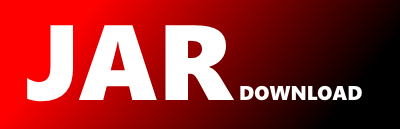
cn.msuno.swagger.spring.boot.autoconfigure.mappers.VendorExtensionsMapper Maven / Gradle / Ivy
/*
*
* Copyright 2015-2018 the original author or authors.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*
*
*/
package cn.msuno.swagger.spring.boot.autoconfigure.mappers;
import static com.google.common.base.Strings.isNullOrEmpty;
import static com.google.common.collect.FluentIterable.from;
import static com.google.common.collect.Maps.newHashMap;
import static com.google.common.collect.Maps.newTreeMap;
import java.util.List;
import java.util.Map;
import org.mapstruct.Mapper;
import com.google.common.base.Function;
import springfox.documentation.service.ListVendorExtension;
import springfox.documentation.service.ObjectVendorExtension;
import springfox.documentation.service.StringVendorExtension;
import springfox.documentation.service.VendorExtension;
@Mapper
public class VendorExtensionsMapper {
public Map mapExtensions(List from) {
Map extensions = newTreeMap();
Iterable listExtensions = from(from)
.filter(ListVendorExtension.class);
for (ListVendorExtension each : listExtensions) {
extensions.put(each.getName(), each.getValue());
}
Iterable
© 2015 - 2024 Weber Informatics LLC | Privacy Policy