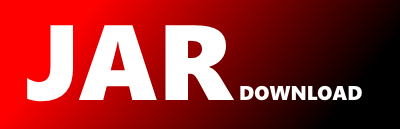
cn.lzgabel.model.grid.Grid Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of bpmn-auto-layout Show documentation
Show all versions of bpmn-auto-layout Show documentation
Tools for auto layout bpmn files
package cn.lzgabel.model.grid;
import java.awt.Dimension;
import java.awt.Point;
import java.util.ArrayList;
import java.util.HashMap;
import java.util.Iterator;
import java.util.List;
import org.activiti.bpmn.model.FlowNode;
public class Grid {
private List>> columns = new ArrayList>>();
private HashMap> cellmap = new HashMap<>();
private GridPosition gridPosition = new GridPosition(0,0);
private Point absolutePosition = new Point();
private Dimension absoluteSize = new Dimension();
private int cellHeight, cellWidth;
private Cell destCell;
private boolean isEmpty = true;
public Grid()
{
columns.add(new ArrayList>());
columns.get(0).add(new Cell(0,0));
}
public GridSize getGridSize()
{
return new GridSize(columns.get(0).size(), columns.size());
}
public void addRowsBelowAndAbovePosition(GridPosition position, int rowCount) {
for (int i = 1; i < rowCount; i++) {
if (i % 2 == 0)
this.addRowAbove(position.row);
if (i % 2 == 1) {
this.addRowBelow(position.row);
}
}
}
public void createAndReserveCells(GridPosition position, Grid spGrid) {
GridPosition subProcessPosition = new GridPosition(position);
for (List> col : spGrid.getColumns()) {
for (Iterator> iterator = col.iterator(); iterator.hasNext(); iterator.next()) {
if(subProcessPosition.row > this.getGridSize().rows()-1)
this.addLastRow();
addValue(null, subProcessPosition);
getCell(subProcessPosition).flag = CellFlag.RESERVED;
subProcessPosition.row++;
}
subProcessPosition.row = position.row;
subProcessPosition.column++;
}
}
public void shiftFlowNode(T node, int distance) throws ArrayIndexOutOfBoundsException{
Cell sourceCell = this.getCellByValue(node);
int col = getColumnOf(sourceCell);
int row = getColumns().get(col).indexOf(sourceCell);
destCell = null;
while (destCell == null) {
List> column = null;
// add columns if the destination column doesn't exist
if (getColumns().size() <= col + distance) {
addColumn();
continue;
}
column = getColumns().get(col + distance);
// add rows if the destination row doesn't exist
if (column.size() <= row) {
column.add(new Cell(col, column.size() - 1));
continue;
}
destCell = column.get(row);
}
moveCellContent(sourceCell, destCell);
}
public void appendGrid(Grid g)
{
isEmpty = false;
int colIndex = 0;
int originalRowSize = getGridSize().rows();
for (List> column : g.getColumns()) {
if (this.columns.size() == colIndex) {
this.addColumn(originalRowSize);
}
for (Cell cell : column) {
this.getColumns().get(colIndex).add(cell);
}
colIndex++;
}
//fill up the remaining columns with cells to match row numbers
while(colIndex != columns.size())
{
while(this.getColumns().get(0).size() != this.getColumns().get(colIndex).size())
this.getColumns().get(colIndex).add(new Cell(0, 0));
colIndex++;
}
cellmap.putAll(g.cellmap);
}
public T getValueFromCell(int row, int col)
{
return (T) columns.get(col).get(row).getValue();
}
public Cell addValueToCell(T value, int row, int col)
{
int freeColumn = col;
while(freeColumn >= columns.size())
addColumn(getGridSize().rows());
Cell cell = columns.get(freeColumn).get(row);
while(cell.getValue() != null)
{
addColumn(getGridSize().rows());
freeColumn++;
cell = columns.get(freeColumn).get(row);
}
if(value != null)
{
cell.setValue(value);
cellmap.put(value, cell);
}
return cell;
}
public Cell getCellByValue(T value)
{
return cellmap.get(value);
}
public void addColumn(int rows) {
ArrayList> col = new ArrayList>();
fillColumnWithRows(col, rows);
columns.add(col);
}
public void addColumn() {
ArrayList> col = new ArrayList>();
fillColumnWithRows(col, getGridSize().rows());
columns.add(col);
}
private void fillColumnWithRows(ArrayList> col, int rows) {
for(int i = 0; i < rows; i++)
col.add(new Cell(i, columns.size()));;
}
public void addRowAbove(int rowIndex) {
for(List> row : columns)
{
row.add(rowIndex, new Cell(rowIndex, row.get(0).gridPosition.column));
for(int i = rowIndex+1; i < row.size(); i++)
{
row.get(i).gridPosition.row++;
}
}
}
public void addRowBelow(int rowIndex) {
for(List> row : columns)
{
row.add(rowIndex+1, new Cell(rowIndex+1, row.get(0).gridPosition.column));
for(int i = rowIndex+2; i < row.size(); i++)
{
row.get(i).gridPosition.row++;
}
}
}
public List>> getColumns() {
return columns;
}
public int getColumnOf(Cell cell) {
for(List> column : columns)
{
if(column.contains(cell))
return columns.indexOf(column);
}
return -1;
}
public void updateCellMap(Cell newCell) {
cellmap.remove(newCell.getValue());
cellmap.put(newCell.getValue(), newCell);
}
public void moveCellContent(Cell sourceCell, Cell destCell) {
destCell.setValue(sourceCell.getValue());
CellFlag sourceFlag = sourceCell.flag;
destCell.flag = sourceFlag;
sourceCell.flag = CellFlag.FREE;
sourceCell.setValue(null);
updateCellMap(destCell);
}
public Cell getCell(GridPosition position) {
try {
return columns.get(position.column).get(position.row);
}catch(IndexOutOfBoundsException e) {
return null;
}
}
public void addValue(T value, GridPosition position) {
addValueToCell(value, position.row, position.column);
}
public void addLastRow() {
addRowBelow(columns.get(0).size() - 1);
}
public void setCellsize(int cellHeight, int cellWidth)
{
this.cellHeight = cellHeight;
this.cellWidth = cellWidth;
}
public GridPosition getGridPosition() {
return gridPosition;
}
public void setGridPosition(GridPosition gridPosition) {
this.gridPosition = gridPosition;
}
public Point getAbsolutePosition() {
absolutePosition.x = gridPosition.column * cellWidth;
absolutePosition.y = gridPosition.row * cellHeight;
return absolutePosition;
}
public Dimension getAbsoluteSize() {
absoluteSize.setSize(columns.size() * cellWidth, columns.get(0).size() * cellHeight);
return absoluteSize;
}
public void moveCellContent(GridPosition sourcePosition, GridPosition destPosition) {
Cell source = getCell(sourcePosition);
Cell target = getCell(destPosition);
moveCellContent(source, target);
}
public boolean isEmpty() {
return cellmap.isEmpty() && isEmpty;
}
public HashMap> getCellMap() {
return cellmap;
}
public Cell getCell(int row, int column) {
return getCell(new GridPosition(row, column));
}
}
| | | | | | | | | | | | |
© 2015 - 2025 Weber Informatics LLC | Privacy Policy