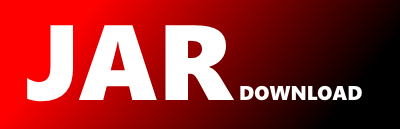
cn.schoolwow.sdk.weiyun.WeiYunAPIImpl Maven / Gradle / Ivy
package cn.schoolwow.sdk.weiyun;
import cn.schoolwow.quickhttp.QuickHttp;
import cn.schoolwow.quickhttp.request.Request;
import cn.schoolwow.quickhttp.response.Response;
import cn.schoolwow.sdk.weiyun.domain.*;
import cn.schoolwow.sdk.weiyun.exception.WeiYunException;
import com.alibaba.fastjson.JSONArray;
import com.alibaba.fastjson.JSONObject;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import java.io.IOException;
import java.io.UnsupportedEncodingException;
import java.net.HttpCookie;
import java.net.URLEncoder;
import java.util.ArrayList;
import java.util.List;
/**
* 微云SDK实现类
*/
public class WeiYunAPIImpl implements WeiYunAPI {
private Logger logger = LoggerFactory.getLogger(WeiYunAPI.class);
private String skey;
private String gtk;
private volatile String rootDirKey = null;
private volatile String mainDirKey = null;
public WeiYunAPIImpl() {
}
public WeiYunAPIImpl(String cookieString) {
setCookie(cookieString);
}
@Override
public void setCookie(String cookieString) {
QuickHttp.clientConfig().cookieOption().addCookieString("weiyun.com", cookieString);
HttpCookie skeyCookie = QuickHttp.clientConfig().cookieOption().getCookie("weiyun.com", "skey");
if (null != skeyCookie) {
skey = skeyCookie.getValue();
}
HttpCookie gtkCookie = QuickHttp.clientConfig().cookieOption().getCookie("weiyun.com", "wyctoken");
if (null != gtkCookie) {
gtk = gtkCookie.getValue();
}
}
@Override
public boolean hasCookieExpire() throws IOException {
String api = "https://www.weiyun.com/webapp/json/weiyunQdiskClient/DiskUserInfoGet?refer=chrome_windows&g_tk=" + gtk + "&r=" + Math.random();
String body = getBody(2201, "\\\".weiyun.DiskUserInfoGetMsgReq_body\\\":{\\\"is_get_upload_flow_flag\\\":true,\\\"is_get_high_speed_flow_info\\\":true,\\\"is_get_weiyun_flag\\\":true}");
Response response = QuickHttp.connect(api)
.method(Request.Method.POST)
.ignoreHttpErrors(true)
.requestBody(body)
.execute();
if (response.statusCode() != 200 || response.body().contains("(190051)您的登录态已失效")) {
return true;
}
return false;
}
@Override
public UserConfig diskUserInfoGet() throws IOException {
String api = "https://www.weiyun.com/webapp/json/weiyunQdiskClient/DiskUserInfoGet?refer=chrome_windows&g_tk=" + gtk + "&r=" + Math.random();
String body = getBody(2201, "\\\".weiyun.DiskUserInfoGetMsgReq_body\\\":{\\\"is_get_upload_flow_flag\\\":true,\\\"is_get_high_speed_flow_info\\\":true,\\\"is_get_weiyun_flag\\\":true}");
Response response = QuickHttp.connect(api)
.method(Request.Method.POST)
.requestBody(body)
.execute();
checkResponse(response);
JSONObject result = response.bodyAsJSONObject().getJSONObject("data").getJSONObject("rsp_body").getJSONObject("RspMsg_body");
UserConfig userConfig = new UserConfig();
userConfig.usedSpace = result.getLong("used_space");
userConfig.totalSpace = result.getLong("total_space");
userConfig.nickName = result.getString("nick_name");
userConfig.uin = result.getString("uin");
userConfig.rootDirKey = result.getString("root_dir_key");
userConfig.mainDirKey = result.getString("main_dir_key");
rootDirKey = userConfig.rootDirKey;
mainDirKey = userConfig.mainDirKey;
return userConfig;
}
@Override
public WeiYunDirectory diskDirBatchList() throws IOException {
diskUserInfoGet();
return diskDirBatchList(rootDirKey, mainDirKey);
}
@Override
public WeiYunDirectory diskDirBatchList(WeiYunDirectory weiYunDirectory) throws IOException {
return diskDirBatchList(weiYunDirectory.pdirKey, weiYunDirectory.dirKey);
}
@Override
public WeiYunDirectoryCreate diskDirCreate(WeiYunDirectory parentDirectory, String dirName) throws IOException {
String api = "https://www.weiyun.com/webapp/json/weiyunQdiskClient/DiskDirCreate?refer=chrome_windows&g_tk=" + gtk + "&r=" + Math.random();
String body = getBody(2614, "\\\".weiyun.DiskDirCreateMsgReq_body\\\":{\\\"pdir_key\\\":\\\"" + parentDirectory.pdirKey + "\\\",\\\"ppdir_key\\\":\\\"" + parentDirectory.ppdirKey + "\\\",\\\"dir_name\\\":\\\"" + dirName + "\\\",\\\"file_exist_option\\\":2,\\\"create_type\\\":1}");
Response response = QuickHttp.connect(api)
.method(Request.Method.POST)
.requestBody(body)
.execute();
checkResponse(response);
JSONObject result = response.bodyAsJSONObject().getJSONObject("data").getJSONObject("rsp_body").getJSONObject("RspMsg_body");
WeiYunDirectoryCreate weiYunDirectoryCreate = new WeiYunDirectoryCreate();
weiYunDirectoryCreate.dirKey = result.getString("dir_key");
weiYunDirectoryCreate.dirName = result.getString("dir_name");
weiYunDirectoryCreate.dirCtime = result.getDate("dir_ctime");
weiYunDirectoryCreate.dirMtime = result.getDate("dir_mtime");
weiYunDirectoryCreate.pdirMtime = result.getDate("pdir_mtime");
weiYunDirectoryCreate.pdirKey = result.getString("pdir_key");
return weiYunDirectoryCreate;
}
@Override
public WeiYunDirectory diskDirFileBatchDeleteEx(List weiYunDirectoryList, List weiYunFileList) throws IOException {
if (null == weiYunDirectoryList) {
weiYunDirectoryList = new ArrayList<>();
}
if (null == weiYunFileList) {
weiYunFileList = new ArrayList<>();
}
String api = "https://www.weiyun.com/webapp/json/weiyunQdiskClient/DiskDirFileBatchDeleteEx?refer=chrome_windows&g_tk=" + gtk + "&r=" + Math.random();
StringBuilder bodyBuilder = new StringBuilder("\\\".weiyun.DiskDirFileBatchDeleteExMsgReq_body\\\":{\\\"file_list\\\":[");
for (WeiYunFile weiYunFile : weiYunFileList) {
bodyBuilder.append("{\\\"ppdir_key\\\":\\\"" + weiYunFile.ppdirKey + "\\\",\\\"pdir_key\\\":\\\"" + weiYunFile.pdirKey + "\\\",\\\"file_id\\\":\\\"" + weiYunFile.fileId + "\\\",\\\"filename\\\":\\\"" + weiYunFile.fileName + "\\\"},");
}
if (weiYunFileList.size() > 0) {
bodyBuilder.deleteCharAt(bodyBuilder.length() - 1);
}
bodyBuilder.append("],\\\"dir_list\\\":[");
for (WeiYunDirectory weiYunDirectory : weiYunDirectoryList) {
bodyBuilder.append("{\\\"ppdir_key\\\":\\\"" + weiYunDirectory.ppdirKey + "\\\",\\\"pdir_key\\\":\\\"" + weiYunDirectory.pdirKey + "\\\",\\\"dir_key\\\":\\\"" + weiYunDirectory.dirKey + "\\\",\\\"dir_name\\\":\\\"" + weiYunDirectory.dirName + "\\\"},");
}
if (weiYunDirectoryList.size() > 0) {
bodyBuilder.deleteCharAt(bodyBuilder.length() - 1);
}
bodyBuilder.append("]}");
String body = getBody(2509, bodyBuilder.toString());
Response response = QuickHttp.connect(api)
.method(Request.Method.POST)
.requestBody(body)
.execute();
checkResponse(response);
JSONObject result = response.bodyAsJSONObject().getJSONObject("data").getJSONObject("rsp_body").getJSONObject("RspMsg_body");
WeiYunDirectory weiYunDirectory = new WeiYunDirectory();
weiYunDirectory.weiYunFileList = getWeiYunFileList(result.getJSONArray("file_list"), null, null);
weiYunDirectory.weiYunDirectoryList = getWeiYunDirectoryList(result.getJSONArray("dir_list"), null, null);
return weiYunDirectory;
}
@Override
public WeiYunFileDownload diskFileBatchDownload(WeiYunFile weiYunFile) throws IOException {
String api = "https://www.weiyun.com/webapp/json/weiyunQdiskClient/DiskFileBatchDownload?refer=chrome_windows&g_tk=" + gtk + "&r=" + Math.random();
String body = getBody(2402, "\\\".weiyun.DiskFileBatchDownloadMsgReq_body\\\":{\\\"file_list\\\":[{\\\"file_id\\\":\\\"" + weiYunFile.fileId + "\\\",\\\"pdir_key\\\":\\\"" + weiYunFile.pdirKey + "\\\"}],\\\"download_type\\\":0}");
Response response = QuickHttp.connect(api)
.method(Request.Method.POST)
.requestBody(body)
.execute();
checkResponse(response);
JSONObject result = response.bodyAsJSONObject().getJSONObject("data").getJSONObject("rsp_body").getJSONObject("RspMsg_body").getJSONArray("file_list").getJSONObject(0);
WeiYunFileDownload weiYunFileDownload = new WeiYunFileDownload();
weiYunFileDownload.retcode = result.getInteger("retcode");
weiYunFileDownload.retmsg = result.getString("retmsg");
weiYunFileDownload.encodeUrl = result.getString("encode_url");
weiYunFileDownload.cookieName = result.getString("cookie_name");
weiYunFileDownload.cookieValue = result.getString("cookie_value");
weiYunFileDownload.fileId = result.getString("file_id");
weiYunFileDownload.fileModifiedTime = result.getDate("file_mtime");
weiYunFileDownload.insideDownloadIP = result.getString("inside_download_ip");
weiYunFileDownload.outsideDownloadIP = result.getString("outside_download_ip");
weiYunFileDownload.serverName = result.getString("server_name");
if (null != result.getString("server_port")) {
weiYunFileDownload.serverPort = result.getInteger("server_port");
}
weiYunFileDownload.downloadUrl = result.getString("download_url");
weiYunFileDownload.httpsServerName = result.getString("https_server_name");
if (null != result.getString("https_server_port")) {
weiYunFileDownload.httpsServerPort = result.getInteger("https_server_port");
}
weiYunFileDownload.httpsDownloadUrl = result.getString("https_download_url");
weiYunFileDownload.fileSha = result.getString("file_sha");
weiYunFileDownload.fileVersion = result.getDate("file_version");
weiYunFileDownload.fileSize = result.getLong("file_size");
weiYunFileDownload.thumbUrl = result.getString("thumb_url");
weiYunFileDownload.httpsThumbUrl = result.getString("https_thumb_url");
return weiYunFileDownload;
}
@Override
public WeiYunFileDownload diskFileBatchDownload(List weiYunDirectoryList, List weiYunFileList) throws IOException {
if (null == weiYunDirectoryList && null == weiYunFileList) {
throw new IllegalArgumentException("文件夹列表和文件列表参数不能同时为空!");
}
String zipName = generateFileName(weiYunDirectoryList, weiYunFileList);
if (null == weiYunDirectoryList) {
weiYunDirectoryList = new ArrayList<>();
}
if (null == weiYunFileList) {
weiYunFileList = new ArrayList<>();
}
String api = "https://www.weiyun.com/webapp/json/weiyunQdiskClient/DiskFileBatchDownload?refer=chrome_windows&g_tk=" + gtk + "&r=" + Math.random();
StringBuilder bodyBuilder = new StringBuilder("\\\".weiyun.DiskFilePackageDownloadMsgReq_body\\\":{\\\"pdir_list\\\":[{\\\"pdir_key\\\":\\\"" + weiYunFileList.get(0).pdirKey + "\\\",\\\"dir_list\\\":[");
for (WeiYunDirectory weiYunDirectory : weiYunDirectoryList) {
bodyBuilder.append("{\\\"dir_key\\\":\\\"" + weiYunDirectory.dirKey + "\\\",\\\"dir_name\\\":\\\"" + weiYunDirectory.dirName + "\\\"},");
}
if (weiYunDirectoryList.size() > 0) {
bodyBuilder.deleteCharAt(bodyBuilder.length() - 1);
}
bodyBuilder.append("],\\\"file_list\\\":[");
for (WeiYunFile weiYunFile : weiYunFileList) {
bodyBuilder.append("{\\\"file_id\\\":\\\"" + weiYunFile.fileId + "\\\",\\\"pdir_key\\\":\\\"" + weiYunFile.pdirKey + "\\\"},");
}
if (weiYunFileList.size() > 0) {
bodyBuilder.deleteCharAt(bodyBuilder.length() - 1);
}
bodyBuilder.append("],\\\"zip_filename\\\":\\\"" + zipName + "\\\"}");
String body = getBody(2403, bodyBuilder.toString());
Response response = QuickHttp.connect(api)
.method(Request.Method.POST)
.requestBody(body)
.execute();
checkResponse(response);
JSONObject result = response.bodyAsJSONObject().getJSONObject("data").getJSONObject("rsp_body").getJSONObject("RspMsg_body");
WeiYunFileDownload weiYunFileDownload = new WeiYunFileDownload();
weiYunFileDownload.cookieName = result.getString("cookie_name");
weiYunFileDownload.cookieValue = result.getString("cookie_value");
weiYunFileDownload.serverName = result.getString("server_name");
weiYunFileDownload.serverPort = result.getInteger("server_port");
weiYunFileDownload.downloadUrl = result.getString("download_url");
weiYunFileDownload.httpsServerName = result.getString("https_server_name");
weiYunFileDownload.httpsServerPort = result.getInteger("https_server_port");
weiYunFileDownload.httpsDownloadUrl = result.getString("https_download_url");
return weiYunFileDownload;
}
@Override
public WeiYunShareView weiyunShareView(String url) throws IOException {
String sharePwd = null;
if(url.contains("#")){
sharePwd = "\\\""+url.substring(url.indexOf("#")+1)+"\\\"";
url = url.substring(0,url.indexOf("#"));
}
String shareKey = url.substring(url.lastIndexOf("/") + 1);
String api = "https://share.weiyun.com/webapp/json/weiyunShare" + (skey == null ? "NoLogin" : "") + "/WeiyunShareView?refer=chrome_windows&g_tk=" + (gtk == null ? "854045919" : gtk) + "&r=" + Math.random();
String body = getBody(12002, "\\\".weiyun.WeiyunShareViewMsgReq_body\\\":{\\\"share_pwd\\\":" + sharePwd + ",\\\"share_key\\\":\\\"" + shareKey + "\\\"}");
Request request = QuickHttp.connect(api)
.method(Request.Method.POST)
.requestBody(body);
if (null == skey) {
QuickHttp.clientConfig().cookieOption().addCookie("share.weiyun.com", "wyctoken", "854045919");
}
Response response = request.execute();
checkResponse(response);
JSONObject result = response.bodyAsJSONObject().getJSONObject("data").getJSONObject("rsp_body").getJSONObject("RspMsg_body");
WeiYunShareView weiYunShare = new WeiYunShareView();
weiYunShare.shareUin = result.getString("share_uin");
weiYunShare.shareNickName = result.getString("share_nick_name");
weiYunShare.downCnt = result.getInteger("down_cnt");
weiYunShare.viewCnt = result.getInteger("view_cnt");
weiYunShare.shareName = result.getString("share_name");
weiYunShare.shareKey = result.getString("share_key");
weiYunShare.fileCreatedTime = result.getDate("create_time");
weiYunShare.pdirKey = result.getString("pdir_key");
weiYunShare.weiYunFileList = getWeiYunFileList(result.getJSONArray("file_list"), weiYunShare.pdirKey, null);
