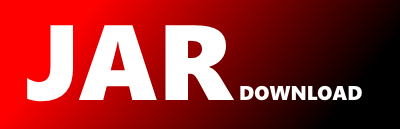
cn.patterncat.converter.JodConverterProperties Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of springboot-starter-jodconverter Show documentation
Show all versions of springboot-starter-jodconverter Show documentation
springboot-starter-jodconverter
The newest version!
package cn.patterncat.converter;
import org.springframework.boot.context.properties.ConfigurationProperties;
/** Configuration class for JODConverter. */
@ConfigurationProperties("jodconverter")
public class JodConverterProperties {
/**
* Enable JODConverter, which means that office instances will be launched. If not set, it
* defaults to false.
*/
private boolean enabled = true;
private int poolMaxTotal = 8;
private int poolMaxIdle = 8; //生产上建议跟max total一致,避免makeObject开销
private int poolMinIdle = 4;
private boolean preparePool = true;
/**
* Represents the office home directory. If not set, it defaults to an auto-detected home
* directory (most recent version of LibreOffice first).
*/
private String officeHome;
/**
* List of ports, separated by commas, used by each JODConverter processing thread. The number of
* office instances is equal to the number of ports, since 1 office will be launched for each port
* number. If not set, it defaults to a list with a single port, 2002.
*/
private String portNumbers = "2002";
/**
* Directory where temporary office profiles will be created. If not set, it defaults to the
* system temporary directory as specified by the java.io.tmpdir system property.
*/
private String workingDir;
/**
* Template profile directory to copy to a created office profile directory when an office
* processed is launched.
*/
private String templateProfileDir;
/**
* Indicates whether we must kill existing office process when an office process already exists
* for the same connection string. If not set, it defaults to true.
*/
private boolean killExistingProcess = true;
/**
* Process timeout (milliseconds). Used when trying to execute an office process call
* (start/terminate). If not set, it defaults to 120000 (2 minutes).
*/
private long processTimeout = 120000L;
/**
* Process retry interval (milliseconds). Used for waiting between office process call tries
* (start/terminate). If not set, it defaults to 250.
*/
private long processRetryInterval = 250L;
/**
* Maximum time allowed to process a task. If the processing time of a task is longer than this
* timeout, this task will be aborted and the next task is processed. If not set, it defaults to
* 120000 (2 minutes).
*/
private long taskExecutionTimeout = 120000L;
/**
* Maximum number of tasks an office process can execute before restarting. If not set, it
* defaults to 200.
*/
private int maxTasksPerProcess = 200;
/**
* Maximum living time of a task in the conversion queue. The task will be removed from the queue
* if the waiting time is longer than this timeout. If not set, it defaults to 30000 (30 seconds).
*/
private long taskQueueTimeout = 30000L;
public boolean isEnabled() {
return enabled;
}
public void setEnabled(final boolean enabled) {
this.enabled = enabled;
}
public String getOfficeHome() {
return officeHome;
}
public void setOfficeHome(final String officeHome) {
this.officeHome = officeHome;
}
public String getPortNumbers() {
return portNumbers;
}
public void setPortNumbers(final String portNumbers) {
this.portNumbers = portNumbers;
}
public String getWorkingDir() {
return workingDir;
}
public void setWorkingDir(final String workingDir) {
this.workingDir = workingDir;
}
public String getTemplateProfileDir() {
return templateProfileDir;
}
public void setTemplateProfileDir(final String templateProfileDir) {
this.templateProfileDir = templateProfileDir;
}
public boolean isKillExistingProcess() {
return killExistingProcess;
}
public void setKillExistingProcess(final boolean killExistingProcess) {
this.killExistingProcess = killExistingProcess;
}
public long getProcessTimeout() {
return processTimeout;
}
public void setProcessTimeout(final long processTimeout) {
this.processTimeout = processTimeout;
}
public long getProcessRetryInterval() {
return processRetryInterval;
}
public void setProcessRetryInterval(final long procesRetryInterval) {
this.processRetryInterval = procesRetryInterval;
}
public long getTaskExecutionTimeout() {
return taskExecutionTimeout;
}
public void setTaskExecutionTimeout(final long taskExecutionTimeout) {
this.taskExecutionTimeout = taskExecutionTimeout;
}
public int getMaxTasksPerProcess() {
return maxTasksPerProcess;
}
public void setMaxTasksPerProcess(final int maxTasksPerProcess) {
this.maxTasksPerProcess = maxTasksPerProcess;
}
public long getTaskQueueTimeout() {
return taskQueueTimeout;
}
public void setTaskQueueTimeout(final long taskQueueTimeout) {
this.taskQueueTimeout = taskQueueTimeout;
}
public int getPoolMaxTotal() {
return poolMaxTotal;
}
public void setPoolMaxTotal(int poolMaxTotal) {
this.poolMaxTotal = poolMaxTotal;
}
public int getPoolMaxIdle() {
return poolMaxIdle;
}
public void setPoolMaxIdle(int poolMaxIdle) {
this.poolMaxIdle = poolMaxIdle;
}
public int getPoolMinIdle() {
return poolMinIdle;
}
public void setPoolMinIdle(int poolMinIdle) {
this.poolMinIdle = poolMinIdle;
}
public boolean isPreparePool() {
return preparePool;
}
public void setPreparePool(boolean preparePool) {
this.preparePool = preparePool;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy