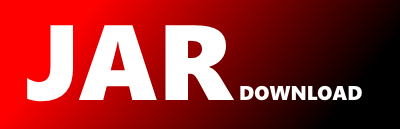
com.mugui.base.client.net.classutil.ClassTool Maven / Gradle / Ivy
The newest version!
package com.mugui.base.client.net.classutil;
import java.io.File;
import java.util.ArrayList;
import java.util.Enumeration;
import java.util.HashMap;
import java.util.List;
import java.util.jar.JarEntry;
import java.util.jar.JarFile;
public class ClassTool {
/**
* 从项目文件获取某包下所有类
*
* @param file 文件路径
* @param className 类名集合
* @param string
* @param string 是否遍历子包
* @return 类的完整名称
*/
public static List> getClassNameByFile(File file, List> className, String string) {
List> myClassName = new ArrayList>();
File[] childFiles = file.listFiles();
for (File childFile : childFiles) {
if (childFile.isDirectory()) {
myClassName.addAll(getClassNameByFile(childFile, myClassName, string));
} else {
String childFilePath = childFile.getPath();
if (childFilePath.endsWith(".class")) {
childFilePath = childFilePath.substring(childFilePath.indexOf("classes\\") + 8,
childFilePath.lastIndexOf("."));
childFilePath = childFilePath.replace("\\", ".");
try {
System.out.println(childFilePath);
myClassName.add(Class.forName(childFilePath));
} catch (ClassNotFoundException e) {
e.printStackTrace();
}
}
}
}
return myClassName;
}
/**
* 从jar获取某包下所有类
*
* @param jarPath jar文件路径
* @param string
* @param string 是否遍历子包
* @return 类的完整名称
*/
public static List> getClassNameByJar(String jarPath, String string) {
List> myClassName = new ArrayList>();
String[] jarInfo = jarPath.split("!");
String jarFilePath = jarInfo[0].substring(jarInfo[0].indexOf("/"));
String packagePath = jarInfo[1].substring(1);
if (packagePath.endsWith(".class")) {
packagePath = packagePath.substring(0, packagePath.lastIndexOf("/"));
}
try {
JarFile jarFile = new JarFile(jarFilePath);
Enumeration entrys = jarFile.entries();
while (entrys.hasMoreElements()) {
JarEntry jarEntry = entrys.nextElement();
String entryName = jarEntry.getName();
if (entryName.endsWith(".class")) {
System.out.println(entryName);
if (entryName.startsWith(packagePath)) {
entryName = entryName.replace("/", ".").substring(0, entryName.lastIndexOf("."));
int len = 0;
if ((len = entryName.indexOf("classes.")) > -1)
entryName = entryName.substring(len + 8);
try {
System.out.println(entryName);
myClassName.add(Thread.currentThread().getContextClassLoader().loadClass(entryName));
} catch (ClassNotFoundException e) {
e.printStackTrace();
}
}
}
}
jarFile.close();
} catch (Exception e) {
e.printStackTrace();
}
return myClassName;
}
/**
* 从jar获取某包下所有类
*
* @param string 是否遍历子包
* @param className
* @param jarPath jar文件路径
* @param string
* @return 类的完整名称
*/
public static List> getClassNameByJar(String jarPath, String className, ClassLoader string) {
HashMap, Boolean> map = new HashMap<>();
String[] jarInfo = jarPath.split("!");
int index = jarInfo[0].indexOf("/");
if (index == -1) {
index = jarInfo[0].indexOf("\\");
}
String jarFilePath = jarInfo[0].substring(index);
String packagePath = null;
if (jarInfo.length != 1)
packagePath = jarInfo[1].substring(1);
try {
JarFile jarFile = new JarFile(jarFilePath);
Enumeration entrys = jarFile.entries();
while (entrys.hasMoreElements()) {
JarEntry jarEntry = entrys.nextElement();
String entryName = jarEntry.getName();
if (entryName.endsWith(".class")) {
int i = entryName.indexOf(className);
if (i >= 0) {
entryName = entryName.substring(i).replace("/", ".");
entryName = entryName.substring(0, entryName.lastIndexOf("."));
int len = 0;
if ((len = entryName.indexOf("classes.")) > -1)
entryName = entryName.substring(len + 8);
try {
Class> class1 = string.loadClass(entryName);
if (map.get(class1) == null) {
map.put(class1, true);
}
} catch (ClassNotFoundException e) {
e.printStackTrace();
}
}
}
}
jarFile.close();
} catch (Exception e) {
e.printStackTrace();
}
return new ArrayList<>(map.keySet());
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy