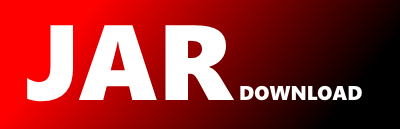
cn.mybatisboost.mapper.CrudMapper Maven / Gradle / Ivy
The newest version!
package cn.mybatisboost.mapper;
import cn.mybatisboost.core.GenericMapper;
import cn.mybatisboost.mapper.provider.Delete;
import cn.mybatisboost.mapper.provider.*;
import cn.mybatisboost.mapper.provider.Insert;
import cn.mybatisboost.mapper.provider.Update;
import org.apache.ibatis.annotations.*;
import org.apache.ibatis.session.RowBounds;
import java.util.List;
public interface CrudMapper extends GenericMapper {
@SelectProvider(type = SelectOrCount.class, method = "reserved")
int count(T entity, String... conditionProperties);
@SelectProvider(type = SelectOrCount.class, method = "reserved")
T selectOne(T entity, String... conditionProperties);
@SelectProvider(type = SelectOrCount.class, method = "reserved")
List select(T entity, String... conditionProperties);
@SelectProvider(type = SelectOrCount.class, method = "reserved")
List selectWithRowBounds(T entity, RowBounds rowBounds, String... conditionProperties);
@SelectProvider(type = SelectOrCountAll.class, method = "reserved")
int countAll();
@SelectProvider(type = SelectOrCountAll.class, method = "reserved")
List selectAll();
@SelectProvider(type = SelectOrCountAll.class, method = "reserved")
List selectAllWithRowBounds(RowBounds rowBounds);
@SelectProvider(type = SelectByIds.class, method = "reserved")
T selectById(@Param("arg0") Object id);
@SelectProvider(type = SelectByIds.class, method = "reserved")
List selectByIds(Object... ids);
@InsertProvider(type = Insert.class, method = "reserved")
int insert(T entity, String... properties);
@InsertProvider(type = Insert.class, method = "reserved")
int batchInsert(List entities, String... properties);
@InsertProvider(type = Insert.class, method = "reserved")
int insertSelective(T entity, String... properties);
@InsertProvider(type = Insert.class, method = "reserved")
int batchInsertSelective(List entities, String... properties);
@UpdateProvider(type = Update.class, method = "reserved")
int update(T entity, String... conditionProperties);
@UpdateProvider(type = Update.class, method = "reserved")
int updatePartial(T entity, String[] properties, String... conditionProperties);
@UpdateProvider(type = Update.class, method = "reserved")
int updateSelective(T entity, String... conditionProperties);
@UpdateProvider(type = Update.class, method = "reserved")
int updatePartialSelective(T entity, String[] properties, String... conditionProperties);
@DeleteProvider(type = Delete.class, method = "reserved")
int delete(T entity, String... conditionProperties);
@DeleteProvider(type = DeleteByIds.class, method = "reserved")
int deleteByIds(Object... ids);
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy