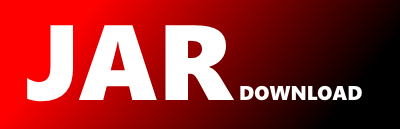
cn.majingjing.http.client.util.HttpFactory Maven / Gradle / Ivy
package cn.majingjing.http.client.util;
import cn.majingjing.http.client.HttpClient;
import cn.majingjing.http.client.config.HttpClientConfig;
import cn.majingjing.http.client.handler.HttpHandler;
import cn.majingjing.http.client.handler.JdkHttpHandler;
import cn.majingjing.http.client.handler.chain.HttpChain;
import cn.majingjing.http.client.handler.chain.RealHttpChain;
import cn.majingjing.http.client.interceptor.HttpInterceptor;
import java.util.List;
import java.util.concurrent.*;
/**
* Http 工厂
*
* @author MaMarion
* @date 2020/5/1
*/
public final class HttpFactory {
/**
* http请求的真实处理器
*/
private static HttpHandler httpHandler = new JdkHttpHandler();
/**
* 异步执行的线程池
*/
private static volatile ExecutorService threadPool = null;
/**
* 异步执行的线程池获取时的锁对象
*/
private static final Object LOCK = new Object();
/**
* 获取http调用链
*
* @return HttpChain
*/
public static HttpChain httpChain() {
return new RealHttpChain(httpHandler, HttpClient.Instance.getConfig().getInterceptors());
}
public static HttpChain httpChain(List httpInterceptorList) {
return new RealHttpChain(httpHandler, httpInterceptorList);
}
/**
* 获取异步执行的线程池
*
* @return ExecutorService
*/
public static ExecutorService getAsyncExecuteThreadPool() {
if (threadPool == null) {
synchronized (LOCK) {
if (threadPool == null) {
HttpLogs.getLogger().fine("---Enable ExecutorService---");
HttpClientConfig config = HttpClient.Instance.getConfig();
ThreadFactory namedThreadFactory = (Runnable r) -> new Thread(r, "HttpClient_Async_Thread_Pool_" + r.hashCode());
threadPool = new ThreadPoolExecutor(config.getAsyncExecuteThreadPoolCorePoolSize(),
config.getAsyncExecuteThreadPoolMaxPoolSize(),
0L,
TimeUnit.MILLISECONDS,
new LinkedBlockingQueue(),
namedThreadFactory,
new ThreadPoolExecutor.AbortPolicy());
}
}
}
return threadPool;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy