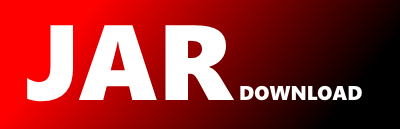
cn.majingjing.http.client.util.SslUtils Maven / Gradle / Ivy
The newest version!
package cn.majingjing.http.client.util;
import cn.majingjing.http.client.exception.HttpClientException;
import javax.net.ssl.*;
import java.security.cert.X509Certificate;
/**
* ssl utils
*
* @author MaMarion
* @date 2020/5/2
*/
public class SslUtils {
private SslUtils(){}
private static SkipHostnameVerifier skipHostnameVerifier = new SkipHostnameVerifier();
private static SkipX509TrustManager skipX509TrustManager = new SkipX509TrustManager();
/**
* 跳过Hostname验证
*/
private static class SkipHostnameVerifier implements HostnameVerifier {
@Override
public boolean verify(String s, SSLSession sslSession) {
return true;
}
}
/**
* 跳过X509信任
*/
private static class SkipX509TrustManager implements X509TrustManager {
@Override
public X509Certificate[] getAcceptedIssuers() {
return new X509Certificate[0];
}
@Override
public void checkClientTrusted(X509Certificate[] chain, String authType) {
}
@Override
public void checkServerTrusted(X509Certificate[] chain, String authType) {
}
}
/**
* 忽略整个应用的HTTPS请求的SSL证书,必须在openConnection之前调用
*/
public static void ignoreAllSsl() {
try {
// TrustAnyTrustManager
TrustManager[] trustAllCerts = new TrustManager[]{skipX509TrustManager};
SSLContext sc = SSLContext.getInstance(HttpConstant.SSL);
sc.init(null, trustAllCerts, null);
HttpsURLConnection.setDefaultSSLSocketFactory(sc.getSocketFactory());
// TrustAnyTrustManager
HttpsURLConnection.setDefaultHostnameVerifier(skipHostnameVerifier);
} catch (Exception e) {
throw new HttpClientException(e);
}
}
/**
* 忽略当前请求链接的HTTPS请求的SSL证书
* @param connection 链接对象
*/
public static void ignoreSsl(HttpsURLConnection connection) {
try {
// TrustAnyTrustManager
TrustManager[] trustAllCerts = new TrustManager[]{skipX509TrustManager};
SSLContext sc = SSLContext.getInstance(HttpConstant.SSL);
sc.init(null, trustAllCerts, null);
connection.setSSLSocketFactory(sc.getSocketFactory());
connection.setHostnameVerifier(skipHostnameVerifier);
} catch (Exception e) {
throw new HttpClientException(e);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy