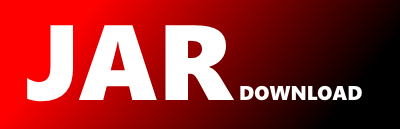
cn.leancloud.ops.CompoundOperation Maven / Gradle / Ivy
package cn.leancloud.ops;
import cn.leancloud.LCObject;
import java.util.*;
public class CompoundOperation extends BaseOperation {
private List operations = new LinkedList();
public CompoundOperation(String field) {
super("Compound", field, null, false);
}
public CompoundOperation(String field, ObjectFieldOperation... ops) {
this(field);
operations.addAll(Arrays.asList(ops));
}
public List getSubOperations() {
return this.operations;
}
@Override
public boolean checkCircleReference(Map markMap) {
boolean result = false;
for (ObjectFieldOperation op : operations) {
result = result || op.checkCircleReference(markMap);
}
return result;
}
public Object apply(Object obj) {
for (ObjectFieldOperation op: operations) {
obj = op.apply(obj);
}
return obj;
}
protected ObjectFieldOperation mergeWithPrevious(ObjectFieldOperation previous) {
operations.add(previous);
return this;
}
public List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy