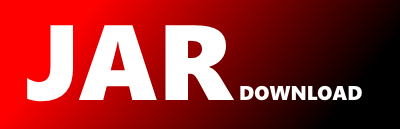
com.mugui.base.util.Other Maven / Gradle / Ivy
package com.mugui.base.util;
import java.io.BufferedInputStream;
import java.io.BufferedOutputStream;
import java.io.BufferedReader;
import java.io.ByteArrayInputStream;
import java.io.ByteArrayOutputStream;
import java.io.DataOutputStream;
import java.io.File;
import java.io.FileInputStream;
import java.io.FileOutputStream;
import java.io.IOException;
import java.io.InputStreamReader;
import java.io.ObjectInputStream;
import java.io.ObjectOutputStream;
import java.io.UnsupportedEncodingException;
import java.math.BigInteger;
import java.net.HttpURLConnection;
import java.net.URL;
import java.security.MessageDigest;
import java.security.NoSuchAlgorithmException;
import java.util.Arrays;
import java.util.Random;
import java.util.Scanner;
import java.util.UUID;
import java.util.regex.Matcher;
import java.util.regex.Pattern;
import java.util.zip.GZIPInputStream;
import java.util.zip.GZIPOutputStream;
import java.util.zip.ZipEntry;
import java.util.zip.ZipInputStream;
/**
* 一些不再更新,因使用习惯加入的杂项工具
*
* @author 木鬼
*
*/
public class Other {
public static void sleep(int i) {
try {
Thread.sleep(i);
} catch (Exception e) {
}
}
public static boolean ObjectToNewObjectBoolean(T obj, Class> toobj) {
return toobj.isAssignableFrom(obj.getClass());
}
public static byte[] intToByteArray(int i) {
byte[] result = new byte[4];
result[0] = (byte) (i & 0xFF);
result[1] = (byte) ((i >> 8) & 0xFF);
result[2] = (byte) ((i >> 16) & 0xFF);
result[3] = (byte) ((i >> 24) & 0xFF);
return result;
}
public static int byteArrayint(byte[] res) {
// 一个byte数据左移24位变成0x??000000,再右移8位变成0x00??0000
int targets = (res[0] & 0xff) | ((res[1] << 8) & 0xff00) // | 表示安位或
| ((res[2] << 24) >>> 8) | (res[3] << 24);
return targets;
}
public static boolean isDouble(String string) {
try {
Double.parseDouble(string);
return true;
} catch (Exception e) {
}
return false;
}
public static boolean isLong(String string) {
try {
Long.parseLong(string);
return true;
} catch (Exception e) {
}
return false;
}
public static final Object ByteArrayToNewObject(byte[] b) {
ByteArrayInputStream bArrayInputStream = null;
ObjectInputStream ois = null;
try {
bArrayInputStream = new ByteArrayInputStream(b, 0, b.length);
ois = new ObjectInputStream(bArrayInputStream);
return ois.readObject();
} catch (Exception e) {
e.printStackTrace();
} finally {
try {
if (ois != null)
ois.close();
if (bArrayInputStream != null)
bArrayInputStream.close();
} catch (Exception e2) {
}
}
return null;
}
public static final byte[] ObjectToNewByteArray(Object obj) {
ByteArrayOutputStream bArrayOutputStream = null;
ObjectOutputStream oos = null;
try {
bArrayOutputStream = new ByteArrayOutputStream();
oos = new ObjectOutputStream(bArrayOutputStream);
oos.writeObject(obj);
return bArrayOutputStream.toByteArray();
} catch (Exception e) {
e.printStackTrace();
} finally {
try {
if (oos != null)
oos.close();
if (bArrayOutputStream != null)
bArrayOutputStream.close();
} catch (Exception e2) {
}
}
return null;
}
public static String ObjectToNewString(Object object) {
try {
return new String(Other.ObjectToNewByteArray(object), "ISO-8859-1");
} catch (UnsupportedEncodingException e) {
e.printStackTrace();
return null;
}
}
public static Object StringToNewObjet(String s) {
try {
return Other.ByteArrayToNewObject(s.getBytes("ISO-8859-1"));
} catch (UnsupportedEncodingException e) {
e.printStackTrace();
return null;
}
}
@SuppressWarnings("unchecked")
public static T copyClassToNewClass(T obj) {
ByteArrayOutputStream baos = null;
ObjectOutputStream oos = null;
ObjectInputStream ois = null;
try {
baos = new ByteArrayOutputStream();
oos = new ObjectOutputStream(baos);
oos.writeObject(obj);
ois = new ObjectInputStream(new ByteArrayInputStream(baos.toByteArray()));
return (T) ois.readObject();
} catch (Exception e) {
e.printStackTrace();
} finally {
try {
if (ois != null)
ois.close();
if (oos != null)
oos.close();
if (baos != null)
baos.close();
} catch (IOException e) {
e.printStackTrace();
}
}
return null;
}
public static boolean isMailString(String mialString) {
Pattern p = Pattern.compile("^([a-zA-Z0-9 _ .-])+@([a-zA-Z0-9 _ .])+(\\.([a-zA-Z0-9 _ .])+)+$");
Matcher m = p.matcher(mialString);
return m.matches();
}
public static T[] ArraysToNewArray(T[] first, T[] second) {
T[] result = Arrays.copyOf(first, first.length + second.length);
System.arraycopy(second, 0, result, first.length, second.length);
return result;
}
public static byte[] ArraysToNewArray(byte[] first, byte[] second) {
byte[] result = Arrays.copyOf(first, first.length + second.length);
System.arraycopy(second, 0, result, first.length, second.length);
return result;
}
public static boolean isInteger(String string) {
try {
Integer.parseInt(string);
return true;
} catch (Exception e) {
}
return false;
}
// 生成一定范围随机数
private static int r(int min, int max) {
int num = 0;
num = new Random().nextInt(max - min) + min;
return num;
}
// 生成一个用户验证码
public static String getVerifyCode(int codeSize) {
String str = "aqzxswedcfrvgtbhyujklp23456789ABCDEFGHIJKLMNOPQRSTUVWXYZ";
char c[] = new char[codeSize];
for (int i = 0; i < codeSize; i++)
c[i] = str.charAt(r(0, str.length()));
return new String(c);
}
public final static String[] chars = new String[] { "a", "b", "c", "d", "e", "f", "g", "h", "i", "j", "k", "l", "m",
"n", "o", "p", "q", "r", "s", "t", "u", "v", "w", "x", "y", "z", "0", "1", "2", "3", "4", "5", "6", "7",
"8", "9", "A", "B", "C", "D", "E", "F", "G", "H", "I", "J", "K", "L", "M", "N", "O", "P", "Q", "R", "S",
"T", "U", "V", "W", "X", "Y", "Z" };
public static String getShortUuid() {
StringBuffer stringBuffer = new StringBuffer();
String uuid = UUID.randomUUID().toString().replace("-", "");
for (int i = 0; i < 8; i++) {
String str = uuid.substring(i % 8 * 4, i % 8 * 4 + 4);
int strInteger = Integer.parseInt(str, 16);
stringBuffer.append(chars[strInteger % 0x3E]);
}
uuid = UUID.randomUUID().toString().replace("-", "");
for (int i = 0; i < 8; i++) {
String str = uuid.substring(i % 8 * 4, i % 8 * 4 + 4);
int strInteger = Integer.parseInt(str, 16);
stringBuffer.append(chars[strInteger % 0x3E]);
}
return stringBuffer.toString();
}
public static boolean isHttpUrl(String http) {
try {
if (!http.startsWith("http")) {
http = "http://" + http;
}
URL url = new URL(http);
url.openConnection().connect();
return true;
} catch (Exception e) {
return false;
}
}
public static String getUUID() {
UUID uuid = UUID.randomUUID();
String str = uuid.toString();
String uuidStr = str.replace("-", "");
return uuidStr;
}
// public static byte[] ZIPComperssor(byte[] body) {
// try {
// return Snappy.compress(body);
// } catch (IOException e) {
// e.printStackTrace();
// }
// return null;
// }
//
// public static byte[] ZIPDecompressor(byte[] body) {
// try {
// return Snappy.uncompress(body);
// } catch (IOException e) {
// e.printStackTrace();
// }
// return null;
//
// }
public static byte[] GZIPComperssor(byte[] body) {
if (body == null || body.length == 0) {
return null;
}
ByteArrayOutputStream out = new ByteArrayOutputStream();
GZIPOutputStream gzip;
try {
gzip = new GZIPOutputStream(out);
gzip.write(body);
gzip.close();
} catch (Exception e) {
e.printStackTrace();
}
return out.toByteArray();
}
public static byte[] GZIPDecompressor(byte[] body) {
if (body == null || body.length == 0) {
return null;
}
ByteArrayOutputStream out = new ByteArrayOutputStream();
ByteArrayInputStream in = new ByteArrayInputStream(body);
try {
GZIPInputStream ungzip = new GZIPInputStream(in);
byte[] buffer = new byte[256];
int n;
while ((n = ungzip.read(buffer)) >= 0) {
out.write(buffer, 0, n);
}
} catch (Exception e) {
e.printStackTrace();
}
return out.toByteArray();
}
/**
* java自带文件解压
*
* @param file
* @param pathFile
*/
public static boolean DZipInput(File file, File pathFile) {
try {
ZipInputStream Zin = new ZipInputStream(new FileInputStream(file));// 输入源zip路径
BufferedInputStream Bin = new BufferedInputStream(Zin);
String Parent = pathFile.getPath(); // 输出路径(文件夹目录)
File Fout = null;
ZipEntry entry;
while ((entry = Zin.getNextEntry()) != null && !entry.isDirectory()) {
Fout = new File(Parent, entry.getName());
if (!Fout.exists()) {
(new File(Fout.getParent())).mkdirs();
}
FileOutputStream out = new FileOutputStream(Fout);
BufferedOutputStream Bout = new BufferedOutputStream(out);
int b;
while ((b = Bin.read()) != -1) {
Bout.write(b);
}
Bout.close();
out.close();
}
Bin.close();
Zin.close();
return true;
} catch (IOException e) {
e.printStackTrace();
return false;
}
}
public static byte[] ArrayBytesDecrypt(byte[] data) {
ByteArrayOutputStream outputStream = new ByteArrayOutputStream();
DataOutputStream dataOutputStream = new DataOutputStream(outputStream);
byte[] key = new byte[16];
try {
int i;
for (i = 0; i < key.length; i++) {
key[i] = (byte) (data[i] - 125);
}
for (; i < data.length; i++) {
data[i] = (byte) (key[i % 16] ^ (data[i] + key[i % 16]));
dataOutputStream.writeByte(data[i]);
}
data = outputStream.toByteArray();
dataOutputStream.close();
outputStream.close();
} catch (IOException e) {
e.printStackTrace();
}
return data;
}
public static byte[] ArrayBytesEncryption(byte[] data) {
byte[] temp = new byte[data.length];
System.arraycopy(data, 0, temp, 0, temp.length);
byte[] string = getShortUuid().getBytes();
ByteArrayOutputStream outputStream = new ByteArrayOutputStream();
DataOutputStream dataOutputStream = new DataOutputStream(outputStream);
try {
int len = string.length;
for (int i = 0; i < len; i++) {
dataOutputStream.writeByte(string[i] + 125);
}
for (int i = 0; i < temp.length; i++) {
temp[i] = (byte) ((string[i % len] ^ temp[i]) - (string[i % len]));
dataOutputStream.writeByte(temp[i]);
}
temp = outputStream.toByteArray();
dataOutputStream.close();
outputStream.close();
} catch (IOException e) {
e.printStackTrace();
}
return temp;
}
/**
*
* @param content 请求的参数 格式为:name=xxx&pwd=xxx
* @param encodingString 服务器端请求编码。如GBK,UTF-8等
* @return
* @throws UnsupportedEncodingException
*/
public static String getAddresses(String content, String encodingString) throws UnsupportedEncodingException {
// 这里调用淘宝API
String urlStr = "http://ip.taobao.com/service/getIpInfo.php";
// 从http://whois.pconline.com.cn取得IP所在的省市区信息
String returnStr = getResult(urlStr, content, encodingString);
if (returnStr != null) {
// 处理返回的省市区信息
returnStr = decodeUnicode(returnStr);
String[] temp = returnStr.split(",");
if (temp.length < 3) {
return "0";// 无效IP,局域网测试
}
return returnStr;
}
return null;
}
/**
* @param urlStr 请求的地址
* @param content 请求的参数 格式为:name=xxx&pwd=xxx
* @param encoding 服务器端请求编码。如GBK,UTF-8等
* @return
*/
private static String getResult(String urlStr, String content, String encoding) {
URL url = null;
HttpURLConnection connection = null;
try {
url = new URL(urlStr + "?" + content);
connection = (HttpURLConnection) url.openConnection();// 新建连接实例
connection.setConnectTimeout(2000);// 设置连接超时时间,单位毫秒
connection.setReadTimeout(2000);// 设置读取数据超时时间,单位毫秒
connection.setDoOutput(true);// 是否打开输出流 true|false
connection.setDoInput(true);// 是否打开输入流true|false
connection.setRequestMethod("POST");// 提交方法POST|GET
connection.setUseCaches(false);// 是否缓存true|false
// DataOutputStream out = new
// DataOutputStream(connection.getOutputStream());// 打开输出流往对端服务器写数据
// out.writeBytes(content);// 写数据,也就是提交你的表单 name=xxx&pwd=xxx
// out.flush();// 刷新
// out.close();// 关闭输出流
if (connection.getResponseCode() == 200) {
BufferedReader reader = new BufferedReader(
new InputStreamReader(connection.getInputStream(), encoding));// 往对端写完数据对端服务器返回数据
// ,以BufferedReader流来读取
StringBuffer buffer = new StringBuffer();
String line = "";
while ((line = reader.readLine()) != null) {
buffer.append(line);
}
reader.close();
return buffer.toString();
}
} catch (IOException e) {
e.printStackTrace();
} finally {
if (connection != null) {
connection.disconnect();// 关闭连接
}
}
return null;
}
/**
* unicode 转换成 中文
*
* @author fanhui 2007-3-15
* @param theString
* @return
*/
public static String decodeUnicode(String theString) {
char aChar;
int len = theString.length();
StringBuffer outBuffer = new StringBuffer(len);
for (int x = 0; x < len;) {
aChar = theString.charAt(x++);
if (aChar == '\\') {
aChar = theString.charAt(x++);
if (aChar == 'u') {
int value = 0;
for (int i = 0; i < 4; i++) {
aChar = theString.charAt(x++);
switch (aChar) {
case '0':
case '1':
case '2':
case '3':
case '4':
case '5':
case '6':
case '7':
case '8':
case '9':
value = (value << 4) + aChar - '0';
break;
case 'a':
case 'b':
case 'c':
case 'd':
case 'e':
case 'f':
value = (value << 4) + 10 + aChar - 'a';
break;
case 'A':
case 'B':
case 'C':
case 'D':
case 'E':
case 'F':
value = (value << 4) + 10 + aChar - 'A';
break;
default:
throw new IllegalArgumentException("Malformed encoding.");
}
}
outBuffer.append((char) value);
} else {
if (aChar == 't') {
aChar = '\t';
} else if (aChar == 'r') {
aChar = '\r';
} else if (aChar == 'n') {
aChar = '\n';
} else if (aChar == 'f') {
aChar = '\f';
}
outBuffer.append(aChar);
}
} else {
outBuffer.append(aChar);
}
}
return outBuffer.toString();
}
public static String getPC_NAME() {
return System.getenv("COMPUTERNAME");
}
public static String getPC_sequence() {
Process process = null;
try {
process = Runtime.getRuntime().exec(new String[] { "wmic", "cpu", "get", "ProcessorId" });
process.getOutputStream().close();
Scanner sc = new Scanner(process.getInputStream());
String property = sc.next();
if (!property.trim().equalsIgnoreCase("ProcessorId")) {
sc.close();
return null;
}
String serial = sc.next();
sc.close();
return serial;
} catch (Exception e) {
e.printStackTrace();
} finally {
if (process != null)
process.destroy();
}
return "FFFFFFFFFFFFFFFF";
}
public static String getPCUser_NAME() {
return System.getProperty("user.name");
}
public static int random(int l, int r) {
return (int) (Math.random() * (r - l) + l);
}
public static String RipeMD128(String plainText) {
return RipeMD128(plainText.getBytes());
}
public static String RipeMD128(byte[] bytes) {
// 定义一个字节数组
byte[] secretBytes = null;
try {
// 生成一个MD5加密计算摘要
MessageDigest md = MessageDigest.getInstance("RipeMD128");
// 对字符串进行加密
md.update(bytes);
// 获得加密后的数据
secretBytes = md.digest();
} catch (NoSuchAlgorithmException e) {
throw new RuntimeException("没有RipeMD128这个算法!");
}
// 将加密后的数据转换为16进制数字
String md5code = new BigInteger(1, secretBytes).toString(16);// 16进制数字
// 如果生成数字未满32位,需要前面补0
for (int i = 0; i < 32 - md5code.length(); i++) {
md5code = "0" + md5code;
}
return md5code;
}
public static String MD5(String plainText) {
if (plainText != null) {
// 定义一个字节数组
byte[] secretBytes = null;
try {
// 生成一个MD5加密计算摘要
MessageDigest md = MessageDigest.getInstance("MD5");
// 对字符串进行加密
md.update(plainText.getBytes());
// 获得加密后的数据
secretBytes = md.digest();
} catch (NoSuchAlgorithmException e) {
throw new RuntimeException("没有md5这个算法!");
}
// 将加密后的数据转换为16进制数字
String md5code = new BigInteger(1, secretBytes).toString(16);// 16进制数字
// 如果生成数字未满32位,需要前面补0
for (int i = 0; i < 32 - md5code.length(); i++) {
md5code = "0" + md5code;
}
return md5code;
}
return null;
}
public static String Sha512(String plainText) {
if (plainText != null) {
// 定义一个字节数组
byte[] secretBytes = null;
try {
// 生成一个MD5加密计算摘要
MessageDigest md = MessageDigest.getInstance("sha512");
// 对字符串进行加密
md.update(plainText.getBytes());
// 获得加密后的数据
secretBytes = md.digest();
} catch (NoSuchAlgorithmException e) {
throw new RuntimeException("没有sha512这个算法!");
}
// 将加密后的数据转换为16进制数字
String md5code = new BigInteger(1, secretBytes).toString(16);// 16进制数字
// 如果生成数字未满512位,需要前面补0
for (int i = 0; i < 128 - md5code.length(); i++) {
md5code = "0" + md5code;
}
return md5code;
}
return null;
}
public static String Sha256(String plainText) {
if (plainText != null) {
// 定义一个字节数组
byte[] secretBytes = null;
try {
// 生成一个MD5加密计算摘要
MessageDigest md = MessageDigest.getInstance("sha256");
// 对字符串进行加密
md.update(plainText.getBytes());
// 获得加密后的数据
secretBytes = md.digest();
} catch (NoSuchAlgorithmException e) {
throw new RuntimeException("没有sha256这个算法!");
}
// 将加密后的数据转换为16进制数字
String md5code = new BigInteger(1, secretBytes).toString(16);// 16进制数字
// 如果生成数字未满512位,需要前面补0
for (int i = 0; i < 64 - md5code.length(); i++) {
md5code = "0" + md5code;
}
return md5code;
}
return null;
}
/**
* 数组截取
*
* @param rec
* @param i
* @param length
* @return
*/
public static byte[] subArrays(byte[] rec, int i, int length) {
byte[] by = new byte[length];
System.arraycopy(rec, i, by, 0, length);
return by;
}
public static byte[] LongToByteArray(long data) {
byte[] bytes = new byte[8];
bytes[0] = (byte) (data & 0xff);
bytes[1] = (byte) ((data >> 8) & 0xff);
bytes[2] = (byte) ((data >> 16) & 0xff);
bytes[3] = (byte) ((data >> 24) & 0xff);
bytes[4] = (byte) ((data >> 32) & 0xff);
bytes[5] = (byte) ((data >> 40) & 0xff);
bytes[6] = (byte) ((data >> 48) & 0xff);
bytes[7] = (byte) ((data >> 56) & 0xff);
return bytes;
}
public static long ByteArrayToLong(byte[] bytes) {
return (0xffL & (long) bytes[0]) | (0xff00L & ((long) bytes[1] << 8)) | (0xff0000L & ((long) bytes[2] << 16))
| (0xff000000L & ((long) bytes[3] << 24)) | (0xff00000000L & ((long) bytes[4] << 32))
| (0xff0000000000L & ((long) bytes[5] << 40)) | (0xff000000000000L & ((long) bytes[6] << 48))
| (0xff00000000000000L & ((long) bytes[7] << 56));
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy