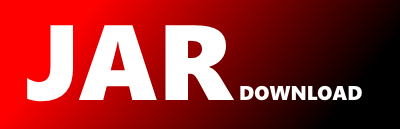
cn.majingjing.http.client.response.HttpResponse Maven / Gradle / Ivy
The newest version!
package cn.majingjing.http.client.response;
import cn.majingjing.http.client.exception.HttpClientException;
import cn.majingjing.http.client.response.handle.DataHandler;
import cn.majingjing.http.client.response.handle.FileDataHandler;
import cn.majingjing.http.client.response.handle.StringDataHandler;
import java.io.File;
import java.net.HttpURLConnection;
import java.nio.charset.StandardCharsets;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
/**
* http响应对象
*
* @author MaMarion
* @date 2020/4/24
*/
public class HttpResponse {
private int statusCode;
private byte[] body;
private HttpClientException exception;
private Map> headers;
public HttpResponse(int statusCode, byte[] body, HttpClientException exception, Map> headers) {
this.statusCode = statusCode;
this.body = body;
this.exception = exception;
// this.headers = headers;
this.headers = new HashMap<>(headers);
}
/**
* 响应状态码
*
* @return int
*/
public int getStatusCode() {
return statusCode;
}
/**
* 请求是否成功
*
* @return boolean 状态码小于400即为成功
*/
public boolean isSucceed() {
return statusCode < HttpURLConnection.HTTP_BAD_REQUEST;
}
/**
* 响应header信息
*
* @return Map>
*/
public Map> getHeaders() {
return headers;
}
/**
* 响应异常信息
*
* @return HttpClientException 如果响应正常,则异常信息为null
*/
public HttpClientException getException() {
if (isSucceed()) {
return null;
}
return exception;
}
/**
* 自定义响应数据处理
*
* @param dataHandler 数据处理器
* @param 数据处理结果对象类型
* @return 数据处理完成后返回的数据类型
*/
public T custom(DataHandler dataHandler) {
checkError();
return dataHandler.handle(body);
}
/**
* 响应数据转换成字节数组
*
* @return byte[]
*/
public byte[] asByteData() {
checkError();
return body;
}
/**
* 响应数据转换成字符串
*
* @return String
*/
public String asString() {
checkError();
return this.custom(new StringDataHandler());
}
/**
* 响应数据转换成文件
*
* @param file 目标保存文件,非空
* @return File
*/
public File asFile(File file) {
if (file == null) {
throw new NullPointerException("file is null");
} else {
this.checkError();
return this.custom(new FileDataHandler(file));
}
}
/**
* 检查请求是否有错误,如果有错误将抛出HttpException
*/
private void checkError() {
if (!isSucceed()) {
throw new HttpClientException("Request failed, status code: " + statusCode + ", response content:" + new String(body, StandardCharsets.UTF_8));
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy