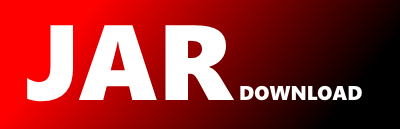
cn.sliew.carp.module.application.vela.api.v1.ApplicationApi Maven / Gradle / Ivy
/*
* Licensed to the Apache Software Foundation (ASF) under one
* or more contributor license agreements. See the NOTICE file
* distributed with this work for additional information
* regarding copyright ownership. The ASF licenses this file
* to you under the Apache License, Version 2.0 (the
* "License"); you may not use this file except in compliance
* with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package cn.sliew.carp.module.application.vela.api.v1;
import cn.sliew.carp.module.application.vela.api.v1.model.v1.*;
import org.springframework.http.ResponseEntity;
import org.springframework.web.bind.annotation.*;
public interface ApplicationApi {
@GetMapping(value = "/api/v1/applications")
ResponseEntity listApplications(@RequestParam(value = "query", required = false) String query, @RequestParam(value = "project", required = false) String project, @RequestParam(value = "env", required = false) String env, @RequestParam(value = "targetName", required = false) String targetName);
@GetMapping(value = "/api/v1/applications/{appName}")
ResponseEntity detailApplication(@PathVariable("appName") String appName);
@GetMapping(value = "/api/v1/applications/{appName}/revisions")
ResponseEntity listApplicationRevisions(@PathVariable("appName") String appName, @RequestParam(value = "envName", required = false) String envName, @RequestParam(value = "status", required = false) String status, @RequestParam(value = "page", required = false) Integer page, @RequestParam(value = "pageSize", required = false) Integer pageSize);
@GetMapping(value = "/api/v1/applications/{appName}/revisions/{revision}")
ResponseEntity detailApplicationRevision(@PathVariable("appName") String appName, @PathVariable("revision") String revision);
@PostMapping(value = "/api/v1/applications/{appName}/revisions/{revision}/rollback")
ResponseEntity rollbackApplicationWithRevision(@PathVariable("appName") String appName, @PathVariable("revision") String revision);
@PostMapping(value = "/api/v1/applications/{appName}/reset")
ResponseEntity resetAppToLatestRevision(@PathVariable("appName") String appName);
@PostMapping(value = "/api/v1/applications/{appName}/compare")
ResponseEntity compareApp(@RequestBody V1AppCompareReq body, @PathVariable("appName") String appName);
@PostMapping(value = "/api/v1/applications")
ResponseEntity createApplication(@RequestBody V1CreateApplicationRequest body);
@PutMapping(value = "/api/v1/applications/{appName}")
ResponseEntity updateApplication(@PathVariable("appName") String appName, @RequestBody V1UpdateApplicationRequest body);
@DeleteMapping(value = "/api/v1/applications/{appName}")
ResponseEntity deleteApplication(@PathVariable("appName") String appName);
@PostMapping(value = "/api/v1/applications/{appName}/dry-run")
ResponseEntity dryRunAppOrRevision(@RequestBody V1AppDryRunReq body, @PathVariable("appName") String appName);
@PostMapping(value = "/api/v1/applications/{appName}/deploy")
ResponseEntity deployApplication(@PathVariable("appName") String appName, @RequestBody V1ApplicationDeployRequest body);
@GetMapping(value = "/api/v1/applications/{appName}/status")
ResponseEntity getApplicationStatusFromAllEnvs(@PathVariable("appName") String appName);
@GetMapping(value = "/api/v1/applications/{appName}/envs/{envName}/status")
ResponseEntity getApplicationStatus(@PathVariable("appName") String appName, @PathVariable("envName") String envName);
@GetMapping(value = "/api/v1/applications/{appName}/records")
ResponseEntity listApplicationRecords(@PathVariable("appName") String appName);
@GetMapping(value = "/api/v1/applications/{appName}/statistics")
ResponseEntity applicationStatistics(@PathVariable("appName") String appName);
@PostMapping(value = "/api/v1/applications/{appName}/template")
ResponseEntity publishApplicationTemplate(@PathVariable("appName") String appName, @RequestBody V1CreateApplicationTemplateRequest body);
@GetMapping(value = "/api/v1/applications/{appName}/envs")
ResponseEntity listApplicationEnvs(@PathVariable("appName") String appName);
@PostMapping(value = "/api/v1/applications/{appName}/envs")
ResponseEntity createApplicationEnv(@PathVariable("appName") String appName, @RequestBody V1CreateApplicationEnvbindingRequest body);
@PutMapping(value = "/api/v1/applications/{appName}/envs/{envName}")
ResponseEntity updateApplicationEnv(@PathVariable("appName") String appName, @PathVariable("envName") String envName, @RequestBody V1PutApplicationEnvBindingRequest body);
@DeleteMapping(value = "/api/v1/applications/{appName}/envs/{envName}")
ResponseEntity deleteApplicationEnv(@PathVariable("appName") String appName, @PathVariable("envName") String envName);
@PostMapping(value = "/api/v1/applications/{appName}/envs/{envName}/recycle")
ResponseEntity recycleApplicationEnv(@PathVariable("appName") String appName, @PathVariable("envName") String envName);
@PostMapping(value = "/api/v1/applications/{appName}/components/{compName}/traits")
ResponseEntity addApplicationTrait(@PathVariable("appName") String appName, @PathVariable("compName") String compName, @RequestBody V1CreateApplicationTraitRequest body);
@PutMapping(value = "/api/v1/applications/{appName}/components/{compName}/traits/{traitType}")
ResponseEntity updateApplicationTrait(@PathVariable("appName") String appName, @PathVariable("compName") String compName, @PathVariable("traitType") String traitType, @RequestBody V1UpdateApplicationTraitRequest body);
@DeleteMapping(value = "/api/v1/applications/{appName}/components/{compName}/traits/{traitType}")
ResponseEntity deleteApplicationTrait(@PathVariable("appName") String appName, @PathVariable("compName") String compName, @PathVariable("traitType") String traitType);
@GetMapping(value = "/api/v1/applications/{appName}/policies")
ResponseEntity listApplicationPolicies(@PathVariable("appName") String appName);
@GetMapping(value = "/api/v1/applications/{appName}/policies/{policyName}")
ResponseEntity detailApplicationPolicy(@PathVariable("appName") String appName, @PathVariable("policyName") String policyName);
@PostMapping(value = "/api/v1/applications/{appName}/policies")
ResponseEntity createApplicationPolicy(@PathVariable("appName") String appName, @RequestBody V1CreatePolicyRequest body);
@PutMapping(value = "/api/v1/applications/{appName}/policies/{policyName}")
ResponseEntity updateApplicationPolicy(@PathVariable("appName") String appName, @PathVariable("policyName") String policyName, @RequestBody V1UpdatePolicyRequest body);
@DeleteMapping(value = "/api/v1/applications/{appName}/policies/{policyName}")
ResponseEntity deleteApplicationPolicy(@PathVariable("appName") String appName, @PathVariable("policyName") String policyName, @RequestParam(value = "force", required = false) Boolean force);
@GetMapping(value = "/api/v1/applications/{appName}/triggers")
ResponseEntity listApplicationTriggers(@PathVariable("appName") String appName);
@PostMapping(value = "/api/v1/applications/{appName}/triggers")
ResponseEntity createApplicationTrigger(@PathVariable("appName") String appName, @RequestBody V1CreateApplicationTriggerRequest body);
@PutMapping(value = "/api/v1/applications/{appName}/triggers/{token}")
ResponseEntity updateApplicationTrigger(@PathVariable("appName") String appName, @PathVariable("token") String token);
@DeleteMapping(value = "/api/v1/applications/{appName}/triggers/{token}")
ResponseEntity deleteApplicationTrigger(@PathVariable("appName") String appName, @PathVariable("token") String token);
@GetMapping(value = "/api/v1/applications/{appName}/components")
ResponseEntity listApplicationComponents(@PathVariable("appName") String appName, @RequestParam(value = "envName", required = false) String envName);
@GetMapping(value = "/api/v1/applications/{appName}/components/{compName}")
ResponseEntity detailComponent(@PathVariable("appName") String appName, @PathVariable("compName") String compName);
@PostMapping(value = "/api/v1/applications/{appName}/components")
ResponseEntity createComponent(@PathVariable("appName") String appName, @RequestBody V1CreateComponentRequest body);
@PutMapping(value = "/api/v1/applications/{appName}/components/{compName}")
ResponseEntity updateComponent(@PathVariable("appName") String appName, @PathVariable("compName") String compName, @RequestBody V1UpdateApplicationComponentRequest body);
@DeleteMapping(value = "/api/v1/applications/{appName}/components/{compName}")
ResponseEntity deleteComponent(@PathVariable("appName") String appName, @PathVariable("compName") String compName);
@GetMapping(value = "/api/v1/applications/{appName}/workflows")
ResponseEntity listApplicationWorkflows(@PathVariable("appName") String appName);
@GetMapping(value = "/api/v1/applications/{appName}/workflows/{workflowName}")
ResponseEntity detailWorkflow(@PathVariable("appName") String appName, @PathVariable("workflowName") String workflowName);
@PostMapping(value = "/api/v1/applications/{appName}/workflows")
ResponseEntity createOrUpdateApplicationWorkflow(@RequestBody V1CreateWorkflowRequest body, @PathVariable("appName") String appName);
@PutMapping(value = "/api/v1/applications/{appName}/workflows/{workflowName}")
ResponseEntity updateWorkflow(@PathVariable("appName") String appName, @PathVariable("workflowName") String workflowName, @RequestBody V1UpdateWorkflowRequest body);
@DeleteMapping(value = "/api/v1/applications/{appName}/workflows/{workflowName}")
ResponseEntity deleteWorkflow(@PathVariable("appName") String appName, @PathVariable("workflowName") String workflowName);
@GetMapping(value = "/api/v1/applications/{appName}/workflows/{workflowName}/records")
ResponseEntity listWorkflowRecords(@PathVariable("appName") String appName, @PathVariable("workflowName") String workflowName, @RequestParam(value = "page", required = false) Integer page, @RequestParam(value = "pageSize", required = false) Integer pageSize);
@GetMapping(value = "/api/v1/applications/{appName}/envs/{envName}/records")
ResponseEntity listWorkflowRecordsFromEnv(@PathVariable("appName") String appName, @PathVariable("envName") String envName, @RequestParam(value = "page", required = false) Integer page, @RequestParam(value = "pageSize", required = false) Integer pageSize);
@GetMapping(value = "/api/v1/applications/{appName}/workflows/{workflowName}/records/{record}")
ResponseEntity detailWorkflowRecord(@PathVariable("appName") String appName, @PathVariable("workflowName") String workflowName, @PathVariable("record") String record);
@GetMapping(value = "/api/v1/applications/{appName}/workflows/{workflowName}/records/{record}/inputs")
ResponseEntity getWorkflowRecordInputs(@PathVariable("appName") String appName, @PathVariable("workflowName") String workflowName, @PathVariable("record") String record, @RequestParam(value = "step", required = true) String step);
@GetMapping(value = "/api/v1/applications/{appName}/workflows/{workflowName}/records/{record}/outputs")
ResponseEntity getWorkflowRecordOutputs(@PathVariable("appName") String appName, @PathVariable("workflowName") String workflowName, @PathVariable("record") String record, @RequestParam(value = "step", required = true) String step);
@GetMapping(value = "/api/v1/applications/{appName}/workflows/{workflowName}/records/{record}/logs")
ResponseEntity getWorkflowRecordLogs(@PathVariable("appName") String appName, @PathVariable("workflowName") String workflowName, @PathVariable("record") String record, @RequestParam(value = "step", required = true) String step);
@GetMapping(value = "/api/v1/applications/{appName}/workflows/{workflowName}/records/{record}/resume")
ResponseEntity resumeWorkflowRecord(@PathVariable("appName") String appName, @PathVariable("workflowName") String workflowName, @PathVariable("record") String record, @RequestParam(value = "step", required = false) String step);
@GetMapping(value = "/api/v1/applications/{appName}/workflows/{workflowName}/records/{record}/rollback")
ResponseEntity rollbackWorkflowRecord(@PathVariable("appName") String appName, @PathVariable("workflowName") String workflowName, @PathVariable("record") String record, @RequestParam(value = "rollbackVersion", required = false) String rollbackVersion);
@GetMapping(value = "/api/v1/applications/{appName}/workflows/{workflowName}/records/{record}/terminate")
ResponseEntity terminateWorkflowRecord(@PathVariable("appName") String appName, @PathVariable("workflowName") String workflowName, @PathVariable("record") String record);
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy