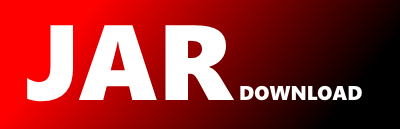
cn.nukkit.metadata.LazyMetadataValue Maven / Gradle / Ivy
package cn.nukkit.metadata;
import cn.nukkit.api.PowerNukkitXOnly;
import cn.nukkit.api.Since;
import cn.nukkit.plugin.Plugin;
import com.dfsek.terra.lib.commons.lang3.Validate;
import java.lang.ref.SoftReference;
import java.util.concurrent.Callable;
/**
* The LazyMetadataValue class implements a type of metadata that is not
* computed until another plugin asks for it.
*
* By making metadata values lazy, no computation is done by the providing
* plugin until absolutely necessary (if ever). Additionally,
* LazyMetadataValue objects cache their values internally unless overridden
* by a {@link CacheStrategy} or invalidated at the individual or plugin
* level. Once invalidated, the LazyMetadataValue will recompute its value
* when asked.
*/
@PowerNukkitXOnly
@Since("1.19.63-r2")
public class LazyMetadataValue extends MetadataValueAdapter {
private Callable
© 2015 - 2025 Weber Informatics LLC | Privacy Policy