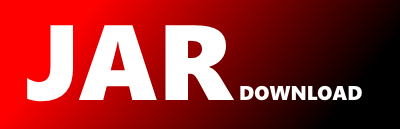
cn.majingjing.http.client.util.ObjectUtils Maven / Gradle / Ivy
The newest version!
package cn.majingjing.http.client.util;
import javax.activation.UnsupportedDataTypeException;
import java.io.*;
import java.util.Objects;
/**
* @author MaMarion
* @date 2021/2/23
*/
public class ObjectUtils {
private ObjectUtils() {
}
public static T getValueOrDefault(Object val, Object defaultVal) {
return (T) (Objects.isNull(val) ? defaultVal : val);
}
public static boolean isNull(Object obj){
return obj == null;
}
public static boolean isNotNull(Object obj){
return obj != null;
}
/**
* Clone object
* @param source object to be cloned
* @param the object type
* @return the target object of cloned
* @throws IOException maybe throw IOException
* @throws ClassNotFoundException maybe throw ClassNotFoundException
*/
@SuppressWarnings("unckecked")
public static T clone(T source) throws IOException, ClassNotFoundException {
T result;
ByteArrayOutputStream bos = new ByteArrayOutputStream();
ObjectOutputStream oos = new ObjectOutputStream(bos);
oos.writeObject(source);
oos.flush();
try (ObjectInputStream ois = new ObjectInputStream(new ByteArrayInputStream(bos.toByteArray()))) {
result = (T) ois.readObject();
}
return result;
}
/**
* Serialize objects into byte []
* @param obj object to be serialized
* @return byte array
* @throws IOException maybe throw IOException
*/
public static byte[] serialize(Object obj) throws IOException {
ByteArrayOutputStream bos = new ByteArrayOutputStream();
try (ObjectOutputStream oos = new ObjectOutputStream(bos)) {
oos.writeObject(obj);
oos.flush();
}
return bos.toByteArray();
}
/**
* Deserialize byte [] into object
*
* @param bytes byte array
* @param de-serialized object type
* @return object with declare type T
* @throws UnsupportedDataTypeException if deserialize error will be throw UnsupportedDataTypeException
*/
public static T deserialize(byte[] bytes) throws UnsupportedDataTypeException {
try {
try (ObjectInputStream ois = new ObjectInputStream(new ByteArrayInputStream(bytes))) {
return (T) ois.readObject();
}
} catch (IOException | ClassNotFoundException e) {
throw new UnsupportedDataTypeException(e.getMessage());
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy