjectMessage.Builder, com.avos.avoscloud.Messages.JsonObjectMessageOrBuilder> resultsBuilder_;
/**
* optional .com.avos.avoscloud.JsonObjectMessage results = 7;
*/
public boolean hasResults() {
return ((bitField0_ & 0x00000040) == 0x00000040);
}
/**
* optional .com.avos.avoscloud.JsonObjectMessage results = 7;
*/
public com.avos.avoscloud.Messages.JsonObjectMessage getResults() {
if (resultsBuilder_ == null) {
return results_ == null ? com.avos.avoscloud.Messages.JsonObjectMessage.getDefaultInstance() : results_;
} else {
return resultsBuilder_.getMessage();
}
}
/**
* optional .com.avos.avoscloud.JsonObjectMessage results = 7;
*/
public Builder setResults(com.avos.avoscloud.Messages.JsonObjectMessage value) {
if (resultsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
results_ = value;
onChanged();
} else {
resultsBuilder_.setMessage(value);
}
bitField0_ |= 0x00000040;
return this;
}
/**
* optional .com.avos.avoscloud.JsonObjectMessage results = 7;
*/
public Builder setResults(
com.avos.avoscloud.Messages.JsonObjectMessage.Builder builderForValue) {
if (resultsBuilder_ == null) {
results_ = builderForValue.build();
onChanged();
} else {
resultsBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00000040;
return this;
}
/**
* optional .com.avos.avoscloud.JsonObjectMessage results = 7;
*/
public Builder mergeResults(com.avos.avoscloud.Messages.JsonObjectMessage value) {
if (resultsBuilder_ == null) {
if (((bitField0_ & 0x00000040) == 0x00000040) &&
results_ != null &&
results_ != com.avos.avoscloud.Messages.JsonObjectMessage.getDefaultInstance()) {
results_ =
com.avos.avoscloud.Messages.JsonObjectMessage.newBuilder(results_).mergeFrom(value).buildPartial();
} else {
results_ = value;
}
onChanged();
} else {
resultsBuilder_.mergeFrom(value);
}
bitField0_ |= 0x00000040;
return this;
}
/**
* optional .com.avos.avoscloud.JsonObjectMessage results = 7;
*/
public Builder clearResults() {
if (resultsBuilder_ == null) {
results_ = null;
onChanged();
} else {
resultsBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000040);
return this;
}
/**
* optional .com.avos.avoscloud.JsonObjectMessage results = 7;
*/
public com.avos.avoscloud.Messages.JsonObjectMessage.Builder getResultsBuilder() {
bitField0_ |= 0x00000040;
onChanged();
return getResultsFieldBuilder().getBuilder();
}
/**
* optional .com.avos.avoscloud.JsonObjectMessage results = 7;
*/
public com.avos.avoscloud.Messages.JsonObjectMessageOrBuilder getResultsOrBuilder() {
if (resultsBuilder_ != null) {
return resultsBuilder_.getMessageOrBuilder();
} else {
return results_ == null ?
com.avos.avoscloud.Messages.JsonObjectMessage.getDefaultInstance() : results_;
}
}
/**
* optional .com.avos.avoscloud.JsonObjectMessage results = 7;
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.avos.avoscloud.Messages.JsonObjectMessage, com.avos.avoscloud.Messages.JsonObjectMessage.Builder, com.avos.avoscloud.Messages.JsonObjectMessageOrBuilder>
getResultsFieldBuilder() {
if (resultsBuilder_ == null) {
resultsBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
com.avos.avoscloud.Messages.JsonObjectMessage, com.avos.avoscloud.Messages.JsonObjectMessage.Builder, com.avos.avoscloud.Messages.JsonObjectMessageOrBuilder>(
getResults(),
getParentForChildren(),
isClean());
results_ = null;
}
return resultsBuilder_;
}
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:com.avos.avoscloud.PubsubCommand)
}
// @@protoc_insertion_point(class_scope:com.avos.avoscloud.PubsubCommand)
private static final com.avos.avoscloud.Messages.PubsubCommand DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new com.avos.avoscloud.Messages.PubsubCommand();
}
public static com.avos.avoscloud.Messages.PubsubCommand getDefaultInstance() {
return DEFAULT_INSTANCE;
}
@java.lang.Deprecated public static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
public PubsubCommand parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new PubsubCommand(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
public com.avos.avoscloud.Messages.PubsubCommand getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface BlacklistCommandOrBuilder extends
// @@protoc_insertion_point(interface_extends:com.avos.avoscloud.BlacklistCommand)
com.google.protobuf.MessageOrBuilder {
/**
* optional string srcCid = 1;
*/
boolean hasSrcCid();
/**
* optional string srcCid = 1;
*/
java.lang.String getSrcCid();
/**
* optional string srcCid = 1;
*/
com.google.protobuf.ByteString
getSrcCidBytes();
/**
* repeated string toPids = 2;
*/
java.util.List
getToPidsList();
/**
* repeated string toPids = 2;
*/
int getToPidsCount();
/**
* repeated string toPids = 2;
*/
java.lang.String getToPids(int index);
/**
* repeated string toPids = 2;
*/
com.google.protobuf.ByteString
getToPidsBytes(int index);
/**
* optional string srcPid = 3;
*/
boolean hasSrcPid();
/**
* optional string srcPid = 3;
*/
java.lang.String getSrcPid();
/**
* optional string srcPid = 3;
*/
com.google.protobuf.ByteString
getSrcPidBytes();
/**
* repeated string toCids = 4;
*/
java.util.List
getToCidsList();
/**
* repeated string toCids = 4;
*/
int getToCidsCount();
/**
* repeated string toCids = 4;
*/
java.lang.String getToCids(int index);
/**
* repeated string toCids = 4;
*/
com.google.protobuf.ByteString
getToCidsBytes(int index);
/**
* optional int32 limit = 5;
*/
boolean hasLimit();
/**
* optional int32 limit = 5;
*/
int getLimit();
/**
* optional string next = 6;
*/
boolean hasNext();
/**
* optional string next = 6;
*/
java.lang.String getNext();
/**
* optional string next = 6;
*/
com.google.protobuf.ByteString
getNextBytes();
/**
* repeated string blockedPids = 8;
*/
java.util.List
getBlockedPidsList();
/**
* repeated string blockedPids = 8;
*/
int getBlockedPidsCount();
/**
* repeated string blockedPids = 8;
*/
java.lang.String getBlockedPids(int index);
/**
* repeated string blockedPids = 8;
*/
com.google.protobuf.ByteString
getBlockedPidsBytes(int index);
/**
* repeated string blockedCids = 9;
*/
java.util.List
getBlockedCidsList();
/**
* repeated string blockedCids = 9;
*/
int getBlockedCidsCount();
/**
* repeated string blockedCids = 9;
*/
java.lang.String getBlockedCids(int index);
/**
* repeated string blockedCids = 9;
*/
com.google.protobuf.ByteString
getBlockedCidsBytes(int index);
/**
* repeated string allowedPids = 10;
*/
java.util.List
getAllowedPidsList();
/**
* repeated string allowedPids = 10;
*/
int getAllowedPidsCount();
/**
* repeated string allowedPids = 10;
*/
java.lang.String getAllowedPids(int index);
/**
* repeated string allowedPids = 10;
*/
com.google.protobuf.ByteString
getAllowedPidsBytes(int index);
/**
* repeated .com.avos.avoscloud.ErrorCommand failedPids = 11;
*/
java.util.List
getFailedPidsList();
/**
* repeated .com.avos.avoscloud.ErrorCommand failedPids = 11;
*/
com.avos.avoscloud.Messages.ErrorCommand getFailedPids(int index);
/**
* repeated .com.avos.avoscloud.ErrorCommand failedPids = 11;
*/
int getFailedPidsCount();
/**
* repeated .com.avos.avoscloud.ErrorCommand failedPids = 11;
*/
java.util.List extends com.avos.avoscloud.Messages.ErrorCommandOrBuilder>
getFailedPidsOrBuilderList();
/**
* repeated .com.avos.avoscloud.ErrorCommand failedPids = 11;
*/
com.avos.avoscloud.Messages.ErrorCommandOrBuilder getFailedPidsOrBuilder(
int index);
/**
* optional int64 t = 12;
*/
boolean hasT();
/**
* optional int64 t = 12;
*/
long getT();
/**
* optional string n = 13;
*/
boolean hasN();
/**
* optional string n = 13;
*/
java.lang.String getN();
/**
* optional string n = 13;
*/
com.google.protobuf.ByteString
getNBytes();
/**
* optional string s = 14;
*/
boolean hasS();
/**
* optional string s = 14;
*/
java.lang.String getS();
/**
* optional string s = 14;
*/
com.google.protobuf.ByteString
getSBytes();
}
/**
* Protobuf type {@code com.avos.avoscloud.BlacklistCommand}
*/
public static final class BlacklistCommand extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:com.avos.avoscloud.BlacklistCommand)
BlacklistCommandOrBuilder {
private static final long serialVersionUID = 0L;
// Use BlacklistCommand.newBuilder() to construct.
private BlacklistCommand(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private BlacklistCommand() {
srcCid_ = "";
toPids_ = com.google.protobuf.LazyStringArrayList.EMPTY;
srcPid_ = "";
toCids_ = com.google.protobuf.LazyStringArrayList.EMPTY;
limit_ = 0;
next_ = "";
blockedPids_ = com.google.protobuf.LazyStringArrayList.EMPTY;
blockedCids_ = com.google.protobuf.LazyStringArrayList.EMPTY;
allowedPids_ = com.google.protobuf.LazyStringArrayList.EMPTY;
failedPids_ = java.util.Collections.emptyList();
t_ = 0L;
n_ = "";
s_ = "";
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private BlacklistCommand(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!parseUnknownField(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
case 10: {
com.google.protobuf.ByteString bs = input.readBytes();
bitField0_ |= 0x00000001;
srcCid_ = bs;
break;
}
case 18: {
com.google.protobuf.ByteString bs = input.readBytes();
if (!((mutable_bitField0_ & 0x00000002) == 0x00000002)) {
toPids_ = new com.google.protobuf.LazyStringArrayList();
mutable_bitField0_ |= 0x00000002;
}
toPids_.add(bs);
break;
}
case 26: {
com.google.protobuf.ByteString bs = input.readBytes();
bitField0_ |= 0x00000002;
srcPid_ = bs;
break;
}
case 34: {
com.google.protobuf.ByteString bs = input.readBytes();
if (!((mutable_bitField0_ & 0x00000008) == 0x00000008)) {
toCids_ = new com.google.protobuf.LazyStringArrayList();
mutable_bitField0_ |= 0x00000008;
}
toCids_.add(bs);
break;
}
case 40: {
bitField0_ |= 0x00000004;
limit_ = input.readInt32();
break;
}
case 50: {
com.google.protobuf.ByteString bs = input.readBytes();
bitField0_ |= 0x00000008;
next_ = bs;
break;
}
case 66: {
com.google.protobuf.ByteString bs = input.readBytes();
if (!((mutable_bitField0_ & 0x00000040) == 0x00000040)) {
blockedPids_ = new com.google.protobuf.LazyStringArrayList();
mutable_bitField0_ |= 0x00000040;
}
blockedPids_.add(bs);
break;
}
case 74: {
com.google.protobuf.ByteString bs = input.readBytes();
if (!((mutable_bitField0_ & 0x00000080) == 0x00000080)) {
blockedCids_ = new com.google.protobuf.LazyStringArrayList();
mutable_bitField0_ |= 0x00000080;
}
blockedCids_.add(bs);
break;
}
case 82: {
com.google.protobuf.ByteString bs = input.readBytes();
if (!((mutable_bitField0_ & 0x00000100) == 0x00000100)) {
allowedPids_ = new com.google.protobuf.LazyStringArrayList();
mutable_bitField0_ |= 0x00000100;
}
allowedPids_.add(bs);
break;
}
case 90: {
if (!((mutable_bitField0_ & 0x00000200) == 0x00000200)) {
failedPids_ = new java.util.ArrayList();
mutable_bitField0_ |= 0x00000200;
}
failedPids_.add(
input.readMessage(com.avos.avoscloud.Messages.ErrorCommand.PARSER, extensionRegistry));
break;
}
case 96: {
bitField0_ |= 0x00000010;
t_ = input.readInt64();
break;
}
case 106: {
com.google.protobuf.ByteString bs = input.readBytes();
bitField0_ |= 0x00000020;
n_ = bs;
break;
}
case 114: {
com.google.protobuf.ByteString bs = input.readBytes();
bitField0_ |= 0x00000040;
s_ = bs;
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
if (((mutable_bitField0_ & 0x00000002) == 0x00000002)) {
toPids_ = toPids_.getUnmodifiableView();
}
if (((mutable_bitField0_ & 0x00000008) == 0x00000008)) {
toCids_ = toCids_.getUnmodifiableView();
}
if (((mutable_bitField0_ & 0x00000040) == 0x00000040)) {
blockedPids_ = blockedPids_.getUnmodifiableView();
}
if (((mutable_bitField0_ & 0x00000080) == 0x00000080)) {
blockedCids_ = blockedCids_.getUnmodifiableView();
}
if (((mutable_bitField0_ & 0x00000100) == 0x00000100)) {
allowedPids_ = allowedPids_.getUnmodifiableView();
}
if (((mutable_bitField0_ & 0x00000200) == 0x00000200)) {
failedPids_ = java.util.Collections.unmodifiableList(failedPids_);
}
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.avos.avoscloud.Messages.internal_static_com_avos_avoscloud_BlacklistCommand_descriptor;
}
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.avos.avoscloud.Messages.internal_static_com_avos_avoscloud_BlacklistCommand_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.avos.avoscloud.Messages.BlacklistCommand.class, com.avos.avoscloud.Messages.BlacklistCommand.Builder.class);
}
private int bitField0_;
public static final int SRCCID_FIELD_NUMBER = 1;
private volatile java.lang.Object srcCid_;
/**
* optional string srcCid = 1;
*/
public boolean hasSrcCid() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
* optional string srcCid = 1;
*/
public java.lang.String getSrcCid() {
java.lang.Object ref = srcCid_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
srcCid_ = s;
}
return s;
}
}
/**
* optional string srcCid = 1;
*/
public com.google.protobuf.ByteString
getSrcCidBytes() {
java.lang.Object ref = srcCid_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
srcCid_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int TOPIDS_FIELD_NUMBER = 2;
private com.google.protobuf.LazyStringList toPids_;
/**
* repeated string toPids = 2;
*/
public com.google.protobuf.ProtocolStringList
getToPidsList() {
return toPids_;
}
/**
* repeated string toPids = 2;
*/
public int getToPidsCount() {
return toPids_.size();
}
/**
* repeated string toPids = 2;
*/
public java.lang.String getToPids(int index) {
return toPids_.get(index);
}
/**
* repeated string toPids = 2;
*/
public com.google.protobuf.ByteString
getToPidsBytes(int index) {
return toPids_.getByteString(index);
}
public static final int SRCPID_FIELD_NUMBER = 3;
private volatile java.lang.Object srcPid_;
/**
* optional string srcPid = 3;
*/
public boolean hasSrcPid() {
return ((bitField0_ & 0x00000002) == 0x00000002);
}
/**
* optional string srcPid = 3;
*/
public java.lang.String getSrcPid() {
java.lang.Object ref = srcPid_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
srcPid_ = s;
}
return s;
}
}
/**
* optional string srcPid = 3;
*/
public com.google.protobuf.ByteString
getSrcPidBytes() {
java.lang.Object ref = srcPid_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
srcPid_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int TOCIDS_FIELD_NUMBER = 4;
private com.google.protobuf.LazyStringList toCids_;
/**
* repeated string toCids = 4;
*/
public com.google.protobuf.ProtocolStringList
getToCidsList() {
return toCids_;
}
/**
* repeated string toCids = 4;
*/
public int getToCidsCount() {
return toCids_.size();
}
/**
* repeated string toCids = 4;
*/
public java.lang.String getToCids(int index) {
return toCids_.get(index);
}
/**
* repeated string toCids = 4;
*/
public com.google.protobuf.ByteString
getToCidsBytes(int index) {
return toCids_.getByteString(index);
}
public static final int LIMIT_FIELD_NUMBER = 5;
private int limit_;
/**
* optional int32 limit = 5;
*/
public boolean hasLimit() {
return ((bitField0_ & 0x00000004) == 0x00000004);
}
/**
* optional int32 limit = 5;
*/
public int getLimit() {
return limit_;
}
public static final int NEXT_FIELD_NUMBER = 6;
private volatile java.lang.Object next_;
/**
* optional string next = 6;
*/
public boolean hasNext() {
return ((bitField0_ & 0x00000008) == 0x00000008);
}
/**
* optional string next = 6;
*/
public java.lang.String getNext() {
java.lang.Object ref = next_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
next_ = s;
}
return s;
}
}
/**
* optional string next = 6;
*/
public com.google.protobuf.ByteString
getNextBytes() {
java.lang.Object ref = next_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
next_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int BLOCKEDPIDS_FIELD_NUMBER = 8;
private com.google.protobuf.LazyStringList blockedPids_;
/**
* repeated string blockedPids = 8;
*/
public com.google.protobuf.ProtocolStringList
getBlockedPidsList() {
return blockedPids_;
}
/**
* repeated string blockedPids = 8;
*/
public int getBlockedPidsCount() {
return blockedPids_.size();
}
/**
* repeated string blockedPids = 8;
*/
public java.lang.String getBlockedPids(int index) {
return blockedPids_.get(index);
}
/**
* repeated string blockedPids = 8;
*/
public com.google.protobuf.ByteString
getBlockedPidsBytes(int index) {
return blockedPids_.getByteString(index);
}
public static final int BLOCKEDCIDS_FIELD_NUMBER = 9;
private com.google.protobuf.LazyStringList blockedCids_;
/**
* repeated string blockedCids = 9;
*/
public com.google.protobuf.ProtocolStringList
getBlockedCidsList() {
return blockedCids_;
}
/**
* repeated string blockedCids = 9;
*/
public int getBlockedCidsCount() {
return blockedCids_.size();
}
/**
* repeated string blockedCids = 9;
*/
public java.lang.String getBlockedCids(int index) {
return blockedCids_.get(index);
}
/**
* repeated string blockedCids = 9;
*/
public com.google.protobuf.ByteString
getBlockedCidsBytes(int index) {
return blockedCids_.getByteString(index);
}
public static final int ALLOWEDPIDS_FIELD_NUMBER = 10;
private com.google.protobuf.LazyStringList allowedPids_;
/**
* repeated string allowedPids = 10;
*/
public com.google.protobuf.ProtocolStringList
getAllowedPidsList() {
return allowedPids_;
}
/**
* repeated string allowedPids = 10;
*/
public int getAllowedPidsCount() {
return allowedPids_.size();
}
/**
* repeated string allowedPids = 10;
*/
public java.lang.String getAllowedPids(int index) {
return allowedPids_.get(index);
}
/**
* repeated string allowedPids = 10;
*/
public com.google.protobuf.ByteString
getAllowedPidsBytes(int index) {
return allowedPids_.getByteString(index);
}
public static final int FAILEDPIDS_FIELD_NUMBER = 11;
private java.util.List failedPids_;
/**
* repeated .com.avos.avoscloud.ErrorCommand failedPids = 11;
*/
public java.util.List getFailedPidsList() {
return failedPids_;
}
/**
* repeated .com.avos.avoscloud.ErrorCommand failedPids = 11;
*/
public java.util.List extends com.avos.avoscloud.Messages.ErrorCommandOrBuilder>
getFailedPidsOrBuilderList() {
return failedPids_;
}
/**
* repeated .com.avos.avoscloud.ErrorCommand failedPids = 11;
*/
public int getFailedPidsCount() {
return failedPids_.size();
}
/**
* repeated .com.avos.avoscloud.ErrorCommand failedPids = 11;
*/
public com.avos.avoscloud.Messages.ErrorCommand getFailedPids(int index) {
return failedPids_.get(index);
}
/**
* repeated .com.avos.avoscloud.ErrorCommand failedPids = 11;
*/
public com.avos.avoscloud.Messages.ErrorCommandOrBuilder getFailedPidsOrBuilder(
int index) {
return failedPids_.get(index);
}
public static final int T_FIELD_NUMBER = 12;
private long t_;
/**
* optional int64 t = 12;
*/
public boolean hasT() {
return ((bitField0_ & 0x00000010) == 0x00000010);
}
/**
* optional int64 t = 12;
*/
public long getT() {
return t_;
}
public static final int N_FIELD_NUMBER = 13;
private volatile java.lang.Object n_;
/**
* optional string n = 13;
*/
public boolean hasN() {
return ((bitField0_ & 0x00000020) == 0x00000020);
}
/**
* optional string n = 13;
*/
public java.lang.String getN() {
java.lang.Object ref = n_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
n_ = s;
}
return s;
}
}
/**
* optional string n = 13;
*/
public com.google.protobuf.ByteString
getNBytes() {
java.lang.Object ref = n_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
n_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int S_FIELD_NUMBER = 14;
private volatile java.lang.Object s_;
/**
* optional string s = 14;
*/
public boolean hasS() {
return ((bitField0_ & 0x00000040) == 0x00000040);
}
/**
* optional string s = 14;
*/
public java.lang.String getS() {
java.lang.Object ref = s_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
s_ = s;
}
return s;
}
}
/**
* optional string s = 14;
*/
public com.google.protobuf.ByteString
getSBytes() {
java.lang.Object ref = s_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
s_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
private byte memoizedIsInitialized = -1;
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
for (int i = 0; i < getFailedPidsCount(); i++) {
if (!getFailedPids(i).isInitialized()) {
memoizedIsInitialized = 0;
return false;
}
}
memoizedIsInitialized = 1;
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (((bitField0_ & 0x00000001) == 0x00000001)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 1, srcCid_);
}
for (int i = 0; i < toPids_.size(); i++) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 2, toPids_.getRaw(i));
}
if (((bitField0_ & 0x00000002) == 0x00000002)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 3, srcPid_);
}
for (int i = 0; i < toCids_.size(); i++) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 4, toCids_.getRaw(i));
}
if (((bitField0_ & 0x00000004) == 0x00000004)) {
output.writeInt32(5, limit_);
}
if (((bitField0_ & 0x00000008) == 0x00000008)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 6, next_);
}
for (int i = 0; i < blockedPids_.size(); i++) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 8, blockedPids_.getRaw(i));
}
for (int i = 0; i < blockedCids_.size(); i++) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 9, blockedCids_.getRaw(i));
}
for (int i = 0; i < allowedPids_.size(); i++) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 10, allowedPids_.getRaw(i));
}
for (int i = 0; i < failedPids_.size(); i++) {
output.writeMessage(11, failedPids_.get(i));
}
if (((bitField0_ & 0x00000010) == 0x00000010)) {
output.writeInt64(12, t_);
}
if (((bitField0_ & 0x00000020) == 0x00000020)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 13, n_);
}
if (((bitField0_ & 0x00000040) == 0x00000040)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 14, s_);
}
unknownFields.writeTo(output);
}
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (((bitField0_ & 0x00000001) == 0x00000001)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(1, srcCid_);
}
{
int dataSize = 0;
for (int i = 0; i < toPids_.size(); i++) {
dataSize += computeStringSizeNoTag(toPids_.getRaw(i));
}
size += dataSize;
size += 1 * getToPidsList().size();
}
if (((bitField0_ & 0x00000002) == 0x00000002)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(3, srcPid_);
}
{
int dataSize = 0;
for (int i = 0; i < toCids_.size(); i++) {
dataSize += computeStringSizeNoTag(toCids_.getRaw(i));
}
size += dataSize;
size += 1 * getToCidsList().size();
}
if (((bitField0_ & 0x00000004) == 0x00000004)) {
size += com.google.protobuf.CodedOutputStream
.computeInt32Size(5, limit_);
}
if (((bitField0_ & 0x00000008) == 0x00000008)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(6, next_);
}
{
int dataSize = 0;
for (int i = 0; i < blockedPids_.size(); i++) {
dataSize += computeStringSizeNoTag(blockedPids_.getRaw(i));
}
size += dataSize;
size += 1 * getBlockedPidsList().size();
}
{
int dataSize = 0;
for (int i = 0; i < blockedCids_.size(); i++) {
dataSize += computeStringSizeNoTag(blockedCids_.getRaw(i));
}
size += dataSize;
size += 1 * getBlockedCidsList().size();
}
{
int dataSize = 0;
for (int i = 0; i < allowedPids_.size(); i++) {
dataSize += computeStringSizeNoTag(allowedPids_.getRaw(i));
}
size += dataSize;
size += 1 * getAllowedPidsList().size();
}
for (int i = 0; i < failedPids_.size(); i++) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(11, failedPids_.get(i));
}
if (((bitField0_ & 0x00000010) == 0x00000010)) {
size += com.google.protobuf.CodedOutputStream
.computeInt64Size(12, t_);
}
if (((bitField0_ & 0x00000020) == 0x00000020)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(13, n_);
}
if (((bitField0_ & 0x00000040) == 0x00000040)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(14, s_);
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof com.avos.avoscloud.Messages.BlacklistCommand)) {
return super.equals(obj);
}
com.avos.avoscloud.Messages.BlacklistCommand other = (com.avos.avoscloud.Messages.BlacklistCommand) obj;
boolean result = true;
result = result && (hasSrcCid() == other.hasSrcCid());
if (hasSrcCid()) {
result = result && getSrcCid()
.equals(other.getSrcCid());
}
result = result && getToPidsList()
.equals(other.getToPidsList());
result = result && (hasSrcPid() == other.hasSrcPid());
if (hasSrcPid()) {
result = result && getSrcPid()
.equals(other.getSrcPid());
}
result = result && getToCidsList()
.equals(other.getToCidsList());
result = result && (hasLimit() == other.hasLimit());
if (hasLimit()) {
result = result && (getLimit()
== other.getLimit());
}
result = result && (hasNext() == other.hasNext());
if (hasNext()) {
result = result && getNext()
.equals(other.getNext());
}
result = result && getBlockedPidsList()
.equals(other.getBlockedPidsList());
result = result && getBlockedCidsList()
.equals(other.getBlockedCidsList());
result = result && getAllowedPidsList()
.equals(other.getAllowedPidsList());
result = result && getFailedPidsList()
.equals(other.getFailedPidsList());
result = result && (hasT() == other.hasT());
if (hasT()) {
result = result && (getT()
== other.getT());
}
result = result && (hasN() == other.hasN());
if (hasN()) {
result = result && getN()
.equals(other.getN());
}
result = result && (hasS() == other.hasS());
if (hasS()) {
result = result && getS()
.equals(other.getS());
}
result = result && unknownFields.equals(other.unknownFields);
return result;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (hasSrcCid()) {
hash = (37 * hash) + SRCCID_FIELD_NUMBER;
hash = (53 * hash) + getSrcCid().hashCode();
}
if (getToPidsCount() > 0) {
hash = (37 * hash) + TOPIDS_FIELD_NUMBER;
hash = (53 * hash) + getToPidsList().hashCode();
}
if (hasSrcPid()) {
hash = (37 * hash) + SRCPID_FIELD_NUMBER;
hash = (53 * hash) + getSrcPid().hashCode();
}
if (getToCidsCount() > 0) {
hash = (37 * hash) + TOCIDS_FIELD_NUMBER;
hash = (53 * hash) + getToCidsList().hashCode();
}
if (hasLimit()) {
hash = (37 * hash) + LIMIT_FIELD_NUMBER;
hash = (53 * hash) + getLimit();
}
if (hasNext()) {
hash = (37 * hash) + NEXT_FIELD_NUMBER;
hash = (53 * hash) + getNext().hashCode();
}
if (getBlockedPidsCount() > 0) {
hash = (37 * hash) + BLOCKEDPIDS_FIELD_NUMBER;
hash = (53 * hash) + getBlockedPidsList().hashCode();
}
if (getBlockedCidsCount() > 0) {
hash = (37 * hash) + BLOCKEDCIDS_FIELD_NUMBER;
hash = (53 * hash) + getBlockedCidsList().hashCode();
}
if (getAllowedPidsCount() > 0) {
hash = (37 * hash) + ALLOWEDPIDS_FIELD_NUMBER;
hash = (53 * hash) + getAllowedPidsList().hashCode();
}
if (getFailedPidsCount() > 0) {
hash = (37 * hash) + FAILEDPIDS_FIELD_NUMBER;
hash = (53 * hash) + getFailedPidsList().hashCode();
}
if (hasT()) {
hash = (37 * hash) + T_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getT());
}
if (hasN()) {
hash = (37 * hash) + N_FIELD_NUMBER;
hash = (53 * hash) + getN().hashCode();
}
if (hasS()) {
hash = (37 * hash) + S_FIELD_NUMBER;
hash = (53 * hash) + getS().hashCode();
}
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static com.avos.avoscloud.Messages.BlacklistCommand parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.avos.avoscloud.Messages.BlacklistCommand parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.avos.avoscloud.Messages.BlacklistCommand parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.avos.avoscloud.Messages.BlacklistCommand parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.avos.avoscloud.Messages.BlacklistCommand parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.avos.avoscloud.Messages.BlacklistCommand parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.avos.avoscloud.Messages.BlacklistCommand parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.avos.avoscloud.Messages.BlacklistCommand parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static com.avos.avoscloud.Messages.BlacklistCommand parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static com.avos.avoscloud.Messages.BlacklistCommand parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static com.avos.avoscloud.Messages.BlacklistCommand parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.avos.avoscloud.Messages.BlacklistCommand parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(com.avos.avoscloud.Messages.BlacklistCommand prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code com.avos.avoscloud.BlacklistCommand}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:com.avos.avoscloud.BlacklistCommand)
com.avos.avoscloud.Messages.BlacklistCommandOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.avos.avoscloud.Messages.internal_static_com_avos_avoscloud_BlacklistCommand_descriptor;
}
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.avos.avoscloud.Messages.internal_static_com_avos_avoscloud_BlacklistCommand_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.avos.avoscloud.Messages.BlacklistCommand.class, com.avos.avoscloud.Messages.BlacklistCommand.Builder.class);
}
// Construct using com.avos.avoscloud.Messages.BlacklistCommand.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
getFailedPidsFieldBuilder();
}
}
public Builder clear() {
super.clear();
srcCid_ = "";
bitField0_ = (bitField0_ & ~0x00000001);
toPids_ = com.google.protobuf.LazyStringArrayList.EMPTY;
bitField0_ = (bitField0_ & ~0x00000002);
srcPid_ = "";
bitField0_ = (bitField0_ & ~0x00000004);
toCids_ = com.google.protobuf.LazyStringArrayList.EMPTY;
bitField0_ = (bitField0_ & ~0x00000008);
limit_ = 0;
bitField0_ = (bitField0_ & ~0x00000010);
next_ = "";
bitField0_ = (bitField0_ & ~0x00000020);
blockedPids_ = com.google.protobuf.LazyStringArrayList.EMPTY;
bitField0_ = (bitField0_ & ~0x00000040);
blockedCids_ = com.google.protobuf.LazyStringArrayList.EMPTY;
bitField0_ = (bitField0_ & ~0x00000080);
allowedPids_ = com.google.protobuf.LazyStringArrayList.EMPTY;
bitField0_ = (bitField0_ & ~0x00000100);
if (failedPidsBuilder_ == null) {
failedPids_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000200);
} else {
failedPidsBuilder_.clear();
}
t_ = 0L;
bitField0_ = (bitField0_ & ~0x00000400);
n_ = "";
bitField0_ = (bitField0_ & ~0x00000800);
s_ = "";
bitField0_ = (bitField0_ & ~0x00001000);
return this;
}
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return com.avos.avoscloud.Messages.internal_static_com_avos_avoscloud_BlacklistCommand_descriptor;
}
public com.avos.avoscloud.Messages.BlacklistCommand getDefaultInstanceForType() {
return com.avos.avoscloud.Messages.BlacklistCommand.getDefaultInstance();
}
public com.avos.avoscloud.Messages.BlacklistCommand build() {
com.avos.avoscloud.Messages.BlacklistCommand result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
public com.avos.avoscloud.Messages.BlacklistCommand buildPartial() {
com.avos.avoscloud.Messages.BlacklistCommand result = new com.avos.avoscloud.Messages.BlacklistCommand(this);
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (((from_bitField0_ & 0x00000001) == 0x00000001)) {
to_bitField0_ |= 0x00000001;
}
result.srcCid_ = srcCid_;
if (((bitField0_ & 0x00000002) == 0x00000002)) {
toPids_ = toPids_.getUnmodifiableView();
bitField0_ = (bitField0_ & ~0x00000002);
}
result.toPids_ = toPids_;
if (((from_bitField0_ & 0x00000004) == 0x00000004)) {
to_bitField0_ |= 0x00000002;
}
result.srcPid_ = srcPid_;
if (((bitField0_ & 0x00000008) == 0x00000008)) {
toCids_ = toCids_.getUnmodifiableView();
bitField0_ = (bitField0_ & ~0x00000008);
}
result.toCids_ = toCids_;
if (((from_bitField0_ & 0x00000010) == 0x00000010)) {
to_bitField0_ |= 0x00000004;
}
result.limit_ = limit_;
if (((from_bitField0_ & 0x00000020) == 0x00000020)) {
to_bitField0_ |= 0x00000008;
}
result.next_ = next_;
if (((bitField0_ & 0x00000040) == 0x00000040)) {
blockedPids_ = blockedPids_.getUnmodifiableView();
bitField0_ = (bitField0_ & ~0x00000040);
}
result.blockedPids_ = blockedPids_;
if (((bitField0_ & 0x00000080) == 0x00000080)) {
blockedCids_ = blockedCids_.getUnmodifiableView();
bitField0_ = (bitField0_ & ~0x00000080);
}
result.blockedCids_ = blockedCids_;
if (((bitField0_ & 0x00000100) == 0x00000100)) {
allowedPids_ = allowedPids_.getUnmodifiableView();
bitField0_ = (bitField0_ & ~0x00000100);
}
result.allowedPids_ = allowedPids_;
if (failedPidsBuilder_ == null) {
if (((bitField0_ & 0x00000200) == 0x00000200)) {
failedPids_ = java.util.Collections.unmodifiableList(failedPids_);
bitField0_ = (bitField0_ & ~0x00000200);
}
result.failedPids_ = failedPids_;
} else {
result.failedPids_ = failedPidsBuilder_.build();
}
if (((from_bitField0_ & 0x00000400) == 0x00000400)) {
to_bitField0_ |= 0x00000010;
}
result.t_ = t_;
if (((from_bitField0_ & 0x00000800) == 0x00000800)) {
to_bitField0_ |= 0x00000020;
}
result.n_ = n_;
if (((from_bitField0_ & 0x00001000) == 0x00001000)) {
to_bitField0_ |= 0x00000040;
}
result.s_ = s_;
result.bitField0_ = to_bitField0_;
onBuilt();
return result;
}
public Builder clone() {
return (Builder) super.clone();
}
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.setField(field, value);
}
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return (Builder) super.clearField(field);
}
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return (Builder) super.clearOneof(oneof);
}
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return (Builder) super.setRepeatedField(field, index, value);
}
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.addRepeatedField(field, value);
}
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.avos.avoscloud.Messages.BlacklistCommand) {
return mergeFrom((com.avos.avoscloud.Messages.BlacklistCommand)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.avos.avoscloud.Messages.BlacklistCommand other) {
if (other == com.avos.avoscloud.Messages.BlacklistCommand.getDefaultInstance()) return this;
if (other.hasSrcCid()) {
bitField0_ |= 0x00000001;
srcCid_ = other.srcCid_;
onChanged();
}
if (!other.toPids_.isEmpty()) {
if (toPids_.isEmpty()) {
toPids_ = other.toPids_;
bitField0_ = (bitField0_ & ~0x00000002);
} else {
ensureToPidsIsMutable();
toPids_.addAll(other.toPids_);
}
onChanged();
}
if (other.hasSrcPid()) {
bitField0_ |= 0x00000004;
srcPid_ = other.srcPid_;
onChanged();
}
if (!other.toCids_.isEmpty()) {
if (toCids_.isEmpty()) {
toCids_ = other.toCids_;
bitField0_ = (bitField0_ & ~0x00000008);
} else {
ensureToCidsIsMutable();
toCids_.addAll(other.toCids_);
}
onChanged();
}
if (other.hasLimit()) {
setLimit(other.getLimit());
}
if (other.hasNext()) {
bitField0_ |= 0x00000020;
next_ = other.next_;
onChanged();
}
if (!other.blockedPids_.isEmpty()) {
if (blockedPids_.isEmpty()) {
blockedPids_ = other.blockedPids_;
bitField0_ = (bitField0_ & ~0x00000040);
} else {
ensureBlockedPidsIsMutable();
blockedPids_.addAll(other.blockedPids_);
}
onChanged();
}
if (!other.blockedCids_.isEmpty()) {
if (blockedCids_.isEmpty()) {
blockedCids_ = other.blockedCids_;
bitField0_ = (bitField0_ & ~0x00000080);
} else {
ensureBlockedCidsIsMutable();
blockedCids_.addAll(other.blockedCids_);
}
onChanged();
}
if (!other.allowedPids_.isEmpty()) {
if (allowedPids_.isEmpty()) {
allowedPids_ = other.allowedPids_;
bitField0_ = (bitField0_ & ~0x00000100);
} else {
ensureAllowedPidsIsMutable();
allowedPids_.addAll(other.allowedPids_);
}
onChanged();
}
if (failedPidsBuilder_ == null) {
if (!other.failedPids_.isEmpty()) {
if (failedPids_.isEmpty()) {
failedPids_ = other.failedPids_;
bitField0_ = (bitField0_ & ~0x00000200);
} else {
ensureFailedPidsIsMutable();
failedPids_.addAll(other.failedPids_);
}
onChanged();
}
} else {
if (!other.failedPids_.isEmpty()) {
if (failedPidsBuilder_.isEmpty()) {
failedPidsBuilder_.dispose();
failedPidsBuilder_ = null;
failedPids_ = other.failedPids_;
bitField0_ = (bitField0_ & ~0x00000200);
failedPidsBuilder_ =
com.google.protobuf.GeneratedMessageV3.alwaysUseFieldBuilders ?
getFailedPidsFieldBuilder() : null;
} else {
failedPidsBuilder_.addAllMessages(other.failedPids_);
}
}
}
if (other.hasT()) {
setT(other.getT());
}
if (other.hasN()) {
bitField0_ |= 0x00000800;
n_ = other.n_;
onChanged();
}
if (other.hasS()) {
bitField0_ |= 0x00001000;
s_ = other.s_;
onChanged();
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
public final boolean isInitialized() {
for (int i = 0; i < getFailedPidsCount(); i++) {
if (!getFailedPids(i).isInitialized()) {
return false;
}
}
return true;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
com.avos.avoscloud.Messages.BlacklistCommand parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (com.avos.avoscloud.Messages.BlacklistCommand) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
private java.lang.Object srcCid_ = "";
/**
* optional string srcCid = 1;
*/
public boolean hasSrcCid() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
* optional string srcCid = 1;
*/
public java.lang.String getSrcCid() {
java.lang.Object ref = srcCid_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
srcCid_ = s;
}
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* optional string srcCid = 1;
*/
public com.google.protobuf.ByteString
getSrcCidBytes() {
java.lang.Object ref = srcCid_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
srcCid_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* optional string srcCid = 1;
*/
public Builder setSrcCid(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000001;
srcCid_ = value;
onChanged();
return this;
}
/**
* optional string srcCid = 1;
*/
public Builder clearSrcCid() {
bitField0_ = (bitField0_ & ~0x00000001);
srcCid_ = getDefaultInstance().getSrcCid();
onChanged();
return this;
}
/**
* optional string srcCid = 1;
*/
public Builder setSrcCidBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000001;
srcCid_ = value;
onChanged();
return this;
}
private com.google.protobuf.LazyStringList toPids_ = com.google.protobuf.LazyStringArrayList.EMPTY;
private void ensureToPidsIsMutable() {
if (!((bitField0_ & 0x00000002) == 0x00000002)) {
toPids_ = new com.google.protobuf.LazyStringArrayList(toPids_);
bitField0_ |= 0x00000002;
}
}
/**
* repeated string toPids = 2;
*/
public com.google.protobuf.ProtocolStringList
getToPidsList() {
return toPids_.getUnmodifiableView();
}
/**
* repeated string toPids = 2;
*/
public int getToPidsCount() {
return toPids_.size();
}
/**
* repeated string toPids = 2;
*/
public java.lang.String getToPids(int index) {
return toPids_.get(index);
}
/**
* repeated string toPids = 2;
*/
public com.google.protobuf.ByteString
getToPidsBytes(int index) {
return toPids_.getByteString(index);
}
/**
* repeated string toPids = 2;
*/
public Builder setToPids(
int index, java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
ensureToPidsIsMutable();
toPids_.set(index, value);
onChanged();
return this;
}
/**
* repeated string toPids = 2;
*/
public Builder addToPids(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
ensureToPidsIsMutable();
toPids_.add(value);
onChanged();
return this;
}
/**
* repeated string toPids = 2;
*/
public Builder addAllToPids(
java.lang.Iterable values) {
ensureToPidsIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, toPids_);
onChanged();
return this;
}
/**
* repeated string toPids = 2;
*/
public Builder clearToPids() {
toPids_ = com.google.protobuf.LazyStringArrayList.EMPTY;
bitField0_ = (bitField0_ & ~0x00000002);
onChanged();
return this;
}
/**
* repeated string toPids = 2;
*/
public Builder addToPidsBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
ensureToPidsIsMutable();
toPids_.add(value);
onChanged();
return this;
}
private java.lang.Object srcPid_ = "";
/**
* optional string srcPid = 3;
*/
public boolean hasSrcPid() {
return ((bitField0_ & 0x00000004) == 0x00000004);
}
/**
* optional string srcPid = 3;
*/
public java.lang.String getSrcPid() {
java.lang.Object ref = srcPid_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
srcPid_ = s;
}
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* optional string srcPid = 3;
*/
public com.google.protobuf.ByteString
getSrcPidBytes() {
java.lang.Object ref = srcPid_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
srcPid_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* optional string srcPid = 3;
*/
public Builder setSrcPid(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000004;
srcPid_ = value;
onChanged();
return this;
}
/**
* optional string srcPid = 3;
*/
public Builder clearSrcPid() {
bitField0_ = (bitField0_ & ~0x00000004);
srcPid_ = getDefaultInstance().getSrcPid();
onChanged();
return this;
}
/**
* optional string srcPid = 3;
*/
public Builder setSrcPidBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000004;
srcPid_ = value;
onChanged();
return this;
}
private com.google.protobuf.LazyStringList toCids_ = com.google.protobuf.LazyStringArrayList.EMPTY;
private void ensureToCidsIsMutable() {
if (!((bitField0_ & 0x00000008) == 0x00000008)) {
toCids_ = new com.google.protobuf.LazyStringArrayList(toCids_);
bitField0_ |= 0x00000008;
}
}
/**
* repeated string toCids = 4;
*/
public com.google.protobuf.ProtocolStringList
getToCidsList() {
return toCids_.getUnmodifiableView();
}
/**
* repeated string toCids = 4;
*/
public int getToCidsCount() {
return toCids_.size();
}
/**
* repeated string toCids = 4;
*/
public java.lang.String getToCids(int index) {
return toCids_.get(index);
}
/**
* repeated string toCids = 4;
*/
public com.google.protobuf.ByteString
getToCidsBytes(int index) {
return toCids_.getByteString(index);
}
/**
* repeated string toCids = 4;
*/
public Builder setToCids(
int index, java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
ensureToCidsIsMutable();
toCids_.set(index, value);
onChanged();
return this;
}
/**
* repeated string toCids = 4;
*/
public Builder addToCids(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
ensureToCidsIsMutable();
toCids_.add(value);
onChanged();
return this;
}
/**
* repeated string toCids = 4;
*/
public Builder addAllToCids(
java.lang.Iterable values) {
ensureToCidsIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, toCids_);
onChanged();
return this;
}
/**
* repeated string toCids = 4;
*/
public Builder clearToCids() {
toCids_ = com.google.protobuf.LazyStringArrayList.EMPTY;
bitField0_ = (bitField0_ & ~0x00000008);
onChanged();
return this;
}
/**
* repeated string toCids = 4;
*/
public Builder addToCidsBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
ensureToCidsIsMutable();
toCids_.add(value);
onChanged();
return this;
}
private int limit_ ;
/**
* optional int32 limit = 5;
*/
public boolean hasLimit() {
return ((bitField0_ & 0x00000010) == 0x00000010);
}
/**
* optional int32 limit = 5;
*/
public int getLimit() {
return limit_;
}
/**
* optional int32 limit = 5;
*/
public Builder setLimit(int value) {
bitField0_ |= 0x00000010;
limit_ = value;
onChanged();
return this;
}
/**
* optional int32 limit = 5;
*/
public Builder clearLimit() {
bitField0_ = (bitField0_ & ~0x00000010);
limit_ = 0;
onChanged();
return this;
}
private java.lang.Object next_ = "";
/**
* optional string next = 6;
*/
public boolean hasNext() {
return ((bitField0_ & 0x00000020) == 0x00000020);
}
/**
* optional string next = 6;
*/
public java.lang.String getNext() {
java.lang.Object ref = next_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
next_ = s;
}
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* optional string next = 6;
*/
public com.google.protobuf.ByteString
getNextBytes() {
java.lang.Object ref = next_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
next_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* optional string next = 6;
*/
public Builder setNext(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000020;
next_ = value;
onChanged();
return this;
}
/**
* optional string next = 6;
*/
public Builder clearNext() {
bitField0_ = (bitField0_ & ~0x00000020);
next_ = getDefaultInstance().getNext();
onChanged();
return this;
}
/**
* optional string next = 6;
*/
public Builder setNextBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000020;
next_ = value;
onChanged();
return this;
}
private com.google.protobuf.LazyStringList blockedPids_ = com.google.protobuf.LazyStringArrayList.EMPTY;
private void ensureBlockedPidsIsMutable() {
if (!((bitField0_ & 0x00000040) == 0x00000040)) {
blockedPids_ = new com.google.protobuf.LazyStringArrayList(blockedPids_);
bitField0_ |= 0x00000040;
}
}
/**
* repeated string blockedPids = 8;
*/
public com.google.protobuf.ProtocolStringList
getBlockedPidsList() {
return blockedPids_.getUnmodifiableView();
}
/**
* repeated string blockedPids = 8;
*/
public int getBlockedPidsCount() {
return blockedPids_.size();
}
/**
* repeated string blockedPids = 8;
*/
public java.lang.String getBlockedPids(int index) {
return blockedPids_.get(index);
}
/**
* repeated string blockedPids = 8;
*/
public com.google.protobuf.ByteString
getBlockedPidsBytes(int index) {
return blockedPids_.getByteString(index);
}
/**
* repeated string blockedPids = 8;
*/
public Builder setBlockedPids(
int index, java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
ensureBlockedPidsIsMutable();
blockedPids_.set(index, value);
onChanged();
return this;
}
/**
* repeated string blockedPids = 8;
*/
public Builder addBlockedPids(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
ensureBlockedPidsIsMutable();
blockedPids_.add(value);
onChanged();
return this;
}
/**
* repeated string blockedPids = 8;
*/
public Builder addAllBlockedPids(
java.lang.Iterable values) {
ensureBlockedPidsIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, blockedPids_);
onChanged();
return this;
}
/**
* repeated string blockedPids = 8;
*/
public Builder clearBlockedPids() {
blockedPids_ = com.google.protobuf.LazyStringArrayList.EMPTY;
bitField0_ = (bitField0_ & ~0x00000040);
onChanged();
return this;
}
/**
* repeated string blockedPids = 8;
*/
public Builder addBlockedPidsBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
ensureBlockedPidsIsMutable();
blockedPids_.add(value);
onChanged();
return this;
}
private com.google.protobuf.LazyStringList blockedCids_ = com.google.protobuf.LazyStringArrayList.EMPTY;
private void ensureBlockedCidsIsMutable() {
if (!((bitField0_ & 0x00000080) == 0x00000080)) {
blockedCids_ = new com.google.protobuf.LazyStringArrayList(blockedCids_);
bitField0_ |= 0x00000080;
}
}
/**
* repeated string blockedCids = 9;
*/
public com.google.protobuf.ProtocolStringList
getBlockedCidsList() {
return blockedCids_.getUnmodifiableView();
}
/**
* repeated string blockedCids = 9;
*/
public int getBlockedCidsCount() {
return blockedCids_.size();
}
/**
* repeated string blockedCids = 9;
*/
public java.lang.String getBlockedCids(int index) {
return blockedCids_.get(index);
}
/**
* repeated string blockedCids = 9;
*/
public com.google.protobuf.ByteString
getBlockedCidsBytes(int index) {
return blockedCids_.getByteString(index);
}
/**
* repeated string blockedCids = 9;
*/
public Builder setBlockedCids(
int index, java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
ensureBlockedCidsIsMutable();
blockedCids_.set(index, value);
onChanged();
return this;
}
/**
* repeated string blockedCids = 9;
*/
public Builder addBlockedCids(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
ensureBlockedCidsIsMutable();
blockedCids_.add(value);
onChanged();
return this;
}
/**
* repeated string blockedCids = 9;
*/
public Builder addAllBlockedCids(
java.lang.Iterable values) {
ensureBlockedCidsIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, blockedCids_);
onChanged();
return this;
}
/**
* repeated string blockedCids = 9;
*/
public Builder clearBlockedCids() {
blockedCids_ = com.google.protobuf.LazyStringArrayList.EMPTY;
bitField0_ = (bitField0_ & ~0x00000080);
onChanged();
return this;
}
/**
* repeated string blockedCids = 9;
*/
public Builder addBlockedCidsBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
ensureBlockedCidsIsMutable();
blockedCids_.add(value);
onChanged();
return this;
}
private com.google.protobuf.LazyStringList allowedPids_ = com.google.protobuf.LazyStringArrayList.EMPTY;
private void ensureAllowedPidsIsMutable() {
if (!((bitField0_ & 0x00000100) == 0x00000100)) {
allowedPids_ = new com.google.protobuf.LazyStringArrayList(allowedPids_);
bitField0_ |= 0x00000100;
}
}
/**
* repeated string allowedPids = 10;
*/
public com.google.protobuf.ProtocolStringList
getAllowedPidsList() {
return allowedPids_.getUnmodifiableView();
}
/**
* repeated string allowedPids = 10;
*/
public int getAllowedPidsCount() {
return allowedPids_.size();
}
/**
* repeated string allowedPids = 10;
*/
public java.lang.String getAllowedPids(int index) {
return allowedPids_.get(index);
}
/**
* repeated string allowedPids = 10;
*/
public com.google.protobuf.ByteString
getAllowedPidsBytes(int index) {
return allowedPids_.getByteString(index);
}
/**
* repeated string allowedPids = 10;
*/
public Builder setAllowedPids(
int index, java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
ensureAllowedPidsIsMutable();
allowedPids_.set(index, value);
onChanged();
return this;
}
/**
* repeated string allowedPids = 10;
*/
public Builder addAllowedPids(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
ensureAllowedPidsIsMutable();
allowedPids_.add(value);
onChanged();
return this;
}
/**
* repeated string allowedPids = 10;
*/
public Builder addAllAllowedPids(
java.lang.Iterable values) {
ensureAllowedPidsIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, allowedPids_);
onChanged();
return this;
}
/**
* repeated string allowedPids = 10;
*/
public Builder clearAllowedPids() {
allowedPids_ = com.google.protobuf.LazyStringArrayList.EMPTY;
bitField0_ = (bitField0_ & ~0x00000100);
onChanged();
return this;
}
/**
* repeated string allowedPids = 10;
*/
public Builder addAllowedPidsBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
ensureAllowedPidsIsMutable();
allowedPids_.add(value);
onChanged();
return this;
}
private java.util.List failedPids_ =
java.util.Collections.emptyList();
private void ensureFailedPidsIsMutable() {
if (!((bitField0_ & 0x00000200) == 0x00000200)) {
failedPids_ = new java.util.ArrayList(failedPids_);
bitField0_ |= 0x00000200;
}
}
private com.google.protobuf.RepeatedFieldBuilderV3<
com.avos.avoscloud.Messages.ErrorCommand, com.avos.avoscloud.Messages.ErrorCommand.Builder, com.avos.avoscloud.Messages.ErrorCommandOrBuilder> failedPidsBuilder_;
/**
* repeated .com.avos.avoscloud.ErrorCommand failedPids = 11;
*/
public java.util.List getFailedPidsList() {
if (failedPidsBuilder_ == null) {
return java.util.Collections.unmodifiableList(failedPids_);
} else {
return failedPidsBuilder_.getMessageList();
}
}
/**
* repeated .com.avos.avoscloud.ErrorCommand failedPids = 11;
*/
public int getFailedPidsCount() {
if (failedPidsBuilder_ == null) {
return failedPids_.size();
} else {
return failedPidsBuilder_.getCount();
}
}
/**
* repeated .com.avos.avoscloud.ErrorCommand failedPids = 11;
*/
public com.avos.avoscloud.Messages.ErrorCommand getFailedPids(int index) {
if (failedPidsBuilder_ == null) {
return failedPids_.get(index);
} else {
return failedPidsBuilder_.getMessage(index);
}
}
/**
* repeated .com.avos.avoscloud.ErrorCommand failedPids = 11;
*/
public Builder setFailedPids(
int index, com.avos.avoscloud.Messages.ErrorCommand value) {
if (failedPidsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureFailedPidsIsMutable();
failedPids_.set(index, value);
onChanged();
} else {
failedPidsBuilder_.setMessage(index, value);
}
return this;
}
/**
* repeated .com.avos.avoscloud.ErrorCommand failedPids = 11;
*/
public Builder setFailedPids(
int index, com.avos.avoscloud.Messages.ErrorCommand.Builder builderForValue) {
if (failedPidsBuilder_ == null) {
ensureFailedPidsIsMutable();
failedPids_.set(index, builderForValue.build());
onChanged();
} else {
failedPidsBuilder_.setMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .com.avos.avoscloud.ErrorCommand failedPids = 11;
*/
public Builder addFailedPids(com.avos.avoscloud.Messages.ErrorCommand value) {
if (failedPidsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureFailedPidsIsMutable();
failedPids_.add(value);
onChanged();
} else {
failedPidsBuilder_.addMessage(value);
}
return this;
}
/**
* repeated .com.avos.avoscloud.ErrorCommand failedPids = 11;
*/
public Builder addFailedPids(
int index, com.avos.avoscloud.Messages.ErrorCommand value) {
if (failedPidsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureFailedPidsIsMutable();
failedPids_.add(index, value);
onChanged();
} else {
failedPidsBuilder_.addMessage(index, value);
}
return this;
}
/**
* repeated .com.avos.avoscloud.ErrorCommand failedPids = 11;
*/
public Builder addFailedPids(
com.avos.avoscloud.Messages.ErrorCommand.Builder builderForValue) {
if (failedPidsBuilder_ == null) {
ensureFailedPidsIsMutable();
failedPids_.add(builderForValue.build());
onChanged();
} else {
failedPidsBuilder_.addMessage(builderForValue.build());
}
return this;
}
/**
* repeated .com.avos.avoscloud.ErrorCommand failedPids = 11;
*/
public Builder addFailedPids(
int index, com.avos.avoscloud.Messages.ErrorCommand.Builder builderForValue) {
if (failedPidsBuilder_ == null) {
ensureFailedPidsIsMutable();
failedPids_.add(index, builderForValue.build());
onChanged();
} else {
failedPidsBuilder_.addMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .com.avos.avoscloud.ErrorCommand failedPids = 11;
*/
public Builder addAllFailedPids(
java.lang.Iterable extends com.avos.avoscloud.Messages.ErrorCommand> values) {
if (failedPidsBuilder_ == null) {
ensureFailedPidsIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, failedPids_);
onChanged();
} else {
failedPidsBuilder_.addAllMessages(values);
}
return this;
}
/**
* repeated .com.avos.avoscloud.ErrorCommand failedPids = 11;
*/
public Builder clearFailedPids() {
if (failedPidsBuilder_ == null) {
failedPids_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000200);
onChanged();
} else {
failedPidsBuilder_.clear();
}
return this;
}
/**
* repeated .com.avos.avoscloud.ErrorCommand failedPids = 11;
*/
public Builder removeFailedPids(int index) {
if (failedPidsBuilder_ == null) {
ensureFailedPidsIsMutable();
failedPids_.remove(index);
onChanged();
} else {
failedPidsBuilder_.remove(index);
}
return this;
}
/**
* repeated .com.avos.avoscloud.ErrorCommand failedPids = 11;
*/
public com.avos.avoscloud.Messages.ErrorCommand.Builder getFailedPidsBuilder(
int index) {
return getFailedPidsFieldBuilder().getBuilder(index);
}
/**
* repeated .com.avos.avoscloud.ErrorCommand failedPids = 11;
*/
public com.avos.avoscloud.Messages.ErrorCommandOrBuilder getFailedPidsOrBuilder(
int index) {
if (failedPidsBuilder_ == null) {
return failedPids_.get(index); } else {
return failedPidsBuilder_.getMessageOrBuilder(index);
}
}
/**
* repeated .com.avos.avoscloud.ErrorCommand failedPids = 11;
*/
public java.util.List extends com.avos.avoscloud.Messages.ErrorCommandOrBuilder>
getFailedPidsOrBuilderList() {
if (failedPidsBuilder_ != null) {
return failedPidsBuilder_.getMessageOrBuilderList();
} else {
return java.util.Collections.unmodifiableList(failedPids_);
}
}
/**
* repeated .com.avos.avoscloud.ErrorCommand failedPids = 11;
*/
public com.avos.avoscloud.Messages.ErrorCommand.Builder addFailedPidsBuilder() {
return getFailedPidsFieldBuilder().addBuilder(
com.avos.avoscloud.Messages.ErrorCommand.getDefaultInstance());
}
/**
* repeated .com.avos.avoscloud.ErrorCommand failedPids = 11;
*/
public com.avos.avoscloud.Messages.ErrorCommand.Builder addFailedPidsBuilder(
int index) {
return getFailedPidsFieldBuilder().addBuilder(
index, com.avos.avoscloud.Messages.ErrorCommand.getDefaultInstance());
}
/**
* repeated .com.avos.avoscloud.ErrorCommand failedPids = 11;
*/
public java.util.List
getFailedPidsBuilderList() {
return getFailedPidsFieldBuilder().getBuilderList();
}
private com.google.protobuf.RepeatedFieldBuilderV3<
com.avos.avoscloud.Messages.ErrorCommand, com.avos.avoscloud.Messages.ErrorCommand.Builder, com.avos.avoscloud.Messages.ErrorCommandOrBuilder>
getFailedPidsFieldBuilder() {
if (failedPidsBuilder_ == null) {
failedPidsBuilder_ = new com.google.protobuf.RepeatedFieldBuilderV3<
com.avos.avoscloud.Messages.ErrorCommand, com.avos.avoscloud.Messages.ErrorCommand.Builder, com.avos.avoscloud.Messages.ErrorCommandOrBuilder>(
failedPids_,
((bitField0_ & 0x00000200) == 0x00000200),
getParentForChildren(),
isClean());
failedPids_ = null;
}
return failedPidsBuilder_;
}
private long t_ ;
/**
* optional int64 t = 12;
*/
public boolean hasT() {
return ((bitField0_ & 0x00000400) == 0x00000400);
}
/**
* optional int64 t = 12;
*/
public long getT() {
return t_;
}
/**
* optional int64 t = 12;
*/
public Builder setT(long value) {
bitField0_ |= 0x00000400;
t_ = value;
onChanged();
return this;
}
/**
* optional int64 t = 12;
*/
public Builder clearT() {
bitField0_ = (bitField0_ & ~0x00000400);
t_ = 0L;
onChanged();
return this;
}
private java.lang.Object n_ = "";
/**
* optional string n = 13;
*/
public boolean hasN() {
return ((bitField0_ & 0x00000800) == 0x00000800);
}
/**
* optional string n = 13;
*/
public java.lang.String getN() {
java.lang.Object ref = n_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
n_ = s;
}
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* optional string n = 13;
*/
public com.google.protobuf.ByteString
getNBytes() {
java.lang.Object ref = n_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
n_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* optional string n = 13;
*/
public Builder setN(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000800;
n_ = value;
onChanged();
return this;
}
/**
* optional string n = 13;
*/
public Builder clearN() {
bitField0_ = (bitField0_ & ~0x00000800);
n_ = getDefaultInstance().getN();
onChanged();
return this;
}
/**
* optional string n = 13;
*/
public Builder setNBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000800;
n_ = value;
onChanged();
return this;
}
private java.lang.Object s_ = "";
/**
* optional string s = 14;
*/
public boolean hasS() {
return ((bitField0_ & 0x00001000) == 0x00001000);
}
/**
* optional string s = 14;
*/
public java.lang.String getS() {
java.lang.Object ref = s_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
s_ = s;
}
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* optional string s = 14;
*/
public com.google.protobuf.ByteString
getSBytes() {
java.lang.Object ref = s_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
s_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* optional string s = 14;
*/
public Builder setS(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00001000;
s_ = value;
onChanged();
return this;
}
/**
* optional string s = 14;
*/
public Builder clearS() {
bitField0_ = (bitField0_ & ~0x00001000);
s_ = getDefaultInstance().getS();
onChanged();
return this;
}
/**
* optional string s = 14;
*/
public Builder setSBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00001000;
s_ = value;
onChanged();
return this;
}
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:com.avos.avoscloud.BlacklistCommand)
}
// @@protoc_insertion_point(class_scope:com.avos.avoscloud.BlacklistCommand)
private static final com.avos.avoscloud.Messages.BlacklistCommand DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new com.avos.avoscloud.Messages.BlacklistCommand();
}
public static com.avos.avoscloud.Messages.BlacklistCommand getDefaultInstance() {
return DEFAULT_INSTANCE;
}
@java.lang.Deprecated public static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
public BlacklistCommand parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new BlacklistCommand(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
public com.avos.avoscloud.Messages.BlacklistCommand getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface GenericCommandOrBuilder extends
// @@protoc_insertion_point(interface_extends:com.avos.avoscloud.GenericCommand)
com.google.protobuf.MessageOrBuilder {
/**
* optional .com.avos.avoscloud.CommandType cmd = 1;
*/
boolean hasCmd();
/**
* optional .com.avos.avoscloud.CommandType cmd = 1;
*/
com.avos.avoscloud.Messages.CommandType getCmd();
/**
* optional .com.avos.avoscloud.OpType op = 2;
*/
boolean hasOp();
/**
* optional .com.avos.avoscloud.OpType op = 2;
*/
com.avos.avoscloud.Messages.OpType getOp();
/**
* optional string appId = 3;
*/
boolean hasAppId();
/**
* optional string appId = 3;
*/
java.lang.String getAppId();
/**
* optional string appId = 3;
*/
com.google.protobuf.ByteString
getAppIdBytes();
/**
* optional string peerId = 4;
*/
boolean hasPeerId();
/**
* optional string peerId = 4;
*/
java.lang.String getPeerId();
/**
* optional string peerId = 4;
*/
com.google.protobuf.ByteString
getPeerIdBytes();
/**
* optional int32 i = 5;
*/
boolean hasI();
/**
* optional int32 i = 5;
*/
int getI();
/**
* optional string installationId = 6;
*/
boolean hasInstallationId();
/**
* optional string installationId = 6;
*/
java.lang.String getInstallationId();
/**
* optional string installationId = 6;
*/
com.google.protobuf.ByteString
getInstallationIdBytes();
/**
* optional int32 priority = 7;
*/
boolean hasPriority();
/**
* optional int32 priority = 7;
*/
int getPriority();
/**
* optional int32 service = 8;
*/
boolean hasService();
/**
* optional int32 service = 8;
*/
int getService();
/**
* optional int64 serverTs = 9;
*/
boolean hasServerTs();
/**
* optional int64 serverTs = 9;
*/
long getServerTs();
/**
* optional .com.avos.avoscloud.DataCommand dataMessage = 101;
*/
boolean hasDataMessage();
/**
* optional .com.avos.avoscloud.DataCommand dataMessage = 101;
*/
com.avos.avoscloud.Messages.DataCommand getDataMessage();
/**
* optional .com.avos.avoscloud.DataCommand dataMessage = 101;
*/
com.avos.avoscloud.Messages.DataCommandOrBuilder getDataMessageOrBuilder();
/**
* optional .com.avos.avoscloud.SessionCommand sessionMessage = 102;
*/
boolean hasSessionMessage();
/**
* optional .com.avos.avoscloud.SessionCommand sessionMessage = 102;
*/
com.avos.avoscloud.Messages.SessionCommand getSessionMessage();
/**
* optional .com.avos.avoscloud.SessionCommand sessionMessage = 102;
*/
com.avos.avoscloud.Messages.SessionCommandOrBuilder getSessionMessageOrBuilder();
/**
* optional .com.avos.avoscloud.ErrorCommand errorMessage = 103;
*/
boolean hasErrorMessage();
/**
* optional .com.avos.avoscloud.ErrorCommand errorMessage = 103;
*/
com.avos.avoscloud.Messages.ErrorCommand getErrorMessage();
/**
* optional .com.avos.avoscloud.ErrorCommand errorMessage = 103;
*/
com.avos.avoscloud.Messages.ErrorCommandOrBuilder getErrorMessageOrBuilder();
/**
* optional .com.avos.avoscloud.DirectCommand directMessage = 104;
*/
boolean hasDirectMessage();
/**
* optional .com.avos.avoscloud.DirectCommand directMessage = 104;
*/
com.avos.avoscloud.Messages.DirectCommand getDirectMessage();
/**
* optional .com.avos.avoscloud.DirectCommand directMessage = 104;
*/
com.avos.avoscloud.Messages.DirectCommandOrBuilder getDirectMessageOrBuilder();
/**
* optional .com.avos.avoscloud.AckCommand ackMessage = 105;
*/
boolean hasAckMessage();
/**
* optional .com.avos.avoscloud.AckCommand ackMessage = 105;
*/
com.avos.avoscloud.Messages.AckCommand getAckMessage();
/**
* optional .com.avos.avoscloud.AckCommand ackMessage = 105;
*/
com.avos.avoscloud.Messages.AckCommandOrBuilder getAckMessageOrBuilder();
/**
* optional .com.avos.avoscloud.UnreadCommand unreadMessage = 106;
*/
boolean hasUnreadMessage();
/**
* optional .com.avos.avoscloud.UnreadCommand unreadMessage = 106;
*/
com.avos.avoscloud.Messages.UnreadCommand getUnreadMessage();
/**
* optional .com.avos.avoscloud.UnreadCommand unreadMessage = 106;
*/
com.avos.avoscloud.Messages.UnreadCommandOrBuilder getUnreadMessageOrBuilder();
/**
* optional .com.avos.avoscloud.ReadCommand readMessage = 107;
*/
boolean hasReadMessage();
/**
* optional .com.avos.avoscloud.ReadCommand readMessage = 107;
*/
com.avos.avoscloud.Messages.ReadCommand getReadMessage();
/**
* optional .com.avos.avoscloud.ReadCommand readMessage = 107;
*/
com.avos.avoscloud.Messages.ReadCommandOrBuilder getReadMessageOrBuilder();
/**
* optional .com.avos.avoscloud.RcpCommand rcpMessage = 108;
*/
boolean hasRcpMessage();
/**
* optional .com.avos.avoscloud.RcpCommand rcpMessage = 108;
*/
com.avos.avoscloud.Messages.RcpCommand getRcpMessage();
/**
* optional .com.avos.avoscloud.RcpCommand rcpMessage = 108;
*/
com.avos.avoscloud.Messages.RcpCommandOrBuilder getRcpMessageOrBuilder();
/**
* optional .com.avos.avoscloud.LogsCommand logsMessage = 109;
*/
boolean hasLogsMessage();
/**
* optional .com.avos.avoscloud.LogsCommand logsMessage = 109;
*/
com.avos.avoscloud.Messages.LogsCommand getLogsMessage();
/**
* optional .com.avos.avoscloud.LogsCommand logsMessage = 109;
*/
com.avos.avoscloud.Messages.LogsCommandOrBuilder getLogsMessageOrBuilder();
/**
* optional .com.avos.avoscloud.ConvCommand convMessage = 110;
*/
boolean hasConvMessage();
/**
* optional .com.avos.avoscloud.ConvCommand convMessage = 110;
*/
com.avos.avoscloud.Messages.ConvCommand getConvMessage();
/**
* optional .com.avos.avoscloud.ConvCommand convMessage = 110;
*/
com.avos.avoscloud.Messages.ConvCommandOrBuilder getConvMessageOrBuilder();
/**
* optional .com.avos.avoscloud.RoomCommand roomMessage = 111;
*/
boolean hasRoomMessage();
/**
* optional .com.avos.avoscloud.RoomCommand roomMessage = 111;
*/
com.avos.avoscloud.Messages.RoomCommand getRoomMessage();
/**
* optional .com.avos.avoscloud.RoomCommand roomMessage = 111;
*/
com.avos.avoscloud.Messages.RoomCommandOrBuilder getRoomMessageOrBuilder();
/**
* optional .com.avos.avoscloud.PresenceCommand presenceMessage = 112;
*/
boolean hasPresenceMessage();
/**
* optional .com.avos.avoscloud.PresenceCommand presenceMessage = 112;
*/
com.avos.avoscloud.Messages.PresenceCommand getPresenceMessage();
/**
* optional .com.avos.avoscloud.PresenceCommand presenceMessage = 112;
*/
com.avos.avoscloud.Messages.PresenceCommandOrBuilder getPresenceMessageOrBuilder();
/**
* optional .com.avos.avoscloud.ReportCommand reportMessage = 113;
*/
boolean hasReportMessage();
/**
* optional .com.avos.avoscloud.ReportCommand reportMessage = 113;
*/
com.avos.avoscloud.Messages.ReportCommand getReportMessage();
/**
* optional .com.avos.avoscloud.ReportCommand reportMessage = 113;
*/
com.avos.avoscloud.Messages.ReportCommandOrBuilder getReportMessageOrBuilder();
/**
* optional .com.avos.avoscloud.PatchCommand patchMessage = 114;
*/
boolean hasPatchMessage();
/**
* optional .com.avos.avoscloud.PatchCommand patchMessage = 114;
*/
com.avos.avoscloud.Messages.PatchCommand getPatchMessage();
/**
* optional .com.avos.avoscloud.PatchCommand patchMessage = 114;
*/
com.avos.avoscloud.Messages.PatchCommandOrBuilder getPatchMessageOrBuilder();
/**
* optional .com.avos.avoscloud.PubsubCommand pubsubMessage = 115;
*/
boolean hasPubsubMessage();
/**
* optional .com.avos.avoscloud.PubsubCommand pubsubMessage = 115;
*/
com.avos.avoscloud.Messages.PubsubCommand getPubsubMessage();
/**
* optional .com.avos.avoscloud.PubsubCommand pubsubMessage = 115;
*/
com.avos.avoscloud.Messages.PubsubCommandOrBuilder getPubsubMessageOrBuilder();
/**
* optional .com.avos.avoscloud.BlacklistCommand blacklistMessage = 116;
*/
boolean hasBlacklistMessage();
/**
* optional .com.avos.avoscloud.BlacklistCommand blacklistMessage = 116;
*/
com.avos.avoscloud.Messages.BlacklistCommand getBlacklistMessage();
/**
* optional .com.avos.avoscloud.BlacklistCommand blacklistMessage = 116;
*/
com.avos.avoscloud.Messages.BlacklistCommandOrBuilder getBlacklistMessageOrBuilder();
}
/**
* Protobuf type {@code com.avos.avoscloud.GenericCommand}
*/
public static final class GenericCommand extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:com.avos.avoscloud.GenericCommand)
GenericCommandOrBuilder {
private static final long serialVersionUID = 0L;
// Use GenericCommand.newBuilder() to construct.
private GenericCommand(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private GenericCommand() {
cmd_ = 0;
op_ = 1;
appId_ = "";
peerId_ = "";
i_ = 0;
installationId_ = "";
priority_ = 0;
service_ = 0;
serverTs_ = 0L;
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private GenericCommand(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!parseUnknownField(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
case 8: {
int rawValue = input.readEnum();
com.avos.avoscloud.Messages.CommandType value = com.avos.avoscloud.Messages.CommandType.valueOf(rawValue);
if (value == null) {
unknownFields.mergeVarintField(1, rawValue);
} else {
bitField0_ |= 0x00000001;
cmd_ = rawValue;
}
break;
}
case 16: {
int rawValue = input.readEnum();
com.avos.avoscloud.Messages.OpType value = com.avos.avoscloud.Messages.OpType.valueOf(rawValue);
if (value == null) {
unknownFields.mergeVarintField(2, rawValue);
} else {
bitField0_ |= 0x00000002;
op_ = rawValue;
}
break;
}
case 26: {
com.google.protobuf.ByteString bs = input.readBytes();
bitField0_ |= 0x00000004;
appId_ = bs;
break;
}
case 34: {
com.google.protobuf.ByteString bs = input.readBytes();
bitField0_ |= 0x00000008;
peerId_ = bs;
break;
}
case 40: {
bitField0_ |= 0x00000010;
i_ = input.readInt32();
break;
}
case 50: {
com.google.protobuf.ByteString bs = input.readBytes();
bitField0_ |= 0x00000020;
installationId_ = bs;
break;
}
case 56: {
bitField0_ |= 0x00000040;
priority_ = input.readInt32();
break;
}
case 64: {
bitField0_ |= 0x00000080;
service_ = input.readInt32();
break;
}
case 72: {
bitField0_ |= 0x00000100;
serverTs_ = input.readInt64();
break;
}
case 810: {
com.avos.avoscloud.Messages.DataCommand.Builder subBuilder = null;
if (((bitField0_ & 0x00000200) == 0x00000200)) {
subBuilder = dataMessage_.toBuilder();
}
dataMessage_ = input.readMessage(com.avos.avoscloud.Messages.DataCommand.PARSER, extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(dataMessage_);
dataMessage_ = subBuilder.buildPartial();
}
bitField0_ |= 0x00000200;
break;
}
case 818: {
com.avos.avoscloud.Messages.SessionCommand.Builder subBuilder = null;
if (((bitField0_ & 0x00000400) == 0x00000400)) {
subBuilder = sessionMessage_.toBuilder();
}
sessionMessage_ = input.readMessage(com.avos.avoscloud.Messages.SessionCommand.PARSER, extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(sessionMessage_);
sessionMessage_ = subBuilder.buildPartial();
}
bitField0_ |= 0x00000400;
break;
}
case 826: {
com.avos.avoscloud.Messages.ErrorCommand.Builder subBuilder = null;
if (((bitField0_ & 0x00000800) == 0x00000800)) {
subBuilder = errorMessage_.toBuilder();
}
errorMessage_ = input.readMessage(com.avos.avoscloud.Messages.ErrorCommand.PARSER, extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(errorMessage_);
errorMessage_ = subBuilder.buildPartial();
}
bitField0_ |= 0x00000800;
break;
}
case 834: {
com.avos.avoscloud.Messages.DirectCommand.Builder subBuilder = null;
if (((bitField0_ & 0x00001000) == 0x00001000)) {
subBuilder = directMessage_.toBuilder();
}
directMessage_ = input.readMessage(com.avos.avoscloud.Messages.DirectCommand.PARSER, extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(directMessage_);
directMessage_ = subBuilder.buildPartial();
}
bitField0_ |= 0x00001000;
break;
}
case 842: {
com.avos.avoscloud.Messages.AckCommand.Builder subBuilder = null;
if (((bitField0_ & 0x00002000) == 0x00002000)) {
subBuilder = ackMessage_.toBuilder();
}
ackMessage_ = input.readMessage(com.avos.avoscloud.Messages.AckCommand.PARSER, extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(ackMessage_);
ackMessage_ = subBuilder.buildPartial();
}
bitField0_ |= 0x00002000;
break;
}
case 850: {
com.avos.avoscloud.Messages.UnreadCommand.Builder subBuilder = null;
if (((bitField0_ & 0x00004000) == 0x00004000)) {
subBuilder = unreadMessage_.toBuilder();
}
unreadMessage_ = input.readMessage(com.avos.avoscloud.Messages.UnreadCommand.PARSER, extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(unreadMessage_);
unreadMessage_ = subBuilder.buildPartial();
}
bitField0_ |= 0x00004000;
break;
}
case 858: {
com.avos.avoscloud.Messages.ReadCommand.Builder subBuilder = null;
if (((bitField0_ & 0x00008000) == 0x00008000)) {
subBuilder = readMessage_.toBuilder();
}
readMessage_ = input.readMessage(com.avos.avoscloud.Messages.ReadCommand.PARSER, extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(readMessage_);
readMessage_ = subBuilder.buildPartial();
}
bitField0_ |= 0x00008000;
break;
}
case 866: {
com.avos.avoscloud.Messages.RcpCommand.Builder subBuilder = null;
if (((bitField0_ & 0x00010000) == 0x00010000)) {
subBuilder = rcpMessage_.toBuilder();
}
rcpMessage_ = input.readMessage(com.avos.avoscloud.Messages.RcpCommand.PARSER, extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(rcpMessage_);
rcpMessage_ = subBuilder.buildPartial();
}
bitField0_ |= 0x00010000;
break;
}
case 874: {
com.avos.avoscloud.Messages.LogsCommand.Builder subBuilder = null;
if (((bitField0_ & 0x00020000) == 0x00020000)) {
subBuilder = logsMessage_.toBuilder();
}
logsMessage_ = input.readMessage(com.avos.avoscloud.Messages.LogsCommand.PARSER, extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(logsMessage_);
logsMessage_ = subBuilder.buildPartial();
}
bitField0_ |= 0x00020000;
break;
}
case 882: {
com.avos.avoscloud.Messages.ConvCommand.Builder subBuilder = null;
if (((bitField0_ & 0x00040000) == 0x00040000)) {
subBuilder = convMessage_.toBuilder();
}
convMessage_ = input.readMessage(com.avos.avoscloud.Messages.ConvCommand.PARSER, extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(convMessage_);
convMessage_ = subBuilder.buildPartial();
}
bitField0_ |= 0x00040000;
break;
}
case 890: {
com.avos.avoscloud.Messages.RoomCommand.Builder subBuilder = null;
if (((bitField0_ & 0x00080000) == 0x00080000)) {
subBuilder = roomMessage_.toBuilder();
}
roomMessage_ = input.readMessage(com.avos.avoscloud.Messages.RoomCommand.PARSER, extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(roomMessage_);
roomMessage_ = subBuilder.buildPartial();
}
bitField0_ |= 0x00080000;
break;
}
case 898: {
com.avos.avoscloud.Messages.PresenceCommand.Builder subBuilder = null;
if (((bitField0_ & 0x00100000) == 0x00100000)) {
subBuilder = presenceMessage_.toBuilder();
}
presenceMessage_ = input.readMessage(com.avos.avoscloud.Messages.PresenceCommand.PARSER, extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(presenceMessage_);
presenceMessage_ = subBuilder.buildPartial();
}
bitField0_ |= 0x00100000;
break;
}
case 906: {
com.avos.avoscloud.Messages.ReportCommand.Builder subBuilder = null;
if (((bitField0_ & 0x00200000) == 0x00200000)) {
subBuilder = reportMessage_.toBuilder();
}
reportMessage_ = input.readMessage(com.avos.avoscloud.Messages.ReportCommand.PARSER, extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(reportMessage_);
reportMessage_ = subBuilder.buildPartial();
}
bitField0_ |= 0x00200000;
break;
}
case 914: {
com.avos.avoscloud.Messages.PatchCommand.Builder subBuilder = null;
if (((bitField0_ & 0x00400000) == 0x00400000)) {
subBuilder = patchMessage_.toBuilder();
}
patchMessage_ = input.readMessage(com.avos.avoscloud.Messages.PatchCommand.PARSER, extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(patchMessage_);
patchMessage_ = subBuilder.buildPartial();
}
bitField0_ |= 0x00400000;
break;
}
case 922: {
com.avos.avoscloud.Messages.PubsubCommand.Builder subBuilder = null;
if (((bitField0_ & 0x00800000) == 0x00800000)) {
subBuilder = pubsubMessage_.toBuilder();
}
pubsubMessage_ = input.readMessage(com.avos.avoscloud.Messages.PubsubCommand.PARSER, extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(pubsubMessage_);
pubsubMessage_ = subBuilder.buildPartial();
}
bitField0_ |= 0x00800000;
break;
}
case 930: {
com.avos.avoscloud.Messages.BlacklistCommand.Builder subBuilder = null;
if (((bitField0_ & 0x01000000) == 0x01000000)) {
subBuilder = blacklistMessage_.toBuilder();
}
blacklistMessage_ = input.readMessage(com.avos.avoscloud.Messages.BlacklistCommand.PARSER, extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(blacklistMessage_);
blacklistMessage_ = subBuilder.buildPartial();
}
bitField0_ |= 0x01000000;
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.avos.avoscloud.Messages.internal_static_com_avos_avoscloud_GenericCommand_descriptor;
}
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.avos.avoscloud.Messages.internal_static_com_avos_avoscloud_GenericCommand_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.avos.avoscloud.Messages.GenericCommand.class, com.avos.avoscloud.Messages.GenericCommand.Builder.class);
}
private int bitField0_;
public static final int CMD_FIELD_NUMBER = 1;
private int cmd_;
/**
* optional .com.avos.avoscloud.CommandType cmd = 1;
*/
public boolean hasCmd() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
* optional .com.avos.avoscloud.CommandType cmd = 1;
*/
public com.avos.avoscloud.Messages.CommandType getCmd() {
com.avos.avoscloud.Messages.CommandType result = com.avos.avoscloud.Messages.CommandType.valueOf(cmd_);
return result == null ? com.avos.avoscloud.Messages.CommandType.session : result;
}
public static final int OP_FIELD_NUMBER = 2;
private int op_;
/**
* optional .com.avos.avoscloud.OpType op = 2;
*/
public boolean hasOp() {
return ((bitField0_ & 0x00000002) == 0x00000002);
}
/**
* optional .com.avos.avoscloud.OpType op = 2;
*/
public com.avos.avoscloud.Messages.OpType getOp() {
com.avos.avoscloud.Messages.OpType result = com.avos.avoscloud.Messages.OpType.valueOf(op_);
return result == null ? com.avos.avoscloud.Messages.OpType.open : result;
}
public static final int APPID_FIELD_NUMBER = 3;
private volatile java.lang.Object appId_;
/**
* optional string appId = 3;
*/
public boolean hasAppId() {
return ((bitField0_ & 0x00000004) == 0x00000004);
}
/**
* optional string appId = 3;
*/
public java.lang.String getAppId() {
java.lang.Object ref = appId_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
appId_ = s;
}
return s;
}
}
/**
* optional string appId = 3;
*/
public com.google.protobuf.ByteString
getAppIdBytes() {
java.lang.Object ref = appId_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
appId_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int PEERID_FIELD_NUMBER = 4;
private volatile java.lang.Object peerId_;
/**
* optional string peerId = 4;
*/
public boolean hasPeerId() {
return ((bitField0_ & 0x00000008) == 0x00000008);
}
/**
* optional string peerId = 4;
*/
public java.lang.String getPeerId() {
java.lang.Object ref = peerId_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
peerId_ = s;
}
return s;
}
}
/**
* optional string peerId = 4;
*/
public com.google.protobuf.ByteString
getPeerIdBytes() {
java.lang.Object ref = peerId_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
peerId_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int I_FIELD_NUMBER = 5;
private int i_;
/**
* optional int32 i = 5;
*/
public boolean hasI() {
return ((bitField0_ & 0x00000010) == 0x00000010);
}
/**
* optional int32 i = 5;
*/
public int getI() {
return i_;
}
public static final int INSTALLATIONID_FIELD_NUMBER = 6;
private volatile java.lang.Object installationId_;
/**
* optional string installationId = 6;
*/
public boolean hasInstallationId() {
return ((bitField0_ & 0x00000020) == 0x00000020);
}
/**
* optional string installationId = 6;
*/
public java.lang.String getInstallationId() {
java.lang.Object ref = installationId_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
installationId_ = s;
}
return s;
}
}
/**
* optional string installationId = 6;
*/
public com.google.protobuf.ByteString
getInstallationIdBytes() {
java.lang.Object ref = installationId_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
installationId_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int PRIORITY_FIELD_NUMBER = 7;
private int priority_;
/**
* optional int32 priority = 7;
*/
public boolean hasPriority() {
return ((bitField0_ & 0x00000040) == 0x00000040);
}
/**
* optional int32 priority = 7;
*/
public int getPriority() {
return priority_;
}
public static final int SERVICE_FIELD_NUMBER = 8;
private int service_;
/**
* optional int32 service = 8;
*/
public boolean hasService() {
return ((bitField0_ & 0x00000080) == 0x00000080);
}
/**
* optional int32 service = 8;
*/
public int getService() {
return service_;
}
public static final int SERVERTS_FIELD_NUMBER = 9;
private long serverTs_;
/**
* optional int64 serverTs = 9;
*/
public boolean hasServerTs() {
return ((bitField0_ & 0x00000100) == 0x00000100);
}
/**
* optional int64 serverTs = 9;
*/
public long getServerTs() {
return serverTs_;
}
public static final int DATAMESSAGE_FIELD_NUMBER = 101;
private com.avos.avoscloud.Messages.DataCommand dataMessage_;
/**
* optional .com.avos.avoscloud.DataCommand dataMessage = 101;
*/
public boolean hasDataMessage() {
return ((bitField0_ & 0x00000200) == 0x00000200);
}
/**
* optional .com.avos.avoscloud.DataCommand dataMessage = 101;
*/
public com.avos.avoscloud.Messages.DataCommand getDataMessage() {
return dataMessage_ == null ? com.avos.avoscloud.Messages.DataCommand.getDefaultInstance() : dataMessage_;
}
/**
* optional .com.avos.avoscloud.DataCommand dataMessage = 101;
*/
public com.avos.avoscloud.Messages.DataCommandOrBuilder getDataMessageOrBuilder() {
return dataMessage_ == null ? com.avos.avoscloud.Messages.DataCommand.getDefaultInstance() : dataMessage_;
}
public static final int SESSIONMESSAGE_FIELD_NUMBER = 102;
private com.avos.avoscloud.Messages.SessionCommand sessionMessage_;
/**
* optional .com.avos.avoscloud.SessionCommand sessionMessage = 102;
*/
public boolean hasSessionMessage() {
return ((bitField0_ & 0x00000400) == 0x00000400);
}
/**
* optional .com.avos.avoscloud.SessionCommand sessionMessage = 102;
*/
public com.avos.avoscloud.Messages.SessionCommand getSessionMessage() {
return sessionMessage_ == null ? com.avos.avoscloud.Messages.SessionCommand.getDefaultInstance() : sessionMessage_;
}
/**
* optional .com.avos.avoscloud.SessionCommand sessionMessage = 102;
*/
public com.avos.avoscloud.Messages.SessionCommandOrBuilder getSessionMessageOrBuilder() {
return sessionMessage_ == null ? com.avos.avoscloud.Messages.SessionCommand.getDefaultInstance() : sessionMessage_;
}
public static final int ERRORMESSAGE_FIELD_NUMBER = 103;
private com.avos.avoscloud.Messages.ErrorCommand errorMessage_;
/**
* optional .com.avos.avoscloud.ErrorCommand errorMessage = 103;
*/
public boolean hasErrorMessage() {
return ((bitField0_ & 0x00000800) == 0x00000800);
}
/**
* optional .com.avos.avoscloud.ErrorCommand errorMessage = 103;
*/
public com.avos.avoscloud.Messages.ErrorCommand getErrorMessage() {
return errorMessage_ == null ? com.avos.avoscloud.Messages.ErrorCommand.getDefaultInstance() : errorMessage_;
}
/**
* optional .com.avos.avoscloud.ErrorCommand errorMessage = 103;
*/
public com.avos.avoscloud.Messages.ErrorCommandOrBuilder getErrorMessageOrBuilder() {
return errorMessage_ == null ? com.avos.avoscloud.Messages.ErrorCommand.getDefaultInstance() : errorMessage_;
}
public static final int DIRECTMESSAGE_FIELD_NUMBER = 104;
private com.avos.avoscloud.Messages.DirectCommand directMessage_;
/**
* optional .com.avos.avoscloud.DirectCommand directMessage = 104;
*/
public boolean hasDirectMessage() {
return ((bitField0_ & 0x00001000) == 0x00001000);
}
/**
* optional .com.avos.avoscloud.DirectCommand directMessage = 104;
*/
public com.avos.avoscloud.Messages.DirectCommand getDirectMessage() {
return directMessage_ == null ? com.avos.avoscloud.Messages.DirectCommand.getDefaultInstance() : directMessage_;
}
/**
* optional .com.avos.avoscloud.DirectCommand directMessage = 104;
*/
public com.avos.avoscloud.Messages.DirectCommandOrBuilder getDirectMessageOrBuilder() {
return directMessage_ == null ? com.avos.avoscloud.Messages.DirectCommand.getDefaultInstance() : directMessage_;
}
public static final int ACKMESSAGE_FIELD_NUMBER = 105;
private com.avos.avoscloud.Messages.AckCommand ackMessage_;
/**
* optional .com.avos.avoscloud.AckCommand ackMessage = 105;
*/
public boolean hasAckMessage() {
return ((bitField0_ & 0x00002000) == 0x00002000);
}
/**
* optional .com.avos.avoscloud.AckCommand ackMessage = 105;
*/
public com.avos.avoscloud.Messages.AckCommand getAckMessage() {
return ackMessage_ == null ? com.avos.avoscloud.Messages.AckCommand.getDefaultInstance() : ackMessage_;
}
/**
* optional .com.avos.avoscloud.AckCommand ackMessage = 105;
*/
public com.avos.avoscloud.Messages.AckCommandOrBuilder getAckMessageOrBuilder() {
return ackMessage_ == null ? com.avos.avoscloud.Messages.AckCommand.getDefaultInstance() : ackMessage_;
}
public static final int UNREADMESSAGE_FIELD_NUMBER = 106;
private com.avos.avoscloud.Messages.UnreadCommand unreadMessage_;
/**
* optional .com.avos.avoscloud.UnreadCommand unreadMessage = 106;
*/
public boolean hasUnreadMessage() {
return ((bitField0_ & 0x00004000) == 0x00004000);
}
/**
* optional .com.avos.avoscloud.UnreadCommand unreadMessage = 106;
*/
public com.avos.avoscloud.Messages.UnreadCommand getUnreadMessage() {
return unreadMessage_ == null ? com.avos.avoscloud.Messages.UnreadCommand.getDefaultInstance() : unreadMessage_;
}
/**
* optional .com.avos.avoscloud.UnreadCommand unreadMessage = 106;
*/
public com.avos.avoscloud.Messages.UnreadCommandOrBuilder getUnreadMessageOrBuilder() {
return unreadMessage_ == null ? com.avos.avoscloud.Messages.UnreadCommand.getDefaultInstance() : unreadMessage_;
}
public static final int READMESSAGE_FIELD_NUMBER = 107;
private com.avos.avoscloud.Messages.ReadCommand readMessage_;
/**
* optional .com.avos.avoscloud.ReadCommand readMessage = 107;
*/
public boolean hasReadMessage() {
return ((bitField0_ & 0x00008000) == 0x00008000);
}
/**
* optional .com.avos.avoscloud.ReadCommand readMessage = 107;
*/
public com.avos.avoscloud.Messages.ReadCommand getReadMessage() {
return readMessage_ == null ? com.avos.avoscloud.Messages.ReadCommand.getDefaultInstance() : readMessage_;
}
/**
* optional .com.avos.avoscloud.ReadCommand readMessage = 107;
*/
public com.avos.avoscloud.Messages.ReadCommandOrBuilder getReadMessageOrBuilder() {
return readMessage_ == null ? com.avos.avoscloud.Messages.ReadCommand.getDefaultInstance() : readMessage_;
}
public static final int RCPMESSAGE_FIELD_NUMBER = 108;
private com.avos.avoscloud.Messages.RcpCommand rcpMessage_;
/**
* optional .com.avos.avoscloud.RcpCommand rcpMessage = 108;
*/
public boolean hasRcpMessage() {
return ((bitField0_ & 0x00010000) == 0x00010000);
}
/**
* optional .com.avos.avoscloud.RcpCommand rcpMessage = 108;
*/
public com.avos.avoscloud.Messages.RcpCommand getRcpMessage() {
return rcpMessage_ == null ? com.avos.avoscloud.Messages.RcpCommand.getDefaultInstance() : rcpMessage_;
}
/**
* optional .com.avos.avoscloud.RcpCommand rcpMessage = 108;
*/
public com.avos.avoscloud.Messages.RcpCommandOrBuilder getRcpMessageOrBuilder() {
return rcpMessage_ == null ? com.avos.avoscloud.Messages.RcpCommand.getDefaultInstance() : rcpMessage_;
}
public static final int LOGSMESSAGE_FIELD_NUMBER = 109;
private com.avos.avoscloud.Messages.LogsCommand logsMessage_;
/**
* optional .com.avos.avoscloud.LogsCommand logsMessage = 109;
*/
public boolean hasLogsMessage() {
return ((bitField0_ & 0x00020000) == 0x00020000);
}
/**
* optional .com.avos.avoscloud.LogsCommand logsMessage = 109;
*/
public com.avos.avoscloud.Messages.LogsCommand getLogsMessage() {
return logsMessage_ == null ? com.avos.avoscloud.Messages.LogsCommand.getDefaultInstance() : logsMessage_;
}
/**
* optional .com.avos.avoscloud.LogsCommand logsMessage = 109;
*/
public com.avos.avoscloud.Messages.LogsCommandOrBuilder getLogsMessageOrBuilder() {
return logsMessage_ == null ? com.avos.avoscloud.Messages.LogsCommand.getDefaultInstance() : logsMessage_;
}
public static final int CONVMESSAGE_FIELD_NUMBER = 110;
private com.avos.avoscloud.Messages.ConvCommand convMessage_;
/**
* optional .com.avos.avoscloud.ConvCommand convMessage = 110;
*/
public boolean hasConvMessage() {
return ((bitField0_ & 0x00040000) == 0x00040000);
}
/**
* optional .com.avos.avoscloud.ConvCommand convMessage = 110;
*/
public com.avos.avoscloud.Messages.ConvCommand getConvMessage() {
return convMessage_ == null ? com.avos.avoscloud.Messages.ConvCommand.getDefaultInstance() : convMessage_;
}
/**
* optional .com.avos.avoscloud.ConvCommand convMessage = 110;
*/
public com.avos.avoscloud.Messages.ConvCommandOrBuilder getConvMessageOrBuilder() {
return convMessage_ == null ? com.avos.avoscloud.Messages.ConvCommand.getDefaultInstance() : convMessage_;
}
public static final int ROOMMESSAGE_FIELD_NUMBER = 111;
private com.avos.avoscloud.Messages.RoomCommand roomMessage_;
/**
* optional .com.avos.avoscloud.RoomCommand roomMessage = 111;
*/
public boolean hasRoomMessage() {
return ((bitField0_ & 0x00080000) == 0x00080000);
}
/**
* optional .com.avos.avoscloud.RoomCommand roomMessage = 111;
*/
public com.avos.avoscloud.Messages.RoomCommand getRoomMessage() {
return roomMessage_ == null ? com.avos.avoscloud.Messages.RoomCommand.getDefaultInstance() : roomMessage_;
}
/**
* optional .com.avos.avoscloud.RoomCommand roomMessage = 111;
*/
public com.avos.avoscloud.Messages.RoomCommandOrBuilder getRoomMessageOrBuilder() {
return roomMessage_ == null ? com.avos.avoscloud.Messages.RoomCommand.getDefaultInstance() : roomMessage_;
}
public static final int PRESENCEMESSAGE_FIELD_NUMBER = 112;
private com.avos.avoscloud.Messages.PresenceCommand presenceMessage_;
/**
* optional .com.avos.avoscloud.PresenceCommand presenceMessage = 112;
*/
public boolean hasPresenceMessage() {
return ((bitField0_ & 0x00100000) == 0x00100000);
}
/**
* optional .com.avos.avoscloud.PresenceCommand presenceMessage = 112;
*/
public com.avos.avoscloud.Messages.PresenceCommand getPresenceMessage() {
return presenceMessage_ == null ? com.avos.avoscloud.Messages.PresenceCommand.getDefaultInstance() : presenceMessage_;
}
/**
* optional .com.avos.avoscloud.PresenceCommand presenceMessage = 112;
*/
public com.avos.avoscloud.Messages.PresenceCommandOrBuilder getPresenceMessageOrBuilder() {
return presenceMessage_ == null ? com.avos.avoscloud.Messages.PresenceCommand.getDefaultInstance() : presenceMessage_;
}
public static final int REPORTMESSAGE_FIELD_NUMBER = 113;
private com.avos.avoscloud.Messages.ReportCommand reportMessage_;
/**
* optional .com.avos.avoscloud.ReportCommand reportMessage = 113;
*/
public boolean hasReportMessage() {
return ((bitField0_ & 0x00200000) == 0x00200000);
}
/**
* optional .com.avos.avoscloud.ReportCommand reportMessage = 113;
*/
public com.avos.avoscloud.Messages.ReportCommand getReportMessage() {
return reportMessage_ == null ? com.avos.avoscloud.Messages.ReportCommand.getDefaultInstance() : reportMessage_;
}
/**
* optional .com.avos.avoscloud.ReportCommand reportMessage = 113;
*/
public com.avos.avoscloud.Messages.ReportCommandOrBuilder getReportMessageOrBuilder() {
return reportMessage_ == null ? com.avos.avoscloud.Messages.ReportCommand.getDefaultInstance() : reportMessage_;
}
public static final int PATCHMESSAGE_FIELD_NUMBER = 114;
private com.avos.avoscloud.Messages.PatchCommand patchMessage_;
/**
* optional .com.avos.avoscloud.PatchCommand patchMessage = 114;
*/
public boolean hasPatchMessage() {
return ((bitField0_ & 0x00400000) == 0x00400000);
}
/**
* optional .com.avos.avoscloud.PatchCommand patchMessage = 114;
*/
public com.avos.avoscloud.Messages.PatchCommand getPatchMessage() {
return patchMessage_ == null ? com.avos.avoscloud.Messages.PatchCommand.getDefaultInstance() : patchMessage_;
}
/**
* optional .com.avos.avoscloud.PatchCommand patchMessage = 114;
*/
public com.avos.avoscloud.Messages.PatchCommandOrBuilder getPatchMessageOrBuilder() {
return patchMessage_ == null ? com.avos.avoscloud.Messages.PatchCommand.getDefaultInstance() : patchMessage_;
}
public static final int PUBSUBMESSAGE_FIELD_NUMBER = 115;
private com.avos.avoscloud.Messages.PubsubCommand pubsubMessage_;
/**
* optional .com.avos.avoscloud.PubsubCommand pubsubMessage = 115;
*/
public boolean hasPubsubMessage() {
return ((bitField0_ & 0x00800000) == 0x00800000);
}
/**
* optional .com.avos.avoscloud.PubsubCommand pubsubMessage = 115;
*/
public com.avos.avoscloud.Messages.PubsubCommand getPubsubMessage() {
return pubsubMessage_ == null ? com.avos.avoscloud.Messages.PubsubCommand.getDefaultInstance() : pubsubMessage_;
}
/**
* optional .com.avos.avoscloud.PubsubCommand pubsubMessage = 115;
*/
public com.avos.avoscloud.Messages.PubsubCommandOrBuilder getPubsubMessageOrBuilder() {
return pubsubMessage_ == null ? com.avos.avoscloud.Messages.PubsubCommand.getDefaultInstance() : pubsubMessage_;
}
public static final int BLACKLISTMESSAGE_FIELD_NUMBER = 116;
private com.avos.avoscloud.Messages.BlacklistCommand blacklistMessage_;
/**
* optional .com.avos.avoscloud.BlacklistCommand blacklistMessage = 116;
*/
public boolean hasBlacklistMessage() {
return ((bitField0_ & 0x01000000) == 0x01000000);
}
/**
* optional .com.avos.avoscloud.BlacklistCommand blacklistMessage = 116;
*/
public com.avos.avoscloud.Messages.BlacklistCommand getBlacklistMessage() {
return blacklistMessage_ == null ? com.avos.avoscloud.Messages.BlacklistCommand.getDefaultInstance() : blacklistMessage_;
}
/**
* optional .com.avos.avoscloud.BlacklistCommand blacklistMessage = 116;
*/
public com.avos.avoscloud.Messages.BlacklistCommandOrBuilder getBlacklistMessageOrBuilder() {
return blacklistMessage_ == null ? com.avos.avoscloud.Messages.BlacklistCommand.getDefaultInstance() : blacklistMessage_;
}
private byte memoizedIsInitialized = -1;
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
if (hasDataMessage()) {
if (!getDataMessage().isInitialized()) {
memoizedIsInitialized = 0;
return false;
}
}
if (hasErrorMessage()) {
if (!getErrorMessage().isInitialized()) {
memoizedIsInitialized = 0;
return false;
}
}
if (hasUnreadMessage()) {
if (!getUnreadMessage().isInitialized()) {
memoizedIsInitialized = 0;
return false;
}
}
if (hasReadMessage()) {
if (!getReadMessage().isInitialized()) {
memoizedIsInitialized = 0;
return false;
}
}
if (hasConvMessage()) {
if (!getConvMessage().isInitialized()) {
memoizedIsInitialized = 0;
return false;
}
}
if (hasPubsubMessage()) {
if (!getPubsubMessage().isInitialized()) {
memoizedIsInitialized = 0;
return false;
}
}
if (hasBlacklistMessage()) {
if (!getBlacklistMessage().isInitialized()) {
memoizedIsInitialized = 0;
return false;
}
}
memoizedIsInitialized = 1;
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (((bitField0_ & 0x00000001) == 0x00000001)) {
output.writeEnum(1, cmd_);
}
if (((bitField0_ & 0x00000002) == 0x00000002)) {
output.writeEnum(2, op_);
}
if (((bitField0_ & 0x00000004) == 0x00000004)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 3, appId_);
}
if (((bitField0_ & 0x00000008) == 0x00000008)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 4, peerId_);
}
if (((bitField0_ & 0x00000010) == 0x00000010)) {
output.writeInt32(5, i_);
}
if (((bitField0_ & 0x00000020) == 0x00000020)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 6, installationId_);
}
if (((bitField0_ & 0x00000040) == 0x00000040)) {
output.writeInt32(7, priority_);
}
if (((bitField0_ & 0x00000080) == 0x00000080)) {
output.writeInt32(8, service_);
}
if (((bitField0_ & 0x00000100) == 0x00000100)) {
output.writeInt64(9, serverTs_);
}
if (((bitField0_ & 0x00000200) == 0x00000200)) {
output.writeMessage(101, getDataMessage());
}
if (((bitField0_ & 0x00000400) == 0x00000400)) {
output.writeMessage(102, getSessionMessage());
}
if (((bitField0_ & 0x00000800) == 0x00000800)) {
output.writeMessage(103, getErrorMessage());
}
if (((bitField0_ & 0x00001000) == 0x00001000)) {
output.writeMessage(104, getDirectMessage());
}
if (((bitField0_ & 0x00002000) == 0x00002000)) {
output.writeMessage(105, getAckMessage());
}
if (((bitField0_ & 0x00004000) == 0x00004000)) {
output.writeMessage(106, getUnreadMessage());
}
if (((bitField0_ & 0x00008000) == 0x00008000)) {
output.writeMessage(107, getReadMessage());
}
if (((bitField0_ & 0x00010000) == 0x00010000)) {
output.writeMessage(108, getRcpMessage());
}
if (((bitField0_ & 0x00020000) == 0x00020000)) {
output.writeMessage(109, getLogsMessage());
}
if (((bitField0_ & 0x00040000) == 0x00040000)) {
output.writeMessage(110, getConvMessage());
}
if (((bitField0_ & 0x00080000) == 0x00080000)) {
output.writeMessage(111, getRoomMessage());
}
if (((bitField0_ & 0x00100000) == 0x00100000)) {
output.writeMessage(112, getPresenceMessage());
}
if (((bitField0_ & 0x00200000) == 0x00200000)) {
output.writeMessage(113, getReportMessage());
}
if (((bitField0_ & 0x00400000) == 0x00400000)) {
output.writeMessage(114, getPatchMessage());
}
if (((bitField0_ & 0x00800000) == 0x00800000)) {
output.writeMessage(115, getPubsubMessage());
}
if (((bitField0_ & 0x01000000) == 0x01000000)) {
output.writeMessage(116, getBlacklistMessage());
}
unknownFields.writeTo(output);
}
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (((bitField0_ & 0x00000001) == 0x00000001)) {
size += com.google.protobuf.CodedOutputStream
.computeEnumSize(1, cmd_);
}
if (((bitField0_ & 0x00000002) == 0x00000002)) {
size += com.google.protobuf.CodedOutputStream
.computeEnumSize(2, op_);
}
if (((bitField0_ & 0x00000004) == 0x00000004)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(3, appId_);
}
if (((bitField0_ & 0x00000008) == 0x00000008)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(4, peerId_);
}
if (((bitField0_ & 0x00000010) == 0x00000010)) {
size += com.google.protobuf.CodedOutputStream
.computeInt32Size(5, i_);
}
if (((bitField0_ & 0x00000020) == 0x00000020)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(6, installationId_);
}
if (((bitField0_ & 0x00000040) == 0x00000040)) {
size += com.google.protobuf.CodedOutputStream
.computeInt32Size(7, priority_);
}
if (((bitField0_ & 0x00000080) == 0x00000080)) {
size += com.google.protobuf.CodedOutputStream
.computeInt32Size(8, service_);
}
if (((bitField0_ & 0x00000100) == 0x00000100)) {
size += com.google.protobuf.CodedOutputStream
.computeInt64Size(9, serverTs_);
}
if (((bitField0_ & 0x00000200) == 0x00000200)) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(101, getDataMessage());
}
if (((bitField0_ & 0x00000400) == 0x00000400)) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(102, getSessionMessage());
}
if (((bitField0_ & 0x00000800) == 0x00000800)) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(103, getErrorMessage());
}
if (((bitField0_ & 0x00001000) == 0x00001000)) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(104, getDirectMessage());
}
if (((bitField0_ & 0x00002000) == 0x00002000)) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(105, getAckMessage());
}
if (((bitField0_ & 0x00004000) == 0x00004000)) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(106, getUnreadMessage());
}
if (((bitField0_ & 0x00008000) == 0x00008000)) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(107, getReadMessage());
}
if (((bitField0_ & 0x00010000) == 0x00010000)) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(108, getRcpMessage());
}
if (((bitField0_ & 0x00020000) == 0x00020000)) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(109, getLogsMessage());
}
if (((bitField0_ & 0x00040000) == 0x00040000)) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(110, getConvMessage());
}
if (((bitField0_ & 0x00080000) == 0x00080000)) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(111, getRoomMessage());
}
if (((bitField0_ & 0x00100000) == 0x00100000)) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(112, getPresenceMessage());
}
if (((bitField0_ & 0x00200000) == 0x00200000)) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(113, getReportMessage());
}
if (((bitField0_ & 0x00400000) == 0x00400000)) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(114, getPatchMessage());
}
if (((bitField0_ & 0x00800000) == 0x00800000)) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(115, getPubsubMessage());
}
if (((bitField0_ & 0x01000000) == 0x01000000)) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(116, getBlacklistMessage());
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof com.avos.avoscloud.Messages.GenericCommand)) {
return super.equals(obj);
}
com.avos.avoscloud.Messages.GenericCommand other = (com.avos.avoscloud.Messages.GenericCommand) obj;
boolean result = true;
result = result && (hasCmd() == other.hasCmd());
if (hasCmd()) {
result = result && cmd_ == other.cmd_;
}
result = result && (hasOp() == other.hasOp());
if (hasOp()) {
result = result && op_ == other.op_;
}
result = result && (hasAppId() == other.hasAppId());
if (hasAppId()) {
result = result && getAppId()
.equals(other.getAppId());
}
result = result && (hasPeerId() == other.hasPeerId());
if (hasPeerId()) {
result = result && getPeerId()
.equals(other.getPeerId());
}
result = result && (hasI() == other.hasI());
if (hasI()) {
result = result && (getI()
== other.getI());
}
result = result && (hasInstallationId() == other.hasInstallationId());
if (hasInstallationId()) {
result = result && getInstallationId()
.equals(other.getInstallationId());
}
result = result && (hasPriority() == other.hasPriority());
if (hasPriority()) {
result = result && (getPriority()
== other.getPriority());
}
result = result && (hasService() == other.hasService());
if (hasService()) {
result = result && (getService()
== other.getService());
}
result = result && (hasServerTs() == other.hasServerTs());
if (hasServerTs()) {
result = result && (getServerTs()
== other.getServerTs());
}
result = result && (hasDataMessage() == other.hasDataMessage());
if (hasDataMessage()) {
result = result && getDataMessage()
.equals(other.getDataMessage());
}
result = result && (hasSessionMessage() == other.hasSessionMessage());
if (hasSessionMessage()) {
result = result && getSessionMessage()
.equals(other.getSessionMessage());
}
result = result && (hasErrorMessage() == other.hasErrorMessage());
if (hasErrorMessage()) {
result = result && getErrorMessage()
.equals(other.getErrorMessage());
}
result = result && (hasDirectMessage() == other.hasDirectMessage());
if (hasDirectMessage()) {
result = result && getDirectMessage()
.equals(other.getDirectMessage());
}
result = result && (hasAckMessage() == other.hasAckMessage());
if (hasAckMessage()) {
result = result && getAckMessage()
.equals(other.getAckMessage());
}
result = result && (hasUnreadMessage() == other.hasUnreadMessage());
if (hasUnreadMessage()) {
result = result && getUnreadMessage()
.equals(other.getUnreadMessage());
}
result = result && (hasReadMessage() == other.hasReadMessage());
if (hasReadMessage()) {
result = result && getReadMessage()
.equals(other.getReadMessage());
}
result = result && (hasRcpMessage() == other.hasRcpMessage());
if (hasRcpMessage()) {
result = result && getRcpMessage()
.equals(other.getRcpMessage());
}
result = result && (hasLogsMessage() == other.hasLogsMessage());
if (hasLogsMessage()) {
result = result && getLogsMessage()
.equals(other.getLogsMessage());
}
result = result && (hasConvMessage() == other.hasConvMessage());
if (hasConvMessage()) {
result = result && getConvMessage()
.equals(other.getConvMessage());
}
result = result && (hasRoomMessage() == other.hasRoomMessage());
if (hasRoomMessage()) {
result = result && getRoomMessage()
.equals(other.getRoomMessage());
}
result = result && (hasPresenceMessage() == other.hasPresenceMessage());
if (hasPresenceMessage()) {
result = result && getPresenceMessage()
.equals(other.getPresenceMessage());
}
result = result && (hasReportMessage() == other.hasReportMessage());
if (hasReportMessage()) {
result = result && getReportMessage()
.equals(other.getReportMessage());
}
result = result && (hasPatchMessage() == other.hasPatchMessage());
if (hasPatchMessage()) {
result = result && getPatchMessage()
.equals(other.getPatchMessage());
}
result = result && (hasPubsubMessage() == other.hasPubsubMessage());
if (hasPubsubMessage()) {
result = result && getPubsubMessage()
.equals(other.getPubsubMessage());
}
result = result && (hasBlacklistMessage() == other.hasBlacklistMessage());
if (hasBlacklistMessage()) {
result = result && getBlacklistMessage()
.equals(other.getBlacklistMessage());
}
result = result && unknownFields.equals(other.unknownFields);
return result;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (hasCmd()) {
hash = (37 * hash) + CMD_FIELD_NUMBER;
hash = (53 * hash) + cmd_;
}
if (hasOp()) {
hash = (37 * hash) + OP_FIELD_NUMBER;
hash = (53 * hash) + op_;
}
if (hasAppId()) {
hash = (37 * hash) + APPID_FIELD_NUMBER;
hash = (53 * hash) + getAppId().hashCode();
}
if (hasPeerId()) {
hash = (37 * hash) + PEERID_FIELD_NUMBER;
hash = (53 * hash) + getPeerId().hashCode();
}
if (hasI()) {
hash = (37 * hash) + I_FIELD_NUMBER;
hash = (53 * hash) + getI();
}
if (hasInstallationId()) {
hash = (37 * hash) + INSTALLATIONID_FIELD_NUMBER;
hash = (53 * hash) + getInstallationId().hashCode();
}
if (hasPriority()) {
hash = (37 * hash) + PRIORITY_FIELD_NUMBER;
hash = (53 * hash) + getPriority();
}
if (hasService()) {
hash = (37 * hash) + SERVICE_FIELD_NUMBER;
hash = (53 * hash) + getService();
}
if (hasServerTs()) {
hash = (37 * hash) + SERVERTS_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getServerTs());
}
if (hasDataMessage()) {
hash = (37 * hash) + DATAMESSAGE_FIELD_NUMBER;
hash = (53 * hash) + getDataMessage().hashCode();
}
if (hasSessionMessage()) {
hash = (37 * hash) + SESSIONMESSAGE_FIELD_NUMBER;
hash = (53 * hash) + getSessionMessage().hashCode();
}
if (hasErrorMessage()) {
hash = (37 * hash) + ERRORMESSAGE_FIELD_NUMBER;
hash = (53 * hash) + getErrorMessage().hashCode();
}
if (hasDirectMessage()) {
hash = (37 * hash) + DIRECTMESSAGE_FIELD_NUMBER;
hash = (53 * hash) + getDirectMessage().hashCode();
}
if (hasAckMessage()) {
hash = (37 * hash) + ACKMESSAGE_FIELD_NUMBER;
hash = (53 * hash) + getAckMessage().hashCode();
}
if (hasUnreadMessage()) {
hash = (37 * hash) + UNREADMESSAGE_FIELD_NUMBER;
hash = (53 * hash) + getUnreadMessage().hashCode();
}
if (hasReadMessage()) {
hash = (37 * hash) + READMESSAGE_FIELD_NUMBER;
hash = (53 * hash) + getReadMessage().hashCode();
}
if (hasRcpMessage()) {
hash = (37 * hash) + RCPMESSAGE_FIELD_NUMBER;
hash = (53 * hash) + getRcpMessage().hashCode();
}
if (hasLogsMessage()) {
hash = (37 * hash) + LOGSMESSAGE_FIELD_NUMBER;
hash = (53 * hash) + getLogsMessage().hashCode();
}
if (hasConvMessage()) {
hash = (37 * hash) + CONVMESSAGE_FIELD_NUMBER;
hash = (53 * hash) + getConvMessage().hashCode();
}
if (hasRoomMessage()) {
hash = (37 * hash) + ROOMMESSAGE_FIELD_NUMBER;
hash = (53 * hash) + getRoomMessage().hashCode();
}
if (hasPresenceMessage()) {
hash = (37 * hash) + PRESENCEMESSAGE_FIELD_NUMBER;
hash = (53 * hash) + getPresenceMessage().hashCode();
}
if (hasReportMessage()) {
hash = (37 * hash) + REPORTMESSAGE_FIELD_NUMBER;
hash = (53 * hash) + getReportMessage().hashCode();
}
if (hasPatchMessage()) {
hash = (37 * hash) + PATCHMESSAGE_FIELD_NUMBER;
hash = (53 * hash) + getPatchMessage().hashCode();
}
if (hasPubsubMessage()) {
hash = (37 * hash) + PUBSUBMESSAGE_FIELD_NUMBER;
hash = (53 * hash) + getPubsubMessage().hashCode();
}
if (hasBlacklistMessage()) {
hash = (37 * hash) + BLACKLISTMESSAGE_FIELD_NUMBER;
hash = (53 * hash) + getBlacklistMessage().hashCode();
}
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static com.avos.avoscloud.Messages.GenericCommand parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.avos.avoscloud.Messages.GenericCommand parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.avos.avoscloud.Messages.GenericCommand parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.avos.avoscloud.Messages.GenericCommand parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.avos.avoscloud.Messages.GenericCommand parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.avos.avoscloud.Messages.GenericCommand parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.avos.avoscloud.Messages.GenericCommand parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.avos.avoscloud.Messages.GenericCommand parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static com.avos.avoscloud.Messages.GenericCommand parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static com.avos.avoscloud.Messages.GenericCommand parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static com.avos.avoscloud.Messages.GenericCommand parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.avos.avoscloud.Messages.GenericCommand parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(com.avos.avoscloud.Messages.GenericCommand prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code com.avos.avoscloud.GenericCommand}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:com.avos.avoscloud.GenericCommand)
com.avos.avoscloud.Messages.GenericCommandOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.avos.avoscloud.Messages.internal_static_com_avos_avoscloud_GenericCommand_descriptor;
}
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.avos.avoscloud.Messages.internal_static_com_avos_avoscloud_GenericCommand_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.avos.avoscloud.Messages.GenericCommand.class, com.avos.avoscloud.Messages.GenericCommand.Builder.class);
}
// Construct using com.avos.avoscloud.Messages.GenericCommand.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
getDataMessageFieldBuilder();
getSessionMessageFieldBuilder();
getErrorMessageFieldBuilder();
getDirectMessageFieldBuilder();
getAckMessageFieldBuilder();
getUnreadMessageFieldBuilder();
getReadMessageFieldBuilder();
getRcpMessageFieldBuilder();
getLogsMessageFieldBuilder();
getConvMessageFieldBuilder();
getRoomMessageFieldBuilder();
getPresenceMessageFieldBuilder();
getReportMessageFieldBuilder();
getPatchMessageFieldBuilder();
getPubsubMessageFieldBuilder();
getBlacklistMessageFieldBuilder();
}
}
public Builder clear() {
super.clear();
cmd_ = 0;
bitField0_ = (bitField0_ & ~0x00000001);
op_ = 1;
bitField0_ = (bitField0_ & ~0x00000002);
appId_ = "";
bitField0_ = (bitField0_ & ~0x00000004);
peerId_ = "";
bitField0_ = (bitField0_ & ~0x00000008);
i_ = 0;
bitField0_ = (bitField0_ & ~0x00000010);
installationId_ = "";
bitField0_ = (bitField0_ & ~0x00000020);
priority_ = 0;
bitField0_ = (bitField0_ & ~0x00000040);
service_ = 0;
bitField0_ = (bitField0_ & ~0x00000080);
serverTs_ = 0L;
bitField0_ = (bitField0_ & ~0x00000100);
if (dataMessageBuilder_ == null) {
dataMessage_ = null;
} else {
dataMessageBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000200);
if (sessionMessageBuilder_ == null) {
sessionMessage_ = null;
} else {
sessionMessageBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000400);
if (errorMessageBuilder_ == null) {
errorMessage_ = null;
} else {
errorMessageBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000800);
if (directMessageBuilder_ == null) {
directMessage_ = null;
} else {
directMessageBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00001000);
if (ackMessageBuilder_ == null) {
ackMessage_ = null;
} else {
ackMessageBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00002000);
if (unreadMessageBuilder_ == null) {
unreadMessage_ = null;
} else {
unreadMessageBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00004000);
if (readMessageBuilder_ == null) {
readMessage_ = null;
} else {
readMessageBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00008000);
if (rcpMessageBuilder_ == null) {
rcpMessage_ = null;
} else {
rcpMessageBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00010000);
if (logsMessageBuilder_ == null) {
logsMessage_ = null;
} else {
logsMessageBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00020000);
if (convMessageBuilder_ == null) {
convMessage_ = null;
} else {
convMessageBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00040000);
if (roomMessageBuilder_ == null) {
roomMessage_ = null;
} else {
roomMessageBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00080000);
if (presenceMessageBuilder_ == null) {
presenceMessage_ = null;
} else {
presenceMessageBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00100000);
if (reportMessageBuilder_ == null) {
reportMessage_ = null;
} else {
reportMessageBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00200000);
if (patchMessageBuilder_ == null) {
patchMessage_ = null;
} else {
patchMessageBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00400000);
if (pubsubMessageBuilder_ == null) {
pubsubMessage_ = null;
} else {
pubsubMessageBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00800000);
if (blacklistMessageBuilder_ == null) {
blacklistMessage_ = null;
} else {
blacklistMessageBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x01000000);
return this;
}
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return com.avos.avoscloud.Messages.internal_static_com_avos_avoscloud_GenericCommand_descriptor;
}
public com.avos.avoscloud.Messages.GenericCommand getDefaultInstanceForType() {
return com.avos.avoscloud.Messages.GenericCommand.getDefaultInstance();
}
public com.avos.avoscloud.Messages.GenericCommand build() {
com.avos.avoscloud.Messages.GenericCommand result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
public com.avos.avoscloud.Messages.GenericCommand buildPartial() {
com.avos.avoscloud.Messages.GenericCommand result = new com.avos.avoscloud.Messages.GenericCommand(this);
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (((from_bitField0_ & 0x00000001) == 0x00000001)) {
to_bitField0_ |= 0x00000001;
}
result.cmd_ = cmd_;
if (((from_bitField0_ & 0x00000002) == 0x00000002)) {
to_bitField0_ |= 0x00000002;
}
result.op_ = op_;
if (((from_bitField0_ & 0x00000004) == 0x00000004)) {
to_bitField0_ |= 0x00000004;
}
result.appId_ = appId_;
if (((from_bitField0_ & 0x00000008) == 0x00000008)) {
to_bitField0_ |= 0x00000008;
}
result.peerId_ = peerId_;
if (((from_bitField0_ & 0x00000010) == 0x00000010)) {
to_bitField0_ |= 0x00000010;
}
result.i_ = i_;
if (((from_bitField0_ & 0x00000020) == 0x00000020)) {
to_bitField0_ |= 0x00000020;
}
result.installationId_ = installationId_;
if (((from_bitField0_ & 0x00000040) == 0x00000040)) {
to_bitField0_ |= 0x00000040;
}
result.priority_ = priority_;
if (((from_bitField0_ & 0x00000080) == 0x00000080)) {
to_bitField0_ |= 0x00000080;
}
result.service_ = service_;
if (((from_bitField0_ & 0x00000100) == 0x00000100)) {
to_bitField0_ |= 0x00000100;
}
result.serverTs_ = serverTs_;
if (((from_bitField0_ & 0x00000200) == 0x00000200)) {
to_bitField0_ |= 0x00000200;
}
if (dataMessageBuilder_ == null) {
result.dataMessage_ = dataMessage_;
} else {
result.dataMessage_ = dataMessageBuilder_.build();
}
if (((from_bitField0_ & 0x00000400) == 0x00000400)) {
to_bitField0_ |= 0x00000400;
}
if (sessionMessageBuilder_ == null) {
result.sessionMessage_ = sessionMessage_;
} else {
result.sessionMessage_ = sessionMessageBuilder_.build();
}
if (((from_bitField0_ & 0x00000800) == 0x00000800)) {
to_bitField0_ |= 0x00000800;
}
if (errorMessageBuilder_ == null) {
result.errorMessage_ = errorMessage_;
} else {
result.errorMessage_ = errorMessageBuilder_.build();
}
if (((from_bitField0_ & 0x00001000) == 0x00001000)) {
to_bitField0_ |= 0x00001000;
}
if (directMessageBuilder_ == null) {
result.directMessage_ = directMessage_;
} else {
result.directMessage_ = directMessageBuilder_.build();
}
if (((from_bitField0_ & 0x00002000) == 0x00002000)) {
to_bitField0_ |= 0x00002000;
}
if (ackMessageBuilder_ == null) {
result.ackMessage_ = ackMessage_;
} else {
result.ackMessage_ = ackMessageBuilder_.build();
}
if (((from_bitField0_ & 0x00004000) == 0x00004000)) {
to_bitField0_ |= 0x00004000;
}
if (unreadMessageBuilder_ == null) {
result.unreadMessage_ = unreadMessage_;
} else {
result.unreadMessage_ = unreadMessageBuilder_.build();
}
if (((from_bitField0_ & 0x00008000) == 0x00008000)) {
to_bitField0_ |= 0x00008000;
}
if (readMessageBuilder_ == null) {
result.readMessage_ = readMessage_;
} else {
result.readMessage_ = readMessageBuilder_.build();
}
if (((from_bitField0_ & 0x00010000) == 0x00010000)) {
to_bitField0_ |= 0x00010000;
}
if (rcpMessageBuilder_ == null) {
result.rcpMessage_ = rcpMessage_;
} else {
result.rcpMessage_ = rcpMessageBuilder_.build();
}
if (((from_bitField0_ & 0x00020000) == 0x00020000)) {
to_bitField0_ |= 0x00020000;
}
if (logsMessageBuilder_ == null) {
result.logsMessage_ = logsMessage_;
} else {
result.logsMessage_ = logsMessageBuilder_.build();
}
if (((from_bitField0_ & 0x00040000) == 0x00040000)) {
to_bitField0_ |= 0x00040000;
}
if (convMessageBuilder_ == null) {
result.convMessage_ = convMessage_;
} else {
result.convMessage_ = convMessageBuilder_.build();
}
if (((from_bitField0_ & 0x00080000) == 0x00080000)) {
to_bitField0_ |= 0x00080000;
}
if (roomMessageBuilder_ == null) {
result.roomMessage_ = roomMessage_;
} else {
result.roomMessage_ = roomMessageBuilder_.build();
}
if (((from_bitField0_ & 0x00100000) == 0x00100000)) {
to_bitField0_ |= 0x00100000;
}
if (presenceMessageBuilder_ == null) {
result.presenceMessage_ = presenceMessage_;
} else {
result.presenceMessage_ = presenceMessageBuilder_.build();
}
if (((from_bitField0_ & 0x00200000) == 0x00200000)) {
to_bitField0_ |= 0x00200000;
}
if (reportMessageBuilder_ == null) {
result.reportMessage_ = reportMessage_;
} else {
result.reportMessage_ = reportMessageBuilder_.build();
}
if (((from_bitField0_ & 0x00400000) == 0x00400000)) {
to_bitField0_ |= 0x00400000;
}
if (patchMessageBuilder_ == null) {
result.patchMessage_ = patchMessage_;
} else {
result.patchMessage_ = patchMessageBuilder_.build();
}
if (((from_bitField0_ & 0x00800000) == 0x00800000)) {
to_bitField0_ |= 0x00800000;
}
if (pubsubMessageBuilder_ == null) {
result.pubsubMessage_ = pubsubMessage_;
} else {
result.pubsubMessage_ = pubsubMessageBuilder_.build();
}
if (((from_bitField0_ & 0x01000000) == 0x01000000)) {
to_bitField0_ |= 0x01000000;
}
if (blacklistMessageBuilder_ == null) {
result.blacklistMessage_ = blacklistMessage_;
} else {
result.blacklistMessage_ = blacklistMessageBuilder_.build();
}
result.bitField0_ = to_bitField0_;
onBuilt();
return result;
}
public Builder clone() {
return (Builder) super.clone();
}
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.setField(field, value);
}
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return (Builder) super.clearField(field);
}
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return (Builder) super.clearOneof(oneof);
}
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return (Builder) super.setRepeatedField(field, index, value);
}
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.addRepeatedField(field, value);
}
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.avos.avoscloud.Messages.GenericCommand) {
return mergeFrom((com.avos.avoscloud.Messages.GenericCommand)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.avos.avoscloud.Messages.GenericCommand other) {
if (other == com.avos.avoscloud.Messages.GenericCommand.getDefaultInstance()) return this;
if (other.hasCmd()) {
setCmd(other.getCmd());
}
if (other.hasOp()) {
setOp(other.getOp());
}
if (other.hasAppId()) {
bitField0_ |= 0x00000004;
appId_ = other.appId_;
onChanged();
}
if (other.hasPeerId()) {
bitField0_ |= 0x00000008;
peerId_ = other.peerId_;
onChanged();
}
if (other.hasI()) {
setI(other.getI());
}
if (other.hasInstallationId()) {
bitField0_ |= 0x00000020;
installationId_ = other.installationId_;
onChanged();
}
if (other.hasPriority()) {
setPriority(other.getPriority());
}
if (other.hasService()) {
setService(other.getService());
}
if (other.hasServerTs()) {
setServerTs(other.getServerTs());
}
if (other.hasDataMessage()) {
mergeDataMessage(other.getDataMessage());
}
if (other.hasSessionMessage()) {
mergeSessionMessage(other.getSessionMessage());
}
if (other.hasErrorMessage()) {
mergeErrorMessage(other.getErrorMessage());
}
if (other.hasDirectMessage()) {
mergeDirectMessage(other.getDirectMessage());
}
if (other.hasAckMessage()) {
mergeAckMessage(other.getAckMessage());
}
if (other.hasUnreadMessage()) {
mergeUnreadMessage(other.getUnreadMessage());
}
if (other.hasReadMessage()) {
mergeReadMessage(other.getReadMessage());
}
if (other.hasRcpMessage()) {
mergeRcpMessage(other.getRcpMessage());
}
if (other.hasLogsMessage()) {
mergeLogsMessage(other.getLogsMessage());
}
if (other.hasConvMessage()) {
mergeConvMessage(other.getConvMessage());
}
if (other.hasRoomMessage()) {
mergeRoomMessage(other.getRoomMessage());
}
if (other.hasPresenceMessage()) {
mergePresenceMessage(other.getPresenceMessage());
}
if (other.hasReportMessage()) {
mergeReportMessage(other.getReportMessage());
}
if (other.hasPatchMessage()) {
mergePatchMessage(other.getPatchMessage());
}
if (other.hasPubsubMessage()) {
mergePubsubMessage(other.getPubsubMessage());
}
if (other.hasBlacklistMessage()) {
mergeBlacklistMessage(other.getBlacklistMessage());
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
public final boolean isInitialized() {
if (hasDataMessage()) {
if (!getDataMessage().isInitialized()) {
return false;
}
}
if (hasErrorMessage()) {
if (!getErrorMessage().isInitialized()) {
return false;
}
}
if (hasUnreadMessage()) {
if (!getUnreadMessage().isInitialized()) {
return false;
}
}
if (hasReadMessage()) {
if (!getReadMessage().isInitialized()) {
return false;
}
}
if (hasConvMessage()) {
if (!getConvMessage().isInitialized()) {
return false;
}
}
if (hasPubsubMessage()) {
if (!getPubsubMessage().isInitialized()) {
return false;
}
}
if (hasBlacklistMessage()) {
if (!getBlacklistMessage().isInitialized()) {
return false;
}
}
return true;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
com.avos.avoscloud.Messages.GenericCommand parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (com.avos.avoscloud.Messages.GenericCommand) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
private int cmd_ = 0;
/**
* optional .com.avos.avoscloud.CommandType cmd = 1;
*/
public boolean hasCmd() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
* optional .com.avos.avoscloud.CommandType cmd = 1;
*/
public com.avos.avoscloud.Messages.CommandType getCmd() {
com.avos.avoscloud.Messages.CommandType result = com.avos.avoscloud.Messages.CommandType.valueOf(cmd_);
return result == null ? com.avos.avoscloud.Messages.CommandType.session : result;
}
/**
* optional .com.avos.avoscloud.CommandType cmd = 1;
*/
public Builder setCmd(com.avos.avoscloud.Messages.CommandType value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000001;
cmd_ = value.getNumber();
onChanged();
return this;
}
/**
* optional .com.avos.avoscloud.CommandType cmd = 1;
*/
public Builder clearCmd() {
bitField0_ = (bitField0_ & ~0x00000001);
cmd_ = 0;
onChanged();
return this;
}
private int op_ = 1;
/**
* optional .com.avos.avoscloud.OpType op = 2;
*/
public boolean hasOp() {
return ((bitField0_ & 0x00000002) == 0x00000002);
}
/**
* optional .com.avos.avoscloud.OpType op = 2;
*/
public com.avos.avoscloud.Messages.OpType getOp() {
com.avos.avoscloud.Messages.OpType result = com.avos.avoscloud.Messages.OpType.valueOf(op_);
return result == null ? com.avos.avoscloud.Messages.OpType.open : result;
}
/**
* optional .com.avos.avoscloud.OpType op = 2;
*/
public Builder setOp(com.avos.avoscloud.Messages.OpType value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000002;
op_ = value.getNumber();
onChanged();
return this;
}
/**
* optional .com.avos.avoscloud.OpType op = 2;
*/
public Builder clearOp() {
bitField0_ = (bitField0_ & ~0x00000002);
op_ = 1;
onChanged();
return this;
}
private java.lang.Object appId_ = "";
/**
* optional string appId = 3;
*/
public boolean hasAppId() {
return ((bitField0_ & 0x00000004) == 0x00000004);
}
/**
* optional string appId = 3;
*/
public java.lang.String getAppId() {
java.lang.Object ref = appId_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
appId_ = s;
}
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* optional string appId = 3;
*/
public com.google.protobuf.ByteString
getAppIdBytes() {
java.lang.Object ref = appId_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
appId_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* optional string appId = 3;
*/
public Builder setAppId(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000004;
appId_ = value;
onChanged();
return this;
}
/**
* optional string appId = 3;
*/
public Builder clearAppId() {
bitField0_ = (bitField0_ & ~0x00000004);
appId_ = getDefaultInstance().getAppId();
onChanged();
return this;
}
/**
* optional string appId = 3;
*/
public Builder setAppIdBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000004;
appId_ = value;
onChanged();
return this;
}
private java.lang.Object peerId_ = "";
/**
* optional string peerId = 4;
*/
public boolean hasPeerId() {
return ((bitField0_ & 0x00000008) == 0x00000008);
}
/**
* optional string peerId = 4;
*/
public java.lang.String getPeerId() {
java.lang.Object ref = peerId_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
peerId_ = s;
}
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* optional string peerId = 4;
*/
public com.google.protobuf.ByteString
getPeerIdBytes() {
java.lang.Object ref = peerId_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
peerId_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* optional string peerId = 4;
*/
public Builder setPeerId(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000008;
peerId_ = value;
onChanged();
return this;
}
/**
* optional string peerId = 4;
*/
public Builder clearPeerId() {
bitField0_ = (bitField0_ & ~0x00000008);
peerId_ = getDefaultInstance().getPeerId();
onChanged();
return this;
}
/**
* optional string peerId = 4;
*/
public Builder setPeerIdBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000008;
peerId_ = value;
onChanged();
return this;
}
private int i_ ;
/**
* optional int32 i = 5;
*/
public boolean hasI() {
return ((bitField0_ & 0x00000010) == 0x00000010);
}
/**
* optional int32 i = 5;
*/
public int getI() {
return i_;
}
/**
* optional int32 i = 5;
*/
public Builder setI(int value) {
bitField0_ |= 0x00000010;
i_ = value;
onChanged();
return this;
}
/**
* optional int32 i = 5;
*/
public Builder clearI() {
bitField0_ = (bitField0_ & ~0x00000010);
i_ = 0;
onChanged();
return this;
}
private java.lang.Object installationId_ = "";
/**
* optional string installationId = 6;
*/
public boolean hasInstallationId() {
return ((bitField0_ & 0x00000020) == 0x00000020);
}
/**
* optional string installationId = 6;
*/
public java.lang.String getInstallationId() {
java.lang.Object ref = installationId_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
installationId_ = s;
}
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* optional string installationId = 6;
*/
public com.google.protobuf.ByteString
getInstallationIdBytes() {
java.lang.Object ref = installationId_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
installationId_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* optional string installationId = 6;
*/
public Builder setInstallationId(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000020;
installationId_ = value;
onChanged();
return this;
}
/**
* optional string installationId = 6;
*/
public Builder clearInstallationId() {
bitField0_ = (bitField0_ & ~0x00000020);
installationId_ = getDefaultInstance().getInstallationId();
onChanged();
return this;
}
/**
* optional string installationId = 6;
*/
public Builder setInstallationIdBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000020;
installationId_ = value;
onChanged();
return this;
}
private int priority_ ;
/**
* optional int32 priority = 7;
*/
public boolean hasPriority() {
return ((bitField0_ & 0x00000040) == 0x00000040);
}
/**
* optional int32 priority = 7;
*/
public int getPriority() {
return priority_;
}
/**
* optional int32 priority = 7;
*/
public Builder setPriority(int value) {
bitField0_ |= 0x00000040;
priority_ = value;
onChanged();
return this;
}
/**
* optional int32 priority = 7;
*/
public Builder clearPriority() {
bitField0_ = (bitField0_ & ~0x00000040);
priority_ = 0;
onChanged();
return this;
}
private int service_ ;
/**
* optional int32 service = 8;
*/
public boolean hasService() {
return ((bitField0_ & 0x00000080) == 0x00000080);
}
/**
* optional int32 service = 8;
*/
public int getService() {
return service_;
}
/**
* optional int32 service = 8;
*/
public Builder setService(int value) {
bitField0_ |= 0x00000080;
service_ = value;
onChanged();
return this;
}
/**
* optional int32 service = 8;
*/
public Builder clearService() {
bitField0_ = (bitField0_ & ~0x00000080);
service_ = 0;
onChanged();
return this;
}
private long serverTs_ ;
/**
* optional int64 serverTs = 9;
*/
public boolean hasServerTs() {
return ((bitField0_ & 0x00000100) == 0x00000100);
}
/**
* optional int64 serverTs = 9;
*/
public long getServerTs() {
return serverTs_;
}
/**
* optional int64 serverTs = 9;
*/
public Builder setServerTs(long value) {
bitField0_ |= 0x00000100;
serverTs_ = value;
onChanged();
return this;
}
/**
* optional int64 serverTs = 9;
*/
public Builder clearServerTs() {
bitField0_ = (bitField0_ & ~0x00000100);
serverTs_ = 0L;
onChanged();
return this;
}
private com.avos.avoscloud.Messages.DataCommand dataMessage_ = null;
private com.google.protobuf.SingleFieldBuilderV3<
com.avos.avoscloud.Messages.DataCommand, com.avos.avoscloud.Messages.DataCommand.Builder, com.avos.avoscloud.Messages.DataCommandOrBuilder> dataMessageBuilder_;
/**
* optional .com.avos.avoscloud.DataCommand dataMessage = 101;
*/
public boolean hasDataMessage() {
return ((bitField0_ & 0x00000200) == 0x00000200);
}
/**
* optional .com.avos.avoscloud.DataCommand dataMessage = 101;
*/
public com.avos.avoscloud.Messages.DataCommand getDataMessage() {
if (dataMessageBuilder_ == null) {
return dataMessage_ == null ? com.avos.avoscloud.Messages.DataCommand.getDefaultInstance() : dataMessage_;
} else {
return dataMessageBuilder_.getMessage();
}
}
/**
* optional .com.avos.avoscloud.DataCommand dataMessage = 101;
*/
public Builder setDataMessage(com.avos.avoscloud.Messages.DataCommand value) {
if (dataMessageBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
dataMessage_ = value;
onChanged();
} else {
dataMessageBuilder_.setMessage(value);
}
bitField0_ |= 0x00000200;
return this;
}
/**
* optional .com.avos.avoscloud.DataCommand dataMessage = 101;
*/
public Builder setDataMessage(
com.avos.avoscloud.Messages.DataCommand.Builder builderForValue) {
if (dataMessageBuilder_ == null) {
dataMessage_ = builderForValue.build();
onChanged();
} else {
dataMessageBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00000200;
return this;
}
/**
* optional .com.avos.avoscloud.DataCommand dataMessage = 101;
*/
public Builder mergeDataMessage(com.avos.avoscloud.Messages.DataCommand value) {
if (dataMessageBuilder_ == null) {
if (((bitField0_ & 0x00000200) == 0x00000200) &&
dataMessage_ != null &&
dataMessage_ != com.avos.avoscloud.Messages.DataCommand.getDefaultInstance()) {
dataMessage_ =
com.avos.avoscloud.Messages.DataCommand.newBuilder(dataMessage_).mergeFrom(value).buildPartial();
} else {
dataMessage_ = value;
}
onChanged();
} else {
dataMessageBuilder_.mergeFrom(value);
}
bitField0_ |= 0x00000200;
return this;
}
/**
* optional .com.avos.avoscloud.DataCommand dataMessage = 101;
*/
public Builder clearDataMessage() {
if (dataMessageBuilder_ == null) {
dataMessage_ = null;
onChanged();
} else {
dataMessageBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000200);
return this;
}
/**
* optional .com.avos.avoscloud.DataCommand dataMessage = 101;
*/
public com.avos.avoscloud.Messages.DataCommand.Builder getDataMessageBuilder() {
bitField0_ |= 0x00000200;
onChanged();
return getDataMessageFieldBuilder().getBuilder();
}
/**
* optional .com.avos.avoscloud.DataCommand dataMessage = 101;
*/
public com.avos.avoscloud.Messages.DataCommandOrBuilder getDataMessageOrBuilder() {
if (dataMessageBuilder_ != null) {
return dataMessageBuilder_.getMessageOrBuilder();
} else {
return dataMessage_ == null ?
com.avos.avoscloud.Messages.DataCommand.getDefaultInstance() : dataMessage_;
}
}
/**
* optional .com.avos.avoscloud.DataCommand dataMessage = 101;
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.avos.avoscloud.Messages.DataCommand, com.avos.avoscloud.Messages.DataCommand.Builder, com.avos.avoscloud.Messages.DataCommandOrBuilder>
getDataMessageFieldBuilder() {
if (dataMessageBuilder_ == null) {
dataMessageBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
com.avos.avoscloud.Messages.DataCommand, com.avos.avoscloud.Messages.DataCommand.Builder, com.avos.avoscloud.Messages.DataCommandOrBuilder>(
getDataMessage(),
getParentForChildren(),
isClean());
dataMessage_ = null;
}
return dataMessageBuilder_;
}
private com.avos.avoscloud.Messages.SessionCommand sessionMessage_ = null;
private com.google.protobuf.SingleFieldBuilderV3<
com.avos.avoscloud.Messages.SessionCommand, com.avos.avoscloud.Messages.SessionCommand.Builder, com.avos.avoscloud.Messages.SessionCommandOrBuilder> sessionMessageBuilder_;
/**
* optional .com.avos.avoscloud.SessionCommand sessionMessage = 102;
*/
public boolean hasSessionMessage() {
return ((bitField0_ & 0x00000400) == 0x00000400);
}
/**
* optional .com.avos.avoscloud.SessionCommand sessionMessage = 102;
*/
public com.avos.avoscloud.Messages.SessionCommand getSessionMessage() {
if (sessionMessageBuilder_ == null) {
return sessionMessage_ == null ? com.avos.avoscloud.Messages.SessionCommand.getDefaultInstance() : sessionMessage_;
} else {
return sessionMessageBuilder_.getMessage();
}
}
/**
* optional .com.avos.avoscloud.SessionCommand sessionMessage = 102;
*/
public Builder setSessionMessage(com.avos.avoscloud.Messages.SessionCommand value) {
if (sessionMessageBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
sessionMessage_ = value;
onChanged();
} else {
sessionMessageBuilder_.setMessage(value);
}
bitField0_ |= 0x00000400;
return this;
}
/**
* optional .com.avos.avoscloud.SessionCommand sessionMessage = 102;
*/
public Builder setSessionMessage(
com.avos.avoscloud.Messages.SessionCommand.Builder builderForValue) {
if (sessionMessageBuilder_ == null) {
sessionMessage_ = builderForValue.build();
onChanged();
} else {
sessionMessageBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00000400;
return this;
}
/**
* optional .com.avos.avoscloud.SessionCommand sessionMessage = 102;
*/
public Builder mergeSessionMessage(com.avos.avoscloud.Messages.SessionCommand value) {
if (sessionMessageBuilder_ == null) {
if (((bitField0_ & 0x00000400) == 0x00000400) &&
sessionMessage_ != null &&
sessionMessage_ != com.avos.avoscloud.Messages.SessionCommand.getDefaultInstance()) {
sessionMessage_ =
com.avos.avoscloud.Messages.SessionCommand.newBuilder(sessionMessage_).mergeFrom(value).buildPartial();
} else {
sessionMessage_ = value;
}
onChanged();
} else {
sessionMessageBuilder_.mergeFrom(value);
}
bitField0_ |= 0x00000400;
return this;
}
/**
* optional .com.avos.avoscloud.SessionCommand sessionMessage = 102;
*/
public Builder clearSessionMessage() {
if (sessionMessageBuilder_ == null) {
sessionMessage_ = null;
onChanged();
} else {
sessionMessageBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000400);
return this;
}
/**
* optional .com.avos.avoscloud.SessionCommand sessionMessage = 102;
*/
public com.avos.avoscloud.Messages.SessionCommand.Builder getSessionMessageBuilder() {
bitField0_ |= 0x00000400;
onChanged();
return getSessionMessageFieldBuilder().getBuilder();
}
/**
* optional .com.avos.avoscloud.SessionCommand sessionMessage = 102;
*/
public com.avos.avoscloud.Messages.SessionCommandOrBuilder getSessionMessageOrBuilder() {
if (sessionMessageBuilder_ != null) {
return sessionMessageBuilder_.getMessageOrBuilder();
} else {
return sessionMessage_ == null ?
com.avos.avoscloud.Messages.SessionCommand.getDefaultInstance() : sessionMessage_;
}
}
/**
* optional .com.avos.avoscloud.SessionCommand sessionMessage = 102;
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.avos.avoscloud.Messages.SessionCommand, com.avos.avoscloud.Messages.SessionCommand.Builder, com.avos.avoscloud.Messages.SessionCommandOrBuilder>
getSessionMessageFieldBuilder() {
if (sessionMessageBuilder_ == null) {
sessionMessageBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
com.avos.avoscloud.Messages.SessionCommand, com.avos.avoscloud.Messages.SessionCommand.Builder, com.avos.avoscloud.Messages.SessionCommandOrBuilder>(
getSessionMessage(),
getParentForChildren(),
isClean());
sessionMessage_ = null;
}
return sessionMessageBuilder_;
}
private com.avos.avoscloud.Messages.ErrorCommand errorMessage_ = null;
private com.google.protobuf.SingleFieldBuilderV3<
com.avos.avoscloud.Messages.ErrorCommand, com.avos.avoscloud.Messages.ErrorCommand.Builder, com.avos.avoscloud.Messages.ErrorCommandOrBuilder> errorMessageBuilder_;
/**
* optional .com.avos.avoscloud.ErrorCommand errorMessage = 103;
*/
public boolean hasErrorMessage() {
return ((bitField0_ & 0x00000800) == 0x00000800);
}
/**
* optional .com.avos.avoscloud.ErrorCommand errorMessage = 103;
*/
public com.avos.avoscloud.Messages.ErrorCommand getErrorMessage() {
if (errorMessageBuilder_ == null) {
return errorMessage_ == null ? com.avos.avoscloud.Messages.ErrorCommand.getDefaultInstance() : errorMessage_;
} else {
return errorMessageBuilder_.getMessage();
}
}
/**
* optional .com.avos.avoscloud.ErrorCommand errorMessage = 103;
*/
public Builder setErrorMessage(com.avos.avoscloud.Messages.ErrorCommand value) {
if (errorMessageBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
errorMessage_ = value;
onChanged();
} else {
errorMessageBuilder_.setMessage(value);
}
bitField0_ |= 0x00000800;
return this;
}
/**
* optional .com.avos.avoscloud.ErrorCommand errorMessage = 103;
*/
public Builder setErrorMessage(
com.avos.avoscloud.Messages.ErrorCommand.Builder builderForValue) {
if (errorMessageBuilder_ == null) {
errorMessage_ = builderForValue.build();
onChanged();
} else {
errorMessageBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00000800;
return this;
}
/**
* optional .com.avos.avoscloud.ErrorCommand errorMessage = 103;
*/
public Builder mergeErrorMessage(com.avos.avoscloud.Messages.ErrorCommand value) {
if (errorMessageBuilder_ == null) {
if (((bitField0_ & 0x00000800) == 0x00000800) &&
errorMessage_ != null &&
errorMessage_ != com.avos.avoscloud.Messages.ErrorCommand.getDefaultInstance()) {
errorMessage_ =
com.avos.avoscloud.Messages.ErrorCommand.newBuilder(errorMessage_).mergeFrom(value).buildPartial();
} else {
errorMessage_ = value;
}
onChanged();
} else {
errorMessageBuilder_.mergeFrom(value);
}
bitField0_ |= 0x00000800;
return this;
}
/**
* optional .com.avos.avoscloud.ErrorCommand errorMessage = 103;
*/
public Builder clearErrorMessage() {
if (errorMessageBuilder_ == null) {
errorMessage_ = null;
onChanged();
} else {
errorMessageBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000800);
return this;
}
/**
* optional .com.avos.avoscloud.ErrorCommand errorMessage = 103;
*/
public com.avos.avoscloud.Messages.ErrorCommand.Builder getErrorMessageBuilder() {
bitField0_ |= 0x00000800;
onChanged();
return getErrorMessageFieldBuilder().getBuilder();
}
/**
* optional .com.avos.avoscloud.ErrorCommand errorMessage = 103;
*/
public com.avos.avoscloud.Messages.ErrorCommandOrBuilder getErrorMessageOrBuilder() {
if (errorMessageBuilder_ != null) {
return errorMessageBuilder_.getMessageOrBuilder();
} else {
return errorMessage_ == null ?
com.avos.avoscloud.Messages.ErrorCommand.getDefaultInstance() : errorMessage_;
}
}
/**
* optional .com.avos.avoscloud.ErrorCommand errorMessage = 103;
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.avos.avoscloud.Messages.ErrorCommand, com.avos.avoscloud.Messages.ErrorCommand.Builder, com.avos.avoscloud.Messages.ErrorCommandOrBuilder>
getErrorMessageFieldBuilder() {
if (errorMessageBuilder_ == null) {
errorMessageBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
com.avos.avoscloud.Messages.ErrorCommand, com.avos.avoscloud.Messages.ErrorCommand.Builder, com.avos.avoscloud.Messages.ErrorCommandOrBuilder>(
getErrorMessage(),
getParentForChildren(),
isClean());
errorMessage_ = null;
}
return errorMessageBuilder_;
}
private com.avos.avoscloud.Messages.DirectCommand directMessage_ = null;
private com.google.protobuf.SingleFieldBuilderV3<
com.avos.avoscloud.Messages.DirectCommand, com.avos.avoscloud.Messages.DirectCommand.Builder, com.avos.avoscloud.Messages.DirectCommandOrBuilder> directMessageBuilder_;
/**
* optional .com.avos.avoscloud.DirectCommand directMessage = 104;
*/
public boolean hasDirectMessage() {
return ((bitField0_ & 0x00001000) == 0x00001000);
}
/**
* optional .com.avos.avoscloud.DirectCommand directMessage = 104;
*/
public com.avos.avoscloud.Messages.DirectCommand getDirectMessage() {
if (directMessageBuilder_ == null) {
return directMessage_ == null ? com.avos.avoscloud.Messages.DirectCommand.getDefaultInstance() : directMessage_;
} else {
return directMessageBuilder_.getMessage();
}
}
/**
* optional .com.avos.avoscloud.DirectCommand directMessage = 104;
*/
public Builder setDirectMessage(com.avos.avoscloud.Messages.DirectCommand value) {
if (directMessageBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
directMessage_ = value;
onChanged();
} else {
directMessageBuilder_.setMessage(value);
}
bitField0_ |= 0x00001000;
return this;
}
/**
* optional .com.avos.avoscloud.DirectCommand directMessage = 104;
*/
public Builder setDirectMessage(
com.avos.avoscloud.Messages.DirectCommand.Builder builderForValue) {
if (directMessageBuilder_ == null) {
directMessage_ = builderForValue.build();
onChanged();
} else {
directMessageBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00001000;
return this;
}
/**
* optional .com.avos.avoscloud.DirectCommand directMessage = 104;
*/
public Builder mergeDirectMessage(com.avos.avoscloud.Messages.DirectCommand value) {
if (directMessageBuilder_ == null) {
if (((bitField0_ & 0x00001000) == 0x00001000) &&
directMessage_ != null &&
directMessage_ != com.avos.avoscloud.Messages.DirectCommand.getDefaultInstance()) {
directMessage_ =
com.avos.avoscloud.Messages.DirectCommand.newBuilder(directMessage_).mergeFrom(value).buildPartial();
} else {
directMessage_ = value;
}
onChanged();
} else {
directMessageBuilder_.mergeFrom(value);
}
bitField0_ |= 0x00001000;
return this;
}
/**
* optional .com.avos.avoscloud.DirectCommand directMessage = 104;
*/
public Builder clearDirectMessage() {
if (directMessageBuilder_ == null) {
directMessage_ = null;
onChanged();
} else {
directMessageBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00001000);
return this;
}
/**
* optional .com.avos.avoscloud.DirectCommand directMessage = 104;
*/
public com.avos.avoscloud.Messages.DirectCommand.Builder getDirectMessageBuilder() {
bitField0_ |= 0x00001000;
onChanged();
return getDirectMessageFieldBuilder().getBuilder();
}
/**
* optional .com.avos.avoscloud.DirectCommand directMessage = 104;
*/
public com.avos.avoscloud.Messages.DirectCommandOrBuilder getDirectMessageOrBuilder() {
if (directMessageBuilder_ != null) {
return directMessageBuilder_.getMessageOrBuilder();
} else {
return directMessage_ == null ?
com.avos.avoscloud.Messages.DirectCommand.getDefaultInstance() : directMessage_;
}
}
/**
* optional .com.avos.avoscloud.DirectCommand directMessage = 104;
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.avos.avoscloud.Messages.DirectCommand, com.avos.avoscloud.Messages.DirectCommand.Builder, com.avos.avoscloud.Messages.DirectCommandOrBuilder>
getDirectMessageFieldBuilder() {
if (directMessageBuilder_ == null) {
directMessageBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
com.avos.avoscloud.Messages.DirectCommand, com.avos.avoscloud.Messages.DirectCommand.Builder, com.avos.avoscloud.Messages.DirectCommandOrBuilder>(
getDirectMessage(),
getParentForChildren(),
isClean());
directMessage_ = null;
}
return directMessageBuilder_;
}
private com.avos.avoscloud.Messages.AckCommand ackMessage_ = null;
private com.google.protobuf.SingleFieldBuilderV3<
com.avos.avoscloud.Messages.AckCommand, com.avos.avoscloud.Messages.AckCommand.Builder, com.avos.avoscloud.Messages.AckCommandOrBuilder> ackMessageBuilder_;
/**
* optional .com.avos.avoscloud.AckCommand ackMessage = 105;
*/
public boolean hasAckMessage() {
return ((bitField0_ & 0x00002000) == 0x00002000);
}
/**
* optional .com.avos.avoscloud.AckCommand ackMessage = 105;
*/
public com.avos.avoscloud.Messages.AckCommand getAckMessage() {
if (ackMessageBuilder_ == null) {
return ackMessage_ == null ? com.avos.avoscloud.Messages.AckCommand.getDefaultInstance() : ackMessage_;
} else {
return ackMessageBuilder_.getMessage();
}
}
/**
* optional .com.avos.avoscloud.AckCommand ackMessage = 105;
*/
public Builder setAckMessage(com.avos.avoscloud.Messages.AckCommand value) {
if (ackMessageBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ackMessage_ = value;
onChanged();
} else {
ackMessageBuilder_.setMessage(value);
}
bitField0_ |= 0x00002000;
return this;
}
/**
* optional .com.avos.avoscloud.AckCommand ackMessage = 105;
*/
public Builder setAckMessage(
com.avos.avoscloud.Messages.AckCommand.Builder builderForValue) {
if (ackMessageBuilder_ == null) {
ackMessage_ = builderForValue.build();
onChanged();
} else {
ackMessageBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00002000;
return this;
}
/**
* optional .com.avos.avoscloud.AckCommand ackMessage = 105;
*/
public Builder mergeAckMessage(com.avos.avoscloud.Messages.AckCommand value) {
if (ackMessageBuilder_ == null) {
if (((bitField0_ & 0x00002000) == 0x00002000) &&
ackMessage_ != null &&
ackMessage_ != com.avos.avoscloud.Messages.AckCommand.getDefaultInstance()) {
ackMessage_ =
com.avos.avoscloud.Messages.AckCommand.newBuilder(ackMessage_).mergeFrom(value).buildPartial();
} else {
ackMessage_ = value;
}
onChanged();
} else {
ackMessageBuilder_.mergeFrom(value);
}
bitField0_ |= 0x00002000;
return this;
}
/**
* optional .com.avos.avoscloud.AckCommand ackMessage = 105;
*/
public Builder clearAckMessage() {
if (ackMessageBuilder_ == null) {
ackMessage_ = null;
onChanged();
} else {
ackMessageBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00002000);
return this;
}
/**
* optional .com.avos.avoscloud.AckCommand ackMessage = 105;
*/
public com.avos.avoscloud.Messages.AckCommand.Builder getAckMessageBuilder() {
bitField0_ |= 0x00002000;
onChanged();
return getAckMessageFieldBuilder().getBuilder();
}
/**
* optional .com.avos.avoscloud.AckCommand ackMessage = 105;
*/
public com.avos.avoscloud.Messages.AckCommandOrBuilder getAckMessageOrBuilder() {
if (ackMessageBuilder_ != null) {
return ackMessageBuilder_.getMessageOrBuilder();
} else {
return ackMessage_ == null ?
com.avos.avoscloud.Messages.AckCommand.getDefaultInstance() : ackMessage_;
}
}
/**
* optional .com.avos.avoscloud.AckCommand ackMessage = 105;
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.avos.avoscloud.Messages.AckCommand, com.avos.avoscloud.Messages.AckCommand.Builder, com.avos.avoscloud.Messages.AckCommandOrBuilder>
getAckMessageFieldBuilder() {
if (ackMessageBuilder_ == null) {
ackMessageBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
com.avos.avoscloud.Messages.AckCommand, com.avos.avoscloud.Messages.AckCommand.Builder, com.avos.avoscloud.Messages.AckCommandOrBuilder>(
getAckMessage(),
getParentForChildren(),
isClean());
ackMessage_ = null;
}
return ackMessageBuilder_;
}
private com.avos.avoscloud.Messages.UnreadCommand unreadMessage_ = null;
private com.google.protobuf.SingleFieldBuilderV3<
com.avos.avoscloud.Messages.UnreadCommand, com.avos.avoscloud.Messages.UnreadCommand.Builder, com.avos.avoscloud.Messages.UnreadCommandOrBuilder> unreadMessageBuilder_;
/**
* optional .com.avos.avoscloud.UnreadCommand unreadMessage = 106;
*/
public boolean hasUnreadMessage() {
return ((bitField0_ & 0x00004000) == 0x00004000);
}
/**
* optional .com.avos.avoscloud.UnreadCommand unreadMessage = 106;
*/
public com.avos.avoscloud.Messages.UnreadCommand getUnreadMessage() {
if (unreadMessageBuilder_ == null) {
return unreadMessage_ == null ? com.avos.avoscloud.Messages.UnreadCommand.getDefaultInstance() : unreadMessage_;
} else {
return unreadMessageBuilder_.getMessage();
}
}
/**
* optional .com.avos.avoscloud.UnreadCommand unreadMessage = 106;
*/
public Builder setUnreadMessage(com.avos.avoscloud.Messages.UnreadCommand value) {
if (unreadMessageBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
unreadMessage_ = value;
onChanged();
} else {
unreadMessageBuilder_.setMessage(value);
}
bitField0_ |= 0x00004000;
return this;
}
/**
* optional .com.avos.avoscloud.UnreadCommand unreadMessage = 106;
*/
public Builder setUnreadMessage(
com.avos.avoscloud.Messages.UnreadCommand.Builder builderForValue) {
if (unreadMessageBuilder_ == null) {
unreadMessage_ = builderForValue.build();
onChanged();
} else {
unreadMessageBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00004000;
return this;
}
/**
* optional .com.avos.avoscloud.UnreadCommand unreadMessage = 106;
*/
public Builder mergeUnreadMessage(com.avos.avoscloud.Messages.UnreadCommand value) {
if (unreadMessageBuilder_ == null) {
if (((bitField0_ & 0x00004000) == 0x00004000) &&
unreadMessage_ != null &&
unreadMessage_ != com.avos.avoscloud.Messages.UnreadCommand.getDefaultInstance()) {
unreadMessage_ =
com.avos.avoscloud.Messages.UnreadCommand.newBuilder(unreadMessage_).mergeFrom(value).buildPartial();
} else {
unreadMessage_ = value;
}
onChanged();
} else {
unreadMessageBuilder_.mergeFrom(value);
}
bitField0_ |= 0x00004000;
return this;
}
/**
* optional .com.avos.avoscloud.UnreadCommand unreadMessage = 106;
*/
public Builder clearUnreadMessage() {
if (unreadMessageBuilder_ == null) {
unreadMessage_ = null;
onChanged();
} else {
unreadMessageBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00004000);
return this;
}
/**
* optional .com.avos.avoscloud.UnreadCommand unreadMessage = 106;
*/
public com.avos.avoscloud.Messages.UnreadCommand.Builder getUnreadMessageBuilder() {
bitField0_ |= 0x00004000;
onChanged();
return getUnreadMessageFieldBuilder().getBuilder();
}
/**
* optional .com.avos.avoscloud.UnreadCommand unreadMessage = 106;
*/
public com.avos.avoscloud.Messages.UnreadCommandOrBuilder getUnreadMessageOrBuilder() {
if (unreadMessageBuilder_ != null) {
return unreadMessageBuilder_.getMessageOrBuilder();
} else {
return unreadMessage_ == null ?
com.avos.avoscloud.Messages.UnreadCommand.getDefaultInstance() : unreadMessage_;
}
}
/**
* optional .com.avos.avoscloud.UnreadCommand unreadMessage = 106;
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.avos.avoscloud.Messages.UnreadCommand, com.avos.avoscloud.Messages.UnreadCommand.Builder, com.avos.avoscloud.Messages.UnreadCommandOrBuilder>
getUnreadMessageFieldBuilder() {
if (unreadMessageBuilder_ == null) {
unreadMessageBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
com.avos.avoscloud.Messages.UnreadCommand, com.avos.avoscloud.Messages.UnreadCommand.Builder, com.avos.avoscloud.Messages.UnreadCommandOrBuilder>(
getUnreadMessage(),
getParentForChildren(),
isClean());
unreadMessage_ = null;
}
return unreadMessageBuilder_;
}
private com.avos.avoscloud.Messages.ReadCommand readMessage_ = null;
private com.google.protobuf.SingleFieldBuilderV3<
com.avos.avoscloud.Messages.ReadCommand, com.avos.avoscloud.Messages.ReadCommand.Builder, com.avos.avoscloud.Messages.ReadCommandOrBuilder> readMessageBuilder_;
/**
* optional .com.avos.avoscloud.ReadCommand readMessage = 107;
*/
public boolean hasReadMessage() {
return ((bitField0_ & 0x00008000) == 0x00008000);
}
/**
* optional .com.avos.avoscloud.ReadCommand readMessage = 107;
*/
public com.avos.avoscloud.Messages.ReadCommand getReadMessage() {
if (readMessageBuilder_ == null) {
return readMessage_ == null ? com.avos.avoscloud.Messages.ReadCommand.getDefaultInstance() : readMessage_;
} else {
return readMessageBuilder_.getMessage();
}
}
/**
* optional .com.avos.avoscloud.ReadCommand readMessage = 107;
*/
public Builder setReadMessage(com.avos.avoscloud.Messages.ReadCommand value) {
if (readMessageBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
readMessage_ = value;
onChanged();
} else {
readMessageBuilder_.setMessage(value);
}
bitField0_ |= 0x00008000;
return this;
}
/**
* optional .com.avos.avoscloud.ReadCommand readMessage = 107;
*/
public Builder setReadMessage(
com.avos.avoscloud.Messages.ReadCommand.Builder builderForValue) {
if (readMessageBuilder_ == null) {
readMessage_ = builderForValue.build();
onChanged();
} else {
readMessageBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00008000;
return this;
}
/**
* optional .com.avos.avoscloud.ReadCommand readMessage = 107;
*/
public Builder mergeReadMessage(com.avos.avoscloud.Messages.ReadCommand value) {
if (readMessageBuilder_ == null) {
if (((bitField0_ & 0x00008000) == 0x00008000) &&
readMessage_ != null &&
readMessage_ != com.avos.avoscloud.Messages.ReadCommand.getDefaultInstance()) {
readMessage_ =
com.avos.avoscloud.Messages.ReadCommand.newBuilder(readMessage_).mergeFrom(value).buildPartial();
} else {
readMessage_ = value;
}
onChanged();
} else {
readMessageBuilder_.mergeFrom(value);
}
bitField0_ |= 0x00008000;
return this;
}
/**
* optional .com.avos.avoscloud.ReadCommand readMessage = 107;
*/
public Builder clearReadMessage() {
if (readMessageBuilder_ == null) {
readMessage_ = null;
onChanged();
} else {
readMessageBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00008000);
return this;
}
/**
* optional .com.avos.avoscloud.ReadCommand readMessage = 107;
*/
public com.avos.avoscloud.Messages.ReadCommand.Builder getReadMessageBuilder() {
bitField0_ |= 0x00008000;
onChanged();
return getReadMessageFieldBuilder().getBuilder();
}
/**
* optional .com.avos.avoscloud.ReadCommand readMessage = 107;
*/
public com.avos.avoscloud.Messages.ReadCommandOrBuilder getReadMessageOrBuilder() {
if (readMessageBuilder_ != null) {
return readMessageBuilder_.getMessageOrBuilder();
} else {
return readMessage_ == null ?
com.avos.avoscloud.Messages.ReadCommand.getDefaultInstance() : readMessage_;
}
}
/**
* optional .com.avos.avoscloud.ReadCommand readMessage = 107;
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.avos.avoscloud.Messages.ReadCommand, com.avos.avoscloud.Messages.ReadCommand.Builder, com.avos.avoscloud.Messages.ReadCommandOrBuilder>
getReadMessageFieldBuilder() {
if (readMessageBuilder_ == null) {
readMessageBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
com.avos.avoscloud.Messages.ReadCommand, com.avos.avoscloud.Messages.ReadCommand.Builder, com.avos.avoscloud.Messages.ReadCommandOrBuilder>(
getReadMessage(),
getParentForChildren(),
isClean());
readMessage_ = null;
}
return readMessageBuilder_;
}
private com.avos.avoscloud.Messages.RcpCommand rcpMessage_ = null;
private com.google.protobuf.SingleFieldBuilderV3<
com.avos.avoscloud.Messages.RcpCommand, com.avos.avoscloud.Messages.RcpCommand.Builder, com.avos.avoscloud.Messages.RcpCommandOrBuilder> rcpMessageBuilder_;
/**
* optional .com.avos.avoscloud.RcpCommand rcpMessage = 108;
*/
public boolean hasRcpMessage() {
return ((bitField0_ & 0x00010000) == 0x00010000);
}
/**
* optional .com.avos.avoscloud.RcpCommand rcpMessage = 108;
*/
public com.avos.avoscloud.Messages.RcpCommand getRcpMessage() {
if (rcpMessageBuilder_ == null) {
return rcpMessage_ == null ? com.avos.avoscloud.Messages.RcpCommand.getDefaultInstance() : rcpMessage_;
} else {
return rcpMessageBuilder_.getMessage();
}
}
/**
* optional .com.avos.avoscloud.RcpCommand rcpMessage = 108;
*/
public Builder setRcpMessage(com.avos.avoscloud.Messages.RcpCommand value) {
if (rcpMessageBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
rcpMessage_ = value;
onChanged();
} else {
rcpMessageBuilder_.setMessage(value);
}
bitField0_ |= 0x00010000;
return this;
}
/**
* optional .com.avos.avoscloud.RcpCommand rcpMessage = 108;
*/
public Builder setRcpMessage(
com.avos.avoscloud.Messages.RcpCommand.Builder builderForValue) {
if (rcpMessageBuilder_ == null) {
rcpMessage_ = builderForValue.build();
onChanged();
} else {
rcpMessageBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00010000;
return this;
}
/**
* optional .com.avos.avoscloud.RcpCommand rcpMessage = 108;
*/
public Builder mergeRcpMessage(com.avos.avoscloud.Messages.RcpCommand value) {
if (rcpMessageBuilder_ == null) {
if (((bitField0_ & 0x00010000) == 0x00010000) &&
rcpMessage_ != null &&
rcpMessage_ != com.avos.avoscloud.Messages.RcpCommand.getDefaultInstance()) {
rcpMessage_ =
com.avos.avoscloud.Messages.RcpCommand.newBuilder(rcpMessage_).mergeFrom(value).buildPartial();
} else {
rcpMessage_ = value;
}
onChanged();
} else {
rcpMessageBuilder_.mergeFrom(value);
}
bitField0_ |= 0x00010000;
return this;
}
/**
* optional .com.avos.avoscloud.RcpCommand rcpMessage = 108;
*/
public Builder clearRcpMessage() {
if (rcpMessageBuilder_ == null) {
rcpMessage_ = null;
onChanged();
} else {
rcpMessageBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00010000);
return this;
}
/**
* optional .com.avos.avoscloud.RcpCommand rcpMessage = 108;
*/
public com.avos.avoscloud.Messages.RcpCommand.Builder getRcpMessageBuilder() {
bitField0_ |= 0x00010000;
onChanged();
return getRcpMessageFieldBuilder().getBuilder();
}
/**
* optional .com.avos.avoscloud.RcpCommand rcpMessage = 108;
*/
public com.avos.avoscloud.Messages.RcpCommandOrBuilder getRcpMessageOrBuilder() {
if (rcpMessageBuilder_ != null) {
return rcpMessageBuilder_.getMessageOrBuilder();
} else {
return rcpMessage_ == null ?
com.avos.avoscloud.Messages.RcpCommand.getDefaultInstance() : rcpMessage_;
}
}
/**
* optional .com.avos.avoscloud.RcpCommand rcpMessage = 108;
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.avos.avoscloud.Messages.RcpCommand, com.avos.avoscloud.Messages.RcpCommand.Builder, com.avos.avoscloud.Messages.RcpCommandOrBuilder>
getRcpMessageFieldBuilder() {
if (rcpMessageBuilder_ == null) {
rcpMessageBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
com.avos.avoscloud.Messages.RcpCommand, com.avos.avoscloud.Messages.RcpCommand.Builder, com.avos.avoscloud.Messages.RcpCommandOrBuilder>(
getRcpMessage(),
getParentForChildren(),
isClean());
rcpMessage_ = null;
}
return rcpMessageBuilder_;
}
private com.avos.avoscloud.Messages.LogsCommand logsMessage_ = null;
private com.google.protobuf.SingleFieldBuilderV3<
com.avos.avoscloud.Messages.LogsCommand, com.avos.avoscloud.Messages.LogsCommand.Builder, com.avos.avoscloud.Messages.LogsCommandOrBuilder> logsMessageBuilder_;
/**
* optional .com.avos.avoscloud.LogsCommand logsMessage = 109;
*/
public boolean hasLogsMessage() {
return ((bitField0_ & 0x00020000) == 0x00020000);
}
/**
* optional .com.avos.avoscloud.LogsCommand logsMessage = 109;
*/
public com.avos.avoscloud.Messages.LogsCommand getLogsMessage() {
if (logsMessageBuilder_ == null) {
return logsMessage_ == null ? com.avos.avoscloud.Messages.LogsCommand.getDefaultInstance() : logsMessage_;
} else {
return logsMessageBuilder_.getMessage();
}
}
/**
* optional .com.avos.avoscloud.LogsCommand logsMessage = 109;
*/
public Builder setLogsMessage(com.avos.avoscloud.Messages.LogsCommand value) {
if (logsMessageBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
logsMessage_ = value;
onChanged();
} else {
logsMessageBuilder_.setMessage(value);
}
bitField0_ |= 0x00020000;
return this;
}
/**
* optional .com.avos.avoscloud.LogsCommand logsMessage = 109;
*/
public Builder setLogsMessage(
com.avos.avoscloud.Messages.LogsCommand.Builder builderForValue) {
if (logsMessageBuilder_ == null) {
logsMessage_ = builderForValue.build();
onChanged();
} else {
logsMessageBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00020000;
return this;
}
/**
* optional .com.avos.avoscloud.LogsCommand logsMessage = 109;
*/
public Builder mergeLogsMessage(com.avos.avoscloud.Messages.LogsCommand value) {
if (logsMessageBuilder_ == null) {
if (((bitField0_ & 0x00020000) == 0x00020000) &&
logsMessage_ != null &&
logsMessage_ != com.avos.avoscloud.Messages.LogsCommand.getDefaultInstance()) {
logsMessage_ =
com.avos.avoscloud.Messages.LogsCommand.newBuilder(logsMessage_).mergeFrom(value).buildPartial();
} else {
logsMessage_ = value;
}
onChanged();
} else {
logsMessageBuilder_.mergeFrom(value);
}
bitField0_ |= 0x00020000;
return this;
}
/**
* optional .com.avos.avoscloud.LogsCommand logsMessage = 109;
*/
public Builder clearLogsMessage() {
if (logsMessageBuilder_ == null) {
logsMessage_ = null;
onChanged();
} else {
logsMessageBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00020000);
return this;
}
/**
* optional .com.avos.avoscloud.LogsCommand logsMessage = 109;
*/
public com.avos.avoscloud.Messages.LogsCommand.Builder getLogsMessageBuilder() {
bitField0_ |= 0x00020000;
onChanged();
return getLogsMessageFieldBuilder().getBuilder();
}
/**
* optional .com.avos.avoscloud.LogsCommand logsMessage = 109;
*/
public com.avos.avoscloud.Messages.LogsCommandOrBuilder getLogsMessageOrBuilder() {
if (logsMessageBuilder_ != null) {
return logsMessageBuilder_.getMessageOrBuilder();
} else {
return logsMessage_ == null ?
com.avos.avoscloud.Messages.LogsCommand.getDefaultInstance() : logsMessage_;
}
}
/**
* optional .com.avos.avoscloud.LogsCommand logsMessage = 109;
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.avos.avoscloud.Messages.LogsCommand, com.avos.avoscloud.Messages.LogsCommand.Builder, com.avos.avoscloud.Messages.LogsCommandOrBuilder>
getLogsMessageFieldBuilder() {
if (logsMessageBuilder_ == null) {
logsMessageBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
com.avos.avoscloud.Messages.LogsCommand, com.avos.avoscloud.Messages.LogsCommand.Builder, com.avos.avoscloud.Messages.LogsCommandOrBuilder>(
getLogsMessage(),
getParentForChildren(),
isClean());
logsMessage_ = null;
}
return logsMessageBuilder_;
}
private com.avos.avoscloud.Messages.ConvCommand convMessage_ = null;
private com.google.protobuf.SingleFieldBuilderV3<
com.avos.avoscloud.Messages.ConvCommand, com.avos.avoscloud.Messages.ConvCommand.Builder, com.avos.avoscloud.Messages.ConvCommandOrBuilder> convMessageBuilder_;
/**
* optional .com.avos.avoscloud.ConvCommand convMessage = 110;
*/
public boolean hasConvMessage() {
return ((bitField0_ & 0x00040000) == 0x00040000);
}
/**
* optional .com.avos.avoscloud.ConvCommand convMessage = 110;
*/
public com.avos.avoscloud.Messages.ConvCommand getConvMessage() {
if (convMessageBuilder_ == null) {
return convMessage_ == null ? com.avos.avoscloud.Messages.ConvCommand.getDefaultInstance() : convMessage_;
} else {
return convMessageBuilder_.getMessage();
}
}
/**
* optional .com.avos.avoscloud.ConvCommand convMessage = 110;
*/
public Builder setConvMessage(com.avos.avoscloud.Messages.ConvCommand value) {
if (convMessageBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
convMessage_ = value;
onChanged();
} else {
convMessageBuilder_.setMessage(value);
}
bitField0_ |= 0x00040000;
return this;
}
/**
* optional .com.avos.avoscloud.ConvCommand convMessage = 110;
*/
public Builder setConvMessage(
com.avos.avoscloud.Messages.ConvCommand.Builder builderForValue) {
if (convMessageBuilder_ == null) {
convMessage_ = builderForValue.build();
onChanged();
} else {
convMessageBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00040000;
return this;
}
/**
* optional .com.avos.avoscloud.ConvCommand convMessage = 110;
*/
public Builder mergeConvMessage(com.avos.avoscloud.Messages.ConvCommand value) {
if (convMessageBuilder_ == null) {
if (((bitField0_ & 0x00040000) == 0x00040000) &&
convMessage_ != null &&
convMessage_ != com.avos.avoscloud.Messages.ConvCommand.getDefaultInstance()) {
convMessage_ =
com.avos.avoscloud.Messages.ConvCommand.newBuilder(convMessage_).mergeFrom(value).buildPartial();
} else {
convMessage_ = value;
}
onChanged();
} else {
convMessageBuilder_.mergeFrom(value);
}
bitField0_ |= 0x00040000;
return this;
}
/**
* optional .com.avos.avoscloud.ConvCommand convMessage = 110;
*/
public Builder clearConvMessage() {
if (convMessageBuilder_ == null) {
convMessage_ = null;
onChanged();
} else {
convMessageBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00040000);
return this;
}
/**
* optional .com.avos.avoscloud.ConvCommand convMessage = 110;
*/
public com.avos.avoscloud.Messages.ConvCommand.Builder getConvMessageBuilder() {
bitField0_ |= 0x00040000;
onChanged();
return getConvMessageFieldBuilder().getBuilder();
}
/**
* optional .com.avos.avoscloud.ConvCommand convMessage = 110;
*/
public com.avos.avoscloud.Messages.ConvCommandOrBuilder getConvMessageOrBuilder() {
if (convMessageBuilder_ != null) {
return convMessageBuilder_.getMessageOrBuilder();
} else {
return convMessage_ == null ?
com.avos.avoscloud.Messages.ConvCommand.getDefaultInstance() : convMessage_;
}
}
/**
* optional .com.avos.avoscloud.ConvCommand convMessage = 110;
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.avos.avoscloud.Messages.ConvCommand, com.avos.avoscloud.Messages.ConvCommand.Builder, com.avos.avoscloud.Messages.ConvCommandOrBuilder>
getConvMessageFieldBuilder() {
if (convMessageBuilder_ == null) {
convMessageBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
com.avos.avoscloud.Messages.ConvCommand, com.avos.avoscloud.Messages.ConvCommand.Builder, com.avos.avoscloud.Messages.ConvCommandOrBuilder>(
getConvMessage(),
getParentForChildren(),
isClean());
convMessage_ = null;
}
return convMessageBuilder_;
}
private com.avos.avoscloud.Messages.RoomCommand roomMessage_ = null;
private com.google.protobuf.SingleFieldBuilderV3<
com.avos.avoscloud.Messages.RoomCommand, com.avos.avoscloud.Messages.RoomCommand.Builder, com.avos.avoscloud.Messages.RoomCommandOrBuilder> roomMessageBuilder_;
/**
* optional .com.avos.avoscloud.RoomCommand roomMessage = 111;
*/
public boolean hasRoomMessage() {
return ((bitField0_ & 0x00080000) == 0x00080000);
}
/**
* optional .com.avos.avoscloud.RoomCommand roomMessage = 111;
*/
public com.avos.avoscloud.Messages.RoomCommand getRoomMessage() {
if (roomMessageBuilder_ == null) {
return roomMessage_ == null ? com.avos.avoscloud.Messages.RoomCommand.getDefaultInstance() : roomMessage_;
} else {
return roomMessageBuilder_.getMessage();
}
}
/**
* optional .com.avos.avoscloud.RoomCommand roomMessage = 111;
*/
public Builder setRoomMessage(com.avos.avoscloud.Messages.RoomCommand value) {
if (roomMessageBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
roomMessage_ = value;
onChanged();
} else {
roomMessageBuilder_.setMessage(value);
}
bitField0_ |= 0x00080000;
return this;
}
/**
* optional .com.avos.avoscloud.RoomCommand roomMessage = 111;
*/
public Builder setRoomMessage(
com.avos.avoscloud.Messages.RoomCommand.Builder builderForValue) {
if (roomMessageBuilder_ == null) {
roomMessage_ = builderForValue.build();
onChanged();
} else {
roomMessageBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00080000;
return this;
}
/**
* optional .com.avos.avoscloud.RoomCommand roomMessage = 111;
*/
public Builder mergeRoomMessage(com.avos.avoscloud.Messages.RoomCommand value) {
if (roomMessageBuilder_ == null) {
if (((bitField0_ & 0x00080000) == 0x00080000) &&
roomMessage_ != null &&
roomMessage_ != com.avos.avoscloud.Messages.RoomCommand.getDefaultInstance()) {
roomMessage_ =
com.avos.avoscloud.Messages.RoomCommand.newBuilder(roomMessage_).mergeFrom(value).buildPartial();
} else {
roomMessage_ = value;
}
onChanged();
} else {
roomMessageBuilder_.mergeFrom(value);
}
bitField0_ |= 0x00080000;
return this;
}
/**
* optional .com.avos.avoscloud.RoomCommand roomMessage = 111;
*/
public Builder clearRoomMessage() {
if (roomMessageBuilder_ == null) {
roomMessage_ = null;
onChanged();
} else {
roomMessageBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00080000);
return this;
}
/**
* optional .com.avos.avoscloud.RoomCommand roomMessage = 111;
*/
public com.avos.avoscloud.Messages.RoomCommand.Builder getRoomMessageBuilder() {
bitField0_ |= 0x00080000;
onChanged();
return getRoomMessageFieldBuilder().getBuilder();
}
/**
* optional .com.avos.avoscloud.RoomCommand roomMessage = 111;
*/
public com.avos.avoscloud.Messages.RoomCommandOrBuilder getRoomMessageOrBuilder() {
if (roomMessageBuilder_ != null) {
return roomMessageBuilder_.getMessageOrBuilder();
} else {
return roomMessage_ == null ?
com.avos.avoscloud.Messages.RoomCommand.getDefaultInstance() : roomMessage_;
}
}
/**
* optional .com.avos.avoscloud.RoomCommand roomMessage = 111;
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.avos.avoscloud.Messages.RoomCommand, com.avos.avoscloud.Messages.RoomCommand.Builder, com.avos.avoscloud.Messages.RoomCommandOrBuilder>
getRoomMessageFieldBuilder() {
if (roomMessageBuilder_ == null) {
roomMessageBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
com.avos.avoscloud.Messages.RoomCommand, com.avos.avoscloud.Messages.RoomCommand.Builder, com.avos.avoscloud.Messages.RoomCommandOrBuilder>(
getRoomMessage(),
getParentForChildren(),
isClean());
roomMessage_ = null;
}
return roomMessageBuilder_;
}
private com.avos.avoscloud.Messages.PresenceCommand presenceMessage_ = null;
private com.google.protobuf.SingleFieldBuilderV3<
com.avos.avoscloud.Messages.PresenceCommand, com.avos.avoscloud.Messages.PresenceCommand.Builder, com.avos.avoscloud.Messages.PresenceCommandOrBuilder> presenceMessageBuilder_;
/**
* optional .com.avos.avoscloud.PresenceCommand presenceMessage = 112;
*/
public boolean hasPresenceMessage() {
return ((bitField0_ & 0x00100000) == 0x00100000);
}
/**
* optional .com.avos.avoscloud.PresenceCommand presenceMessage = 112;
*/
public com.avos.avoscloud.Messages.PresenceCommand getPresenceMessage() {
if (presenceMessageBuilder_ == null) {
return presenceMessage_ == null ? com.avos.avoscloud.Messages.PresenceCommand.getDefaultInstance() : presenceMessage_;
} else {
return presenceMessageBuilder_.getMessage();
}
}
/**
* optional .com.avos.avoscloud.PresenceCommand presenceMessage = 112;
*/
public Builder setPresenceMessage(com.avos.avoscloud.Messages.PresenceCommand value) {
if (presenceMessageBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
presenceMessage_ = value;
onChanged();
} else {
presenceMessageBuilder_.setMessage(value);
}
bitField0_ |= 0x00100000;
return this;
}
/**
* optional .com.avos.avoscloud.PresenceCommand presenceMessage = 112;
*/
public Builder setPresenceMessage(
com.avos.avoscloud.Messages.PresenceCommand.Builder builderForValue) {
if (presenceMessageBuilder_ == null) {
presenceMessage_ = builderForValue.build();
onChanged();
} else {
presenceMessageBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00100000;
return this;
}
/**
* optional .com.avos.avoscloud.PresenceCommand presenceMessage = 112;
*/
public Builder mergePresenceMessage(com.avos.avoscloud.Messages.PresenceCommand value) {
if (presenceMessageBuilder_ == null) {
if (((bitField0_ & 0x00100000) == 0x00100000) &&
presenceMessage_ != null &&
presenceMessage_ != com.avos.avoscloud.Messages.PresenceCommand.getDefaultInstance()) {
presenceMessage_ =
com.avos.avoscloud.Messages.PresenceCommand.newBuilder(presenceMessage_).mergeFrom(value).buildPartial();
} else {
presenceMessage_ = value;
}
onChanged();
} else {
presenceMessageBuilder_.mergeFrom(value);
}
bitField0_ |= 0x00100000;
return this;
}
/**
* optional .com.avos.avoscloud.PresenceCommand presenceMessage = 112;
*/
public Builder clearPresenceMessage() {
if (presenceMessageBuilder_ == null) {
presenceMessage_ = null;
onChanged();
} else {
presenceMessageBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00100000);
return this;
}
/**
* optional .com.avos.avoscloud.PresenceCommand presenceMessage = 112;
*/
public com.avos.avoscloud.Messages.PresenceCommand.Builder getPresenceMessageBuilder() {
bitField0_ |= 0x00100000;
onChanged();
return getPresenceMessageFieldBuilder().getBuilder();
}
/**
* optional .com.avos.avoscloud.PresenceCommand presenceMessage = 112;
*/
public com.avos.avoscloud.Messages.PresenceCommandOrBuilder getPresenceMessageOrBuilder() {
if (presenceMessageBuilder_ != null) {
return presenceMessageBuilder_.getMessageOrBuilder();
} else {
return presenceMessage_ == null ?
com.avos.avoscloud.Messages.PresenceCommand.getDefaultInstance() : presenceMessage_;
}
}
/**
* optional .com.avos.avoscloud.PresenceCommand presenceMessage = 112;
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.avos.avoscloud.Messages.PresenceCommand, com.avos.avoscloud.Messages.PresenceCommand.Builder, com.avos.avoscloud.Messages.PresenceCommandOrBuilder>
getPresenceMessageFieldBuilder() {
if (presenceMessageBuilder_ == null) {
presenceMessageBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
com.avos.avoscloud.Messages.PresenceCommand, com.avos.avoscloud.Messages.PresenceCommand.Builder, com.avos.avoscloud.Messages.PresenceCommandOrBuilder>(
getPresenceMessage(),
getParentForChildren(),
isClean());
presenceMessage_ = null;
}
return presenceMessageBuilder_;
}
private com.avos.avoscloud.Messages.ReportCommand reportMessage_ = null;
private com.google.protobuf.SingleFieldBuilderV3<
com.avos.avoscloud.Messages.ReportCommand, com.avos.avoscloud.Messages.ReportCommand.Builder, com.avos.avoscloud.Messages.ReportCommandOrBuilder> reportMessageBuilder_;
/**
* optional .com.avos.avoscloud.ReportCommand reportMessage = 113;
*/
public boolean hasReportMessage() {
return ((bitField0_ & 0x00200000) == 0x00200000);
}
/**
* optional .com.avos.avoscloud.ReportCommand reportMessage = 113;
*/
public com.avos.avoscloud.Messages.ReportCommand getReportMessage() {
if (reportMessageBuilder_ == null) {
return reportMessage_ == null ? com.avos.avoscloud.Messages.ReportCommand.getDefaultInstance() : reportMessage_;
} else {
return reportMessageBuilder_.getMessage();
}
}
/**
* optional .com.avos.avoscloud.ReportCommand reportMessage = 113;
*/
public Builder setReportMessage(com.avos.avoscloud.Messages.ReportCommand value) {
if (reportMessageBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
reportMessage_ = value;
onChanged();
} else {
reportMessageBuilder_.setMessage(value);
}
bitField0_ |= 0x00200000;
return this;
}
/**
* optional .com.avos.avoscloud.ReportCommand reportMessage = 113;
*/
public Builder setReportMessage(
com.avos.avoscloud.Messages.ReportCommand.Builder builderForValue) {
if (reportMessageBuilder_ == null) {
reportMessage_ = builderForValue.build();
onChanged();
} else {
reportMessageBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00200000;
return this;
}
/**
* optional .com.avos.avoscloud.ReportCommand reportMessage = 113;
*/
public Builder mergeReportMessage(com.avos.avoscloud.Messages.ReportCommand value) {
if (reportMessageBuilder_ == null) {
if (((bitField0_ & 0x00200000) == 0x00200000) &&
reportMessage_ != null &&
reportMessage_ != com.avos.avoscloud.Messages.ReportCommand.getDefaultInstance()) {
reportMessage_ =
com.avos.avoscloud.Messages.ReportCommand.newBuilder(reportMessage_).mergeFrom(value).buildPartial();
} else {
reportMessage_ = value;
}
onChanged();
} else {
reportMessageBuilder_.mergeFrom(value);
}
bitField0_ |= 0x00200000;
return this;
}
/**
* optional .com.avos.avoscloud.ReportCommand reportMessage = 113;
*/
public Builder clearReportMessage() {
if (reportMessageBuilder_ == null) {
reportMessage_ = null;
onChanged();
} else {
reportMessageBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00200000);
return this;
}
/**
* optional .com.avos.avoscloud.ReportCommand reportMessage = 113;
*/
public com.avos.avoscloud.Messages.ReportCommand.Builder getReportMessageBuilder() {
bitField0_ |= 0x00200000;
onChanged();
return getReportMessageFieldBuilder().getBuilder();
}
/**
* optional .com.avos.avoscloud.ReportCommand reportMessage = 113;
*/
public com.avos.avoscloud.Messages.ReportCommandOrBuilder getReportMessageOrBuilder() {
if (reportMessageBuilder_ != null) {
return reportMessageBuilder_.getMessageOrBuilder();
} else {
return reportMessage_ == null ?
com.avos.avoscloud.Messages.ReportCommand.getDefaultInstance() : reportMessage_;
}
}
/**
* optional .com.avos.avoscloud.ReportCommand reportMessage = 113;
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.avos.avoscloud.Messages.ReportCommand, com.avos.avoscloud.Messages.ReportCommand.Builder, com.avos.avoscloud.Messages.ReportCommandOrBuilder>
getReportMessageFieldBuilder() {
if (reportMessageBuilder_ == null) {
reportMessageBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
com.avos.avoscloud.Messages.ReportCommand, com.avos.avoscloud.Messages.ReportCommand.Builder, com.avos.avoscloud.Messages.ReportCommandOrBuilder>(
getReportMessage(),
getParentForChildren(),
isClean());
reportMessage_ = null;
}
return reportMessageBuilder_;
}
private com.avos.avoscloud.Messages.PatchCommand patchMessage_ = null;
private com.google.protobuf.SingleFieldBuilderV3<
com.avos.avoscloud.Messages.PatchCommand, com.avos.avoscloud.Messages.PatchCommand.Builder, com.avos.avoscloud.Messages.PatchCommandOrBuilder> patchMessageBuilder_;
/**
* optional .com.avos.avoscloud.PatchCommand patchMessage = 114;
*/
public boolean hasPatchMessage() {
return ((bitField0_ & 0x00400000) == 0x00400000);
}
/**
* optional .com.avos.avoscloud.PatchCommand patchMessage = 114;
*/
public com.avos.avoscloud.Messages.PatchCommand getPatchMessage() {
if (patchMessageBuilder_ == null) {
return patchMessage_ == null ? com.avos.avoscloud.Messages.PatchCommand.getDefaultInstance() : patchMessage_;
} else {
return patchMessageBuilder_.getMessage();
}
}
/**
* optional .com.avos.avoscloud.PatchCommand patchMessage = 114;
*/
public Builder setPatchMessage(com.avos.avoscloud.Messages.PatchCommand value) {
if (patchMessageBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
patchMessage_ = value;
onChanged();
} else {
patchMessageBuilder_.setMessage(value);
}
bitField0_ |= 0x00400000;
return this;
}
/**
* optional .com.avos.avoscloud.PatchCommand patchMessage = 114;
*/
public Builder setPatchMessage(
com.avos.avoscloud.Messages.PatchCommand.Builder builderForValue) {
if (patchMessageBuilder_ == null) {
patchMessage_ = builderForValue.build();
onChanged();
} else {
patchMessageBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00400000;
return this;
}
/**
* optional .com.avos.avoscloud.PatchCommand patchMessage = 114;
*/
public Builder mergePatchMessage(com.avos.avoscloud.Messages.PatchCommand value) {
if (patchMessageBuilder_ == null) {
if (((bitField0_ & 0x00400000) == 0x00400000) &&
patchMessage_ != null &&
patchMessage_ != com.avos.avoscloud.Messages.PatchCommand.getDefaultInstance()) {
patchMessage_ =
com.avos.avoscloud.Messages.PatchCommand.newBuilder(patchMessage_).mergeFrom(value).buildPartial();
} else {
patchMessage_ = value;
}
onChanged();
} else {
patchMessageBuilder_.mergeFrom(value);
}
bitField0_ |= 0x00400000;
return this;
}
/**
* optional .com.avos.avoscloud.PatchCommand patchMessage = 114;
*/
public Builder clearPatchMessage() {
if (patchMessageBuilder_ == null) {
patchMessage_ = null;
onChanged();
} else {
patchMessageBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00400000);
return this;
}
/**
* optional .com.avos.avoscloud.PatchCommand patchMessage = 114;
*/
public com.avos.avoscloud.Messages.PatchCommand.Builder getPatchMessageBuilder() {
bitField0_ |= 0x00400000;
onChanged();
return getPatchMessageFieldBuilder().getBuilder();
}
/**
* optional .com.avos.avoscloud.PatchCommand patchMessage = 114;
*/
public com.avos.avoscloud.Messages.PatchCommandOrBuilder getPatchMessageOrBuilder() {
if (patchMessageBuilder_ != null) {
return patchMessageBuilder_.getMessageOrBuilder();
} else {
return patchMessage_ == null ?
com.avos.avoscloud.Messages.PatchCommand.getDefaultInstance() : patchMessage_;
}
}
/**
* optional .com.avos.avoscloud.PatchCommand patchMessage = 114;
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.avos.avoscloud.Messages.PatchCommand, com.avos.avoscloud.Messages.PatchCommand.Builder, com.avos.avoscloud.Messages.PatchCommandOrBuilder>
getPatchMessageFieldBuilder() {
if (patchMessageBuilder_ == null) {
patchMessageBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
com.avos.avoscloud.Messages.PatchCommand, com.avos.avoscloud.Messages.PatchCommand.Builder, com.avos.avoscloud.Messages.PatchCommandOrBuilder>(
getPatchMessage(),
getParentForChildren(),
isClean());
patchMessage_ = null;
}
return patchMessageBuilder_;
}
private com.avos.avoscloud.Messages.PubsubCommand pubsubMessage_ = null;
private com.google.protobuf.SingleFieldBuilderV3<
com.avos.avoscloud.Messages.PubsubCommand, com.avos.avoscloud.Messages.PubsubCommand.Builder, com.avos.avoscloud.Messages.PubsubCommandOrBuilder> pubsubMessageBuilder_;
/**
* optional .com.avos.avoscloud.PubsubCommand pubsubMessage = 115;
*/
public boolean hasPubsubMessage() {
return ((bitField0_ & 0x00800000) == 0x00800000);
}
/**
* optional .com.avos.avoscloud.PubsubCommand pubsubMessage = 115;
*/
public com.avos.avoscloud.Messages.PubsubCommand getPubsubMessage() {
if (pubsubMessageBuilder_ == null) {
return pubsubMessage_ == null ? com.avos.avoscloud.Messages.PubsubCommand.getDefaultInstance() : pubsubMessage_;
} else {
return pubsubMessageBuilder_.getMessage();
}
}
/**
* optional .com.avos.avoscloud.PubsubCommand pubsubMessage = 115;
*/
public Builder setPubsubMessage(com.avos.avoscloud.Messages.PubsubCommand value) {
if (pubsubMessageBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
pubsubMessage_ = value;
onChanged();
} else {
pubsubMessageBuilder_.setMessage(value);
}
bitField0_ |= 0x00800000;
return this;
}
/**
* optional .com.avos.avoscloud.PubsubCommand pubsubMessage = 115;
*/
public Builder setPubsubMessage(
com.avos.avoscloud.Messages.PubsubCommand.Builder builderForValue) {
if (pubsubMessageBuilder_ == null) {
pubsubMessage_ = builderForValue.build();
onChanged();
} else {
pubsubMessageBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00800000;
return this;
}
/**
* optional .com.avos.avoscloud.PubsubCommand pubsubMessage = 115;
*/
public Builder mergePubsubMessage(com.avos.avoscloud.Messages.PubsubCommand value) {
if (pubsubMessageBuilder_ == null) {
if (((bitField0_ & 0x00800000) == 0x00800000) &&
pubsubMessage_ != null &&
pubsubMessage_ != com.avos.avoscloud.Messages.PubsubCommand.getDefaultInstance()) {
pubsubMessage_ =
com.avos.avoscloud.Messages.PubsubCommand.newBuilder(pubsubMessage_).mergeFrom(value).buildPartial();
} else {
pubsubMessage_ = value;
}
onChanged();
} else {
pubsubMessageBuilder_.mergeFrom(value);
}
bitField0_ |= 0x00800000;
return this;
}
/**
* optional .com.avos.avoscloud.PubsubCommand pubsubMessage = 115;
*/
public Builder clearPubsubMessage() {
if (pubsubMessageBuilder_ == null) {
pubsubMessage_ = null;
onChanged();
} else {
pubsubMessageBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00800000);
return this;
}
/**
* optional .com.avos.avoscloud.PubsubCommand pubsubMessage = 115;
*/
public com.avos.avoscloud.Messages.PubsubCommand.Builder getPubsubMessageBuilder() {
bitField0_ |= 0x00800000;
onChanged();
return getPubsubMessageFieldBuilder().getBuilder();
}
/**
* optional .com.avos.avoscloud.PubsubCommand pubsubMessage = 115;
*/
public com.avos.avoscloud.Messages.PubsubCommandOrBuilder getPubsubMessageOrBuilder() {
if (pubsubMessageBuilder_ != null) {
return pubsubMessageBuilder_.getMessageOrBuilder();
} else {
return pubsubMessage_ == null ?
com.avos.avoscloud.Messages.PubsubCommand.getDefaultInstance() : pubsubMessage_;
}
}
/**
* optional .com.avos.avoscloud.PubsubCommand pubsubMessage = 115;
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.avos.avoscloud.Messages.PubsubCommand, com.avos.avoscloud.Messages.PubsubCommand.Builder, com.avos.avoscloud.Messages.PubsubCommandOrBuilder>
getPubsubMessageFieldBuilder() {
if (pubsubMessageBuilder_ == null) {
pubsubMessageBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
com.avos.avoscloud.Messages.PubsubCommand, com.avos.avoscloud.Messages.PubsubCommand.Builder, com.avos.avoscloud.Messages.PubsubCommandOrBuilder>(
getPubsubMessage(),
getParentForChildren(),
isClean());
pubsubMessage_ = null;
}
return pubsubMessageBuilder_;
}
private com.avos.avoscloud.Messages.BlacklistCommand blacklistMessage_ = null;
private com.google.protobuf.SingleFieldBuilderV3<
com.avos.avoscloud.Messages.BlacklistCommand, com.avos.avoscloud.Messages.BlacklistCommand.Builder, com.avos.avoscloud.Messages.BlacklistCommandOrBuilder> blacklistMessageBuilder_;
/**
* optional .com.avos.avoscloud.BlacklistCommand blacklistMessage = 116;
*/
public boolean hasBlacklistMessage() {
return ((bitField0_ & 0x01000000) == 0x01000000);
}
/**
* optional .com.avos.avoscloud.BlacklistCommand blacklistMessage = 116;
*/
public com.avos.avoscloud.Messages.BlacklistCommand getBlacklistMessage() {
if (blacklistMessageBuilder_ == null) {
return blacklistMessage_ == null ? com.avos.avoscloud.Messages.BlacklistCommand.getDefaultInstance() : blacklistMessage_;
} else {
return blacklistMessageBuilder_.getMessage();
}
}
/**
* optional .com.avos.avoscloud.BlacklistCommand blacklistMessage = 116;
*/
public Builder setBlacklistMessage(com.avos.avoscloud.Messages.BlacklistCommand value) {
if (blacklistMessageBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
blacklistMessage_ = value;
onChanged();
} else {
blacklistMessageBuilder_.setMessage(value);
}
bitField0_ |= 0x01000000;
return this;
}
/**
* optional .com.avos.avoscloud.BlacklistCommand blacklistMessage = 116;
*/
public Builder setBlacklistMessage(
com.avos.avoscloud.Messages.BlacklistCommand.Builder builderForValue) {
if (blacklistMessageBuilder_ == null) {
blacklistMessage_ = builderForValue.build();
onChanged();
} else {
blacklistMessageBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x01000000;
return this;
}
/**
* optional .com.avos.avoscloud.BlacklistCommand blacklistMessage = 116;
*/
public Builder mergeBlacklistMessage(com.avos.avoscloud.Messages.BlacklistCommand value) {
if (blacklistMessageBuilder_ == null) {
if (((bitField0_ & 0x01000000) == 0x01000000) &&
blacklistMessage_ != null &&
blacklistMessage_ != com.avos.avoscloud.Messages.BlacklistCommand.getDefaultInstance()) {
blacklistMessage_ =
com.avos.avoscloud.Messages.BlacklistCommand.newBuilder(blacklistMessage_).mergeFrom(value).buildPartial();
} else {
blacklistMessage_ = value;
}
onChanged();
} else {
blacklistMessageBuilder_.mergeFrom(value);
}
bitField0_ |= 0x01000000;
return this;
}
/**
* optional .com.avos.avoscloud.BlacklistCommand blacklistMessage = 116;
*/
public Builder clearBlacklistMessage() {
if (blacklistMessageBuilder_ == null) {
blacklistMessage_ = null;
onChanged();
} else {
blacklistMessageBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x01000000);
return this;
}
/**
* optional .com.avos.avoscloud.BlacklistCommand blacklistMessage = 116;
*/
public com.avos.avoscloud.Messages.BlacklistCommand.Builder getBlacklistMessageBuilder() {
bitField0_ |= 0x01000000;
onChanged();
return getBlacklistMessageFieldBuilder().getBuilder();
}
/**
* optional .com.avos.avoscloud.BlacklistCommand blacklistMessage = 116;
*/
public com.avos.avoscloud.Messages.BlacklistCommandOrBuilder getBlacklistMessageOrBuilder() {
if (blacklistMessageBuilder_ != null) {
return blacklistMessageBuilder_.getMessageOrBuilder();
} else {
return blacklistMessage_ == null ?
com.avos.avoscloud.Messages.BlacklistCommand.getDefaultInstance() : blacklistMessage_;
}
}
/**
* optional .com.avos.avoscloud.BlacklistCommand blacklistMessage = 116;
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.avos.avoscloud.Messages.BlacklistCommand, com.avos.avoscloud.Messages.BlacklistCommand.Builder, com.avos.avoscloud.Messages.BlacklistCommandOrBuilder>
getBlacklistMessageFieldBuilder() {
if (blacklistMessageBuilder_ == null) {
blacklistMessageBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
com.avos.avoscloud.Messages.BlacklistCommand, com.avos.avoscloud.Messages.BlacklistCommand.Builder, com.avos.avoscloud.Messages.BlacklistCommandOrBuilder>(
getBlacklistMessage(),
getParentForChildren(),
isClean());
blacklistMessage_ = null;
}
return blacklistMessageBuilder_;
}
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:com.avos.avoscloud.GenericCommand)
}
// @@protoc_insertion_point(class_scope:com.avos.avoscloud.GenericCommand)
private static final com.avos.avoscloud.Messages.GenericCommand DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new com.avos.avoscloud.Messages.GenericCommand();
}
public static com.avos.avoscloud.Messages.GenericCommand getDefaultInstance() {
return DEFAULT_INSTANCE;
}
@java.lang.Deprecated public static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
public GenericCommand parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new GenericCommand(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
public com.avos.avoscloud.Messages.GenericCommand getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_com_avos_avoscloud_JsonObjectMessage_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_com_avos_avoscloud_JsonObjectMessage_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_com_avos_avoscloud_UnreadTuple_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_com_avos_avoscloud_UnreadTuple_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_com_avos_avoscloud_LogItem_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_com_avos_avoscloud_LogItem_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_com_avos_avoscloud_ConvMemberInfo_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_com_avos_avoscloud_ConvMemberInfo_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_com_avos_avoscloud_DataCommand_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_com_avos_avoscloud_DataCommand_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_com_avos_avoscloud_SessionCommand_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_com_avos_avoscloud_SessionCommand_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_com_avos_avoscloud_ErrorCommand_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_com_avos_avoscloud_ErrorCommand_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_com_avos_avoscloud_DirectCommand_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_com_avos_avoscloud_DirectCommand_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_com_avos_avoscloud_AckCommand_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_com_avos_avoscloud_AckCommand_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_com_avos_avoscloud_UnreadCommand_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_com_avos_avoscloud_UnreadCommand_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_com_avos_avoscloud_ConvCommand_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_com_avos_avoscloud_ConvCommand_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_com_avos_avoscloud_RoomCommand_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_com_avos_avoscloud_RoomCommand_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_com_avos_avoscloud_LogsCommand_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_com_avos_avoscloud_LogsCommand_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_com_avos_avoscloud_RcpCommand_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_com_avos_avoscloud_RcpCommand_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_com_avos_avoscloud_ReadTuple_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_com_avos_avoscloud_ReadTuple_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_com_avos_avoscloud_MaxReadTuple_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_com_avos_avoscloud_MaxReadTuple_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_com_avos_avoscloud_ReadCommand_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_com_avos_avoscloud_ReadCommand_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_com_avos_avoscloud_PresenceCommand_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_com_avos_avoscloud_PresenceCommand_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_com_avos_avoscloud_ReportCommand_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_com_avos_avoscloud_ReportCommand_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_com_avos_avoscloud_PatchItem_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_com_avos_avoscloud_PatchItem_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_com_avos_avoscloud_PatchCommand_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_com_avos_avoscloud_PatchCommand_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_com_avos_avoscloud_PubsubCommand_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_com_avos_avoscloud_PubsubCommand_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_com_avos_avoscloud_BlacklistCommand_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_com_avos_avoscloud_BlacklistCommand_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_com_avos_avoscloud_GenericCommand_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_com_avos_avoscloud_GenericCommand_fieldAccessorTable;
public static com.google.protobuf.Descriptors.FileDescriptor
getDescriptor() {
return descriptor;
}
private static com.google.protobuf.Descriptors.FileDescriptor
descriptor;
static {
java.lang.String[] descriptorData = {
"\n\016messages.proto\022\022com.avos.avoscloud\"!\n\021" +
"JsonObjectMessage\022\014\n\004data\030\001 \002(\t\"\266\001\n\013Unre" +
"adTuple\022\013\n\003cid\030\001 \002(\t\022\016\n\006unread\030\002 \002(\005\022\013\n\003" +
"mid\030\003 \001(\t\022\021\n\ttimestamp\030\004 \001(\003\022\014\n\004from\030\005 \001" +
"(\t\022\014\n\004data\030\006 \001(\t\022\026\n\016patchTimestamp\030\007 \001(\003" +
"\022\021\n\tmentioned\030\010 \001(\010\022\021\n\tbinaryMsg\030\t \001(\014\022\020" +
"\n\010convType\030\n \001(\005\"\306\001\n\007LogItem\022\014\n\004from\030\001 \001" +
"(\t\022\014\n\004data\030\002 \001(\t\022\021\n\ttimestamp\030\003 \001(\003\022\r\n\005m" +
"sgId\030\004 \001(\t\022\r\n\005ackAt\030\005 \001(\003\022\016\n\006readAt\030\006 \001(" +
"\003\022\026\n\016patchTimestamp\030\007 \001(\003\022\022\n\nmentionAll\030",
"\010 \001(\010\022\023\n\013mentionPids\030\t \003(\t\022\013\n\003bin\030\n \001(\010\022" +
"\020\n\010convType\030\013 \001(\005\";\n\016ConvMemberInfo\022\013\n\003p" +
"id\030\001 \001(\t\022\014\n\004role\030\002 \001(\t\022\016\n\006infoId\030\003 \001(\t\"_" +
"\n\013DataCommand\022\013\n\003ids\030\001 \003(\t\0222\n\003msg\030\002 \003(\0132" +
"%.com.avos.avoscloud.JsonObjectMessage\022\017" +
"\n\007offline\030\003 \001(\010\"\321\002\n\016SessionCommand\022\t\n\001t\030" +
"\001 \001(\003\022\t\n\001n\030\002 \001(\t\022\t\n\001s\030\003 \001(\t\022\n\n\002ua\030\004 \001(\t\022" +
"\t\n\001r\030\005 \001(\010\022\013\n\003tag\030\006 \001(\t\022\020\n\010deviceId\030\007 \001(" +
"\t\022\026\n\016sessionPeerIds\030\010 \003(\t\022\034\n\024onlineSessi" +
"onPeerIds\030\t \003(\t\022\n\n\002st\030\n \001(\t\022\r\n\005stTtl\030\013 \001",
"(\005\022\014\n\004code\030\014 \001(\005\022\016\n\006reason\030\r \001(\t\022\023\n\013devi" +
"ceToken\030\016 \001(\t\022\n\n\002sp\030\017 \001(\010\022\016\n\006detail\030\020 \001(" +
"\t\022\033\n\023lastUnreadNotifTime\030\021 \001(\003\022\025\n\rlastPa" +
"tchTime\030\022 \001(\003\022\024\n\014configBitmap\030\023 \001(\003\"k\n\014E" +
"rrorCommand\022\014\n\004code\030\001 \002(\005\022\016\n\006reason\030\002 \002(" +
"\t\022\017\n\007appCode\030\003 \001(\005\022\016\n\006detail\030\004 \001(\t\022\014\n\004pi" +
"ds\030\005 \003(\t\022\016\n\006appMsg\030\006 \001(\t\"\336\002\n\rDirectComma" +
"nd\022\013\n\003msg\030\001 \001(\t\022\013\n\003uid\030\002 \001(\t\022\022\n\nfromPeer" +
"Id\030\003 \001(\t\022\021\n\ttimestamp\030\004 \001(\003\022\017\n\007offline\030\005" +
" \001(\010\022\017\n\007hasMore\030\006 \001(\010\022\021\n\ttoPeerIds\030\007 \003(\t",
"\022\t\n\001r\030\n \001(\010\022\013\n\003cid\030\013 \001(\t\022\n\n\002id\030\014 \001(\t\022\021\n\t" +
"transient\030\r \001(\010\022\n\n\002dt\030\016 \001(\t\022\016\n\006roomId\030\017 " +
"\001(\t\022\020\n\010pushData\030\020 \001(\t\022\014\n\004will\030\021 \001(\010\022\026\n\016p" +
"atchTimestamp\030\022 \001(\003\022\021\n\tbinaryMsg\030\023 \001(\014\022\023" +
"\n\013mentionPids\030\024 \003(\t\022\022\n\nmentionAll\030\025 \001(\010\022" +
"\020\n\010convType\030\026 \001(\005\"\266\001\n\nAckCommand\022\014\n\004code" +
"\030\001 \001(\005\022\016\n\006reason\030\002 \001(\t\022\013\n\003mid\030\003 \001(\t\022\013\n\003c" +
"id\030\004 \001(\t\022\t\n\001t\030\005 \001(\003\022\013\n\003uid\030\006 \001(\t\022\016\n\006from" +
"ts\030\007 \001(\003\022\014\n\004tots\030\010 \001(\003\022\014\n\004type\030\t \001(\t\022\013\n\003" +
"ids\030\n \003(\t\022\017\n\007appCode\030\013 \001(\005\022\016\n\006appMsg\030\014 \001",
"(\t\"R\n\rUnreadCommand\022.\n\005convs\030\001 \003(\0132\037.com" +
".avos.avoscloud.UnreadTuple\022\021\n\tnotifTime" +
"\030\002 \001(\003\"\374\006\n\013ConvCommand\022\t\n\001m\030\001 \003(\t\022\021\n\ttra" +
"nsient\030\002 \001(\010\022\016\n\006unique\030\003 \001(\010\022\013\n\003cid\030\004 \001(" +
"\t\022\r\n\005cdate\030\005 \001(\t\022\016\n\006initBy\030\006 \001(\t\022\014\n\004sort" +
"\030\007 \001(\t\022\r\n\005limit\030\010 \001(\005\022\014\n\004skip\030\t \001(\005\022\014\n\004f" +
"lag\030\n \001(\005\022\r\n\005count\030\013 \001(\005\022\r\n\005udate\030\014 \001(\t\022" +
"\t\n\001t\030\r \001(\003\022\t\n\001n\030\016 \001(\t\022\t\n\001s\030\017 \001(\t\022\021\n\tstat" +
"usSub\030\020 \001(\010\022\021\n\tstatusPub\030\021 \001(\010\022\021\n\tstatus" +
"TTL\030\022 \001(\005\022\020\n\010uniqueId\030\023 \001(\t\022\026\n\016targetCli",
"entId\030\024 \001(\t\022\030\n\020maxReadTimestamp\030\025 \001(\003\022\027\n" +
"\017maxAckTimestamp\030\026 \001(\003\022\027\n\017queryAllMember" +
"s\030\027 \001(\010\0227\n\rmaxReadTuples\030\030 \003(\0132 .com.avo" +
"s.avoscloud.MaxReadTuple\022\014\n\004cids\030\031 \003(\t\0220" +
"\n\004info\030\032 \001(\0132\".com.avos.avoscloud.ConvMe" +
"mberInfo\022\020\n\010tempConv\030\033 \001(\010\022\023\n\013tempConvTT" +
"L\030\034 \001(\005\022\023\n\013tempConvIds\030\035 \003(\t\022\023\n\013allowedP" +
"ids\030\036 \003(\t\0224\n\nfailedPids\030\037 \003(\0132 .com.avos" +
".avoscloud.ErrorCommand\022\014\n\004next\030( \001(\t\0226\n" +
"\007results\030d \001(\0132%.com.avos.avoscloud.Json",
"ObjectMessage\0224\n\005where\030e \001(\0132%.com.avos." +
"avoscloud.JsonObjectMessage\0223\n\004attr\030g \001(" +
"\0132%.com.avos.avoscloud.JsonObjectMessage" +
"\022;\n\014attrModified\030h \001(\0132%.com.avos.avoscl" +
"oud.JsonObjectMessage\"x\n\013RoomCommand\022\016\n\006" +
"roomId\030\001 \001(\t\022\t\n\001s\030\002 \001(\t\022\t\n\001t\030\003 \001(\003\022\t\n\001n\030" +
"\004 \001(\t\022\021\n\ttransient\030\005 \001(\010\022\023\n\013roomPeerIds\030" +
"\006 \003(\t\022\020\n\010byPeerId\030\007 \001(\t\"\326\002\n\013LogsCommand\022" +
"\013\n\003cid\030\001 \001(\t\022\t\n\001l\030\002 \001(\005\022\r\n\005limit\030\003 \001(\005\022\t" +
"\n\001t\030\004 \001(\003\022\n\n\002tt\030\005 \001(\003\022\014\n\004tmid\030\006 \001(\t\022\013\n\003m",
"id\030\007 \001(\t\022\020\n\010checksum\030\010 \001(\t\022\016\n\006stored\030\t \001" +
"(\010\022F\n\tdirection\030\n \001(\0162..com.avos.avosclo" +
"ud.LogsCommand.QueryDirection:\003OLD\022\021\n\ttI" +
"ncluded\030\013 \001(\010\022\022\n\nttIncluded\030\014 \001(\010\022\016\n\006lct" +
"ype\030\r \001(\005\022)\n\004logs\030i \003(\0132\033.com.avos.avosc" +
"loud.LogItem\"\"\n\016QueryDirection\022\007\n\003OLD\020\001\022" +
"\007\n\003NEW\020\002\"L\n\nRcpCommand\022\n\n\002id\030\001 \001(\t\022\013\n\003ci" +
"d\030\002 \001(\t\022\t\n\001t\030\003 \001(\003\022\014\n\004read\030\004 \001(\010\022\014\n\004from" +
"\030\005 \001(\t\"8\n\tReadTuple\022\013\n\003cid\030\001 \002(\t\022\021\n\ttime" +
"stamp\030\002 \001(\003\022\013\n\003mid\030\003 \001(\t\"N\n\014MaxReadTuple",
"\022\013\n\003pid\030\001 \001(\t\022\027\n\017maxAckTimestamp\030\002 \001(\003\022\030" +
"\n\020maxReadTimestamp\030\003 \001(\003\"V\n\013ReadCommand\022" +
"\013\n\003cid\030\001 \001(\t\022\014\n\004cids\030\002 \003(\t\022,\n\005convs\030\003 \003(" +
"\0132\035.com.avos.avoscloud.ReadTuple\"f\n\017Pres" +
"enceCommand\022.\n\006status\030\001 \001(\0162\036.com.avos.a" +
"voscloud.StatusType\022\026\n\016sessionPeerIds\030\002 " +
"\003(\t\022\013\n\003cid\030\003 \001(\t\"?\n\rReportCommand\022\022\n\nini" +
"tiative\030\001 \001(\010\022\014\n\004type\030\002 \001(\t\022\014\n\004data\030\003 \001(" +
"\t\"\340\001\n\tPatchItem\022\013\n\003cid\030\001 \001(\t\022\013\n\003mid\030\002 \001(" +
"\t\022\021\n\ttimestamp\030\003 \001(\003\022\016\n\006recall\030\004 \001(\010\022\014\n\004",
"data\030\005 \001(\t\022\026\n\016patchTimestamp\030\006 \001(\003\022\014\n\004fr" +
"om\030\007 \001(\t\022\021\n\tbinaryMsg\030\010 \001(\014\022\022\n\nmentionAl" +
"l\030\t \001(\010\022\023\n\013mentionPids\030\n \003(\t\022\021\n\tpatchCod" +
"e\030\013 \001(\003\022\023\n\013patchReason\030\014 \001(\t\"U\n\014PatchCom" +
"mand\022.\n\007patches\030\001 \003(\0132\035.com.avos.avosclo" +
"ud.PatchItem\022\025\n\rlastPatchTime\030\002 \001(\003\"\246\001\n\r" +
"PubsubCommand\022\013\n\003cid\030\001 \001(\t\022\014\n\004cids\030\002 \003(\t" +
"\022\r\n\005topic\030\003 \001(\t\022\020\n\010subtopic\030\004 \001(\t\022\016\n\006top" +
"ics\030\005 \003(\t\022\021\n\tsubtopics\030\006 \003(\t\0226\n\007results\030" +
"\007 \001(\0132%.com.avos.avoscloud.JsonObjectMes",
"sage\"\205\002\n\020BlacklistCommand\022\016\n\006srcCid\030\001 \001(" +
"\t\022\016\n\006toPids\030\002 \003(\t\022\016\n\006srcPid\030\003 \001(\t\022\016\n\006toC" +
"ids\030\004 \003(\t\022\r\n\005limit\030\005 \001(\005\022\014\n\004next\030\006 \001(\t\022\023" +
"\n\013blockedPids\030\010 \003(\t\022\023\n\013blockedCids\030\t \003(\t" +
"\022\023\n\013allowedPids\030\n \003(\t\0224\n\nfailedPids\030\013 \003(" +
"\0132 .com.avos.avoscloud.ErrorCommand\022\t\n\001t" +
"\030\014 \001(\003\022\t\n\001n\030\r \001(\t\022\t\n\001s\030\016 \001(\t\"\345\010\n\016Generic" +
"Command\022,\n\003cmd\030\001 \001(\0162\037.com.avos.avosclou" +
"d.CommandType\022&\n\002op\030\002 \001(\0162\032.com.avos.avo" +
"scloud.OpType\022\r\n\005appId\030\003 \001(\t\022\016\n\006peerId\030\004",
" \001(\t\022\t\n\001i\030\005 \001(\005\022\026\n\016installationId\030\006 \001(\t\022" +
"\020\n\010priority\030\007 \001(\005\022\017\n\007service\030\010 \001(\005\022\020\n\010se" +
"rverTs\030\t \001(\003\0224\n\013dataMessage\030e \001(\0132\037.com." +
"avos.avoscloud.DataCommand\022:\n\016sessionMes" +
"sage\030f \001(\0132\".com.avos.avoscloud.SessionC" +
"ommand\0226\n\014errorMessage\030g \001(\0132 .com.avos." +
"avoscloud.ErrorCommand\0228\n\rdirectMessage\030" +
"h \001(\0132!.com.avos.avoscloud.DirectCommand" +
"\0222\n\nackMessage\030i \001(\0132\036.com.avos.avosclou" +
"d.AckCommand\0228\n\runreadMessage\030j \001(\0132!.co",
"m.avos.avoscloud.UnreadCommand\0224\n\013readMe" +
"ssage\030k \001(\0132\037.com.avos.avoscloud.ReadCom" +
"mand\0222\n\nrcpMessage\030l \001(\0132\036.com.avos.avos" +
"cloud.RcpCommand\0224\n\013logsMessage\030m \001(\0132\037." +
"com.avos.avoscloud.LogsCommand\0224\n\013convMe" +
"ssage\030n \001(\0132\037.com.avos.avoscloud.ConvCom" +
"mand\0224\n\013roomMessage\030o \001(\0132\037.com.avos.avo" +
"scloud.RoomCommand\022<\n\017presenceMessage\030p " +
"\001(\0132#.com.avos.avoscloud.PresenceCommand" +
"\0228\n\rreportMessage\030q \001(\0132!.com.avos.avosc",
"loud.ReportCommand\0226\n\014patchMessage\030r \001(\013" +
"2 .com.avos.avoscloud.PatchCommand\0228\n\rpu" +
"bsubMessage\030s \001(\0132!.com.avos.avoscloud.P" +
"ubsubCommand\022>\n\020blacklistMessage\030t \001(\0132$" +
".com.avos.avoscloud.BlacklistCommand*\213\002\n" +
"\013CommandType\022\013\n\007session\020\000\022\010\n\004conv\020\001\022\n\n\006d" +
"irect\020\002\022\007\n\003ack\020\003\022\007\n\003rcp\020\004\022\n\n\006unread\020\005\022\010\n" +
"\004logs\020\006\022\t\n\005error\020\007\022\t\n\005login\020\010\022\010\n\004data\020\t\022" +
"\010\n\004room\020\n\022\010\n\004read\020\013\022\014\n\010presence\020\014\022\n\n\006rep" +
"ort\020\r\022\010\n\004echo\020\016\022\014\n\010loggedin\020\017\022\n\n\006logout\020",
"\020\022\r\n\tloggedout\020\021\022\t\n\005patch\020\022\022\n\n\006pubsub\020\023\022" +
"\r\n\tblacklist\020\024\022\n\n\006goaway\020\025*\215\010\n\006OpType\022\010\n" +
"\004open\020\001\022\007\n\003add\020\002\022\n\n\006remove\020\003\022\t\n\005close\020\004\022" +
"\n\n\006opened\020\005\022\n\n\006closed\020\006\022\t\n\005query\020\007\022\020\n\014qu" +
"ery_result\020\010\022\014\n\010conflict\020\t\022\t\n\005added\020\n\022\013\n" +
"\007removed\020\013\022\013\n\007refresh\020\014\022\r\n\trefreshed\020\r\022\t" +
"\n\005start\020\036\022\013\n\007started\020\037\022\n\n\006joined\020 \022\022\n\016me" +
"mbers_joined\020!\022\010\n\004left\020\'\022\020\n\014members_left" +
"\020(\022\013\n\007results\020*\022\t\n\005count\020+\022\n\n\006result\020,\022\n" +
"\n\006update\020-\022\013\n\007updated\020.\022\010\n\004mute\020/\022\n\n\006unm",
"ute\0200\022\n\n\006status\0201\022\013\n\007members\0202\022\014\n\010max_re" +
"ad\0203\022\r\n\tis_member\0204\022\026\n\022member_info_updat" +
"e\0205\022\027\n\023member_info_updated\0206\022\027\n\023member_i" +
"nfo_changed\0207\022\010\n\004join\020P\022\n\n\006invite\020Q\022\t\n\005l" +
"eave\020R\022\010\n\004kick\020S\022\n\n\006reject\020T\022\013\n\007invited\020" +
"U\022\n\n\006kicked\020V\022\n\n\006upload\020d\022\014\n\010uploaded\020e\022" +
"\r\n\tsubscribe\020x\022\016\n\nsubscribed\020y\022\017\n\013unsubs" +
"cribe\020z\022\020\n\014unsubscribed\020{\022\021\n\ris_subscrib" +
"ed\020|\022\013\n\006modify\020\226\001\022\r\n\010modified\020\227\001\022\n\n\005bloc" +
"k\020\252\001\022\014\n\007unblock\020\253\001\022\014\n\007blocked\020\254\001\022\016\n\tunbl",
"ocked\020\255\001\022\024\n\017members_blocked\020\256\001\022\026\n\021member" +
"s_unblocked\020\257\001\022\020\n\013check_block\020\260\001\022\021\n\014chec" +
"k_result\020\261\001\022\017\n\nadd_shutup\020\264\001\022\022\n\rremove_s" +
"hutup\020\265\001\022\021\n\014query_shutup\020\266\001\022\021\n\014shutup_ad" +
"ded\020\267\001\022\023\n\016shutup_removed\020\270\001\022\022\n\rshutup_re" +
"sult\020\271\001\022\r\n\010shutuped\020\272\001\022\017\n\nunshutuped\020\273\001\022" +
"\025\n\020members_shutuped\020\274\001\022\027\n\022members_unshut" +
"uped\020\275\001\022\021\n\014check_shutup\020\276\001*\035\n\nStatusType" +
"\022\006\n\002on\020\001\022\007\n\003off\020\002B\007\242\002\004AVIM"
};
com.google.protobuf.Descriptors.FileDescriptor.InternalDescriptorAssigner assigner =
new com.google.protobuf.Descriptors.FileDescriptor. InternalDescriptorAssigner() {
public com.google.protobuf.ExtensionRegistry assignDescriptors(
com.google.protobuf.Descriptors.FileDescriptor root) {
descriptor = root;
return null;
}
};
com.google.protobuf.Descriptors.FileDescriptor
.internalBuildGeneratedFileFrom(descriptorData,
new com.google.protobuf.Descriptors.FileDescriptor[] {
}, assigner);
internal_static_com_avos_avoscloud_JsonObjectMessage_descriptor =
getDescriptor().getMessageTypes().get(0);
internal_static_com_avos_avoscloud_JsonObjectMessage_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_com_avos_avoscloud_JsonObjectMessage_descriptor,
new java.lang.String[] { "Data", });
internal_static_com_avos_avoscloud_UnreadTuple_descriptor =
getDescriptor().getMessageTypes().get(1);
internal_static_com_avos_avoscloud_UnreadTuple_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_com_avos_avoscloud_UnreadTuple_descriptor,
new java.lang.String[] { "Cid", "Unread", "Mid", "Timestamp", "From", "Data", "PatchTimestamp", "Mentioned", "BinaryMsg", "ConvType", });
internal_static_com_avos_avoscloud_LogItem_descriptor =
getDescriptor().getMessageTypes().get(2);
internal_static_com_avos_avoscloud_LogItem_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_com_avos_avoscloud_LogItem_descriptor,
new java.lang.String[] { "From", "Data", "Timestamp", "MsgId", "AckAt", "ReadAt", "PatchTimestamp", "MentionAll", "MentionPids", "Bin", "ConvType", });
internal_static_com_avos_avoscloud_ConvMemberInfo_descriptor =
getDescriptor().getMessageTypes().get(3);
internal_static_com_avos_avoscloud_ConvMemberInfo_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_com_avos_avoscloud_ConvMemberInfo_descriptor,
new java.lang.String[] { "Pid", "Role", "InfoId", });
internal_static_com_avos_avoscloud_DataCommand_descriptor =
getDescriptor().getMessageTypes().get(4);
internal_static_com_avos_avoscloud_DataCommand_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_com_avos_avoscloud_DataCommand_descriptor,
new java.lang.String[] { "Ids", "Msg", "Offline", });
internal_static_com_avos_avoscloud_SessionCommand_descriptor =
getDescriptor().getMessageTypes().get(5);
internal_static_com_avos_avoscloud_SessionCommand_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_com_avos_avoscloud_SessionCommand_descriptor,
new java.lang.String[] { "T", "N", "S", "Ua", "R", "Tag", "DeviceId", "SessionPeerIds", "OnlineSessionPeerIds", "St", "StTtl", "Code", "Reason", "DeviceToken", "Sp", "Detail", "LastUnreadNotifTime", "LastPatchTime", "ConfigBitmap", });
internal_static_com_avos_avoscloud_ErrorCommand_descriptor =
getDescriptor().getMessageTypes().get(6);
internal_static_com_avos_avoscloud_ErrorCommand_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_com_avos_avoscloud_ErrorCommand_descriptor,
new java.lang.String[] { "Code", "Reason", "AppCode", "Detail", "Pids", "AppMsg", });
internal_static_com_avos_avoscloud_DirectCommand_descriptor =
getDescriptor().getMessageTypes().get(7);
internal_static_com_avos_avoscloud_DirectCommand_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_com_avos_avoscloud_DirectCommand_descriptor,
new java.lang.String[] { "Msg", "Uid", "FromPeerId", "Timestamp", "Offline", "HasMore", "ToPeerIds", "R", "Cid", "Id", "Transient", "Dt", "RoomId", "PushData", "Will", "PatchTimestamp", "BinaryMsg", "MentionPids", "MentionAll", "ConvType", });
internal_static_com_avos_avoscloud_AckCommand_descriptor =
getDescriptor().getMessageTypes().get(8);
internal_static_com_avos_avoscloud_AckCommand_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_com_avos_avoscloud_AckCommand_descriptor,
new java.lang.String[] { "Code", "Reason", "Mid", "Cid", "T", "Uid", "Fromts", "Tots", "Type", "Ids", "AppCode", "AppMsg", });
internal_static_com_avos_avoscloud_UnreadCommand_descriptor =
getDescriptor().getMessageTypes().get(9);
internal_static_com_avos_avoscloud_UnreadCommand_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_com_avos_avoscloud_UnreadCommand_descriptor,
new java.lang.String[] { "Convs", "NotifTime", });
internal_static_com_avos_avoscloud_ConvCommand_descriptor =
getDescriptor().getMessageTypes().get(10);
internal_static_com_avos_avoscloud_ConvCommand_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_com_avos_avoscloud_ConvCommand_descriptor,
new java.lang.String[] { "M", "Transient", "Unique", "Cid", "Cdate", "InitBy", "Sort", "Limit", "Skip", "Flag", "Count", "Udate", "T", "N", "S", "StatusSub", "StatusPub", "StatusTTL", "UniqueId", "TargetClientId", "MaxReadTimestamp", "MaxAckTimestamp", "QueryAllMembers", "MaxReadTuples", "Cids", "Info", "TempConv", "TempConvTTL", "TempConvIds", "AllowedPids", "FailedPids", "Next", "Results", "Where", "Attr", "AttrModified", });
internal_static_com_avos_avoscloud_RoomCommand_descriptor =
getDescriptor().getMessageTypes().get(11);
internal_static_com_avos_avoscloud_RoomCommand_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_com_avos_avoscloud_RoomCommand_descriptor,
new java.lang.String[] { "RoomId", "S", "T", "N", "Transient", "RoomPeerIds", "ByPeerId", });
internal_static_com_avos_avoscloud_LogsCommand_descriptor =
getDescriptor().getMessageTypes().get(12);
internal_static_com_avos_avoscloud_LogsCommand_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_com_avos_avoscloud_LogsCommand_descriptor,
new java.lang.String[] { "Cid", "L", "Limit", "T", "Tt", "Tmid", "Mid", "Checksum", "Stored", "Direction", "TIncluded", "TtIncluded", "Lctype", "Logs", });
internal_static_com_avos_avoscloud_RcpCommand_descriptor =
getDescriptor().getMessageTypes().get(13);
internal_static_com_avos_avoscloud_RcpCommand_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_com_avos_avoscloud_RcpCommand_descriptor,
new java.lang.String[] { "Id", "Cid", "T", "Read", "From", });
internal_static_com_avos_avoscloud_ReadTuple_descriptor =
getDescriptor().getMessageTypes().get(14);
internal_static_com_avos_avoscloud_ReadTuple_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_com_avos_avoscloud_ReadTuple_descriptor,
new java.lang.String[] { "Cid", "Timestamp", "Mid", });
internal_static_com_avos_avoscloud_MaxReadTuple_descriptor =
getDescriptor().getMessageTypes().get(15);
internal_static_com_avos_avoscloud_MaxReadTuple_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_com_avos_avoscloud_MaxReadTuple_descriptor,
new java.lang.String[] { "Pid", "MaxAckTimestamp", "MaxReadTimestamp", });
internal_static_com_avos_avoscloud_ReadCommand_descriptor =
getDescriptor().getMessageTypes().get(16);
internal_static_com_avos_avoscloud_ReadCommand_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_com_avos_avoscloud_ReadCommand_descriptor,
new java.lang.String[] { "Cid", "Cids", "Convs", });
internal_static_com_avos_avoscloud_PresenceCommand_descriptor =
getDescriptor().getMessageTypes().get(17);
internal_static_com_avos_avoscloud_PresenceCommand_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_com_avos_avoscloud_PresenceCommand_descriptor,
new java.lang.String[] { "Status", "SessionPeerIds", "Cid", });
internal_static_com_avos_avoscloud_ReportCommand_descriptor =
getDescriptor().getMessageTypes().get(18);
internal_static_com_avos_avoscloud_ReportCommand_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_com_avos_avoscloud_ReportCommand_descriptor,
new java.lang.String[] { "Initiative", "Type", "Data", });
internal_static_com_avos_avoscloud_PatchItem_descriptor =
getDescriptor().getMessageTypes().get(19);
internal_static_com_avos_avoscloud_PatchItem_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_com_avos_avoscloud_PatchItem_descriptor,
new java.lang.String[] { "Cid", "Mid", "Timestamp", "Recall", "Data", "PatchTimestamp", "From", "BinaryMsg", "MentionAll", "MentionPids", "PatchCode", "PatchReason", });
internal_static_com_avos_avoscloud_PatchCommand_descriptor =
getDescriptor().getMessageTypes().get(20);
internal_static_com_avos_avoscloud_PatchCommand_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_com_avos_avoscloud_PatchCommand_descriptor,
new java.lang.String[] { "Patches", "LastPatchTime", });
internal_static_com_avos_avoscloud_PubsubCommand_descriptor =
getDescriptor().getMessageTypes().get(21);
internal_static_com_avos_avoscloud_PubsubCommand_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_com_avos_avoscloud_PubsubCommand_descriptor,
new java.lang.String[] { "Cid", "Cids", "Topic", "Subtopic", "Topics", "Subtopics", "Results", });
internal_static_com_avos_avoscloud_BlacklistCommand_descriptor =
getDescriptor().getMessageTypes().get(22);
internal_static_com_avos_avoscloud_BlacklistCommand_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_com_avos_avoscloud_BlacklistCommand_descriptor,
new java.lang.String[] { "SrcCid", "ToPids", "SrcPid", "ToCids", "Limit", "Next", "BlockedPids", "BlockedCids", "AllowedPids", "FailedPids", "T", "N", "S", });
internal_static_com_avos_avoscloud_GenericCommand_descriptor =
getDescriptor().getMessageTypes().get(23);
internal_static_com_avos_avoscloud_GenericCommand_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_com_avos_avoscloud_GenericCommand_descriptor,
new java.lang.String[] { "Cmd", "Op", "AppId", "PeerId", "I", "InstallationId", "Priority", "Service", "ServerTs", "DataMessage", "SessionMessage", "ErrorMessage", "DirectMessage", "AckMessage", "UnreadMessage", "ReadMessage", "RcpMessage", "LogsMessage", "ConvMessage", "RoomMessage", "PresenceMessage", "ReportMessage", "PatchMessage", "PubsubMessage", "BlacklistMessage", });
}
// @@protoc_insertion_point(outer_class_scope)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy