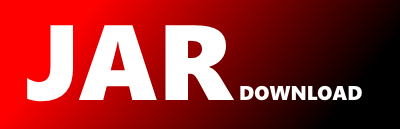
com.mugui.base.client.net.task.TaskCycleImpl Maven / Gradle / Ivy
The newest version!
package com.mugui.base.client.net.task;
import java.util.concurrent.ConcurrentLinkedDeque;
import com.mugui.util.Other;
import lombok.Getter;
public abstract class TaskCycleImpl extends TaskImpl {
@Getter
ConcurrentLinkedDeque cycleList = new ConcurrentLinkedDeque<>();
@Override
public void run() {
while (true) {
try {
handle();
} catch (Exception e) {
e.printStackTrace();
}
Other.sleep(10000);
}
}
private void handle() throws InterruptedException {
while (true) {
T poll = cycleList.poll();
if (poll == null) {
synchronized (cycleList) {
poll = cycleList.poll();
if (poll == null) {
cycleList.wait();
continue;
}
}
}
handle(poll);
}
}
/**
* 最终处理
* @param poll
*/
protected abstract void handle(T poll);
public void add(T data) {
synchronized (cycleList) {
cycleList.push(data);
cycleList.notifyAll();
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy