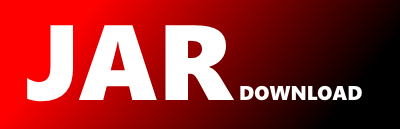
cn.msuno.swagger.spring.boot.autoconfigure.mappers.ServiceModelToSwagger2Mapper Maven / Gradle / Ivy
/*
*
* Copyright 2015 the original author or authors.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*
*
*/
package cn.msuno.swagger.spring.boot.autoconfigure.mappers;
import static cn.msuno.swagger.spring.boot.autoconfigure.mappers.ModelMapper.modelRefToProperty;
import static com.google.common.collect.FluentIterable.from;
import static com.google.common.collect.Lists.newArrayList;
import static com.google.common.collect.Maps.EntryTransformer;
import static com.google.common.collect.Maps.newHashMap;
import static com.google.common.collect.Maps.newTreeMap;
import static com.google.common.collect.Maps.transformEntries;
import static springfox.documentation.builders.BuilderDefaults.nullToEmptyList;
import java.util.List;
import java.util.Map;
import java.util.Set;
import org.mapstruct.Mapper;
import org.mapstruct.Mapping;
import org.mapstruct.Mappings;
import com.google.common.base.Function;
import com.google.common.base.Optional;
import com.google.common.collect.Maps;
import com.google.common.collect.Multimap;
import io.swagger.models.Contact;
import io.swagger.models.Info;
import io.swagger.models.Operation;
import io.swagger.models.Path;
import io.swagger.models.Response;
import io.swagger.models.Scheme;
import io.swagger.models.Swagger;
import io.swagger.models.Tag;
import io.swagger.models.properties.Property;
import springfox.documentation.schema.ModelReference;
import springfox.documentation.service.ApiDescription;
import springfox.documentation.service.ApiInfo;
import springfox.documentation.service.ApiListing;
import springfox.documentation.service.AuthorizationScope;
import springfox.documentation.service.Documentation;
import springfox.documentation.service.Header;
import springfox.documentation.service.ResponseMessage;
@Mapper(uses = {
ModelMapper.class,
ParameterMapper.class,
SecurityMapper.class,
LicenseMapper.class,
VendorExtensionsMapper.class
})
public abstract class ServiceModelToSwagger2Mapper {
@Mappings({
@Mapping(target = "info", source = "resourceListing.info"),
@Mapping(target = "paths", source = "apiListings"),
@Mapping(target = "host", source = "host"),
@Mapping(target = "schemes", source = "schemes"),
@Mapping(target = "definitions", source = "apiListings"),
@Mapping(target = "securityDefinitions", source = "resourceListing"),
@Mapping(target = "securityRequirement", ignore = true),
@Mapping(target = "security", ignore = true),
@Mapping(target = "swagger", ignore = true),
@Mapping(target = "parameters", ignore = true),
@Mapping(target = "responses", ignore = true),
@Mapping(target = "externalDocs", ignore = true),
@Mapping(target = "vendorExtensions", source = "vendorExtensions")
})
public abstract Swagger mapDocumentation(Documentation from);
@Mappings({
@Mapping(target = "license", source = "from",
qualifiedBy = { LicenseMapper.LicenseTranslator.class, LicenseMapper.License.class }),
@Mapping(target = "contact", source = "from.contact"),
@Mapping(target = "termsOfService", source = "termsOfServiceUrl"),
@Mapping(target = "vendorExtensions", source = "vendorExtensions")
})
protected abstract Info mapApiInfo(ApiInfo from);
protected abstract Contact map(springfox.documentation.service.Contact from);
@Mappings({
@Mapping(target = "description", source = "notes"),
@Mapping(target = "operationId", source = "uniqueId"),
@Mapping(target = "schemes", source = "protocol"),
@Mapping(target = "security", source = "securityReferences"),
@Mapping(target = "responses", source = "responseMessages"),
@Mapping(target = "vendorExtensions", source = "vendorExtensions"),
@Mapping(target = "externalDocs", ignore = true)
})
protected abstract Operation mapOperation(springfox.documentation.service.Operation from);
@Mappings({
@Mapping(target = "externalDocs", ignore = true),
@Mapping(target = "vendorExtensions", source = "vendorExtensions")
})
protected abstract Tag mapTag(springfox.documentation.service.Tag from);
protected List mapSchemes(List from) {
return from(from).transform(toScheme()).toList();
}
protected List
© 2015 - 2024 Weber Informatics LLC | Privacy Policy