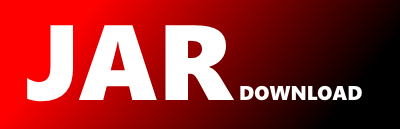
cn.schoolwow.quickdao.module.database.dml.flow.common.CheckFieldConstraintFlow Maven / Gradle / Ivy
package cn.schoolwow.quickdao.module.database.dml.flow.common;
import cn.schoolwow.quickdao.domain.entity.Property;
import cn.schoolwow.quickflow.domain.FlowContext;
import cn.schoolwow.quickflow.flow.BusinessFlow;
import java.math.BigDecimal;
public class CheckFieldConstraintFlow implements BusinessFlow {
@Override
public void executeBusinessFlow(FlowContext flowContext) throws Exception {
Property property = (Property) flowContext.checkData("property");
Object value = flowContext.getData("value");
if(property.id||property.createdAt||property.updateAt){
return;
}
if(null!=property.notNull&&property.notNull&&null==value){
flowContext.putData("reason", "字段"+property.column+"的值为空");
return;
}
if(null==value){
return;
}
String reason = null;
switch (property.columnType.toLowerCase()){
case "mediumint":{
int intValue = (int) value;
if(intValue<-8388608||intValue>16777215){
reason = "字段"+property.column+"类型为mediumint,限制范围为[-8388608,16777215],当前值为"+intValue;
}
}break;
case "char":{
int valueLength = value.toString().length();
if(valueLength>255){
reason = "字段"+property.column+"类型为char,限制长度为[0,255],当前长度为"+valueLength;
}
}break;
case "varchar":{
if(null==property.range){
break;
}
int valueLength = value.toString().length();
int maxLength = null== property.range?65535:Math.min(Integer.parseInt(property.range), 65535);
if(valueLength>maxLength){
reason = "字段"+property.column+"类型为varchar,限制长度为[0,"+maxLength+"],当前长度为"+valueLength;
}
}break;
case "text":{
int valueLength = value.toString().length();
if(valueLength>65535){
reason = "字段"+property.column+"类型为text,限制长度为[0,65535],当前长度为"+valueLength;
}
}break;
case "decimal":{
if(null==property.range){
break;
}
BigDecimal number = (BigDecimal) value;
int integerDigits = number.precision() - number.scale();
int decimalDigits = number.scale();
int limitIntegerDigits = Integer.parseInt(property.range.substring(0,property.range.indexOf(",")));
if(integerDigits>limitIntegerDigits){
reason = "字段"+property.column+"类型为decimal("+property.range+"),整数位数超过限制,当前整数位数为"+integerDigits+",小数位数为"+decimalDigits+",数值为"+number;
break;
}
int limitDecimalDigits = Integer.parseInt(property.range.substring(property.range.indexOf(",")+1));
if(decimalDigits>limitDecimalDigits){
reason = "字段"+property.column+"类型为decimal("+property.range+"),小数位数超过限制,当前整数位数为"+integerDigits+",小数位数为"+decimalDigits+",数值为"+number;
break;
}
}break;
}
flowContext.putData("reason", reason);
}
@Override
public String name() {
return "检查字段约束";
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy