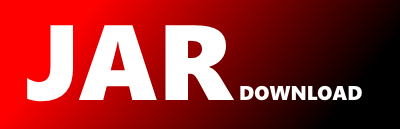
cn.majingjing.http.client.request.PostRequest Maven / Gradle / Ivy
The newest version!
package cn.majingjing.http.client.request;
import cn.majingjing.http.client.exception.HttpClientException;
import cn.majingjing.http.client.util.HttpConstant;
import cn.majingjing.http.client.util.ObjectUtils;
import cn.majingjing.http.client.util.StringUtils;
/**
* Post方式的请求对象
*
* @author MaMarion
* @date 2020/4/24
*/
public class PostRequest extends BaseRequest {
public PostRequest(String url) {
super(url, "POST");
}
/**
* body为纯文本
*
* @param body 请求体
* @return PostRequest
*/
public PostRequest text(String body) {
if (ObjectUtils.isNotNull(this.body)) {
throw new HttpClientException("PostRequest body can not be set multiple times");
}
this.body = StringUtils.toBytes(body);
super.header(HttpConstant.CONTENT_TYPE, "text/plain");
return this;
}
/**
* body为json格式
* @param body 请求体
* @return PostRequest
*/
public PostRequest json(String body) {
if (ObjectUtils.isNotNull(this.body)) {
throw new HttpClientException("PostRequest body can not be set multiple times");
}
this.body = StringUtils.toBytes(body);
super.header(HttpConstant.CONTENT_TYPE, "application/json");
return this;
}
/**
* body为xml格式
* @param body 请求体
* @return PostRequest
*/
public PostRequest xml(String body) {
if (ObjectUtils.isNotNull(this.body)) {
throw new HttpClientException("PostRequest body can not be set multiple times");
}
this.body = StringUtils.toBytes(body);
super.header(HttpConstant.CONTENT_TYPE, "application/xml");
return this;
}
/**
* body为xml格式
* @param body 请求体
* @return PostRequest
*/
public PostRequest stream(byte[] body) {
if (ObjectUtils.isNotNull(this.body)) {
throw new HttpClientException("PostRequest body can not be set multiple times");
}
this.body = body;
return this;
}
// /**
// * body为form表单
// *
// * @param name 表单域内的属性名称
// * @param value 表单域内的属性值
// * @return PostRequest
// */
// public PostRequest form(String name, String value) {
// if (StringUtils.isNotEmpty(this.body) && !"application/x-www-form-urlencoded".equals(super.headers.get("Content-Type"))) {
// throw new HttpClientException("PostRequest body can not be set multiple times");
// }
// if (StringUtils.isEmpty(this.body)) {
// this.body = String.format("%s=%s", name, value);
// } else {
// this.body = String.format("%s&%s=%s", this.body, name, value);
// }
// super.header("Content-Type", "application/x-www-form-urlencoded");
// return this;
// }
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy