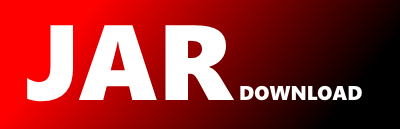
cn.nukkit.entity.data.property.EntityProperty Maven / Gradle / Ivy
package cn.nukkit.entity.data.property;
import cn.nukkit.nbt.tag.CompoundTag;
import cn.nukkit.nbt.tag.ListTag;
import cn.nukkit.network.protocol.SyncEntityPropertyPacket;
import java.util.*;
/**
* @author Peng_Lx
*/
public abstract class EntityProperty {
private static final String PLAYER_KEY = "minecraft:player";
private static final String PROPERTIES_KEY = "properties";
private static final Map> entityPropertyMap = new HashMap<>();
private static List packetCache = new ArrayList<>();
private static CompoundTag playerPropertyCache = new CompoundTag("");
private final String identifier;
public EntityProperty(String identifier) {
this.identifier = identifier;
}
public static boolean register(String entityIdentifier, EntityProperty property) {
List entityProperties = entityPropertyMap.getOrDefault(entityIdentifier, new ArrayList<>());
for (EntityProperty entityProperty : entityProperties) {
if (Objects.equals(entityProperty.identifier, property.identifier)) return false;
}
entityProperties.add(property);
entityPropertyMap.put(entityIdentifier, entityProperties);
return true;
}
public static void buildPacket() {
List packetList = new ArrayList<>();
for (Map.Entry> entry : entityPropertyMap.entrySet()) {
ListTag listProperty = buildPropertyList(entry.getValue());
CompoundTag nbt = new CompoundTag().putList(PROPERTIES_KEY, listProperty).putString("type", entry.getKey());
SyncEntityPropertyPacket packet = new SyncEntityPropertyPacket();
packet.setData(nbt);
packetList.add(packet);
}
packetCache = packetList;
}
public static void buildPlayerProperty() {
List properties = entityPropertyMap.get(PLAYER_KEY);
if (properties == null) {
playerPropertyCache = new CompoundTag("");
return;
}
ListTag listProperty = buildPropertyList(properties);
playerPropertyCache = new CompoundTag().putList(PROPERTIES_KEY, listProperty).putString("type", PLAYER_KEY);
}
public static List getPacketCache() {
return packetCache;
}
public static CompoundTag getPlayerPropertyCache() {
return playerPropertyCache;
}
public static List getEntityProperty(String identifier) {
return entityPropertyMap.getOrDefault(identifier, new ArrayList<>());
}
public String getIdentifier() {
return identifier;
}
public abstract void populateTag(CompoundTag tag);
private static ListTag buildPropertyList(List properties) {
ListTag listProperty = new ListTag<>();
for (EntityProperty entityProperty : properties) {
CompoundTag propertyTag = new CompoundTag();
propertyTag.putString("name", entityProperty.getIdentifier());
entityProperty.populateTag(propertyTag);
listProperty.add(propertyTag);
}
return listProperty;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy