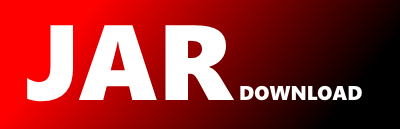
cn.schoolwow.workflow.util.ResourceUtil Maven / Gradle / Ivy
package cn.schoolwow.workflow.util;
import java.io.File;
import java.io.FileInputStream;
import java.io.IOException;
import java.io.InputStream;
import java.net.JarURLConnection;
import java.net.URL;
import java.nio.file.*;
import java.nio.file.attribute.BasicFileAttributes;
import java.util.jar.JarFile;
public class ResourceUtil {
public static void deleteFile(String directoryPath) throws IOException {
Files.walkFileTree(Paths.get(directoryPath), new FileVisitor(){
@Override
public FileVisitResult preVisitDirectory(Path dir, BasicFileAttributes attrs) throws IOException {
return null;
}
@Override
public FileVisitResult visitFile(Path file, BasicFileAttributes attrs) throws IOException {
Files.deleteIfExists(file);
return FileVisitResult.CONTINUE;
}
@Override
public FileVisitResult visitFileFailed(Path file, IOException exc) throws IOException {
return null;
}
@Override
public FileVisitResult postVisitDirectory(Path dir, IOException exc) throws IOException {
Files.deleteIfExists(dir);
return FileVisitResult.CONTINUE;
}
});
}
/**获取指定资源*/
public static InputStream getInputStreamByPath(String path) {
ClassLoader classLoader = Thread.currentThread().getContextClassLoader();
URL url = classLoader.getResource(path);
if (url == null) {
throw new IllegalArgumentException("路径不存在!路径:"+path);
}
try {
switch (url.getProtocol()) {
case "file": {
File file = new File(url.getFile());
FileInputStream fis = new FileInputStream(file);
return fis;
}
case "jar": {
JarURLConnection jarURLConnection = (JarURLConnection) url.openConnection();
JarFile jarFile = jarURLConnection.getJarFile();
InputStream inputStream = jarFile.getInputStream(jarFile.getJarEntry(path));
return inputStream;
}
}
}catch (IOException e){
throw new RuntimeException(e);
}
throw new IllegalArgumentException("不支持的路径!"+path);
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy