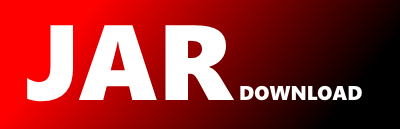
cn.ocoop.shiro.cache.ShiroRealmCacheManager Maven / Gradle / Ivy
package cn.ocoop.shiro.cache;
import cn.ocoop.spring.App;
import org.apache.shiro.authc.AuthenticationInfo;
import org.apache.shiro.authz.AuthorizationInfo;
import org.apache.shiro.cache.Cache;
import org.apache.shiro.cache.CacheManager;
import org.apache.shiro.mgt.DefaultSecurityManager;
import org.apache.shiro.realm.AuthenticatingRealm;
import org.apache.shiro.realm.AuthorizingRealm;
import org.apache.shiro.realm.Realm;
import org.apache.shiro.subject.PrincipalCollection;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import java.util.Collection;
import java.util.Optional;
public class ShiroRealmCacheManager {
private static final Logger log = LoggerFactory.getLogger(ShiroRealmCacheManager.class);
public static void clearCachedAuthenticationInfo(PrincipalCollection principals) {
if (principals == null) return;
getRealms().forEach(realm -> {
if (realm instanceof AuthenticatingRealm) {
Optional.ofNullable(getAuthenticationCache((AuthenticatingRealm) realm))
.ifPresent(cache -> cache.remove(principals));
}
});
}
public static void clearCachedAuthenticationInfo(PrincipalCollection principals, Class extends AuthenticatingRealm>... realms) {
if (principals == null) return;
getRealms().forEach(realm -> {
for (Class realmClass : realms) {
if (realmClass.isAssignableFrom(realm.getClass())) {
Optional.ofNullable(getAuthenticationCache((AuthenticatingRealm) realm))
.ifPresent(cache -> cache.remove(principals));
}
}
});
}
public static void clearCachedAuthenticationInfo(Class extends AuthenticatingRealm>... realms) {
getRealms().forEach(realm -> {
for (Class realmClass : realms) {
if (realmClass.isAssignableFrom(realm.getClass())) {
Optional.ofNullable(getAuthenticationCache((AuthenticatingRealm) realm))
.ifPresent(cache -> cache.clear());
}
}
});
}
public static void clearAllCachedAuthenticationInfo() {
getRealms().forEach(realm -> {
if (realm instanceof AuthenticatingRealm) {
Optional.ofNullable(getAuthenticationCache((AuthenticatingRealm) realm))
.ifPresent(cache -> cache.clear());
}
});
}
private static Cache
© 2015 - 2025 Weber Informatics LLC | Privacy Policy