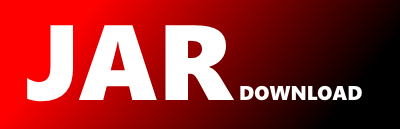
cn.majingjing.http.client.request.BaseRequest Maven / Gradle / Ivy
package cn.majingjing.http.client.request;
import cn.majingjing.http.client.HttpClient;
import cn.majingjing.http.client.interceptor.HttpInterceptor;
import cn.majingjing.http.client.response.HttpResponse;
import cn.majingjing.http.client.response.callback.HttpCallBack;
import cn.majingjing.http.client.util.HttpConstant;
import cn.majingjing.http.client.util.HttpFactory;
import cn.majingjing.http.client.util.StringUtils;
import java.util.*;
/**
* 基础请求对象
*
* @author MaMarion
* @date 2020/4/24
*/
public abstract class BaseRequest> {
protected List interceptors = new ArrayList<>(1);
/**
* 请求方式
*/
protected String method;
/**
* 请求地址
*/
protected String url;
/**
* 存储请求头信息
*/
protected Map headers;
/**
* url上的请求参数
*/
protected String param;
/**
* 请求体内容
*/
protected byte[] body;
/**
* 连接超时时间 (默认10s)
*/
protected int connectTimeout = 10000;
/**
* 流读取超时时间 (默认10s)
*/
protected int readTimeout = 10000;
/**
* 流写入超时时间
*/
protected int writeTimeout;
/**
* 失败最大重试次数 (默认不重试)
*/
protected int maxRetry = 0;
protected String traceId;
/**
* 基础请求对象构造方法
*
* @param url 请求地址
* @param method 请求方式
*/
public BaseRequest(String url, String method) {
this.url = url;
this.method = method;
this.headers = new LinkedHashMap<>(4);
this.header("accept", "*/*");
this.header("Connection", "Keep-Alive");
this.header("Charset", "UTF-8");
// this.header("User-Agent", "Mozilla/5.0 (Macintosh; Intel Mac OS X 10_15_1) AppleWebKit/605.1.15 (KHTML, like Gecko) Version/13.0.3 Safari/605.1.15");
this.header("User-Agent", HttpConstant.USER_AGENT_VALUE);
}
/**
* 添加请求头
*
* @param key 键
* @param value 值
* @return 返回当前类 {@linkplain Req}的对象自己
*/
public Req header(String key, String value) {
if (StringUtils.isNotEmpty(key) && null != value) {
this.headers.put(key, value);
}
return (Req) this;
}
public Req addInterceptor(HttpInterceptor httpInterceptor){
this.interceptors.add(httpInterceptor);
return (Req) this;
}
/**
* 删除请求头
*
* @param key 键
* @return 返回当前类 {@linkplain Req}的对象自己
*/
public Req removeHeader(String key) {
if (StringUtils.isNotEmpty(key)) {
this.headers.remove(key);
}
return (Req) this;
}
/**
* 添加url请求参数
*
* @param name 键
* @param value 值
* @return 返回当前类 {@linkplain Req}的对象自己
*/
public Req param(String name, String value) {
if (StringUtils.isEmpty(this.param)) {
this.param = String.format("%s=%s", name, value);
} else {
this.param = String.format("%s&%s=%s", this.param, name, value);
}
return (Req) this;
}
/**
* 设置路由参数
* /web/blogs/{id}.html => id:123 => /web/blogs/123.html
* @param name 键
* @param value 值
* @return 返回当前类 {@linkplain Req}的对象自己
*/
public Req routeParam(String name, String value) {
this.url = this.url.replace("{" + name + "}", value);
return (Req) this;
}
/**
* 设置网络链接时间
*
* @param connectTimeout 链接时间(单位秒)
* @return 返回当前类 {@linkplain Req}的对象自己
*/
public Req connectTimeout(int connectTimeout) {
this.connectTimeout = connectTimeout;
return (Req) this;
}
/**
* 设置请求读取时间
*
* @param readTimeout 读取时间(单位秒)
* @return 返回当前类 {@linkplain Req}的对象自己
*/
public Req readTimeout(int readTimeout) {
this.readTimeout = readTimeout;
return (Req) this;
}
/**
* 设置最大重试次数(不包含第一次调用失败次数,即总执行次数为 maxRetry+1)
*
* @param maxRetry 最大重试次数
* @return 返回当前类 {@linkplain Req}的对象自己
*/
public Req maxRetry(int maxRetry) {
this.maxRetry = maxRetry;
return (Req) this;
}
public Req traceId(String traceId) {
this.traceId = traceId;
return (Req) this;
}
/**
* 同步-执行HTTP请求
* @return HttpResponse 响应对象
*/
public HttpResponse execute() {
if (StringUtils.isNotEmpty(param)) {
this.url = String.format("%s%s%s", this.url, this.url.contains("?") ? "&" : "?", param);
}
HttpRequest httpRequest = new HttpRequest(this);
if(Objects.isNull(httpRequest.getInterceptors()) || httpRequest.getInterceptors().isEmpty()){
HttpResponse response = HttpFactory.httpChain().proceed(httpRequest);
return response;
}
List globalInterceptorList = HttpClient.Instance.getConfig().getInterceptors();
List interceptors = new ArrayList<>(globalInterceptorList.size()+httpRequest.getInterceptors().size());
interceptors.addAll(globalInterceptorList);
interceptors.addAll(httpRequest.getInterceptors());
HttpResponse response = HttpFactory.httpChain(interceptors).proceed(httpRequest);
return response;
}
/**
* 异步-执行HTTP请求
*
* @param callBack 回调函数
*/
public void executeAsync(HttpCallBack callBack) {
HttpFactory.getAsyncExecuteThreadPool().execute(() -> callBack.onComplete(new HttpRequest(this), execute()));
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy