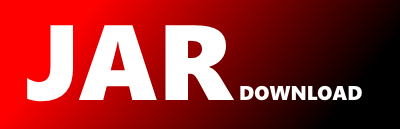
cn.schoolwow.quickdao.domain.entity.SqlLog Maven / Gradle / Ivy
package cn.schoolwow.quickdao.domain.entity;
import cn.schoolwow.quickdao.annotation.*;
import cn.schoolwow.quickdao.domain.database.parent.DatabaseType;
import java.time.LocalDateTime;
@Ignore
@TableName("quickdao_sql_log")
@Comment("SQL日志")
public class SqlLog {
@Id
private long id;
@Comment("类型(0:查询,1:更新)")
private Integer type;
@Comment("名称")
@ColumnType("varchar(128)")
private String name;
@Comment("用时-毫秒")
private Long consumeTime;
@Comment("原始语句")
@ColumnType(value = "longtext", databaseType = DatabaseType.Mysql)
@ColumnType(value = "longtext", databaseType = DatabaseType.MariaDB)
@ColumnType(value = "text", databaseType = DatabaseType.CURRENT)
private String statement;
@Comment("格式化语句")
@ColumnType(value = "longtext", databaseType = DatabaseType.Mysql)
@ColumnType(value = "longtext", databaseType = DatabaseType.MariaDB)
@ColumnType(value = "text", databaseType = DatabaseType.CURRENT)
private String formatStatement;
@Comment("参数列表")
@ColumnType(value = "longtext", databaseType = DatabaseType.Mysql)
@ColumnType(value = "longtext", databaseType = DatabaseType.MariaDB)
@ColumnType(value = "text", databaseType = DatabaseType.CURRENT)
private String parameters;
@Comment("更新影响行数")
private Integer effect;
@Comment("异常信息")
@ColumnType(value = "mediumtext", databaseType = DatabaseType.Mysql)
@ColumnType(value = "mediumtext", databaseType = DatabaseType.MariaDB)
@ColumnType(value = "text", databaseType = DatabaseType.CURRENT)
private String exception;
@TableField(createdAt = true)
private LocalDateTime createdAt;
@TableField(updatedAt = true)
private LocalDateTime updatedAt;
public long getId() {
return id;
}
public void setId(long id) {
this.id = id;
}
public Integer getType() {
return type;
}
public void setType(Integer type) {
this.type = type;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public Long getConsumeTime() {
return consumeTime;
}
public void setConsumeTime(Long consumeTime) {
this.consumeTime = consumeTime;
}
public String getStatement() {
return statement;
}
public void setStatement(String statement) {
this.statement = statement;
}
public String getFormatStatement() {
return formatStatement;
}
public void setFormatStatement(String formatStatement) {
this.formatStatement = formatStatement;
}
public String getParameters() {
return parameters;
}
public void setParameters(String parameters) {
this.parameters = parameters;
}
public Integer getEffect() {
return effect;
}
public void setEffect(Integer effect) {
this.effect = effect;
}
public String getException() {
return exception;
}
public void setException(String exception) {
this.exception = exception;
}
public LocalDateTime getCreatedAt() {
return createdAt;
}
public void setCreatedAt(LocalDateTime createdAt) {
this.createdAt = createdAt;
}
public LocalDateTime getUpdatedAt() {
return updatedAt;
}
public void setUpdatedAt(LocalDateTime updatedAt) {
this.updatedAt = updatedAt;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy