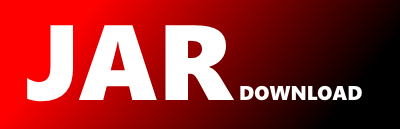
cn.schoolwow.data.thread.flow.work.ExecuteMapWorkFlow Maven / Gradle / Ivy
package cn.schoolwow.data.thread.flow.work;
import cn.schoolwow.data.thread.domain.DataThreadProgress;
import cn.schoolwow.data.thread.flow.handler.WorkTryCatchFinallyHandler;
import cn.schoolwow.data.thread.flow.work.map.GetMapFileFlow;
import cn.schoolwow.data.thread.listener.ProgressListener;
import cn.schoolwow.data.thread.work.map.MapDataThreadHandler;
import cn.schoolwow.data.thread.work.map.MapFile;
import cn.schoolwow.quickflow.domain.FlowContext;
import cn.schoolwow.quickflow.flow.BusinessFlow;
import cn.schoolwow.quickflow.flow.FunctionFlow;
import org.apache.commons.io.FileUtils;
import java.io.File;
import java.util.ArrayList;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import java.util.concurrent.ThreadPoolExecutor;
import java.util.concurrent.atomic.AtomicInteger;
public class ExecuteMapWorkFlow implements BusinessFlow {
@Override
public void executeBusinessFlow(FlowContext flowContext) throws Exception {
String name = (String) flowContext.checkData("name");
ThreadPoolExecutor threadPoolExecutor = (ThreadPoolExecutor) flowContext.checkData("threadPoolExecutor");
List mapDataThreadHandlerList = (List) flowContext.checkData("mapDataThreadHandlerList");
ProgressListener progressListener = (ProgressListener) flowContext.getData("progressListener");
Map threadExceptionMap = (Map) flowContext.getData("threadExceptionMap", new HashMap<>());
DataThreadProgress dataThreadProgress = (DataThreadProgress) flowContext.checkData("dataThreadProgress");
dataThreadProgress.total = mapDataThreadHandlerList.size();
dataThreadProgress.type = "Map";
AtomicInteger progress = new AtomicInteger(1);
final List idList = new ArrayList<>();
flowContext.putTemporaryData("idList", idList);
for(MapDataThreadHandler mapDataThreadHandler:mapDataThreadHandlerList){
threadPoolExecutor.execute(()->{
flowContext.startFlow("执行Map单个线程任务")
.tryCatchFinallyHandler(new WorkTryCatchFinallyHandler())
.putThreadLocalData("delta", 1)
.putThreadLocalData("handleFunction", new FunctionFlow() {
@Override
public void executeFunction() throws Exception {
MapFile mapFile = mapDataThreadHandler.map();
if(null==mapFile){
return;
}
synchronized (idList){
idList.add(mapFile.id);
}
File file = (File) flowContext.startFlow(new GetMapFileFlow())
.putThreadLocalData("workName", name)
.putThreadLocalData("id", mapFile.id)
.execute()
.checkData("mapFile");
FileUtils.writeStringToFile(file, mapFile.content, "UTF-8");
}
})
.execute();
});
}
}
@Override
public String name() {
return "执行Map类型任务";
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy