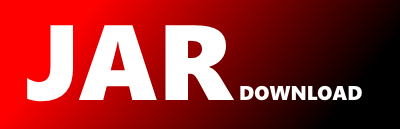
com.avos.avoscloud.ops.RemoveOp Maven / Gradle / Ivy
package com.avos.avoscloud.ops;
import java.util.Collection;
import java.util.HashSet;
import java.util.LinkedList;
import java.util.List;
import java.util.Map;
import java.util.Set;
import com.avos.avoscloud.AVUtils;
public class RemoveOp extends CollectionOp {
private Set
© 2015 - 2025 Weber Informatics LLC | Privacy Policy