weiYunShare.weiYunDirectoryList = getWeiYunDirectoryList(result.getJSONArray("dir_list"), weiYunShare.pdirKey, null);
return weiYunShare;
}
@Override
public WeiYunDirectory weiyunShareDirList(WeiYunShareView weiYunShareView, WeiYunDirectory weiYunDirectory) throws IOException {
String api = "https://share.weiyun.com/webapp/json/weiyunShare" + (skey == null ? "NoLogin" : "") + "/WeiyunShareDirList?refer=chrome_windows&g_tk=" + (gtk == null ? "854045919" : gtk) + "&r=" + Math.random();
String body = getBody(12031, "\\\".weiyun.WeiyunShareDirListMsgReq_body\\\":{\\\"share_key\\\":\\\"" + weiYunShareView.shareKey + "\\\",\\\"share_pwd\\\":\\\"\\\",\\\"dir_key\\\":\\\"" + weiYunDirectory.dirKey + "\\\",\\\"dir_name\\\":\\\"" + weiYunDirectory.dirName + "\\\",\\\"get_type\\\":0,\\\"start\\\":0,\\\"count\\\":100,\\\"get_abstract_url\\\":true}");
Request request = QuickHttp.connect(api)
.method(Request.Method.POST)
.requestBody(body);
if (null == skey) {
QuickHttp.clientConfig().cookieOption().addCookie("share.weiyun.com", "wyctoken", "854045919");
}
Response response = request.execute();
checkResponse(response);
JSONObject result = response.bodyAsJSONObject().getJSONObject("data").getJSONObject("rsp_body").getJSONObject("RspMsg_body");
WeiYunDirectory weiYunDirectory1 = new WeiYunDirectory();
JSONArray dirList = result.getJSONArray("dir_list");
weiYunDirectory1.weiYunDirectoryList = getWeiYunDirectoryList(dirList, weiYunDirectory.pdirKey, weiYunDirectory.ppdirKey);
JSONArray fileList = result.getJSONArray("file_list");
weiYunDirectory1.weiYunFileList = getWeiYunFileList(fileList, weiYunDirectory.pdirKey, weiYunDirectory.ppdirKey);
return weiYunDirectory1;
}
@Override
public WeiYunShareSave weiyunSharePartSaveData(WeiYunShareView weiYunShareView, WeiYunDirectory weiYunDirectory) throws IOException {
String api = "https://share.weiyun.com/webapp/json/weiyunShare/WeiyunSharePartSaveData?refer=chrome_windows&g_tk=" + gtk + "&r=" + Math.random();
StringBuilder bodyBuilder = new StringBuilder("\\\".weiyun.WeiyunSharePartSaveDataMsgReq_body\\\":{\\\"os_info\\\":\\\"windows\\\",\\\"browser\\\":\\\"chrome\\\",\\\"share_key\\\":\\\"" + weiYunShareView.shareKey + "\\\",\\\"pwd\\\":\\\"\\\",");
bodyBuilder.append("\\\"dst_ppdir_key\\\":\\\"" + weiYunDirectory.pdirKey + "\\\",\\\"dst_pdir_key\\\":\\\"" + weiYunDirectory.dirKey + "\\\",\\\"src_pdir_key\\\":\\\"\\\",\\\"dir_list\\\":[],\\\"note_list\\\":[],");
bodyBuilder.append("\\\"file_list\\\":[");
for (WeiYunFile weiYunFile : weiYunShareView.weiYunFileList) {
bodyBuilder.append("{\\\"pdir_key\\\":\\\"" + weiYunFile.pdirKey + "\\\",\\\"file_id\\\":\\\"" + weiYunFile.fileId + "\\\",\\\"filename\\\":\\\"" + weiYunFile.fileName + "\\\",\\\"file_size\\\":" + weiYunFile.fileSize + "},");
}
bodyBuilder.deleteCharAt(bodyBuilder.length() - 1);
bodyBuilder.append("],\\\"is_save_all\\\":true}");
String body = getBody(12025, bodyBuilder.toString());
Response response = QuickHttp.connect(api)
.method(Request.Method.POST)
.requestBody(body)
.execute();
checkResponse(response);
JSONObject result = response.bodyAsJSONObject().getJSONObject("data").getJSONObject("rsp_body").getJSONObject("RspMsg_body");
WeiYunShareSave weiYunShareSave = new WeiYunShareSave();
weiYunShareSave.downloadCountInfo = result.getString("download_count_info");
weiYunShareSave.saveSessionKey = result.getString("save_session_key\n");
return weiYunShareSave;
}
@Override
public List weiyunShareList() throws IOException {
String api = "https://www.weiyun.com/webapp/json/weiyunShare/WeiyunShareList?refer=chrome_windows&g_tk=" + gtk + "&r=" + Math.random();
String body = getBody(12008, "\\\".weiyun.WeiyunShareListMsgReq_body\\\":{\\\"offset\\\":0,\\\"size\\\":20,\\\"order\\\":0}");
Response response = QuickHttp.connect(api)
.method(Request.Method.POST)
.requestBody(body)
.execute();
checkResponse(response);
JSONArray array = response.bodyAsJSONObject().getJSONObject("data").getJSONObject("rsp_body").getJSONObject("RspMsg_body").getJSONArray("item");
List weiYunShareAddList = new ArrayList<>();
for (int i = 0; i < array.size(); i++) {
weiYunShareAddList.add(getWeiYunShareAdd(array.getJSONObject(i)));
}
return weiYunShareAddList;
}
@Override
public WeiYunShare weiyunShareAddV2(List weiYunDirectoryList, List weiYunFileList) throws IOException {
if (null == weiYunDirectoryList && null == weiYunFileList) {
throw new IllegalArgumentException("文件夹列表和文件列表参数不能同时为空!");
}
String zipName = generateFileName(weiYunDirectoryList, weiYunFileList);
if (null == weiYunDirectoryList) {
weiYunDirectoryList = new ArrayList<>();
}
if (null == weiYunFileList) {
weiYunFileList = new ArrayList<>();
}
String api = "https://www.weiyun.com/webapp/json/weiyunShare/WeiyunShareAddV2?refer=chrome_windows&g_tk=" + gtk + "&r=" + Math.random();
StringBuilder bodyBuilder = new StringBuilder("\\\".weiyun.WeiyunShareAddV2MsgReq_body\\\":{\\\"note_list\\\":[],\\\"dir_list\\\":[");
for (WeiYunDirectory weiYunDirectory : weiYunDirectoryList) {
bodyBuilder.append("{\\\"pdir_key\\\":\\\"" + weiYunDirectory.pdirKey + "\\\",\\\"dir_key\\\":\\\"" + weiYunDirectory.dirKey + "\\\"},");
}
if (weiYunDirectoryList.size() > 0) {
bodyBuilder.deleteCharAt(bodyBuilder.length() - 1);
}
bodyBuilder.append("],\\\"file_list\\\":[");
for (WeiYunFile weiYunFile : weiYunFileList) {
bodyBuilder.append("{\\\"pdir_key\\\":\\\"" + weiYunFile.pdirKey + "\\\",\\\"file_id\\\":\\\"" + weiYunFile.fileId + "\\\"},");
}
if (weiYunFileList.size() > 0) {
bodyBuilder.deleteCharAt(bodyBuilder.length() - 1);
}
bodyBuilder.append("],\\\"share_type\\\":0,\\\"share_name\\\":\\\"" + zipName + "\\\"}");
String body = getBody(12100, bodyBuilder.toString());
Response response = QuickHttp.connect(api)
.method(Request.Method.POST)
.requestBody(body)
.execute();
checkResponse(response);
JSONObject result = response.bodyAsJSONObject().getJSONObject("data").getJSONObject("rsp_body").getJSONObject("RspMsg_body");
return getWeiYunShareAdd(result);
}
@Override
public void weiyunSharePwdCreate(WeiYunShare weiYunShare, String pwd) throws IOException {
if (null == weiYunShare) {
throw new IllegalArgumentException("分享文件不能为空!");
}
if (!pwd.matches("[A-Z|a-z|0-9]{6}")) {
throw new IllegalArgumentException("密码必须为6位英文数字的组合!!");
}
String api = "https://www.weiyun.com/webapp/json/weiyunShare/WeiyunSharePwdCreate?refer=chrome_windows&g_tk=" + gtk + "&r=" + Math.random();
String body = getBody(12012, "\\\".weiyun.WeiyunSharePwdCreateMsgReq_body\\\":{\\\"share_key\\\":\\\"" + weiYunShare.shareKey + "\\\",\\\"share_pwd\\\":\\\"" + pwd + "\\\"}");
Response response = QuickHttp.connect(api)
.method(Request.Method.POST)
.requestBody(body)
.execute();
checkResponse(response);
}
@Override
public void weiyunSharePwdModify(WeiYunShare weiYunShare, String pwd) throws IOException {
if (null == weiYunShare) {
throw new IllegalArgumentException("分享文件不能为空!");
}
if (!pwd.matches("[A-Z|a-z|0-9]{6}")) {
throw new IllegalArgumentException("密码必须为6位英文数字的组合!!");
}
String api = "https://www.weiyun.com/webapp/json/weiyunShare/WeiyunSharePwdModify?refer=chrome_windows&g_tk=" + gtk + "&r=" + Math.random();
String body = getBody(12013, "\\\".weiyun.WeiyunSharePwdModifyMsgReq_body\\\":{\\\"share_key\\\":\\\"" + weiYunShare.shareKey + "\\\",\\\"share_new_pwd\\\":\\\"" + pwd + "\\\"}");
Response response = QuickHttp.connect(api)
.method(Request.Method.POST)
.requestBody(body)
.execute();
checkResponse(response);
}
@Override
public List weiyunShareDelete(List weiYunShareAddList) throws IOException {
if (null == weiYunShareAddList) {
throw new IllegalArgumentException("待删除分享列表不能为空!");
}
String api = "https://www.weiyun.com/webapp/json/weiyunShare/WeiyunShareDelete?refer=chrome_windows&g_tk=" + gtk + "&r=" + Math.random();
StringBuilder bodyBuilder = new StringBuilder("\\\".weiyun.WeiyunShareDeleteMsgReq_body\\\":{\\\"share_key_list\\\":[");
for (WeiYunShare weiYunShareAdd : weiYunShareAddList) {
bodyBuilder.append("\\\"" + weiYunShareAdd.shareKey + "\\\",");
}
if (weiYunShareAddList.size() > 0) {
bodyBuilder.deleteCharAt(bodyBuilder.length() - 1);
}
bodyBuilder.append("]}");
String body = getBody(12007, bodyBuilder.toString());
Response response = QuickHttp.connect(api)
.method(Request.Method.POST)
.requestBody(body)
.execute();
checkResponse(response);
JSONArray array = response.bodyAsJSONObject().getJSONObject("data").getJSONObject("rsp_body").getJSONObject("RspMsg_body").getJSONArray("ret_item_list");
List weiYunShareDeleteList = new ArrayList<>();
for (int i = 0; i < array.size(); i++) {
JSONObject o = array.getJSONObject(i);
WeiYunShareDelete weiYunShareDelete = new WeiYunShareDelete();
weiYunShareDelete.ret = o.getInteger("ret");
weiYunShareDelete.shareKey = o.getString("share_key");
weiYunShareDeleteList.add(weiYunShareDelete);
}
return weiYunShareDeleteList;
}
@Override
public WeiYunDirectory fileSearchbyKeyWord(String keyword) throws IOException {
String api = "https://www.weiyun.com/webapp/json/weiyunFileSearch/FileSearchbyKeyWord?refer=chrome_windows&g_tk=" + gtk + "&r=" + Math.random();
String body = getBody(247251, "\\\".weiyun.FileSearchbyKeyWordMsgReq_body\\\":{\\\"type\\\":0,\\\"key_word\\\":\\\"" + keyword + "\\\",\\\"local_context\\\":\\\"\\\",\\\"location\\\":0}");
Response response = QuickHttp.connect(api)
.method(Request.Method.POST)
.requestBody(body)
.execute();
checkResponse(response);
JSONArray array = response.bodyAsJSONObject().getJSONObject("data").getJSONObject("rsp_body").getJSONObject("RspMsg_body").getJSONArray("search_result_list");
WeiYunDirectory weiYunDirectory = new WeiYunDirectory();
for (int i = 0; i < array.size(); i++) {
JSONObject o = array.getJSONObject(i);
if (o.containsKey("type") && o.getInteger("type") == 2) {
JSONObject dirItem = o.getJSONObject("dir_item");
WeiYunDirectory subWeiYunDirectory = new WeiYunDirectory();
subWeiYunDirectory.dirCreatedTime = dirItem.getDate("dir_ctime");
subWeiYunDirectory.dirKey = dirItem.getString("dir_key");
subWeiYunDirectory.dirName = dirItem.getString("dir_name");
subWeiYunDirectory.pdirKey = dirItem.getString("pdir_key");
weiYunDirectory.weiYunDirectoryList.add(subWeiYunDirectory);
} else if (o.containsKey("type") && o.getInteger("type") == 1) {
JSONObject fileItem = o.getJSONObject("file_item");
WeiYunFile subWeiYunFile = new WeiYunFile();
subWeiYunFile.fileCreatedTime = fileItem.getDate("file_ctime");
subWeiYunFile.fileId = fileItem.getString("file_id");
subWeiYunFile.fileSha = fileItem.getString("file_sha");
subWeiYunFile.fileSize = fileItem.getLong("file_size");
subWeiYunFile.fileName = fileItem.getString("filename");
subWeiYunFile.pdirKey = fileItem.getString("pdir_key");
weiYunDirectory.weiYunFileList.add(subWeiYunFile);
}
}
return weiYunDirectory;
}
@Override
public WeiYunDirectory diskRecycleList() throws IOException {
String api = "https://www.weiyun.com/webapp/json/weiyunQdiskClient/DiskRecycleList?refer=chrome_windows&g_tk=" + gtk + "&r=" + Math.random();
String body = getBody(2702, "\\\".weiyun.DiskRecycleListMsgReq_body\\\":{\\\"start\\\":0,\\\"count\\\":100,\\\"sort_field\\\":2,\\\"reverse_order\\\":false,\\\"get_abstract_url\\\":true}");
Response response = QuickHttp.connect(api)
.method(Request.Method.POST)
.requestBody(body)
.execute();
checkResponse(response);
JSONObject result = response.bodyAsJSONObject().getJSONObject("data").getJSONObject("rsp_body").getJSONObject("RspMsg_body");
WeiYunDirectory weiYunDirectory = new WeiYunDirectory();
weiYunDirectory.weiYunFileList = getWeiYunFileList(result.getJSONArray("file_list"), null, null);
weiYunDirectory.weiYunDirectoryList = getWeiYunDirectoryList(result.getJSONArray("dir_list"), null, null);
return weiYunDirectory;
}
@Override
public WeiYunDirectoryDelete diskRecycleDirFileBatchRestore(List weiYunDirectoryList, List weiYunFileList) throws IOException {
if (null == weiYunDirectoryList) {
weiYunDirectoryList = new ArrayList<>();
}
if (null == weiYunFileList) {
weiYunFileList = new ArrayList<>();
}
String api = "https://www.weiyun.com/webapp/json/weiyunQdiskClient/DiskRecycleDirFileBatchRestore?refer=chrome_windows&g_tk=" + gtk + "&r=" + Math.random();
StringBuilder bodyBuilder = new StringBuilder("\\\".weiyun.DiskRecycleDirFileBatchRestoreMsgReq_body\\\":{\\\"file_list\\\":[");
for (WeiYunFile weiYunFile : weiYunFileList) {
bodyBuilder.append("{\\\"recycle_file_id\\\":\\\"" + weiYunFile.fileId + "\\\",\\\"recycle_filename\\\":\\\"" + weiYunFile.fileName + "\\\"},");
}
if (weiYunFileList.size() > 0) {
bodyBuilder.deleteCharAt(bodyBuilder.length() - 1);
}
bodyBuilder.append("],\\\"dir_list\\\":[");
for (WeiYunDirectory weiYunDirectory : weiYunDirectoryList) {
bodyBuilder.append("{\\\"recycle_dir_key\\\":\\\"" + weiYunDirectory.dirKey + "\\\",\\\"recycle_dir_name\\\":\\\"" + weiYunDirectory.dirName + "\\\"},");
}
if (weiYunDirectoryList.size() > 0) {
bodyBuilder.deleteCharAt(bodyBuilder.length() - 1);
}
bodyBuilder.append("]}");
String body = getBody(2708, bodyBuilder.toString());
Response response = QuickHttp.connect(api)
.method(Request.Method.POST)
.requestBody(body)
.execute();
checkResponse(response);
return getWeiYunDirectoryDelete(response);
}
@Override
public void diskRecycleClear() throws IOException {
String api = "https://www.weiyun.com/webapp/json/weiyunQdiskClient/DiskRecycleClear?refer=chrome_windows&g_tk=" + gtk + "&r=" + Math.random();
String body = getBody(2703, "\\\".weiyun.DiskRecycleClearMsgReq_body\\\":{}");
Response response = QuickHttp.connect(api)
.method(Request.Method.POST)
.requestBody(body)
.execute();
checkResponse(response);
}
@Override
public WeiYunDirectoryDelete diskRecycleDirFileClear(List weiYunDirectoryList, List weiYunFileList) throws IOException {
if (null == weiYunDirectoryList) {
weiYunDirectoryList = new ArrayList<>();
}
if (null == weiYunFileList) {
weiYunFileList = new ArrayList<>();
}
String api = "https://www.weiyun.com/webapp/json/weiyunQdiskClient/DiskRecycleDirFileClear?refer=chrome_windows&g_tk=" + gtk + "&r=" + Math.random();
StringBuilder bodyBuilder = new StringBuilder("\\\".weiyun.DiskRecycleDirFileClearMsgReq_body\\\":{\\\"file_list\\\":[");
for (WeiYunFile weiYunFile : weiYunFileList) {
bodyBuilder.append("{\\\"file_id\\\":\\\"" + weiYunFile.fileId + "\\\",\\\"filename\\\":\\\"" + weiYunFile.fileName + "\\\"},");
}
if (weiYunFileList.size() > 0) {
bodyBuilder.deleteCharAt(bodyBuilder.length() - 1);
}
bodyBuilder.append("],\\\"dir_list\\\":[");
for (WeiYunDirectory weiYunDirectory : weiYunDirectoryList) {
bodyBuilder.append("{\\\"dir_key\\\":\\\"" + weiYunDirectory.dirKey + "\\\",\\\"dir_name\\\":\\\"" + weiYunDirectory.dirName + "\\\"},");
}
if (weiYunDirectoryList.size() > 0) {
bodyBuilder.deleteCharAt(bodyBuilder.length() - 1);
}
bodyBuilder.append("]}");
String body = getBody(2710, bodyBuilder.toString());
Response response = QuickHttp.connect(api)
.method(Request.Method.POST)
.requestBody(body)
.execute();
checkResponse(response);
return getWeiYunDirectoryDelete(response);
}
private WeiYunDirectory diskDirBatchList(String pdirKey, String dirKey) throws IOException {
String api = "https://www.weiyun.com/webapp/json/weiyunQdisk/DiskDirBatchList?refer=chrome_windows&g_tk=" + gtk + "&r=" + Math.random();
String body = getBody(2209, "\\\".weiyun.DiskDirBatchListMsgReq_body\\\":{\\\"pdir_key\\\":\\\"" + pdirKey + "\\\",\\\"dir_list\\\":[{\\\"dir_key\\\":\\\"" + dirKey + "\\\",\\\"get_type\\\":0,\\\"start\\\":0,\\\"count\\\":100,\\\"sort_field\\\":2,\\\"reverse_order\\\":false,\\\"get_abstract_url\\\":true,\\\"get_dir_detail_info\\\":true}]}");
Response response = QuickHttp.connect(api)
.method(Request.Method.POST)
.requestBody(body)
.execute();
checkResponse(response);
JSONObject result = response.bodyAsJSONObject().getJSONObject("data").getJSONObject("rsp_body").getJSONObject("RspMsg_body").getJSONArray("dir_list").getJSONObject(0);
WeiYunDirectory weiYunDirectory = new WeiYunDirectory();
weiYunDirectory.pdirKey = result.getString("pdir_key");
weiYunDirectory.weiYunFileList = getWeiYunFileList(result.getJSONArray("file_list"), dirKey, pdirKey);
weiYunDirectory.weiYunDirectoryList = getWeiYunDirectoryList(result.getJSONArray("dir_list"), dirKey, pdirKey);
return weiYunDirectory;
}
private WeiYunShare getWeiYunShareAdd(JSONObject result) {
WeiYunShare weiYunShareAdd = new WeiYunShare();
weiYunShareAdd.rawUrl = result.getString("raw_url");
weiYunShareAdd.shortUrl = result.getString("short_url");
weiYunShareAdd.shareName = result.getString("share_name");
weiYunShareAdd.sharePwd = result.getString("share_pwd");
weiYunShareAdd.shareKey = result.getString("share_key");
weiYunShareAdd.thumbUrl = result.getString("thumb_url");
return weiYunShareAdd;
}
private WeiYunDirectoryDelete getWeiYunDirectoryDelete(Response response) throws IOException {
JSONObject result = response.bodyAsJSONObject().getJSONObject("data").getJSONObject("rsp_body").getJSONObject("RspMsg_body");
WeiYunDirectoryDelete weiYunDirectoryDelete = new WeiYunDirectoryDelete();
if (result.containsKey("dir_list")) {
JSONArray array = result.getJSONArray("dir_list");
for (int i = 0; i < array.size(); i++) {
JSONObject o = array.getJSONObject(i);
WeiYunDirectoryDelete subWeiYunDirectoryDelete = new WeiYunDirectoryDelete();
subWeiYunDirectoryDelete.retcode = o.getInteger("retcode");
subWeiYunDirectoryDelete.retmsg = o.getString("retmsg");
subWeiYunDirectoryDelete.recycleDirKey = o.getString("recycle_dir_key");
subWeiYunDirectoryDelete.restoreDirName = o.getString("restore_dir_name");
weiYunDirectoryDelete.weiYunDirectoryDeleteList.add(subWeiYunDirectoryDelete);
}
}
if (result.containsKey("file_list")) {
JSONArray array = result.getJSONArray("file_list");
for (int i = 0; i < array.size(); i++) {
JSONObject o = array.getJSONObject(i);
WeiYunFileDelete weiYunFileDelete = new WeiYunFileDelete();
weiYunFileDelete.retcode = o.getInteger("retcode");
weiYunFileDelete.retmsg = o.getString("retmsg");
weiYunFileDelete.recycleFileId = o.getString("recycle_file_id");
weiYunFileDelete.restoreFilename = o.getString("restore_filename");
weiYunDirectoryDelete.weiYunFileDeleteList.add(weiYunFileDelete);
}
}
return weiYunDirectoryDelete;
}
private List getWeiYunDirectoryList(JSONArray directoryList, String pdirKey, String ppdirKey) {
List weiYunDirectoryList = new ArrayList<>();
for (int i = 0; i < directoryList.size(); i++) {
JSONObject o = directoryList.getJSONObject(i);
WeiYunDirectory subWeiYunDirectory = new WeiYunDirectory();
subWeiYunDirectory.dirKey = o.getString("dir_key");
subWeiYunDirectory.dirName = o.getString("dir_name");
subWeiYunDirectory.dirCreatedTime = o.getDate("dir_ctime");
subWeiYunDirectory.pdirKey = o.getString("pdir_key");
if (o.containsKey("space")) {
subWeiYunDirectory.space = o.getInteger("space");
}
if (null == subWeiYunDirectory.pdirKey) {
subWeiYunDirectory.pdirKey = pdirKey;
}
subWeiYunDirectory.ppdirKey = o.getString("ppdir_key");
if (null == subWeiYunDirectory.ppdirKey) {
subWeiYunDirectory.ppdirKey = ppdirKey;
}
weiYunDirectoryList.add(subWeiYunDirectory);
}
return weiYunDirectoryList;
}
private List getWeiYunFileList(JSONArray fileList, String pdirKey, String ppdirKey) {
List weiYunFileList = new ArrayList<>();
for (int i = 0; i < fileList.size(); i++) {
JSONObject o = fileList.getJSONObject(i);
WeiYunFile weiYunFile = new WeiYunFile();
weiYunFile.fileId = o.getString("file_id");
weiYunFile.fileName = o.getString("filename");
if (null == weiYunFile.fileName) {
weiYunFile.fileName = o.getString("file_name");
}
if (null != weiYunFile.fileName) {
if (weiYunFile.fileName.lastIndexOf(".") > 0) {
weiYunFile.fileNameSuffix = weiYunFile.fileName.substring(weiYunFile.fileName.lastIndexOf(".") + 1);
}
}
if (o.containsKey("file_size")) {
weiYunFile.fileSize = o.getLong("file_size");
}
weiYunFile.fileCreatedTime = o.getDate("file_ctime");
if (null == weiYunFile.fileCreatedTime) {
weiYunFile.fileCreatedTime = o.getDate("file_mtime");
}
weiYunFile.pdirKey = o.getString("pdir_key");
if (null == weiYunFile.pdirKey) {
weiYunFile.pdirKey = pdirKey;
}
weiYunFile.ppdirKey = o.getString("ppdir_key");
if (null == weiYunFile.ppdirKey) {
weiYunFile.ppdirKey = ppdirKey;
}
weiYunFileList.add(weiYunFile);
}
return weiYunFileList;
}
/**
* 检查请求是否成功
*/
private void checkResponse(Response response) throws IOException {
JSONObject result = response.bodyAsJSONObject();
if (result.containsKey("ret") && 0 != result.getInteger("ret")) {
throw new WeiYunException(result.getInteger("ret"), result.getString("msg"));
}
JSONObject resHeader = result.getJSONObject("data").getJSONObject("rsp_header");
if (0 == resHeader.getInteger("retcode")) {
return;
}
throw new WeiYunException(resHeader.getInteger("retcode"), resHeader.getString("retmsg"));
}
/**
* 获取请求体
*/
private String getBody(int cmd, String condition) {
return "{\"req_header\":\"{\\\"seq\\\":15677596144092318,\\\"type\\\":1,\\\"cmd\\\":" + cmd + ",\\\"appid\\\":30013,\\\"version\\\":3,\\\"major_version\\\":3,\\\"minor_version\\\":3,\\\"fix_version\\\":3,\\\"wx_openid\\\":\\\"\\\",\\\"user_flag\\\":0}\",\"req_body\":\"{\\\"ReqMsg_body\\\":{\\\"ext_req_head\\\":{\\\"token_info\\\":{\\\"token_type\\\":0,\\\"login_key_type\\\":1,\\\"login_key_value\\\":\\\"" + (skey == null ? "" : skey) + "\\\"},\\\"language_info\\\":{\\\"language_type\\\":2052}}," + condition + "}}\"}";
}
/**
* 生成文件名
*/
private String generateFileName(List weiYunDirectoryList, List weiYunFileList) throws UnsupportedEncodingException {
String zipName = null;
if (null == weiYunDirectoryList) {
String firstFileName = weiYunFileList.get(0).fileName;
zipName = weiYunFileList.size() == 1 ? firstFileName : firstFileName + URLEncoder.encode("等" + weiYunFileList.size() + "个文件", "utf-8");
}
if (null == weiYunFileList) {
String firstDirName = weiYunDirectoryList.get(0).dirName;
zipName = weiYunDirectoryList.size() == 1 ? firstDirName : firstDirName + URLEncoder.encode("等" + weiYunDirectoryList.size() + "个文件", "utf-8");
}
if (null == zipName) {
zipName = weiYunFileList.get(0).fileName + URLEncoder.encode("等" + (weiYunDirectoryList.size() + weiYunFileList.size()) + "个文件", "utf-8");
}
return zipName;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy