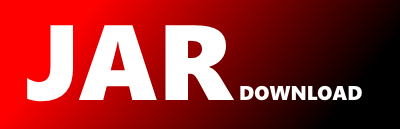
cn.maarlakes.common.tuple.Tuple2 Maven / Gradle / Ivy
package cn.maarlakes.common.tuple;
import jakarta.annotation.Nonnull;
import java.util.Objects;
import java.util.Optional;
import java.util.function.Function;
/**
* @author linjpxc
*/
public class Tuple2 implements Tuple {
private static final long serialVersionUID = 6000042060179342005L;
protected final T1 item1;
protected final T2 item2;
public Tuple2(T1 item1, T2 item2) {
this.item1 = item1;
this.item2 = item2;
}
@Override
public final int size() {
return 2;
}
public final T1 item1() {
return this.item1;
}
public final T2 item2() {
return this.item2;
}
public final Optional optionalItem1() {
return Optional.ofNullable(this.item1);
}
public final Optional optionalItem2() {
return Optional.ofNullable(this.item2);
}
public Tuple2 with(T1 item1, T2 item2) {
return new Tuple2<>(item1, item2);
}
public Tuple2 withItem1(T1 item1) {
return new Tuple2<>(item1, this.item2);
}
public Tuple2 withItem2(T2 item2) {
return new Tuple2<>(this.item1, item2);
}
public Tuple2 map(Function map1, Function map2) {
return new Tuple2<>(map1.apply(this.item1), map2.apply(this.item2));
}
public Tuple2 mapItem1(Function map1) {
return new Tuple2<>(map1.apply(this.item1), this.item2);
}
public Tuple2 mapItem2(Function map2) {
return new Tuple2<>(this.item1, map2.apply(this.item2));
}
@Override
@SuppressWarnings("unchecked")
public T get(int index) {
switch (index) {
case 0:
return (T) this.item1;
case 1:
return (T) this.item2;
default:
throw new IndexOutOfBoundsException();
}
}
@Nonnull
@Override
@SuppressWarnings("unchecked")
public Tuple2 clone() {
try {
return (Tuple2) super.clone();
} catch (CloneNotSupportedException e) {
throw new IllegalStateException(e);
}
}
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
final Tuple2, ?> tuple2 = (Tuple2, ?>) o;
return Objects.equals(item1, tuple2.item1) && Objects.equals(item2, tuple2.item2);
}
@Override
public int hashCode() {
return Objects.hash(item1, item2);
}
@Override
public String toString() {
return "{" + this.item1 + ", " + this.item2 + "}";
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy