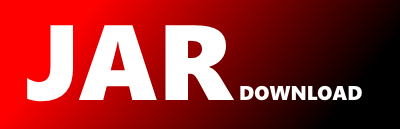
cn.opencodes.framework.autoconfigure.MVCAutoConfiguration Maven / Gradle / Ivy
package cn.opencodes.framework.autoconfigure;
import java.util.List;
import java.util.Map;
import javax.servlet.DispatcherType;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.boot.web.servlet.FilterRegistrationBean;
import org.springframework.context.annotation.Bean;
import org.springframework.context.annotation.DependsOn;
import org.springframework.web.cors.CorsConfiguration;
import org.springframework.web.cors.UrlBasedCorsConfigurationSource;
import org.springframework.web.filter.CorsFilter;
import org.springframework.web.servlet.config.annotation.InterceptorRegistry;
import org.springframework.web.servlet.config.annotation.ResourceHandlerRegistry;
import org.springframework.web.servlet.config.annotation.WebMvcConfigurer;
import org.springframework.web.servlet.handler.HandlerInterceptorAdapter;
import cn.opencodes.framework.autoconfigure.properties.AlphaProperties;
import cn.opencodes.framework.core.interceptor.AccessInterceptor;
import cn.opencodes.framework.core.interceptor.MaliciousInterceptor;
import cn.opencodes.framework.core.utils.SpringUtils;
import cn.opencodes.framework.tools.xss.XssFilter;
/**
* MVC配置
* @author HJ
*/
@DependsOn("springUtils")
public class MVCAutoConfiguration implements WebMvcConfigurer{
@Autowired
private AlphaProperties props;
@Autowired(required=false)
private MaliciousInterceptor maliciousInterceptor;
@Autowired(required=false)
private AccessInterceptor accessInterceptor;
@Override
public void addInterceptors(InterceptorRegistry registry) {
// 访问请求拦截
if (accessInterceptor != null) {
registry.addInterceptor(accessInterceptor).addPathPatterns("/"+props.getModule()+"/**");
}
// 恶意请求拦截
if (maliciousInterceptor != null) {
registry.addInterceptor(maliciousInterceptor).addPathPatterns("/"+props.getModule()+"/**");
}
// 其他模块自定义拦截器
Map interceptors = props.getInterceptors();
if (interceptors != null) {
for (String k : interceptors.keySet()) {
HandlerInterceptorAdapter interceptor = SpringUtils.getBean(k, HandlerInterceptorAdapter.class);
registry.addInterceptor(interceptor).addPathPatterns(interceptors.get(k));
}
}
}
@Override
public void addResourceHandlers(ResourceHandlerRegistry registry) {
registry.addResourceHandler("doc.html").addResourceLocations("classpath:/META-INF/resources/");
registry.addResourceHandler("swagger-ui.html").addResourceLocations("classpath:/META-INF/resources/");
registry.addResourceHandler("/webjars/**").addResourceLocations("classpath:/META-INF/resources/webjars/");
List resources = props.getResources();
if (resources != null) {
for (String res : resources) {
String[] fs = res.split(":");
registry.addResourceHandler(fs[0].trim()).addResourceLocations(fs[1].trim());
}
}
}
@Bean
public FilterRegistrationBean corsFilter() {
UrlBasedCorsConfigurationSource source = new UrlBasedCorsConfigurationSource();
CorsConfiguration config = new CorsConfiguration();
config.setAllowCredentials(true);
// 设置你要允许的网站域名,如果全允许则设为 *
config.addAllowedOrigin("*");
// 如果要限制 HEADER 或 METHOD 请自行更改
config.addAllowedHeader("*");
config.addAllowedMethod("*");
source.registerCorsConfiguration("/"+props.getModule()+"/**", config);
FilterRegistrationBean bean = new FilterRegistrationBean<>(new CorsFilter(source));
// 这个顺序很重要哦,为避免麻烦请设置在最前
bean.setOrder(0);
return bean;
}
@Bean
public FilterRegistrationBean xssFilterRegistration() {
FilterRegistrationBean registration = new FilterRegistrationBean<>();
registration.setDispatcherTypes(DispatcherType.REQUEST);
registration.setFilter(new XssFilter());
registration.addUrlPatterns("/*");
registration.setName("xssFilter");
registration.setOrder(Integer.MAX_VALUE);
return registration;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy